import pygame
import random
# 初始化pygame
pygame.init()
# 设置屏幕大小
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
# 定义一些工具函数
def random_color():
return random.randint(100, 255), random.randint(100, 255), random.randint(100, 255)
def draw_tail(screen, positions, color):
if len(positions) > 1:
for i in range(len(positions) - 1):
pygame.draw.line(screen, color, positions[i], positions[i+1], 2)
class Particle:
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
self.radius = random.randint(4, 7)
self.vel_x = random.uniform(-1, 1) * random.randint(3, 7)
self.vel_y = random.uniform(-1, 1) * random.randint(3, 7)
self.lifetime = random.randint(50, 100)
self.positions = []
def update(self):
self.x += self.vel_x
self.y += self.vel_y
self.lifetime -= 1
self.vel_y += 0.15 # 模拟重力
self.positions.append((self.x, self.y))
if len(self.positions) > 5:
self.positions.pop(0)
def draw(self):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), max(1, self.radius * self.lifetime / 60))
draw_tail(screen, self.positions, self.color)
class Firework:
def __init__(self):
self.x = random.randint(0, width)
self.y = height
self.color = random_color()
self.vel_y = random.randint(-18, -8)
self.exploded = False
self.particles = []
self.positions = []
def explode(self):
for _ in range(100): # 烟花爆炸产生的粒子数
self.particles.append(Particle(self.x, self.y, random_color()))
def update(self):
if not self.exploded:
self.y += self.vel_y
self.vel_y += 0.1 # 模拟重力
self.positions.append((self.x, self.y))
if len(self.positions) > 10:
self.positions.pop(0)
if self.vel_y >= 0:
self.exploded = True
self.explode()
else:
for particle in self.particles:
particle.update()
def draw(self):
if not self.exploded:
pygame.draw.circle(screen, self.color, (self.x, int(self.y)), 5)
draw_tail(screen, self.positions, self.color)
else:
for particle in self.particles:
particle.draw()
fireworks = []
running = True
while running:
screen.fill((0, 0, 0))
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if random.random() < 0.03: # 控制烟花生成的频率
fireworks.append(Firework())
for firework in fireworks[:]:
firework.update()
firework.draw()
if firework.exploded and all(p.lifetime <= 0 for p in firework.particles):
fireworks.remove(firework)
pygame.display.flip()
pygame.time.delay(30)
pygame.quit()

JKooll
- 粉丝: 1264
- 资源: 22
最新资源
- 2024年最新更新!!!!水系数据(全国/分省/分城市/)
- SS928V100 VI 输入场景详细说明.xlsx
- 基于springboot的毕业生信息招聘平台源码(java毕业设计完整源码+LW).zip
- (12006218)数控稳压电源
- (13167858)Java坦克大战
- (172746840)个人对8255实现简易电子琴的理解1
- 基于springboot的餐厅点餐系统源码(java毕业设计完整源码+LW).zip
- (173003038)Java电子相册源码.zip
- (174127818)(完整word版)PLC工业洗衣机.doc
- (174715434)Java小游戏-坦克大战
- 西门子s7 200smart与3台三菱e740变频器通讯程序目的:西门子s7 200smart 控制3台三菱变频器通讯,通讯稳定,可靠 器件:西门子s7 200 smart的PLC,昆仑通态触摸屏(带
- LLM图书 四本《Building An LLM from scratch》《Hands-on LLMs》《AI Engineering》《LLM Engineer’s Handbook》
- (175082856)ensp模拟企业网实例(精品拓扑).zip
- (175183422)clustering的经典k-mean算法源程序,matlab
- (175497242)基于51单片机的数字时钟设计
- (175580038)数字图像处理期末考试模拟题
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


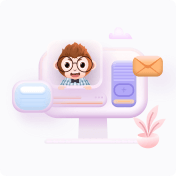