XML Data Bindings {#mainpage}
=================
[TOC]
Introduction {#intro}
============
This is a detailed overview of the gSOAP XML data bindings concepts, usage, and
implementation. At the end of this document two examples are given to
illustrate the application of XML data bindings.
The first simple example `address.cpp` shows how to use wsdl2h to bind an XML
schema to C++. The C++ application reads and writes an XML file into and from
a C++ "address book" data structure. The C++ data structure is an STL vector
of address objects.
The second example `graph.cpp` shows how XML is serialized as a tree, digraph,
and cyclic graph. The digraph and cyclic graph serialization rules are similar
to SOAP 1.1/1.2 encoded multi-ref elements with id-ref attributes to link
elements through IDREF XML references, creating a an XML graph with pointers to
XML nodes.
These examples demonstrate XML data bindings only for relatively simple data
structures and types. The gSOAP tools support more than just these type of
structures to serialize in XML. There are practically no limits to
enable XML serialization of C and C++ types.
Support for XML schema components is unlimited. The wsdl2h tool maps schemas
to C and C++ using built-in intuitive mapping rules, while allowing the
mappings to be customized using a `typemap.dat` file with mapping instructions
for wsdl2h.
The information in this document is applicable to gSOAP 2.8.26 and later
versions that support C++11 features. However, C++11 is not required to use
this material and the examples included, unless we need smart pointers and
scoped enumerations. While most of the examples in this document are given in
C++, the concepts also apply to C with the exception of containers, smart
pointers, classes and their methods. None of these exceptions limit the use of
the gSOAP tools for C in any way.
The data binding concepts described in this document were first envisioned in
1999 by Prof. Robert van Engelen at the Florida State University. An
implementation was created in 2000, named "stub/skeleton compiler". The first
articles on its successor version "gSOAP" appeared in 2002. The principle of
mapping XSD components to C/C++ types and vice versa is now widely adopted in
systems and programming languages, including Java web services and by C# WCF.
We continue to be committed to our goal to empower C/C++ developers with
powerful autocoding tools for XML. Our commitment started in the very early
days of SOAP by actively participating in
[SOAP interoperability testing](http://www.whitemesa.com/interop.htm),
participating in the development and testing of the
[W3C XML Schema Patterns for Databinding Interoperability](http://www.w3.org/2002/ws/databinding),
and continues by contributing to the development of
[OASIS open standards](https://www.oasis-open.org) in partnership with leading
IT companies.
Mapping WSDL and XML schemas to C/C++ {#tocpp}
=====================================
To convert WSDL and XML schemas (XSD files) to code, use the wsdl2h command to
generate the data binding interface code that is saved to a special gSOAP
header file with WSDL service declarations and the data binding interface:
wsdl2h [options] -o file.h ... XSD and WSDL files ...
This command converts WSDL and XSD files to C++ (or pure C with wsdl2h option
`-c`) and saves the data binding interface to a gSOAP header file `file.h` that
uses familiar C/C++ syntax extended with `//gsoap` [directives](#directives)
and annotations. Notational conventions are used in the data binding interface
to declare serializable C/C++ types and functions for Web service operations.
The WSDL 1.1/2.0, SOAP 1.1/1.2, and XSD 1.0/1.1 standards are supported by the
gSOAP tools. In addition, the most popular WS specifications are also
supported, including WS-Addressing, WS-ReliableMessaging, WS-Discovery,
WS-Security, WS-Policy, WS-SecurityPolicy, and WS-SecureConversation.
This document focusses on XML data bindings. XML data bindings for C/C++ bind
XML schema types to C/C++ types. So integers in XML are bound to C integers,
strings in XML are bound to C or C++ strings, complex types in XML are bound to
C structs or C++ classes, and so on.
A data binding is dual. Either you start with WSDLs and/or XML schemas that
are mapped to equivalent C/C++ types, or you start with C/C++ types that are
mapped to XSD types. Either way, the end result is that you can serialize
C/C++ types in XML such that your XML is an instance of XML schema(s) and is
validated against these schema(s).
This covers all of the following standard XSD components with their optional
attributes and properties:
| XSD Component | Attributes and Properties |
| -------------- | ------------------------------------------------------------------------------------------------------------------- |
| schema | targetNamespace, version, elementFormDefault, attributeFormDefault, defaultAttributes |
| attribute | name, ref, type, use, default, fixed, form, targetNamespace, wsdl:arrayType |
| element | name, ref, type, default, fixed, form, nillable, abstract, substitutionGroup, minOccurs, maxOccurs, targetNamespace |
| simpleType | name |
| complexType | name, abstract, mixed, defaultAttributesApply |
| all | |
| choice | minOccurs, maxOccurs |
| sequence | minOccurs, maxOccurs |
| group | name, ref, minOccurs, maxOccurs |
| attributeGroup | name, ref |
| any | minOccurs, maxOccurs |
| anyAttribute | |
And also the following standard XSD directives are covered:
| Directive | Description |
| ---------- | ---------------------------------------------------------- |
| import | Imports a schema into the importing schema for referencing |
| include | Include schema component definitions into a schema |
| override | Override by replacing schema component definitions |
| redefine | Extend or restrict schema component definitions |
| annotation | Annotates a component |
The XSD facets and their mappings to C/C++ are:
| XSD Facet | Maps to |
| -------------- | ------------------------------------------------------------------------------------------- |
| enumeration | `enum` |
| simpleContent | class/struct wrapper with `__item` member |
| complexContent | class/struct |
| list | `enum*` bitmask (`enum*` enum

kalymm
- 粉丝: 0
- 资源: 8
最新资源
- 几何物体检测43-YOLO(v5至v9)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 基于cruise的燃料电池功率跟随仿真,按照丰田氢能源车型搭建,在wltc工况下跟随效果好,最高车速175,最大爬坡30,百公里9s均已实现 1.模型通过cruise simulink联合仿真,策略
- C#源码 上位机 联合Visionpro 通用框架开发源码,已应用于多个项目,整套设备程序,可以根据需求编出来,具体Vpp功能自己编 程序包含功能 1.自动设置界面窗体个数及分布 2.照方式以命令触
- 程序名称:悬架设计计算程序 开发平台:基于matlab平台 计算内容:悬架偏频刚度挠度;螺旋弹簧,多片簧,少片簧,稳定杆,减震器的匹配计算;悬架垂向纵向侧向力学、纵倾、侧倾校核等;独立悬架杠杆比,等效
- 华为OD+真题及解析+智能驾驶
- jQuery信息提示插件
- 基于stm32的通信系统,sim800c与服务器通信,无线通信监测,远程定位,服务器通信系统,gps,sim800c,心率,温度,stm32 由STM32F103ZET6单片机核心板电路、DS18B2
- 充电器检测9-YOLO(v5至v11)、COCO、Create充电器检测9L、Paligemma、TFRecord、VOC数据集合集.rar
- 华为OD+考试真题+实现过程
- 保险箱检测51-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


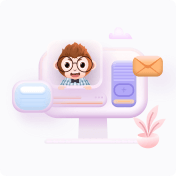