package com.niudada.controller;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.niudada.entity.*;
import com.niudada.service.*;
import com.niudada.utils.MapControl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.*;
import javax.servlet.http.HttpSession;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Controller
@RequestMapping("/section")
public class SectionController {
private static final String LIST = "section/list";
private static final String ADD = "section/add";
private static final String UPDATE = "section/update";
@Autowired
private SectionService sectionService;
@Autowired
private SubjectService subjectService;
@Autowired
private ClazzService clazzService;
@Autowired
private TeacherService teacherService;
@Autowired
private CourseService courseService;
@Autowired
private StudentService studentService;
@Autowired
public ScoreService scoreService;
//跳转添加页面
@GetMapping("/add")
public String create(Integer clazzId, ModelMap modelMap) {
//查询所有的老师,存储到request域
List<Teacher> teachers = teacherService.query(null);
//查询所有的课程,存储到request域
List<Course> courses = courseService.query(null);
modelMap.addAttribute("teachers", teachers);
modelMap.addAttribute("courses", courses);
modelMap.addAttribute("clazzId", clazzId);
return ADD;
}
//添加操作
@PostMapping("/create")
@ResponseBody
public Map<String, Object> create(@RequestBody Section section) {
int result = sectionService.create(section);
if (result <= 0) {
return MapControl.getInstance().error().getMap();
}
return MapControl.getInstance().success().getMap();
}
//根据id删除
@PostMapping("/delete/{id}")
@ResponseBody
public Map<String, Object> delete(@PathVariable("id") Integer id) {
int result = sectionService.delete(id);
if (result <= 0) {
return MapControl.getInstance().error().getMap();
}
return MapControl.getInstance().success().getMap();
}
//批量删除
@PostMapping("/delete")
@ResponseBody
public Map<String, Object> delete(String ids) {
int result = sectionService.delete(ids);
if (result <= 0) {
return MapControl.getInstance().error().getMap();
}
return MapControl.getInstance().success().getMap();
}
//修改操作
@PostMapping("/update")
@ResponseBody
public Map<String, Object> update(@RequestBody Section section) {
int result = sectionService.update(section);
if (result <= 0) {
return MapControl.getInstance().error().getMap();
}
return MapControl.getInstance().success().getMap();
}
//根据id查询,跳转修改页面
@GetMapping("/detail/{id}")
public String detail(@PathVariable("id") Integer id, ModelMap modelMap) {
//查询出要修改的班级
Section section = sectionService.detail(id);
//查询所有的老师
List<Teacher> teachers = teacherService.query(null);
//查询所有的课程
List<Course> courses = courseService.query(null);
modelMap.addAttribute("teachers", teachers);
modelMap.addAttribute("courses", courses);
modelMap.addAttribute("section", section);
return UPDATE;
}
//查询所有
@PostMapping("/query")
@ResponseBody
public Map<String, Object> query(@RequestBody Section section) {
//查询所有的开课信息
List<Section> list = sectionService.query(section);
//查询所有的老师
List<Teacher> teachers = teacherService.query(null);
//查询所有的班级
List<Course> courses = courseService.query(null);
//设置关联
list.forEach(entity -> {
teachers.forEach(teacher -> {
//判断开课表的teacherId和老师表的id是否一致
if (teacher.getId() == entity.getTeacherId()) {
entity.setTeacher(teacher);
}
});
courses.forEach(course -> {
//判断开课表中的courseId和课程表的id是否一致
if (course.getId() == entity.getCourseId()) {
entity.setCourse(course);
}
});
});
//查询宗记录条数
Integer count = sectionService.count(section);
return MapControl.getInstance().success().page(list, count).getMap();
}
//跳转列表页面
@GetMapping("/list")
public String list() {
return LIST;
}
//生成zTree树形
@PostMapping("/tree")
@ResponseBody
public List<Map> tree() {
List<Subject> subjects = subjectService.query(null);
List<Clazz> clazzes = clazzService.query(null);
List<Map> list = new ArrayList<>();
subjects.forEach(subject -> {
Map<String, Object> map = new HashMap<>();
map.put("id", subject.getId());
map.put("name", subject.getSubjectName());
map.put("parentId", 0);
List<Map<String, Object>> childrenList = new ArrayList<>();
clazzes.forEach(clazz -> {
if (subject.getId() == clazz.getSubjectId()) {
Map<String, Object> children = new HashMap<>();
children.put("id", clazz.getId());
children.put("name", clazz.getClazzName());
children.put("parentId", subject.getId());
childrenList.add(children);
}
});
map.put("children", childrenList);
list.add(map);
});
ObjectMapper objectMapper = new ObjectMapper();
try {
String jsonString = objectMapper.writeValueAsString(list);
} catch (JsonProcessingException e) {
e.printStackTrace();
}
return list;
}
//跳转开课信息列表页面
@GetMapping("/student_section")
public String student_section() {
return "section/student_section";
}
@PostMapping("/query_student_section")
@ResponseBody
public Map<String, Object> student_section(HttpSession session) {
Student student = (Student) session.getAttribute("user");
List<Section> sections = sectionService.queryByStudent(student.getId());
List<Clazz> clazzes = clazzService.query(null);
List<Teacher> teachers = teacherService.query(null);
List<Course> courses = courseService.query(null);
sections.forEach(section -> {
clazzes.forEach(clazz -> {
if (section.getClazzId() == clazz.getId()) {
section.setClazz(clazz);
}
});
teachers.forEach(teacher -> {
if (section.getTeacherId() == teacher.getId()) {
section.setTeacher(teacher);
}
});
courses.forEach(course -> {
if (section.getCourseId() == course.getId()) {
section.setCourse(course);
}
});
});
return MapControl.getInstance().success().add("data", sections).getMap();
}
@GetMapping("/teacher_section")
public String teacher_section() {
return "section/teacher_section";
}
@PostMapping("/query_teacher_section")
@ResponseBody
public Map<String, Object> query_teacher_section(HttpSession session) {
//获取登录老师的信息
Teacher teacher = (T
没有合适的资源?快使用搜索试试~ 我知道了~
MF00929-Java学籍管理系统源码.zip
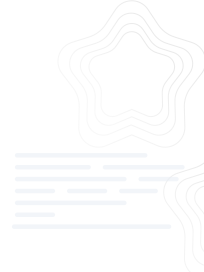
共976个文件
gif:306个
js:136个
class:122个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 111 浏览量
2023-08-14
23:23:39
上传
评论
收藏 32.11MB ZIP 举报
温馨提示
Java学籍管理系统源码带本地搭建教程 注意:不带技术支持,有帮助文件,虚拟商品,发货不退,看好再拍。 开发语言 : JAVA 数据库 : MySQL 开发工具 : Eclipse 源码类型 : WebForm 技术框架:ssm + layui + jsp + echarts + mybatis + bootstrap + jquery + mysql5.7 运行环境:jdk8 + nginx1.20 + tomcat9 + IntelliJ IDEA + maven + 宝塔面板 系统功能介绍 管理员:专业管理、班级管理、学生管理、老师管理、课程管理、开课管理、用户管理 教师:成绩管理、学生查询 学生:选课管理、查看成绩 系统概览:专业数量、班级数量、课程数量、老师数量、开课数量、学生数量
资源推荐
资源详情
资源评论
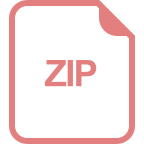
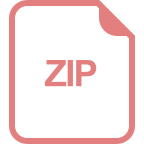
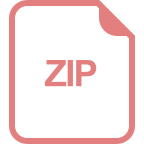
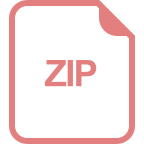
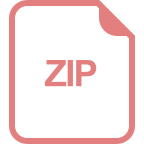
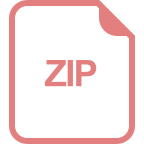
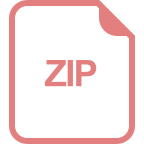
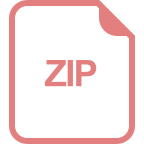
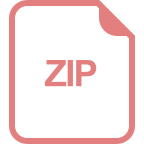
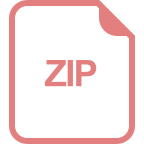
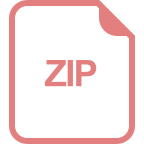
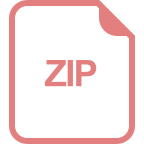
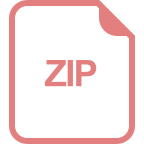
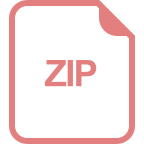
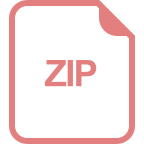
收起资源包目录

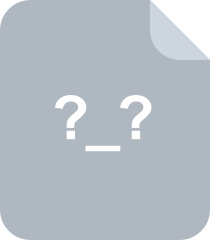
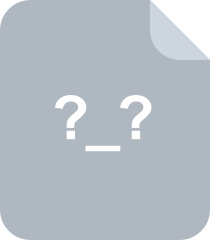
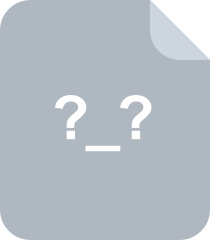
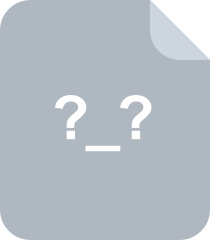
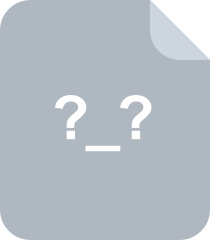
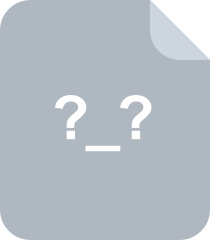
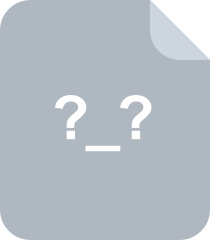
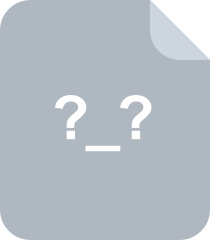
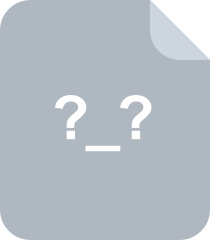
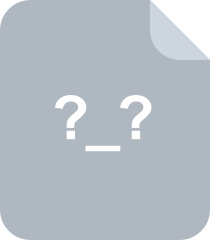
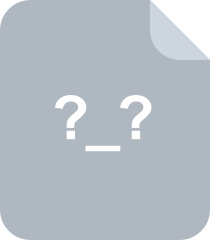
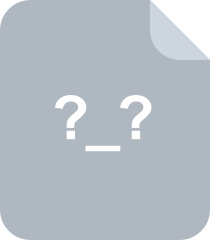
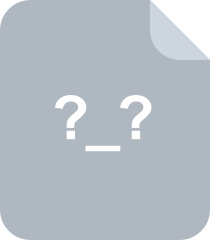
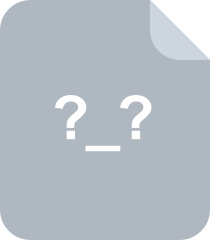
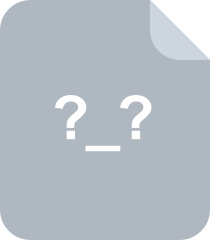
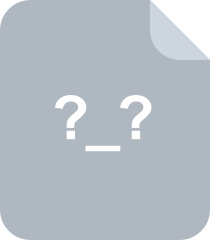
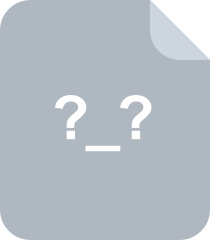
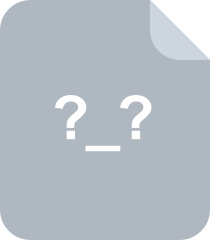
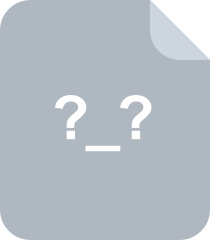
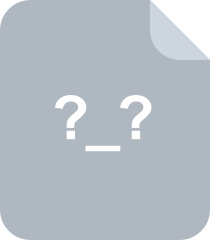
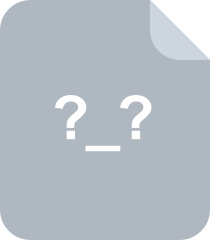
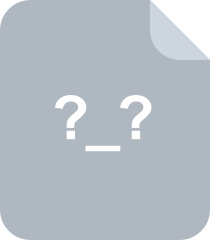
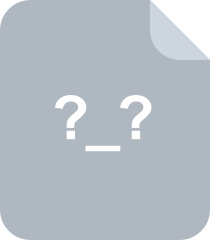
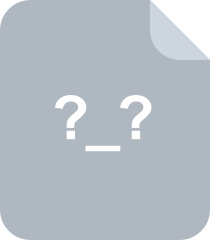
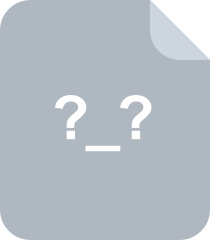
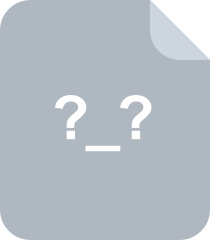
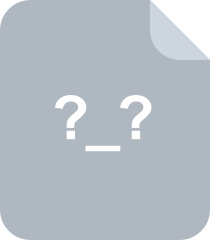
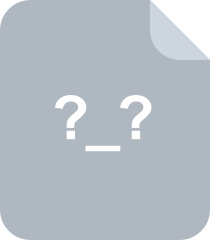
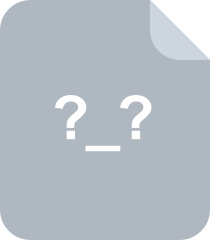
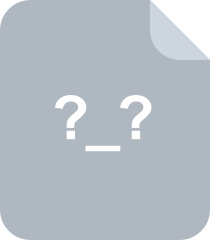
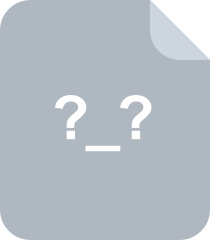
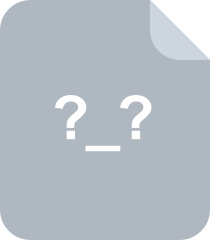
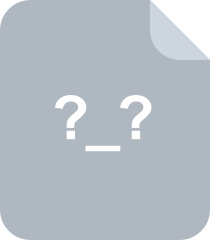
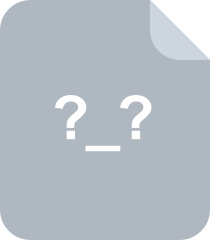
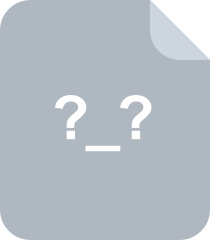
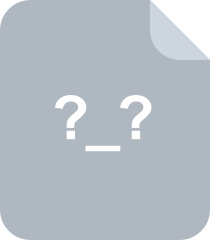
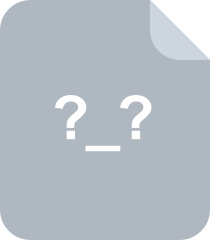
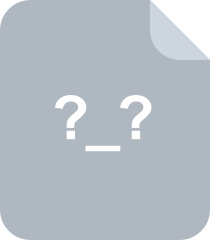
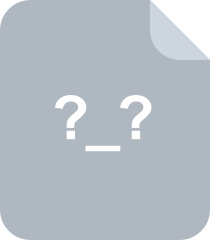
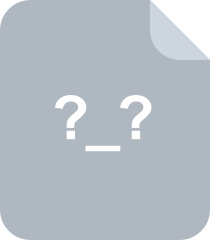
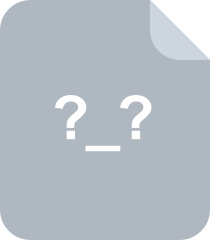
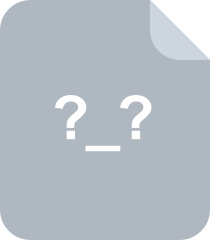
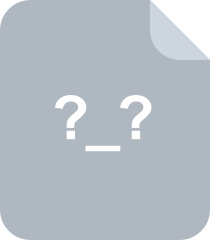
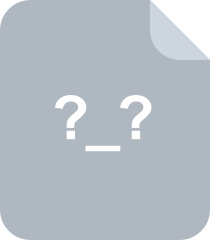
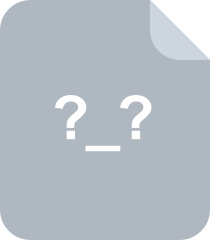
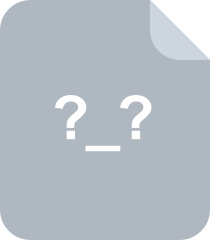
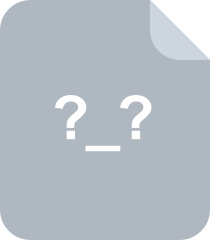
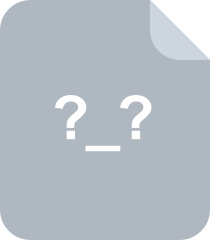
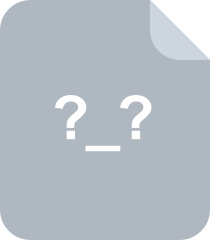
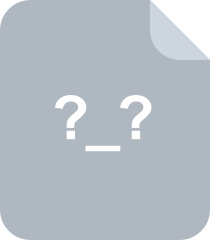
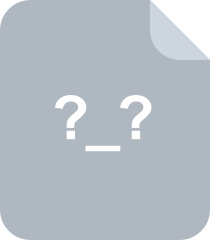
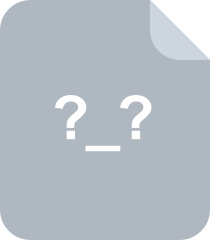
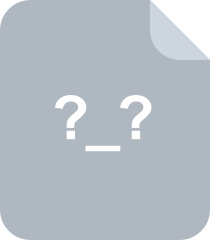
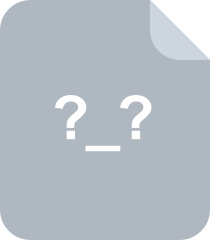
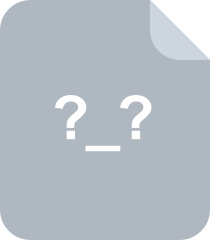
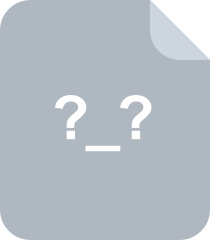
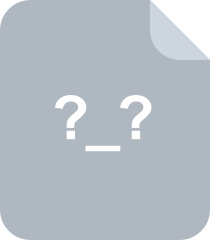
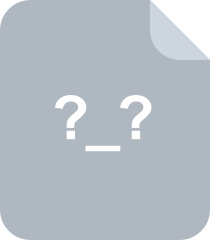
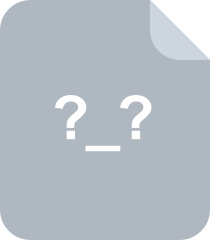
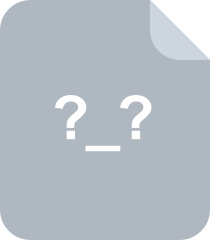
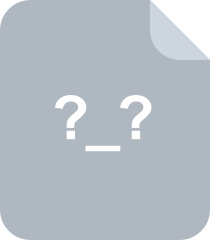
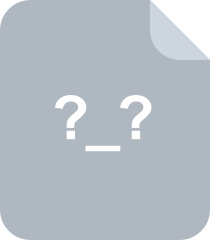
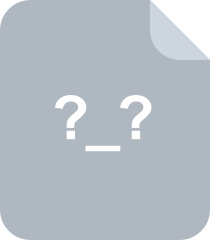
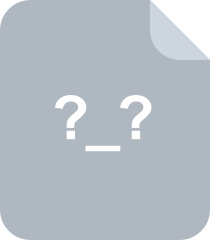
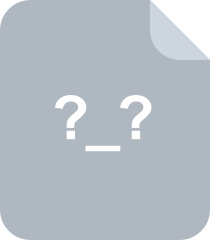
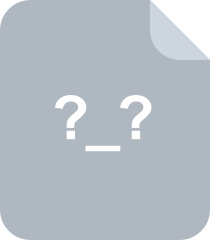
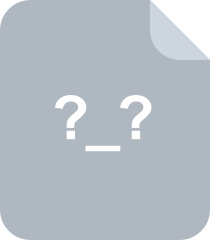
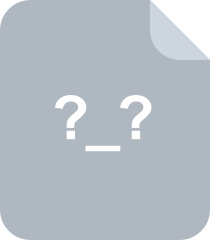
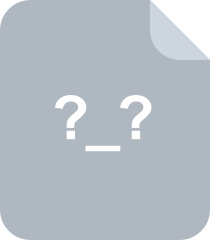
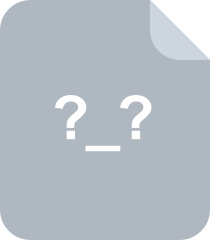
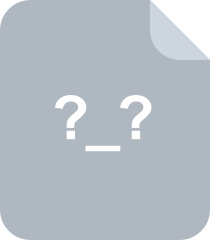
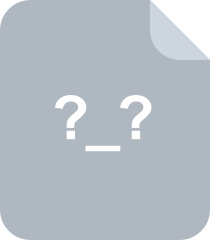
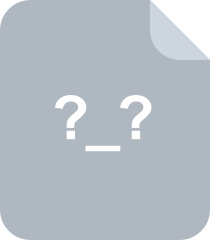
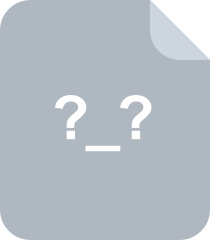
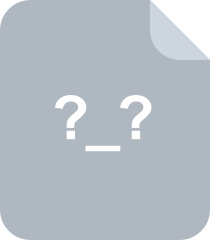
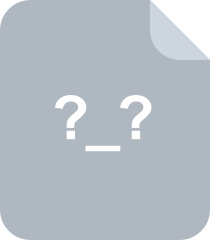
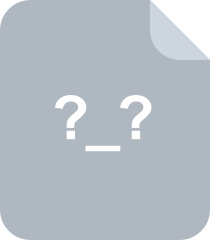
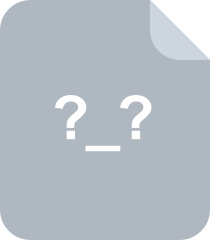
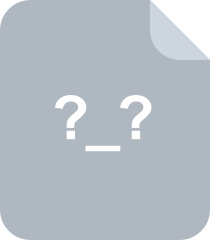
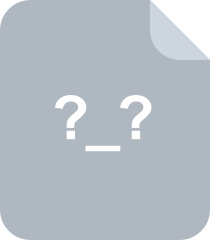
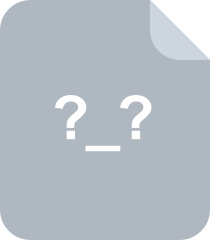
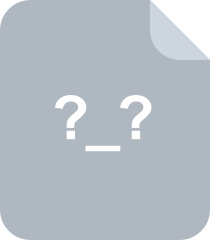
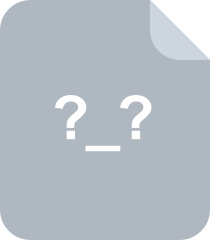
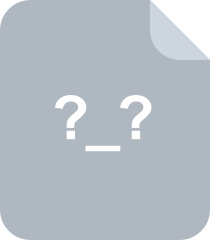
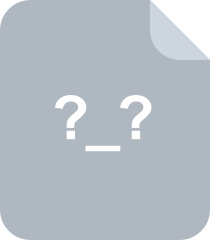
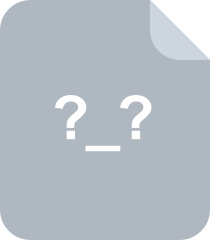
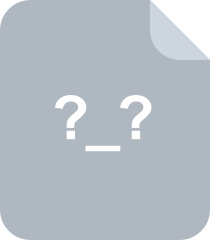
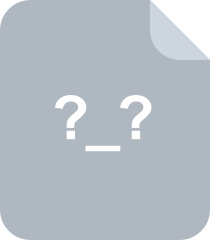
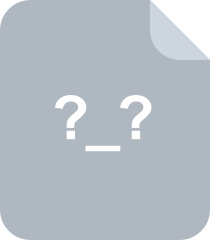
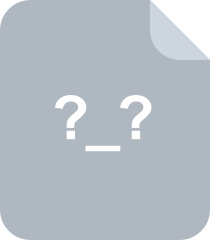
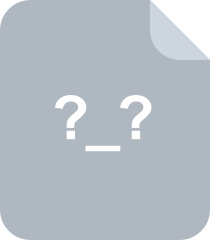
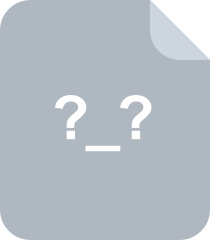
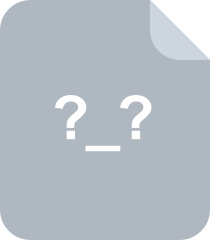
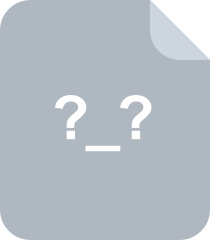
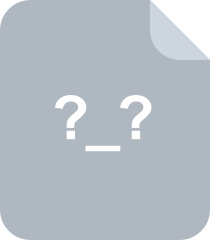
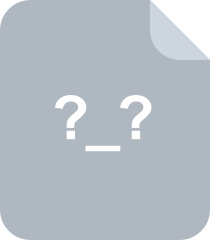
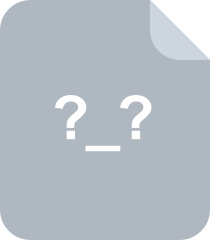
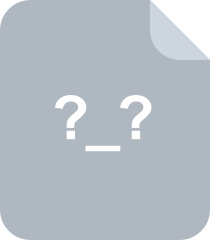
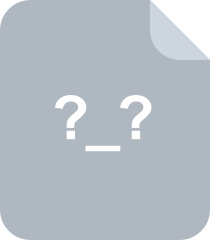
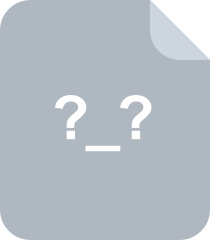
共 976 条
- 1
- 2
- 3
- 4
- 5
- 6
- 10
资源评论
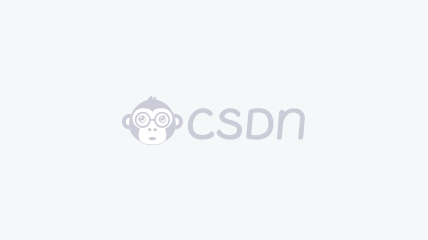

jane9872
- 粉丝: 109
- 资源: 7795

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

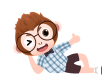
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


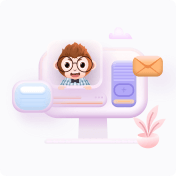
安全验证
文档复制为VIP权益,开通VIP直接复制
