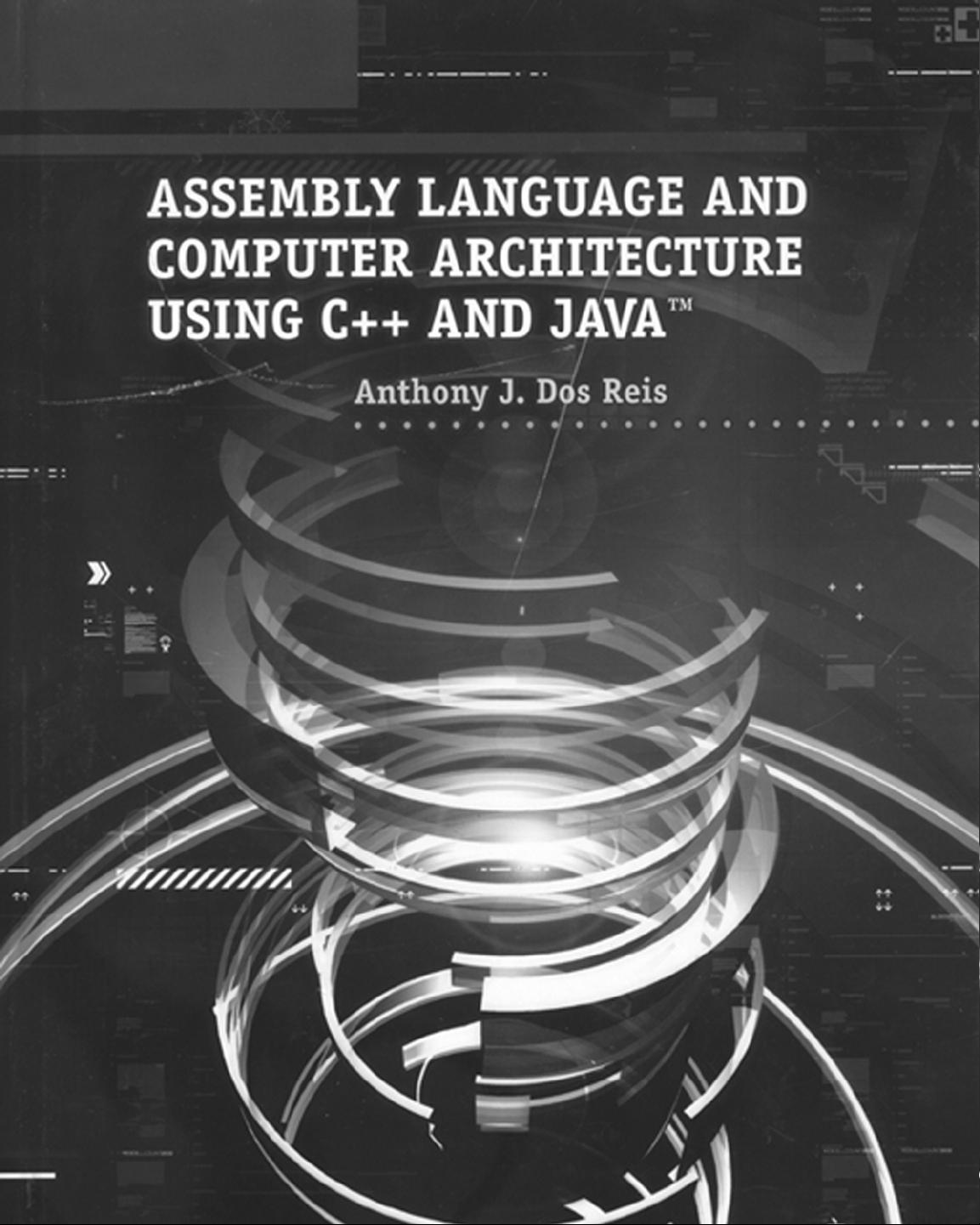
Licensed to:
iChapters User
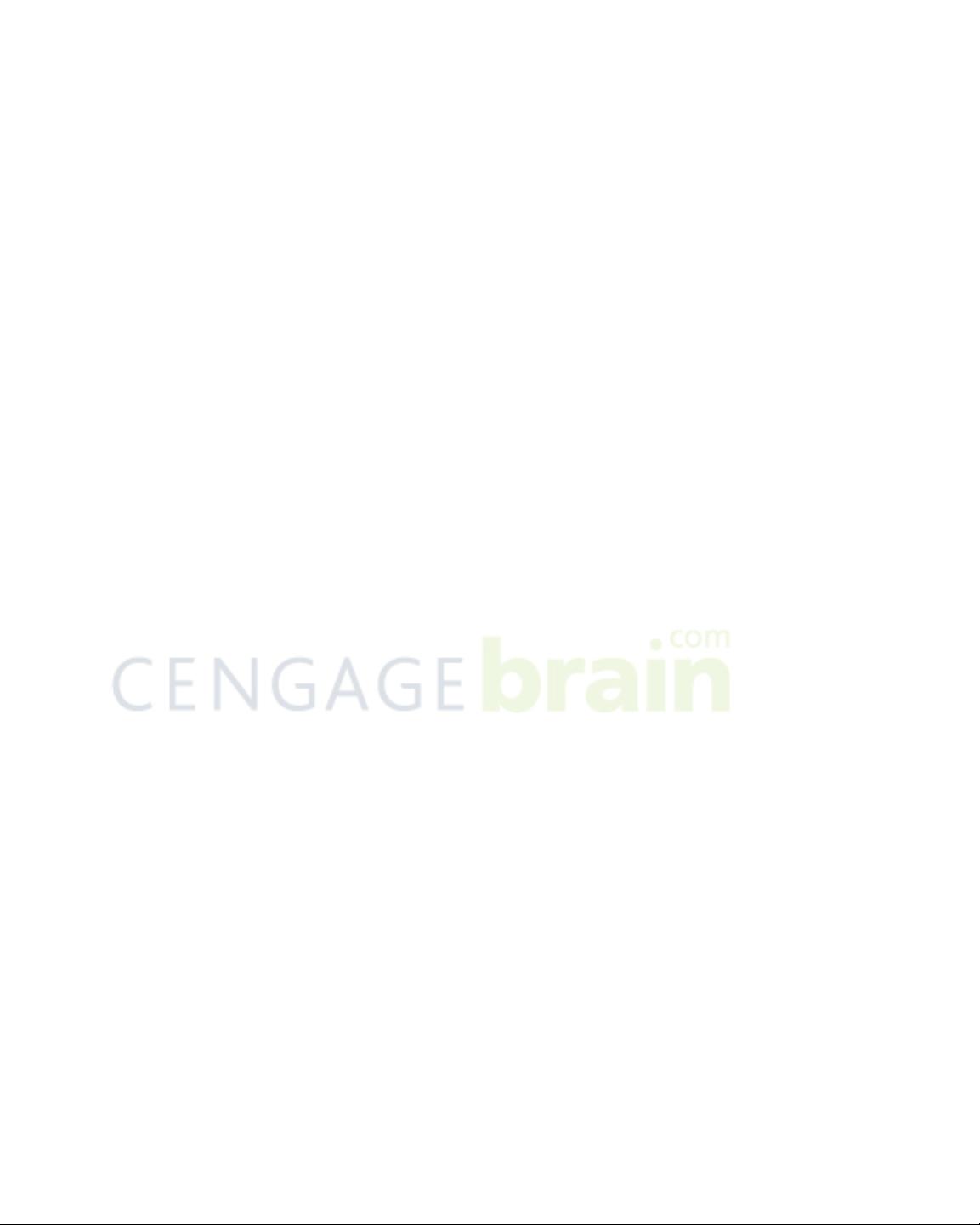
Managing Editor:
Jennifer Muroff
Editorial Assistant:
Amanda Piantedosi
Manufacturing Coordinator:
Trevor Kallop
Cover Design:
Roy Neuhaus
Compositor:
WestWords, Inc.
COPYRIGHT © 2004 Course
Technology, a division of Thom-
son Learning, Inc. Thomson
Learning™ is a trademark used
herein under license.
Printed in the United States
of America
123456789PX0706050403
For more information, contact
Course Technology, 25 Thomson
Place, Boston Massachusetts,
02210.
Or find us on the World Wide
Web at : www.course.com
Disclaimer
Course Technology reserves the
right to revise this publication
and make changes from time to
time in its content without notice.
ISBN 0-534-40527-4
ALL RIGHTS RESERVED.
No part of this work covered by
the copyright hereon may be re-
produced or used in any form or
by any means—graphic, electron-
ic, or mechanical,
including photocopying, record-
ing, taping, Web distribution, or
information storage and retrieval
systems—without the written
permission of the publisher.
For permission to use material
fromthis text or product, contact
us by
Tel (800) 730-2214
Fax (800) 730-2215
www.thomsonrights.com
Associate Product Manager:
Mirella Misiaszek
Designer:
Roy Neuhaus
Assembly Language and Computer Architecture Using C++ and Java
by Anthony J. Dos Reis
Copyright 2009 Cengage Learning, Inc. All Rights Reserved. May not be copied, scanned, or duplicated, in whole or in part.
Licensed to:
iChapters User
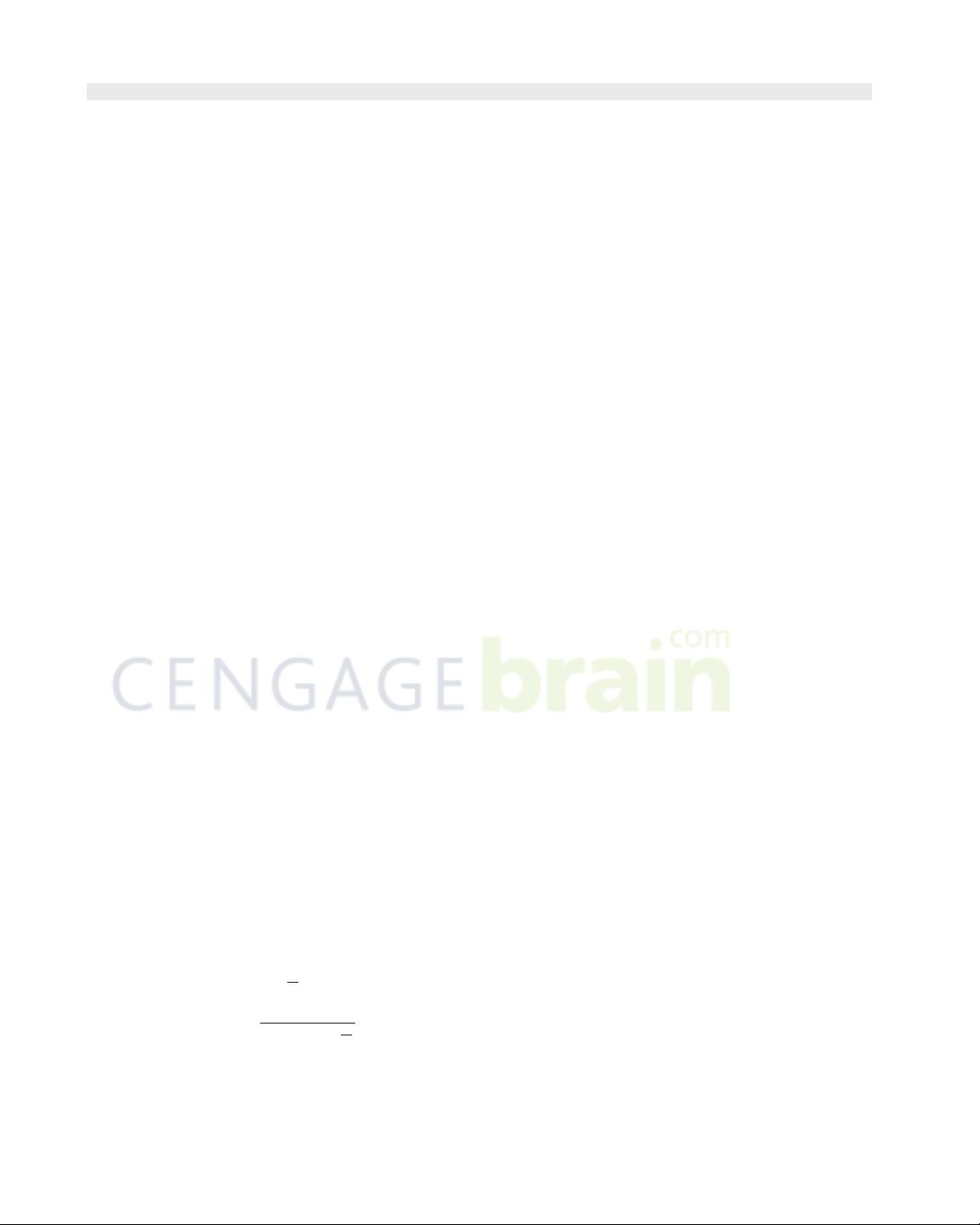
Chapter One
NUMBER SYSTEMS
1
1.1 INTRODUCTION
• • • • • • • • • • • • • • • • • • • • • • • • • • • •
In a well-known joke, a graduate of a speed-reading course proudly announces
that he was able to read Tolstoy’s War and Peace in 10 minutes. When asked what
the book was about, the graduate replied, “I think it was about Russia.” Students
completing computer courses—particularly courses in assembly language and
computer architecture—often have similarly inadequate answers about their
courses. Computers, after all, are complex systems that span a multitude of tech-
nologies. To thoroughly understand even one computer system can take years of
diligent study. There is, however, one way to quickly acquire an understanding of
the fundamental concepts of computers: study a computer that is well suited for
learning these concepts. We will do precisely that in this book. We will study a
computer that is simple enough that it can be mastered quickly, but realistic
enough that it accurately models the essential features of modern computers.
In this chapter, we start with the study of number systems. We humans use
the decimal number system. Computers, however, use a different number system,
called binary. Decimal numbers use 10 symbols (0, 1, 9) called digits. Binary
numbers use only two symbols (0 and 1), called bits. A third number system, hexa-
decimal (or hex, for short), can be used as a convenient shorthand representation of
the binary numbers inside a computer. For this reason, hexadecimal as well as bi-
nary numbers are important in the computer field. Hexadecimal numbers use 16
symbols (0, 1, 9, A, B, C, D, E, F) called hex digits or, when the context im-
plies hex, simply digits.
1.2 POSITIONAL NUMBER SYSTEMS
• • • • • • • • • • • • • • • • • • • • • • • • • • • •
Decimal, binary, and hexadecimal are all positional number systems. In a positional
number system, each position is associated with a different weight. For example,
in the decimal number 25.4, the weights of the three positions, from right to left,
are 1, and 10:
weights
The weight of each position determines how much the digit in that position con-
tributes to the value of the number. For example, because the “2” in 25.4 occupies
the 10’s position (i.e., the position with weight 10), it contributes to2 3 10 5 20
1 0
1
1
10
2
5
?
4
1
10
,
2, c,
2, c,
Copyright 2009 Cengage Learning, Inc. All Rights Reserved. May not be copied, scanned, or duplicated, in whole or in part.
Licensed to:
iChapters User
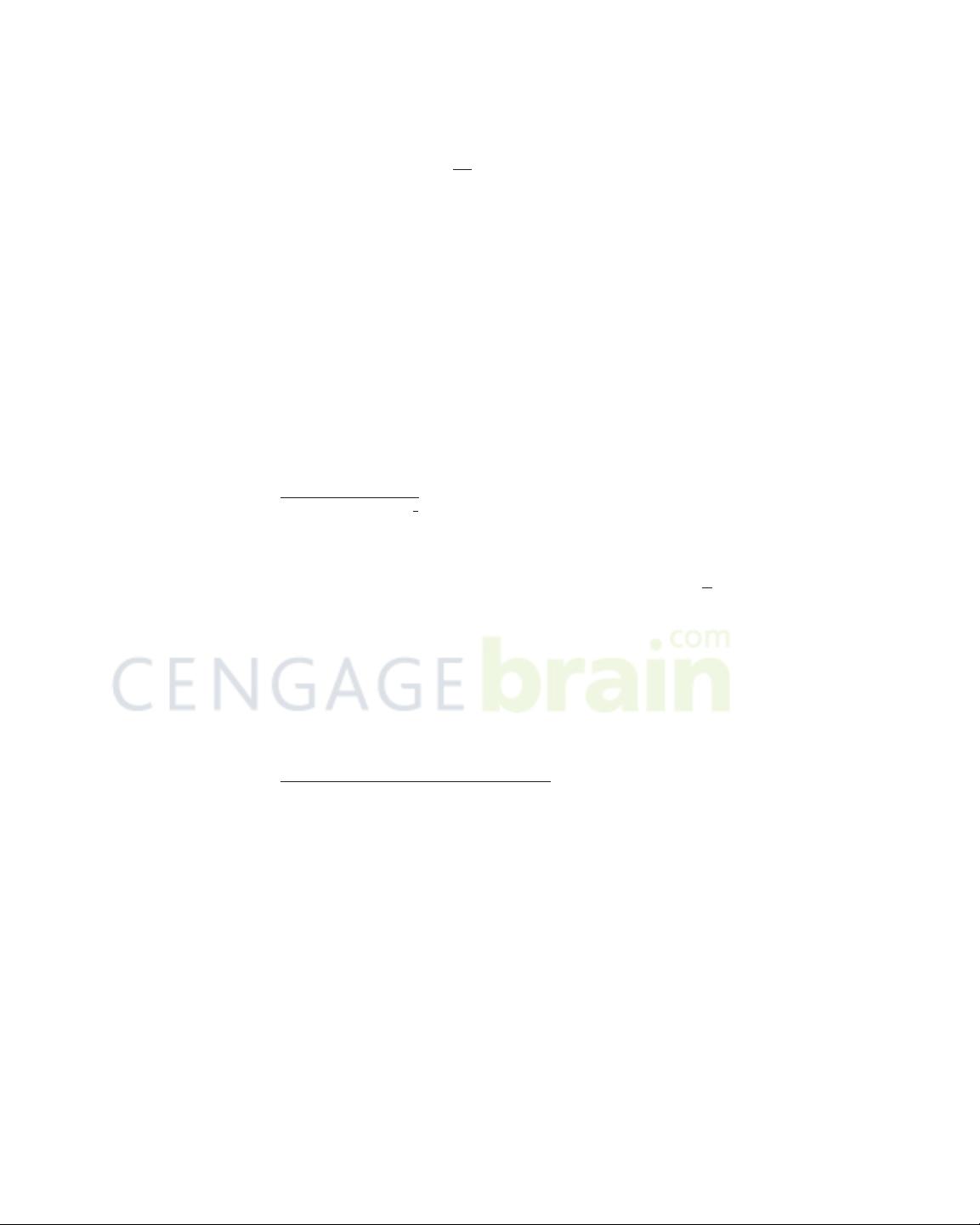
2 Chapter 1 Number Systems
Decimal Binary Hexadecimal
0 0000 0
1 0001 1
2 0010 2
3 0011 3
4 0100 4
5 0101 5
6 0110 6
7 0111 7
8 1000 8
9 1001 9
10 1010 A
11 1011 B
12 1100 C
13 1101 D
14 1110 E
15 1111 F
FIGURE 1.1
the value of the number. The value of a decimal number is the sum of each digit
times its weight. Thus, the value of 25.4 is
In a decimal number, weights increase by a factor of 10 from right to left from
one position to the next. The weight of the position to the immediate left of the
decimal point is always 1. Because decimal numbers have 10 symbols (0 to 9), and
have weights that increase by a factor of 10, we call decimal a base 10 or radix 10
positional number system.
We call the period in a number that divides the whole part from the fractional
part the radix point. When we are dealing with decimal numbers, we usually call
the radix point a decimal point. In binary and hexadecimal numbers, we can simi-
larly call the radix point the binary point and the hexadecimal point, respectively.
Binary is the base 2 positional number system. Thus, in the binary number
1011.1, the weights of each position increase by a factor of 2 from right to left. As in
decimal, the position to the immediate left of the radix point has weight 1. Thus, in
the binary number 1011.1, the weights are
weights (in decimal)
and its value in decimal is
Because bits are either 1 or 0, the value of a binary number is simply the sum of the
weights whose corresponding bits are 1.
Hexadecimal is the base 16 positional number system. For its 16 symbols, we
use 0 to 9 and A to F. The symbols 0 to 9 have the same values as they do in deci-
mal. The symbols A, B, C, D, E, and F have values equal to decimal 10, 11, 12, 13,
14, and 15, respectively (see Figure 1.1).
1 3 8 1 0 3 4 1 1 3 2 1 1 3 1 1 1 3
1
2
5 11.5
8
4
2
1
1
2
1
0
1
1
?
1
2 3 10 1 5 3 1 1 4 3
1
10
Copyright 2009 Cengage Learning, Inc. All Rights Reserved. May not be copied, scanned, or duplicated, in whole or in part.
Licensed to:
iChapters User
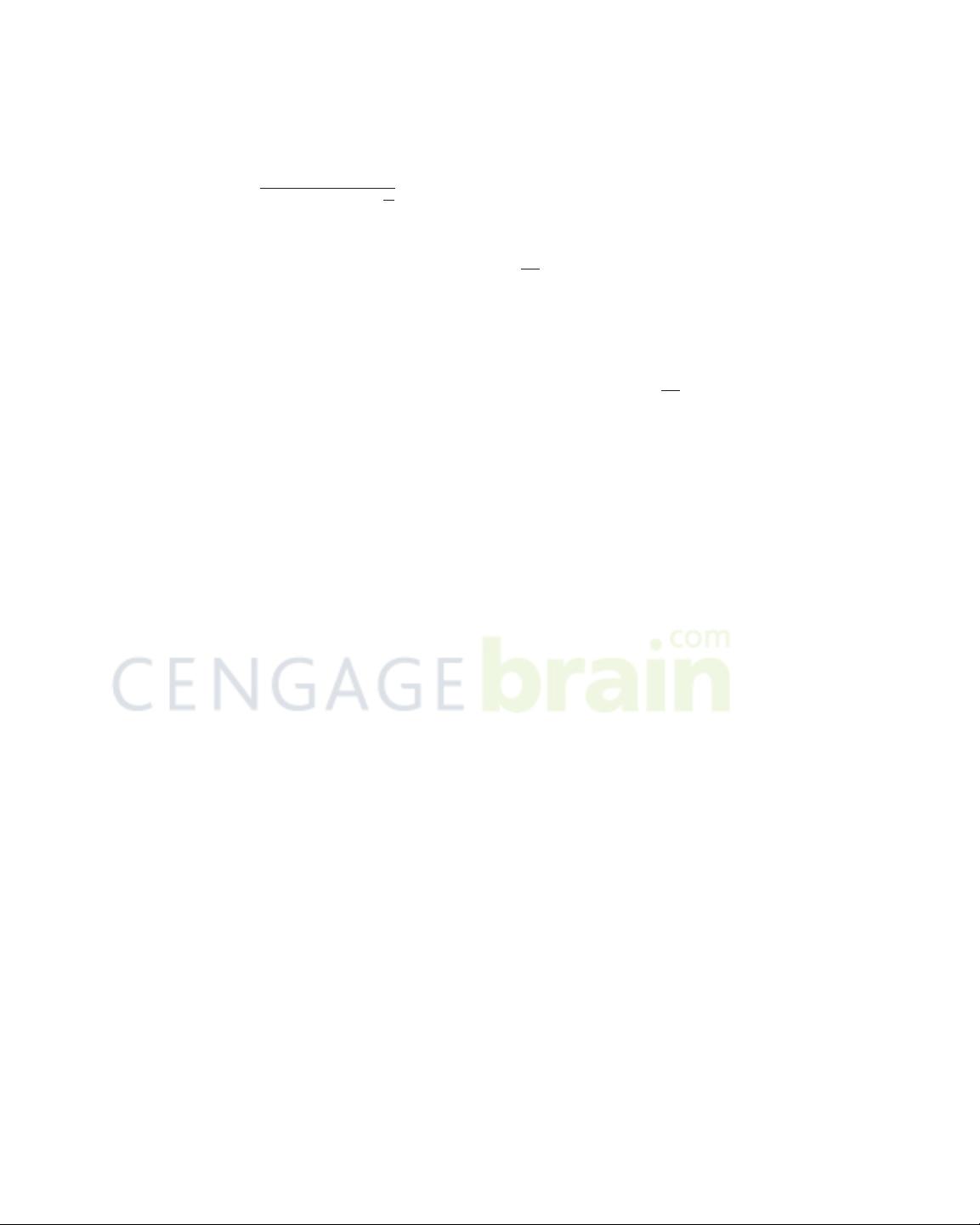
1.2 Positional Number Systems 3
The weights in a hexadecimal number increase by a factor of 16. As in decimal
and binary, the weight of the position to the immediate left of the radix point is 1.
For example, in the hexadecimal number 1CB.8, the weights are
weights (in decimal)
and its value is
Substituting the decimal equivalents for the symbols B (11 decimal) and C (12 dec-
imal), we get an all-decimal expression from which we can compute its decimal
value:
Because the leftmost position in a positional number has the most weight, we
call the symbol in that position the most significant. The symbol in the rightmost
position is the least significant. For example, in the binary number 0101, the 0 in the
leftmost position is the most significant bit (MSB), and the 1 in the rightmost posi-
tion is the least significant bit (LSB). In decimal and hexadecimal numbers, we use
the terms most significant digit (MSD) and least significant digit (LSD).
The complement of a symbol s in the base b positional number system is the
symbol whose value is For example, the complement of 3 in decimal
is In binary, the complement of 1 is the com-
plement of 0 is A symbol in base b plus its complement always
equals the value of the symbol in base b with the largest value. For example, in
decimal, 3 plus its complement, 6, equals 9; in hexadecimal, 3 plus its comple-
ment, C, equals F.
To complement a symbol means to change it to its complement. For example,
if we complement 1 binary, 1 decimal, and 1 hexadecimal, we get 0, 8, and E, re-
spectively. In binary, to flip a bit means to complement it. Thus, if we flip 1, we get
0; if we flip 0, we get 1.
Let’s determine how many distinct numbers can be represented in base b using
three positions. Because there are b possible symbols for each of the three positions,
combinatorial mathematics tells us that there are possible numbers.
For example, in a decimal number, there are 10 possible symbols for each position.
Thus, with three digits, there are possible decimal
numbers. Similarly, with three bits, there are possible binary
numbers. Generalizing, we can state that with n positions, there are possible
base b numbers. Figure 1.2 shows a table containing the number of distinct binary
numbers for various sizes that commonly appear in computers. You should famil-
iarize yourself with this table—it will prove useful as you study the rest of the
book.
A byte is a sequence of 8 bits. One byte can represent numbers. Thus,
if we use all 8 bits to hold consecutive positive binary numbers starting from
zero, numbers can range from 0 to Similarly, with 16 bits, numbers
can range from 0 to With n bits, numbers can range from 0 to
Computer circuits are designed to operate on chunks of data of a specific
length. These chunks of data are called words. A word typically contains some
2
n
2 1.
2
16
2 1 5 65,535.
2
8
2 1 5 255.
2
8
5 256
b
n
2 3 2 3 2 5 2
3
5 8
10 3 10 3 10 5 10
3
5 1000
b 3 b 3 b 5 b
3
12 2 122 0 5 1.
12 2 122 1 5 0;110 2 122 3 5 6.
1b 2 122 s.
1 3 256 1 12 3 16 1 11 3 1 1 8 3
1
16
5 459.5
1 3 256 1 C 3 16 1 B 3 1 1 8 3
1
16
256
1 6
1
1
16
1
C
B
?
8
Copyright 2009 Cengage Learning, Inc. All Rights Reserved. May not be copied, scanned, or duplicated, in whole or in part.
Licensed to:
iChapters User