c调用windows api文件夹增删改
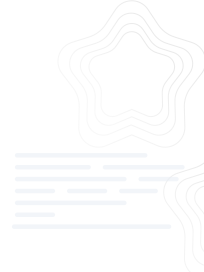


在C语言中调用Windows API来操作文件夹的增删改是常见的系统编程技术。Windows API是一组函数库,提供了操作系统级别的服务,包括文件系统管理。以下将详细讲解如何使用C语言结合Windows API进行文件夹的操作。 我们需要包含必要的头文件,如`windows.h`,它包含了大部分Windows API函数的声明: ```c #include <windows.h> ``` **增加文件夹:** 创建新文件夹可以使用`CreateDirectory`函数。这个函数需要两个参数,一个是新文件夹的完整路径,另一个是安全属性指针(一般设为NULL)。 ```c BOOL CreateDirectory(LPCTSTR lpPathName, LPSECURITY_ATTRIBUTES lpSecurityAttributes); ``` 例如: ```c #include <windows.h> #include <stdio.h> int main() { if (CreateDirectory("C:\\NewFolder", NULL)) { printf("新文件夹创建成功。\n"); } else { printf("创建文件夹失败,错误代码:%d\n", GetLastError()); } return 0; } ``` **删除文件夹:** 删除空文件夹可以使用`RemoveDirectory`函数,但如果是非空文件夹,则需要先删除其中的所有文件和子文件夹。 ```c BOOL RemoveDirectory(LPCTSTR lpPathName); ``` 例如: ```c #include <windows.h> #include <stdio.h> int main() { if (RemoveDirectory("C:\\NewFolder")) { printf("文件夹删除成功。\n"); } else { printf("删除文件夹失败,错误代码:%d\n", GetLastError()); } return 0; } ``` **修改文件夹:** 文件夹的修改通常涉及到重命名操作,这可以通过`MoveFile`或`MoveFileEx`函数完成。这两个函数都可以用来移动或重命名文件或文件夹。 ```c BOOL MoveFile(LPCSTR lpExistingFileName, LPCSTR lpNewFileName); BOOL MoveFileEx(LPCSTR lpExistingFileName, LPCSTR lpNewFileName, DWORD dwFlags); ``` 例如,重命名文件夹: ```c #include <windows.h> #include <stdio.h> int main() { if (MoveFile("C:\\NewFolder", "C:\\RenamedFolder")) { printf("文件夹重命名成功。\n"); } else { printf("重命名文件夹失败,错误代码:%d\n", GetLastError()); } return 0; } ``` 在实际应用中,可能还需要处理更复杂的情况,如递归删除非空文件夹、权限检查等。此外,对于文件操作,Windows API还提供了`FindFirstFile`、`FindNextFile`等函数用于遍历文件夹内容,以及`CopyFile`、`DeleteFile`等函数用于文件操作。这些函数在进行文件夹管理时都非常有用。 总结来说,C语言调用Windows API进行文件夹操作涉及创建、删除和重命名等基本功能,需要理解并正确使用相应的API函数,同时注意错误处理和权限控制。在开发过程中,确保程序的健壮性和安全性至关重要。通过实践和学习,你可以熟练掌握这些技巧,为你的项目提供强大的文件系统管理能力。
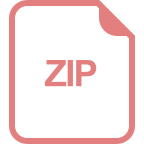
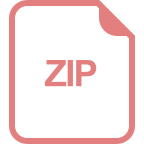
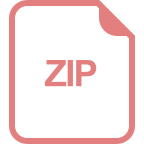
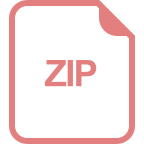
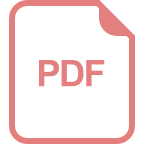
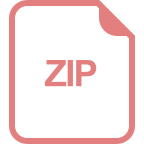
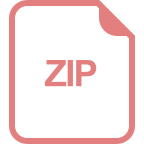
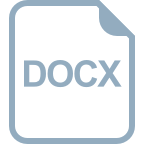
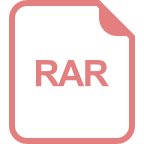
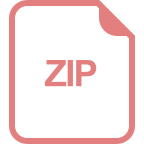
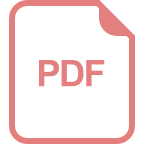
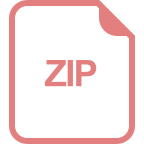
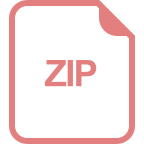
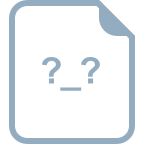
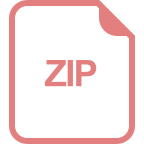
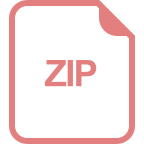
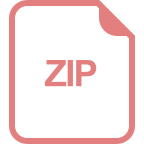
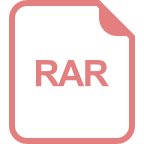
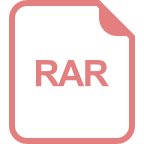
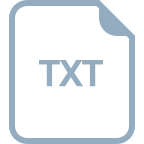
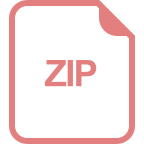


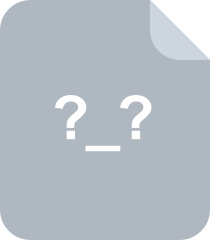
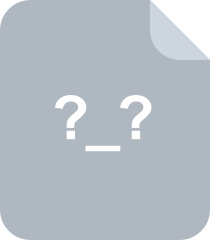
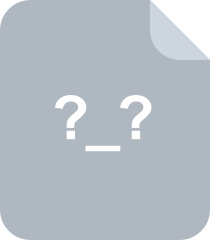
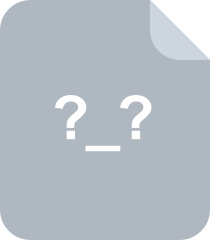
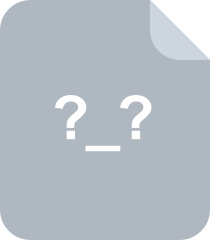

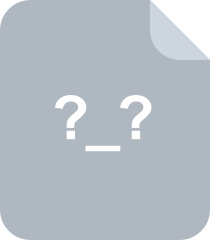
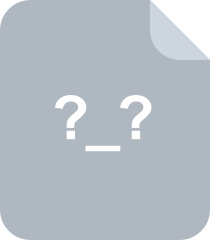


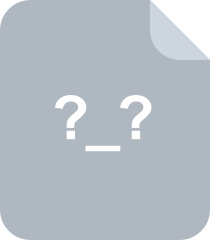
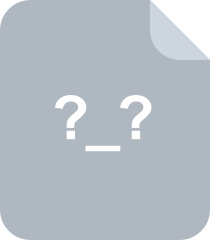
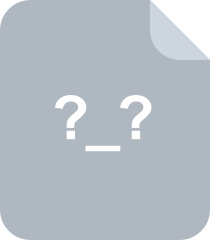


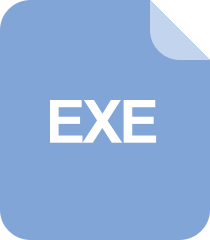
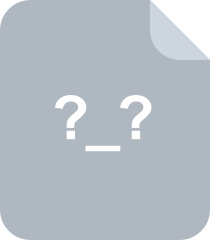
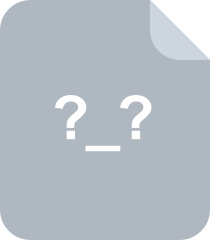
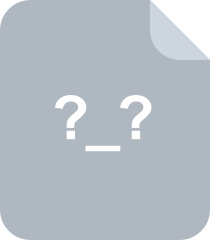
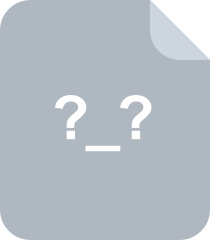
- 1

- 粉丝: 13
- 资源: 37
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

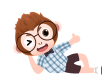
最新资源
- 新年倒计时网页基础教程
- Python编程初学者快速入门基础教程
- 新年倒计时编程基础教程
- 峰会报告自动化处理基础教程
- UE4UE5游戏开发基础教程:从零开始构建你的世界
- DataStructure-拓扑排序
- Front-end-learning-to-organize-notes-新年主题资源
- QPython Plus-Python资源
- baidulite-新年主题资源
- CnOCR-Python资源
- Golang_Puzzlers-新年主题资源
- Python开源扫雷游戏PyMine-Python资源
- Golang_Puzzlers-新年主题资源
- pyporter-Python资源
- Golang_Puzzlers-新年主题资源
- mulan-rework-Python资源

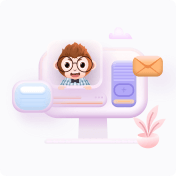

- 1
- 2
前往页