在C语言中,字符串处理是不可或缺的一部分,它涉及到多种函数,可以帮助我们进行字符串的创建、复制、连接、比较以及搜索等各种操作。以下是一些常用的C语言字符串处理函数的详解及示例程序: 1. **stpcpy()**: - 功能:此函数将源字符串(source)的内容复制到目标字符串(destin)中,并返回目标字符串的结束位置。 - 用法:`char *stpcpy(char *destin, char *source);` - 示例程序: ```c #include <stdio.h> #include <string.h> int main(void) { char string[10]; char *str1 = "abcdefghi"; stpcpy(string, str1); printf("%s\n", string); return 0; } ``` 2. **strcat()**: - 功能:将源字符串(source)追加到目标字符串(destin)的末尾。 - 用法:`char *strcat(char *destin, char *source);` - 示例程序: ```c #include <string.h> #include <stdio.h> int main(void) { char destination[25]; char *blank = " ", *c = "C++", *Borland = "Borland"; strcpy(destination, Borland); strcat(destination, blank); strcat(destination, c); printf("%s\n", destination); return 0; } ``` 3. **strchr()**: - 功能:在字符串(str)中查找指定字符(c)的首次出现的位置。 - 用法:`char *strchr(char *str, char c);` - 示例程序: ```c #include <string.h> #include <stdio.h> int main(void) { char string[15]; char *ptr, c = 'r'; strcpy(string, "This is a string"); ptr = strchr(string, c); if (ptr) printf("The character %c is at position: %d\n", c, ptr-string); else printf("The character was not found\n"); return 0; } ``` 4. **strcmp()**: - 功能:比较两个字符串(str1 和 str2),根据ASCII码值判断它们的相对顺序。 - 用法:`int strcmp(char *str1, char *str2);` - 示例程序: ```c #include <string.h> #include <stdio.h> int main(void) { char *buf1 = "aaa", *buf2 = "bbb", *buf3 = "ccc"; int ptr; ptr = strcmp(buf2, buf1); if (ptr > 0) printf("buffer 2 is greater than buffer 1\n"); else printf("buffer 2 is less than buffer 1\n"); ptr = strcmp(buf2, buf3); if (ptr > 0) printf("buffer 2 is greater than buffer 3\n"); else printf("buffer 2 is less than buffer 3\n"); return 0; } ``` 5. **strncmpi()**(注意:这个函数并不是标准C库中的函数,可能是某些库或特定平台提供的): - 功能:不区分大小写地比较两个字符串(str1 和 str2)的前maxlen个字符。 - 用法:`int strncmpi(char *str1, char *str2, unsigned maxlen);` - 示例程序(可能需要根据实际库或平台替换为相应的函数,例如`strcasecmp`): ```c #include <string.h> #include <stdio.h> int main(void) { char *buf1 = "BBB", *buf2 = "bbb"; int ptr; ptr = strcasecmp(buf2, buf1); // 替换为实际函数 if (ptr > 0) printf("buffer 2 is greater than buffer 1\n"); if (ptr < 0) printf("buffer 2 is less than buffer 1\n"); return 0; } ``` 除了上述函数,C语言还提供了许多其他字符串处理函数,如`strcpy()`用于完全复制字符串,`strlen()`用于计算字符串长度,`strncpy()`用于复制指定长度的字符串,`strncat()`用于有限长度的字符串连接,`strstr()`用于在一个字符串中查找子字符串,等等。掌握这些函数的使用,对于编写C语言程序特别是涉及文本处理的系统开发至关重要。通过实践和理解每个函数的用法,可以更加高效地处理字符串数据,从而提高编程效率。
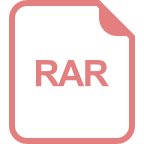
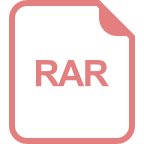
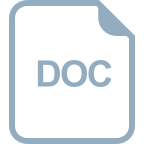
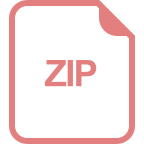
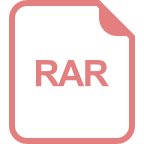
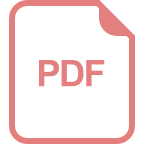
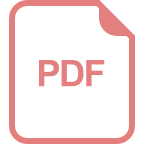
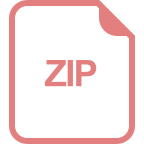
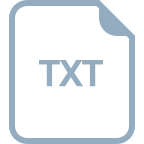
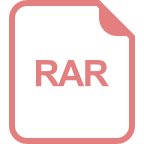
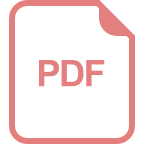
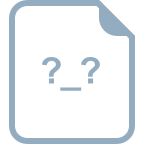
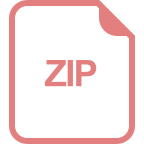
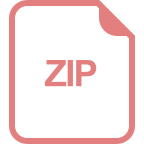
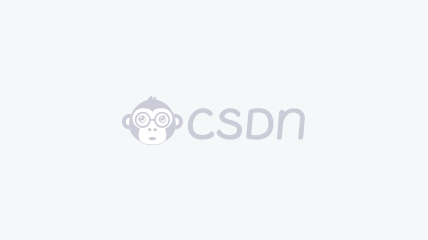

- 粉丝: 49
- 资源: 31
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

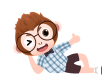
最新资源
- 一个线程安全的并发映射.zip
- 一个用于与任意 JSON 交互的 Go 包.zip
- 一个用于 go 的 cron 库.zip
- 基于BJUI + Spring MVC + Spring + Mybatis框架的办公自动化系统设计源码
- 基于百度地图的Java+HTML+JavaScript+CSS高速公路设备管理系统设计源码
- 基于Django Web框架的母婴商城实践项目设计源码
- 一个使用 Go 编程语言和 WebAssembly 构建渐进式 Web 应用程序的包 .zip
- 基于Python桌面画笔的自动画图设计源码
- 基于Java语言的中医通病例问询子系统设计源码
- 基于Java语言的云南旅游主题设计源码

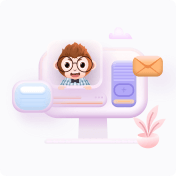
