没有合适的资源?快使用搜索试试~ 我知道了~
ASP-NET-Web-API-2-Building-a-REST-Service-from-Start-to-Finish

温馨提示
The ASP.NET MVC Framework has always been a good platform on which to implement REST-based services, but the introduction of the ASP.NET Web API Framework raised the bar to a whole new level. Now in release version 2.1, the Web API Framework has evolved into a powerful and refreshingly usable platform. This concise book provides technical background.
资源推荐
资源详情
资源评论
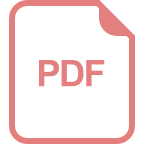
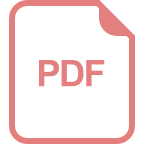
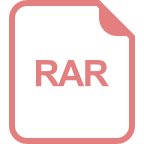
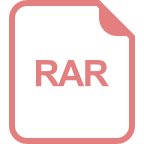
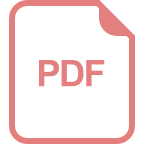
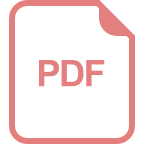
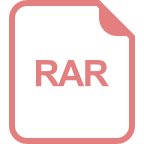
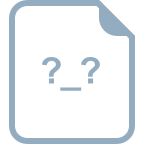
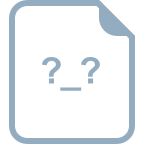
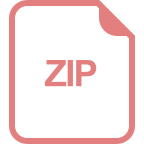
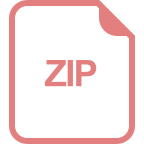
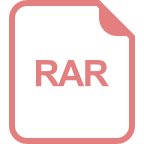
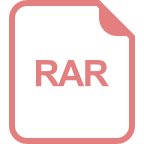
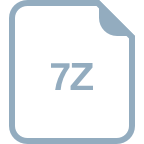
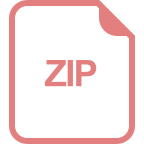
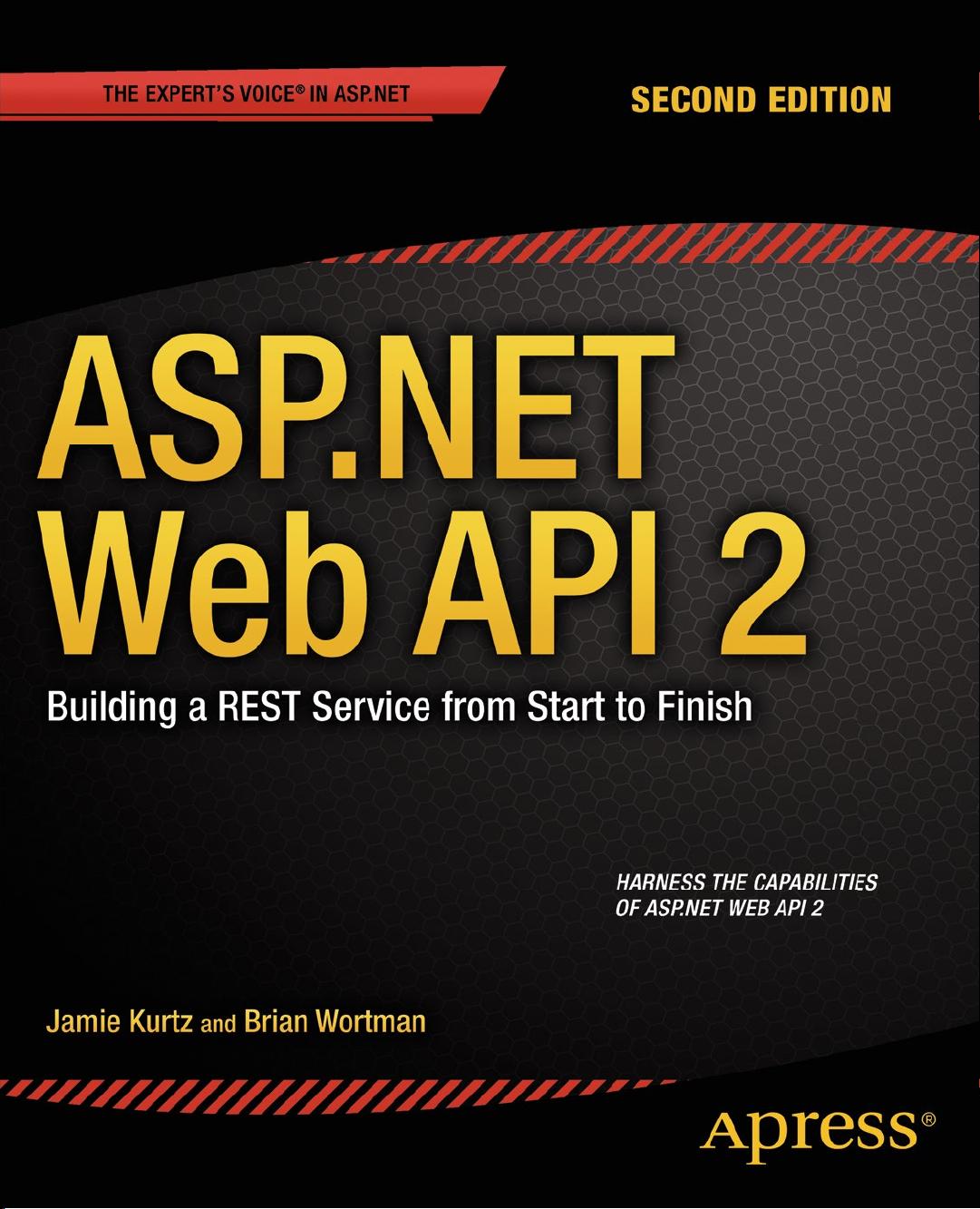
Kurtz
Wortman
Shelve in
.NET
User level:
Beginning–Advanced
www.apress.com
SOURCE CODE ONLINE
BOOKS FOR PROFESSIONALS BY PROFESSIONALS
®
ASP.NET Web API 2: Building a REST
Service from Start to Finish
The ASP.NET MVC Framework has always been a good platform on which to
implement REST-based services, but the introduction of the ASP.NET Web API
Framework raised the bar to a whole new level. Now in release version 2.1, the Web
API Framework has evolved into a powerful and refreshingly usable platform. This
concise book provides technical background and guidance that will enable you to
best use the ASP.NET Web API 2 Framework to build world-class REST services.
In this book you’ll learn:
• How to design a REST API
• New capabilities in ASP.NET Web API 2
• Understanding ASP.NET Web API controller activation
• Automatic lifetime management for database connections and transactions
• Using NHibernate with ASP.NET Web API
• Easily secure a REST service, using standards-based authentication and
authorization and JSON Web Tokens
• Supporting legacy SOAP callers with ASP.NET Web API
• How to expose relationships between resources
• Supporting partial resource updates under REST
• Web API versioning
Get ready for authors Jamie Kurtz and Brian Wortman to take you from zero to REST
service hero in no time at all. No prior experience with ASP.NET Web API is required; all
Web API-related concepts are introduced from basic principles and developed to the
point where you can use them in a production system. A good working knowledge of
C# and the .NET Framework are the only prerequisites to best benefit from this book.
SECOND
EDITION
RELATED
9781484 201107
52999
ISBN 978-1-4842-0110-7
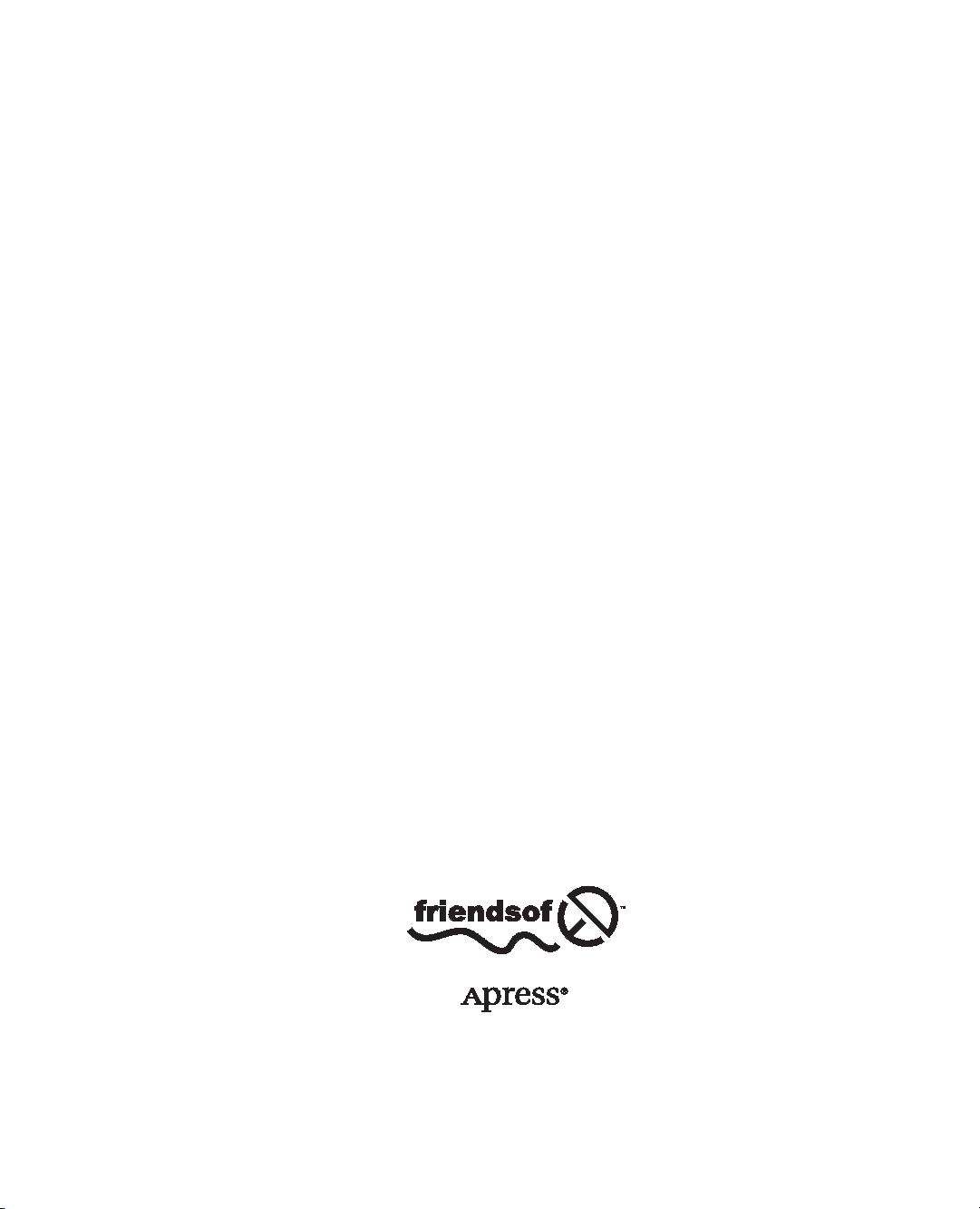
For your convenience Apress has placed some of the front
matter material after the index. Please use the Bookmarks
and Contents at a Glance links to access them.
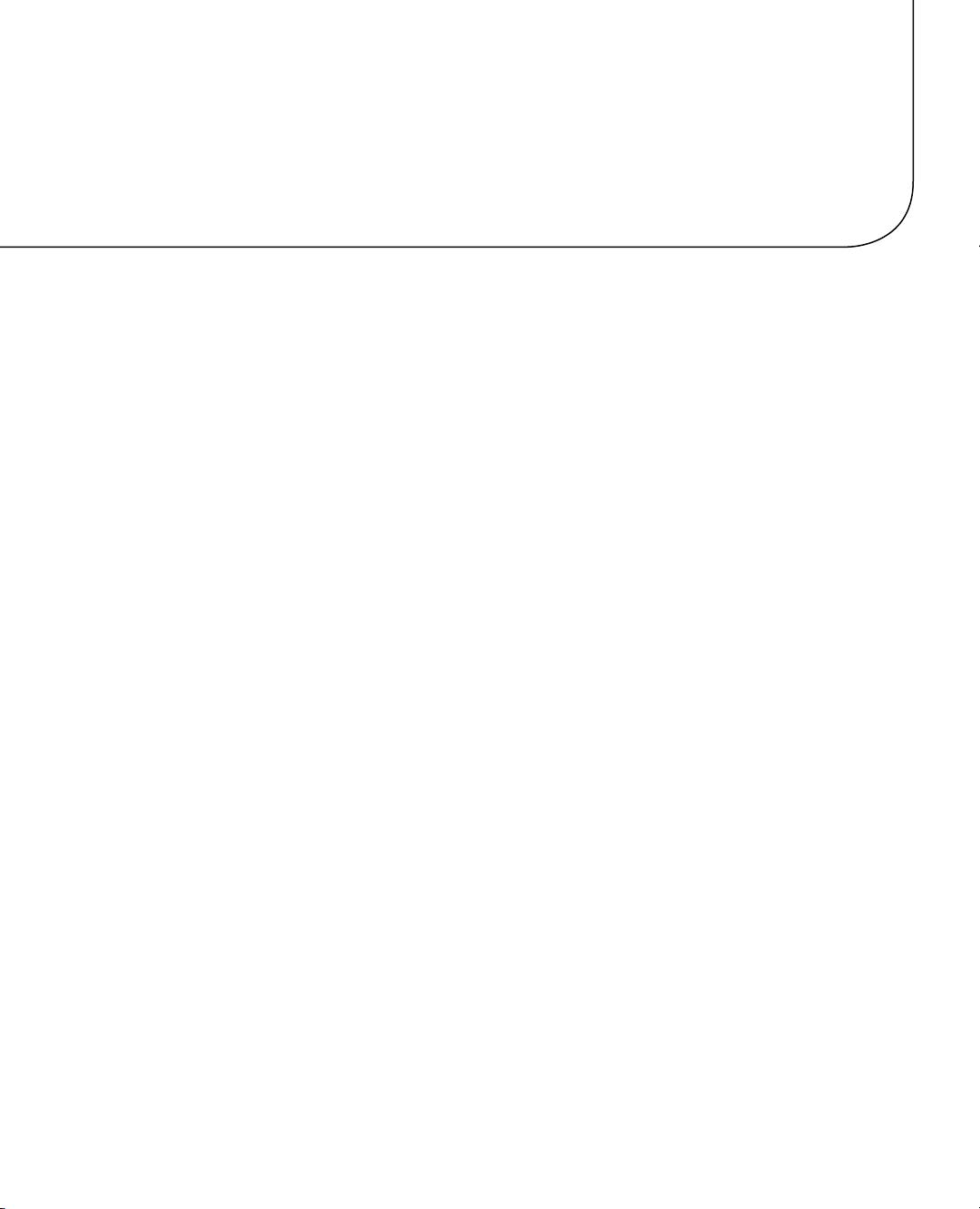
v
Contents at a Glance
About the Authors ��������������������������������������������������������������������������������������������������������������xiii
About the Technical Reviewer �������������������������������������������������������������������������������������������� xv
Acknowledgments ������������������������������������������������������������������������������������������������������������ xvii
Foreword ��������������������������������������������������������������������������������������������������������������������������� xix
Introduction ����������������������������������������������������������������������������������������������������������������������� xxi
Chapter 1: ASP�NET as a Service Framework ■ ��������������������������������������������������������������������1
Chapter 2: What Is RESTful? ■ ����������������������������������������������������������������������������������������������9
Chapter 3: Designing the Sample REST API ■ ���������������������������������������������������������������������21
Chapter 4: Building the Environment and Creating the Source Tree ■ �������������������������������31
Chapter 5: Up and Down the Stack with a POST ■ ��������������������������������������������������������������49
Chapter 6: Securing the Service ■ ������������������������������������������������������������������������������������117
Chapter 7: Dealing with Relationships, Partial Updates, and Other Complexities ■ ���������157
Chapter 8: Supporting Diverse Clients ■ ���������������������������������������������������������������������������209
Chapter 9: Completing the Picture ■ ��������������������������������������������������������������������������������221
Index ���������������������������������������������������������������������������������������������������������������������������������251
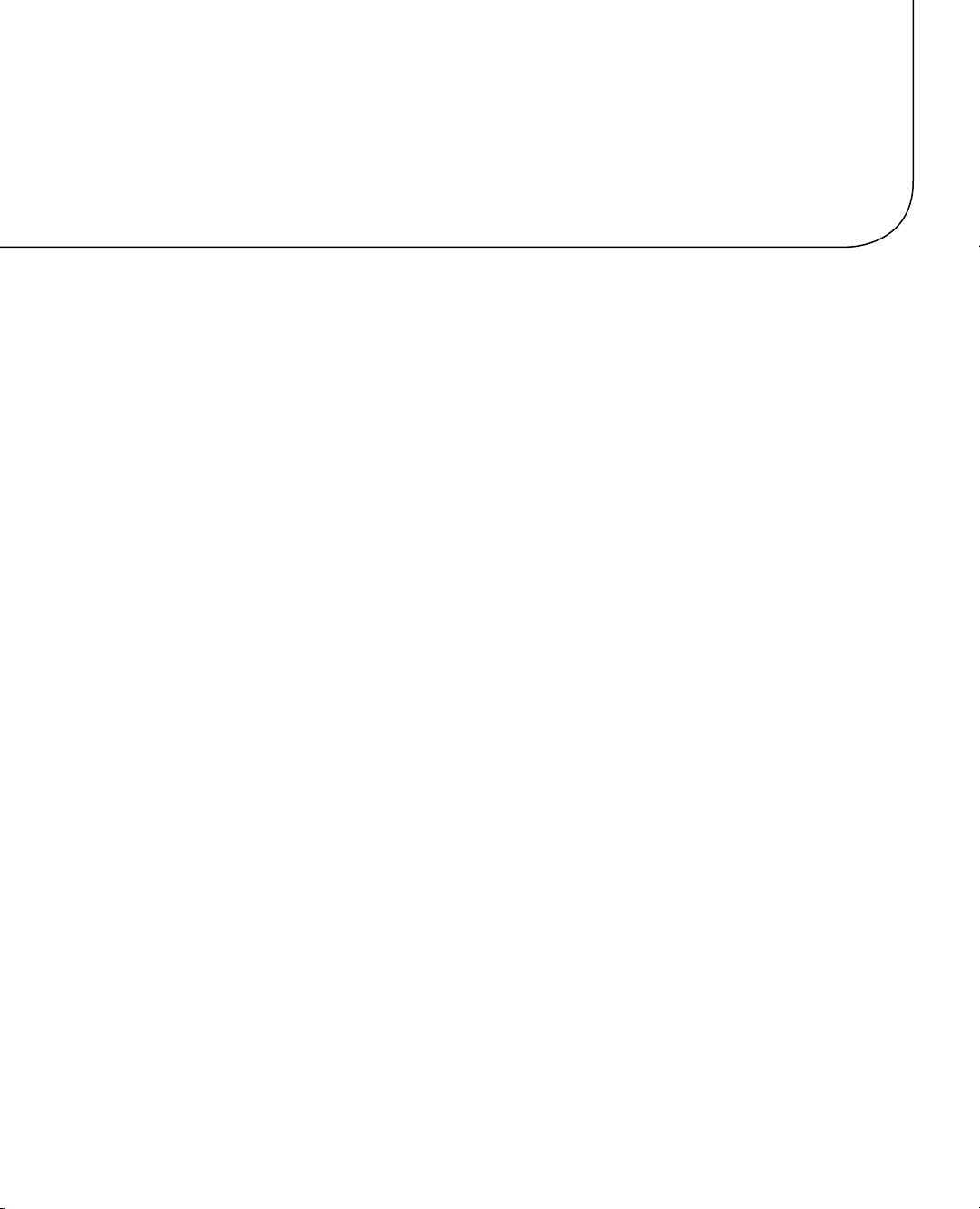
xxi
Introduction
With the introduction of services technology over a decade ago, Microsoft made it relatively easy to build and
support web services with the .NET Framework. Starting with XML Web Services, and then adding the broadly
capable Windows Communication Foundation (WCF) several years later, Microsoft gave .NET developers many
options for building SOAP-based services. With some basic conguration changes, you could support a wide array
of communication protocols, authentication schemes, message formats, and WS-* standards with WCF. But as
the world of connected devices evolved, the need arose within the community for a simple HTTP-only services
framework—without all of the capabilities (and complexity) of WCF. Developers realized that most of their newer
services did not require federated authentication or message encryption, nor did they require transactions or Web
Services Description Language (WSDL)–based discovery. And the services really only needed to communicate over
HTTP, not named pipes or MSMQ.
In short, the demand for mobile-to-service communication and browser-based, single-page applications started
increasing exponentially. It was no longer just large enterprise services talking SOAP/RPC to each other. Now a
developer needed to be able to whip up a JavaScript application, or 99-cent mobile app, in a matter of days—and
those applications needed a simple HTTP-only, JSON-compatible back end. e Internet needs of these applications
looked more and more like Roy Fielding’s vision of connected systems (i.e., REST).
And so Microsoft responded by creating the ASP.NET Web API, a super-simple yet very powerful framework
for building HTTP-only, JSON-by-default web services without all the fuss of WCF. Model binding works out of the
box, and returning Plain Old CLR Objects is drop-dead easy. Conguration is available (though almost completely
unnecessary), and you can spin up a RESTful service in a matter of minutes.
e previous edition of this book, ASP.NET MVC 4 and the Web API: Building a REST Service from Start to Finish,
spent a couple chapters describing REST and then dove into building a sample service with the rst version of
ASP.NET Web API. In a little over a hundred pages, you were guided through the process of implementing a working
service. But based on reader feedback, I discovered that a better job needed to be done in two major areas: fewer
opinions about patterns and best practices and various open source libraries, and more details on the ASP.NET Web
API itself. So when the second version of the ASP.NET Web API was released, Brian Wortman and I decided it was time
to release a version 2 of the book. Brian wanted to help me correct some glaring “bugs,” and also incorporate some
great new features found in ASP.NET Web API 2. And so this book was born.
In this second edition, we will cover all major features and capabilities of the ASP.NET Web API (version 2).
We also show you how to support API versioning, input validation, non-resource APIs, legacy/SOAP clients (this
is super cool!), partial updates with PATCH, adding hypermedia links to responses, and securing your service with
OAuth-compatible JSON Web Tokens. Improving upon the book’s rst edition, we continue to evolve the message
and techniques around REST principles, controller activation, dependency injection, database connection and
transaction management, and error handling. While we continue to leverage certain open source NuGet packages,
we have eliminated the chatter and opinions around those choices. We also spend more time on the code—lots of
code. Unit tests and all. And in the end, we build a simple KnockoutJS-based Single Page Application (SPA) that
demonstrates both JSON Web Token authentication and use of our new service.
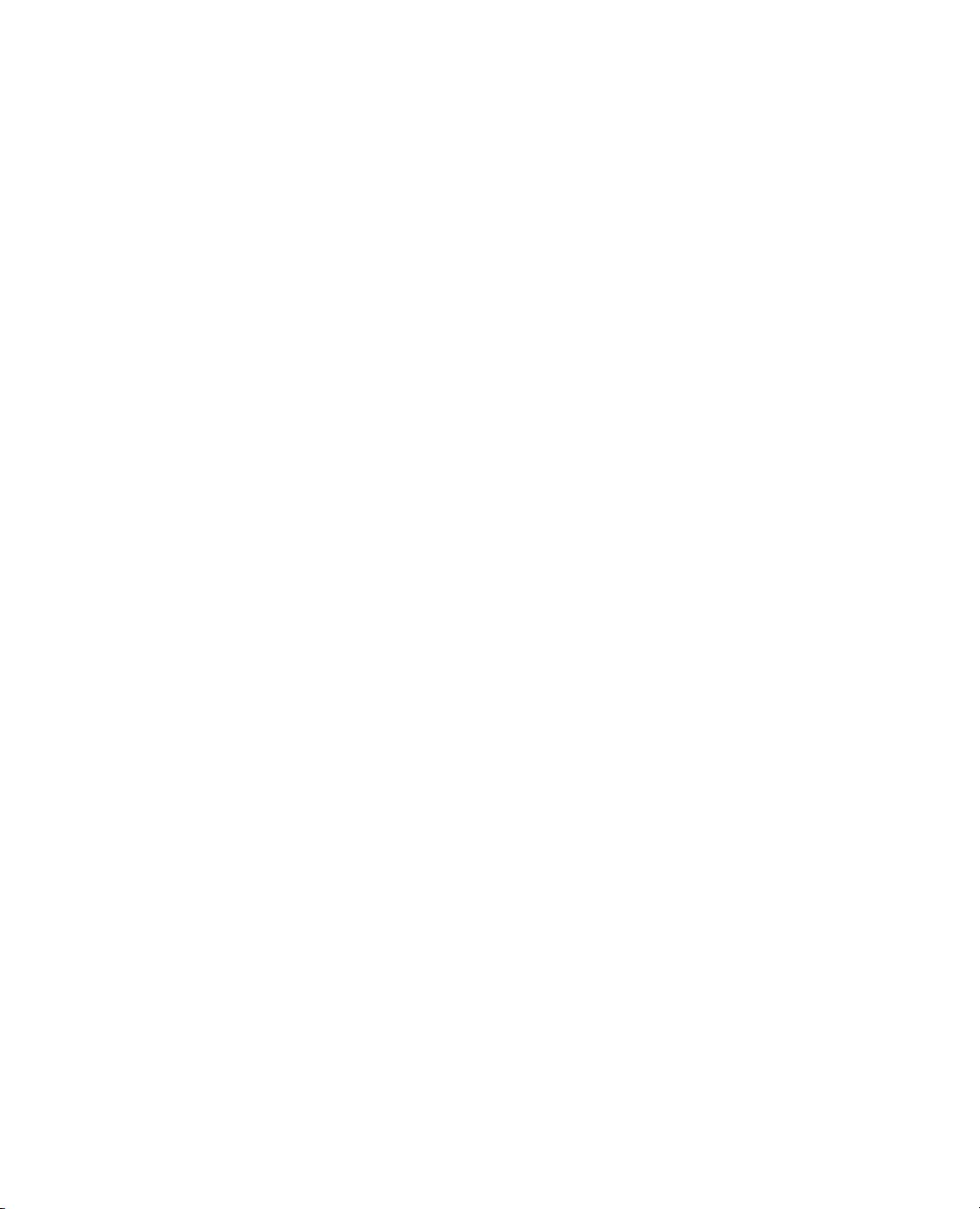
■ IntroduCtIon
xxii
We have also made improvements in response to your feedback regarding the source code that accompanied
the rst book. erefore, on GitHub you will nd a git repository containing all of the code for the task-management
service we will build together (https://github.com/jamiekurtz/WebAPI2Book). e repository contains one
branch per chapter, with multiple check-ins (or “commits”) per branch to help guide you step-by-step through the
implementation. e repository also includes a branch containing the completed task-management service, with
additional code to help reinforce the concepts that we cover in the book. Of course, feel free to use any of this code in
your own projects.
I am very excited about this book. Both Brian and I are rm believers in the “Agile way,” which at its heart is all
about feedback. So we carefully triaged each and every comment I received from the rst book and did our best to
make associated improvements. And I’m really excited about all the new features in the second version of ASP.NET
Web API. So many capabilities have been added, but Microsoft has managed to maintain the framework’s simplicity
and ease of use.
We hope you not only nd this book useful in your daily developer lives, but also nd it a pleasure to read. As
always, please share any feedback you have. We not only love to hear it, but your feedback is key in making continuous
improvements.
Cheers,
—Jamie Kurtz
(Brian Wortman)
剩余265页未读,继续阅读
资源评论
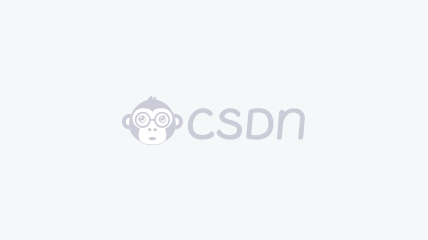
- u0107131592018-07-03读完集成理论,再通过源代码进行实践,从而打通了从应用集成理论到实际操作的桥梁。
- dpf4433982019-03-21很好,不错的资料

keokoo
- 粉丝: 0
- 资源: 7
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

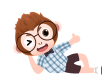
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


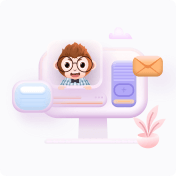
安全验证
文档复制为VIP权益,开通VIP直接复制
