Java编程语言在软件开发中扮演着重要角色,而软件度量是评估代码质量和复杂性的重要工具。本资源提供了一个简单的Java实现,用于计算代码行数,这是度量软件规模的一个基本指标,也是估算软件开发成本的关键因素。这个桌面应用程序旨在为开发者提供一个直观的方式来了解他们的项目规模,并据此进行优化。 软件度量通常包括多种指标,例如: 1. **代码行数(LOC,Lines of Code)**:这是最基础的度量,虽然有时被认为过于简单,但依然被广泛使用。它可以帮助初略估计项目的大小和工作量。该Java工具能够计算源代码中的行数,包括空行、注释行和实际的语句行。 2. **功能点(Function Point)**:这是一种更高级的度量方法,考虑了软件的功能性和用户输入、输出、存储等。不过,这个Java程序可能不包含功能点的计算,因为它主要关注代码行数。 3. **复杂度度量**:例如Cyclomatic复杂度,衡量代码分支和决策的数量。高复杂度的代码往往更难维护和测试。虽然描述中没有明确指出,但可以假设这个简单的工具可能只计算LOC,不涉及复杂的度量。 4. **类和对象的数量**:在面向对象编程中,类和对象的数量能反映设计的模块化程度,是评估设计质量的一个方面。 5. **重复代码(Duplication)**:检测代码重复有助于减少冗余和提高代码的可维护性。这个简单的工具可能未包含这部分功能。 6. **圈复杂度(Cyclomatic Complexity)**:通过分析控制流图来确定代码的复杂性,通常用于测试覆盖率的计算。如果此工具仅基于代码行数,那么它可能不提供这一指标。 7. **软件成本估算**:根据代码行数,可以使用一些经验公式或模型(如COCOMO模型)来估算开发时间和成本。描述中提到的这个工具可能提供了这种估算功能。 使用这样的工具,开发者可以定期检查项目的状态,及时发现潜在的问题,比如代码过于复杂或者存在大量重复。对于团队协作来说,统一的度量标准也有助于提升沟通效率和代码质量。然而,需要注意的是,度量只是手段,真正的目标是提高软件的可读性、可维护性和性能。在依赖这些工具的同时,开发者应结合其他实践,如代码审查、单元测试和持续集成,来全面评估和改进软件质量。
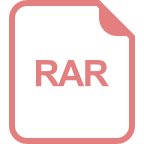
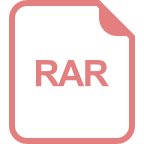
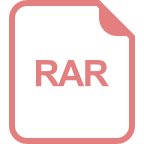
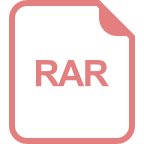
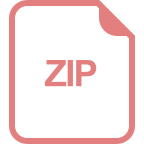
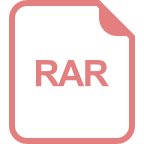
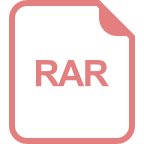
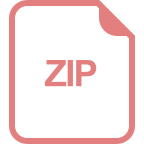
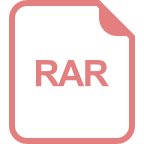
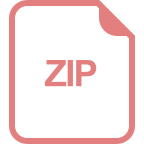
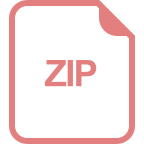
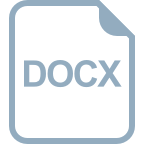
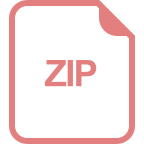
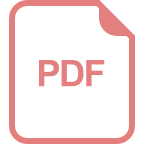
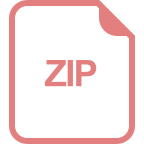
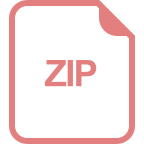
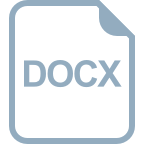
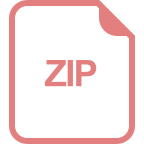
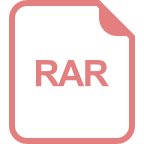
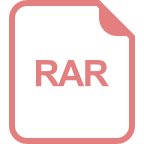
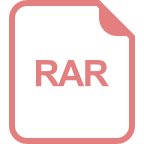
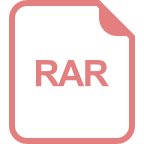
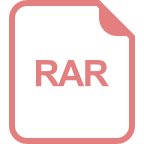
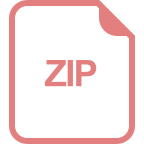
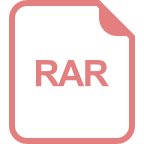
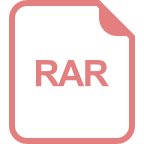








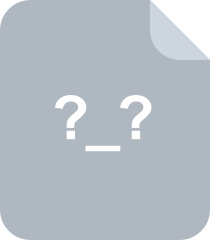
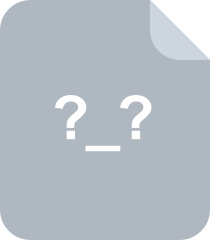
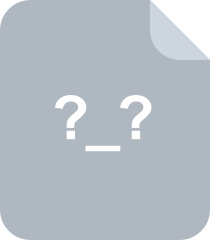



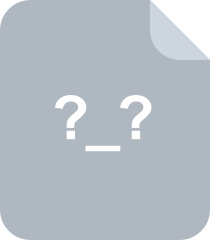
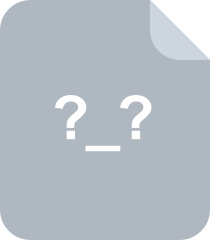
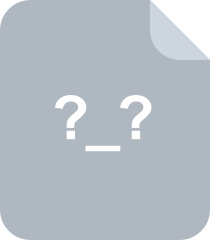

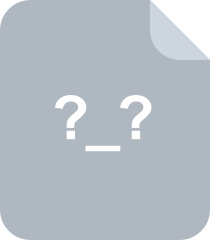
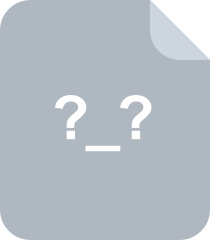

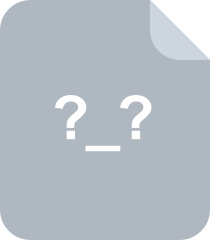
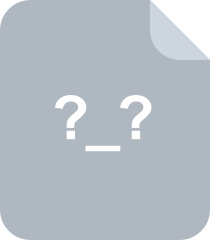
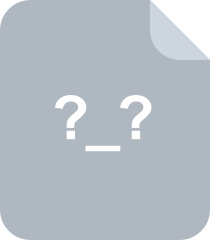
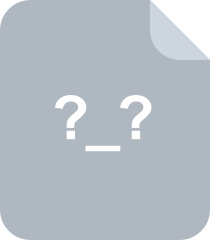
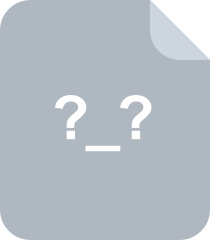
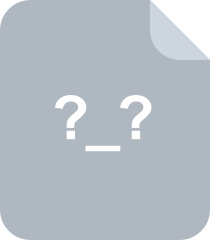
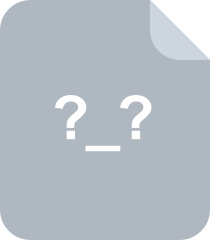
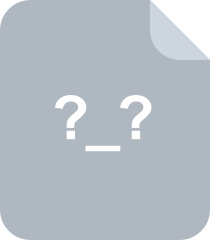
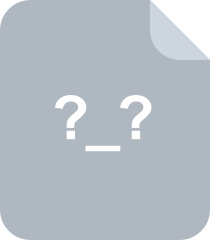
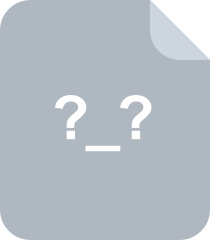
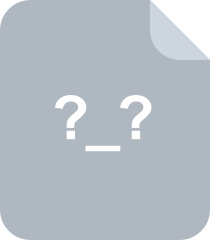

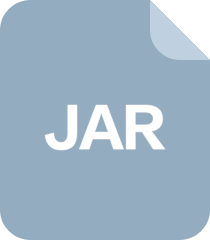

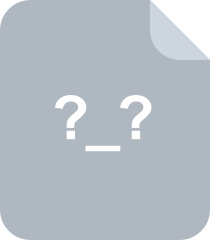






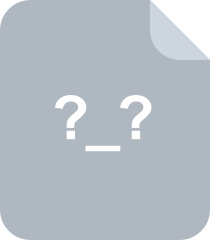
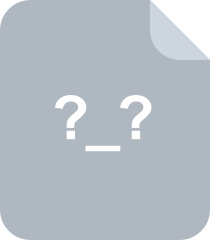
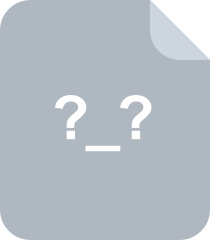



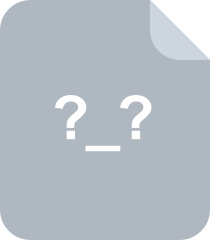
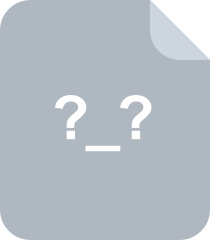
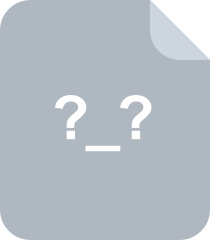

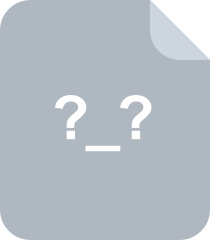
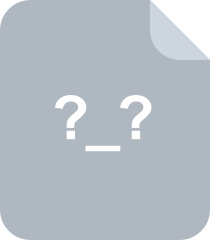

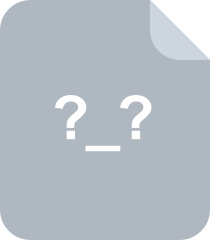
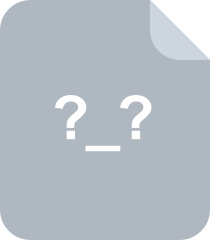
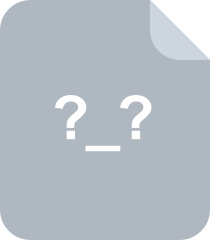
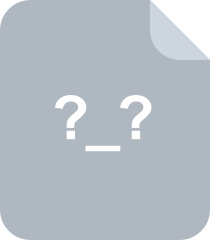
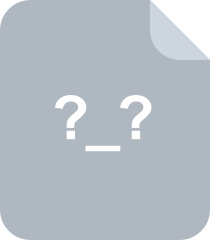
- 1
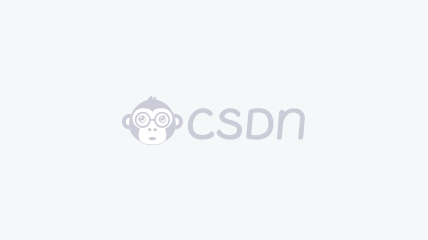
- yessica_Chen2014-05-27很好的小工具,通过度量代码行,来计算软件规模。可以运行~~
- jiangzt_leo2014-06-09很好用的小工具,可以用。
- Sunshing123152014-05-13很好,给我帮助很大
- 青梦维心2015-04-23好东西~帮助很大,很有收获~

- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

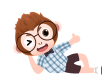
最新资源
- 全氟聚醚行业市场调研报告:全球前10强生产商排名及市场份额
- 自动裁切装PIN设备(含,BOM) sw17可编辑全套技术开发资料100%好用.zip
- C语言编程中圣诞树打印技术实现与教学
- STM32 ADC采样的十种滤波加程序
- 文件上传神器,ftp文件上传到服务器
- (176820022)基于遗传算法(GA)优化高斯过程回归(GA-GPR)的数据回归预测,matlab代码,多变量输入模型 评价指标包括:R2、M
- Python实现控制台打印圣诞树图案
- (176739420)遗传算法(GA)优化极限学习机ELM回归预测,GA-ELM回归预测,多变量输入模型 评价指标包括:R2、MAE、MSE、RM
- (175488410)基于 SSM java源码 仿buy京东商城源码 京东JavaWeb项目源代码+数据库(Java毕业设计,包括源码,教程)
- 自动编带包装机step全套技术开发资料100%好用.zip
- 基于STM32单片机的智能晾衣架项目源码(高分项目)
- 微信小程序开发入门与项目构建指南
- 自动翻转涂胶机(含工程图)sw16可编辑全套技术开发资料100%好用.zip
- (175488396)基于 SSM 的JAVAWEB校园订餐系统项目源码+数据库(Java毕业设计,包括源码,教程).zip
- (177358030)Python 爬虫基金.zip
- LLC板桥震荡参数计算

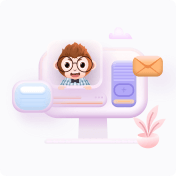
