# BrowserMob Proxy
BrowserMob Proxy is a simple utility that makes it easy to capture performance data from browsers, typically written using automation toolkits such as Selenium and Watir.
The latest version of BrowserMobProxy is 2.1.0, powered by [LittleProxy](https://github.com/adamfisk/LittleProxy).
To use BrowserMob Proxy in your tests or application, add the `browsermob-core` dependency to your pom:
```xml
<dependency>
<groupId>net.lightbody.bmp</groupId>
<artifactId>browsermob-core</artifactId>
<version>2.1.0</version>
<scope>test</scope>
</dependency>
```
To run in standalone mode from the command line, download the latest release from the [releases page](https://github.com/lightbody/browsermob-proxy/releases), or [build the latest from source](#building-the-latest-from-source).
For more information on using BrowserMob Proxy with Selenium, see the [Using with Selenium](#using-with-selenium) section.
## Changes since the 2.1-beta series
**The `browsermob-core-littleproxy` module is now `browsermob-core`**
After six beta releases, the LittleProxy implementation now supports more features and is more stable than the legacy implementation. To reflect that level of maturity and long-term support, the `browsermob-core` module now uses LittleProxy by default.
**Note about Legacy support**: In the 2.1-betas, if you were using the `ProxyServer` or `LegacyProxyServer` classes, use the `browsermob-core-legacy` module in 2.1.0.
*LittleProxy support for `LegacyProxyServer` has moved to `BrowserMobProxyServerLegacyAdapter`*. Using the LittleProxy implementation with the `LegacyProxyServer` interface is still fully supported as a means to help you transition from 2.0.0. Unlike the 2.1-beta series, the `BrowserMobProxyServer` class
no longer implements `LegacyProxyServer`; however, the `BrowserMobProxyServerLegacyAdapter` can be used to integrate legacy code with the new LittleProxy interface. You must still use the `browsermob-core-legacy` module when using the LegacyAdapter.
```java
LegacyProxyServer proxy = new BrowserMobProxyServerLegacyAdapter();
proxy.setPort(8081); // method only supported by the legacy interface
proxy.start();
```
## Changes since 2.0.0
The new [BrowserMobProxyServer class](browsermob-core/src/main/java/net/lightbody/bmp/BrowserMobProxyServer.java) has replaced the legacy ProxyServer implementation. The legacy implementation is no longer actively supported; all new code should use `BrowserMobProxyServer`. We highly recommend that existing code migrate to the new implementation.
The most important changes from 2.0 are:
- [Separate REST API and Embedded Mode modules](#embedded-mode). Include only the functionality you need.
- [New BrowserMobProxy interface](browsermob-core/src/main/java/net/lightbody/bmp/BrowserMobProxy.java). The new interface will completely replace the legacy 2.0 ProxyServer contract in version 3.0 and higher.
- [LittleProxy support](#littleproxy-support). More stable and more powerful than the legacy Jetty back-end.
### New BrowserMobProxy API
BrowserMob Proxy 2.1 includes a [new BrowserMobProxy interface](browsermob-core/src/main/java/net/lightbody/bmp/BrowserMobProxy.java) to interact with BrowserMob Proxy programmatically. The new interface defines the functionality that BrowserMob Proxy will support in future releases (including 3.0+). To ease migration, both the legacy (Jetty-based) ProxyServer class and the new, LittleProxy-powered BrowserMobProxy class support the new BrowserMobProxy interface.
We _highly_ recommend migrating existing code to the BrowserMobProxy interface using the `BrowserMobProxyServer` class.
### Using the LittleProxy implementation with 2.0.0 code
The legacy interface, implicitly defined by the ProxyServer class, has been extracted into `net.lightbody.bmp.proxy.LegacyProxyServer` and is now officially deprecated. The new LittleProxy-based implementation will implement LegacyProxyServer for all 2.1.x releases. This means you can switch to the LittleProxy-powered implementation with minimal change to existing code ([with the exception of interceptors](#http-request-manipulation)):
```java
// With the Jetty-based 2.0.0 release, BMP was created like this:
ProxyServer proxyServer = new ProxyServer();
proxyServer.start();
// [...]
// To use the LittleProxy-powered 2.1.0 release, simply change to
// the LegacyProxyServer interface and the adapter for the new
// LittleProxy-based implementation:
LegacyProxyServer proxyServer = new BrowserMobProxyServerLegacyAdapter();
proxyServer.start();
// Almost all deprecated 2.0.0 methods are supported by the
// new BrowserMobProxyServerLegacyAdapter implementation, so in most cases,
// no further code changes are necessary
```
LegacyProxyServer will not be supported after 3.0 is released, so we recommend migrating to the `BrowserMobProxy` interface as soon as possible. The new interface provides additional functionality and is compatible with both the legacy Jetty-based ProxyServer implementation [(with some exceptions)](new-interface-compatibility.md) and the new LittleProxy implementation.
If you must continue using the legacy Jetty-based implementation, include the `browsermob-core-legacy` artifact instead of `browsermob-core`.
## Features and Usage
The proxy is programmatically controlled via a REST interface or by being embedded directly inside Java-based programs and unit tests. It captures performance data in the [HAR format](http://groups.google.com/group/http-archive-specification). In addition it can actually control HTTP traffic, such as:
- blacklisting and whitelisting certain URL patterns
- simulating various bandwidth and latency
- remapping DNS lookups
- flushing DNS caching
- controlling DNS and request timeouts
- automatic BASIC authorization
### REST API
**New in 2.1:** The REST API now supports LittleProxy. As of 2.1.0-beta-3, LittleProxy is the default implementation. (You may specify `--use-littleproxy false` to disable LittleProxy in favor of the legacy Jetty 5-based implementation.)
To get started, first start the proxy by running `browsermob-proxy` or `browsermob-proxy.bat` in the bin directory:
$ sh browsermob-proxy -port 9090
INFO 05/31 03:12:48 o.b.p.Main - Starting up...
2011-05-30 20:12:49.517:INFO::jetty-7.3.0.v20110203
2011-05-30 20:12:49.689:INFO::started o.e.j.s.ServletContextHandler{/,null}
2011-05-30 20:12:49.820:INFO::Started SelectChannelConnector@0.0.0.0:9090
Once started, there won't be an actual proxy running until you create a new proxy. You can do this by POSTing to /proxy:
[~]$ curl -X POST http://localhost:9090/proxy
{"port":9091}
or optionally specify your own port:
[~]$ curl -X POST -d 'port=9099' http://localhost:9090/proxy
{"port":9099}
or if running BrowserMob Proxy in a multi-homed environment, specify a desired bind address (default is `0.0.0.0`):
[~]$ curl -X POST -d 'bindAddress=192.168.1.222' http://localhost:9090/proxy
{"port":9096}
Once that is done, a new proxy will be available on the port returned. All you have to do is point a browser to that proxy on that port and you should be able to browse the internet. The following additional APIs will then be available:
Description | HTTP method | Request path | Request parameters
--- | :---: | :---: | ---
Get a list of ports attached to `ProxyServer` instances managed by `ProxyManager` | GET | */proxy* ||
<a name="harcreate">Creates a new HAR</a> attached to the proxy and returns the HAR content if there was a previous HAR. *[port]* in request path it is port where your proxy was started | PUT |*/proxy/[port]/har* |<p>*captureHeaders* - Boolean, capture headers or not. Optional, default to "true".</p><p>*captureContent* - Boolean, capture content bodies or not. Optional, default to "false".</p><p>*captureBinaryContent* - Boolean, capture binary content or not

jjter2002
- 粉丝: 1
- 资源: 8
最新资源
- 燃油泵结构图sw18可编辑全套技术资料100%好用.zip
- NPC三电平逆变器 SVPWM plecs c语言 电压电流双闭环控制 SVPWM使用c-script模块使用c语言编写 工况如下 直流电压Vdc 800V 负载侧电压幅值控制到311V具体波形
- 三轴模组坐标机械手sw20全套技术资料100%好用.zip
- 基于matlab上的DES和RSA两种算法的双重加密,附带显示界面,可更改DES密钥,明文消息(在显示界面中),可在代码中更改RSA对应的p,q,e等数据,代码可附加注释和对应要求修改
- 基于simulink的35kv变电站三相故障仿真,包含变压器和线路
- 格子玻尔兹曼方法 LBM- IBM 模拟下坠(流固耦合)包含球碰撞及和壁面碰撞 C++代码
- COA-CNN-BiGRU-Attention分类 基于浣熊优化算法优化卷积神经网络(CNN)-双向门控循环单元(BGRU)结合注意力机制(Attention)的数据分类预测(可更为回归 单变量 多变
- 三相不平衡电压下并网逆变器并网控制,三相两电平逆变器控制 1.采用正负序分离锁相环以及正序PI控制,负序PI控制 2.SPWM 3.提供参考文献 提供仿真源文件,电流环参数设计,正负序分离方法详解
- 基于有限差分-嵌入式离散裂缝网络(FDM-EDFM)的油气藏地层压力场计算,通过matlab代码实现,可提供理论指导和相关问题,可计算不同裂缝网络的压力分布
- 2023大型企业数字化转型管控平台解决方案.pdf
- 《大模型安全实践(2024)》白皮书.pdf
- 2023金融业数据分类分级与保护应用研究.pdf
- 2023年车路协同算力网络白皮书.pdf
- 2023年通用人工智能AGI等级保护白皮书.pdf
- 2023年中医诊疗数字化发展白皮书.pdf
- 2023人工智能大模型体验报告3.0.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


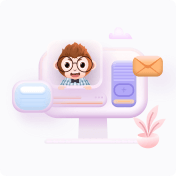