package com.weather.service.impl;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.weather.service.WeatherDataService;
import com.weather.vo.WeatherResponse;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
@Slf4j
@Service
public class WeatherDataServiceImpl implements WeatherDataService {
@Autowired
private RestTemplate restTemplate;
@Autowired
private StringRedisTemplate redisTemplate;
private static final long TIME_OUT = 1800L; //redis超时时间为30分钟
private static final String WEATHER_URI = "http://wthrcdn.etouch.cn/weather_mini?";
@Override
public WeatherResponse getDataByCityId(final String cityId) {
String uri = WEATHER_URI + "citykey=" + cityId;
return this.doGetWeather(uri);
}
@Override
public WeatherResponse getDataByCityName(final String cityName) {
String uri = WEATHER_URI + "city=" + cityName;
return this.doGetWeather(uri);
}
private WeatherResponse doGetWeather(String uri) {
ObjectMapper mapper = new ObjectMapper();
WeatherResponse resp = null;
String strBody = null;
// 先从缓存中查询数据
if (redisTemplate.hasKey(uri)) {
strBody = redisTemplate.opsForValue().get(uri);
} else {
// 缓存中没有就调用接口查询天气数据
ResponseEntity<String> respString = restTemplate.getForEntity(uri, String.class);
if (respString.getStatusCodeValue() == 200) {
strBody = respString.getBody();
}
// 把查询出来的数据写入缓存中
redisTemplate.opsForValue().set(uri,strBody,TIME_OUT,TimeUnit.SECONDS);
}
try {
resp = mapper.readValue(strBody, WeatherResponse.class);
} catch (IOException e) {
//e.printStackTrace();
log.error("Error!",e);
}
return resp;
}
/**
* 根据城市id来同步天气数据
*/
@Override
public void syncDataByCityId(String cityId) {
String uri = WEATHER_URI + "citykey=" + cityId;
this.saveWeatherDate(uri);
}
/**
* 把天气数据放入缓存中
*/
private void saveWeatherDate(String uri) {
String strBody = null;
ResponseEntity<String> respString = restTemplate.getForEntity(uri, String.class);
if (respString.getStatusCodeValue() == 200) {
strBody = respString.getBody();
}
// 把查询出来的数据写入缓存中
redisTemplate.opsForValue().set(uri,strBody,TIME_OUT,TimeUnit.SECONDS);
}
}

c++服务器开发
- 粉丝: 3185
- 资源: 4461
最新资源
- DeepSeek多模态接口开发指南:图文混合处理在自动驾驶中的应用.pdf
- DeepSeek接口版本管理实战:灰度发布与回滚机制设计指南.pdf
- DeepSeek多模态实战:文本生成+知识图谱构建的完整Pipeline设计.pdf
- DeepSeek三大API全解析:搜索、生成、语义分析接口的底层逻辑与参数调优.pdf
- DeepSeek三大核心能力实战:文本生成、语义搜索、智能对话开发指南.pdf
- DeepSeek三大核心API实战:搜索、生成与语义分析的最佳实践指南.pdf
- DeepSeek微调实战:用自有数据训练行业专属模型的完整流程.pdf
- DeepSeek与GPT-4接口对比评测:十分之一成本实现代码生成.pdf
- DeepSeek文本生成API的7大隐藏参数:控制temperature与top_p的进阶技巧.pdf
- DeepSeek长文本处理秘籍:突破128K上下文窗口的3种工程方案.pdf
- DualPipe算法实战:5倍加速DeepSeekAPI响应的底层逻辑.pdf
- FP8混合精度训练揭秘:DeepSeek如何平衡速度与精度?.pdf
- IDEA神器CodeGPT:DeepSeek模型无缝接入与私有化部署指南.pdf
- Markdown空行陷阱:代码生成接口调试实录.pdf
- MLA注意力机制源码解析:DeepSeek如何实现多层级语义捕捉?.pdf
- Postman调试DeepSeekAPI全流程:从鉴权到流式响应的进阶技巧.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


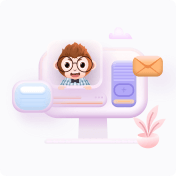