/* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/*
* httpd.c: simple http daemon for answering WWW file requests
*
*
* 03-21-93 Rob McCool wrote original code (up to NCSA HTTPd 1.3)
*
* 03-06-95 blong
* changed server number for child-alone processes to 0 and changed name
* of processes
*
* 03-10-95 blong
* Added numerous speed hacks proposed by Robert S. Thau (rst@ai.mit.edu)
* including set group before fork, and call gettime before to fork
* to set up libraries.
*
* 04-14-95 rst / rh
* Brandon's code snarfed from NCSA 1.4, but tinkered to work with the
* Apache server, and also to have child processes do accept() directly.
*
* April-July '95 rst
* Extensive rework for Apache.
*/
#ifndef SHARED_CORE_BOOTSTRAP
#ifndef SHARED_CORE_TIESTATIC
#ifdef SHARED_CORE
#define REALMAIN ap_main
int ap_main(int argc, char *argv[]);
#else
#define REALMAIN main
#endif
#define CORE_PRIVATE
#include "httpd.h"
#include "http_main.h"
#include "http_log.h"
#include "http_config.h" /* for read_config */
#include "http_protocol.h" /* for read_request */
#include "http_request.h" /* for process_request */
#include "http_conf_globals.h"
#include "http_core.h" /* for get_remote_host */
#include "http_vhost.h"
#include "util_script.h" /* to force util_script.c linking */
#include "util_uri.h"
#include "scoreboard.h"
#include "multithread.h"
#include <sys/stat.h>
#ifdef USE_SHMGET_SCOREBOARD
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#endif
#ifdef SecureWare
#include <sys/security.h>
#include <sys/audit.h>
#include <prot.h>
#endif
#ifdef WIN32
#include "../os/win32/getopt.h"
#elif !defined(BEOS) && !defined(TPF) && !defined(NETWARE) && !defined(OS390) && !defined(CYGWIN)
#include <netinet/tcp.h>
#endif
#ifdef HAVE_BSTRING_H
#include <bstring.h> /* for IRIX, FD_SET calls bzero() */
#endif
#ifdef HAVE_SET_DUMPABLE /* certain levels of Linux */
#include <sys/prctl.h>
#endif
#ifdef MULTITHREAD
/* special debug stuff -- PCS */
/* Set this non-zero if you are prepared to put up with more than one log entry per second */
#define SEVERELY_VERBOSE 0
/* APD1() to APD5() are macros to help us debug. They can either
* log to the screen or the error_log file. In release builds, these
* macros do nothing. In debug builds, they send messages at priority
* "debug" to the error log file, or if DEBUG_TO_CONSOLE is defined,
* to the console.
*/
# ifdef _DEBUG
# ifndef DEBUG_TO_CONSOLE
# define APD1(a) ap_log_error(APLOG_MARK,APLOG_DEBUG|APLOG_NOERRNO,server_conf,a)
# define APD2(a,b) ap_log_error(APLOG_MARK,APLOG_DEBUG|APLOG_NOERRNO,server_conf,a,b)
# define APD3(a,b,c) ap_log_error(APLOG_MARK,APLOG_DEBUG|APLOG_NOERRNO,server_conf,a,b,c)
# define APD4(a,b,c,d) ap_log_error(APLOG_MARK,APLOG_DEBUG|APLOG_NOERRNO,server_conf,a,b,c,d)
# define APD5(a,b,c,d,e) ap_log_error(APLOG_MARK,APLOG_DEBUG|APLOG_NOERRNO,server_conf,a,b,c,d,e)
# else
# define APD1(a) printf("%s\n",a)
# define APD2(a,b) do { printf(a,b);putchar('\n'); } while(0);
# define APD3(a,b,c) do { printf(a,b,c);putchar('\n'); } while(0);
# define APD4(a,b,c,d) do { printf(a,b,c,d);putchar('\n'); } while(0);
# define APD5(a,b,c,d,e) do { printf(a,b,c,d,e);putchar('\n'); } while(0);
# endif
# else /* !_DEBUG */
# define APD1(a)
# define APD2(a,b)
# define APD3(a,b,c)
# define APD4(a,b,c,d)
# define APD5(a,b,c,d,e)
# endif /* _DEBUG */
#endif /* MULTITHREAD */
/* This next function is never used. It is here to ensure that if we
* make all the modules into shared libraries that core httpd still
* includes the full Apache API. Without this function the objects in
* main/util_script.c would not be linked into a minimal httpd.
* And the extra prototype is to make gcc -Wmissing-prototypes quiet.
*/
API_EXPORT(void) ap_force_library_loading(void);
API_EXPORT(void) ap_force_library_loading(void) {
ap_add_cgi_vars(NULL);
}
#include "explain.h"
#if !defined(max)
#define max(a,b) (a > b ? a : b)
#endif
#ifdef WIN32
#include "../os/win32/service.h"
#include "../os/win32/registry.h"
#define DEFAULTSERVICENAME "Apache"
#define PATHSEPARATOR '\\'
#else
#define PATHSEPARATOR '/'
#endif
#ifdef MINT
long _stksize = 32768;
#endif
#ifdef USE_OS2_SCOREBOARD
/* Add MMAP style functionality to OS/2 */
#define INCL_DOSMEMMGR
#define INCL_DOSEXCEPTIONS
#define INCL_DOSSEMAPHORES
#include <os2.h>
#include <umalloc.h>
#include <stdio.h>
caddr_t create_shared_heap(const char *, size_t);
caddr_t get_shared_heap(const char *);
#endif
DEF_Explain
/* Defining GPROF when compiling uses the moncontrol() function to
* disable gprof profiling in the parent, and enable it only for
* request processing in children (or in one_process mode). It's
* absolutely required to get useful gprof results under linux
* because the profile itimers and such are disabled across a
* fork(). It's probably useful elsewhere as well.
*/
#ifdef GPROF
extern void moncontrol(int);
#define MONCONTROL(x) moncontrol(x)
#else
#define MONCONTROL(x)
#endif
#ifndef MULTITHREAD
/* this just need to be anything non-NULL */
void *ap_dummy_mutex = &ap_dummy_mutex;
#endif
/*
* Actual definitions of config globals... here because this is
* for the most part the only code that acts on 'em. (Hmmm... mod_main.c?)
*/
#ifdef NETWARE
BOOL ap_main_finished = FALSE;
unsigned int ap_thread_stack_size = 65536;
#endif
int ap_thread_count = 0;
API_VAR_EXPORT int ap_standalone=0;
API_VAR_EXPORT int ap_configtestonly=0;
int ap_docrootcheck=1;
API_VAR_EXPORT uid_t ap_user_id=0;
API_VAR_EXPORT char *ap_user_name=NULL;
API_VAR_EXPORT gid_t ap_group_id=0;
#ifdef MULTIPLE_GROUPS
gid_t group_id_list[NGROUPS_MAX];
#endif
API_VAR_EXPORT int ap_max_requests_per_child=0;
API_VAR_EXPORT int ap_threads_per_child=0;
API_VAR_EXPORT int ap_excess_requests_per_child=0;
API_VAR_EXPORT char *ap_pid_fname=NULL;
API_VAR_EXPORT char *ap_scoreboard_fname=NULL;
API_VAR_EXPORT char *ap_lock_fname=NULL;
API_VAR_EXPORT char *ap_server_argv0=NULL;
API_VAR_EXPORT struct in_addr ap_bind_address={0};
API_VAR_EXPORT int ap_daemons_to_start=0;
API_VAR_EXPORT int ap_daemons_min_free=0;
API_VAR_EXPORT int ap_daemons_max_free=0;
API_VAR_EXPORT int ap_daemons_limit=0;
API_VAR_EXPORT time_t ap_restart_time=0;
API_VAR_EXPORT int ap_suexec_enabled = 0;
API_VAR_EXPORT int ap_listenbacklog=0;
#ifdef AP_ENABLE_EXCEPTION_HOOK
int ap_exception_hook_enabled=0;
#endif
struct accept_mutex_methods_s {
void (*child_init)(pool *p);
void (*init)(pool *p);
void (*on)(void);
void (*off)(void);
char *name;
};
typedef struct accept_mutex_methods_s accept_mutex_methods_s;
accept_mutex_methods_s *amutex;
#ifdef SO_ACCEPTFILTER
int ap_acceptfilter =
#ifdef AP_ACCEPTFILTER_OFF
0;
#else
1;
#endif
#endif
int ap_dump_settings = 0;
API_VAR_EXPORT int ap_extended_status = 0;
/*
* The max child slot ever assigned, preserved across restarts. Necessary
* to deal with MaxClients changes across SIGUSR1 restarts. We use this
* value to optimize routines that have to scan the entire scoreboard.
*/
static int max_daemons_limit = -1;
/*
* During config time, listeners is treated as a NULL-terminated list.
* child_main previously would star
没有合适的资源?快使用搜索试试~ 我知道了~
apache_1.3.37.tar.gz

温馨提示
apache web服务 作为最流行的Web服务器,Apache Server提供了较好的安全特性,使其能够应对可能的安全威胁和信息泄漏。 1、 采用选择性访问控制和强制性访问控制的安全策略 从Apache 或Web的角度来讲,选择性访问控制DAC(Discretionary Access Control)仍是基于用户名和密码的,强制性访问控制MAC(Mandatory Access Control)则是依据发出请求的客户端的IP地址或所在的域号来进行界定的。对于DAC方式,如输入错误,那么用户还有机会更正,从新输入正确的的密码;如果用户通过不了MAC关卡,那么用户将被禁止做进一步的操作,除非服务器作出安全策略调整,否则用户的任何努力都将无济于事。 2、Apache 的安全模块 Apache 的一个优势便是其灵活的模块结构,其设计思想也是围绕模块(Modules)概念而展开的。安全模块是Apache Server中的极其重要的组成部分。这些安全模块负责提供Apache Server的访问控制和认证、授权等一系列至关重要的安全服务。
资源推荐
资源详情
资源评论
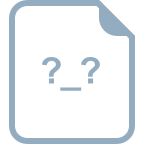
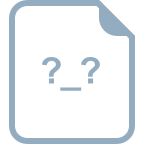
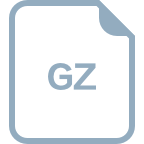
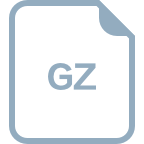
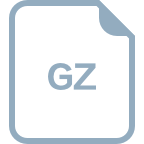
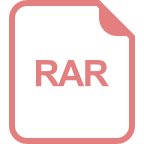
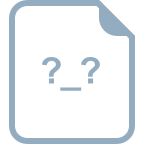
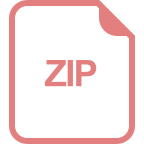
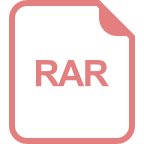
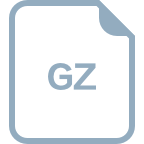
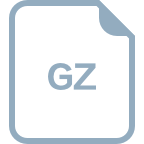
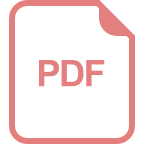
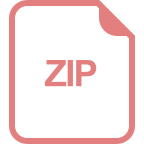
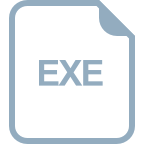
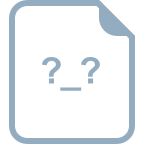
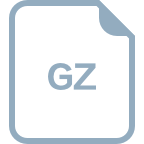
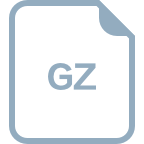
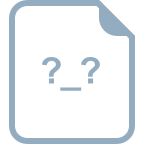
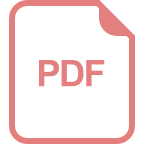
收起资源包目录

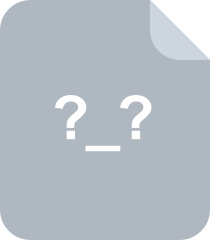
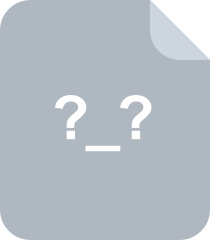
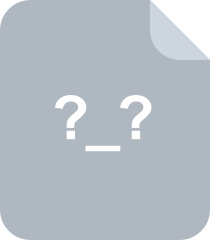
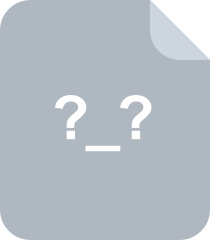
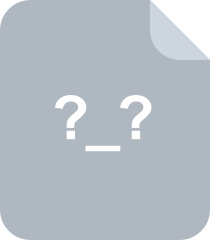
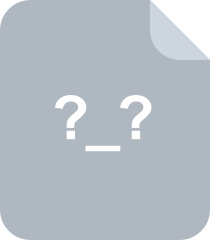
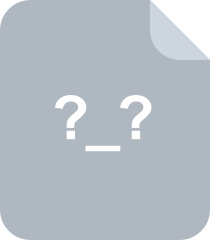
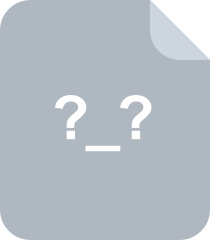
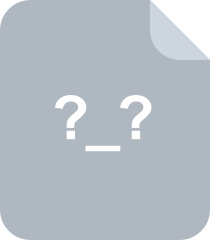
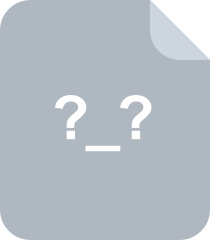
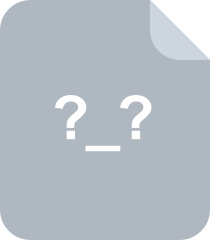
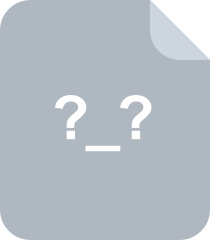
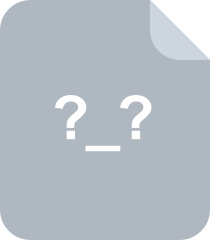
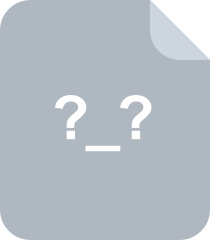
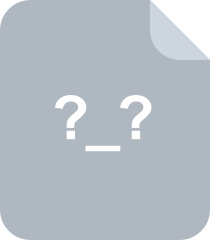
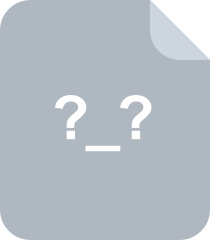
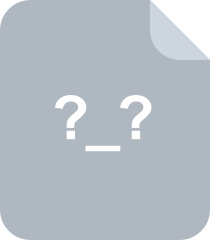
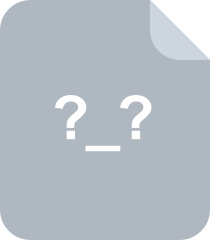
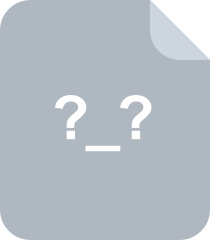
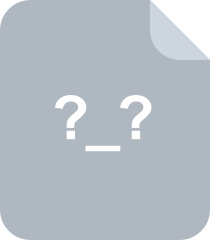
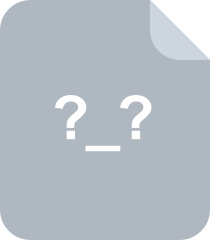
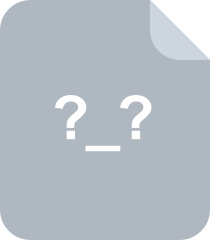
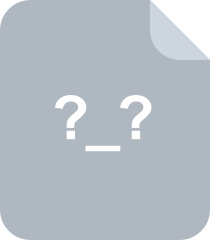
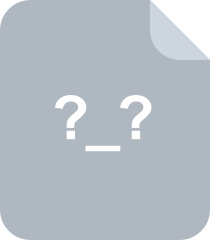
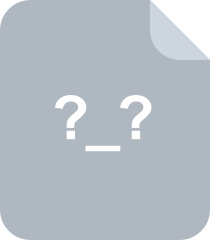
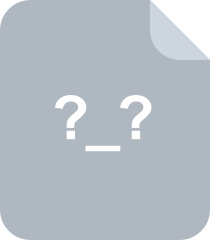
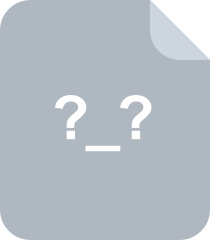
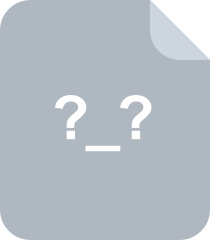
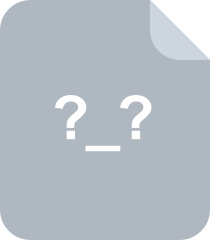
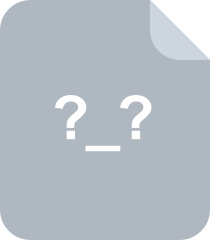
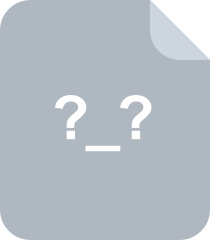
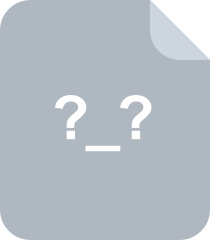
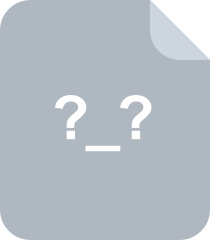
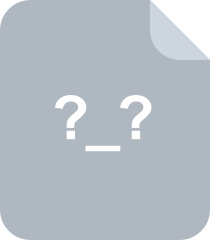
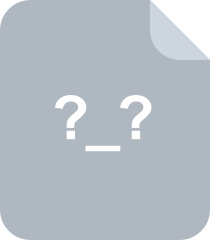
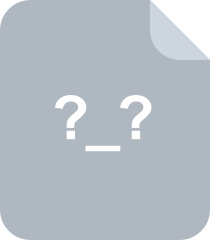
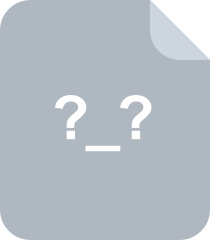
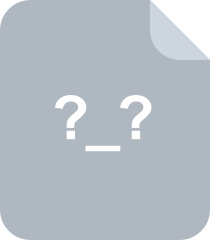
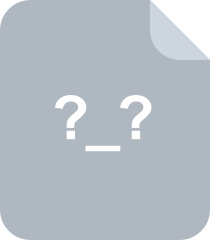
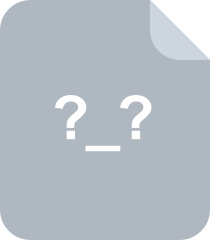
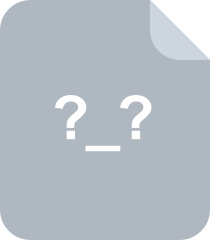
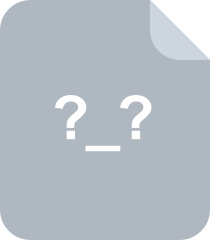
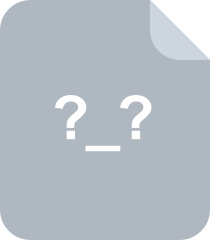
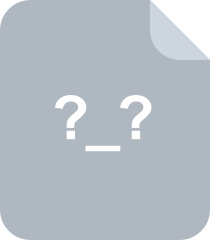
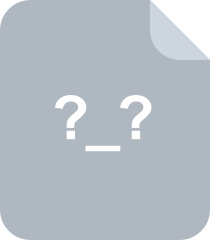
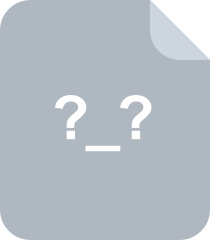
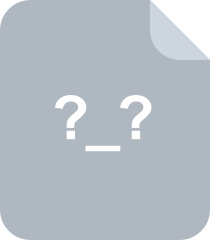
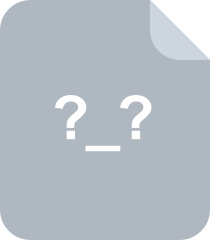
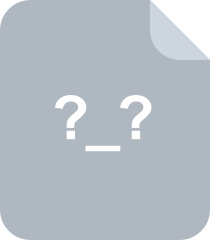
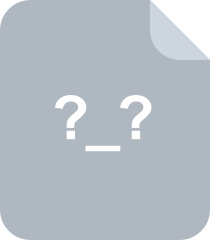
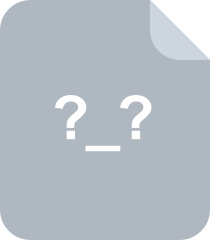
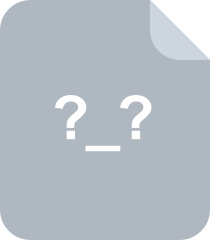
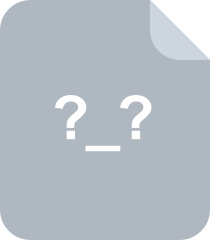
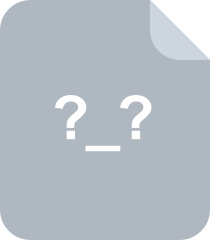
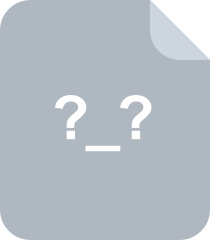
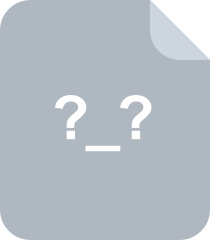
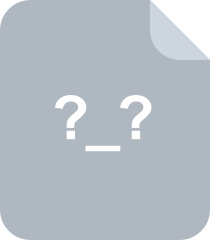
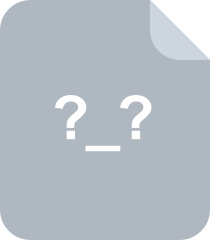
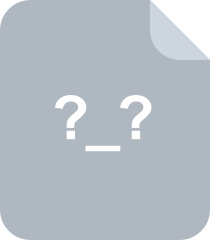
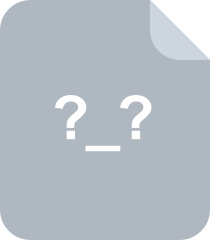
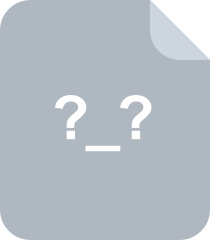
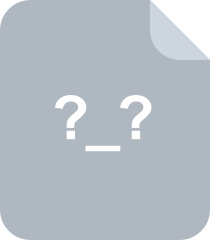
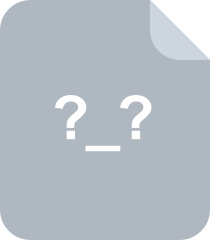
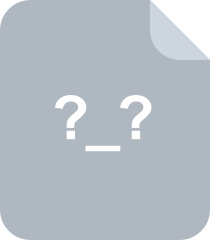
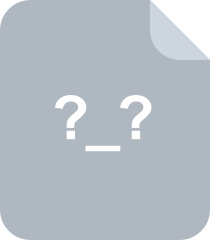
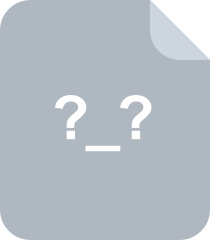
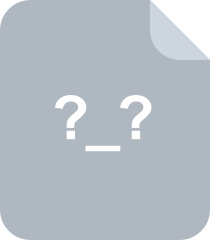
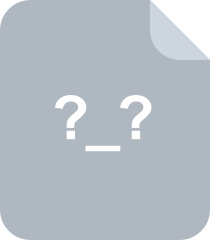
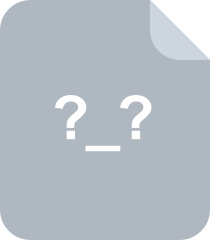
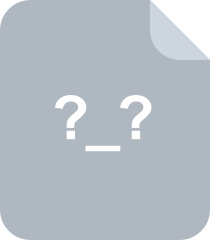
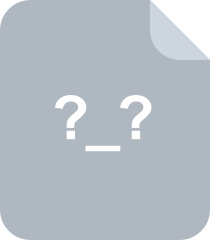
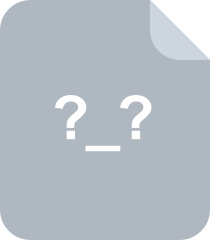
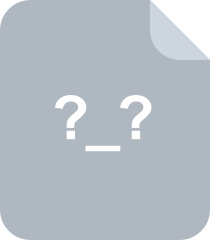
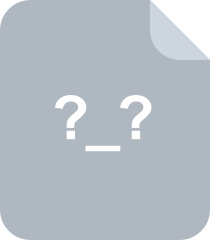
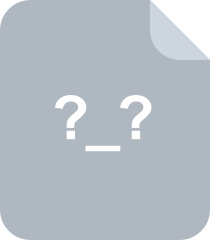
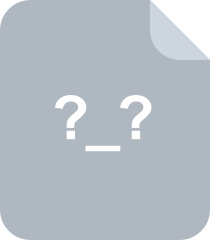
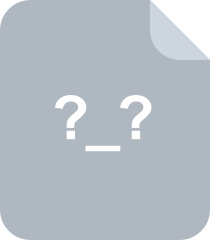
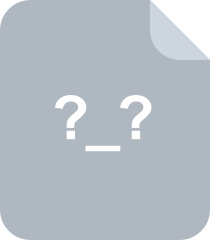
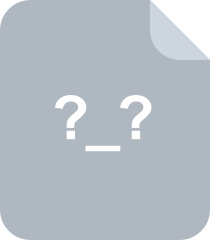
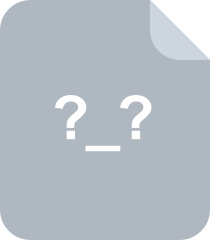
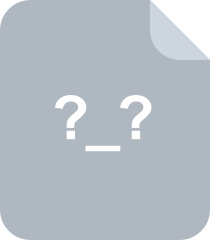
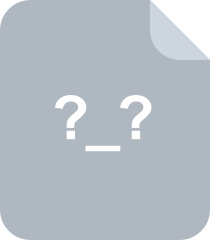
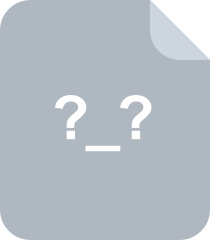
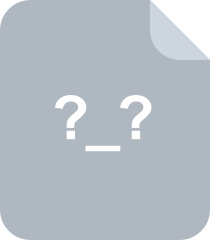
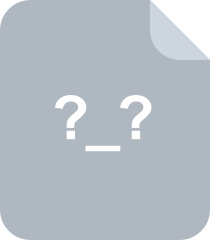
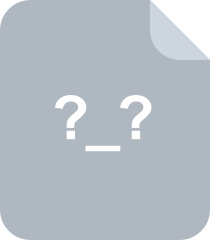
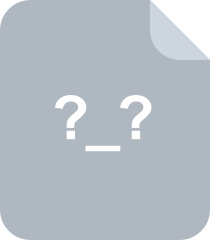
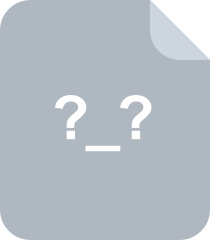
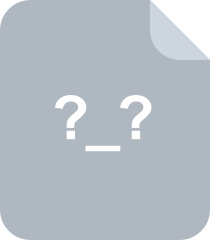
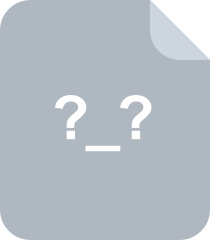
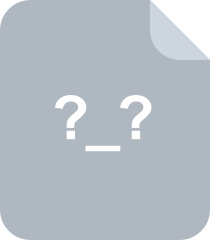
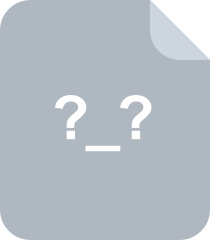
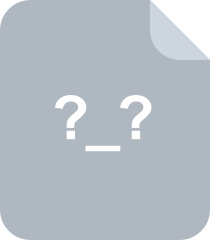
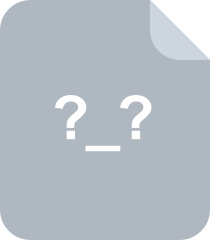
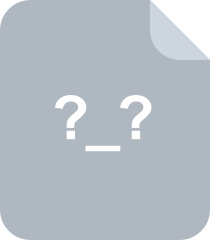
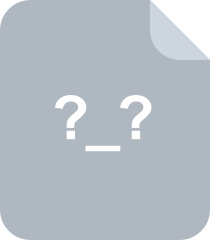
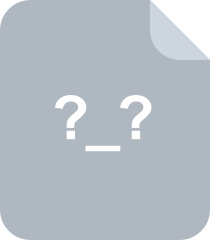
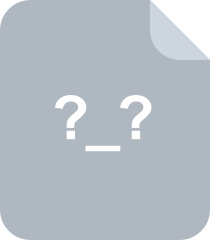
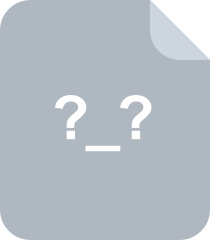
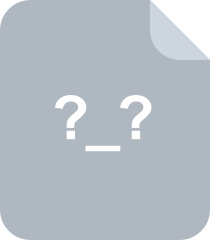
共 1076 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11
资源评论
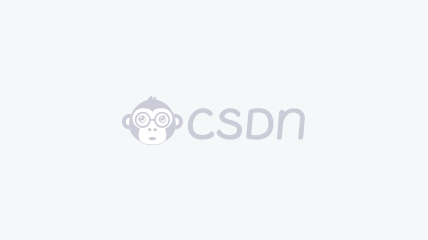
- zhaosun11112014-08-10正在学习Linux ,需要这些旧版本的东西.感谢!
- 天泰路小王爷2013-12-19解压出来安装了,没有问题,谢谢了
- pony042014-07-08安装成功,谢谢楼主
- 宁宁爱然然2013-11-04安装成功,感谢楼主

johnny6789
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

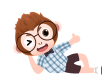
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


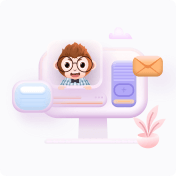
安全验证
文档复制为VIP权益,开通VIP直接复制
