FIR滤波器在DSP的实现主程序C语言

### FIR滤波器在DSP中的C语言实现 #### 概述 FIR(Finite Impulse Response)滤波器是数字信号处理(DSP)领域中一种非常重要的线性时不变滤波器类型。与IIR滤波器不同,FIR滤波器具有线性相位特性,并且稳定,这使得它在很多应用场合中都具有优势。本文档提供了一个基于C语言的FIR滤波器在DSP中的实现方法。 #### 程序结构 该C语言程序主要包括以下几个部分: 1. **头文件包含**: 包含必要的标准库文件。 2. **宏定义**: 定义了一些宏,例如数据类型和数组大小。 3. **初始化函数**: `clear()` 函数用于清空延迟线。 4. **基本FIR滤波器**: `fir_basic()` 实现了最简单的FIR滤波器算法。 5. **循环FIR滤波器**: `fir_circular()` 使用循环缓冲区技术实现了FIR滤波器。 6. **交错FIR滤波器**: `fir_shuffle()` 是一种高效的FIR滤波器实现方式。 7. **分段FIR滤波器**: `fir_split()` 实现了一种分段式FIR滤波器算法。 8. **双缓存FIR滤波器**: `fir_double_z()` 利用双缓存技术提高了FIR滤波器的效率。 #### 代码详解 ##### 1. 头文件包含和宏定义 ```c #include<stdio.h> #ifdef WIN32 #include<conio.h> #endif #define SAMPLE double /*定义数据样本的类型*/ void clear(int ntaps, SAMPLE z[]); ``` 这部分首先包含了`stdio.h`和条件包含`conio.h`(仅在Windows系统下)。`SAMPLE`宏定义了数据类型的符号常量,这里使用了`double`类型来存储样本数据。 ##### 2. 初始化函数 ```c void clear(int ntaps, SAMPLE z[]) { int ii; for (ii = 0; ii < ntaps; ii++) { z[ii] = 0; } } ``` `clear()`函数用于初始化延迟线数组`z`,将其所有元素置零,为后续滤波操作做准备。 ##### 3. 基本FIR滤波器 ```c SAMPLE fir_basic(SAMPLE input, int ntaps, const SAMPLE h[], SAMPLE z[]) { int ii; SAMPLE accum; z[0] = input; /*将输入值存储到延迟线的头部*/ accum = 0; for (ii = 0; ii < ntaps; ii++) { accum += h[ii] * z[ii]; } for (ii = ntaps - 2; ii >= 0; ii--) { z[ii + 1] = z[ii]; /*移动延迟线中的数据*/ } return accum; } ``` `fir_basic()`函数实现了基本的FIR滤波器,包括卷积运算和延迟线更新。 ##### 4. 循环FIR滤波器 ```c SAMPLE fir_circular(SAMPLE input, int ntaps, const SAMPLE h[], SAMPLE z[], int *p_state) { int ii, state; SAMPLE accum; state = *p_state; z[state] = input; if (++state >= ntaps) { state = 0; } accum = 0; for (ii = ntaps - 1; ii >= 0; ii--) { accum += h[ii] * z[state]; if (++state >= ntaps) { state = 0; } } *p_state = state; return accum; } ``` `fir_circular()`函数通过循环缓冲区实现了FIR滤波器,可以更高效地利用内存资源。 ##### 5. 交错FIR滤波器 ```c SAMPLE fir_shuffle(SAMPLE input, int ntaps, const SAMPLE h[], SAMPLE z[]) { int ii; SAMPLE accum; z[0] = input; accum = h[ntaps - 1] * z[ntaps - 1]; for (ii = ntaps - 2; ii >= 0; ii--) { accum += h[ii] * z[ii]; z[ii + 1] = z[ii]; } return accum; } ``` `fir_shuffle()`函数采用了交错的方法进行FIR滤波器计算,可以在一定程度上提高计算效率。 ##### 6. 分段FIR滤波器 ```c SAMPLE fir_split(SAMPLE input, int ntaps, const SAMPLE h[], SAMPLE z[], int *p_state) { int ii, end_ntaps, state = *p_state; SAMPLE accum; SAMPLE const *p_h; SAMPLE *p_z; accum = 0; p_h = h; p_z = z + state; *p_z = input; end_ntaps = ntaps - state; for (ii = 0; ii < end_ntaps; ii++) { accum += *p_h++ * *p_z++; } p_z = z; for (ii = 0; ii < state; ii++) { accum += *p_h++ * *p_z++; } if (--state < 0) { state += ntaps; } *p_state = state; return accum; } ``` `fir_split()`函数通过分段的方式实现了FIR滤波器,适用于处理较大的滤波器系数数组。 ##### 7. 双缓存FIR滤波器 ```c SAMPLE fir_double_z(SAMPLE input, int ntaps, const SAMPLE h[], SAMPLE z[], int *p_state) { SAMPLE accum; int ii, state = *p_state; SAMPLE const *p_h, *p_z; z[state] = z[state + ntaps] = input; // ... (省略部分代码) return accum; } ``` `fir_double_z()`函数使用了双缓存技术来提高FIR滤波器的效率,适用于需要高精度计算的应用场景。 #### 总结 以上代码展示了FIR滤波器在DSP中的几种不同的实现方法。每种方法都有其特点和适用场景,可以根据实际需求选择合适的方式来实现FIR滤波器。这些实现方法不仅能够帮助理解FIR滤波器的工作原理,也为进一步的研究和开发提供了基础。
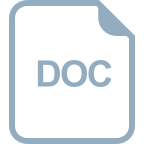
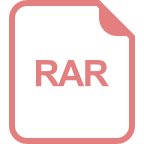
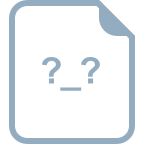
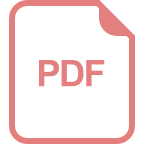
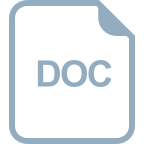
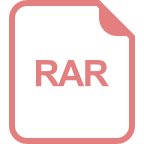
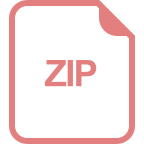
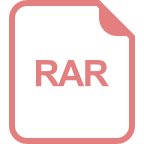
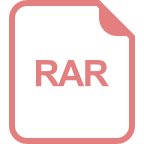
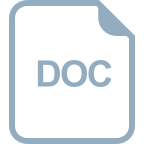
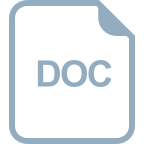
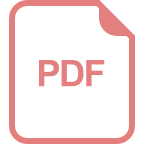
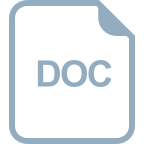
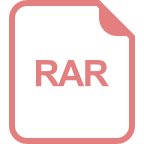
#ifdef WIN32
#include <conio.h>
#endif
#define SAMPLE double /* define the type used for data samples */
void clear(int ntaps, SAMPLE z[])
{
int ii;
for (ii = 0; ii < ntaps; ii++) {
z[ii] = 0;
}
}
SAMPLE fir_basic(SAMPLE input, int ntaps, const SAMPLE h[], SAMPLE z[])
{
int ii;
SAMPLE accum;
/* store input at the beginning of the delay line */
z[0] = input;
/* calc FIR */
accum = 0;
for (ii = 0; ii < ntaps; ii++) {
accum += h[ii] * z[ii];
}
for (ii = ntaps - 2; ii >= 0; ii--) {
z[ii + 1] = z[ii];
}
return accum;
}
SAMPLE fir_circular(SAMPLE input, int ntaps, const SAMPLE h[], SAMPLE z[],
int *p_state)
{
int ii, state;
SAMPLE accum;
state = *p_state; /* copy the filter's state to a local */
/* store input at the beginning of the delay line */
z[state] = input;
if (++state >= ntaps) { /* incr state and check for wrap */
state = 0;
}
/* calc FIR and shift data */
accum = 0;
for (ii = ntaps - 1; ii >= 0; ii--) {
accum += h[ii] * z[state];
if (++state >= ntaps) { /* incr state and check for wrap */
state = 0;
}
剩余8页未读,继续阅读

- 粉丝: 28
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

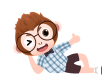
最新资源
- 学校课程软件工程常见10道题目以及答案demo
- javaweb新手开发中常见的目录结构讲解
- 新手小白的git使用的手册入门学习demo
- 基于Java观察者模式的info-express多对多广播通信框架设计源码
- 利用python爬取豆瓣电影评分简单案例demo
- 机器人开发中常见的几道问题以及答案demo
- 基于SpringBoot和layuimini的简洁美观后台权限管理系统设计源码
- 实验报告五六代码.zip
- hdw-dubbo-ui基于vue、element-ui构建开发,实现后台管理前端功能.zip
- (Grafana + Zabbix + ASP.NET Core 2.1 + ECharts + Dapper + Swagger + layuiAdmin)基于角色授权的权限体系.zip

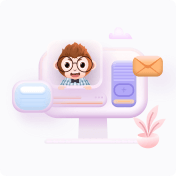

- 1
- 2
前往页