# POSIX Interface
## Introduction to Pthreads
POSIX Threads is abbreviated as Pthreads. POSIX is the abbreviation of "Portable Operating System Interface". POSIX is a set of standards established by IEEE Computer Society to improve the compatibility of different operating systems and the portability of applications. Pthreads is a threaded POSIX standard defined in the POSIX.1c, Threads extensions (IEEE Std1003.1c-1995) standard, which defines a set of C programming language types, functions, and constants. Defined in the `pthread.h` header file and a thread library, there are about 100 APIs, all of which have a "`pthread_`" prefix and can be divided into 4 categories:
- **Thread management**: Thread creating, detaching, joining, and setting and querying thread attributes.
- **Mutex**: Abbreviation for "mutual exclusion", which restricts thread access to shared data and protects the integrity of shared data. This includes creating, destroying, locking, and unlocking mutex and some functions for setting or modifying mutex properties.
- **Condition variable**: Communication between threads used to share a mutex. It includes functions such as creation, destruction, waiting condition variables, and sending signal .
- **Read/write locks and barriers**: including the creation, destruction, wait, and related property settings of read-write locks and barriers.
POSIX semaphores are used with Pthreads, but are not part of the Pthreads standard definition and are defined in POSIX.1b, Real-time extensions (IEEE Std1003.1b-1993). Therefore the prefix of the semaphore correlation function is "`sem_`" instead of "`pthread_`".
Message queues, like semaphores, are used with Pthreads and are not part of the Pthreads standard definition and are defined in the IEEE Std 1003.1-2001 standard. The prefix of the message queue related function is "`mq_`".
| Function Prefix | Function Group |
|----------------------|----------------------|
| `pthread_ ` | Thread itself and various related functions |
| `pthread_attr_` | Thread attribute object |
| `Pthread_mutex_` | Mutex |
| `pthread_mutexattr_` | Mutex attribute object |
| `pthread_cond_ ` | Conditional variable |
| `pthread_condattr_` | Condition variable attribute object |
| `pthread_rwlock_` | Read-write lock |
| `pthread_rwlockattr_` | Read-write lock attribute object |
| `pthread_spin_` | Spin lock |
| `pthread_barrier_ ` | Barrier |
| `pthread_barrierattr_` | Barrier attribute object |
| `sem_` | Semaphore |
| `mq_ ` | Message queue |
Most Pthreads functions return a value of 0 if they succeed, and an error code contained in the `errno.h` header file if unsuccessful. Many operating systems support Pthreads, such as Linux, MacOSX, Android, and Solaris, so applications written using Pthreads functions are very portable and can be compiled and run directly on many platforms that support Pthreads.
### Use POSIX in RT-Thread
Using the POSIX API interface in RT-Thread includes several parts: libc (for example, newlib), filesystem, pthread, and so on. Need to open the relevant options in rtconfig.h:
``` c
#define RT_USING_LIBC
#define RT_USING_DFS
#define RT_USING_DFS_DEVFS
#define RT_USING_PTHREADS
```
RT-Thread implements most of the functions and constants of Pthreads, defined in the pthread.h, mqueue.h, semaphore.h, and sched.h header files according to the POSIX standard. Pthreads is a sublibrary of libc, and Pthreads in RT-Thread are based on the encapsulation of RT-Thread kernel functions, making them POSIX compliant. The Pthreads functions and related functions implemented in RT-Thread are described in detail in the following sections.
## Thread
### Thread Handle
``` c
typedef rt_thread_t pthread_t;
```
`Pthread_t` is a redefinition of the `rt_thread_t` type, defined in the `pthread.h` header file. rt_thread_t is the thread handle (or thread identifier) of the RT-Thread and is a pointer to the thread control block. You need to define a variable of type pthread_t before creating a thread. Each thread corresponds to its own thread control block, which is a data structure used by the operating system to control threads. It stores some information about the thread, such as priority, thread name, and thread stack address. Thread control blocks and thread specific information are described in detail in the [Thread Management](../thread/thread.md) chapter.
### Create Thread
``` c
int pthread_create (pthread_t *tid,
const pthread_attr_t *attr,
void *(*start) (void *), void *arg);
```
| **Parameter** | **Description** |
|----------|------------------------------------------------------|
| tid | Pointer to thread handle (thread identifier), cannot be NULL |
| attr | Pointer to the thread property, if NULL is used, the default thread property is used |
| start | Thread entry function address |
| arg | The argument passed to the thread entry function |
|**return**| —— |
| 0 | succeeded |
| EINVAL | Invalid parameter |
| ENOMEM | Dynamic allocation of memory failed |
This function creates a pthread thread. This function dynamically allocates the POSIX thread data block and the RT-Thread thread control block, and saves the start address (thread ID) of the thread control block in the memory pointed to by the parameter tid, which can be used to operate in other threads. This thread; and the thread attribute pointed to by attr, the thread entry function pointed to by start, and the entry function parameter arg are stored in the thread data block and the thread control block. If the thread is created successfully, the thread immediately enters the ready state and participates in the scheduling of the system. If the thread creation fails, the resources occupied by the thread are released.
Thread properties and related functions are described in detail in the *Thread Advanced Programming* chapter. In general, the default properties can be used.
> After the pthread thread is created, if the thread needs to be created repeatedly, you need to set the pthread thread to detach mode, or use pthread_join to wait for the created pthread thread to finish.
#### Example Code for Creating Thread
The following program initializes two threads, which have a common entry function, but their entry parameters are not the same. Others, they have the same priority and are scheduled for rotation in time slices.
``` c
#include <pthread.h>
#include <unistd.h>
#include <stdio.h>
/* Thread control block */
static pthread_t tid1;
static pthread_t tid2;
/* Function return value check */
static void check_result(char* str,int result)
{
if (0 == result)
{
printf("%s successfully!\n",str);
}
else
{
printf("%s failed! error code is %d\n",str,result);
}
}
/* Thread entry function */
static void* thread_entry(void* parameter)
{
int count = 0;
int no = (int) parameter; /* Obtain the thread's entry parameters */
while (1)
{
/* Printout thread count value */
printf("thread%d count: %d\n", no, count ++);
sleep(2); /* Sleep for 2 seconds */
}
}
/* User application portal */
int rt_application_init()
{
int result;
/* Create thread 1, the property is the default value, the entry function is thread_entry, and the entry function parameter is 1 */
result = pthread_create(&tid1,NULL,thread_entry,(void*)1);
check_result("thread1 created", result);
/* Create thread 2, the property is the default value, the entry function
没有合适的资源?快使用搜索试试~ 我知道了~
移植rt-thread+freemodbus到gd32f10x工程
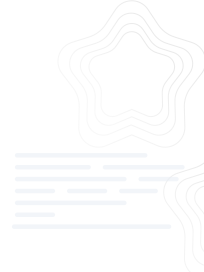
共2000个文件
h:838个
c:575个
md:477个

需积分: 0 6 下载量 5 浏览量
2023-09-14
10:15:04
上传
评论 1
收藏 676.65MB 7Z 举报
温馨提示
1.版本 RT-Thread v5.0.1,Freemodbus REL_1_6_0 2.使用了uart0,115200, 8N1串口配置,PA8作为收发控制引脚 3.已经调试好的工程目录rt-thread-master\bsp\gd32\arm\gd32103c-start,通过上位机发送01 06 31 00 00 0F C7 32后,gd32会0.5s发送一次该数据到上位机。 4.解决了gd32发送漏掉最后一个字节的bug。 5.支持env下使用menuconfig配置内核
资源推荐
资源详情
资源评论
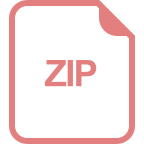
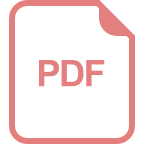
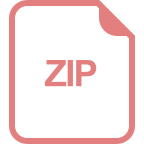
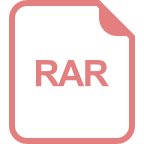
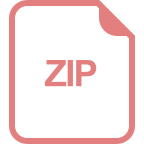
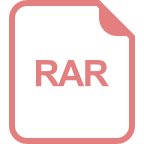
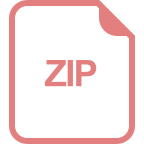
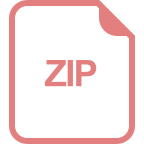
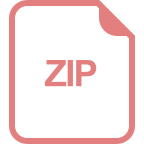
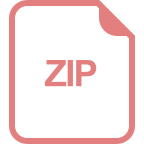
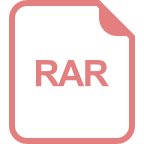
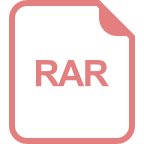
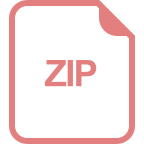
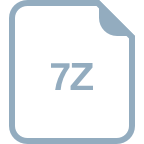
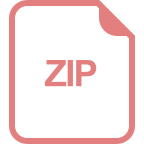
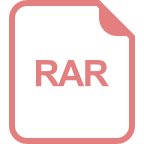
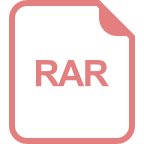
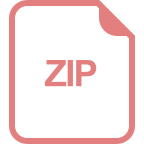
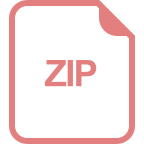
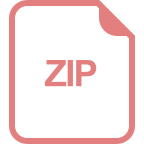
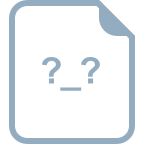
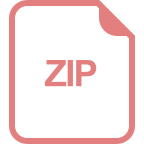
收起资源包目录

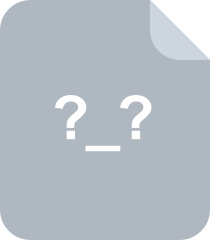
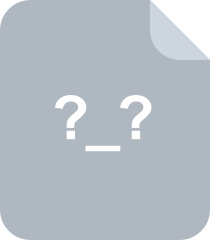
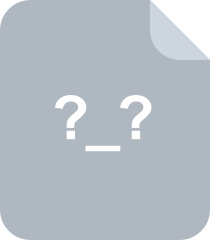
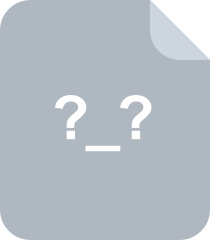
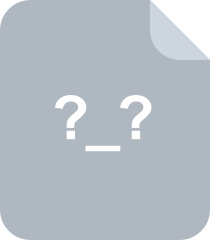
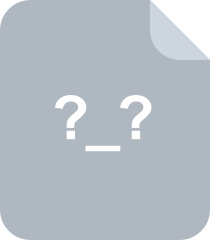
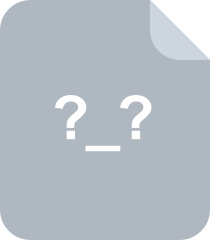
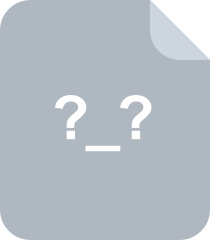
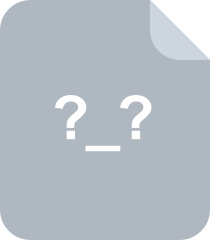
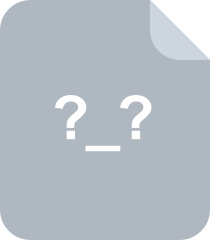
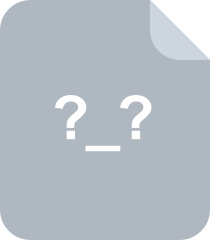
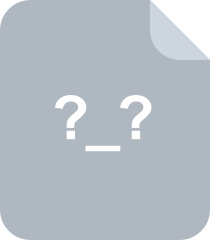
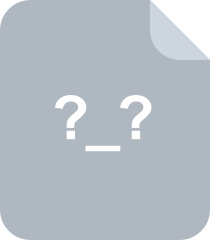
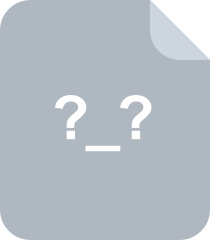
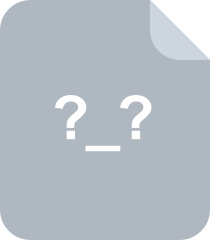
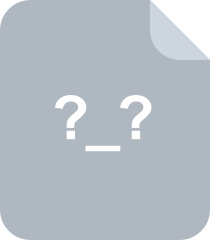
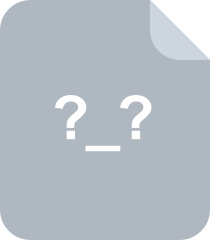
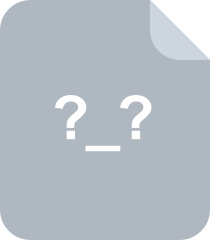
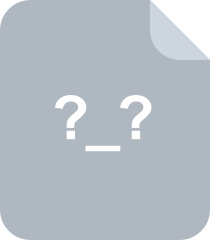
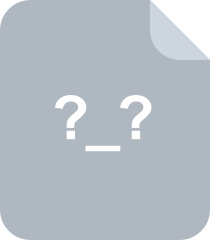
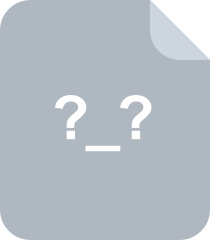
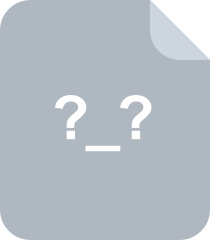
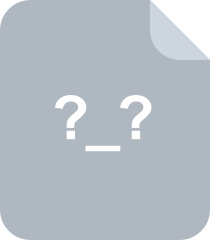
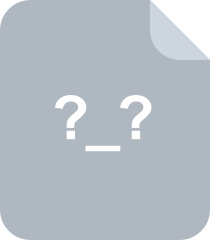
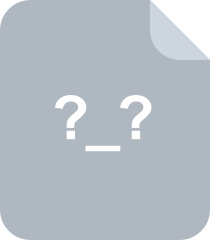
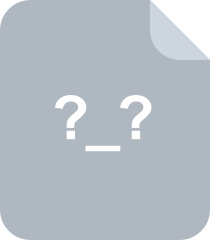
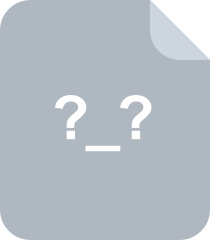
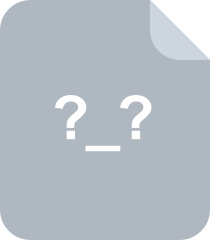
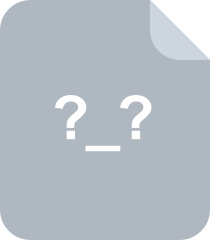
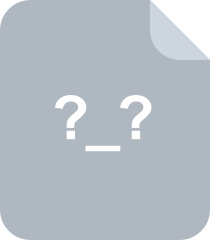
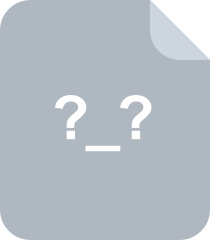
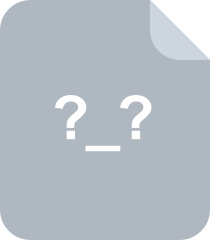
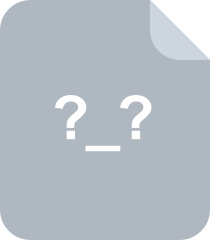
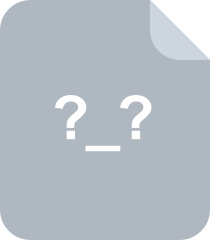
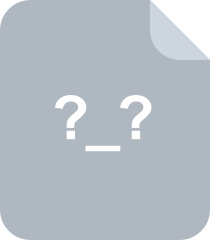
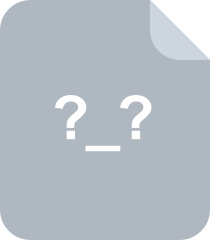
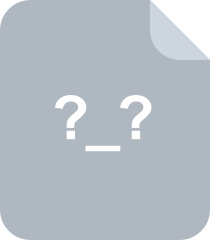
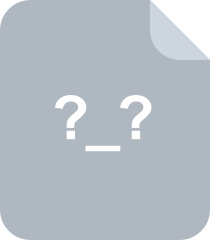
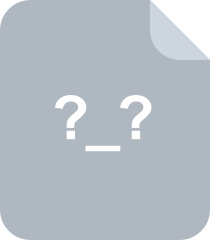
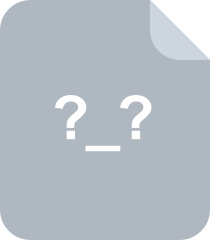
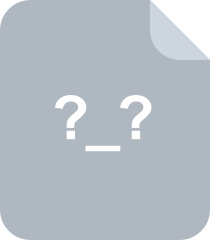
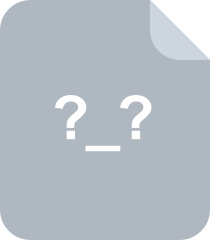
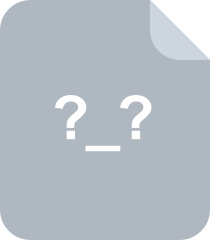
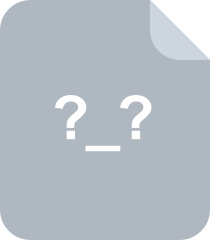
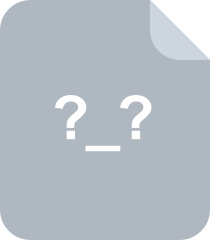
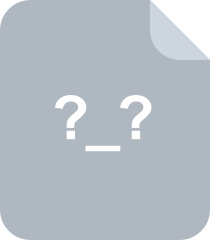
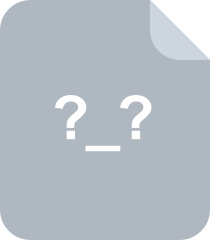
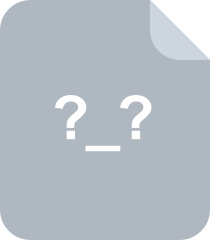
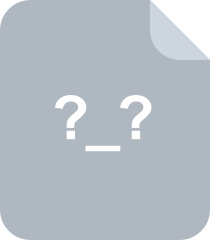
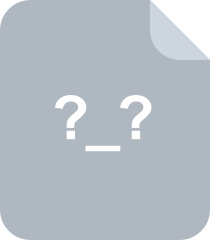
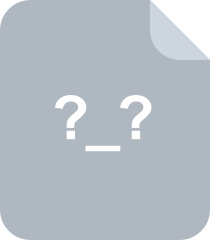
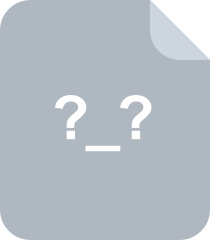
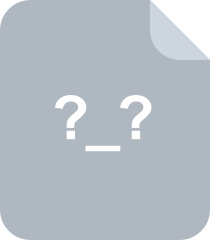
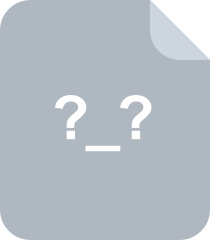
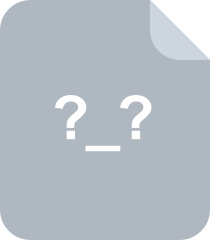
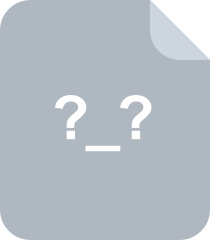
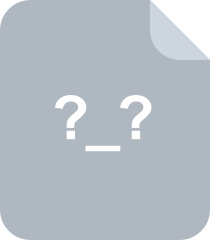
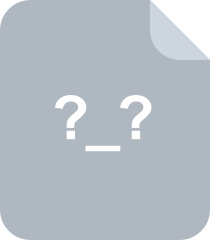
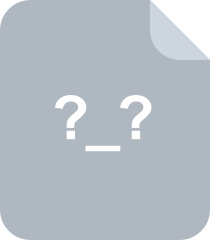
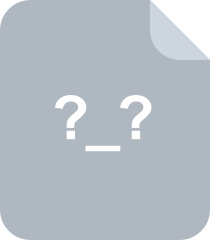
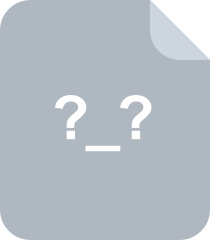
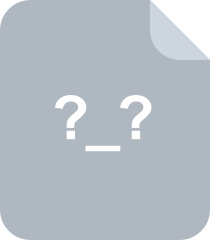
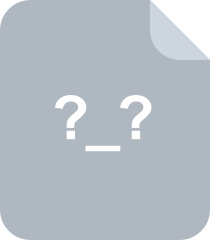
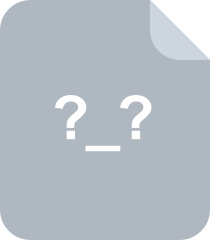
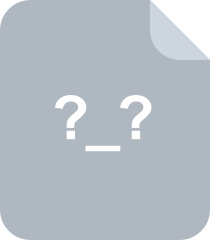
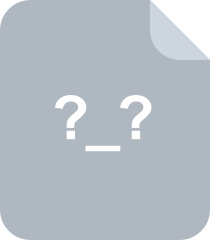
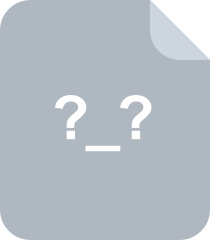
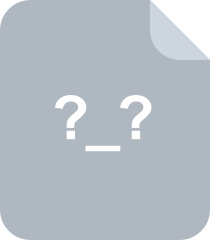
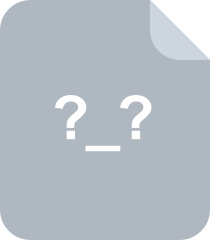
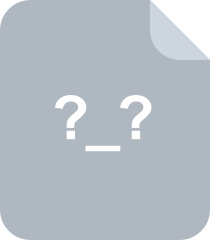
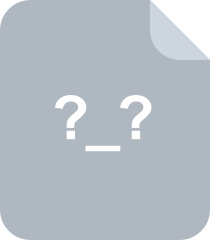
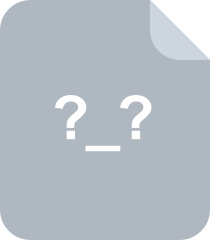
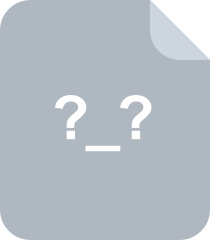
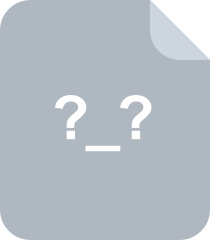
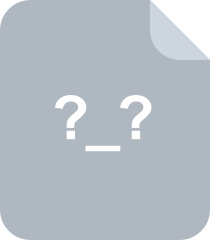
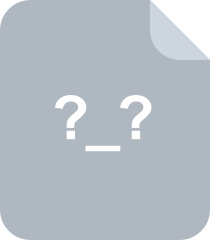
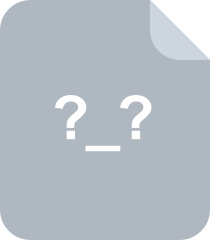
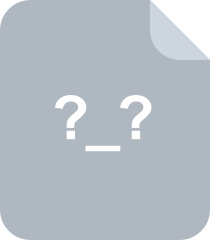
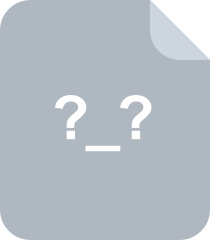
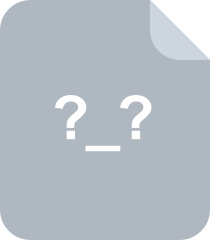
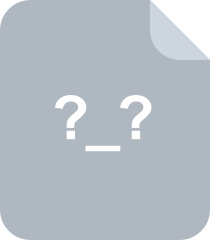
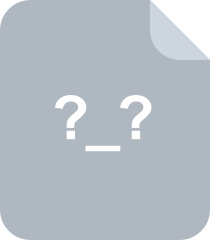
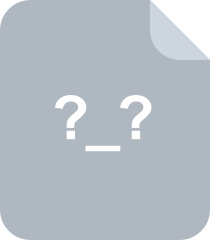
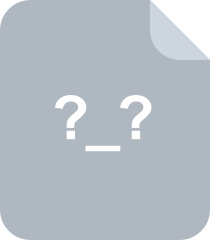
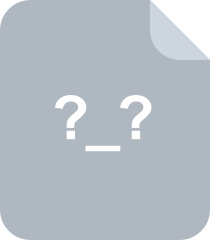
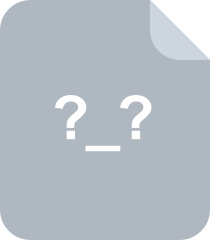
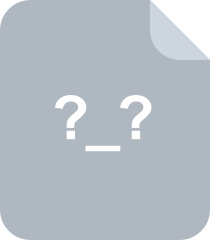
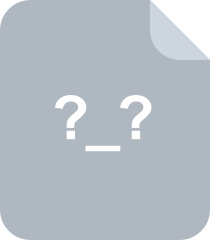
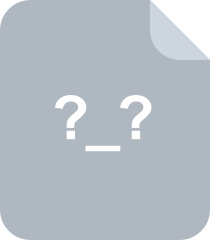
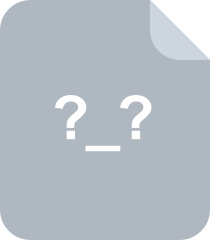
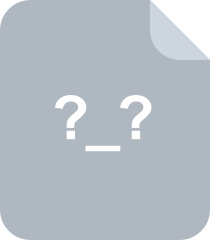
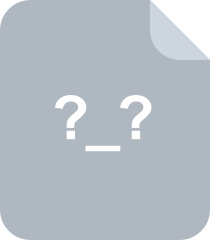
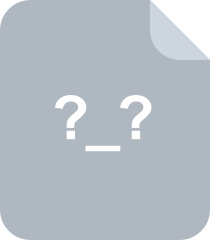
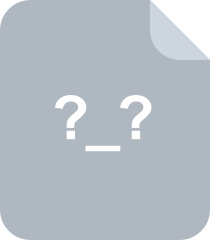
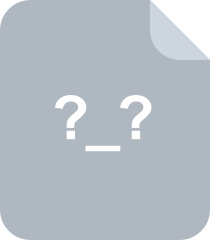
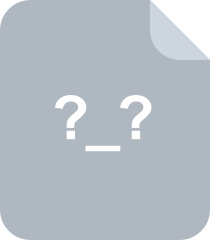
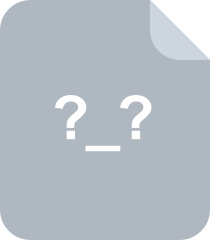
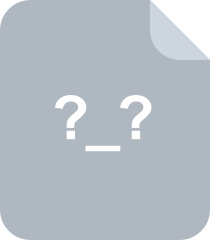
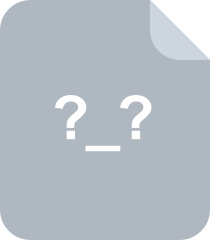
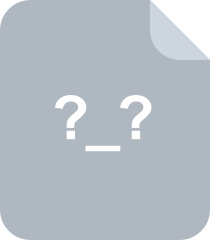
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
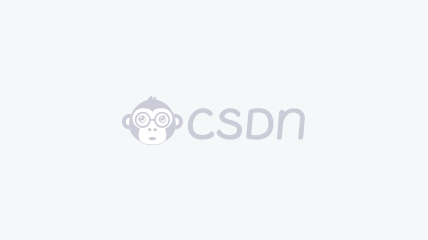

kevinlovejia
- 粉丝: 32
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

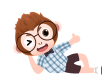
最新资源
- bdwptqmxgj11.zip
- onnxruntime-win-x86
- onnxruntime-win-x64-gpu-1.20.1.zip
- vs2019 c++20 语法规范 头文件 <ratio> 的源码阅读与注释,处理分数的存储,加减乘除,以及大小比较等运算
- 首次尝试使用 Win,DirectX C++ 中的形状渲染套件.zip
- 预乘混合模式是一种用途广泛的三合一混合模式 它已经存在很长时间了,但似乎每隔几年就会被重新发现 该项目包括使用预乘 alpha 的描述,示例和工具 .zip
- 项目描述 DirectX 引擎支持版本 9、10、11 库 Microsoft SDK 功能相机视图、照明、加载网格、动画、蒙皮、层次结构界面、动画控制器、网格容器、碰撞系统 .zip
- 项目 wiki 文档中使用的代码教程的源代码库.zip
- 面向对象的通用GUI框架.zip
- 基于Java语言的PlayerBase游戏角色设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


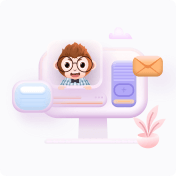
安全验证
文档复制为VIP权益,开通VIP直接复制
