package com.lxyz.controller;
import org.springframework.beans.propertyeditors.CustomDateEditor;
import org.springframework.web.bind.WebDataBinder;
import org.springframework.web.bind.annotation.InitBinder;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
import java.text.SimpleDateFormat;
import java.util.Date;
/**
* Created by 18761 on 2017/12/22.
*/
public class BaseController {
/**
* @param binder
*/
@InitBinder
public void initBinder(WebDataBinder binder) {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
dateFormat.setLenient(false);
binder.registerCustomEditor(Date.class, new CustomDateEditor(dateFormat, true));
}
/**
* @param binder
*/
@InitBinder("pagination")
public void paginationBinder(WebDataBinder binder) {
binder.setFieldDefaultPrefix("pagination.");
}
/**
* 输出流. 缓冲区大小为1024.
*
* @param response
* @param input 输入流.本方法会自动关闭流对象,不需要独立关闭.
* @throws java.io.IOException 流出错.
* @see #outputStream(javax.servlet.http.HttpServletResponse,
* java.io.InputStream, int)
*/
protected void outputStream(HttpServletResponse response, InputStream input) throws IOException {
outputStream(response, input, 1024);
}
/**
* 输出流
*
* @param response
* @param input 输入流.本方法会自动关闭流对象,不需要独立关闭.
* @param bufferSize 缓冲区大小.
* @throws java.io.IOException 流出错.
*/
protected void outputStream(HttpServletResponse response, InputStream input, int bufferSize) throws IOException {
OutputStream out = null;
try {
out = response.getOutputStream();
byte[] bs = new byte[1024];
int s;
while ((s = input.read(bs)) >= 0) {
out.write(bs, 0, s);
}
out.flush();
} catch (IOException ex) {
ex.printStackTrace();
} finally {
try {
if (input != null) {
input.close();
}
if (out != null) {
out.close();
}
} catch (IOException ex) {
throw ex;
}
}
}
/**
* 绘制临时文件
* @param network_resource_file_path
* @param tempFile
*/
public void drawingFile(String network_resource_file_path,File tempFile){
InputStream inStream = null;
FileOutputStream fos = null;
try{
URL url = new URL(network_resource_file_path);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setConnectTimeout(5 * 1000);
inStream = new BufferedInputStream(conn.getInputStream());
fos = new FileOutputStream(tempFile);
byte[] data = castInputStreamToByteArray(inStream);
fos.write(data);
}catch(Exception ex){
ex.printStackTrace();
} finally {
try {
if (fos != null) {
fos.close();
}
if (inStream != null) {
inStream.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
/**
* @param inStream
* @return
* @throws Exception
*/
public byte[] castInputStreamToByteArray(InputStream inStream) throws Exception {
ByteArrayOutputStream outStream = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len = 0;
while ((len = inStream.read(buffer)) != -1) {
outStream.write(buffer, 0, len);
}
inStream.close();
return outStream.toByteArray();
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
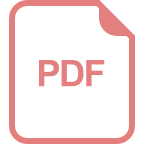
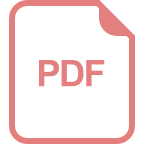
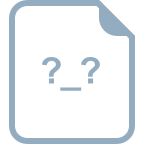
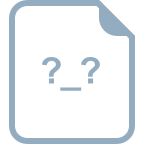
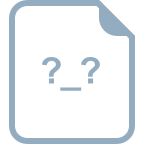
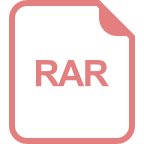
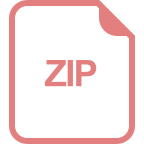
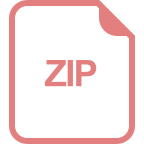
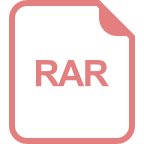
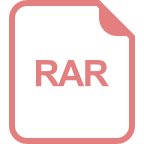
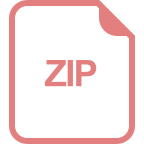
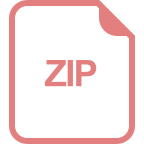
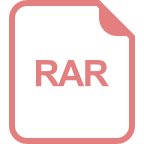
收起资源包目录

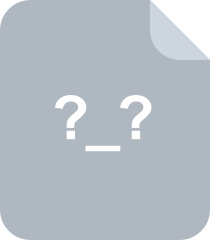
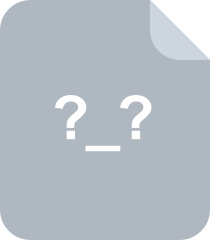
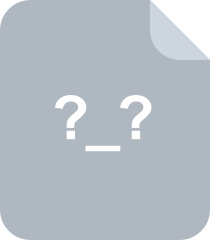
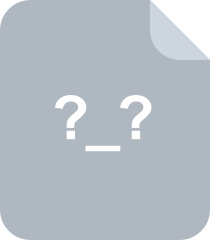
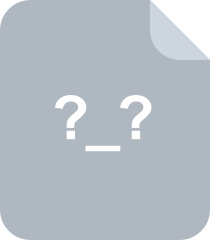
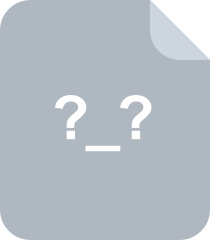
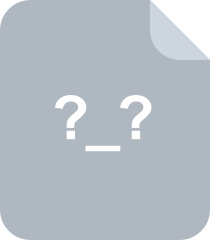
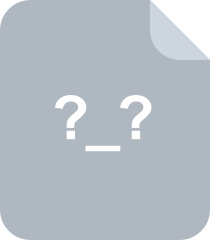
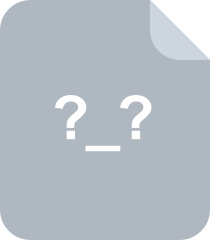
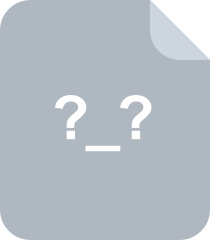
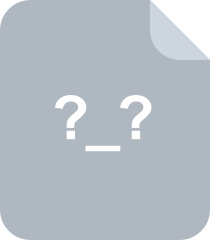
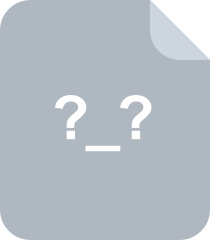
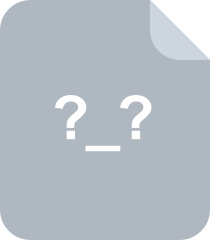
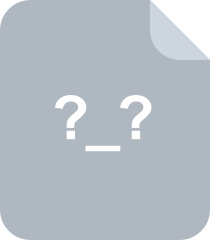
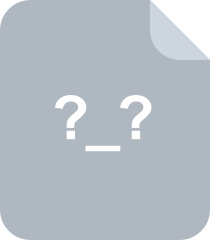
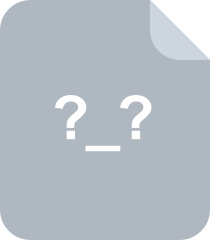
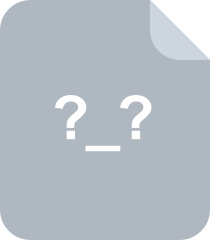
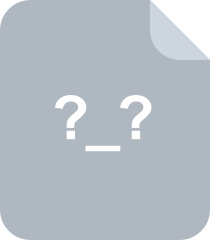
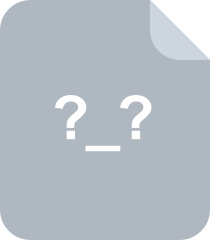
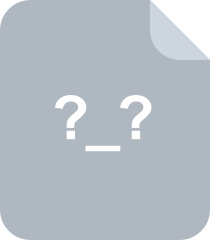
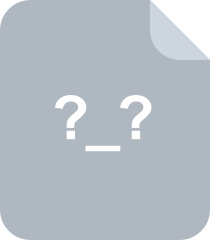
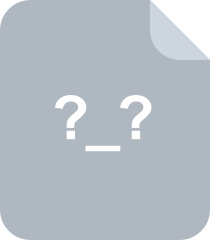
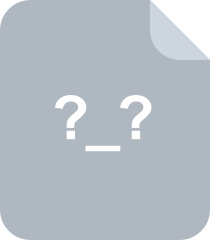
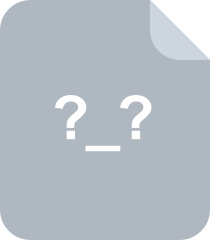
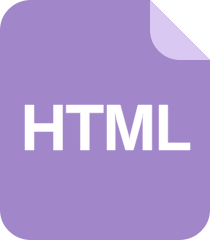
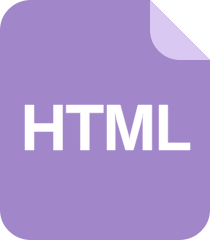
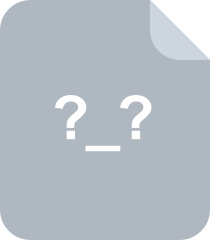
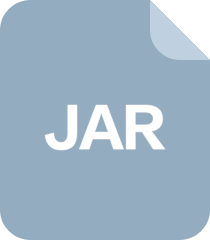
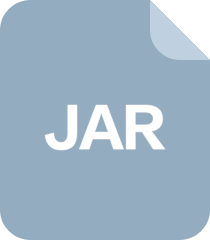
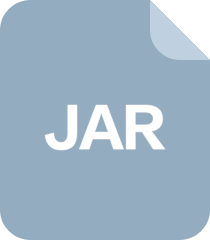
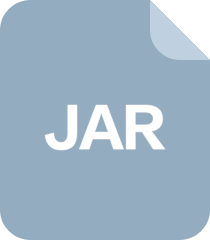
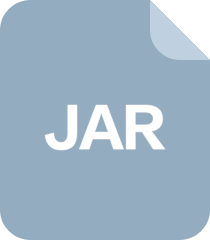
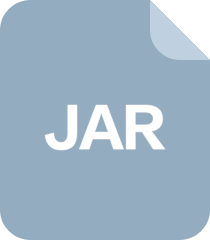
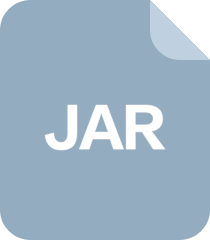
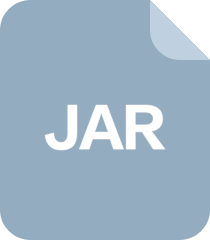
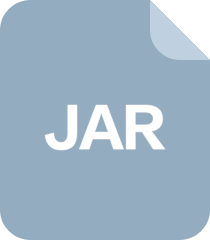
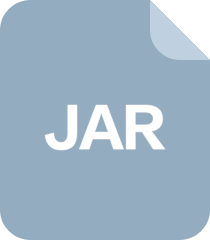
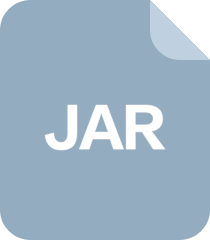
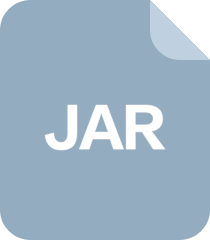
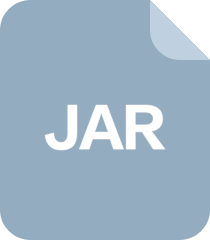
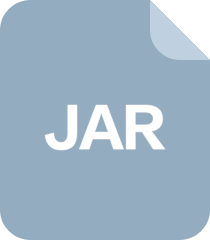
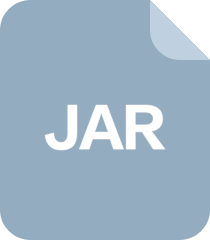
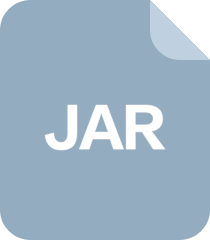
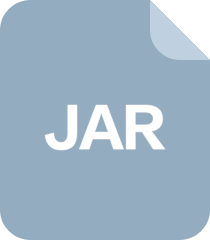
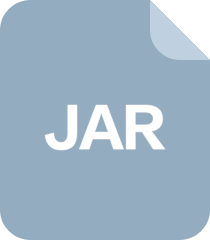
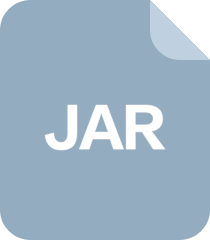
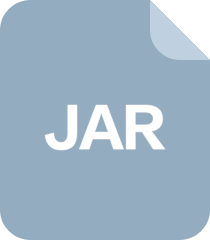
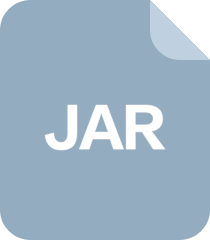
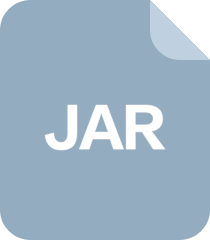
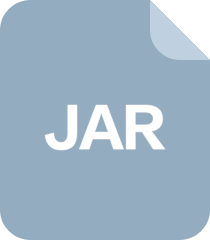
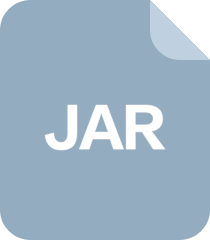
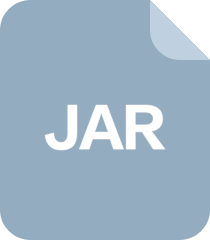
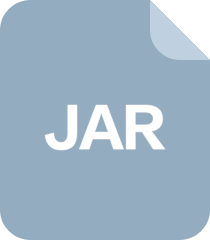
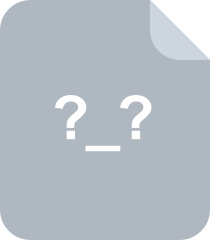
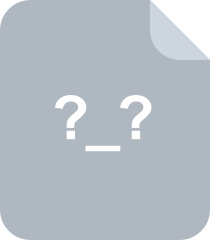
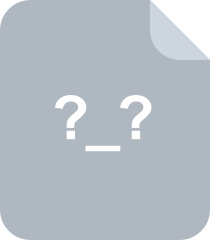
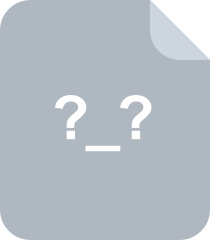
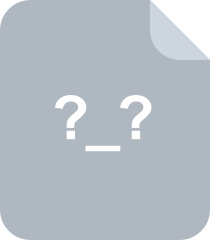
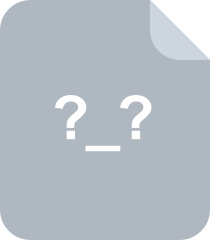
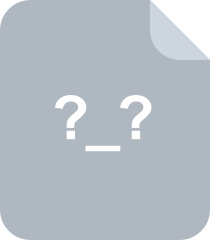
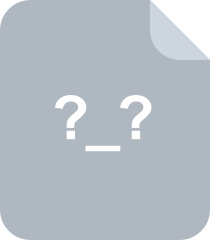
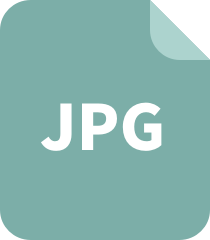
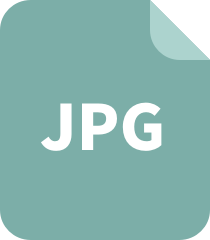
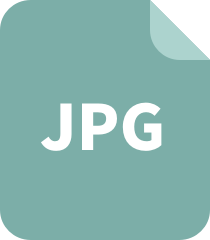
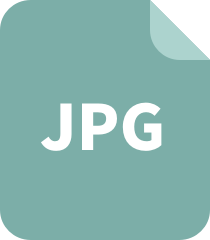
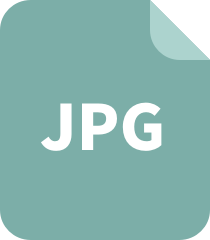
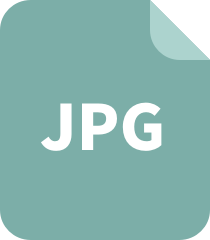
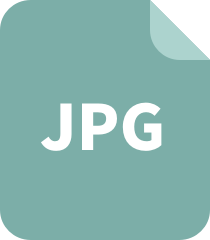
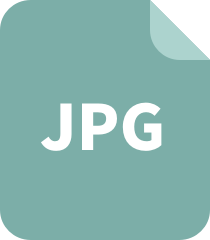
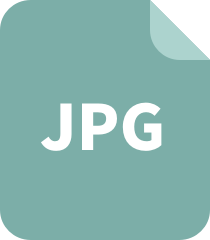
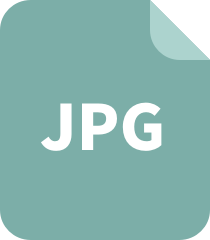
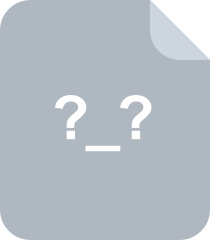
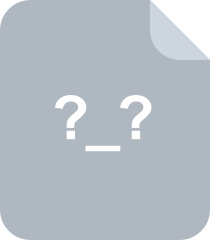
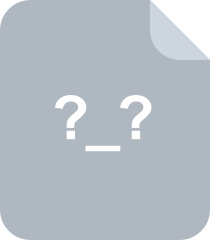
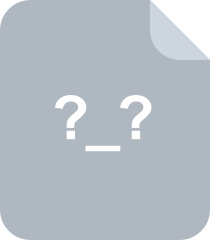
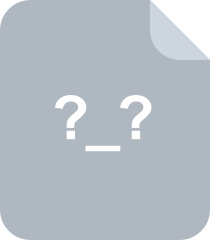
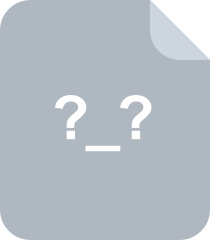
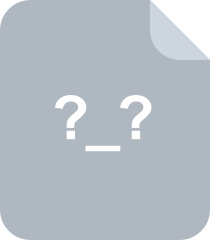
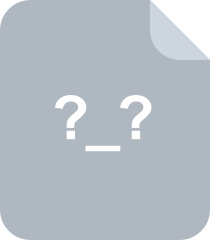
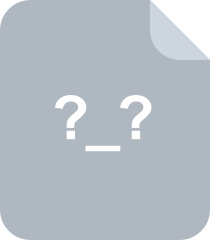
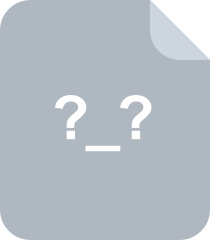
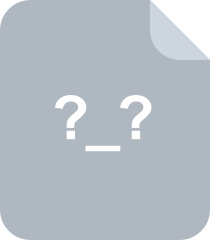
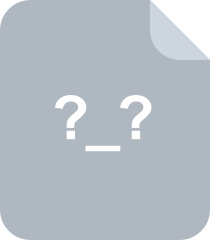
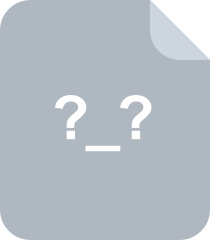
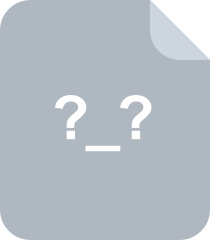
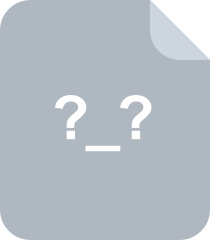
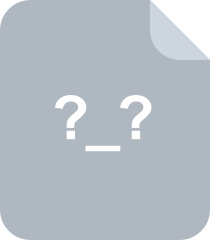
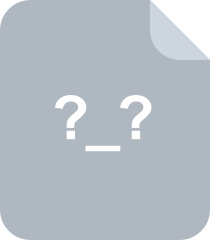
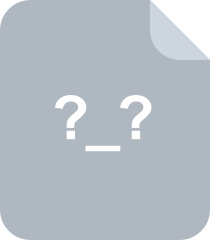
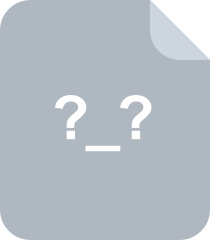
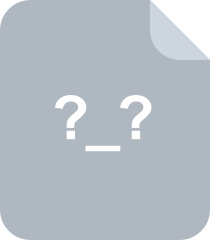
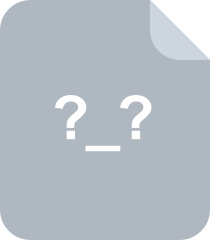
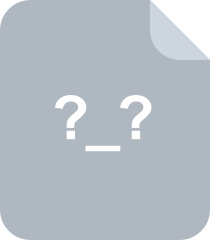
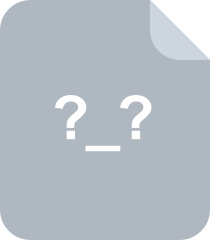
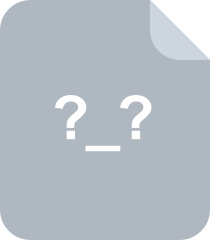
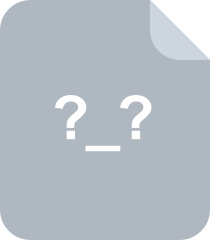
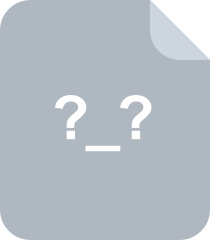
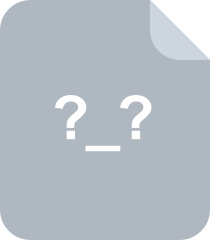
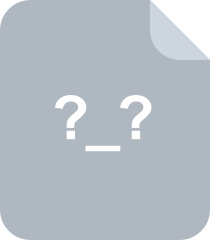
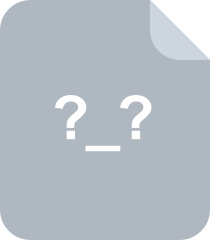
共 120 条
- 1
- 2
资源评论
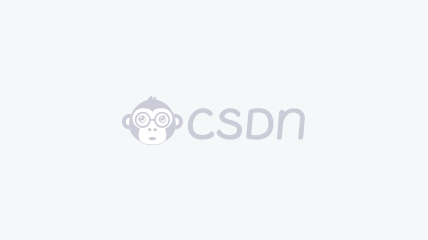

scaling_heights
- 粉丝: 257
- 资源: 49
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

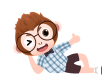
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


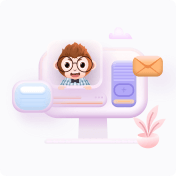
安全验证
文档复制为VIP权益,开通VIP直接复制
