springboot整合redis的测试案例
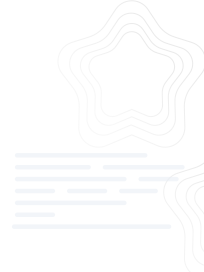

在本文中,我们将深入探讨如何在Spring Boot应用中整合Redis,以此实现高效的缓存管理和数据存储。Spring Boot简化了配置过程,并提供了与Redis的无缝集成,使得开发人员能够快速搭建和部署应用程序。以下是对"springboot整合redis的测试案例"的详细解析。 让我们从环境准备开始。在Spring Boot项目中,我们需要引入Spring Data Redis模块,这可以通过在`pom.xml`文件中添加对应的Maven依赖来完成。根据描述,这个案例使用的Maven版本是2019.11.6,因此依赖可能会像这样: ```xml <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> <version>2.2.5.RELEASE</version> <!-- 或者匹配2019.11.6时期的版本 --> </dependency> ``` 请注意,这里使用的版本可能需要与描述中的日期对应,但通常推荐使用较新的稳定版本以获取最新的特性和修复。 接下来,配置Redis连接。在`application.properties`或`application.yml`文件中,我们需设置Redis服务器的相关信息,如主机名、端口、密码等: ```properties spring.redis.host=localhost spring.redis.port=6379 # 可选:spring.redis.password=your_password # 可选:spring.redis.database=0 (默认数据库编号) ``` 然后,创建Redis配置类,以进一步自定义连接池和其他高级配置: ```java @Configuration public class RedisConfig { @Value("${spring.redis.host}") private String host; @Value("${spring.redis.port}") private int port; @Bean public LettuceConnectionFactory connectionFactory() { RedisStandaloneConfiguration config = new RedisStandaloneConfiguration(host, port); return new LettuceConnectionFactory(config); } @Bean public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory factory) { RedisTemplate<String, Object> template = new RedisTemplate<>(); template.setConnectionFactory(factory); // 配置序列化器,例如使用Jackson2JsonRedisSerializer Jackson2JsonRedisSerializer<Object> serializer = new Jackson2JsonRedisSerializer<>(Object.class); ObjectMapper om = new ObjectMapper(); om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY); om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL); serializer.setObjectMapper(om); template.setValueSerializer(serializer); template.setKeySerializer(new StringRedisSerializer()); template.afterPropertiesSet(); return template; } } ``` 现在,我们可以创建一个Redis服务接口和其实现,用于操作Redis: ```java public interface RedisService { void set(String key, Object value); Object get(String key); void delete(String key); // 其他Redis操作... } @Service public class RedisServiceImpl implements RedisService { @Autowired private RedisTemplate<String, Object> redisTemplate; @Override public void set(String key, Object value) { redisTemplate.opsForValue().set(key, value); } @Override public Object get(String key) { return redisTemplate.opsForValue().get(key); } @Override public void delete(String key) { redisTemplate.delete(key); } // 其他Redis操作的实现... } ``` 至此,我们已经完成了Spring Boot应用与Redis的基本整合。现在可以创建测试用例来验证这些配置是否正常工作。`Demo`类通常包含一些测试方法,比如: ```java @SpringBootTest public class DemoApplicationTests { @Autowired private RedisService redisService; @Test public void testRedisOperations() { String key = "testKey"; String value = "testValue"; // 存储数据到Redis redisService.set(key, value); // 检查数据是否已存储 assertEquals(value, redisService.get(key)); // 删除数据 redisService.delete(key); // 验证数据已被删除 assertNull(redisService.get(key)); } } ``` 在实际项目中,你可以扩展这些基本操作,使用Redis的更多功能,如哈希、集合、有序集合、发布/订阅等。同时,还可以考虑使用Spring Cache抽象来自动管理缓存,提高代码的可维护性。 总结,Spring Boot与Redis的整合主要涉及引入相关依赖、配置连接信息、创建Redis模板和自定义配置、实现业务服务以及编写测试用例。通过这种方式,我们可以轻松地在Spring Boot应用中利用Redis的强大功能,实现高效的数据存储和缓存管理。

























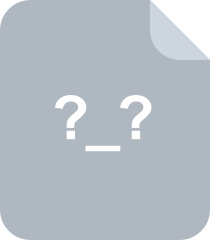
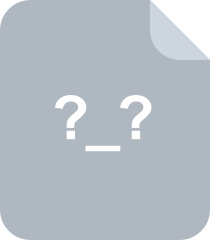
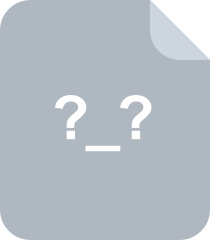
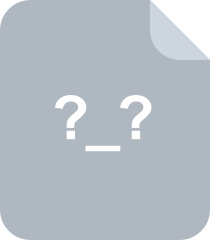
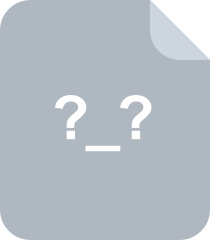
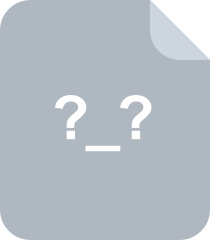
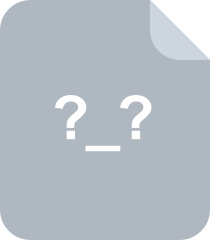
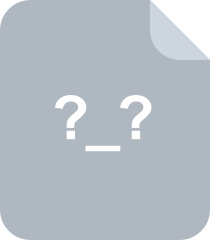
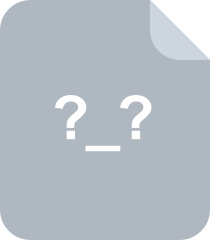
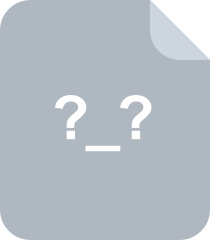
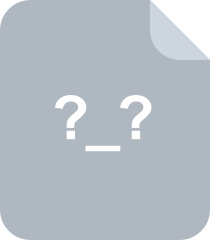
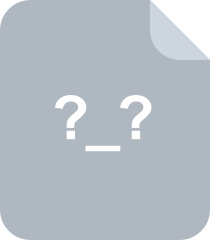
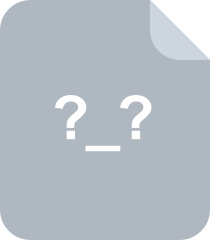
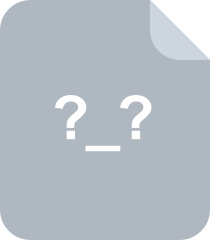
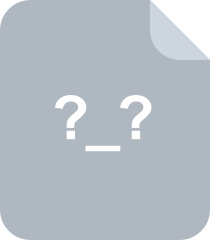
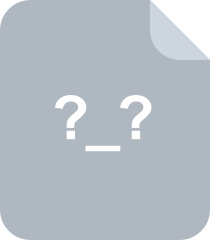
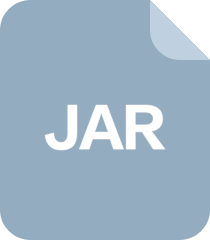
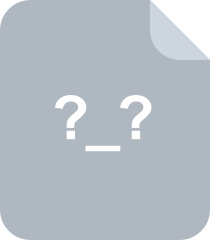
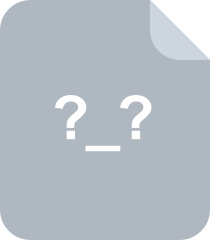
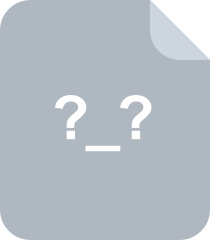
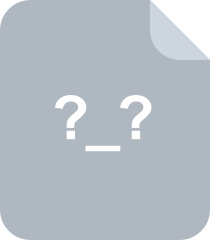
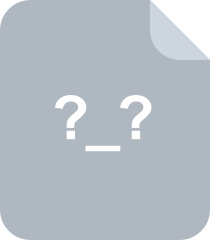
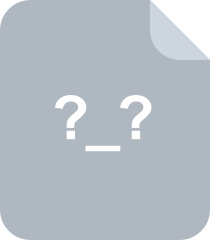
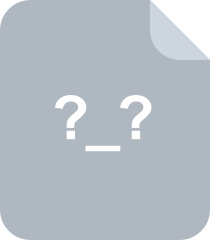
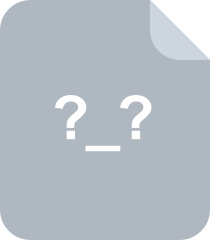
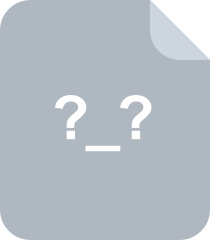
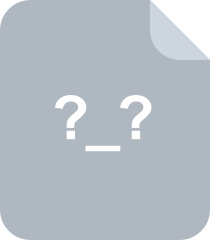
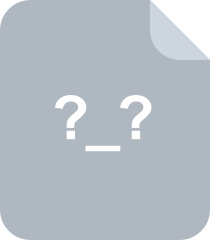
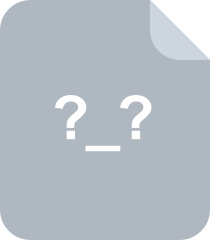
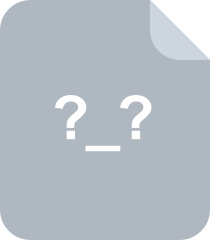
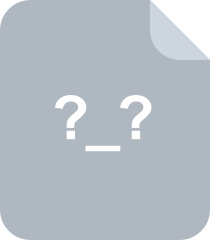
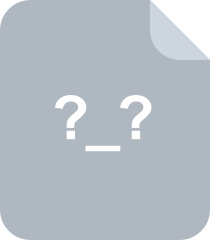
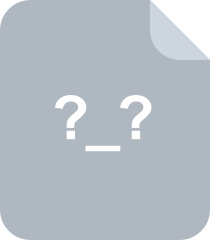
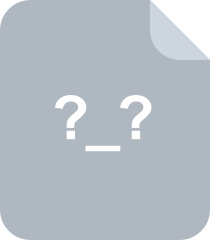
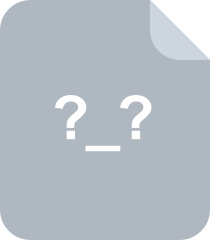
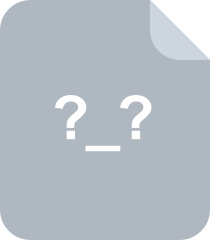
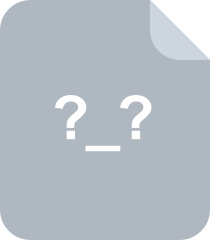
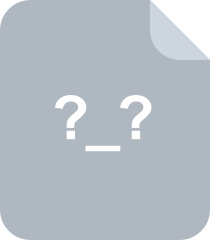
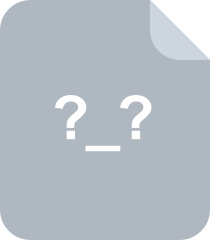
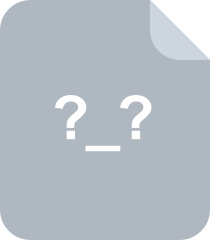
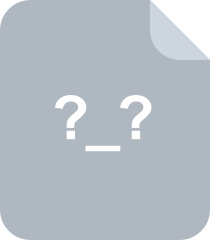
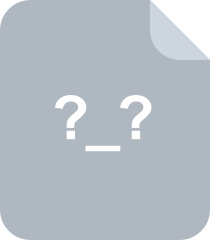
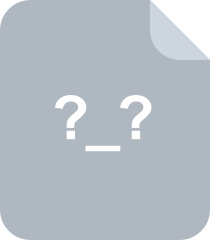
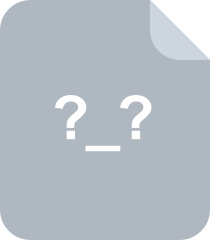
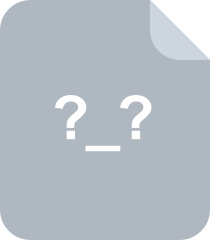
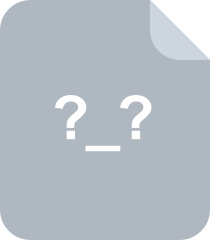
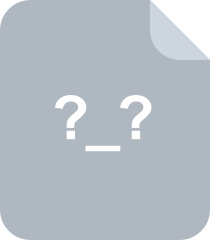
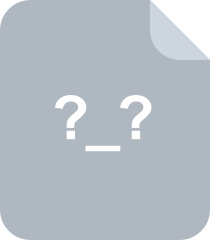
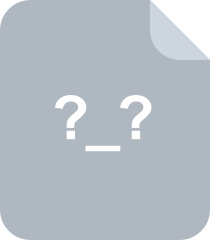
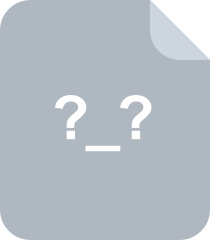
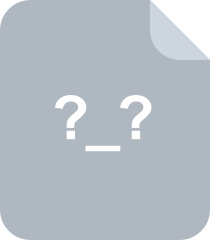
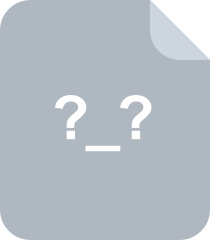
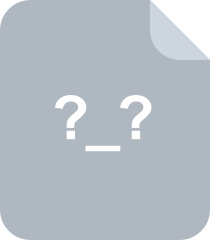
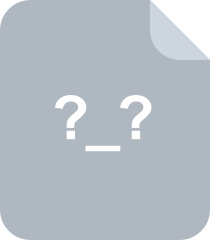
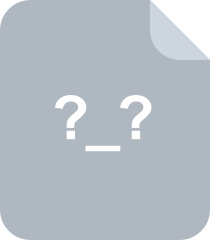
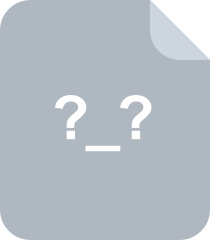
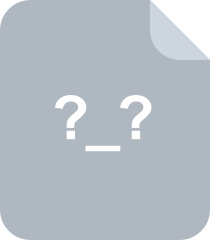
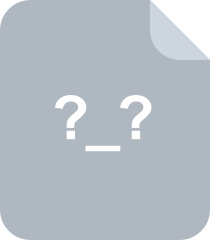
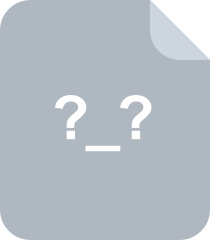
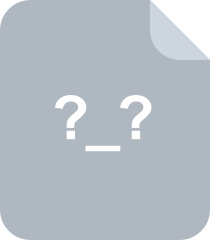
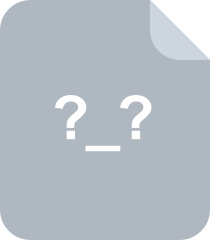
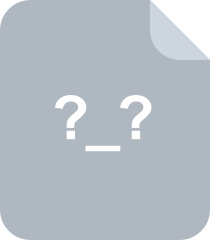
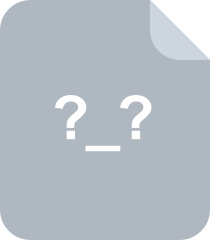
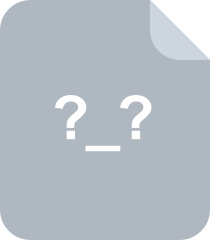
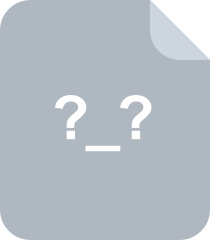
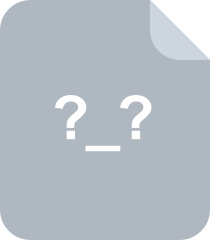
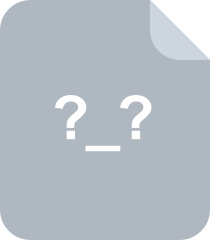
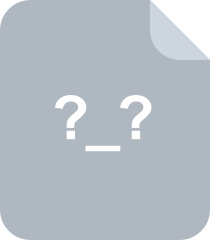
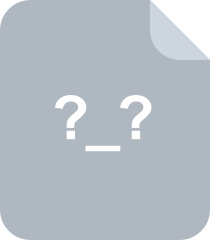
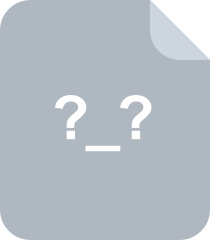
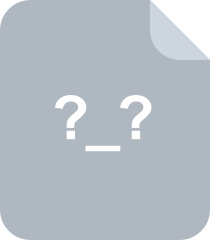
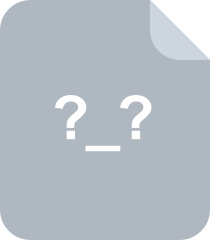
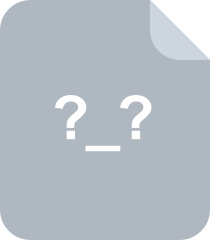
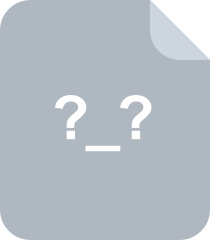
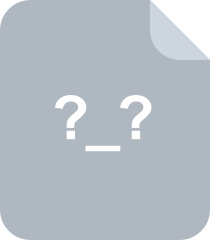
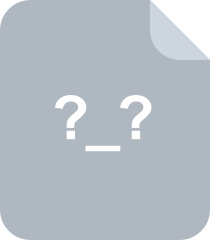
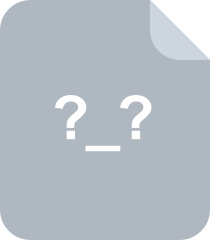
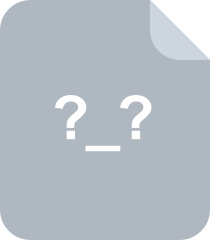
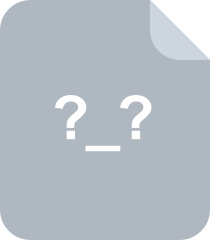
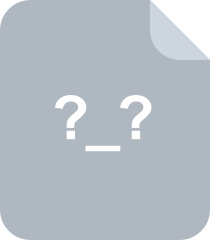
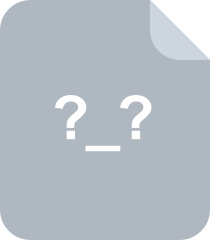
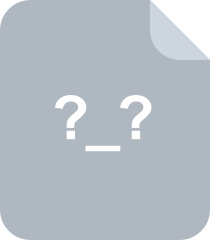
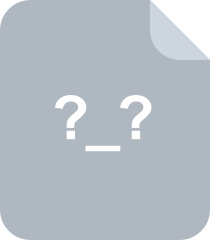
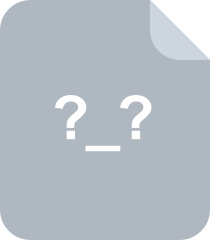
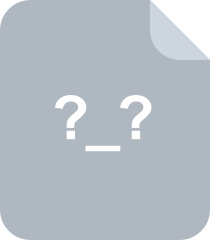
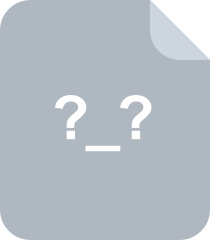
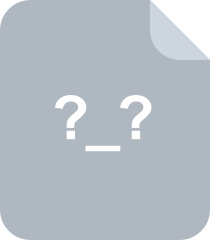
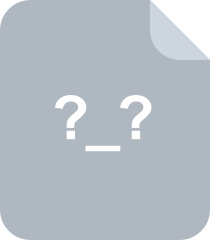
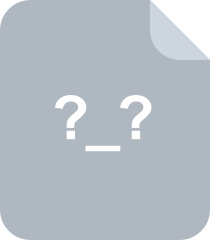
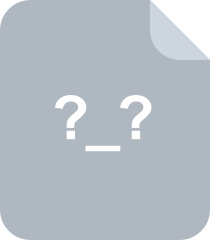
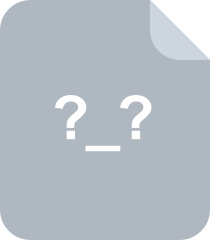
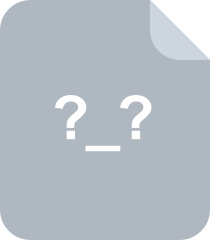
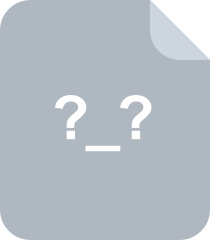
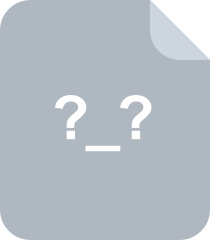
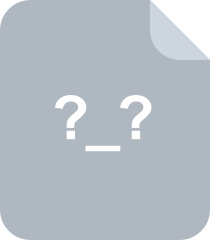
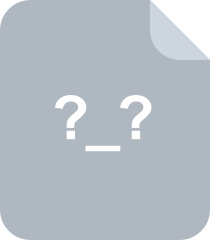
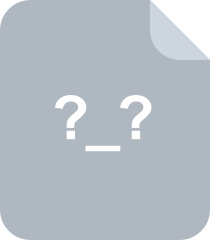
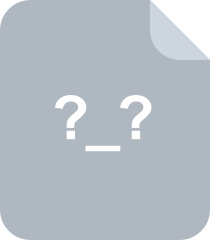
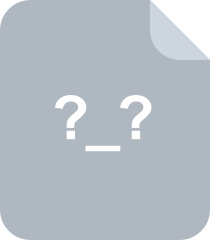
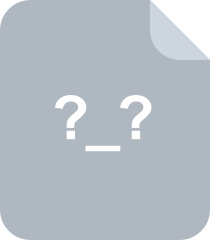
- 1
- 2
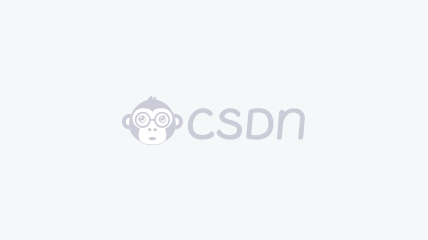

- 粉丝: 1191
- 资源: 25
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

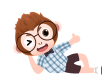
最新资源
- Python资源集锦_框架库软件资源_1741398287.zip
- 编程语言_Python_2022周活动模板_学习工具_1741402725.zip
- 知识领域_Python_环境变量管理_开发辅助.zip
- tebelorg_RPA-Python_1741401250.zip
- 编程语言交互_PythonKit_桥接框架_开发工具_1741400489.zip
- davidbombal_red-python-scripts_1741401291.zip
- ipinfo_python_1741399042.zip
- 网络认证_OAuth10_python实现_通用库_1741403060.zip
- 人工智能_奥拉玛_Python库_项目集成_1741401273.zip
- DevOps_Python基础进阶_编程技能_培训课程_1741399033.zip
- 网络爬虫_代理IP池_ProxyPool_防封堵_1741401288.zip
- 编程语言_Python_代码段_随机应用_1741403080.zip
- calistus-igwilo_python_1741400290.zip
- 面试基础知识_Python实现_编程语言_数据结构_1741403581.zip
- achillean_shodan-python_1741403441.zip
- Python高级特性_单例模式实现技巧_1741398532.zip

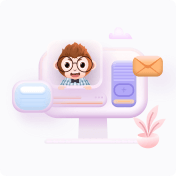
