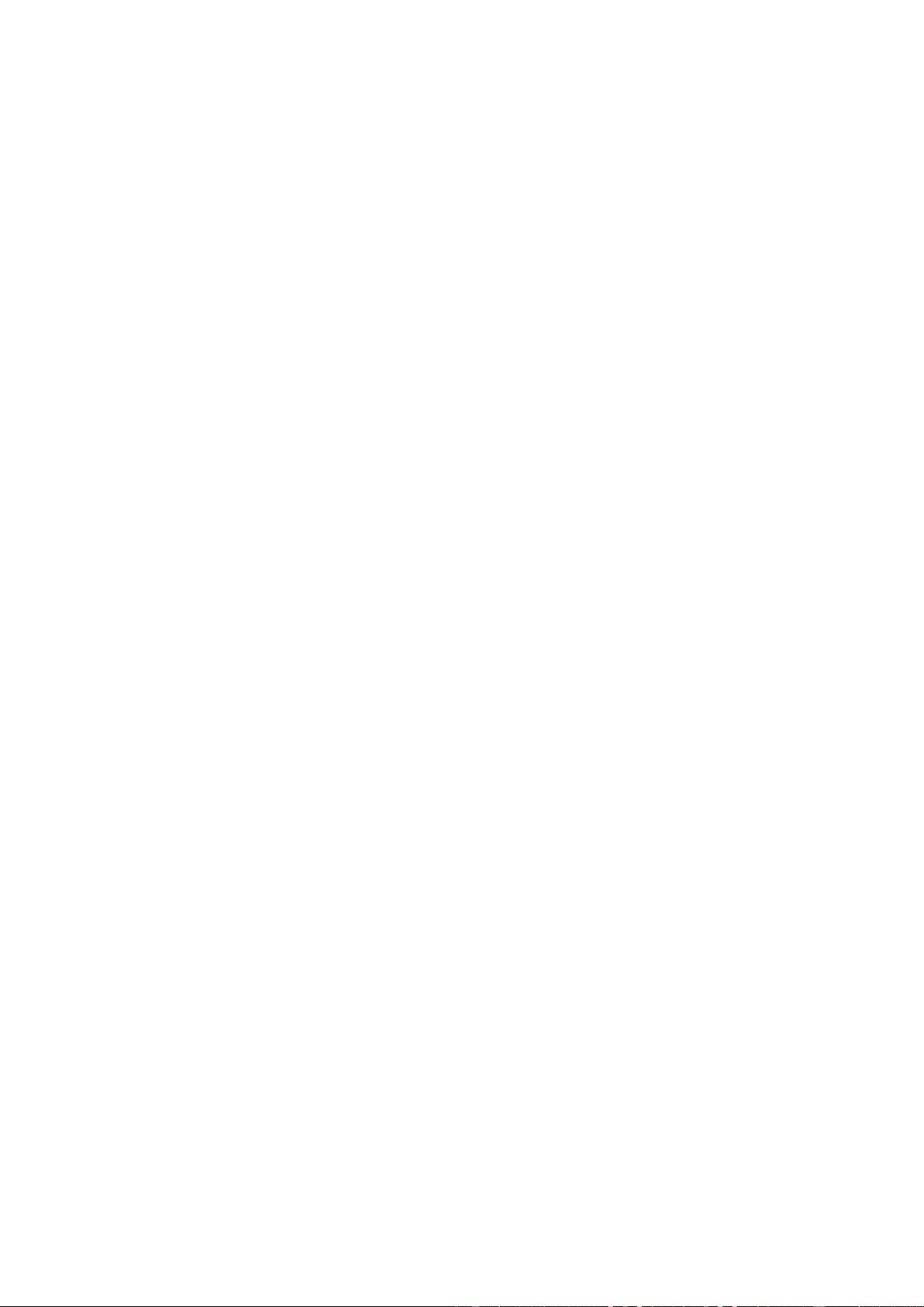
c o n t e n t s
Preface ...................................................................................... 1
Part 1—Overview....................................................................... 2
file structure.................................................................................................. 2
simple program ........................................................................................... 2
a few details................................................................................................. 3
hands-on—enter the program on page 2................................... 4
part 2—Language Elements ...................................................... 5
functions ....................................................................................................... 5
types.............................................................................................................. 6
statements.................................................................................................... 7
hands-on—a larger program...................................................... 9
part 3—Style and Idioms ........................................................... 10
switch ............................................................................................................ 10
loops.............................................................................................................. 10
arguments .................................................................................................... 10
pointers and returning values................................................................... 11
arrays, pointer arithmetic and array arguments .................................... 11
hands-on—sorting strings.......................................................... 13
part 4—Structured Types........................................................... 14
struct.............................................................................................................. 14
typedef .......................................................................................................... 14
putting it together: array of struct.............................................................. 15
hands-on—sorting employee records........................................ 17
part 5—Advanced Topics........................................................... 18
preprocessor................................................................................................ 18
function pointers.......................................................................................... 19
traps and pitfalls.......................................................................................... 20
hands-on—generalizing a sort .................................................. 21
part 6—Programming in the Large............................................. 22
file structure revisited ................................................................................. 22
maintainability............................................................................................. 22
portability...................................................................................................... 22
hiding the risky parts .................................................................................. 23
performance vs. maintainability ............................................................... 23
hands-on—porting a program from Unix.................................... 25
part 7—Object-Oriented Design................................................. 26
identifying objects....................................................................................... 26
object relationships .................................................................................... 26
entities vs. actions....................................................................................... 27
example: event-driven program............................................................... 27
design task—simple event-driven user interface....................... 28
part 8—OOD and C.................................................................... 29
language elements..................................................................................... 29
example........................................................................................................ 29
hands-on—implementation........................................................ 32
part 9—Object-Oriented Design and C++.................................. 33
OOD summary............................................................................................. 33
objects in C++.............................................................................................. 33
stream I/O ..................................................................................................... 35
differences from C....................................................................................... 35
hands-on—simple example ....................................................... 37
part 10—Classes in More Detail................................................ 38