// ---------------------------------------------------------------------------------------------------------------------
#include "QVector"
#include "QTextCodec"
#include "QDebug"
#include "QJson.h"
namespace JSK
{
JSONObject::JSONObject(const QString& key)
{
init();
setKey(key);
}
void JSONObject::init()
{
m_key = "";
m_type = vtfNull;
m_string = "";
m_int = 0;
m_double = 0.0;
m_bool = false;
m_datetime = QDateTime();
}
JSONObject::~JSONObject()
{
for( int i=0;i<m_list.count();i++ )
{
JSONObject* item = m_list.at(i);
delete item;
}
}
QString JSONObject::dequote(QString text)
{
int idx;
while((idx=text.indexOf("\\u"))>=0)
{
int nHex = text.mid(idx+2, 4).toInt(0, 16);
text.replace(idx, 6, QChar(nHex));
}
return text;
}
QString JSONObject::quote(const QString& Text)
{
QString ret;
QStdWString ws = Text.toStdWString();
const wchar_t* ptr = ws.c_str();
while(*ptr)
{
switch(*ptr)
{
case L'\\': ret += "\\\\"; break;
case L'\"': ret += "\\\""; break;
case L'\b': ret += "\\b"; break;
case L'\t': ret += "\\t"; break;
case L'\n': ret += "\\n"; break;
case L'\f': ret += "\\f"; break;
case L'\r': ret += "\\r"; break;
default:
if ( *ptr > 255 )
{
uint16_t v = *ptr;
QString S = QString::number(v, 16);
ret += "\\u" + S;
}else
{
ret += *ptr;
}
break;
}
ptr++;
}
return ret;
}
QString JSONObject::getKey() const
{
return m_key;
}
void JSONObject::setKey(QString value)
{
m_key = value.trimmed();
}
QString JSONObject::getJsonKey() const
{
return "\"" + quote(m_key) + "\"";
}
QString JSONObject::getJsonValue() const
{
QString ret;
switch(m_type)
{
case 0: // null
ret = "null";
break;
case 1: // string
ret = "\"" + quote(m_string) + "\"";
break;
case 2: // int
ret = QString::number(m_int);
break;
case 3: // double
ret = QString::number(m_double);
break;
case 4: // bool
ret = m_bool ? "true" : "false";
break;
case 5: // datetime
ret = "\"" + m_datetime.toString("yyyy/MM/dd HH:mm:ss.zzz") + "\"";
break;
default:
ret = "json.value.type error.";
break;
}
return ret;
}
JSONObject* JSONObject::addChild(const QString& key, int type)
{
JSONObject* ret = new JSONObject(key);
ret->setType(type);
m_list.append(ret);
return ret;
}
JSONObject* JSONObject::add(const QString& key, const char* value)
{
JSONObject* ret = new JSONObject(key);
ret->setValue(value);
m_list.append(ret);
return ret;
}
JSONObject* JSONObject::add(const QString& key)
{
JSONObject* ret = new JSONObject(key);
m_list.append(ret);
return ret;
}
JSONObject* JSONObject::add(const QString& key, const QString& value)
{
JSONObject* ret = new JSONObject(key);
ret->setValue(value);
m_list.append(ret);
return ret;
}
JSONObject* JSONObject::add(const QString& key, int value)
{
JSONObject* ret = new JSONObject(key);
ret->setValue(value);
m_list.append(ret);
return ret;
}
JSONObject* JSONObject::add(const QString& key, double value)
{
JSONObject* ret = new JSONObject(key);
ret->setValue(value);
m_list.append(ret);
return ret;
}
JSONObject* JSONObject::add(const QString& key, bool value)
{
JSONObject* ret = new JSONObject(key);
ret->setValue(value);
m_list.append(ret);
return ret;
}
JSONObject* JSONObject::add(const QString& key, QDateTime value)
{
JSONObject* ret = new JSONObject(key);
ret->setValue(value);
m_list.append(ret);
return ret;
}
int JSONObject::deleteChildren(const QString& key)
{
int ret = 0;
for( int i=m_list.count();i>0;i-- )
{
JSONObject* item = m_list.at(i-1);
if ( item->m_key.compare(key)==0 )
{
m_list.removeAt(i-1);
delete item;
ret ++;
}
}
return ret;
}
void JSONObject::clearChildren()
{
for( int i=0;i<m_list.count();i++ )
{
JSONObject* item = m_list.at(i);
delete item;
}
m_list.clear();
}
void JSONObject::clear()
{
init();
clearChildren();
}
QStringList JSONObject::keys() const
{
QStringList ret;
for( int i=0;i<m_list.count();i++ )
{
JSONObject* item = m_list.at(i);
ret.append(item->getKey());
}
return ret;
}
JSONObject* JSONObject::get(const QString& key) const
{
JSONObject* ret = NULL;
for( int i=0;i<m_list.count();i++ )
{
JSONObject* item = m_list.at(i);
if ( item->m_key.compare(key)==0 )
{
ret = item;
break;
}
}
return ret;
}
QString JSONObject::getString(const QString& key) const
{
JSONObject* res = get(key);
return res != NULL ? res->getString() : QString();
}
int JSONObject::getInt(const QString& key) const
{
JSONObject* res = get(key);
return res != NULL ? res->getInt() : 0;
}
double JSONObject::getDouble(const QString& key) const
{
JSONObject* res = get(key);
return res != NULL ? res->getDouble() : 0.0;
}
bool JSONObject::getBoolean(const QString& key) const
{
JSONObject* res = get(key);
return res != NULL ? res->getBoolean() : false;
}
QDateTime JSONObject::getDateTime(const QString& key) const
{
JSONObject* res = get(key);
return res != NULL ? res->getDateTime() : QDateTime();
}
QString JSONObject::toString() const
{
QString values;
if ( m_list.count() >0 )
{
for( int i=0;i<m_list.count();i++ )
{
JSONObject* item = m_list.at(i);
if ( m_type == vtfArray )
{
values += "," + item->getJsonValue();
}else
{
values += "," + item->toString(); //item->getJsonKey() + ":" + item->getJsonValue();
}
}
if ( ! values.isEmpty() )
{
values.remove(0, 1);
}
if ( m_type == vtfArray )
{
values = "[" + values + "]";
}else
{
values = "{" + values + "}";
}
}else
{
if ( m_type == vtfArray )
{
values = "[]";
}else
{
values = getJsonValue();
}
}
return m_key.isEmpty() ? values : getJsonKey() + ":" + values;
}
QString JSONObject::getString() const
{
QString ret;
switch(m_type)
{
default:
case 0: // null
ret = "";
break;
case 1: // string
ret = m_string;
break;
case 2: // int
ret = QString::number(m_int);
break;
case 3: // double
ret = QString::number(m_double);
break;
case 4: // bool
ret = m_bool ? "true" : "false";
break;
case 5: // datetime
ret = "\"" + m_datetime.toString("yyyy/MM/dd HH:mm:ss.zzz") + "\"";
break;
}
return ret;
}
int JSONObject::getInt() const
{
int ret;
switch(m_type)
{
default:
case 0: // null
ret = 0;
break;
case 1: // string
ret = m_string.toInt();
break;
case 2: // int
ret = m_int;
break;
case 3: // double
ret = m_double;
break;
case 4: // bool
ret = m_bool ? 1 : 0;
break;
case 5: // datetime
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
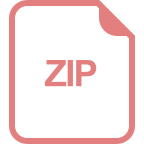
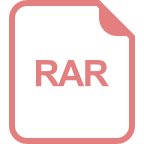
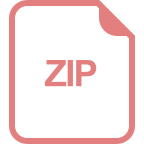
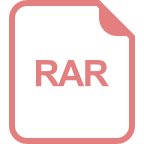
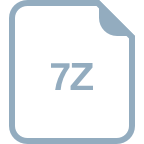
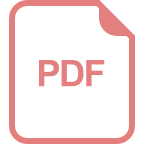
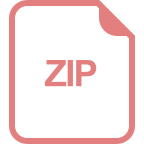
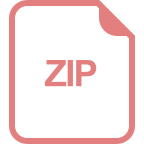
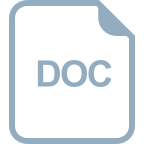
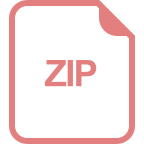
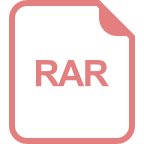
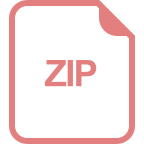
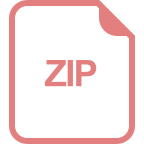
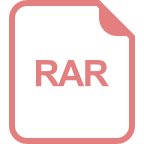
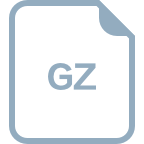
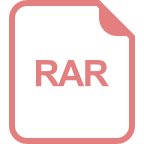
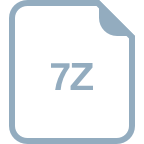
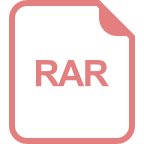
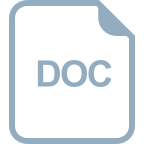
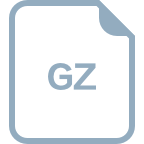
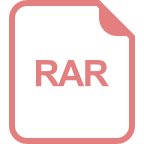
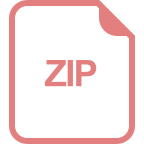
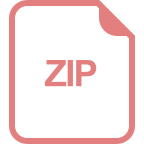
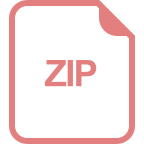
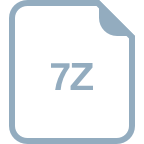
收起资源包目录

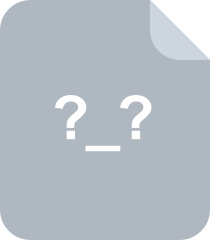
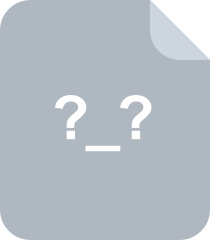
共 2 条
- 1
资源评论
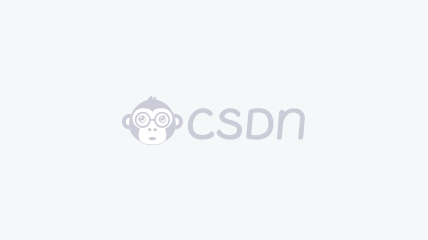
- wushuang_guan2014-05-08一个头文件,一个cpp文件,但还是知道该怎么使用,不过谢谢 了
- wjp3286707122012-12-23只有一个.H和一个.c文件,看了像是写了json的使用函数!我用的平台已有了这些库函数不是我想要的使用方法例子,不过还是感谢分享把!
- qq_343781962017-11-06没太多用,仅作参考
- pan_fenf2014-07-29解析这块不是很好
- vacantChan2015-01-04使用了qt5后自带json,还是比较 方便的,升级还是好的!!!

Jonix
- 粉丝: 78
- 资源: 13
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

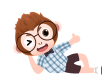
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


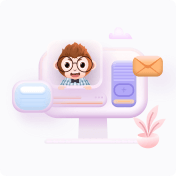
安全验证
文档复制为VIP权益,开通VIP直接复制
