package zicox.esc;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
import android.app.Activity;
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.bluetooth.BluetoothSocket;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.content.IntentFilter;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.ListView;
import android.widget.Toast;
import android.widget.ToggleButton;
public class Demo_ad_escActivity extends Activity
{
//---------------------------------------------------
public static String ErrorMessage;
Button btnSearch, btnDis, btnExit;
ToggleButton tbtnSwitch;
ListView lvBTDevices;
ArrayAdapter<String> adtDevices;
List<String> lstDevices = new ArrayList<String>();
BluetoothAdapter btAdapt;
public static BluetoothSocket btSocket;
//---------------------------------------------------
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
// if(!ListBluetoothDevice())finish();
Button Button1 = (Button) findViewById(R.id.button1);
ErrorMessage = "";
//---------------------------------------------------
btnSearch = (Button) this.findViewById(R.id.btnSearch);
btnSearch.setOnClickListener(new ClickEvent());
btnExit = (Button) this.findViewById(R.id.btnExit);
btnExit.setOnClickListener(new ClickEvent());
btnDis = (Button) this.findViewById(R.id.btnDis);
btnDis.setOnClickListener(new ClickEvent());
// ToogleButton设置
tbtnSwitch = (ToggleButton) this.findViewById(R.id.toggleButton1);
tbtnSwitch.setOnClickListener(new ClickEvent());
// ListView及其数据源 适配器
lvBTDevices = (ListView) this.findViewById(R.id.listView1);
adtDevices = new ArrayAdapter<String>(this,
android.R.layout.simple_list_item_1, lstDevices);
lvBTDevices.setAdapter(adtDevices);
lvBTDevices.setOnItemClickListener(new ItemClickEvent());
btAdapt = BluetoothAdapter.getDefaultAdapter();// 初始化本机蓝牙功能
if (btAdapt.isEnabled())
tbtnSwitch.setChecked(false);
else
tbtnSwitch.setChecked(true);
// 注册Receiver来获取蓝牙设备相关的结果
String ACTION_PAIRING_REQUEST = "android.bluetooth.device.action.PAIRING_REQUEST";
IntentFilter intent = new IntentFilter();
intent.addAction(BluetoothDevice.ACTION_FOUND);// 用BroadcastReceiver来取得搜索结果
intent.addAction(BluetoothDevice.ACTION_BOND_STATE_CHANGED);
intent.addAction(ACTION_PAIRING_REQUEST);
intent.addAction(BluetoothAdapter.ACTION_SCAN_MODE_CHANGED);
intent.addAction(BluetoothAdapter.ACTION_STATE_CHANGED);
registerReceiver(searchDevices, intent);
Button1.setOnClickListener(new Button.OnClickListener()
{
public void onClick(View arg0)
{
// Print1(SelectedBDAddress);
}
});
}
//---------------------------------------------------
private BroadcastReceiver searchDevices = new BroadcastReceiver() {
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
Bundle b = intent.getExtras();
Object[] lstName = b.keySet().toArray();
// 显示所有收到的消息及其细节
for (int i = 0; i < lstName.length; i++) {
String keyName = lstName[i].toString();
Log.e(keyName, String.valueOf(b.get(keyName)));
}
BluetoothDevice device = null;
// 搜索设备时,取得设备的MAC地址
if (BluetoothDevice.ACTION_FOUND.equals(action)) {
device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE);
if (device.getBondState() == BluetoothDevice.BOND_NONE) {
String str = "未配对|" + device.getName() + "|"
+ device.getAddress();
if (lstDevices.indexOf(str) == -1)// 防止重复添加
lstDevices.add(str); // 获取设备名称和mac地址
adtDevices.notifyDataSetChanged();
try {
ClsUtils.setPin(device.getClass(),device,"0000");
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
try {
ClsUtils.cancelPairingUserInput(device.getClass(), device);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}else if(BluetoothDevice.ACTION_BOND_STATE_CHANGED.equals(action)){
device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE);
switch (device.getBondState()) {
case BluetoothDevice.BOND_BONDING:
Log.d("BlueToothTestActivity", "正在配对......");
break;
case BluetoothDevice.BOND_BONDED:
Log.d("BlueToothTestActivity", "完成配对");
connect(device);//连接设备
break;
case BluetoothDevice.BOND_NONE:
Log.d("BlueToothTestActivity", "取消配对");
default:
break;
}
}
if (intent.getAction().equals("android.bluetooth.device.action.PAIRING_REQUEST"))
{
Log.e("tag11111111111111111111111", "ddd");
BluetoothDevice btDevice = intent
.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE);
// byte[] pinBytes = BluetoothDevice.convertPinToBytes("1234");
// device.setPin(pinBytes);
try
{
ClsUtils.setPin(btDevice.getClass(), btDevice, "0000"); // 手机和蓝牙采集器配对
ClsUtils.createBond(btDevice.getClass(), btDevice);
ClsUtils.cancelPairingUserInput(btDevice.getClass(), btDevice);
}
catch (Exception e)
{
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
};
class ItemClickEvent implements AdapterView.OnItemClickListener {
@Override
public void onItemClick(AdapterView<?> arg0, View arg1, int arg2,
long arg3) {
if(btAdapt.isDiscovering())btAdapt.cancelDiscovery();
String str = lstDevices.get(arg2);
String[] values = str.split("\\|");
String address = values[2];
Log.e("address", values[2]);
BluetoothDevice btDev = btAdapt.getRemoteDevice(address);
try {
Boolean returnValue = false;
if (btDev.getBondState() == BluetoothDevice.BOND_NONE) {
//利用反射方法调用BluetoothDevice.createBond(BluetoothDevice remoteDevice);
// Method createBondMethod = BluetoothDevice.class.getMethod("createBond");
// Log.d("BlueToothTestActivity", "开始配对");
//

jilong17
- 粉丝: 59
- 资源: 11
最新资源
- C# Winform简单的俄罗斯方块小游戏源码2.zip
- 混合动力汽车动态规划算法理论油耗计算与视频教学,使用matlab编写快速计算程序,整个工程结构模块化,可以快速改为串联,并联,混联等 控制量可以快速扩展为档位,转矩,转速等 状态量一般为SOC,目
- 全国职业院校技能大赛网络建设与运维规程
- agv 1223.fbx
- 考虑泄流效应的光伏无功优化matlab 以IEEE33节点为例,分析泄流效应下,最佳网络无功补偿方案,程序运行稳定
- jetbra插件工具,方便开发者快速开发
- 云计算2401班课程设计资料.zip
- 企业宣传PPT模板, 企业宣传PPT模板
- 微环谐振腔的光学频率梳matlab仿真 微腔光频梳仿真 包括求解LLE方程(Lugiato-Lefever equation)实现微环中的光频梳,同时考虑了色散,克尔非线性,外部泵浦等因素,具有可延展
- 生菜生长记录数据集.zip
- 基于Springboot+Vue健身房管理系统-毕业源码案例设计(高分项目).zip
- 中国风格, 节日 主题, PPT模板
- lcd取模工具,很难找的有用的LCD显示开发工具
- 基于Springboot+Vue健身房管理系统-毕业源码案例设计(源码+数据库).zip
- 基于Springboot+Vue江理工文档管理系统的设计与实现-毕业源码案例设计(源码+论文).zip
- 基于Springboot+Vue教师工作量管理系统-毕业源码案例设计(高分毕业设计).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


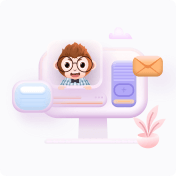
- 1
- 2
- 3
前往页