package lib.base;
import java.io.File;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.HttpURLConnection;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import net.sf.json.JSONObject;
import utils.HttpDeleteUtil;
import utils.RequestEncoder;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.methods.HttpPut;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.protocol.HTTP;
import org.apache.http.util.EntityUtils;
import config.AppConfig;
import lib.Mail;
import lib.Message;
/**
* 这个类实现了ISender,包含了在发送之前处理发生的事件的常用方法
* 创建了一个签名并构建请求数据
* @author submail
*
*/
public class Sender implements ISender {
protected AppConfig config = null;
private static final String API_TIMESTAMP = "http://api.submail.cn/service/timestamp.json";
public static final String APPID = "appid";
public static final String TIMESTAMP = "timestamp";
public static final String SIGN_TYPE = "sign_type";
public static final String SIGNATURE = "signature";
public static final String APPKEY = "appkey";
private CloseableHttpClient closeableHttpClient = null;
public Sender() {
closeableHttpClient = HttpClientBuilder.create().build();
}
@Override
public String send(Map<String, Object> data) {
// TODO Auto-generated method stub
return null;
}
@Override
public String xsend(Map<String, Object> data) {
// TODO Auto-generated method stub
return null;
}
@Override
public String subscribe(Map<String, Object> data) {
// TODO Auto-generated method stub
return null;
}
@Override
public String unsubscribe(Map<String, Object> data) {
// TODO Auto-generated method stub
return null;
}
@Override
public String multixsend(Map<String, Object> data) {
// TODO Auto-generated method stub
return null;
}
@Override
public String verify(Map<String, Object> data) {
// TODO Auto-generated method stub
return null;
}
@Override
public String log(Map<String, Object> data) {
// TODO Auto-generated method stub
return null;
}
@Override
public String get(Map<String, Object> data) {
// TODO Auto-generated method stub
return null;
}
@Override
public String post(Map<String, Object> data) {
// TODO Auto-generated method stub
return null;
}
@Override
public String put(Map<String, Object> data) {
// TODO Auto-generated method stub
return null;
}
@Override
public String delete(Map<String, Object> data) {
// TODO Auto-generated method stub
return null;
}
@Override
public String selMobiledata(Map<String, Object> data) {
// TODO Auto-generated method stub
return null;
}
@Override
public String toService(Map<String, Object> data) {
// TODO Auto-generated method stub
return null;
}
@Override
public String charge(Map<String, Object> data) {
// TODO Auto-generated method stub
return null;
}
/**
* 请求时间戳
* @return timestamp
* */
protected String getTimestamp() {
HttpGet httpget = new HttpGet(API_TIMESTAMP);
HttpResponse response;
try {
response = closeableHttpClient.execute(httpget);
HttpEntity httpEntity = response.getEntity();
String jsonStr = EntityUtils.toString(httpEntity, "UTF-8");
if (jsonStr != null) {
JSONObject json = JSONObject.fromObject(jsonStr);
return json.getString("timestamp");
}
closeableHttpClient.close();
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
protected String createSignature(String data) {
if (AppConfig.TYPE_NORMAL.equals(config.getSignType())) {
return config.getAppKey();
} else {
return buildSignature(data);
}
}
/**
* 当 {@link AppConfig#setSignType(String)} 不正常时,创建
* 一个签名类型
*
* @param data
* 请求数据
* @return signature
* */
private String buildSignature(String data) {
String app = config.getAppId();
String appKey = config.getAppKey();
// order is confirmed
String jointData = app + appKey + data + app + appKey;
if (AppConfig.TYPE_MD5.equals(config.getSignType())) {
return RequestEncoder.encode(RequestEncoder.MD5, jointData);
} else if (AppConfig.TYPE_SHA1.equals(config.getSignType())) {
return RequestEncoder.encode(RequestEncoder.SHA1, jointData);
}
return null;
}
/**
* 请求数据 post提交
*
* @param url
* @param data
* @return boolean
* */
protected String request(String url, Map<String, Object> data) {
HttpPost httppost = new HttpPost(url);
httppost.addHeader("charset", "UTF-8");
httppost.setEntity(build(data));
try {
HttpResponse response = closeableHttpClient.execute(httppost);
HttpEntity httpEntity = response.getEntity();
if (httpEntity != null) {
String jsonStr = EntityUtils.toString(httpEntity, "UTF-8");
System.out.println(jsonStr);
return jsonStr;
}
closeableHttpClient.close();
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
/**
* 短信模板:get请求方法
* @param url
* @param data
* @return
*/
protected String getMethodRequest(String url, Map<String, Object> data) {
data.put(APPID, config.getAppId());
data.put(TIMESTAMP, this.getTimestamp());
data.put(SIGNATURE, config.getAppKey());
String urlGet = url + "?";
for(Map.Entry<String, Object> entry: data.entrySet()){
String key = entry.getKey();
Object value = entry.getValue();
if(value instanceof String){
urlGet += key + "=" + String.valueOf(value) + "&";
}
}
urlGet = urlGet.substring(0, urlGet.length()-1);
HttpGet httpGet = new HttpGet(urlGet);
try{
CloseableHttpClient closeableHttpClient = HttpClientBuilder.create().build();
HttpResponse response = closeableHttpClient.execute(httpGet);
HttpEntity httpEntity = response.getEntity();
if(httpEntity != null){
String jsonStr = EntityUtils.toString(httpEntity, "UTF-8");
System.out.println(jsonStr);
return jsonStr;
}
closeableHttpClient.close();
}catch(ClientProtocolException e){
e.printStackTrace();
}catch(IOException e){
e.printStackTrace();
}
return null;
}
/**
* 短信模板:put方法
* @param url
* @param data
* @return
*/
protected String putMethodRequest(String url, Map<String, Object> data) {
HttpPut httpput = new HttpPut(url);
httpput.addHeader("charset", "UTF-8");
data.put(APPID, config.getAppId());
data.put(TIMESTAMP, this.getTimestamp());
data.put(SIGNATURE, config.getAppKey());
List<NameValuePair> params=new ArrayList<NameValuePair>();
for(Map.Entry<String, Object> entry: data.entrySet()){
String key = entry.getKey();
String value = (String) entry.getValue();
params.add(new BasicNameValuePair(key,value));
}
try {
httpput.setEntity(new UrlEncodedFormEntity(params,HTTP.UTF_8));
HttpResponse response = closeableHttpClient.execute(httpput);
HttpEntity httpEntity = response.getEntity();
if (httpEntity !=
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
Submail 接入 ------------- submai_demo 是我们提供的一个测试代码示列,每个类对应的一个单独的接口,带入参数执行main方法即可请求我们平台接口。 submail_sdk 使我们平台提供的sdk代码文档,您可以在项目集成我们的sdk,在配置文件里配置您的appid,appkey,signtype,即可调用,接口调用方法请参考sdk里的demo包。 SDK-MAVEN 是通过maven实现管理项目,相比sdk多了一个pom文件,方便您管理jar包。 Subhook1 是一个我们平台subhook获取推送状态的示列代码。 Submail-sdk.jar 是我们平台提供的对接jar包。里面集成我们平台的sdk以及其他三方jar包,只需导入我们提供的jar包,即可调用。调用的示列代码在jar包里的com.submail.demo包里。
资源推荐
资源详情
资源评论
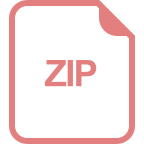
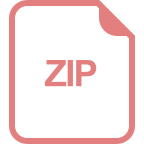
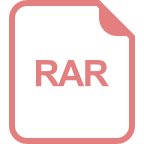
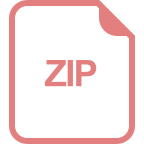
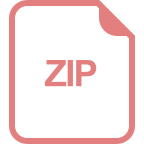
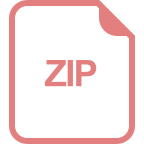
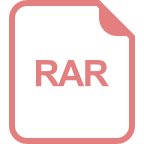
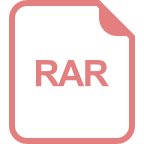
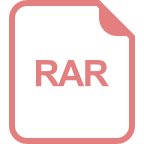
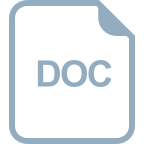
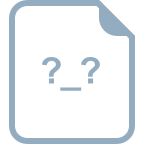
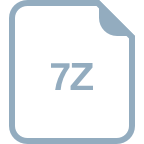
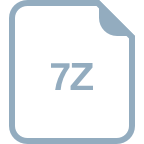
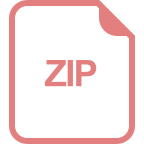
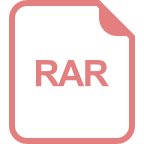
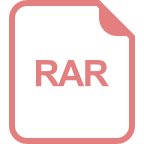
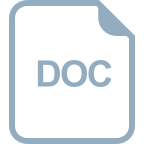
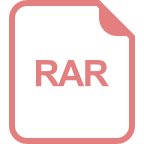
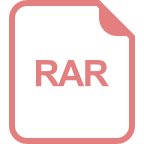
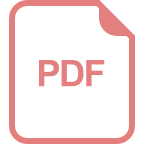
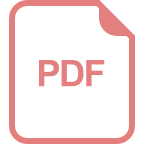
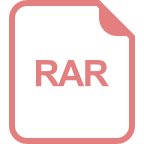
收起资源包目录

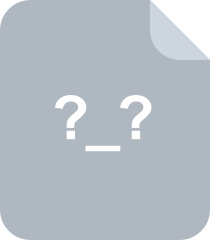
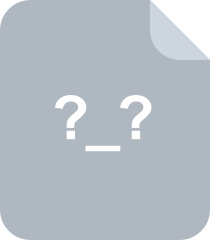
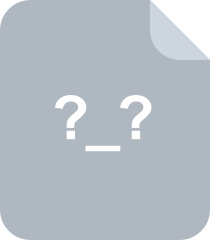
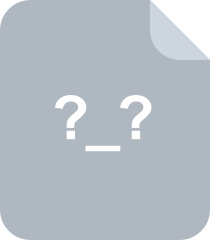
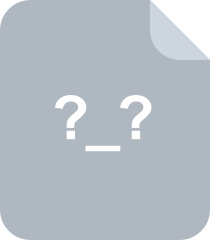
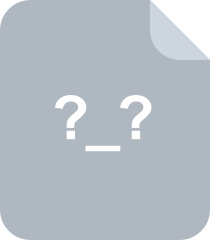
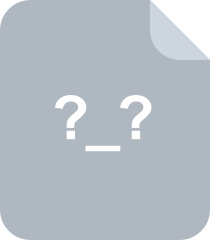
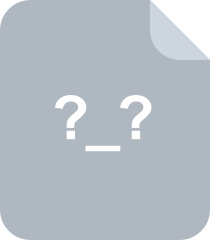
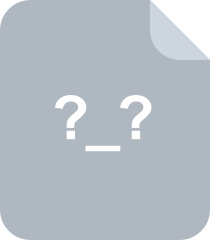
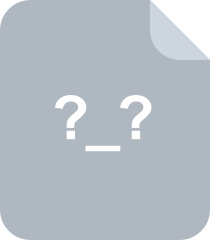
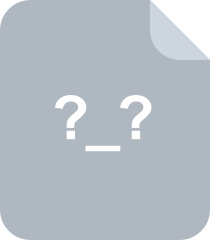
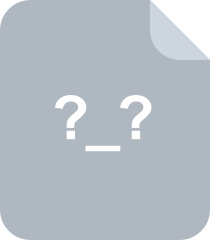
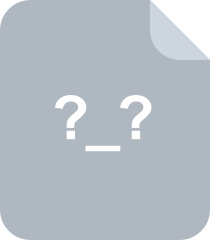
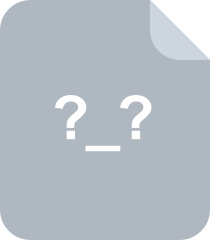
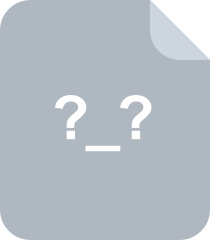
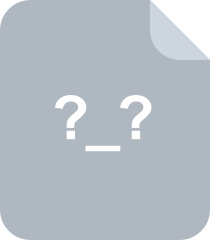
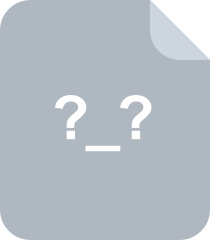
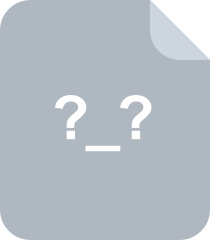
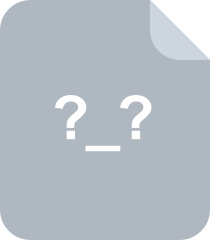
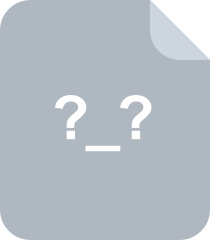
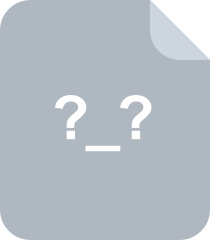
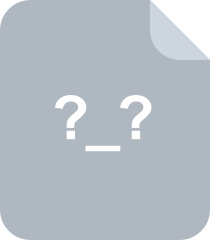
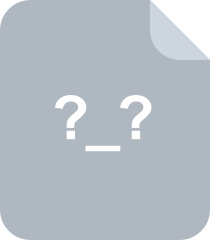
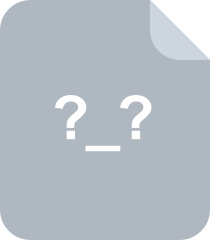
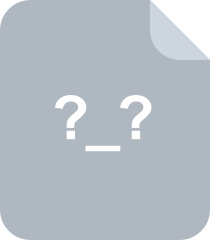
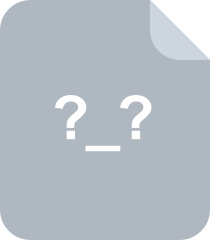
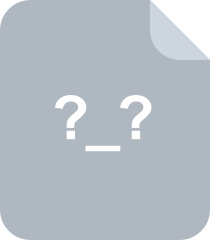
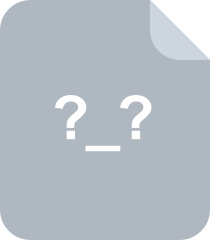
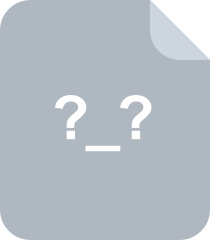
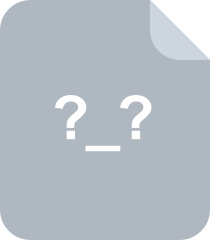
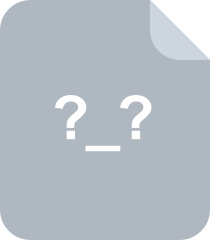
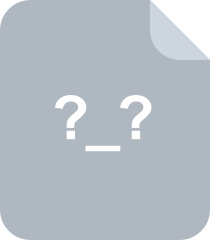
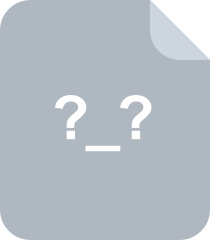
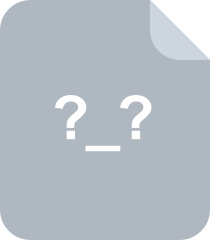
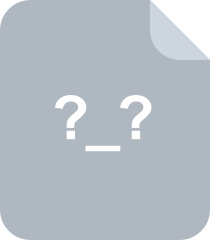
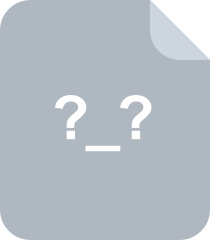
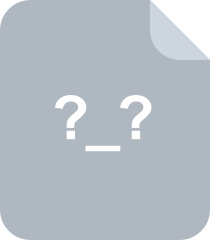
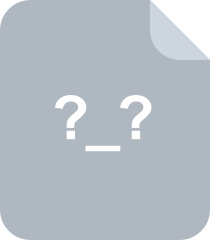
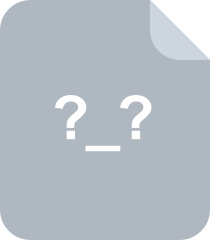
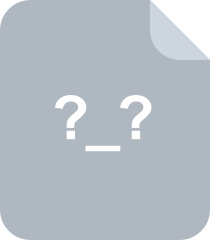
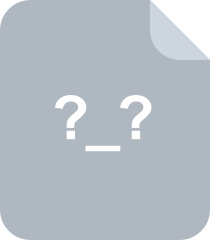
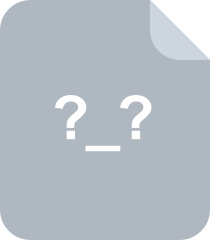
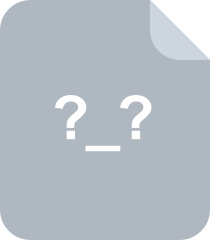
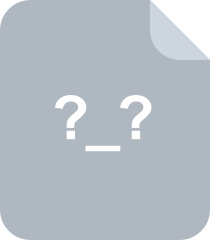
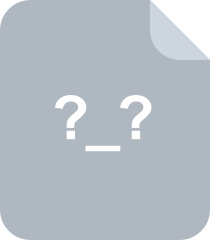
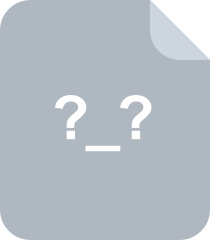
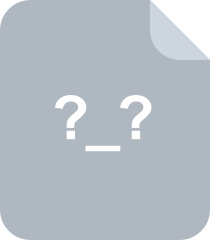
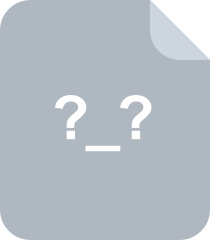
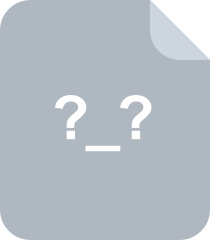
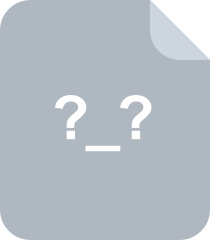
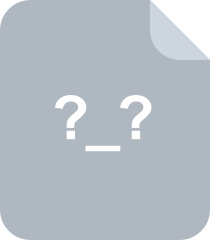
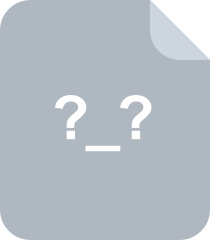
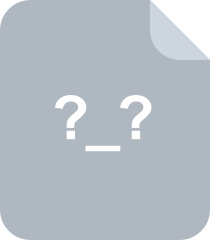
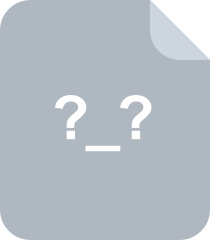
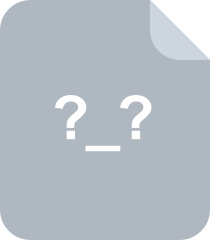
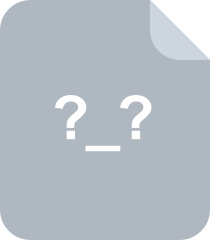
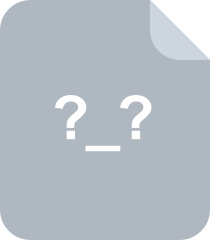
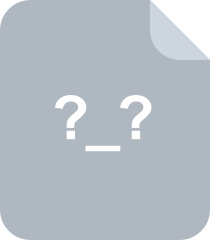
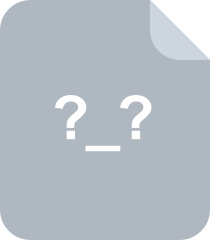
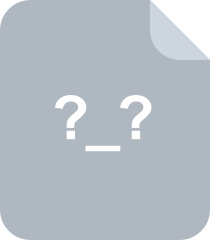
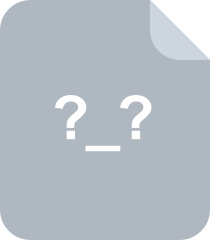
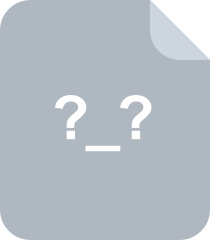
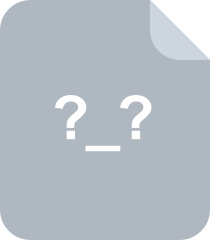
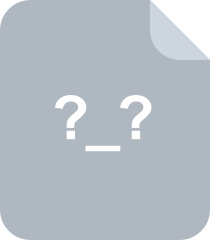
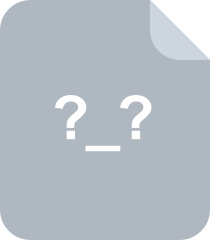
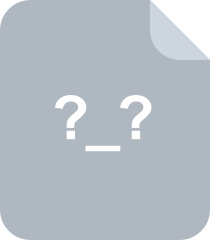
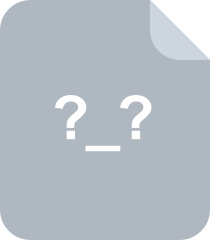
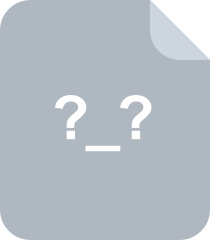
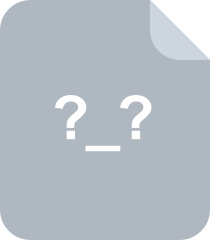
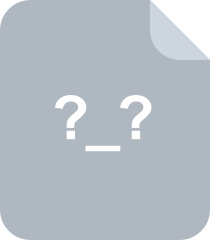
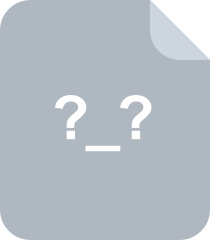
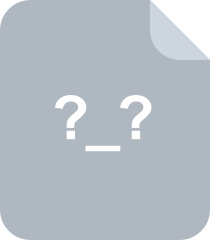
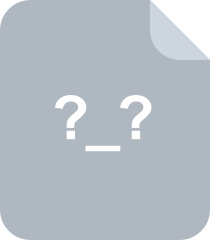
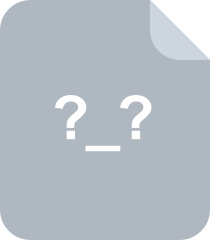
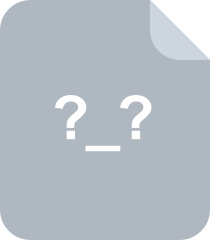
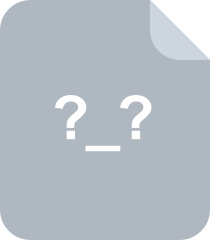
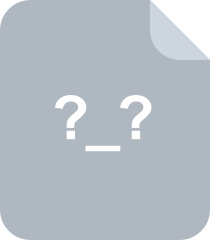
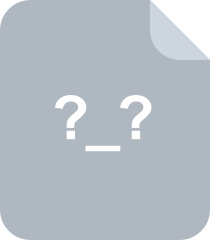
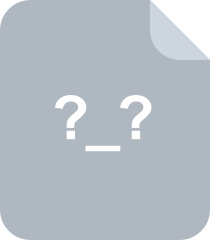
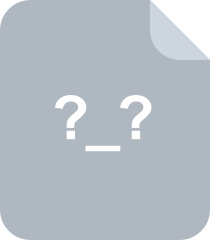
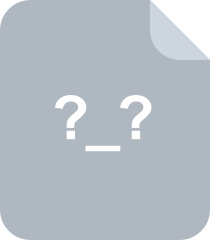
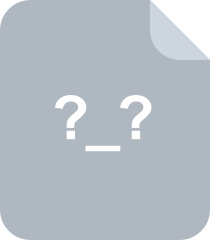
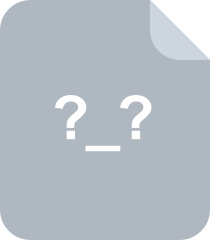
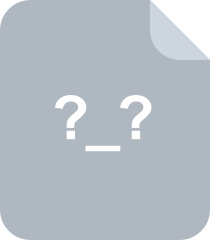
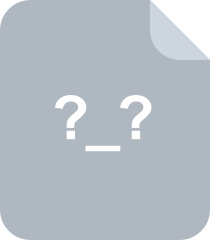
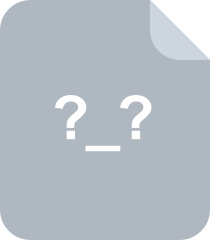
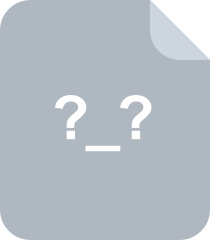
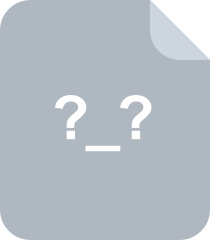
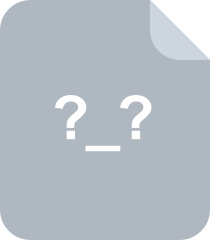
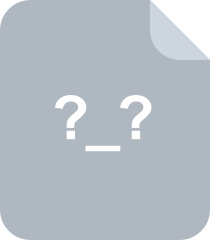
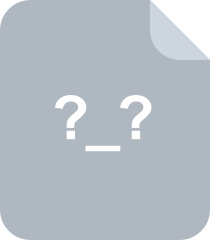
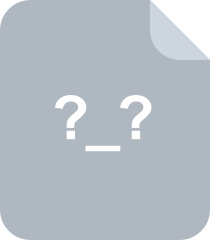
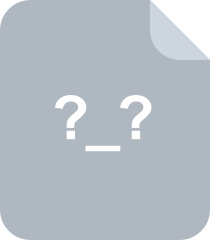
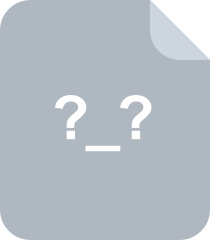
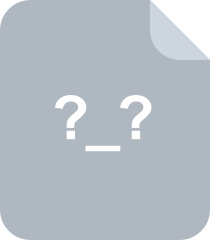
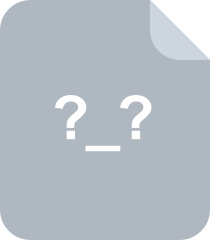
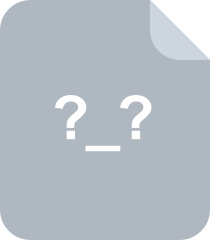
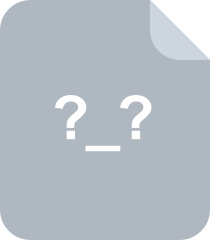
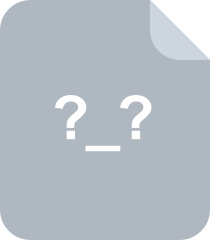
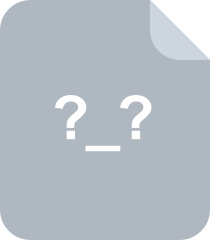
共 383 条
- 1
- 2
- 3
- 4
资源评论
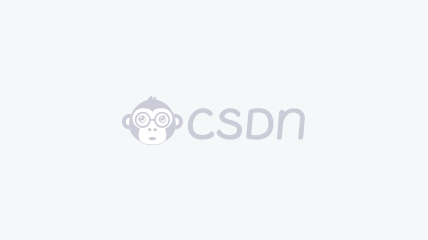

jlj224
- 粉丝: 2
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

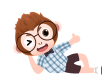
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


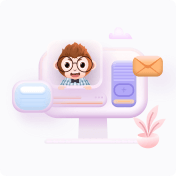
安全验证
文档复制为VIP权益,开通VIP直接复制
