### 打印Windows窗体知识点解析 #### 一、概述 在Windows应用程序开发过程中,有时候我们需要将当前窗体的内容打印出来。本知识点基于一个简单的Windows Forms应用实例,讲解如何实现打印功能。通过分析代码,我们可以了解到如何利用.NET Framework提供的类库来完成这一任务。 #### 二、关键概念与技术 ##### 1. Windows Forms (WinForms) Windows Forms是.NET Framework的一部分,它为创建桌面应用程序提供了一种简单直观的方式。WinForms提供了丰富的控件和事件处理机制,使得开发者能够快速构建用户界面。 ##### 2. PrintDocument 类 `PrintDocument` 类位于 `System.Drawing.Printing` 命名空间中,用于表示要打印的文档。它是所有打印文档的基础类,可以用于创建打印文档并处理打印操作中的各种事件。 ##### 3. PrintPageEvent 类 `PrintPageEvent` 类同样位于 `System.Drawing.Printing` 命名空间中,用于处理 `PrintDocument` 的 `PrintPage` 事件。当打印页面时,该事件会被触发,允许开发者在页面上绘制内容。 ##### 4. Bitmap 和 Graphics 类 - **Bitmap**:位于 `System.Drawing` 命名空间中,用于表示位图图像。 - **Graphics**:也位于 `System.Drawing` 命名空间中,提供了一系列用于绘制图形的方法和属性。 这两个类在本例中被用来捕捉屏幕上的内容,并将其转换为可以在打印页面上使用的图像。 #### 三、代码解析 ##### 1. 类定义和初始化 ```csharp public partial class Form1 : Form { private Button printButton = new Button(); private PrintDocument printDocument = new PrintDocument(); public Form1() { // 初始化按钮和事件绑定 printButton.Text = "PrintForm"; printButton.Click += new EventHandler(printButton_Click); printDocument.PrintPage += new PrintPageEventHandler(printDocument1_PrintPage); this.Controls.Add(printButton); } ``` 这里定义了一个名为 `Form1` 的窗体类,其中包含一个按钮 `printButton` 和一个 `PrintDocument` 对象 `printDocument`。按钮点击事件和 `PrintDocument` 的 `PrintPage` 事件分别绑定了对应的事件处理方法。 ##### 2. 按钮点击事件处理 ```csharp private void printButton_Click(object sender, EventArgs e) { Bitmap bmp = new Bitmap(this.Width, this.Height); Graphics newGraphics = Graphics.FromImage(bmp); newGraphics.CopyFromScreen(this.Location, Point.Empty, this.Size); Clipboard.SetImage(bmp); // SendKeys.Send("%{PRTSC}"); // (Alt+PrtSc) printDocument.Print(); } ``` 当点击按钮时,这段代码首先创建一个与当前窗体大小相同的 `Bitmap` 对象,然后通过 `Graphics.FromImage` 方法获取一个 `Graphics` 对象。接着调用 `CopyFromScreen` 方法将当前窗体的内容复制到 `Bitmap` 中,并将其设置为剪贴板内容。最后调用 `PrintDocument` 的 `Print` 方法进行打印。 ##### 3. 打印页面事件处理 ```csharp private void printDocument1_PrintPage(object sender, PrintPageEventArgs e) { e.Graphics.DrawImage(Clipboard.GetImage(), 0, 0); } ``` 这个方法会在每次打印页面时被调用。通过 `e.Graphics.DrawImage` 方法将剪贴板中的图像绘制到打印页面上。 #### 四、总结 通过以上代码示例,我们学习了如何在Windows Forms应用程序中实现打印功能。这涉及到使用 `PrintDocument` 类及其事件来控制打印过程,以及利用 `Bitmap` 和 `Graphics` 类来捕捉和处理图像数据。这些技术对于需要在Windows应用程序中添加打印功能的开发者来说是非常有用的。
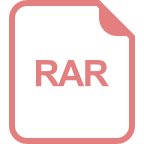
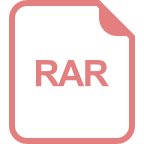
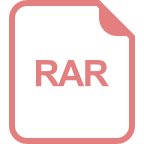
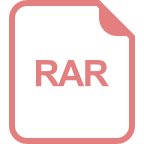
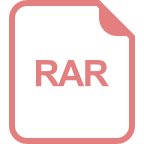
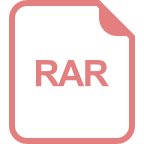
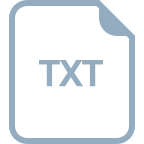
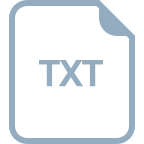
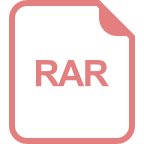
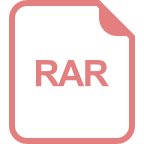
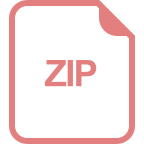
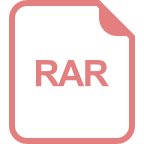
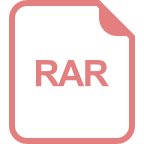
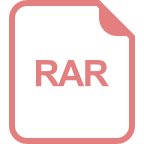
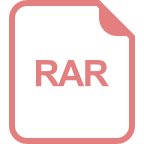
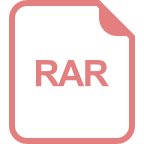
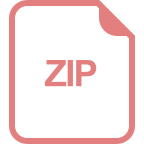
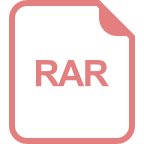
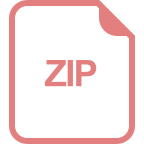
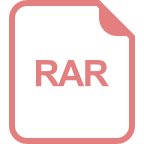
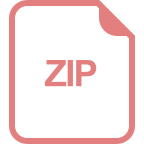
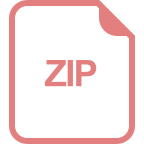
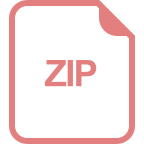
using System.Drawing;
using System.Drawing.Printing;
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
private Button printButton = new Button();
private PrintDocument printDocument = new PrintDocument();
public Form1()
{
printButton.Text = "Print Form";
printButton.Click += new EventHandler(printButton_Click);
printDocument.PrintPage += new PrintPageEventHandler(printDocument1_PrintPage);
this.Controls.Add(printButton);
}
private void printButton_Click(object sender, EventArgs e)
{
Bitmap bmp = new Bitmap(this.Width, this.Height);
Graphics newGraphics = Graphics.FromImage(bmp);
newGraphics.CopyFromScreen(this.Location, Point.Empty, this.Size);
Clipboard.SetImage(bmp);
//SendKeys.Send("%{PRTSC}"); // (Alt + PrtSc)
printDocument.Print();
}
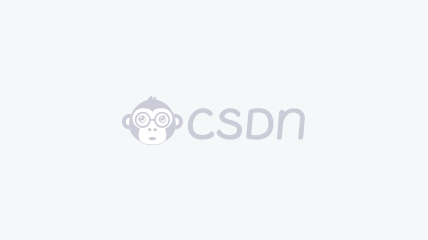

- 粉丝: 21
- 资源: 295
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

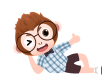
最新资源
- 20190313-100538-非对称电容在变压器油中10kv高压电作用下产生力的现象
- GB材料数据库(!请注意鉴别其中的材料参数并不是完全正确!)
- JAVA商城,支持小程序商城、 供应链商城 小程序商城 H5商城 app商城超全商城模式官网 支持小程序商城 H5商城 APP商城 PC商城
- springboot的在线商城系统设计与开发源码
- springboot的飘香水果购物网站的设计与实现 源码
- NO.4学习样本,请参考第4章的内容配合学习使用
- 20190312-084407-旋转磁体产生的场对周围空间长度的影响-数值越大距离越短
- 嵌入式系统应用-LVGL的应用-智能时钟 part 2
- 国家安全教育课程结课论文要求.docx
- FIR数字滤波器设计与软件实现.pdf

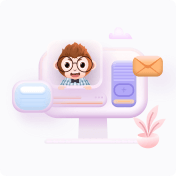
