/*
* Copyright (C) 2009 The Undried Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.android.superdeskclock;
import static android.os.BatteryManager.BATTERY_STATUS_CHARGING;
import static android.os.BatteryManager.BATTERY_STATUS_FULL;
import static android.os.BatteryManager.BATTERY_STATUS_UNKNOWN;
import java.util.Calendar;
import java.util.Date;
import java.util.Random;
import android.app.Activity;
import android.app.AlarmManager;
import android.app.PendingIntent;
import android.content.BroadcastReceiver;
import android.content.ComponentName;
import android.content.Context;
import android.content.Intent;
import android.content.IntentFilter;
import android.content.pm.PackageManager;
import android.content.res.Configuration;
import android.content.res.Resources;
import android.database.ContentObserver;
import android.database.Cursor;
import android.graphics.Rect;
import android.graphics.drawable.Drawable;
import android.net.Uri;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.provider.Settings;
import android.text.TextUtils;
import android.text.format.DateFormat;
import android.util.DisplayMetrics;
import android.util.Log;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.MenuItem;
import android.view.MotionEvent;
import android.view.View;
import android.view.ViewGroup;
import android.view.ViewTreeObserver;
import android.view.Window;
import android.view.WindowManager;
import android.view.animation.AnimationUtils;
import android.widget.AbsoluteLayout;
import android.widget.ImageButton;
import android.widget.ImageView;
import android.widget.TextView;
/**
* DeskClock clock view for desk docks.
*/
@SuppressWarnings("deprecation")
public class DeskClock extends Activity {
private static final boolean DEBUG = false;
private static final String LOG_TAG = "DeskClock";
// Alarm action for midnight (so we can update the date display).
private static final String ACTION_MIDNIGHT = "com.android.superdeskclock.MIDNIGHT";
// Interval between forced polls of the weather widget.
private final long QUERY_WEATHER_DELAY = 60 * 60 * 1000; // 1 hr
// Intent to broadcast for dock settings.
private static final String DOCK_SETTINGS_ACTION = "com.android.settings.DOCK_SETTINGS";
// Delay before engaging the burn-in protection mode (green-on-black).
private final long SCREEN_SAVER_TIMEOUT = 5 * 60 * 1000; // 5 min
// Repositioning delay in screen saver.
private final long SCREEN_SAVER_MOVE_DELAY = 60 * 1000; // 1 min
// Color to use for text & graphics in screen saver mode.
private final int SCREEN_SAVER_COLOR = 0xFF308030;
private final int SCREEN_SAVER_COLOR_DIM = 0xFF183018;
// Opacity of black layer between clock display and wallpaper.
private final float DIM_BEHIND_AMOUNT_NORMAL = 0.4f;
private final float DIM_BEHIND_AMOUNT_DIMMED = 0.8f; // higher contrast when display dimmed
// Internal message IDs.
private final int QUERY_WEATHER_DATA_MSG = 0x1000;
private final int UPDATE_WEATHER_DISPLAY_MSG = 0x1001;
private final int SCREEN_SAVER_TIMEOUT_MSG = 0x2000;
private final int SCREEN_SAVER_MOVE_MSG = 0x2001;
// Weather widget query information.
private static final String GENIE_PACKAGE_ID = "com.google.android.apps.genie.geniewidget";
private static final String WEATHER_CONTENT_AUTHORITY = GENIE_PACKAGE_ID + ".weather";
private static final String WEATHER_CONTENT_PATH = "/weather/current";
private static final String[] WEATHER_CONTENT_COLUMNS = new String[] {
"location",
"timestamp",
"temperature",
"highTemperature",
"lowTemperature",
"iconUrl",
"iconResId",
"description",
};
private static final String ACTION_GENIE_REFRESH = "com.google.android.apps.genie.REFRESH";
// State variables follow.
private DigitalClock mTime;
private TextView mDate;
private TextView mNextAlarm = null;
private TextView mBatteryDisplay;
private TextView mWeatherCurrentTemperature;
private TextView mWeatherHighTemperature;
private TextView mWeatherLowTemperature;
private TextView mWeatherLocation;
private ImageView mWeatherIcon;
private String mWeatherCurrentTemperatureString;
private String mWeatherHighTemperatureString;
private String mWeatherLowTemperatureString;
private String mWeatherLocationString;
private Drawable mWeatherIconDrawable;
private Resources mGenieResources = null;
private boolean mDimmed = false;
private boolean mScreenSaverMode = false;
private String mDateFormat;
private int mBatteryLevel = -1;
private boolean mPluggedIn = false;
private boolean mLaunchedFromDock = false;
private Random mRNG;
private PendingIntent mMidnightIntent;
private final BroadcastReceiver mIntentReceiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
final String action = intent.getAction();
if (DEBUG) Log.d(LOG_TAG, "mIntentReceiver.onReceive: action=" + action + ", intent=" + intent);
if (Intent.ACTION_DATE_CHANGED.equals(action) || ACTION_MIDNIGHT.equals(action)) {
refreshDate();
} else if (Intent.ACTION_BATTERY_CHANGED.equals(action)) {
handleBatteryUpdate(
intent.getIntExtra("status", BATTERY_STATUS_UNKNOWN),
intent.getIntExtra("level", 0));
}
// else if (UiModeManager.ACTION_EXIT_DESK_MODE.equals(action)) {
// if (mLaunchedFromDock) {
// // moveTaskToBack(false);
// finish();
// }
// mLaunchedFromDock = false;
// }
}
};
private final Handler mHandy = new Handler() {
@Override
public void handleMessage(Message m) {
if (m.what == QUERY_WEATHER_DATA_MSG) {
new Thread() { public void run() { queryWeatherData(); } }.start();
scheduleWeatherQueryDelayed(QUERY_WEATHER_DELAY);
} else if (m.what == UPDATE_WEATHER_DISPLAY_MSG) {
updateWeatherDisplay();
} else if (m.what == SCREEN_SAVER_TIMEOUT_MSG) {
saveScreen();
} else if (m.what == SCREEN_SAVER_MOVE_MSG) {
moveScreenSaver();
}
}
};
private final ContentObserver mContentObserver = new ContentObserver(mHandy) {
@Override
public void onChange(boolean selfChange) {
if (DEBUG) Log.d(LOG_TAG, "content observer notified that weather changed");
refreshWeather();
}
};
private void moveScreenSaver() {
moveScreenSaverTo(-1,-1);
}
private void moveScreenSaverTo(int x, int y) {
if (!mScreenSaverMode) return;
final View saver_view = findViewById(R.id.saver_view);
DisplayMetrics metrics = new DisplayMetrics();
getWindowManager().getDefaultDisplay().getMetrics(metrics);
if (x < 0 ||

kiduo08
- 粉丝: 76
- 资源: 12
最新资源
- 2024金融数据安全治理白皮书.pdf
- 2024基于风险驱动的交付模式转型探索与实践.pdf
- Java源码springboot+vue线上教育系统(vue)-毕业设计论文-大作业.zip
- Java源码springboot+vue响应式企业员工绩效考评系统-毕业设计论文-大作业.zip
- Java源码springboot+vue线上阅读系统-毕业设计论文-大作业.zip
- Java源码springboot+vue校企合作项目管理系统-毕业设计论文-大作业.zip
- Java源码springboot+vue校园爱心捐赠互助管理系统-毕业设计论文-大作业.zip
- Java源码springboot+vue小区物业管理系统-毕业设计论文-大作业.zip
- 2024面向AIGC的数智广电新质生产力构建白皮书.pdf
- Java源码springboot+vue学生心理咨询评估系统-毕业设计论文-大作业.zip
- Java源码springboot+vue鞋类商品购物商城系统-毕业设计论文-大作业.zip
- Java源码springboot+vue学校田径运动会管理系统(vue)-毕业设计论文-大作业.zip
- Java源码springboot+vue药品信息管理系统(vue)-毕业设计论文-大作业.zip
- Java源码springboot+vue养老服务管理系统(vue)-毕业设计论文-大作业.zip
- Java毕业设计-基于SpringBoot遗传算法的学校排课系统源码+数据库
- 2024年GPT与通信白皮书.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


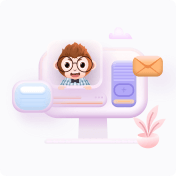
- 1
- 2
前往页