Java爬虫是编程领域中一个重要的实践技术,主要用于自动化地从互联网上收集和处理数据,进行数据挖掘。在这个“java爬虫完整代码”压缩包中,我们预计会找到一系列用Java语言编写的爬虫程序,可能包括核心的网络请求、HTML解析、数据提取等模块。以下是对这些知识点的详细解释: 1. **Java编程基础**:Java是一种广泛使用的面向对象的编程语言,具有跨平台性,适合开发大型和小型项目。在爬虫开发中,Java提供了丰富的库和工具,如HttpClient用于网络请求,Jsoup或JsoupParser进行HTML解析。 2. **网络请求**:爬虫首先需要发送HTTP或HTTPS请求到目标网站获取网页内容。Java中的HttpURLConnection或者Apache HttpClient库可以实现这个功能。它们允许设置各种请求头,如User-Agent,处理cookies,甚至模拟登录。 3. **HTML解析**:接收到网页内容后,需要解析HTML来提取所需数据。Jsoup是一个强大的库,能够解析HTML并提供DOM操作接口,便于查找和提取特定元素。通过CSS选择器,XPath等方法可以高效定位数据。 4. **数据提取**:在HTML解析后,数据通常嵌入在标签属性或文本中。Jsoup提供了便利的方法,如`.text()`获取元素文本,`.attr()`获取属性值,可以方便地提取出数据。 5. **异步处理**:对于大量页面的爬取,可以使用Java的并发和多线程技术,如ExecutorService,以提高爬取速度。异步请求库如AsyncHttpClient也可以实现非阻塞I/O,进一步提升效率。 6. **数据存储**:爬取到的数据通常需要存储,可能涉及数据库(如MySQL,MongoDB)或者文件系统(CSV,JSON)。Java的JDBC接口用于连接数据库,而Jackson或Gson库可以方便地处理JSON数据。 7. **反爬机制应对**:许多网站有反爬策略,如验证码、IP限制和User-Agent检查。Java爬虫可能需要实现验证码识别(如OCR),使用代理IP池,以及定期更换User-Agent来应对这些挑战。 8. **数据挖掘**:爬取到的数据经过清洗和预处理后,可以进行数据分析和挖掘。Java提供了诸如Weka这样的机器学习库,以及Apache Mahout,可以进行分类、聚类、关联规则等分析。 9. **异常处理和日志记录**:在爬虫运行过程中,错误处理和日志记录至关重要。使用try-catch语句块捕获异常,结合Log4j或SLF4J记录运行状态和错误信息,有助于调试和优化。 10. **持续集成与自动化**:将爬虫项目整合到持续集成工具如Jenkins中,可以自动化构建、测试和部署,确保代码质量和稳定性。 这个压缩包“java爬虫完整代码”可能包含了实现上述所有步骤的Java代码,对于学习和实践Java爬虫技术来说,是一个宝贵的学习资源。通过深入理解和运用这些知识点,可以创建出高效、稳定的网络爬虫,用于数据挖掘和分析。
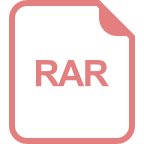
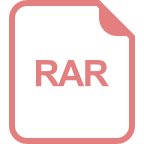
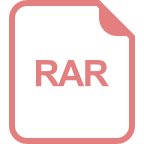
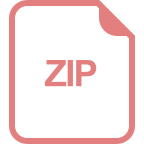
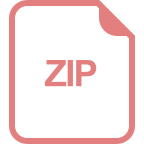
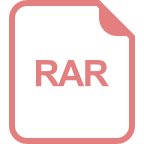
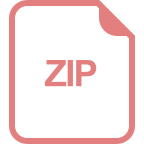
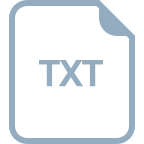
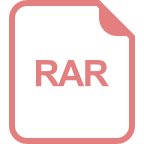




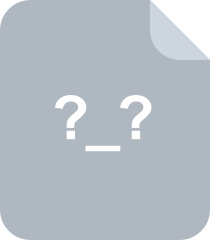

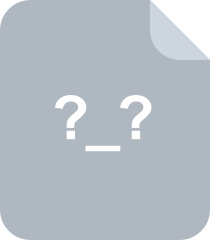

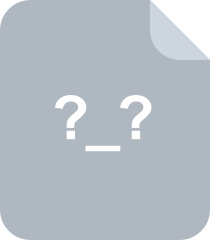
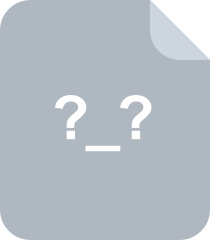

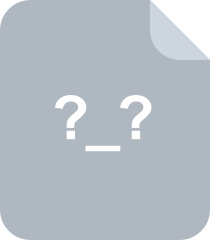


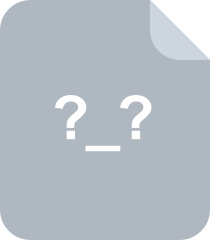

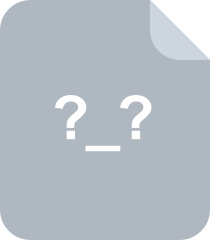

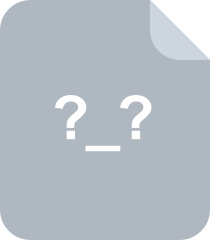
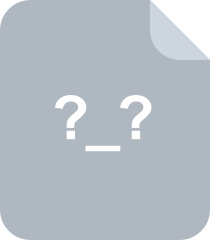
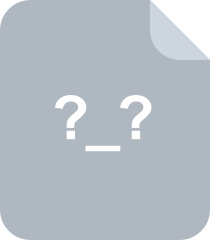
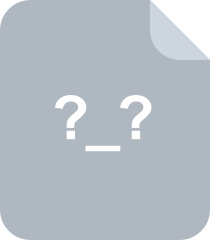
- 1

- 粉丝: 960
- 资源: 4
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

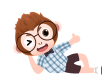
最新资源
- 基于lua-nginx-module,可以多维度检查和拦截恶意网络请求,具有简单易用、高性能、轻量级的特点
- 一个基于qt开发的包含各种基础图像处理技术的桌面应用,图像处理算法基于halcon,有直接调用halcon脚本和执行halcon
- 【带个人免签支付】宝宝取名源码 易经在线起名网 周易新生儿取名 生辰八字取名系统
- 微信公众号批量下载工具
- 创维8A06机芯 E750A系列 通用主程序 电视刷机 固件升级包 Ver01.01
- LxRunOffline-v3.5.0-11-gfdab71a-msvc.zip
- 惠普Laser Jet Professional P1100(系列)打印机驱动下载
- C#毕业设计基于leap motion和CNN的手语识别系统源代码+数据集+项目文档+演示视频
- 绑定halcon显示控件,可实现ROI交互,用于机器视觉领域.zip
- java连接数据库,jdbc连接数据库,并实现在控制台显示输入书名查询书本

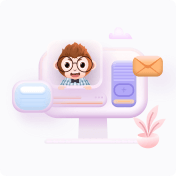

- 1
- 2
- 3
- 4
前往页