### C语言字符串操作大全 #### 一、stpcpy - 拷贝一个字符串到另一个 **函数原型:** ```c char* stpcpy(char* destin, char* source); ``` **功能描述:** `stpcpy` 函数用于将源字符串(包括终止空字符)复制到目标字符串,并返回指向目标字符串末尾(即终止空字符的位置)的指针。 **示例代码:** ```c #include <stdio.h> #include <string.h> int main(void) { char string[10]; char *str1 = "abcdefghi"; stpcpy(string, str1); printf("%s\n", string); return 0; } ``` **运行结果:** 该示例代码会输出 `abcdefghi`,展示了如何使用 `stpcpy` 将一个字符串完整地复制到另一个字符串中。 --- #### 二、strcat - 字符串拼接函数 **函数原型:** ```c char* strcat(char* destin, char* source); ``` **功能描述:** `strcat` 函数用于将一个字符串附加到另一个字符串的末尾。目标字符串必须有足够的空间来容纳添加的新字符串。 **示例代码:** ```c #include <string.h> #include <stdio.h> int main(void) { char destination[25]; char *blank = "", *c = "C++", *Borland = "Borland"; strcpy(destination, Borland); strcat(destination, blank); // 这里可以省略,因为 blank 是空字符串 strcat(destination, c); printf("%s\n", destination); return 0; } ``` **运行结果:** 该示例代码输出 `BorlandC++`,展示了如何使用 `strcat` 进行字符串的拼接。 --- #### 三、strchr - 查找给定字符的第一个匹配之处 **函数原型:** ```c char* strchr(char* str, char c); ``` **功能描述:** `strchr` 函数用于在指定字符串中查找第一次出现的指定字符的位置,并返回指向该位置的指针。如果未找到,则返回 NULL。 **示例代码:** ```c #include <string.h> #include <stdio.h> int main(void) { char string[15]; char *ptr, c = 'r'; strcpy(string, "Thisisastring"); ptr = strchr(string, c); if (ptr) printf("The character %c is at position: %d\n", c, ptr - string); else printf("The character was not found\n"); return 0; } ``` **运行结果:** 该示例代码会输出 `The character r is at position: 9`,展示了如何使用 `strchr` 查找字符在字符串中的位置。 --- #### 四、strcmp - 串比较 **函数原型:** ```c int strcmp(char* str1, char* str2); ``` **功能描述:** `strcmp` 函数用于比较两个字符串。如果 str1 > str2,则返回正值;如果 str1 == str2,则返回 0;如果 str1 < str2,则返回负值。 **示例代码:** ```c #include <string.h> #include <stdio.h> int main(void) { char *buf1 = "aaa", *buf2 = "bbb", *buf3 = "ccc"; int ptr; ptr = strcmp(buf2, buf1); if (ptr > 0) printf("buffer2 is greater than buffer1\n"); else printf("buffer2 is less than buffer1\n"); ptr = strcmp(buf2, buf3); if (ptr > 0) printf("buffer2 is greater than buffer3\n"); else printf("buffer2 is less than buffer3\n"); return 0; } ``` **运行结果:** 该示例代码输出 `buffer2 is greater than buffer1` 和 `buffer2 is less than buffer3`,展示了如何使用 `strcmp` 对字符串进行比较。 --- #### 五、strncmpi - 比较字符串,忽略大小写 **函数原型:** ```c int strncmpi(char* str1, char* str2, unsigned maxlen); ``` **功能描述:** `strncmpi` 函数用于比较两个字符串的前 maxlen 个字符,但忽略大小写的差异。 **示例代码:** ```c #include <string.h> #include <stdio.h> int main(void) { char *buf1 = "BBB", *buf2 = "bbb"; int ptr; ptr = strncmpi(buf2, buf1, 3); if (ptr > 0) printf("buffer2 is greater than buffer1\n"); else if (ptr < 0) printf("buffer2 is less than buffer1\n"); else printf("buffer2 equals buffer1\n"); return 0; } ``` **运行结果:** 该示例代码输出 `buffer2 equals buffer1`,展示了如何使用 `strncmpi` 忽略大小写进行字符串比较。 --- #### 六、strcpy - 串拷贝 **函数原型:** ```c char* strcpy(char* str1, char* str2); ``` **功能描述:** `strcpy` 函数用于将源字符串复制到目标字符串。源字符串会被完全复制到目标字符串中,包括终止空字符。 **示例代码:** ```c #include <stdio.h> #include <string.h> int main(void) { char string[10]; char *str1 = "abcdefghi"; strcpy(string, str1); printf("%s\n", string); return 0; } ``` **运行结果:** 该示例代码输出 `abcdefghi`,展示了如何使用 `strcpy` 复制字符串。 --- #### 七、strcspn - 在串中查找第一个给定字符集内容的段 **函数原型:** ```c int strcspn(char* str1, char* str2); ``` **功能描述:** `strcspn` 函数用于计算在字符串 str1 的起始部分中没有出现在字符串 str2 中的字符的最大长度。 **示例代码:** ```c #include <stdio.h> #include <string.h> #include <alloc.h> int main(void) { char string1[20] = "Thisisastring"; char *set = "aeiou"; // 查找不包含这些元音的最长子串 int length = strcspn(string1, set); printf("Length of the segment without vowels: %d\n", length); return 0; } ``` **运行结果:** 该示例代码输出 `Length of the segment without vowels: 3`,展示了如何使用 `strcspn` 计算字符串起始部分中不包含特定字符集合的最长子串长度。 通过以上示例,我们可以看到 C 语言提供了丰富的字符串处理函数,可以帮助开发者轻松地实现字符串的各种操作。掌握这些基本的字符串处理函数对于编写高效的 C 语言程序非常重要。
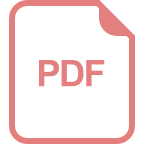
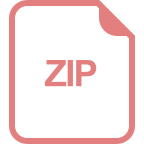
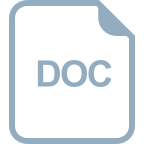
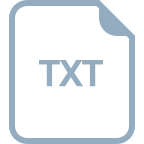
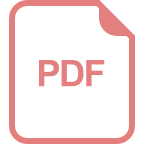
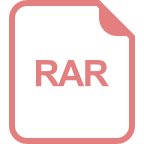
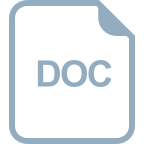
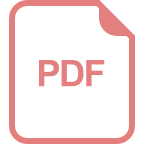
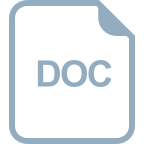
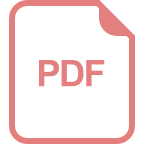
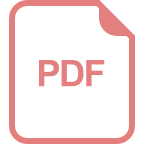
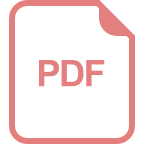
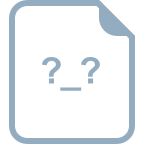
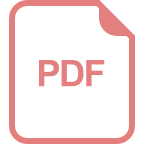
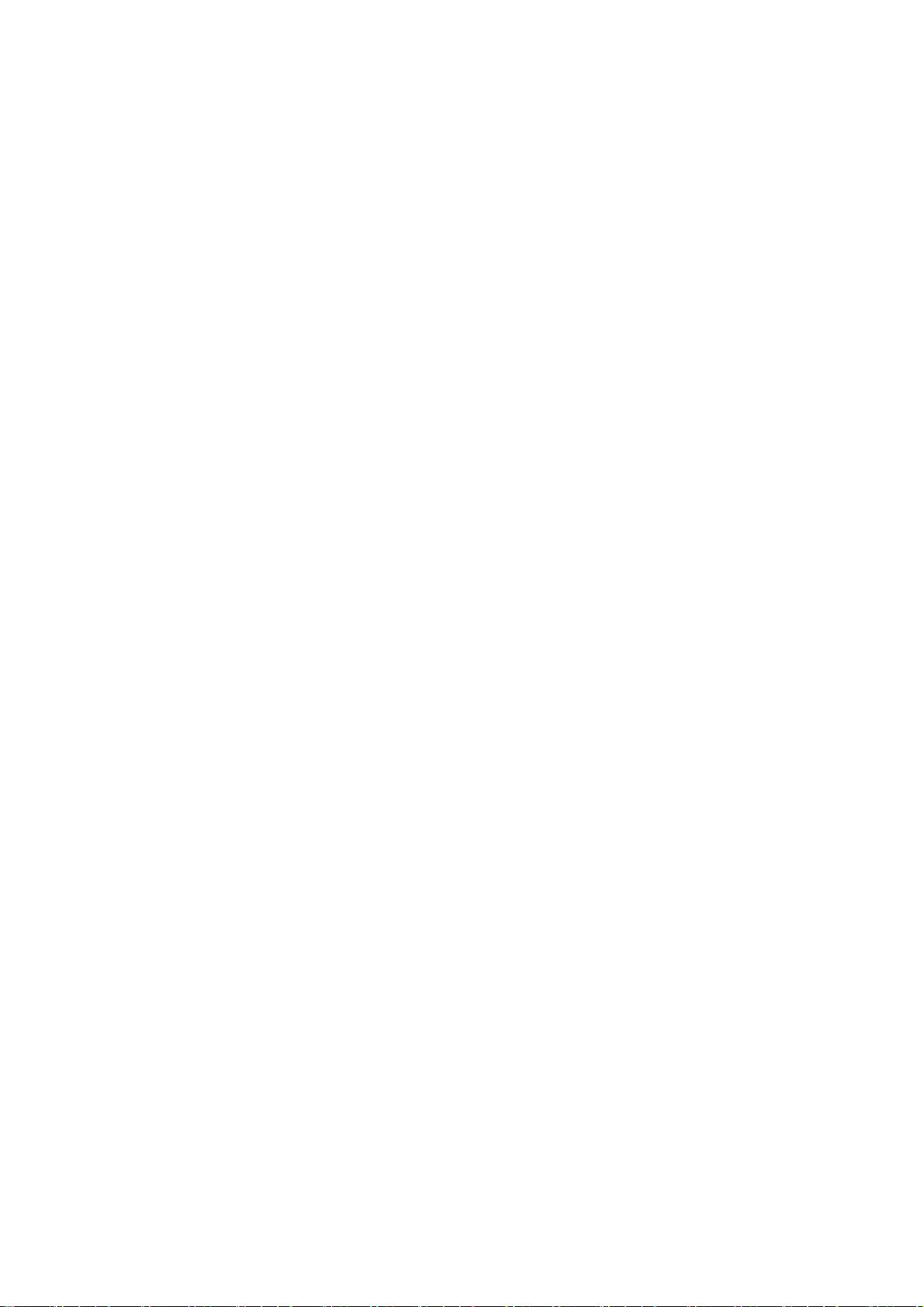
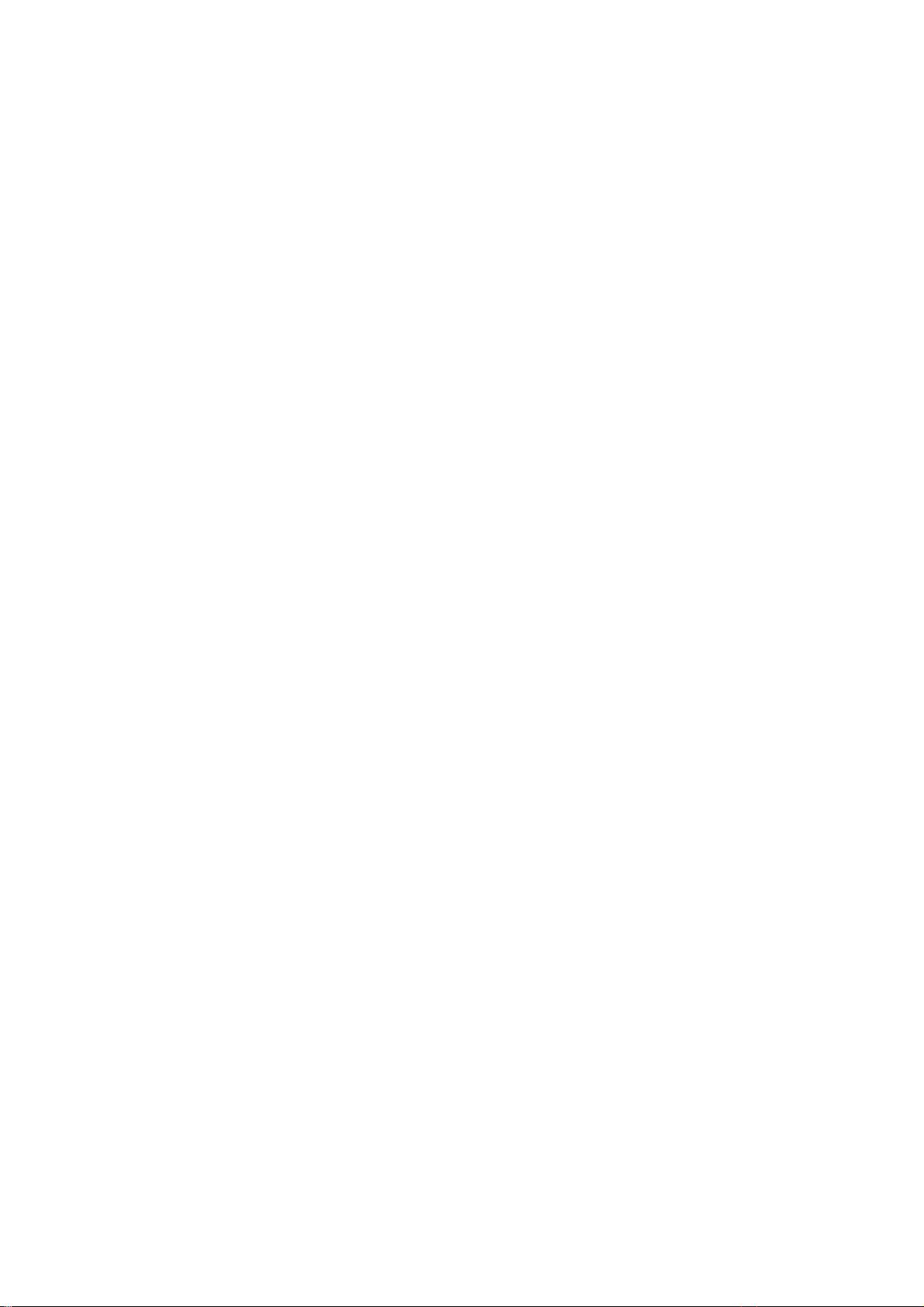
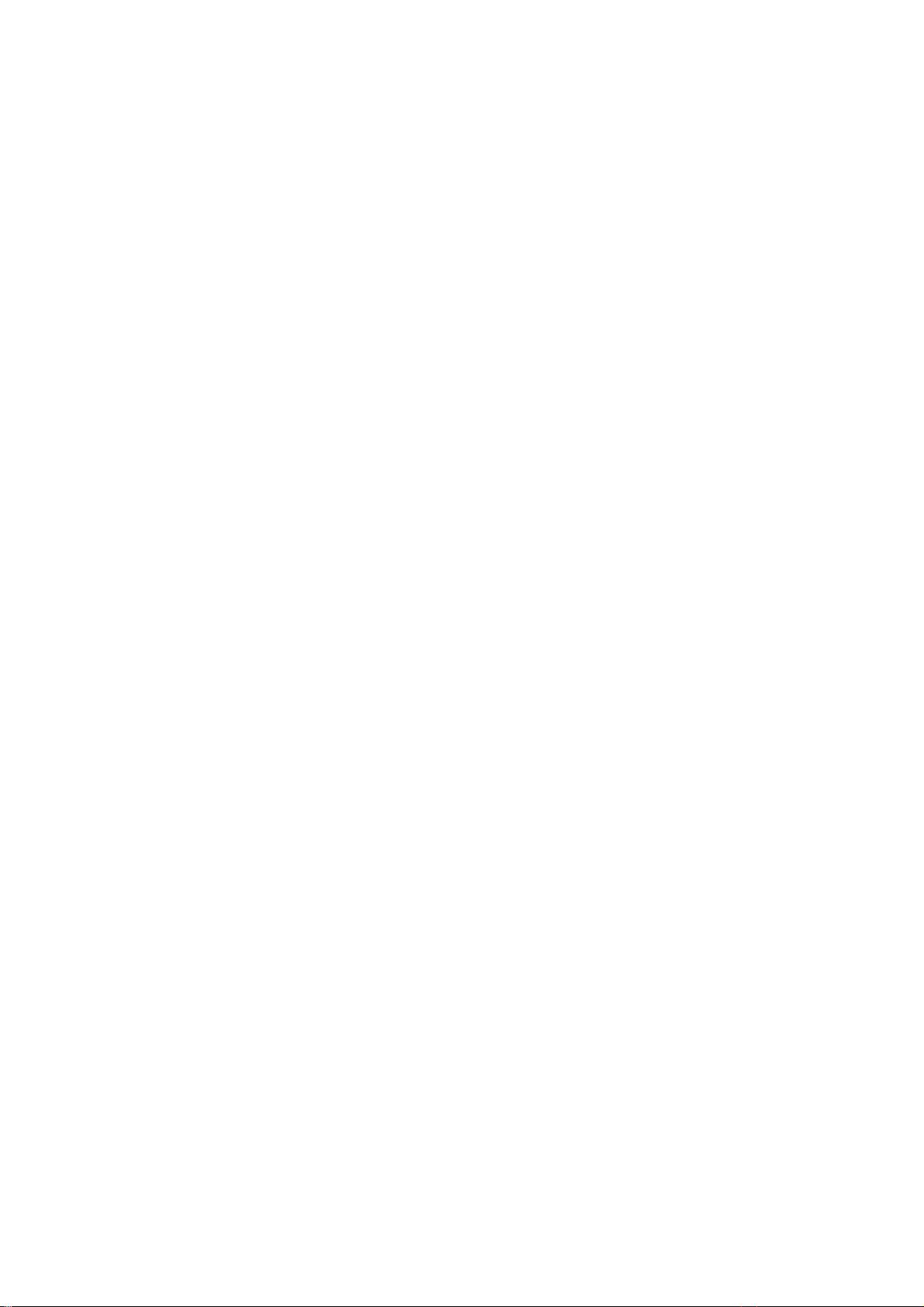
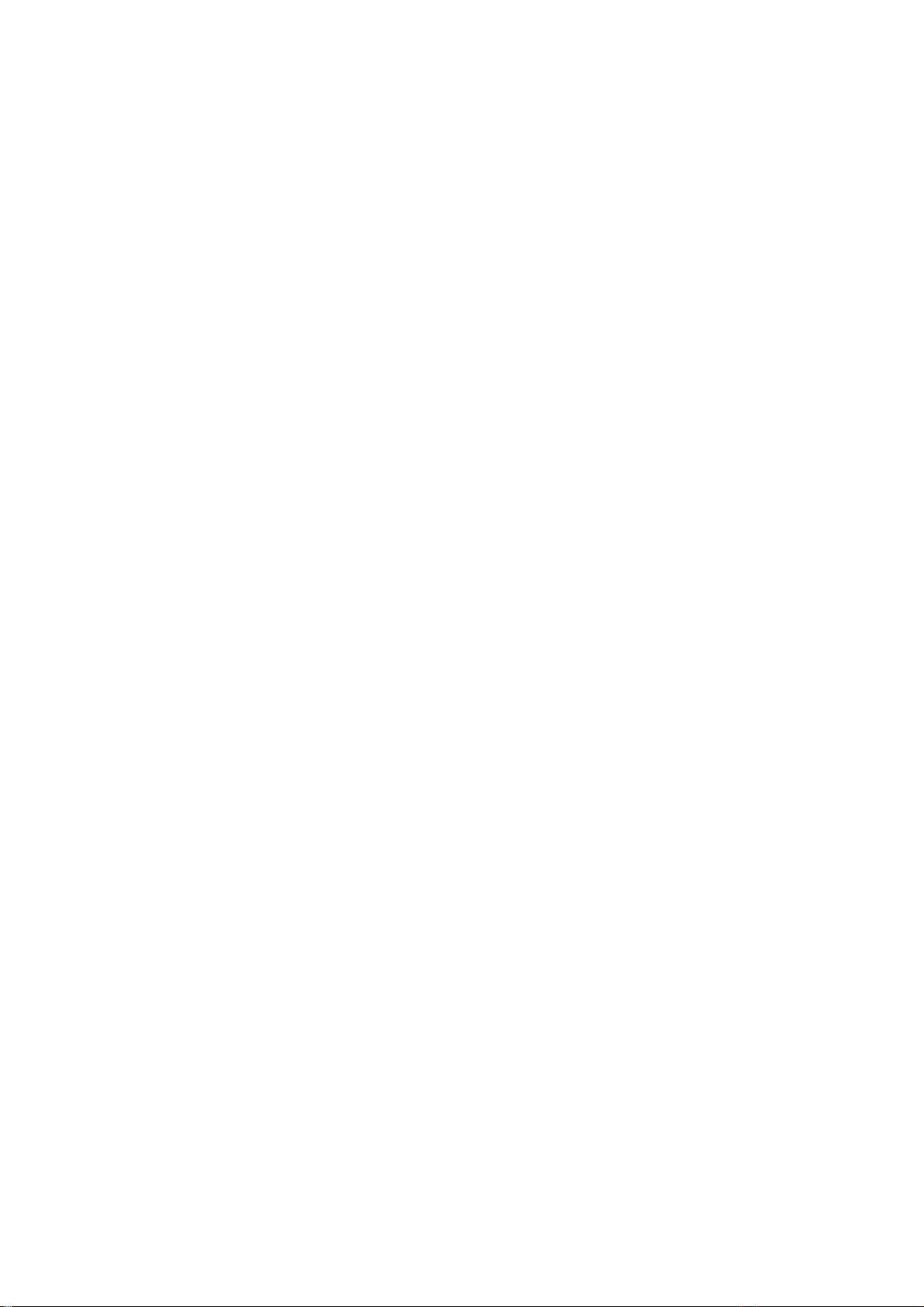
剩余23页未读,继续阅读
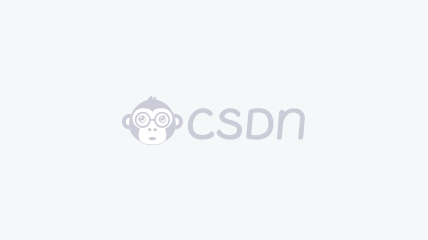
- koself2017-11-14没有什么好的价值,就是几个字符串函数的使用

- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

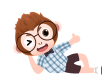
最新资源

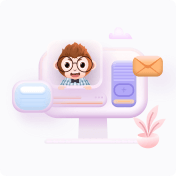
