# jsGrid Lightweight Grid jQuery Plugin
[](https://travis-ci.org/tabalinas/jsgrid)
Project site [js-grid.com](http://js-grid.com/)
**jsGrid** is a lightweight client-side data grid control based on jQuery.
It supports basic grid operations like inserting, filtering, editing, deleting, paging, sorting, and validating.
jsGrid is tunable and allows to customize appearance and components.

## Table of contents
* [Demos](#demos)
* [Installation](#installation)
* [Basic Usage](#basic-usage)
* [Configuration](#configuration)
* [Grid Fields](#grid-fields)
* [Methods](#methods)
* [Callbacks](#callbacks)
* [Grid Controller](#grid-controller)
* [Validation](#validation)
* [Localization](#localization)
* [Sorting Strategies](#sorting-strategies)
* [Load Strategies](#load-strategies)
* [Load Indication](#load-indication)
* [Requirement](#requirement)
* [Compatibility](#compatibility)
## Demos
See [Demos](http://js-grid.com/demos/) on project site.
Sample projects showing how to use jsGrid with the most popular backend technologies
* **PHP** - https://github.com/tabalinas/jsgrid-php
* **ASP.NET WebAPI** - https://github.com/tabalinas/jsgrid-webapi
* **Express (NodeJS)** - https://github.com/tabalinas/jsgrid-express
* **Ruby on Rail** - https://github.com/tabalinas/jsgrid-rails
* **Django (Python)** - https://github.com/tabalinas/jsgrid-django
## Installation
Install jsgrid with bower:
```bash
$ bower install js-grid --save
```
Find jsGrid cdn links [here](https://cdnjs.com/libraries/jsgrid).
## Basic Usage
Ensure that jQuery library of version 1.8.3 or later is included.
Include `jsgrid.min.js`, `jsgrid-theme.min.css`, and `jsgrid.min.css` files into the web page.
Create grid applying jQuery plugin `jsGrid` with grid config as follows:
```javascript
$("#jsGrid").jsGrid({
width: "100%",
height: "400px",
filtering: true,
editing: true,
sorting: true,
paging: true,
data: db.clients,
fields: [
{ name: "Name", type: "text", width: 150 },
{ name: "Age", type: "number", width: 50 },
{ name: "Address", type: "text", width: 200 },
{ name: "Country", type: "select", items: db.countries, valueField: "Id", textField: "Name" },
{ name: "Married", type: "checkbox", title: "Is Married", sorting: false },
{ type: "control" }
]
});
```
## Configuration
The config object may contain following options (default values are specified below):
```javascript
{
fields: [],
data: [],
autoload: false,
controller: {
loadData: $.noop,
insertItem: $.noop,
updateItem: $.noop,
deleteItem: $.noop
},
width: "auto",
height: "auto",
heading: true,
filtering: false,
inserting: false,
editing: false,
selecting: true,
sorting: false,
paging: false,
pageLoading: false,
rowClass: function(item, itemIndex) { ... },
rowClick: function(args) { ... },
rowDoubleClick: function(args) { ... },
noDataContent: "Not found",
confirmDeleting: true,
deleteConfirm: "Are you sure?",
pagerContainer: null,
pageIndex: 1,
pageSize: 20,
pageButtonCount: 15,
pagerFormat: "Pages: {first} {prev} {pages} {next} {last} {pageIndex} of {pageCount}",
pagePrevText: "Prev",
pageNextText: "Next",
pageFirstText: "First",
pageLastText: "Last",
pageNavigatorNextText: "...",
pageNavigatorPrevText: "...",
invalidNotify: function(args) { ... }
invalidMessage: "Invalid data entered!",
loadIndication: true,
loadIndicationDelay: 500,
loadMessage: "Please, wait...",
loadShading: true,
loadIndicator: function(config) { ... }
loadStrategy: function(config) { ... }
updateOnResize: true,
rowRenderer: null,
headerRowRenderer: null,
filterRowRenderer: null,
insertRowRenderer: null,
editRowRenderer: null,
pagerRenderer: null
}
```
### fields
An array of fields (columns) of the grid.
Each field has general options and specific options depending on field type.
General options peculiar to all field types:
```javascript
{
type: "",
name: "",
title: "",
align: "",
width: 100,
visible: true,
css: "",
headercss: "",
filtercss: "",
insertcss: "",
editcss: "",
filtering: true,
inserting: true,
editing: true,
sorting: true,
sorter: "string",
headerTemplate: function() { ... },
itemTemplate: function(value, item) { ... },
filterTemplate: function() { ... },
insertTemplate: function() { ... },
editTemplate: function(value, item) { ... },
filterValue: function() { ... },
insertValue: function() { ... },
editValue: function() { ... },
cellRenderer: null,
validate: null
}
```
- **type** is a string key of field (`"text"|"number"|"checkbox"|"select"|"textarea"|"control"`) in fields registry `jsGrid.fields` (the registry can be easily extended with custom field types).
- **name** is a property of data item associated with the column.
- **title** is a text to be displayed in the header of the column. If `title` is not specified, the `name` will be used instead.
- **align** is alignment of text in the cell. Accepts following values `"left"|"center"|"right"`.
- **width** is a width of the column.
- **visible** is a boolean specifying whether to show a column or not. (version added: 1.3)
- **css** is a string representing css classes to be attached to the table cell.
- **headercss** is a string representing css classes to be attached to the table header cell. If not specified, then **css** is attached instead.
- **filtercss** is a string representing css classes to be attached to the table filter row cell. If not specified, then **css** is attached instead.
- **insertcss** is a string representing css classes to be attached to the table insert row cell. If not specified, then **css** is attached instead.
- **editcss** is a string representing css classes to be attached to the table edit row cell. If not specified, then **css** is attached instead.
- **filtering** is a boolean specifying whether or not column has filtering (`filterTemplate()` is rendered and `filterValue()` is included in load filter object).
- **inserting** is a boolean specifying whether or not column has inserting (`insertTemplate()` is rendered and `insertValue()` is included in inserting item).
- **editing** is a boolean specifying whether or not column has editing (`editTemplate()` is rendered and `editValue()` is included in editing item).
- **sorting** is a boolean specifying whether or not column has sorting ability.
- **sorter** is a string or a function specifying how to sort item by the field. The string is a key of sorting strategy in the registry `jsGrid.sortStrategies` (the registry can be easily extended with custom sorting functions). Sorting function has the signature `function(value1, value2) { return -1|0|1; }`.
- **headerTemplate** is a function to create column header content. It should return markup as string, DomNode or jQueryElement.
- **itemTemplate** is a function to create cell content. It should return markup as string, DomNode or jQueryElement. The function signature is `function(value, item)`, where `value` is a value of column property of data item, and `item` is a row data item.
- **filterTemplate** is a function to create filter row cell content. It should return markup as string, DomNode or jQueryElement.
- **insertTemplate** is a function to create insert row cell content. It should return markup as string, DomNode or jQueryElement.
- **editTemplate** is a function to create cell content of editing row. It should return markup as string, DomNode or jQueryElement.

jane9872
- 粉丝: 109
- 资源: 7797
最新资源
- 基于MPC的永磁同步电机非线性终端滑模控制仿真研究 matlab simulink 无参考文件
- 本科生课程设计封面.doc
- 基于动物群体行为优化的多椭圆检测算法及其在图像处理的应用
- 适用方向:基于LQR控制算法的直接横摆力矩控制(DYC)的四轮独立电驱动汽车的横向稳定性控制研究 主要内容:利用carsim建模,在simulink中搭建控制器,然后进行联合 实现汽车在高速低附着路
- 永磁同步电机模型预测电流控制Simulink仿真,单矢量控制,带一份报告介绍
- Sim-EKB-Install-2024-12-08
- 跟网型逆变器小干扰稳定性分析与控制策略优化simulink仿真模型和代码 现代逆变技术 阻抗重塑 双锁相环 可附赠参考文献(英文) 和一份与模型完全对应的中文版报告
- 冲压废料收集装置sw18可编辑全套技术资料100%好用.zip
- 【西门子1500吉利(柯马)汽车SICAR项目程序源码】西门子PLC&HMI整套设计资料(源码+注释) 西门子1500 PLC, TP1200触摸屏HMI 非常标准的汽车行业程序(SICAR),修改套
- C++ 基于opencv 4.5 仿halcon 基于形状的模板匹配 ,支持目标缩放以及旋转,支持亚像素精度,源码,支持C#
- 深度学习技术中混沌时间序列预测-基于LSTM、Transformer与CNN的多专家混合模型应用-含详细代码及解释
- 双馈风机惯性控制+下垂控制参与系统一次调频的Matlab Simulink模型,调频结束后转速回复,造成频率二次跌落 系统为三机九节点模型,所有参数已调好且可调,可直接运行,风电渗透率19.4% 风机
- iOS 7.0 ~ 16.7 DeviceSupport.zip
- 软件工程期末复习总结.xmind
- Kriging代理模型 克里金模型 回归预测 根据样本数据建立代理模型,进行预测 Matlab编程
- 高速永磁同步电机的电磁设计 高速永磁电机的体积远小于同等功率的中低速电机,且功率密度高,近年来得到了广泛的发展,在离心压缩机、新能源汽车、航空航天、医疗器械等领域备受青睐 但高速永磁电机的研究主要集
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


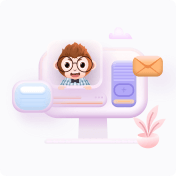