// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: market.proto
package com.gc.android.market.api.model;
public final class Market {
private Market() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public enum AppType
implements com.google.protobuf.ProtocolMessageEnum {
NONE(0, 0),
APPLICATION(1, 1),
RINGTONE(2, 2),
WALLPAPER(3, 3),
GAME(4, 4),
;
public static final int NONE_VALUE = 0;
public static final int APPLICATION_VALUE = 1;
public static final int RINGTONE_VALUE = 2;
public static final int WALLPAPER_VALUE = 3;
public static final int GAME_VALUE = 4;
public final int getNumber() { return value; }
public static AppType valueOf(int value) {
switch (value) {
case 0: return NONE;
case 1: return APPLICATION;
case 2: return RINGTONE;
case 3: return WALLPAPER;
case 4: return GAME;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap<AppType>
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap<AppType>
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap<AppType>() {
public AppType findValueByNumber(int number) {
return AppType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.gc.android.market.api.model.Market.getDescriptor().getEnumTypes().get(0);
}
private static final AppType[] VALUES = {
NONE, APPLICATION, RINGTONE, WALLPAPER, GAME,
};
public static AppType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private AppType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:AppType)
}
public interface AppsRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional .AppType appType = 1;
boolean hasAppType();
com.gc.android.market.api.model.Market.AppType getAppType();
// optional string query = 2;
boolean hasQuery();
String getQuery();
// optional string categoryId = 3;
boolean hasCategoryId();
String getCategoryId();
// optional string appId = 4;
boolean hasAppId();
String getAppId();
// optional bool withExtendedInfo = 6;
boolean hasWithExtendedInfo();
boolean getWithExtendedInfo();
// optional .AppsRequest.OrderType orderType = 7 [default = NONE];
boolean hasOrderType();
com.gc.android.market.api.model.Market.AppsRequest.OrderType getOrderType();
// optional uint64 startIndex = 8;
boolean hasStartIndex();
long getStartIndex();
// optional int32 entriesCount = 9;
boolean hasEntriesCount();
int getEntriesCount();
// optional .AppsRequest.ViewType viewType = 10 [default = ALL];
boolean hasViewType();
com.gc.android.market.api.model.Market.AppsRequest.ViewType getViewType();
}
public static final class AppsRequest extends
com.google.protobuf.GeneratedMessage
implements AppsRequestOrBuilder {
// Use AppsRequest.newBuilder() to construct.
private AppsRequest(Builder builder) {
super(builder);
}
private AppsRequest(boolean noInit) {}
private static final AppsRequest defaultInstance;
public static AppsRequest getDefaultInstance() {
return defaultInstance;
}
public AppsRequest getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.gc.android.market.api.model.Market.internal_static_AppsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.gc.android.market.api.model.Market.internal_static_AppsRequest_fieldAccessorTable;
}
public enum OrderType
implements com.google.protobuf.ProtocolMessageEnum {
NONE(0, 0),
POPULAR(1, 1),
NEWEST(2, 2),
FEATURED(3, 3),
;
public static final int NONE_VALUE = 0;
public static final int POPULAR_VALUE = 1;
public static final int NEWEST_VALUE = 2;
public static final int FEATURED_VALUE = 3;
public final int getNumber() { return value; }
public static OrderType valueOf(int value) {
switch (value) {
case 0: return NONE;
case 1: return POPULAR;
case 2: return NEWEST;
case 3: return FEATURED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap<OrderType>
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap<OrderType>
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap<OrderType>() {
public OrderType findValueByNumber(int number) {
return OrderType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.gc.android.market.api.model.Market.AppsRequest.getDescriptor().getEnumTypes().get(0);
}
private static final OrderType[] VALUES = {
NONE, POPULAR, NEWEST, FEATURED,
};
public static OrderType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private OrderType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:AppsRequest.OrderType)
}
public enum ViewType
implements com.google.protobuf.ProtocolMessageEnum {
ALL(0, 0),
FREE(1, 1),
PAID(2, 2),
;
public static final int ALL_VALUE = 0;
public static final int FREE_VALUE = 1;
public static final int PAID_VALUE = 2;
public final int getNumber() { return value; }
public static ViewType valueOf(int value) {
switch (value) {
case 0: return ALL;
case 1: return FREE;
case 2: return PAID;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap<ViewType>
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.
没有合适的资源?快使用搜索试试~ 我知道了~
google play获取app的详细信息 源码
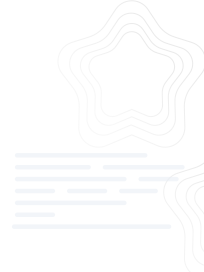
共241个文件
svn-base:136个
class:53个
entries:18个


温馨提示
程序中取得一些Android应用的信息,Google的Android Market公布了api,可以取得Android Market上的Android应用的包括名字、作者、应用的描述、评论、图标与软件截图等信息。 基于Google Protocol Buffers 议实现
资源推荐
资源详情
资源评论
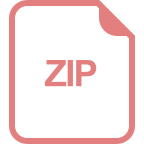
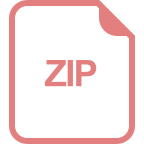
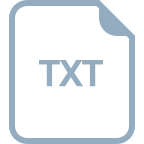
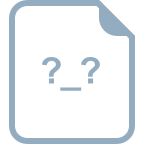
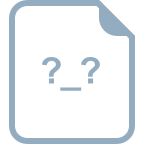
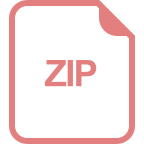
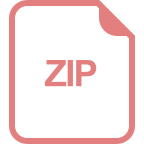
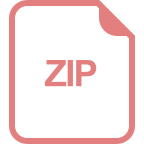
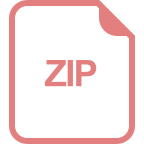
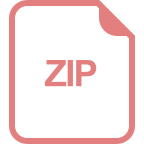
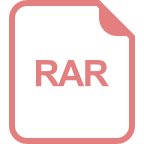
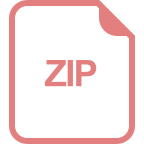
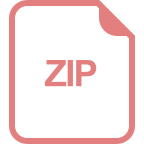
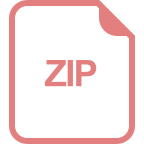
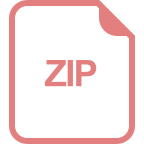
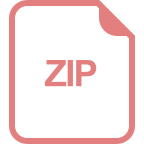
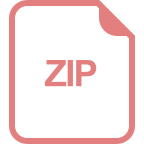
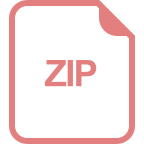
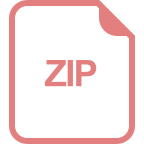
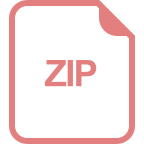
收起资源包目录

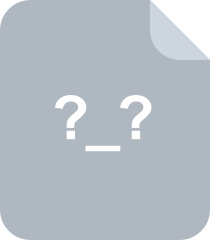
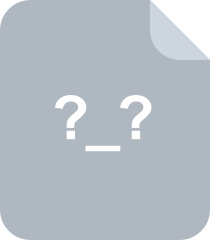
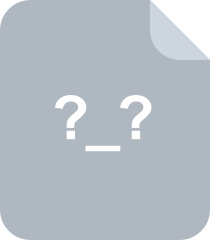
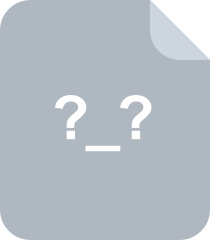
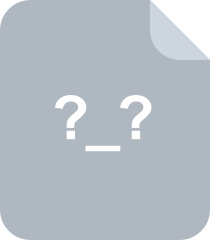
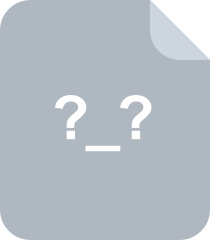
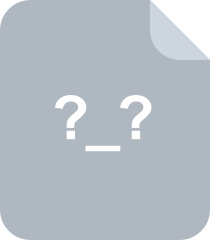
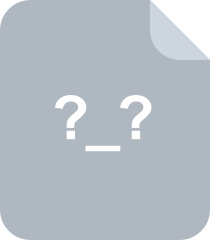
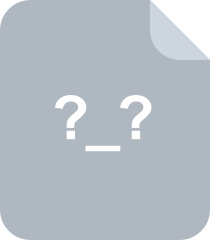
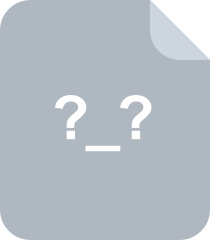
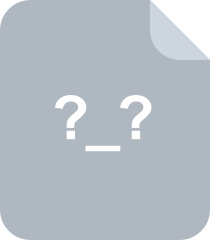
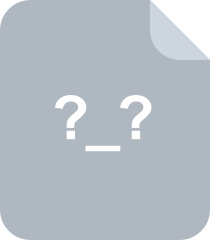
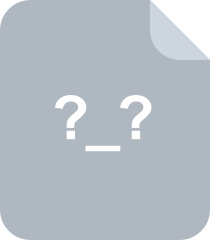
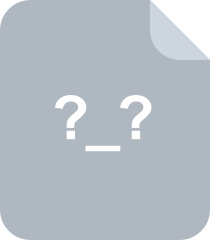
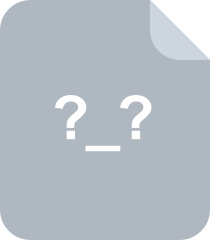
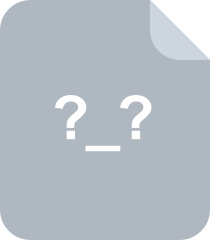
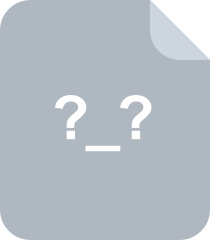
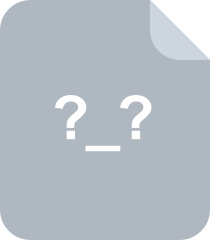
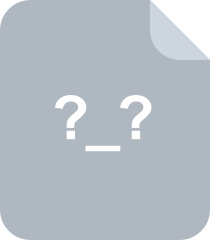
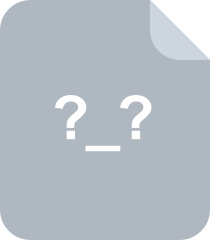
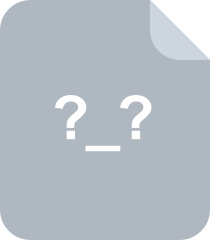
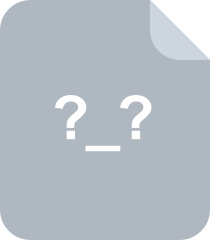
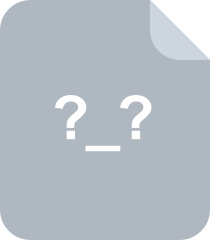
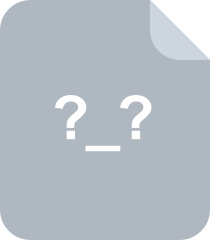
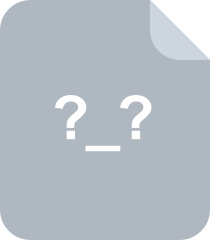
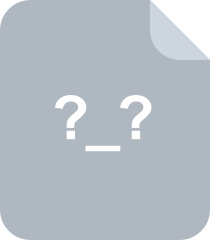
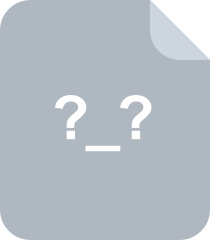
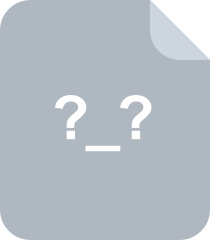
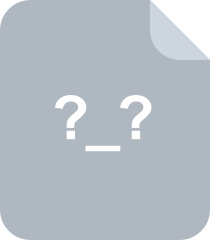
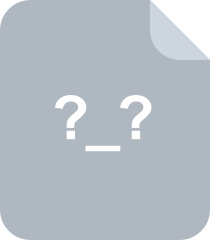
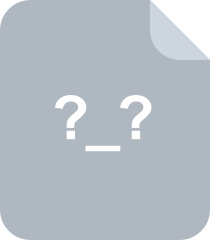
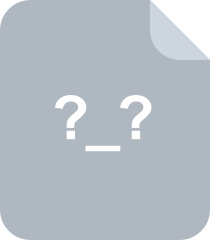
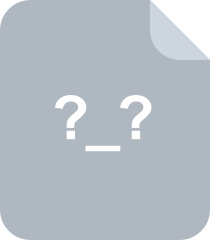
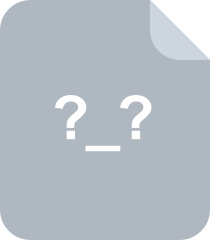
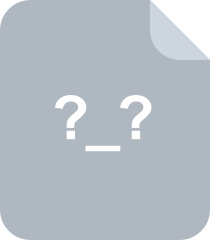
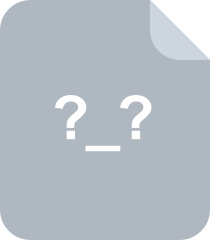
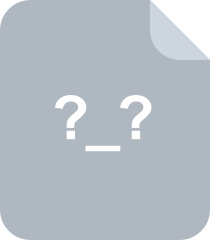
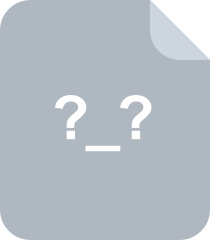
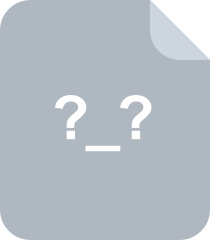
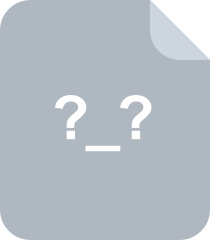
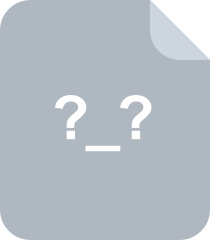
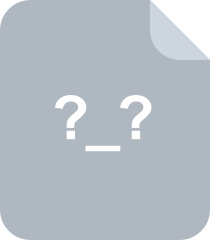
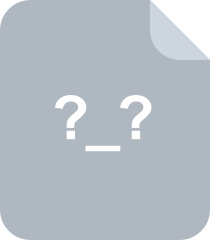
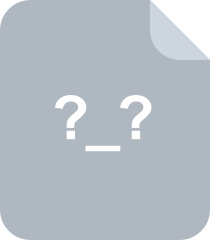
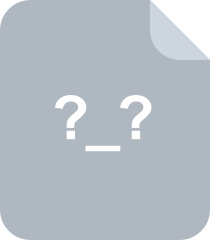
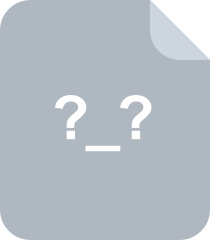
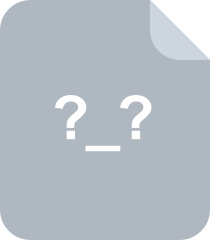
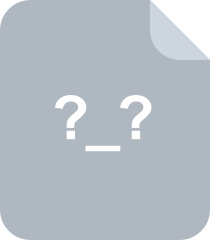
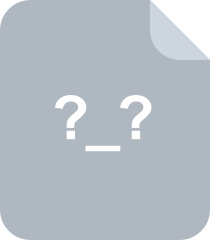
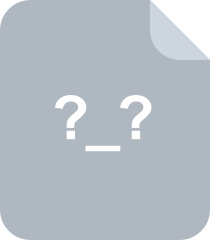
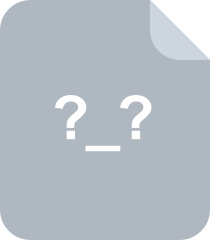
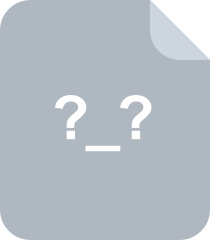
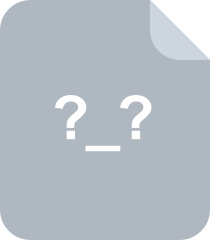
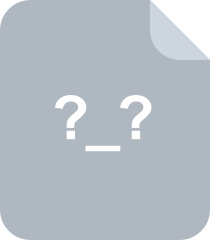
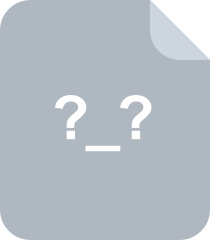
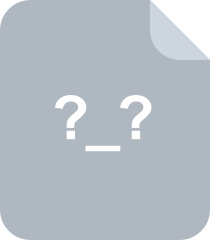
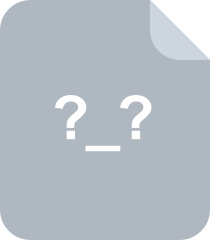
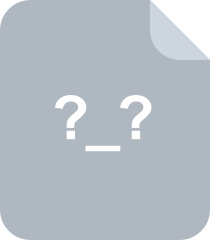
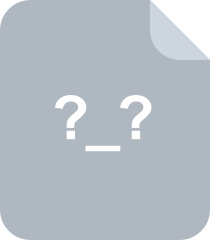
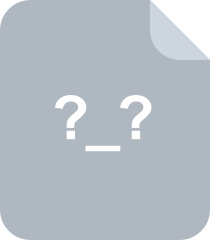
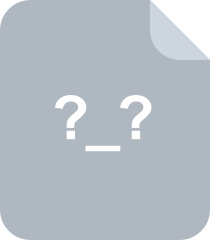
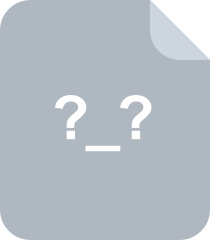
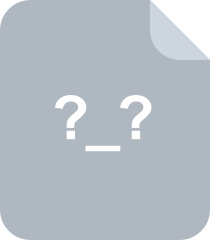
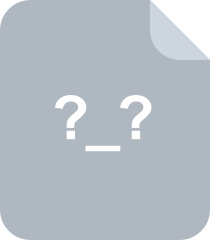
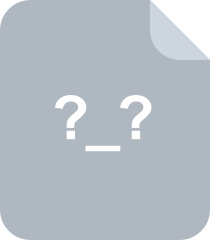
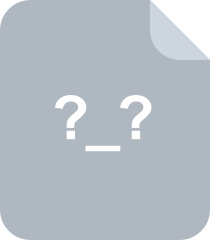
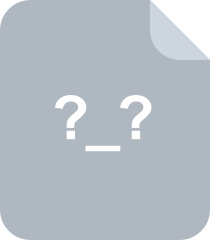
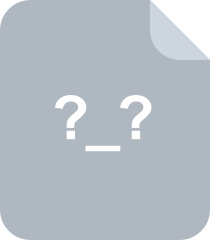
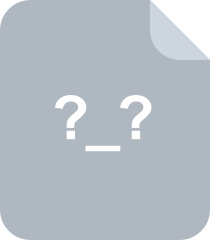
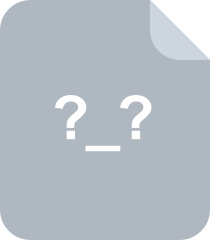
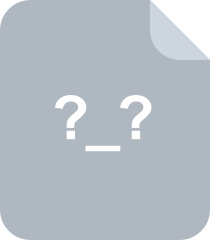
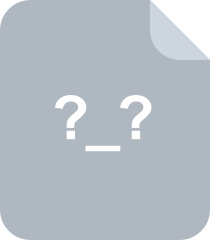
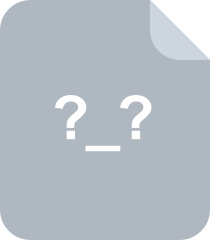
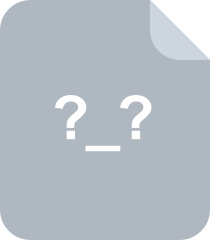
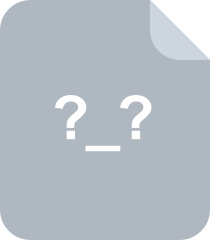
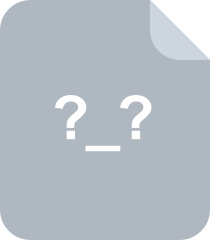
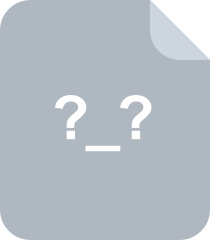
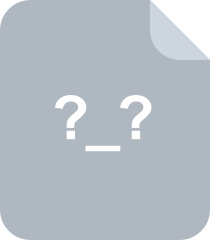
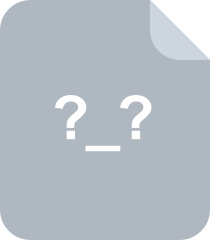
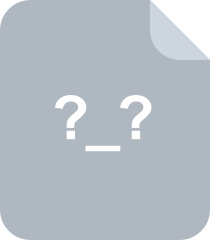
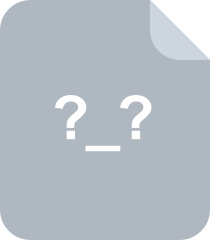
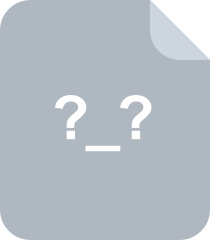
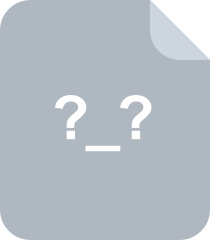
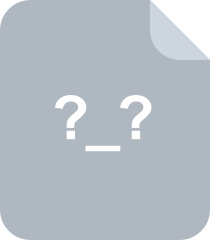
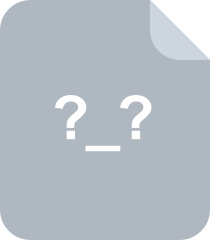
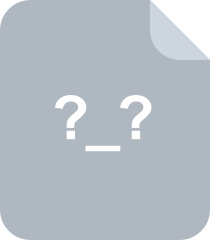
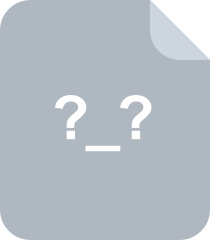
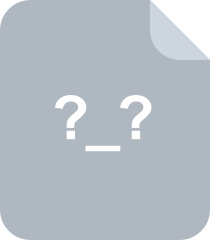
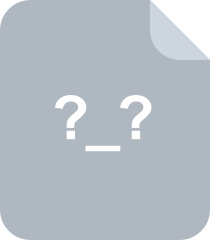
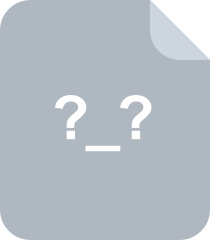
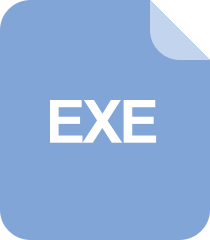
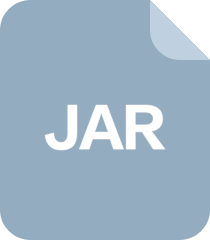
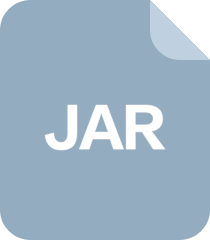
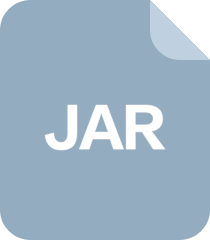
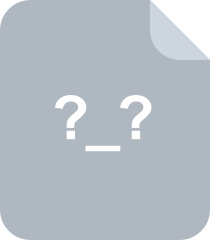
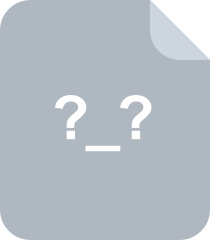
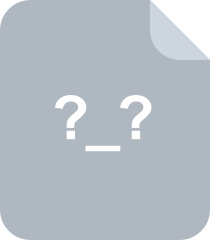
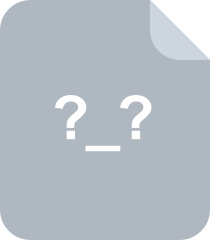
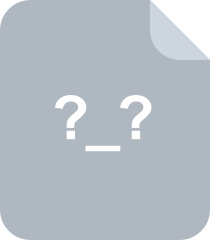
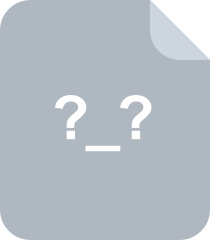
共 241 条
- 1
- 2
- 3

kf42470
- 粉丝: 2
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

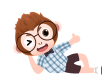
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


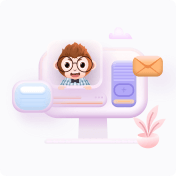
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
前往页