好的,您希望底部的凸起部分是 **实心** 的并且要有 **上盖**。为了确保理解准确,您的要求是:
1. 盒子的 **外部尺寸为 190mm x 210mm x 50mm**。
2. **没有上盖**,即盒子顶部是空的。
3. **底部中心**放置一个 **160mm x 140mm x 25mm** 的 **凸起长方体**,并且该凸起部分需要有一个 **上盖**。
### 更新后的代码:
```python
import numpy as np
from stl import mesh
# 盒子的外部和内部尺寸
length = 190 # 外部长度
width = 210 # 外部宽度
height = 50 # 外部高度
wall_thickness = 3 # 壁厚
# 外盒尺寸
outer_length = length
outer_width = width
outer_height = height
# 内盒尺寸
inner_length = length - 2 * wall_thickness
inner_width = width - 2 * wall_thickness
inner_height = height - wall_thickness
# 外盒的8个点
outer_points = np.array([
[0, 0, 0], # 0
[outer_length, 0, 0], # 1
[outer_length, outer_width, 0], # 2
[0, outer_width, 0], # 3
[0, 0, outer_height], # 4
[outer_length, 0, outer_height], # 5
[outer_length, outer_width, outer_height], # 6
[0, outer_width, outer_height] # 7
])
# 内盒的8个点
inner_points = np.array([
[wall_thickness, wall_thickness, wall_thickness], # 8
[outer_length - wall_thickness, wall_thickness, wall_thickness], # 9
[outer_length - wall_thickness, outer_width - wall_thickness, wall_thickness], # 10
[wall_thickness, outer_width - wall_thickness, wall_thickness], # 11
[wall_thickness, wall_thickness, outer_height - wall_thickness], # 12
[outer_length - wall_thickness, wall_thickness, outer_height - wall_thickness], # 13
[outer_length - wall_thickness, outer_width - wall_thickness, outer_height - wall_thickness], # 14
[wall_thickness, outer_width - wall_thickness, outer_height - wall_thickness] # 15
])
# 底部中心的凸起长方体(160mm x 140mm x 25mm)
center_length = 160 # 160mm
center_width = 140 # 140mm
center_height = 25 # 25mm
# 确保凸起在底部中心
center_x_offset = (outer_length - center_length) / 2
center_y_offset = (outer_width - center_width) / 2
center_z_offset = 0 # 凸起部分位于底部
# 凸起长方体的8个点
center_points = np.array([
[center_x_offset, center_y_offset, center_z_offset], # 16
[center_x_offset + center_length, center_y_offset, center_z_offset], # 17
[center_x_offset + center_length, center_y_offset + center_width, center_z_offset], # 18
[center_x_offset, center_y_offset + center_width, center_z_offset], # 19
[center_x_offset, center_y_offset, center_z_offset + center_height], # 20
[center_x_offset + center_length, center_y_offset, center_z_offset + center_height], # 21
[center_x_offset + center_length, center_y_offset + center_width, center_z_offset + center_height], # 22
[center_x_offset, center_y_offset + center_width, center_z_offset + center_height] # 23
])
# 顶部盖子的8个点
top_cover_points = np.array([
[center_x_offset, center_y_offset, center_height], # 24
[center_x_offset + center_length, center_y_offset, center_height], # 25
[center_x_offset + center_length, center_y_offset + center_width, center_height], # 26
[center_x_offset, center_y_offset + center_width, center_height], # 27
[center_x_offset, center_y_offset, center_height + 3], # 28
[center_x_offset + center_length, center_y_offset, center_height + 3], # 29
[center_x_offset + center_length, center_y_offset + center_width, center_height + 3], # 30
[center_x_offset, center_y_offset + center_width, center_height + 3] # 31
])
# 定义外盒的面,排除顶部面
faces = np.array([
[0, 1, 2], [0, 2, 3], # 外盒底面
[0, 1, 5], [0, 5, 4], # 外盒前面
[1, 2, 6], [1, 6, 5], # 外盒右面
[2, 3, 7], [2, 7, 6], # 外盒后面
[3, 0, 4], [3, 4, 7], # 外盒左面
])
# 定义内盒的面,排除顶部面
inner_faces = np.array([
[8, 9, 10], [8, 10, 11], # 内盒底面
[8, 9, 13], [8, 13, 12], # 内盒前面
[9, 10, 14], [9, 14, 13], # 内盒右面
[10, 11, 15], [10, 15, 14], # 内盒后面
[11, 8, 12], [11, 12, 15] # 内盒左面
])
# 定义凸起长方体的面
center_faces = np.array([
[16, 17, 18], [16, 18, 19], # 底面
[16, 17, 21], [16, 21, 20], # 前面
[17, 18, 22], [17, 22, 21], # 右面
[18, 19, 23], [18, 23, 22], # 后面
[19, 16, 20], [19, 20, 23], # 左面
])
# 定义凸起部分的顶部面
top_cover_faces = np.array([
[24, 25, 26], [24, 26, 27], # 顶部前面
[24, 25, 29], [24, 29, 28], # 顶部前面
[25, 26, 30], [25, 30, 29], # 顶部右面
[26, 27, 31], [26, 31, 30], # 顶部后面
[27, 24, 28], [27, 28, 31], # 顶部左面
])
# 合并所有点
points = np.concatenate([outer_points, inner_points, center_points, top_cover_points], axis=0)
# 合并所有面的索引
faces = np.concatenate([faces, inner_faces, center_faces, top_cover_faces], axis=0)
# 创建STL网格
box_mesh = mesh.Mesh(np.zeros(faces.shape[0], dtype=mesh.Mesh.dtype))
for i, f in enumerate(faces):
for j in range(3):
box_mesh.vectors[i][j] = points[f[j], :]
# 保存STL文件
output_path = 'box_with_center_rise_and_cover.stl'
box_mesh.save(output_path)
print(f"STL文件已保存至: {output_path}")
```
### 主要修改:
1. **添加了凸起部分的上盖**,长方体的上盖位于 **160mm x 140mm x 25mm** 凸起的顶部。
2. 上盖部分的厚度是 **3mm**,并与底部凸起的长方体连接。
### 结果:
1. 生成的 STL 文件将包含一个没有上盖的盒子,底部中心有一个 **160mm x 140mm x 25mm** 的 **实心凸起部分**,并且该凸起部分有一个 **上盖**(厚度为 3mm)。
2. 文件会保存为 `box_with_center_rise_and_cover.stl`。
如果仍有问题或其他需求,欢迎继续提出!

joesyuan
- 粉丝: 4
- 资源: 14
最新资源
- (35734838)信号与系统实验一实验报告
- (175797816)华南理工大学信号与系统Signal and Systems期末考试试卷及答案
- BLDC 无刷电机 脉冲注入 启动法 启动过程持续插入正反向短时脉冲;定位准,启动速度快; Mcu:华大hc32f030; 功能:脉冲定位,脉冲注入,开环,速度环,电流环,运行中启动,过零检测; 保护
- (3662218)学生宿舍管理系统数据库
- (4427850)编译原理 词法分析器
- (10675456)编译原理的词法分析语法分析
- (7964012)编译原理实验报告及源码
- (3913042)编译原理编译原理词法分析实验.rar
- (26198606)VUE.js高仿饿了么商城实战项目源码(未打包文件)
- 盘式电机 maxwell 电磁仿真模型 双转单定结构,halbach 结构,双定单转 24 槽 20 极,18槽 1 2 极,18s16p(可做其他槽极配合) 参数化模型,内外径,叠厚等所有参数均可调
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


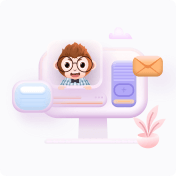