### PRBS Check Module in Verilog The provided Verilog code snippet outlines the implementation of a Pseudo-Random Binary Sequence (PRBS) checker and error injector module. PRBS is a sequence of binary values that is statistically random but deterministic, often used in digital communication systems for testing purposes. The PRBS checker verifies the integrity of received data by comparing it against an expected PRBS pattern, while the error injector intentionally introduces errors into the data stream to test the system’s ability to detect and correct them. #### Key Components of the PRBS Checker Module 1. **Finite State Machine (FSM):** - The FSM is the core component of the PRBS checker, controlling its operation through various states. It transitions between states based on input signals such as `DIN` (Data In) and `DVAL` (Data Valid Strobe). - The FSM has several states: - `ST_IDLE`: Waits for valid data to arrive. - `ST_LOAD0`, `ST_LOAD1`, `ST_LOAD2`, `ST_LOAD3`, `ST_LOAD4`: Sequentially load the received bits into the Linear Feedback Shift Register (LFSR). - `ST_MATCH`: Indicates that the sequence lock has been achieved. - Each state transition is controlled by the presence of valid data (`DVAL`), which triggers the loading of new bits into the LFSR. 2. **Linear Feedback Shift Register (LFSR):** - An N-bit LFSR is instantiated with parameters defined within the code. The LFSR generates the expected PRBS pattern based on predefined taps (`tap0`, `tap1`, etc.). - The LFSR is loaded with incoming data bits (`DIN`) during the `ST_LOAD*` states and shifts during normal operation when `LFSR_SHIFT` is set to `1`. - The LFSR output (`LFSR_DOUT`) is compared with the incoming data to determine if the received sequence matches the expected PRBS pattern. 3. **Control Signals:** - `LFSR_LOAD`: Enables the loading of data into the LFSR. - `LFSR_SHIFT`: Controls the shifting of the LFSR. - `LOAD_EN`: Enables the loading of data into the LFSR based on the current state and the presence of valid data. - `LOCK`: Indicates whether the PRBS lock has been achieved. If `1`, the sequence matches the expected PRBS pattern. 4. **Synchronization Logic:** - Delayed versions of `DIN` and `DVAL` (`DEL_DIN` and `DEL_DVAL`) are used to ensure proper synchronization and timing within the FSM. #### Detailed Explanation of the Code Snippet - **Module Declaration:** - The module is declared as `modulePRBS_CHK(CLK, RESET, DIN, DVAL, LOCK);`. It takes five inputs: `CLK` (Clock signal), `RESET` (Reset signal), `DIN` (Data In), `DVAL` (Data Valid Strobe), and one output `LOCK` (PRBS Lock Indicator). - **Parameters:** - Parameters define the states of the FSM and the size of the LFSR (`N`). - `ST_IDLE`, `ST_LOAD0`, `ST_LOAD1`, `ST_LOAD2`, `ST_LOAD3`, `ST_LOAD4`, `ST_MATCH`: FSM states. - `N`: Size of the LFSR (5 bits in this case). - **Inputs and Outputs:** - `CLK`: Clock signal. - `RESET`: Asynchronous reset (active-high). - `DIN`: Input data. - `DVAL`: Data valid strobe. Indicates when `DIN` contains a valid PRBS bit. - `LOCK`: PRBS lock indicator. `1` indicates the sequence lock is achieved, `0` means `DIN` is not a valid sequence. - **Registers:** - `LOCK`, `LOCK_N`: Current and next state of the `LOCK` output. - `STATE`, `STATE_N`: Current and next state of the FSM. - `LOAD_EN`, `LOAD_EN_N`: Enables loading into the LFSR. - `DEL_DIN`, `DEL_DVAL`: Delayed versions of `DIN` and `DVAL`. - `LFSR_SHIFT`, `LFSR_SHIFT_N`: Controls shifting in the LFSR. - **Instantiation of the LFSR:** - The LFSR is instantiated using the `LFSR` module with parameters defining its size and tap positions. - The LFSR outputs (`LFSR_DOUT`) are compared with the incoming data to determine if the sequence matches the expected PRBS pattern. - **Behavioral Description:** - The `always` block describes the behavior of the FSM and control signals. - The FSM transitions between states based on the presence of valid data (`DVAL`). - The `LFSR_LOAD` signal enables the loading of data into the LFSR. - The FSM transitions from `ST_IDLE` to `ST_LOAD0` upon receiving the first valid data bit, and then sequentially loads each subsequent bit until the `ST_LOAD4` state. - Once all bits are loaded, the FSM transitions to `ST_MATCH`, indicating that the sequence lock has been achieved. In summary, the provided code snippet implements a PRBS checker module in Verilog that verifies the integrity of received data by comparing it against an expected PRBS pattern. The module uses an FSM to control the loading and shifting of data into an LFSR, and provides a `LOCK` output to indicate when the sequence lock is achieved. This module is essential for testing and verifying the functionality of digital communication systems.
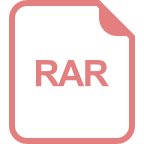
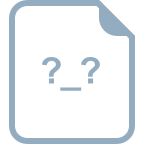
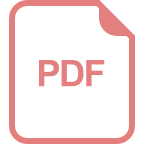
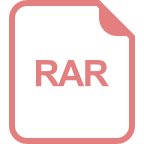
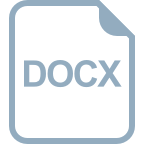
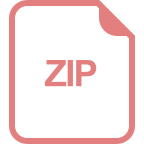
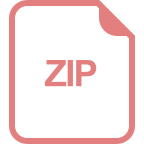
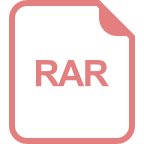
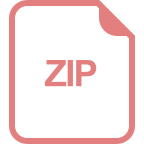
parameter ST_IDLE = 0;
parameter ST_LOAD0 = 1;
parameter ST_LOAD1 = 2;
parameter ST_LOAD2 = 3;
parameter ST_LOAD3 = 4;
parameter ST_LOAD4 = 5;
parameter ST_MATCH = 6;
// LFSR size
parameter N = 5;
input CLK;
input RESET; // Asynchronous reset (active-high)
input DIN; // Input data
input DVAL; // Data valid strobe
// 1 => DIN is PRBS
// 0 => DIN needs to be disregarded
output LOCK; // PRBS lock indicator
// 1 => Sequence lock achieved
// 0 => DIN is not a valid sequence
reg LOCK, LOCK_N; // Next state of LOCK output
reg [2:0] STATE, STATE_N; // State variable for checker FSM
reg LOAD_EN, LOAD_EN_N; // Enables LFSR load signal
reg DEL_DVAL; // DVAL delayed by 1 clock cycle
reg LFSR_SHIFT, LFSR_SHIFT_N; // LFSR's SHIFT
wire [N-1:0] LFSR_DOUT; // LFSR output
wire LFSR_LOAD; // LFSR's LOAD
// Instantiate the LFSR with parameters
defparam lfsr.N = N; // N-bit LFSR
defparam lfsr.tap0 = 0;
defparam lfsr.tap1 = 2;
defparam lfsr.tap2 = 2;
defparam lfsr.tap3 = 2;
LFSR lfsr (
.CLK(CLK),
.RESET(RESET),
.DIN(DIN),
.LOAD(LFSR_LOAD),
.SHIFT(LFSR_SHIFT),
.Q(LFSR_DOUT)
);
always @ (posedge CLK or posedge RESET)
begin
if (RESET) begin
DEL_DIN <= #1 1'b0;
LOCK <= #1 1'b0;
剩余5页未读,继续阅读
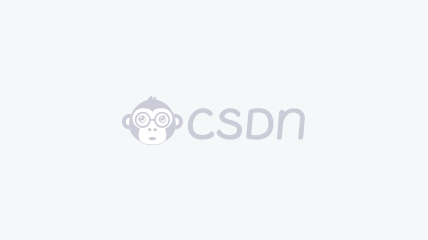
- 木箫思木2019-02-21下载后没什么用处。
- liyangxian2016-02-27无实用和参考价值。本科生做个毕业设计还可以用用,无应用价值。

- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

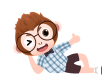
最新资源

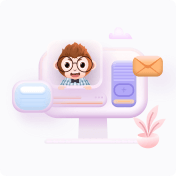
