---
title: File
description: Read/write files on the device.
---
<!--
# license: Licensed to the Apache Software Foundation (ASF) under one
# or more contributor license agreements. See the NOTICE file
# distributed with this work for additional information
# regarding copyright ownership. The ASF licenses this file
# to you under the Apache License, Version 2.0 (the
# "License"); you may not use this file except in compliance
# with the License. You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing,
# software distributed under the License is distributed on an
# "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
# KIND, either express or implied. See the License for the
# specific language governing permissions and limitations
# under the License.
-->
# cordova-plugin-file
[](https://github.com/apache/cordova-plugin-file/actions/workflows/android.yml) [](https://github.com/apache/cordova-plugin-file/actions/workflows/chrome.yml) [](https://github.com/apache/cordova-plugin-file/actions/workflows/ios.yml) [](https://github.com/apache/cordova-plugin-file/actions/workflows/lint.yml)
This plugin implements a File API allowing read/write access to files residing on the device.
This plugin is based on several specs, including :
The HTML5 File API
[http://www.w3.org/TR/FileAPI/](http://www.w3.org/TR/FileAPI/)
The Directories and System extensions
Latest:
[http://www.w3.org/TR/2012/WD-file-system-api-20120417/](http://www.w3.org/TR/2012/WD-file-system-api-20120417/)
Although most of the plugin code was written when an earlier spec was current:
[http://www.w3.org/TR/2011/WD-file-system-api-20110419/](http://www.w3.org/TR/2011/WD-file-system-api-20110419/)
It also implements the FileWriter spec :
[http://dev.w3.org/2009/dap/file-system/file-writer.html](http://dev.w3.org/2009/dap/file-system/file-writer.html)
>*Note* While the W3C FileSystem spec is deprecated for web browsers, the FileSystem APIs are supported in Cordova applications with this plugin for the platforms listed in the _Supported Platforms_ list, with the exception of the Browser platform.
To get a few ideas how to use the plugin, check out the [sample](#sample) at the bottom of this page. For additional examples (browser focused), see the HTML5 Rocks' [FileSystem article.](http://www.html5rocks.com/en/tutorials/file/filesystem/)
For an overview of other storage options, refer to Cordova's
[storage guide](http://cordova.apache.org/docs/en/latest/cordova/storage/storage.html).
This plugin defines a global `cordova.file` object.
Although the object is in the global scope, it is not available to applications until after the `deviceready` event fires.
document.addEventListener("deviceready", onDeviceReady, false);
function onDeviceReady() {
console.log(cordova.file);
}
## Installation
cordova plugin add cordova-plugin-file
## Supported Platforms
- Android
- iOS
- OS X
- Windows*
- Browser
\* _These platforms do not support `FileReader.readAsArrayBuffer` nor `FileWriter.write(blob)`._
## Where to Store Files
As of v1.2.0, URLs to important file-system directories are provided.
Each URL is in the form _file:///path/to/spot/_, and can be converted to a
`DirectoryEntry` using `window.resolveLocalFileSystemURL()`.
* `cordova.file.applicationDirectory` - Read-only directory where the application
is installed. (_iOS_, _Android_, _BlackBerry 10_, _OSX_, _windows_)
* `cordova.file.applicationStorageDirectory` - Root directory of the application's
sandbox; on iOS & windows this location is read-only (but specific subdirectories [like
`/Documents` on iOS or `/localState` on windows] are read-write). All data contained within
is private to the app. (_iOS_, _Android_, _BlackBerry 10_, _OSX_)
* `cordova.file.dataDirectory` - Persistent and private data storage within the
application's sandbox using internal memory (on Android, if you need to use
external memory, use `.externalDataDirectory`). On iOS, this directory is not
synced with iCloud (use `.syncedDataDirectory`). (_iOS_, _Android_, _BlackBerry 10_, _windows_)
* `cordova.file.cacheDirectory` - Directory for cached data files or any files
that your app can re-create easily. The OS may delete these files when the device
runs low on storage, nevertheless, apps should not rely on the OS to delete files
in here. (_iOS_, _Android_, _BlackBerry 10_, _OSX_, _windows_)
* `cordova.file.externalApplicationStorageDirectory` - Application space on
external storage. (_Android_)
* `cordova.file.externalDataDirectory` - Where to put app-specific data files on
external storage. (_Android_)
* `cordova.file.externalCacheDirectory` - Application cache on external storage.
(_Android_)
* `cordova.file.externalRootDirectory` - External storage (SD card) root. (_Android_, _BlackBerry 10_)
* `cordova.file.tempDirectory` - Temp directory that the OS can clear at will. Do not
rely on the OS to clear this directory; your app should always remove files as
applicable. (_iOS_, _OSX_, _windows_)
* `cordova.file.syncedDataDirectory` - Holds app-specific files that should be synced
(e.g. to iCloud). (_iOS_, _windows_)
* `cordova.file.documentsDirectory` - Files private to the app, but that are meaningful
to other application (e.g. Office files). Note that for _OSX_ this is the user's `~/Documents` directory. (_iOS_, _OSX_)
* `cordova.file.sharedDirectory` - Files globally available to all applications (_BlackBerry 10_)
## File System Layouts
Although technically an implementation detail, it can be very useful to know how
the `cordova.file.*` properties map to physical paths on a real device.
### iOS File System Layout
| Device Path | `cordova.file.*` | `iosExtraFileSystems` | r/w? | persistent? | OS clears | sync | private |
|:-----------------------------------------------|:----------------------------|:----------------------|:----:|:-----------:|:---------:|:----:|:-------:|
| `/var/mobile/Applications/<UUID>/` | applicationStorageDirectory | - | r | N/A | N/A | N/A | Yes |
| `appname.app/` | applicationDirectory | bundle | r | N/A | N/A | N/A | Yes |
| `www/` | - | - | r | N/A | N/A | N/A | Yes |
| `Documents/` | documentsDirectory | documents | r/w | Yes | No | Yes | Yes |
| `NoCloud/` | - | documents-nosync | r/w | Yes | No | No | Yes |
| `Library` | - | library | r/w | Yes | No | Yes? | Yes |
| `NoCloud/` | dataDirectory | library-nosync | r/w | Yes | No | No | Yes |
| `Cloud/` | syncedDataDirectory | - | r/w | Yes | No | Yes | Yes |
| `Caches/` | cacheDirectory | cache | r/w | Yes* | Yes\*\*\*| No | Yes |
| &n
没有合适的资源?快使用搜索试试~ 我知道了~
将justep的X5 Androidsdk 升级到30 备忘
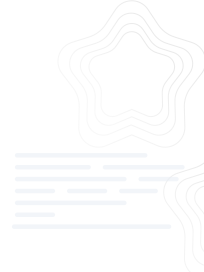
共8271个文件
js:2431个
class:967个
dex:731个

0 下载量 132 浏览量
2023-07-05
18:21:35
上传
评论
收藏 117.67MB ZIP 举报
温馨提示
X5 Android sdk升级
资源推荐
资源详情
资源评论
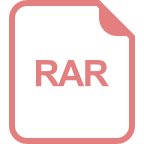
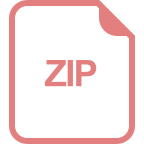
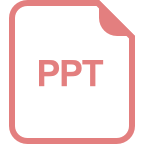
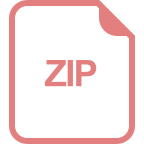
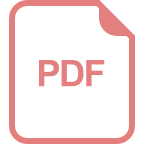
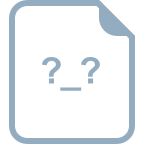
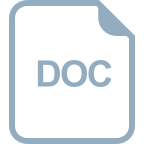
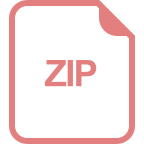
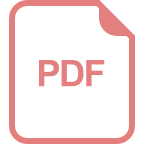
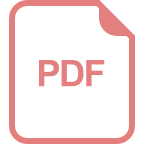
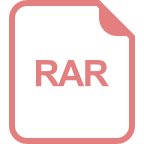
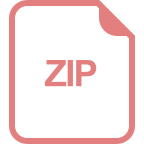
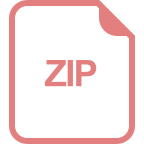
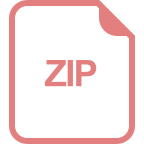
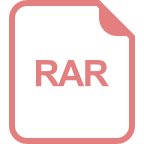
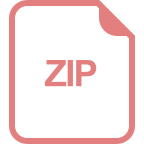
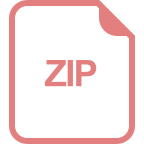
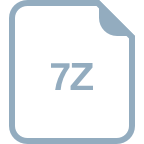
收起资源包目录

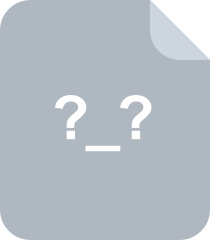
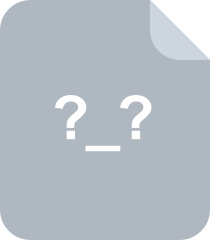
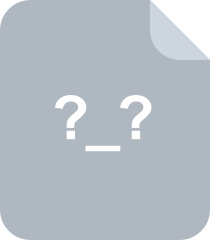
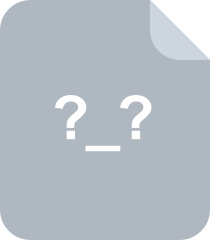
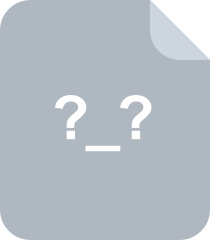
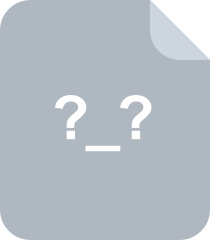
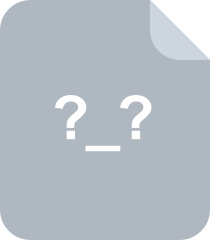
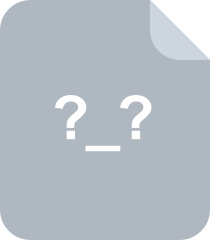
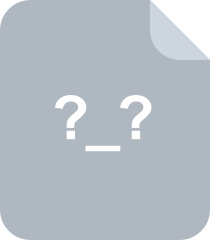
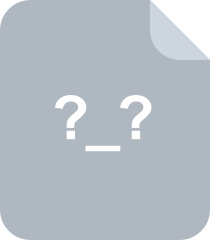
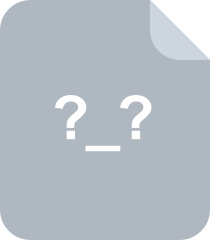
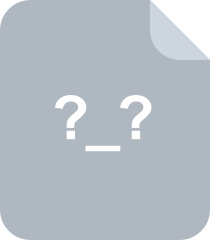
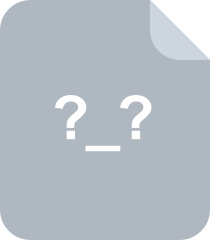
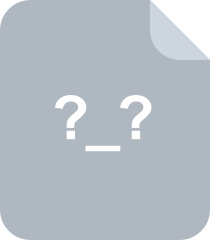
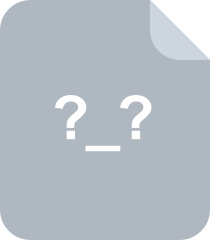
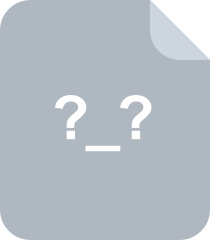
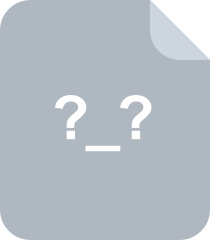
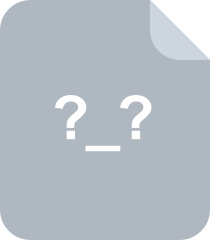
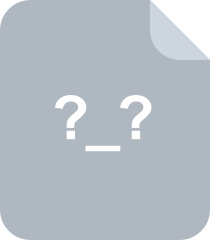
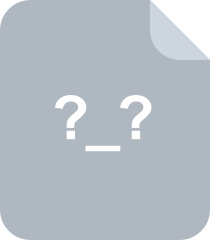
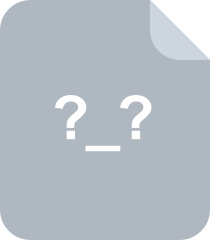
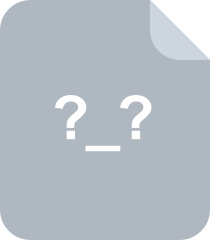
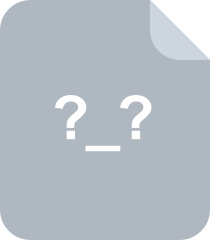
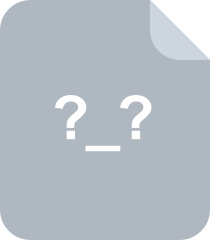
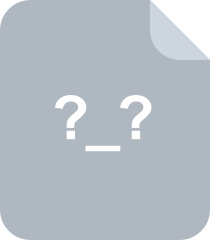
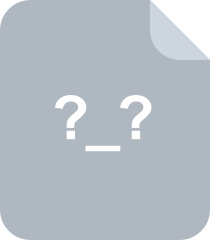
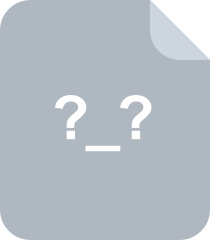
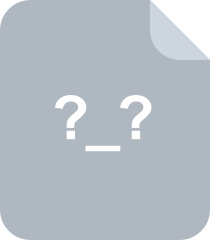
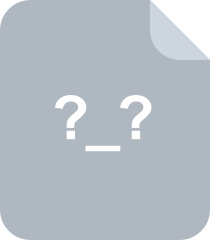
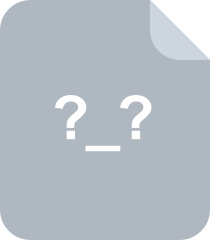
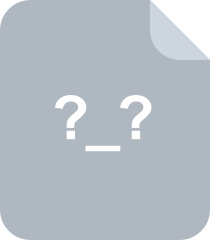
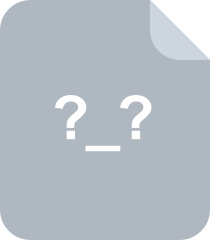
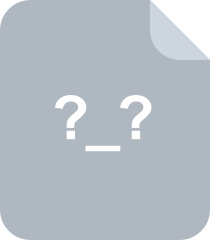
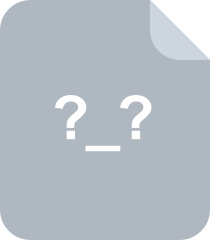
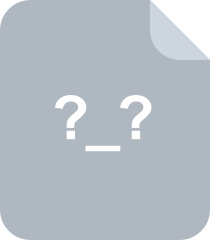
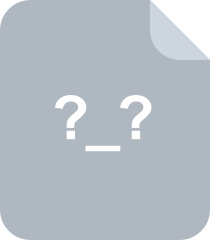
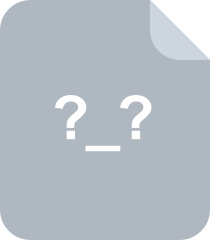
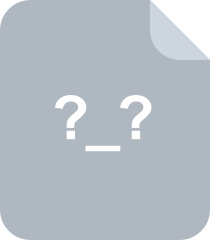
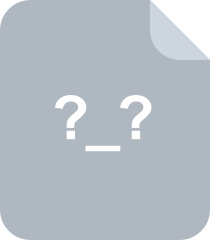
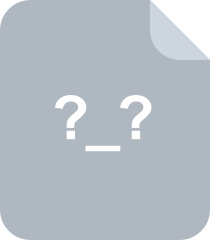
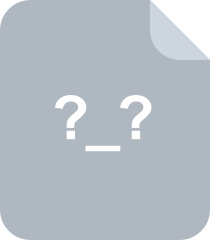
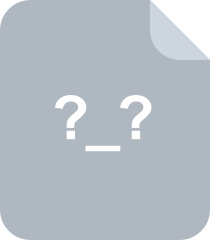
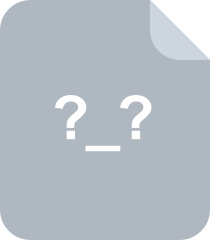
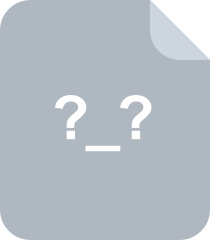
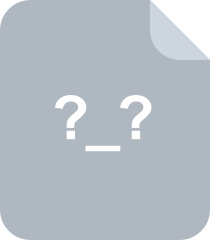
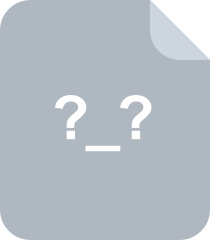
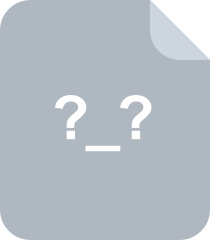
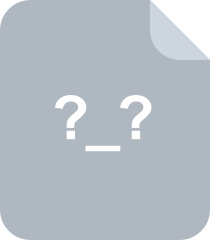
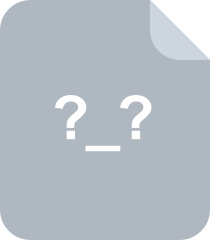
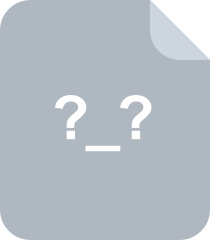
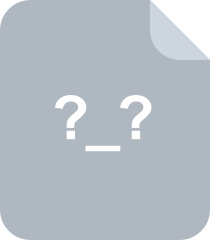
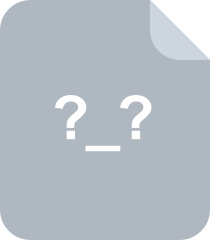
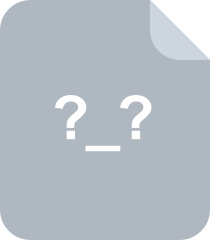
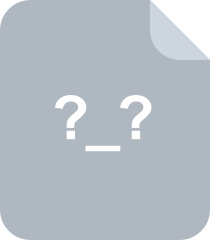
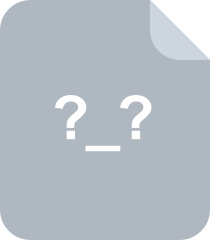
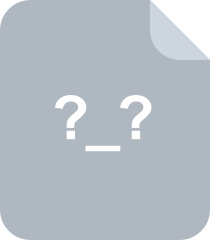
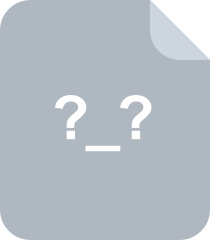
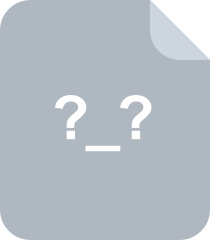
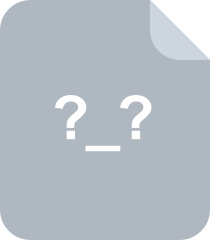
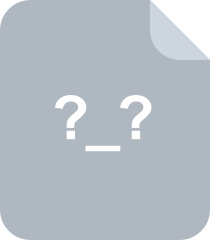
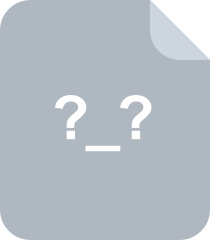
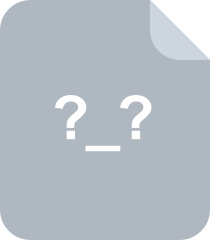
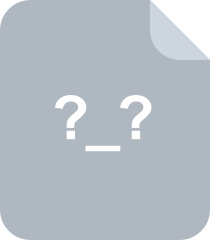
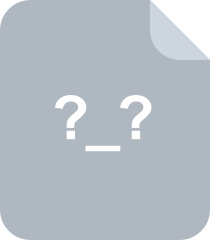
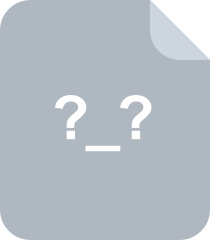
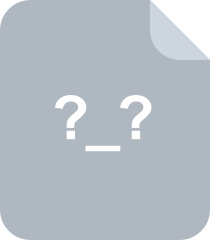
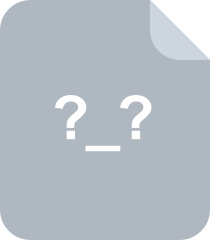
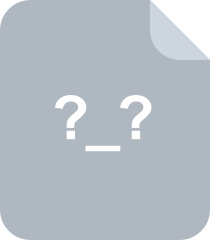
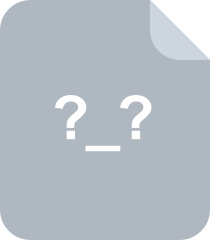
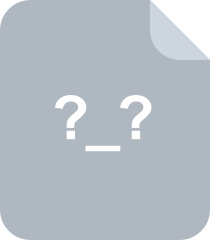
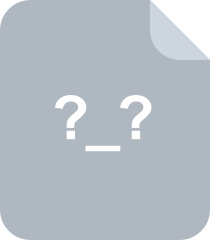
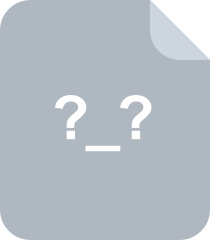
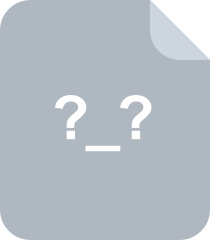
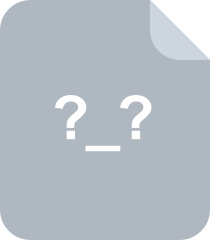
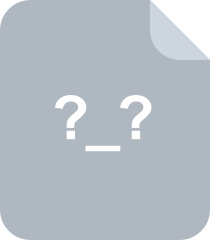
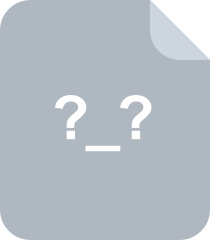
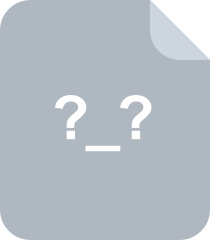
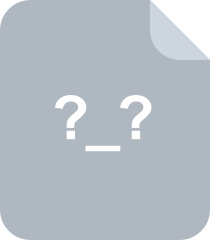
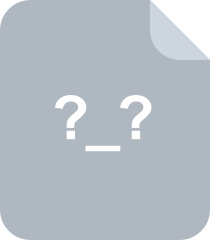
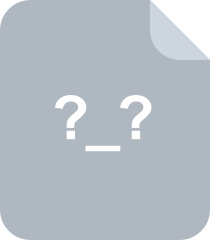
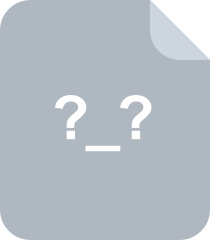
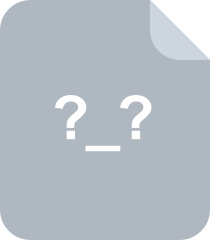
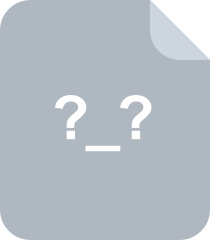
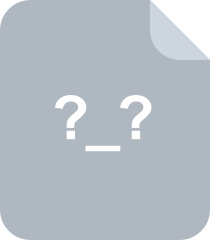
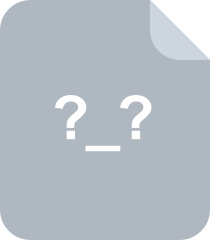
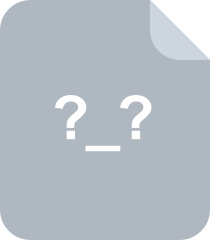
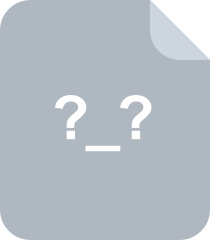
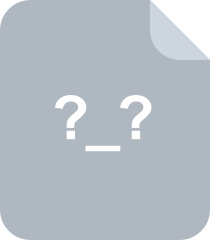
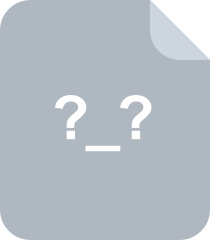
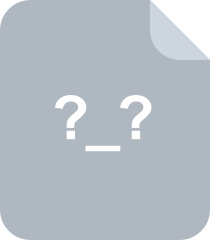
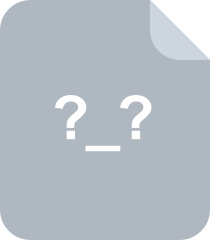
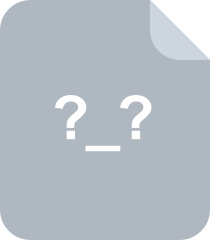
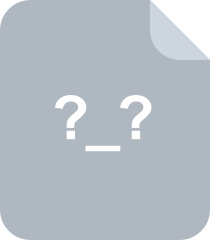
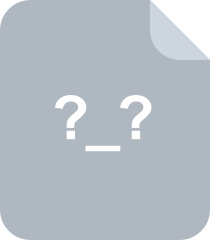
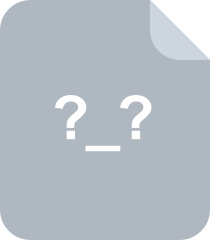
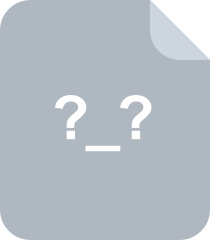
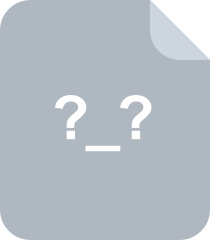
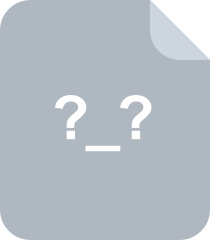
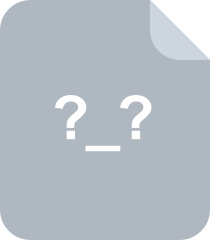
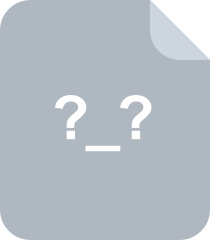
共 8271 条
- 1
- 2
- 3
- 4
- 5
- 6
- 83
资源评论
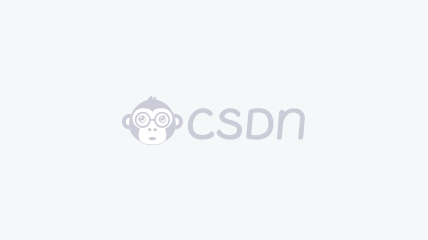

jia_dojo
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

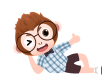
最新资源
- #P0015. 全排列 超级简单
- pta题库答案c语言之排序4统计工龄.zip
- pta题库答案c语言之树结构7堆中的路径.zip
- pta题库答案c语言之树结构3TreeTraversalsAgain.zip
- pta题库答案c语言之树结构2ListLeaves.zip
- pta题库答案c语言之树结构1树的同构.zip
- 基于C++实现民航飞行与地图简易管理系统可执行程序+说明+详细注释.zip
- pta题库答案c语言之复杂度1最大子列和问题.zip
- 三维装箱问题(Three-Dimensional Bin Packing Problem,3D-BPP)是一个经典的组合优化问题
- 以下是一些关于Linux线程同步的基本概念和方法.txt
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


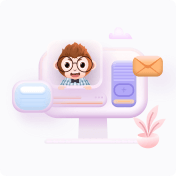
安全验证
文档复制为VIP权益,开通VIP直接复制
