/*!
* FormValidation (http://formvalidation.io)
* The best jQuery plugin to validate form fields. Support Bootstrap, Foundation, Pure, SemanticUI, UIKit and custom frameworks
*
* @version v0.6.2-dev, built on 2015-03-13 8:15:45 AM
* @author https://twitter.com/nghuuphuoc
* @copyright (c) 2013 - 2015 Nguyen Huu Phuoc
* @license http://formvalidation.io/license/
*/
// Register the namespace
window.FormValidation = {
AddOn: {}, // Add-ons
Framework: {}, // Supported frameworks
I18n: {}, // i18n
Validator: {} // Available validators
};
if (typeof jQuery === 'undefined') {
throw new Error('FormValidation requires jQuery');
}
(function ($) {
var version = $.fn.jquery.split(' ')[0].split('.');
if ((+version[0] < 3 && +version[1] < 9) || (+version[0] === 3 && +version[1] === 0 && +version[2] < 0)) {
throw new Error('FormValidation requires jQuery version 3.0.0 or higher');
}
}(jQuery));
(function ($) {
// TODO: Remove backward compatibility in v0.7.0
/**
* Constructor
*
* @param {jQuery|String} form The form element or selector
* @param {Object} options The options
* @param {String} [namespace] The optional namespace which is used for data-{namespace}-xxx attributes and internal data.
* Currently, it's used to support backward version
* @constructor
*/
FormValidation.Base = function (form, options, namespace) {
this.$form = $(form);
this.options = $.extend({}, $.fn.formValidation.DEFAULT_OPTIONS, options);
this._namespace = namespace || 'fv';
this.$invalidFields = $([]); // Array of invalid fields
this.$submitButton = null; // The submit button which is clicked to submit form
this.$hiddenButton = null;
// Validating status
this.STATUS_NOT_VALIDATED = 'NOT_VALIDATED';
this.STATUS_VALIDATING = 'VALIDATING';
this.STATUS_INVALID = 'INVALID';
this.STATUS_VALID = 'VALID';
this.STATUS_IGNORED = 'IGNORED';
// Determine the event that is fired when user change the field value
// Most modern browsers supports input event except IE 7, 8.
// IE 9 supports input event but the event is still not fired if I press the backspace key.
// Get IE version
// https://gist.github.com/padolsey/527683/#comment-7595
var ieVersion = (function () {
var v = 3, div = document.createElement('div'), a = div.all || [];
while (div.innerHTML = '<!--[if gt IE ' + (++v) + ']><br><![endif]-->', a[0]) {
}
return v > 4 ? v : !v;
}());
var el = document.createElement('div');
this._changeEvent = (ieVersion === 9 || !('oninput' in el)) ? 'keyup' : 'input';
// The flag to indicate that the form is ready to submit when a remote/callback validator returns
this._submitIfValid = null;
// Field elements
this._cacheFields = {};
this._init();
};
FormValidation.Base.prototype = {
constructor: FormValidation.Base,
/**
* Check if the number of characters of field value exceed the threshold or not
*
* @param {jQuery} $field The field element
* @returns {Boolean}
*/
_exceedThreshold: function ($field) {
var ns = this._namespace,
field = $field.attr('data-' + ns + '-field'),
threshold = this.options.fields[field].threshold || this.options.threshold;
if (!threshold) {
return true;
}
var cannotType = $.inArray($field.attr('type'), ['button', 'checkbox', 'file', 'hidden', 'image', 'radio', 'reset', 'submit']) !== -1;
return (cannotType || $field.val().length >= threshold);
},
/**
* Init form
*/
_init: function () {
var that = this,
ns = this._namespace,
options = {
addOns: {},
autoFocus: this.$form.attr('data-' + ns + '-autofocus'),
button: {
selector: this.$form.attr('data-' + ns + '-button-selector') || this.$form.attr('data-' + ns + '-submitbuttons'), // Support backward
disabled: this.$form.attr('data-' + ns + '-button-disabled')
},
control: {
valid: this.$form.attr('data-' + ns + '-control-valid'),
invalid: this.$form.attr('data-' + ns + '-control-invalid')
},
err: {
clazz: this.$form.attr('data-' + ns + '-err-clazz'),
container: this.$form.attr('data-' + ns + '-err-container') || this.$form.attr('data-' + ns + '-container'), // Support backward
parent: this.$form.attr('data-' + ns + '-err-parent')
},
events: {
formInit: this.$form.attr('data-' + ns + '-events-form-init'),
formError: this.$form.attr('data-' + ns + '-events-form-error'),
formSuccess: this.$form.attr('data-' + ns + '-events-form-success'),
fieldAdded: this.$form.attr('data-' + ns + '-events-field-added'),
fieldRemoved: this.$form.attr('data-' + ns + '-events-field-removed'),
fieldInit: this.$form.attr('data-' + ns + '-events-field-init'),
fieldError: this.$form.attr('data-' + ns + '-events-field-error'),
fieldSuccess: this.$form.attr('data-' + ns + '-events-field-success'),
fieldStatus: this.$form.attr('data-' + ns + '-events-field-status'),
localeChanged: this.$form.attr('data-' + ns + '-events-locale-changed'),
validatorError: this.$form.attr('data-' + ns + '-events-validator-error'),
validatorSuccess: this.$form.attr('data-' + ns + '-events-validator-success'),
validatorIgnored: this.$form.attr('data-' + ns + '-events-validator-ignored')
},
excluded: this.$form.attr('data-' + ns + '-excluded'),
icon: {
valid: this.$form.attr('data-' + ns + '-icon-valid') || this.$form.attr('data-' + ns + '-feedbackicons-valid'), // Support backward
invalid: this.$form.attr('data-' + ns + '-icon-invalid') || this.$form.attr('data-' + ns + '-feedbackicons-invalid'), // Support backward
validating: this.$form.attr('data-' + ns + '-icon-validating') || this.$form.attr('data-' + ns + '-feedbackicons-validating'), // Support backward
feedback: this.$form.attr('data-' + ns + '-icon-feedback')
},
live: this.$form.attr('data-' + ns + '-live'),
locale: this.$form.attr('data-' + ns + '-locale'),
message: this.$form.attr('data-' + ns + '-message'),
onError: this.$form.attr('data-' + ns + '-onerror'),
onSuccess: this.$form.attr('data-' + ns + '-onsuccess'),
row: {
selector: this.$form.attr('data-' + ns + '-row-selector') || this.$form.attr('data-' + ns + '-group'), // Support backward
valid: this.$form.attr('data-' + ns + '-row-valid'),
invalid: this.$form.attr('data-' + ns + '-row-invalid'),
feedback: this.$form.attr('data-' + ns + '-row-feedback')
},
threshold: this.$form.attr('data-' + ns + '-threshold'),
trigger: this.$form.attr('data-' + ns + '-trigger'),
verbose: this.$form.attr('data-' + ns + '
没有合适的资源?快使用搜索试试~ 我知道了~
bootstrap框架后台管理系统示例
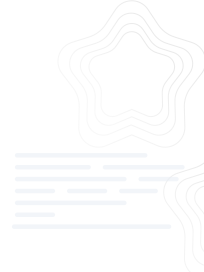
共616个文件
js:295个
png:119个
css:51个

需积分: 1 1 下载量 190 浏览量
2023-12-15
09:14:00
上传
评论
收藏 89.98MB ZIP 举报
温馨提示
Bootstrap是一个由Twitter公司的设计师Mark Otto和Jacob Thornton合作基于HTML、CSS、JavaScript开发的简洁、直观、强悍的前端开发框架,使得Web开发更加快捷。 Bootstrap提供了优雅的HTML和CSS规范,它即是由动态CSS语言Less写成。Bootstrap一经推出后颇受欢迎,一直是GitHub上的热门开源项目,包括NASA的MSNBC(微软全国广播公司)的Breaking News都使用了该项目。国内一些移动开发者较为熟悉的框架,如WeX5前端开源框架等,也是基于Bootstrap源码进行性能优化而来。 Bootstrap是一个用于快速开发Web应用程序和网站的前端框架。它的优势包括: 1. 浏览器支持:所有的主流浏览器都支持Bootstrap。 2. 容易上手:只要具备HTML和CSS的基础知识,就可以开始学习Bootstrap。 3. 响应式设计:Bootstrap的响应式CSS能够自适应于台式机、平板电脑和手机。 4. 提供功能强大的内置组件,易于定制。 5. 提供基于Web的定制。
资源推荐
资源详情
资源评论
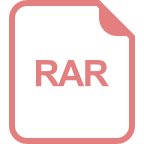
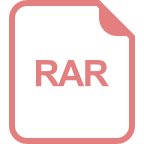
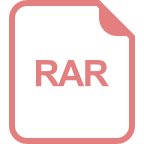
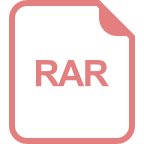
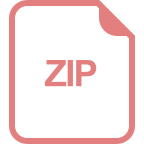
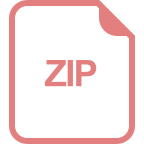
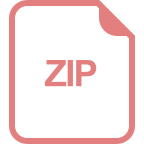
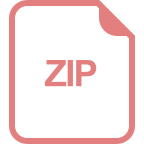
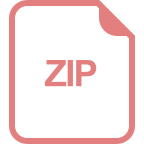
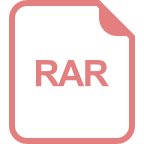
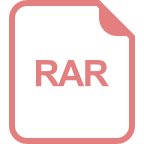
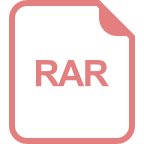
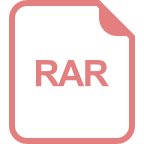
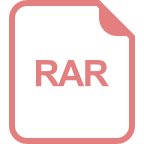
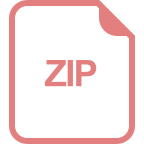
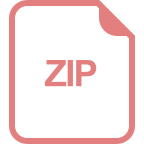
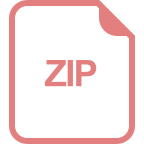
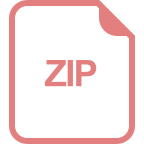
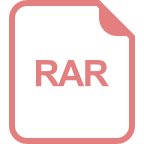
收起资源包目录

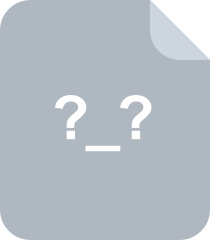
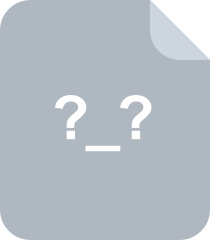
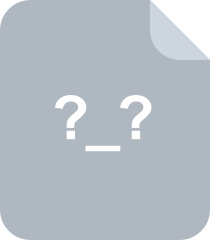
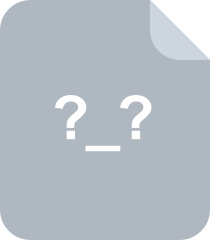
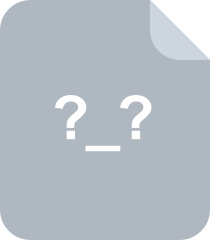
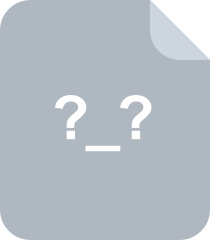
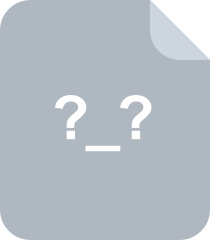
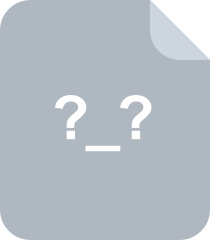
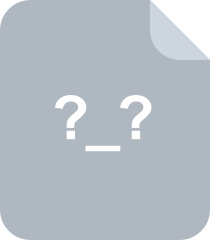
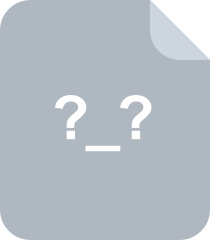
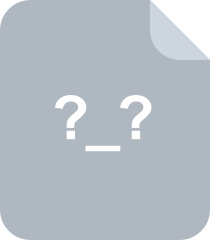
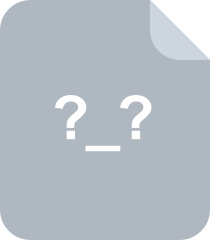
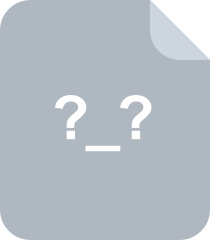
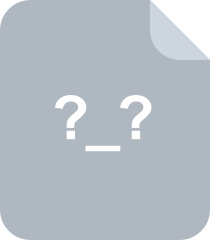
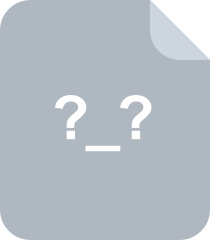
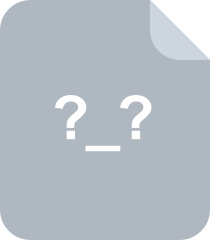
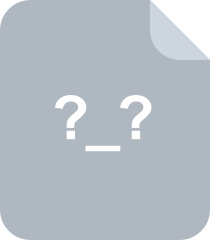
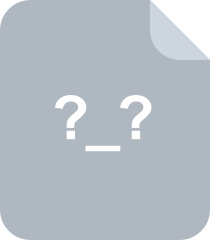
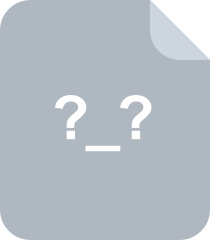
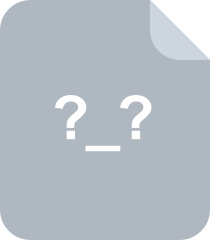
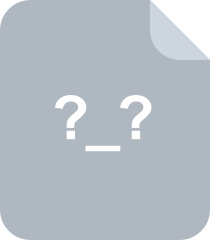
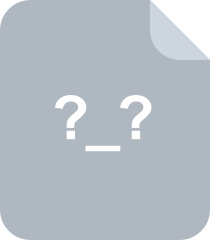
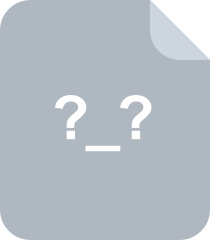
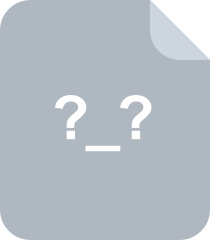
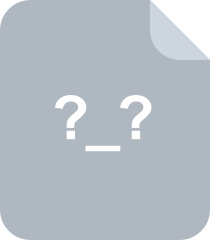
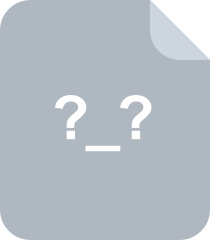
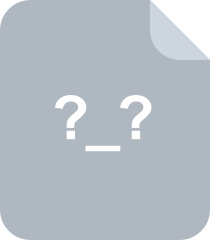
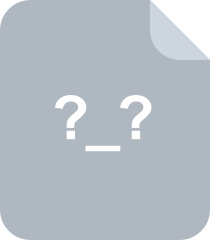
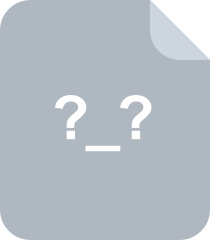
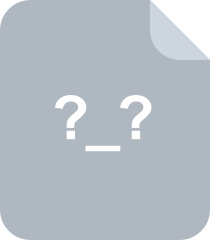
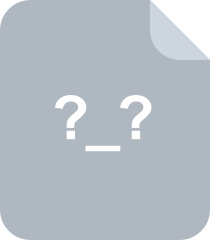
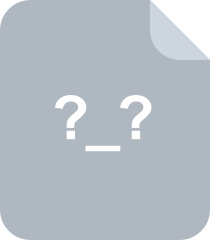
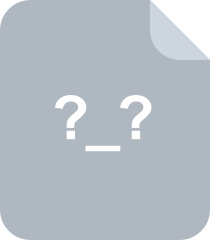
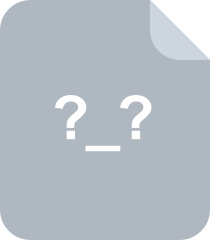
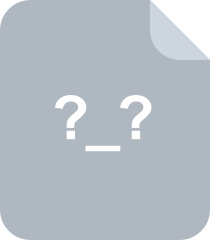
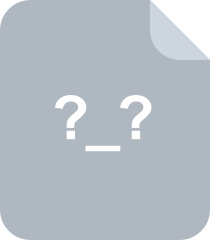
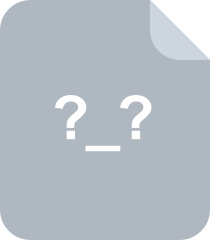
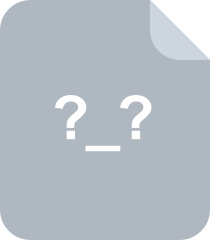
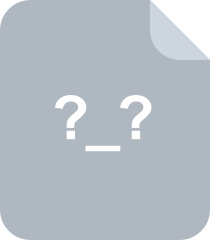
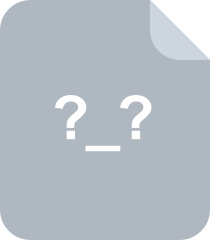
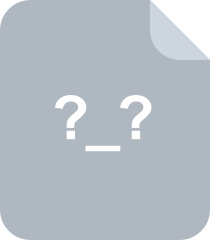
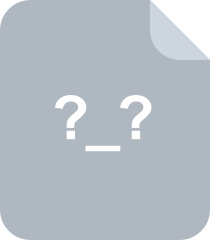
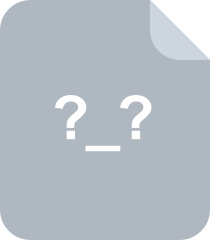
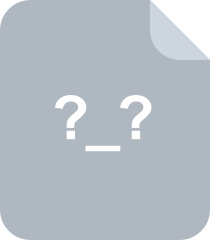
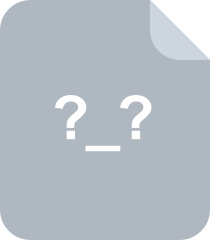
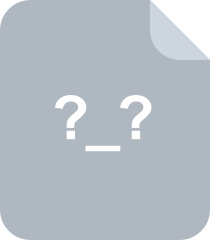
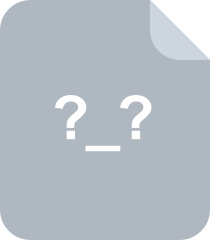
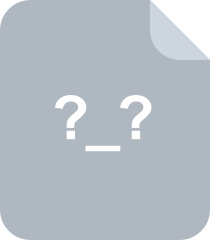
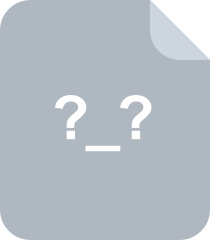
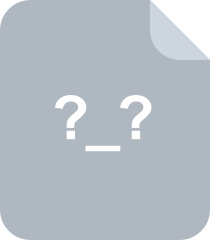
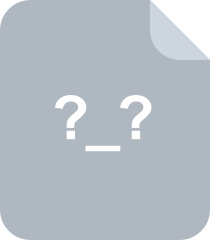
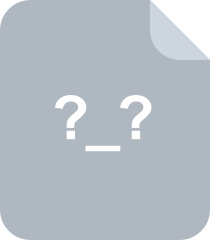
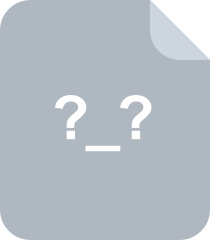
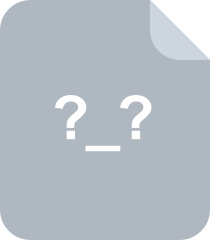
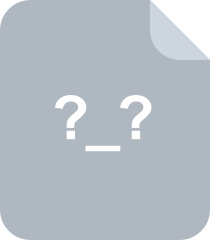
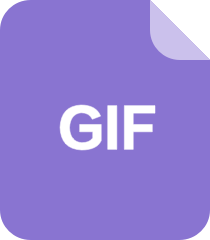
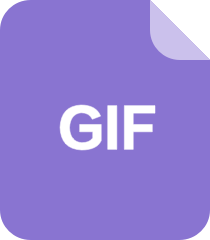
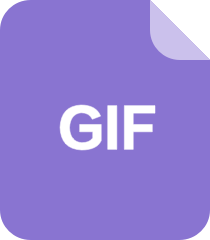
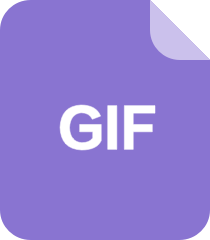
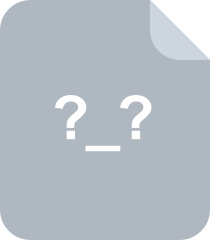
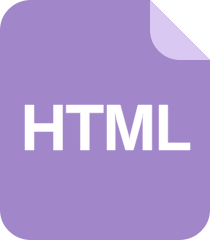
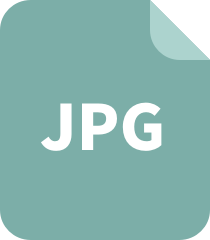
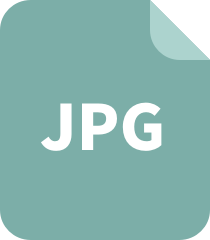
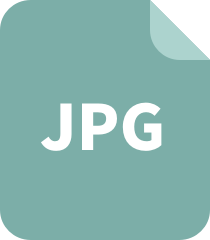
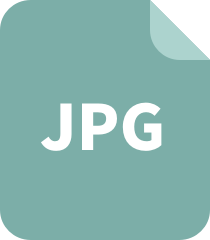
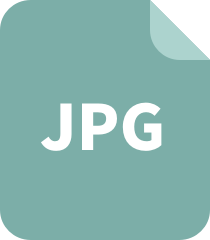
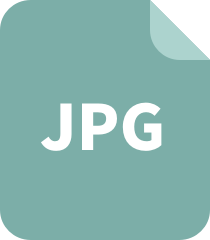
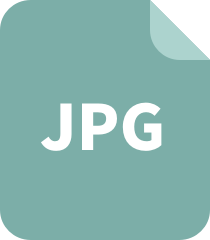
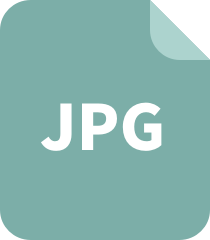
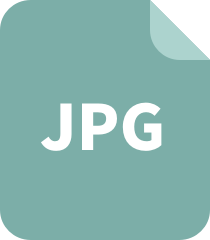
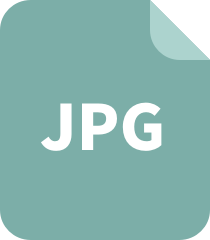
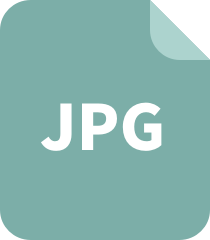
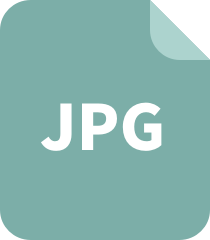
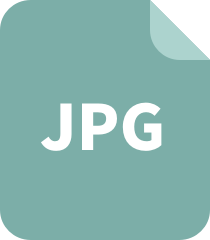
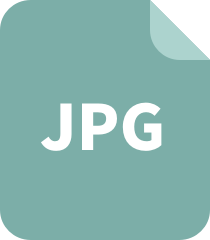
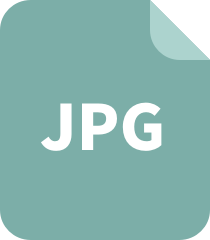
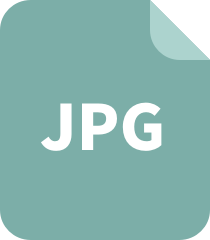
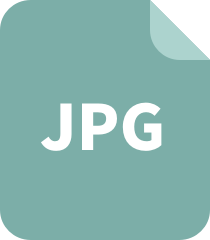
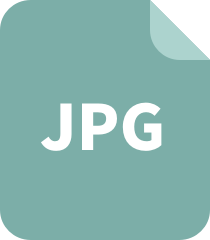
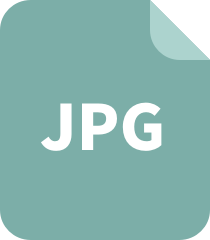
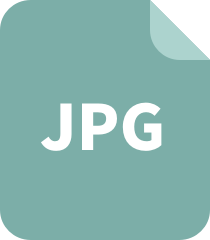
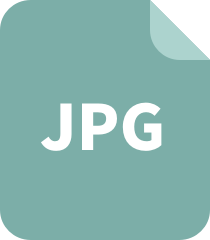
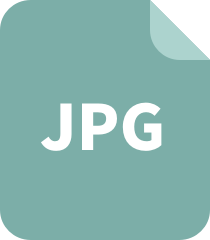
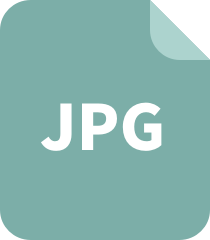
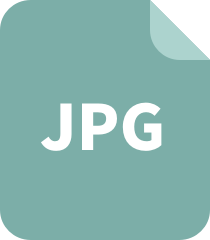
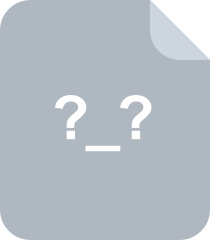
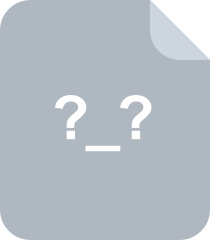
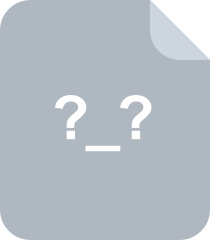
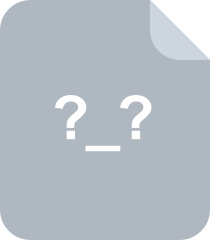
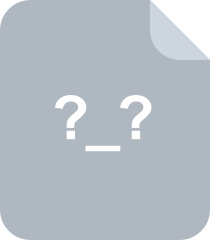
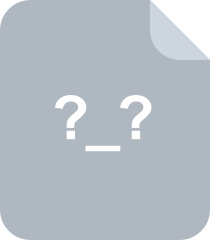
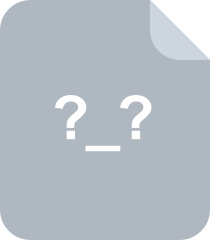
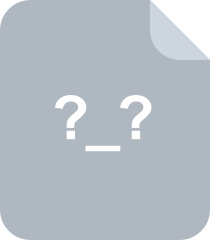
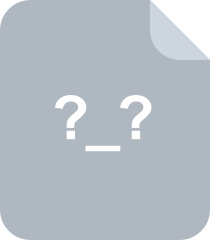
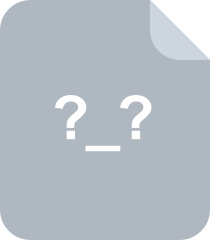
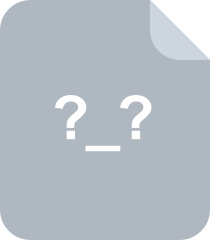
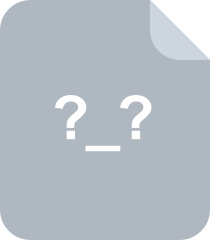
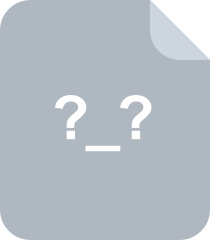
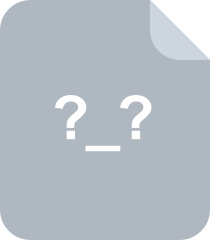
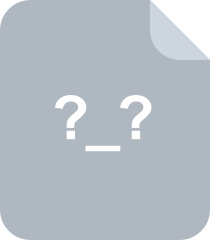
共 616 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
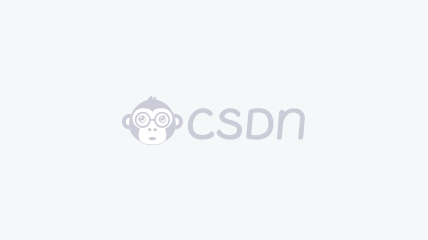

crmeb专业二开
- 粉丝: 732
- 资源: 180
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

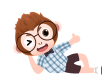
最新资源
- 基于语音控制的智能家居系统,实现使用android端来远程控制LED灯和收集温湿度传感器信息,图表展示温湿度走势全部资料+详细文档+优秀项目.zip
- 基于语音开放平台,包含技能开发、语音设备接入及智能家居接入的文档、SDK 及示例代码全部资料+详细文档+优秀项目.zip
- 基于智能家居板载程序全部资料+详细文档+优秀项目.zip
- 基于智能家居Android App全部资料+详细文档+优秀项目.zip
- 基于智能家居 、控制、物联网、摄像头、开关全部资料+详细文档+优秀项目.zip
- 基于智能家居管理系统全部资料+详细文档+优秀项目.zip
- 基于智能家居规则集构建全部资料+详细文档+优秀项目.zip
- 基于智能家居服务器全部资料+详细文档+优秀项目.zip
- 基于智能家居系统的移动终端,采用Qt编写,主要实现电能的监控和管理全部资料+详细文档+优秀项目.zip
- 基于智能家居物联网项目-enOcean全部资料+详细文档+优秀项目.zip
- 基于智能家居-万能遥控器全部资料+详细文档+优秀项目.zip
- 基于智能家居行为识别全部资料+详细文档+优秀项目.zip
- 基于智能家居远程监控系统全部资料+详细文档+优秀项目.zip
- 基于智能家居遥控器 Android端全部资料+详细文档+优秀项目.zip
- 基于智能家居在线全部资料+详细文档+优秀项目.zip
- 基于智能家居终端(可通过zigbee控制家中电器)全部资料+详细文档+优秀项目.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


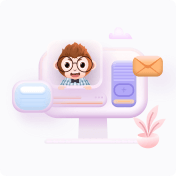
安全验证
文档复制为VIP权益,开通VIP直接复制
