/**
**********************************************************************************************************************
* @file stm32h5xx_hal_i3c.c
* @author MCD Application Team
* @brief I3C HAL module driver.
* This file provides firmware functions to manage the following
* functionalities of the Improvement Inter Integrated Circuit (I3C) peripheral:
* + Initialization and de-initialization functions
* + IO operation functions
* + Peripheral State and Errors functions
*
**********************************************************************************************************************
* @attention
*
* Copyright (c) 2023 STMicroelectronics.
* All rights reserved.
*
* This software is licensed under terms that can be found in the LICENSE file
* in the root directory of this software component.
* If no LICENSE file comes with this software, it is provided AS-IS.
*
**********************************************************************************************************************
@verbatim
======================================================================================================================
##### How to use this driver #####
======================================================================================================================
[..]
The I3C HAL driver can be used as follows:
(#) Declare a I3C_HandleTypeDef handle structure, for example:
I3C_HandleTypeDef hi3c;
(#) Declare a I3C_XferTypeDef transfer descriptor structure, for example:
I3C_XferTypeDef ContextBuffers;
(#)Initialize the I3C low level resources by implementing the HAL_I3C_MspInit() API:
(##) Enable the I3Cx interface clock
(##) I3C pins configuration
(+++) Enable the clock for the I3C GPIOs
(+++) Configure I3C pins as alternate function push-pull with no-pull
(##) NVIC configuration if you need to use interrupt process
(+++) Configure the I3Cx interrupt priority
(+++) Enable the NVIC I3C IRQ Channel
(##) DMA Configuration if you need to use DMA process
(+++) Declare a DMA_HandleTypeDef handle structure for
the Command Common Code (CCC) management channel
(+++) Declare a DMA_HandleTypeDef handle structure for
the transmit channel
(+++) Declare a DMA_HandleTypeDef handle structure for
the receive channel
(+++) Declare a DMA_HandleTypeDef handle structure for
the status channel
(+++) Enable the DMAx interface clock
(+++) Configure the DMA handle parameters
(+++) Configure the DMA Command Common Code (CCC) channel
(+++) Configure the DMA Tx channel
(+++) Configure the DMA Rx channel
(+++) Configure the DMA Status channel
(+++) Associate the initialized DMA handle to the hi3c DMA CCC, Tx, Rx or Status handle as necessary
(+++) Configure the priority and enable the NVIC for the transfer complete interrupt on
the DMA CCC, Tx, Rx or Status instance
(#) Configure the HAL I3C Communication Mode as Controller or Target in the hi3c Init structure.
(#) Configure the Controller Communication Bus characterics for Controller mode.
This mean, configure the parameters SDAHoldTime, WaitTime, SCLPPLowDuration,
SCLI3CHighDuration, SCLODLowDuration, SCLI2CHighDuration, BusFreeDuration,
BusIdleDuration in the LL_I3C_CtrlBusConfTypeDef structure through h3c Init structure.
(#) Configure the Target Communication Bus characterics for Target mode.
This mean, configure the parameter BusAvailableDuration in the LL_I3C_TgtBusConfTypeDef structure
through h3c Init structure.
All these parameters for Controller or Target can be configured directly in user code or
by using CubeMx generation.
To help the computation of the different parameters, the recommendation is to use CubeMx.
Those parameters can be modified after the hi3c initialization by using
HAL_I3C_Ctrl_BusCharacteristicConfig() for controller and
HAL_I3C_Tgt_BusCharacteristicConfig() for target.
(#) Initialize the I3C registers by calling the HAL_I3C_Init(), configures also the low level Hardware
(GPIO, CLOCK, NVIC...etc) by calling the customized HAL_I3C_MspInit(&hi3c) API.
(#) Configure the different FIFO parameters in I3C_FifoConfTypeDef structure as RxFifoThreshold, TxFifoThreshold
for Controller or Target mode.
And enable/disable the Control or Status FIFO only for Controller Mode.
Use HAL_I3C_SetConfigFifo() function to finalize the configuration, and HAL_I3C_GetConfigFifo() to retrieve
FIFO configuration.
Possibility to clear the FIFO configuration by using HAL_I3C_ClearConfigFifo() which reset the configuration
FIFO to their default hardware value
(#) Configure the different additional Controller configuration in I3C_CtrlConfTypeDef structure as DynamicAddr,
StallTime, HotJoinAllowed, ACKStallState, CCCStallState, TxStallState, RxStallState, HighKeeperSDA.
Use HAL_I3C_Ctrl_Config() function to finalize the Controller configuration.
(#) Configure the different additional Target configuration in I3C_TgtConfTypeDef structure as Identifier,
MIPIIdentifier, CtrlRoleRequest, HotJoinRequest, IBIRequest, IBIPayload, IBIPayloadSize, MaxReadDataSize,
MaxWriteDataSize, CtrlCapability, GroupAddrCapability, DataTurnAroundDuration, MaxReadTurnAround,
MaxDataSpeed, MaxSpeedLimitation, HandOffActivityState, HandOffDelay, PendingReadMDB.
Use HAL_I3C_Tgt_Config() function to finalize the Target configuration.
(#) Before initiate any IO operation, the application must launch an assignment of the different
Target dynamic address by using HAL_I3C_Ctrl_DynAddrAssign() in polling mode or
HAL_I3C_Ctrl_DynAddrAssign_IT() in interrupt mode.
This procedure is named Enter Dynamic Address Assignment (ENTDAA CCC command).
For the initiation of ENTDAA procedure from the controller, each target connected and powered on the I3C bus
must repond to this particular Command Common Code by sending its proper Payload (a amount of 48bits which
contain the target characteristics)
Each time a target responds to ENTDAA sequence, the application is informed through
HAL_I3C_TgtReqDynamicAddrCallback() of the reception of the target paylaod.
And then application must send a associated dynamic address through HAL_I3C_Ctrl_SetDynAddr().
This procedure in loop automatically in hardware side until a target respond to repeated ENTDAA sequence.
The application is informed of the end of the procedure at reception of HAL_I3C_CtrlDAACpltCallback().
At the end of procedure, the function HAL_I3C_Ctrl_ConfigBusDevices() must be called to store in hardware
register part the target capabilities as Dynamic address, IBI support with or without additional data byte,
Controller role request support, Controller stop transfer after IBI through I3C_DeviceConfTypeDef structure.
(#) Other action to be done, before initiate any IO operation, the application must prepare the different frame
descriptor with its associated buffer allocation in their side.
Configure the different information related to CCC transfer through I3C_CCCTypeDef structure
Configure the different information related to Private or I2C transfer through I3C_PrivateTypeDef structure
Configure the different buffer pointers and associated size needed for the driver communication
through I3C_XferTypeDef structure
The I3C_XferTypeDef structur
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
STM32F4DISCOVERY 是 ST 推出的一款基于 ARM Cortex-M4 内核的开发板,最高主频为 168Mhz,该开发板具有丰富的板载资源,可以充分发挥 STM32F407 的芯片性能。MCU:STM32F407VGT6,主频 168MHz,1024KB FLASH ,192KB RAM,本章节是为需要在 RT-Thread 操作系统上使用更多开发板资源的开发者准备的。通过使用 ENV 工具对 BSP 进行配置,可以开启更多板载资源,实现更多高级功能。本 BSP 为开发者提供 MDK4、MDK5 和 IAR 工程,并且支持 GCC 开发环境。下面以 MDK5 开发环境为例,介绍如何将系统运行起来。
资源推荐
资源详情
资源评论
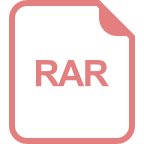
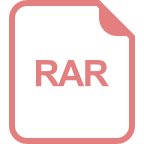
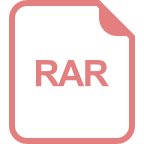
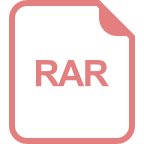
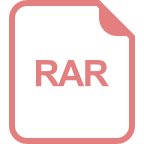
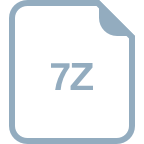
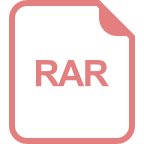
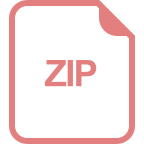
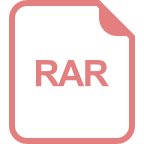
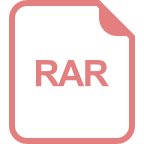
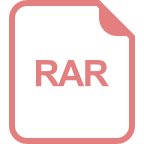
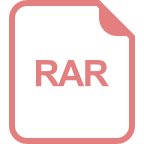
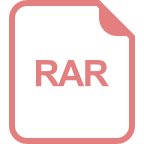
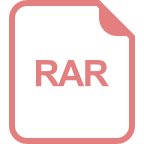
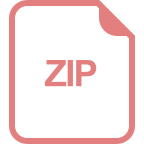
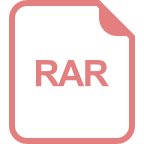
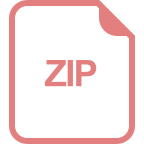
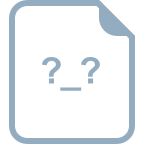
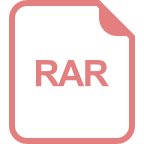
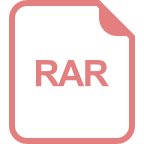
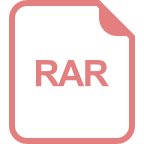
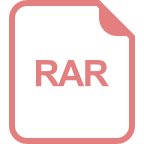
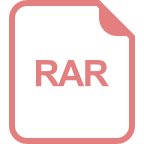
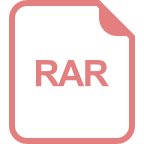
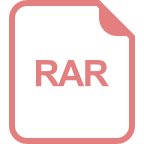
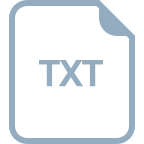
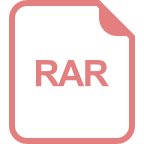
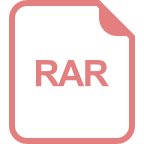
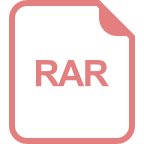
收起资源包目录

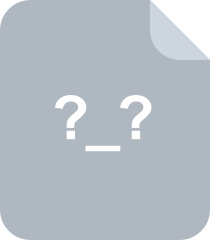
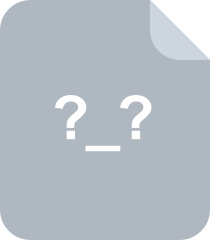
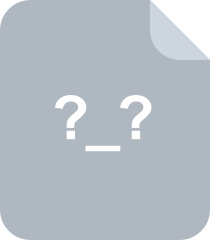
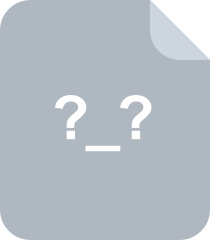
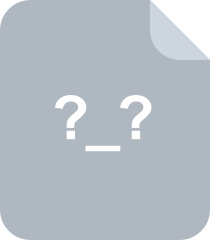
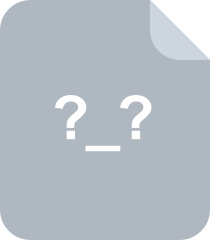
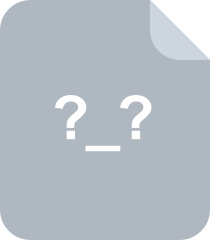
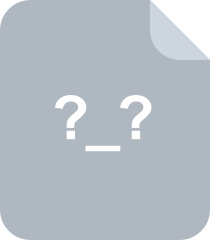
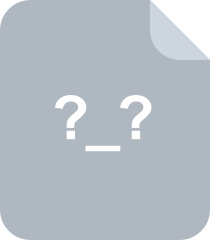
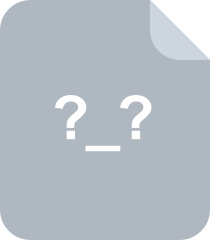
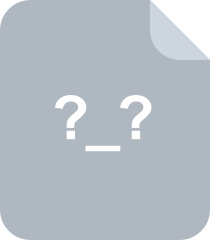
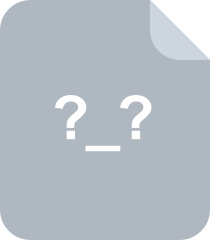
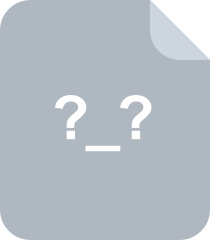
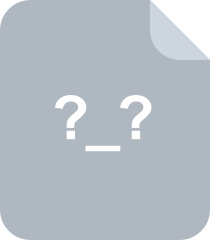
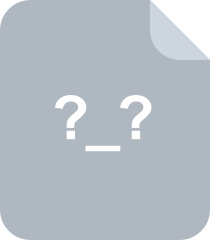
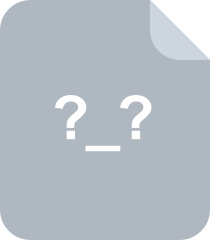
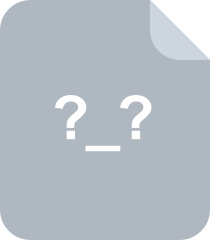
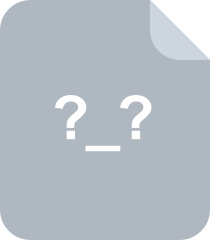
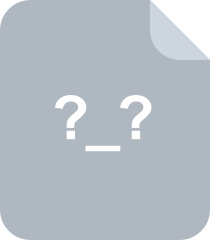
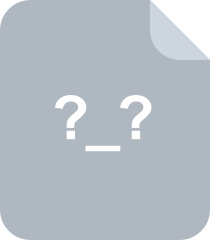
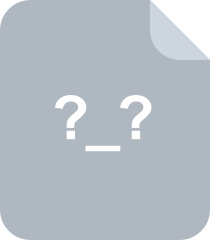
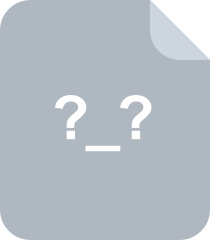
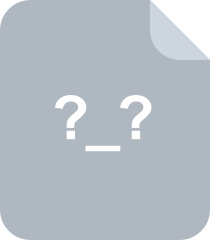
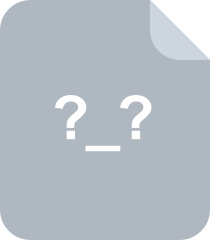
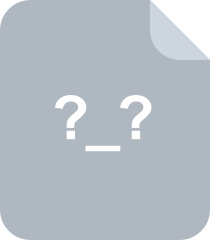
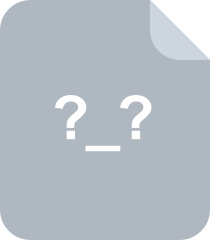
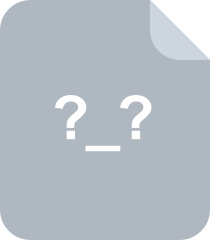
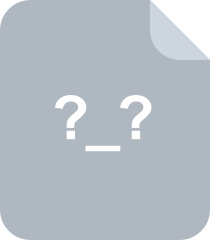
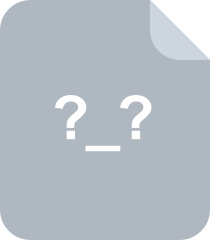
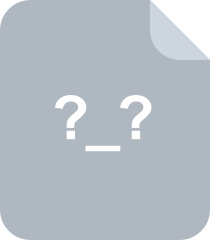
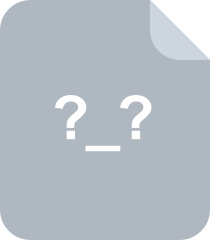
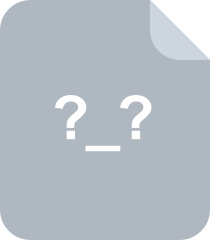
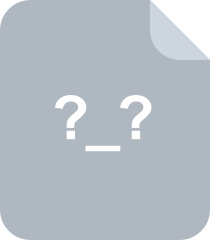
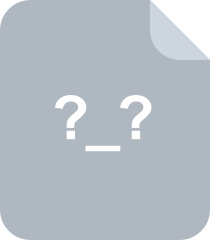
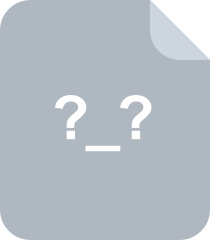
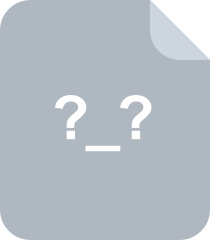
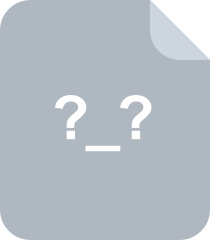
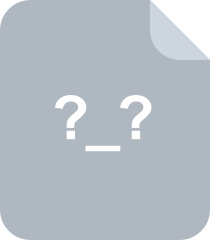
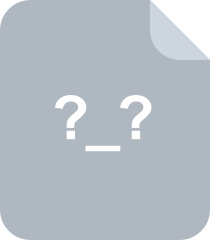
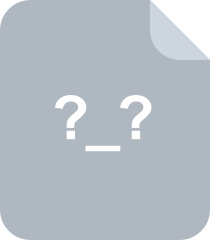
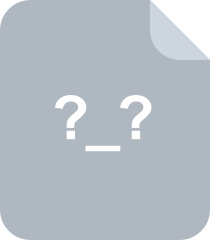
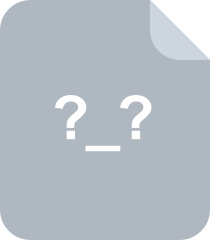
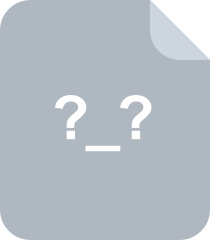
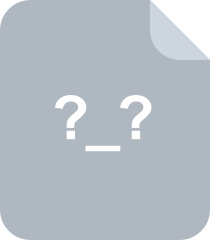
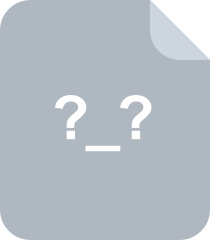
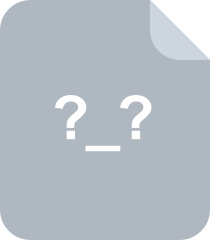
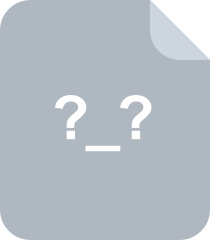
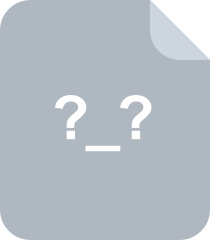
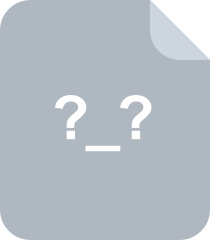
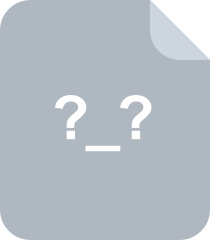
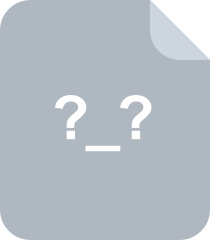
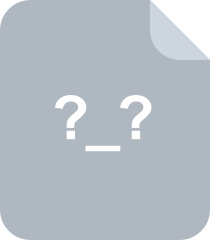
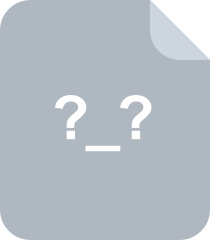
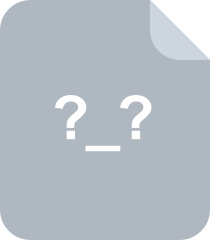
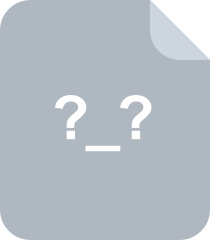
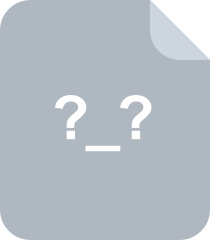
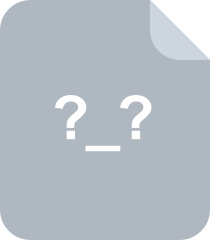
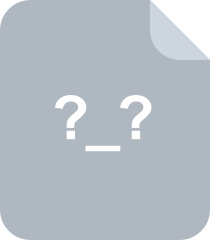
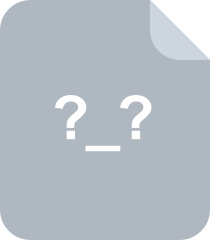
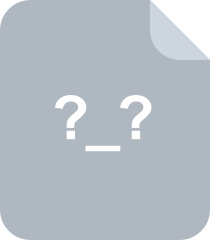
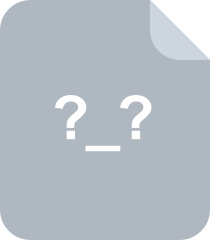
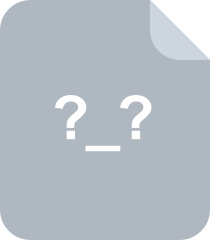
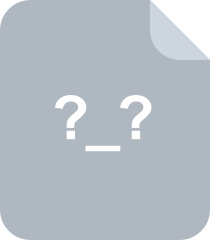
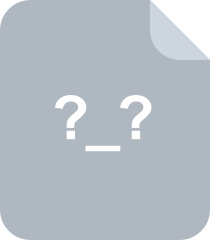
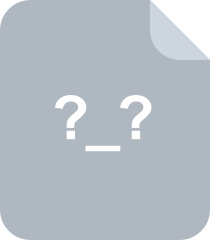
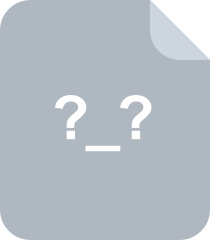
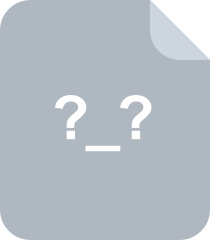
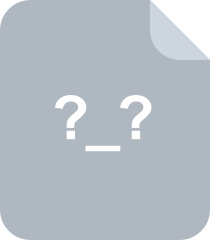
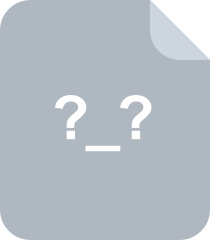
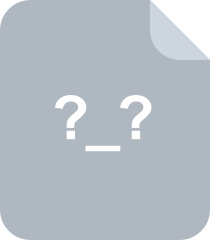
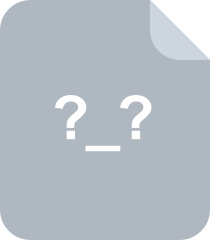
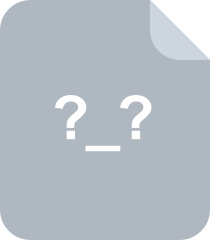
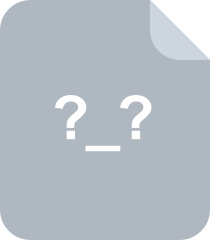
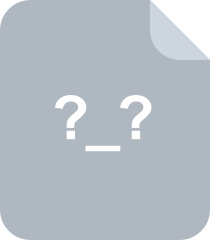
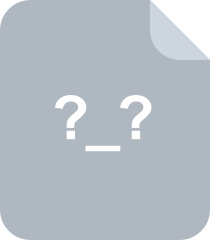
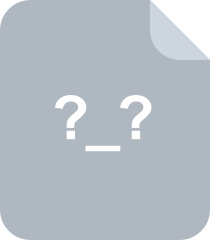
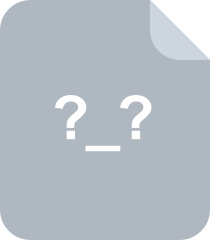
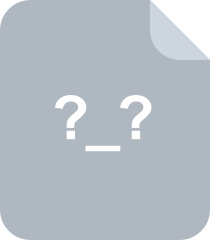
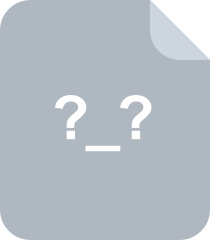
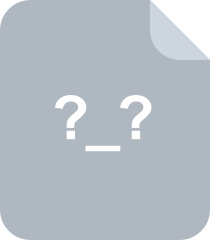
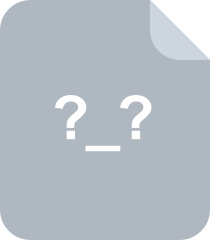
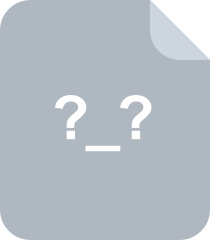
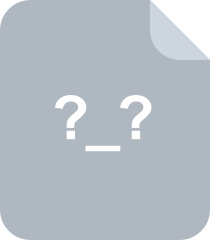
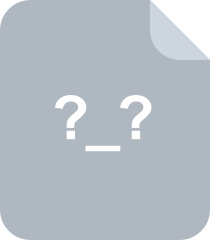
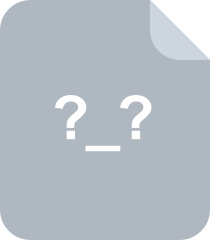
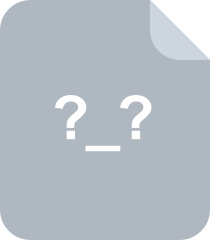
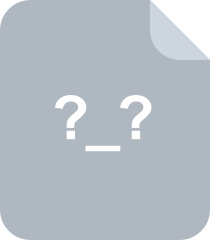
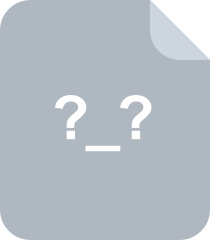
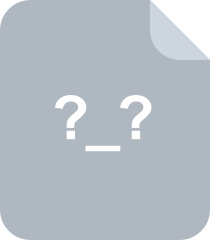
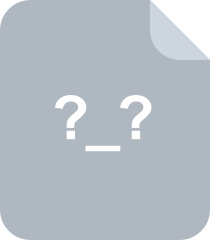
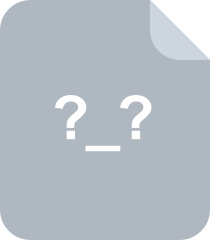
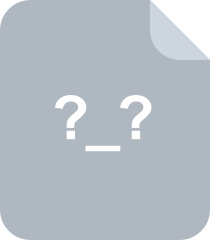
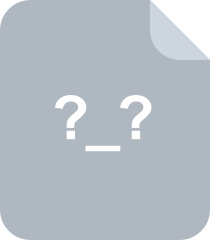
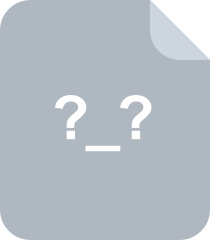
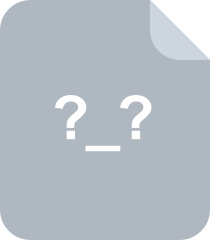
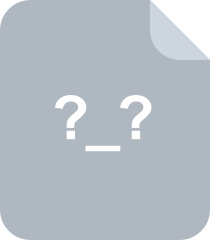
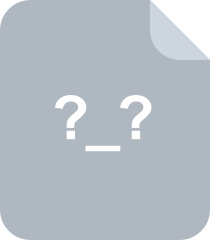
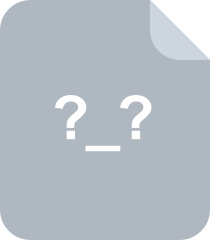
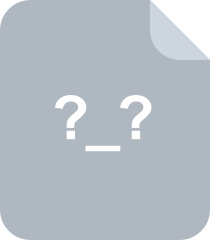
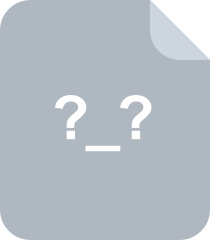
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
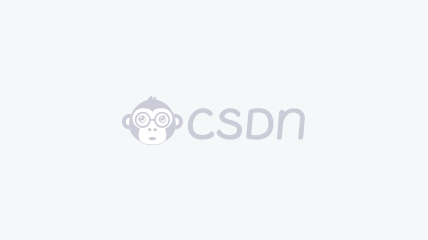


华为奋斗者精神
- 粉丝: 1w+
- 资源: 241
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

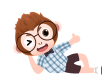
最新资源
- TOWER DEFENSE ZOMBIE WAR [1.01].zip
- GBT 27930 国标充电CAN报文解析 DBC文件
- 毕业设计基于C++和QT开发的智能售货系统(饮料售卖机)源码(高分毕设)
- TH2024005基于微信平台的文玩交易小程序ssm.zip
- java高校职工工资管理系统
- 零基础学AI-python语言:python基础语法(课件部分)
- IMT5G推进组发布5G无人机应用白皮书
- 基于Java SSM写的停车场管理系统,加入了车牌识别和数据分析
- 2025年P气瓶充装模拟考试卷
- 【java毕业设计】基于spring boot心理健康服务系统(springboot+vue+mysql+说明文档).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


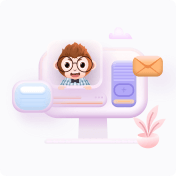
安全验证
文档复制为VIP权益,开通VIP直接复制
