/*
* Copyright (c) 2008-2009 Apple Inc. All rights reserved.
*
* This document is the property of Apple Inc.
* It is considered confidential and proprietary.
*
* This document may not be reproduced or transmitted in any form,
* in whole or in part, without the express written permission of
* Apple Inc.
*/
#define TIME_TREE 0
#define AND_TRACE_LAYER FTL
#include "yaFTL_whoami.h"
#include "WMRConfig.h"
#include "ANDTypes.h"
#include "WMROAM.h"
#include "WMRBuf.h"
#include "VFLBuffer.h"
#include "VFL.h"
#include "yaFTLTypes.h"
#include "FTL.h"
#include "yaFTL_Defines.h"
#include "yaFTL_gc.h"
#include "yaFTL_BTOC.h"
#include "L2V/L2V_Extern.h"
#if TIME_TREE
#include <kern/clock.h>
#endif
#include "WMRFeatures.h"
#ifndef ENABLE_L2V_TREE
#error ENABLE_L2V_TREE must be set to 0 or 1 in WMRFeatures.h
#endif
#define kYaftlMinorVersion (1)
#if !NAND_RAW && !NAND_PPN
#error PPN or raw?
#endif
#ifdef AND_COLLECT_STATISTICS
FTLStatistics stFTLStatistics;
#endif /* AND_COLLECT_STATISTICS */
#define CXT_VER "CX01"
FTLWMRDeviceInfo yaFTL_FTLDeviceInfo;
VFLFunctions yaFTL_VFLFunctions;
yaFTL_t yaFTL;
static BOOL32 yaftl_init_done = FALSE32;
/* static functions declaration section */
static void YAFTL_FreeMemory(void);
static Int32 YAFTL_Init(VFLFunctions *pVFLFunctions);
static Int32 YAFTL_Open(UInt32 *pTotalScts,
UInt32 * pdwSectorSize,
BOOL32 nandFullRestore,
BOOL32 justFormatted,
UInt32 dwMinorVer,
UInt32 dwOptions);
static Int32 YAFTL_Read(UInt32 nLpn, UInt32 nNumOfScts,
UInt8 *pBuf);
static void YAFTL_Close(void);
static BOOL32 YAFTL_GetStruct(UInt32 dwStructType,
void * pvoidStructBuffer,
UInt32 * pdwStructSize);
Int32 _readPage(UInt32 vpn, UInt8 *pageData,
PageMeta_t *pMeta,
BOOL32 bInternalOp, BOOL32 boolCleanCheck, BOOL32 scrubOnUECC);
BOOL32 _readMultiPages(UInt32 * padwVpn,
UInt16 wNumPagesToRead, UInt8 * pbaData,
UInt8 * pbaSpare,
BOOL32 bInternalOp, BOOL32 scrubOnUECC);
#if ENABLE_L2V_TREE
static void PopulateFromIndexCache(UInt32 indexPageNo, UInt32 *indexPageData);
#endif //ENABLE_L2V_TREE
static void PopulateTreesOnBoot_Fast(void);
#ifndef AND_READONLY
static Int32 YAFTL_Write(UInt32 nLpn, UInt32 nNumOfScts,
UInt8 *pBuf, BOOL32 isStatic);
static Int32 YAFTL_Format(UInt32 dwOptions);
static Int32 YAFTL_WearLevel(void);
static BOOL32 YAFTL_GarbageCollect(void);
static BOOL32 YAFTL_ShutdownNotify(BOOL32 boolMergeLogs);
Int32 _writePage(UInt32 vpn, UInt8 *pageData,
PageMeta_t *,
BOOL32 bInternalOp);
static ANDStatus writeIndexPage(UInt8 *pageBuffer, PageMeta_t *mdPtr,
UInt8 flag);
UInt32 _allocateBlock(UInt32 blockNo, UInt8 flag, UInt8 type);
BOOL32 _writeMultiPages(UInt16 vbn, UInt16 pageOffset,
UInt16 wNumPagesToWrite, UInt8 *pageData,
PageMeta_t *mdPtr,
BOOL32 bInternalOp);
UInt32* IndexLoadDirty(UInt32 lba, UInt32 *indexOfs);
UInt32* IndexLoadClean(UInt32 lba, UInt32 *indexOfs, UInt32 *d_tocEntry);
void IndexMarkDirty(UInt32 tocEntry);
ANDStatus invalidateCXT(void);
BOOL32 isBlockInEraseNowList(UInt16 blockNo);
BOOL32 removeBlockFromEraseNowList(UInt16 blockNo);
BOOL32 addBlockToEraseNowList(UInt16 blockNo);
#endif // ! AND_READONLY
/*
static variables/structures declaration section
*/
typedef struct blockList
{
WeaveSeq_t weaveSeq;
UInt16 blockNo;
struct blockList *next;
struct blockList *prev;
} BlockListType;
typedef struct BlockRange
{
UInt32 start;
UInt32 end;
} BlockRangeType;
#if NAND_PPN
typedef struct YAFTLControlInfo
{
UInt32 versionNo;
UInt32 indexSize; /* index size in blocks */
UInt32 logicalPartitionSize; /* partititon size in pages exposed to file system */
UInt32 wrState_data_block; /* current physical block being used for updates */
UInt32 wrState_data_weaveSeq_Lo;
UInt32 wrState_data_weaveSeq_Hi;
UInt32 wrState_index_block; /* current physical block being used for updates */
UInt32 wrState_index_weaveSeq_Lo;
UInt32 wrState_index_weaveSeq_Hi;
UInt32 seaState_data_freeBlocks; /* number of free ( erased ) blocks */
UInt32 seaState_index_freeBlocks; /* number of free ( erased ) blocks */
UInt32 seaState_data_allocdBlocks;
UInt32 seaState_index_allocdBlocks;
UInt32 indexCacheSize;
UInt32 FTLRestoreCnt;
UInt32 reserved32[10];
UInt16 TOCtableEntriesNo; /* number of entries in index TOC table */
UInt16 controlPageNo;
UInt16 indexPageRatio; /* TOC of each block contains Lpa for each page written to block */
UInt16 freeCachePages;
UInt16 nextFreeCachePage;
UInt16 wrState_data_nextPage; /* offset in current block of next free page */
UInt16 wrState_index_nextPage; /* offset in current block of next free page */
UInt32 reserved16[10];
UInt8 exportedRatio;
UInt8 cxtEraseCounter;
UInt8 reserved8[10];
} YAFTLControlInfoType;
#elif NAND_RAW
typedef struct YAFTLControlInfo
{
UInt32 versionNo;
UInt32 indexSize; /* index size in blocks */
UInt32 logicalPartitionSize; /* partititon size in pages exposed to file system */
UInt32 wrState_data_block; /* current physical block being used for updates */
UInt32 wrState_data_weaveSeq;
UInt32 wrState_index_block; /* current physical block being used for updates */
UInt32 wrState_index_weaveSeq;
UInt32 seaState_data_freeBlocks; /* number of free ( erased ) blocks */
UInt32 seaState_index_freeBlocks; /* number of free ( erased ) blocks */
UInt32 seaState_data_allocdBlocks;
UInt32 seaState_index_allocdBlocks;
UInt32 indexCacheSize;
UInt32 FTLRestoreCnt;
UInt32 reserved32[10];
UInt16 TOCtableEntriesNo; /* number of entries in index TOC table */
UInt16 controlPageNo;
UInt16 indexPageRatio; /* TOC of each block contains Lpa for each page written to block */
UInt16 freeCachePages;
UInt16 nextFreeCachePage;
UInt16 wrState_data_nextPage; /* offset in current block of next free page */
UInt16 wrState_index_nextPage; /* offset in current block of next free page */
UInt32 reserved16[10];
UInt8 exportedRatio;
UInt8 cxtEraseCounter;
UInt8 reserved8[10];
} YAFTLControlInfoType;
#else
#error PPN or raw?
#endif
static BOOL32 _allocateRestoreIndexBuffers(BlockRangeType **blockRangeBuffer, UInt32 **indexTable, UInt32 *indexTableSize);
#ifdef AND_COLLECT_STATISTICS
#ifndef AND_READONLY
static BOOL32 _FTLGetStatisticsToCxt(UInt8 * pabData);
#endif //ifndef AND_READONLY
static BOOL32 _FTLSetStatisticsFromCxt(UInt8 * pabData);
#endif
#ifndef AND_READONLY
static BOOL32 YAFTL_WriteStats(void);
#define CXT_ERASE_GAP 50
#define MOVE_CXT_TRESHOLD 1000
#define ERASE_CXT_UPDATE_THRESHOLD (FTL_AREA_SIZE>>4)
#define WRITE_CXT_UPDATE_THRESHOLD (USER_PAGES_TOTAL>>4)
#endif
static UInt32 tempIndexBufferSize = 0;
/*****************************************************************************/
/* Code Implementation */
/*****************************************************************************/
void freeBlockList(BlockListType * listHead)
{
BlockListType * tmpPtr = listHead;
while(tmpPtr != NULL)
{
listHea
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
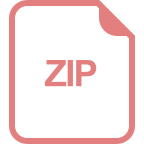
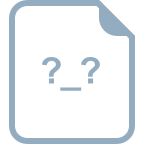
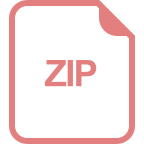
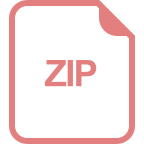
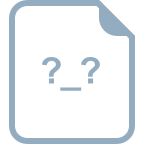
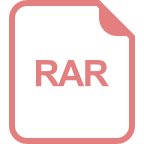
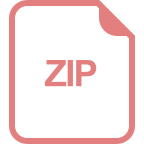
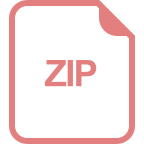
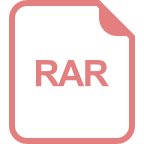
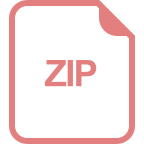
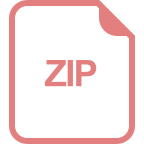
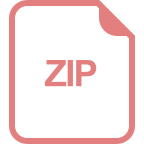
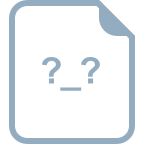
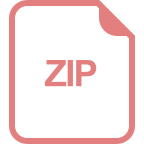
收起资源包目录

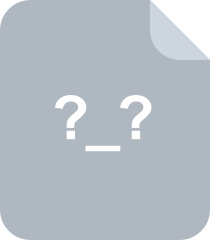
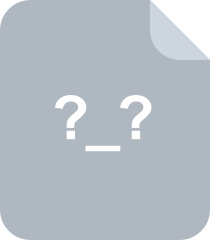
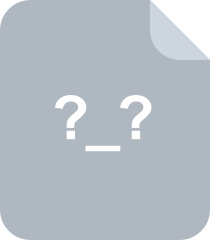
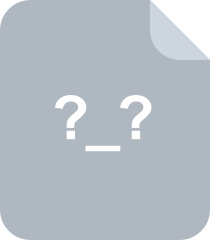
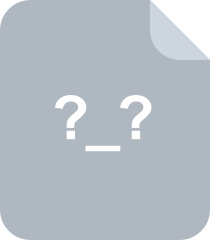
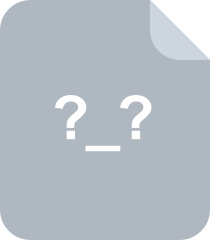
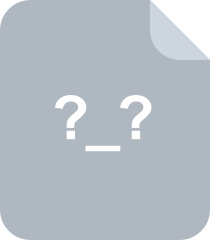
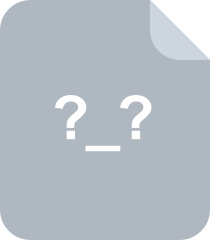
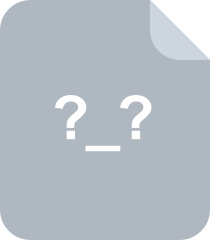
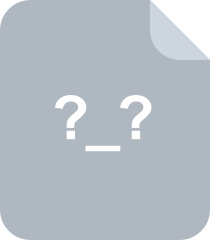
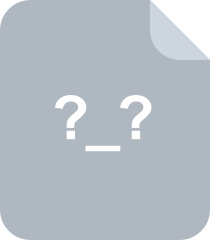
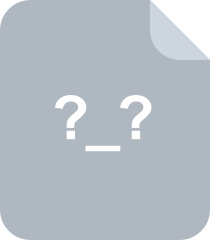
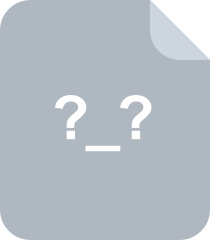
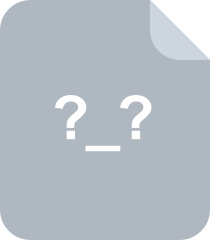
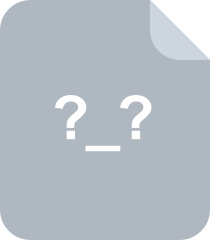
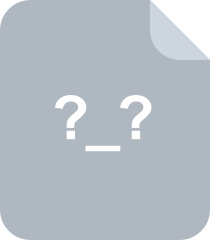
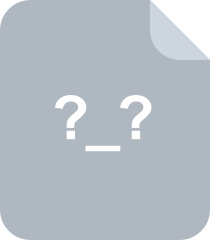
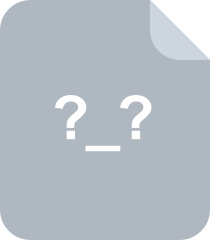
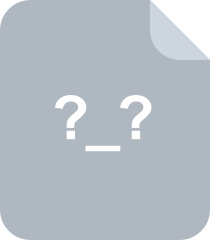
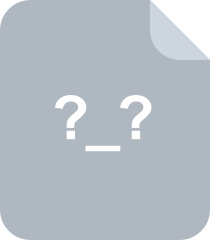
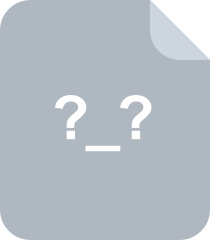
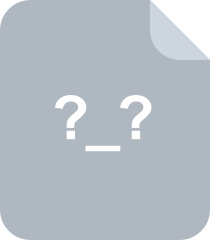
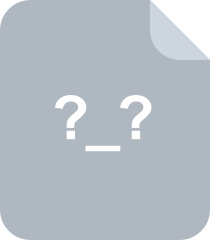
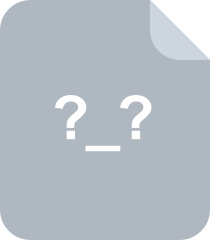
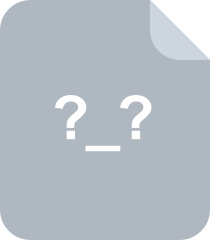
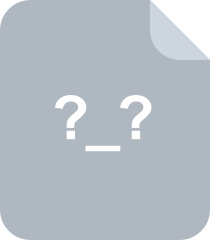
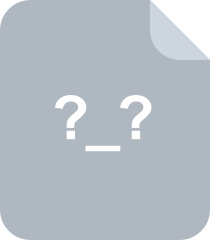
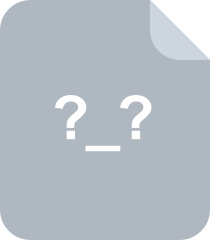
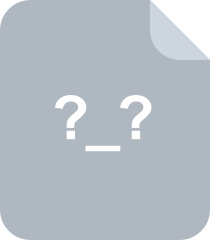
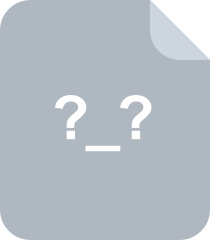
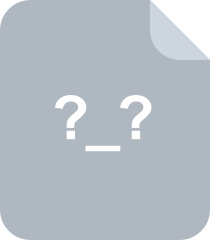
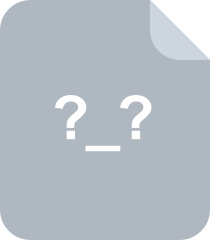
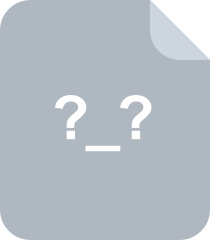
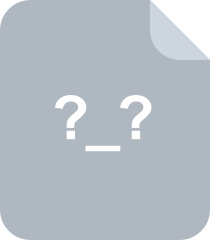
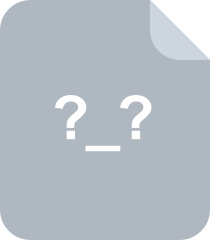
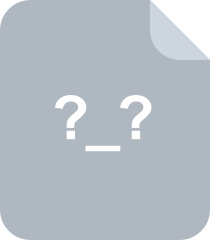
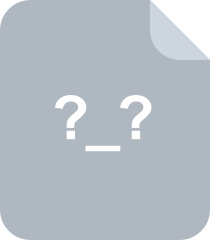
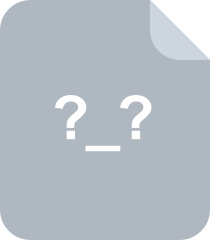
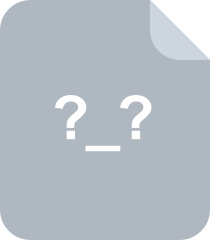
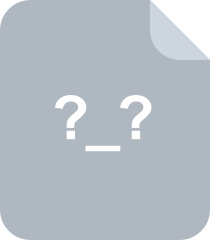
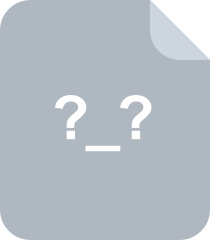
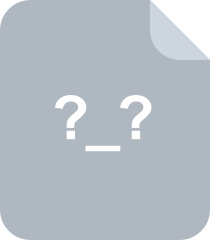
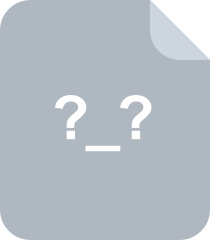
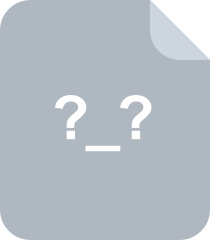
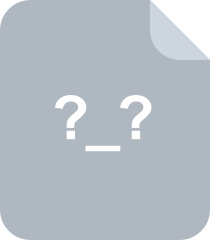
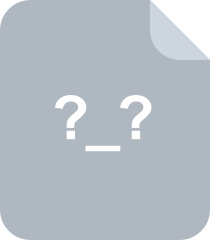
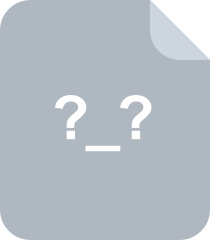
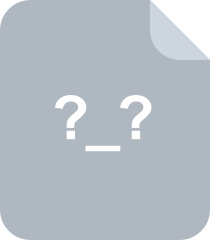
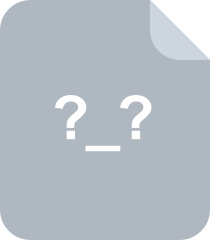
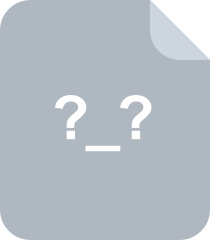
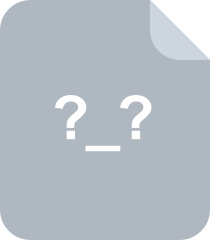
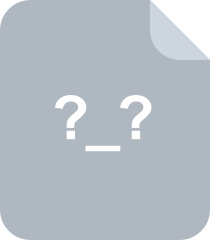
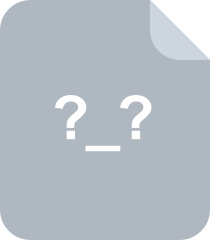
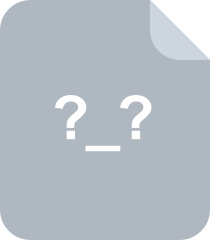
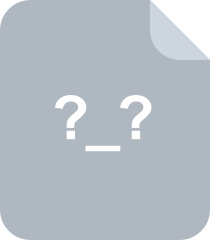
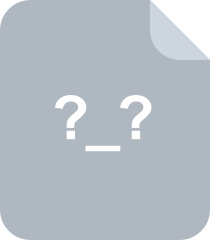
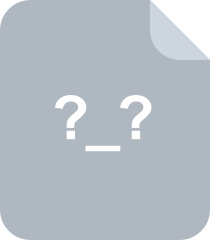
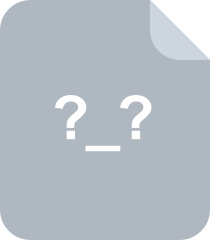
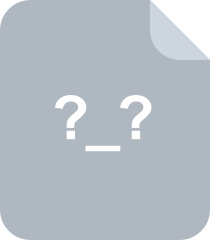
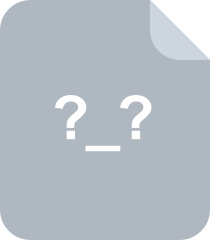
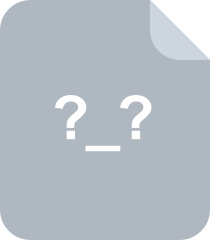
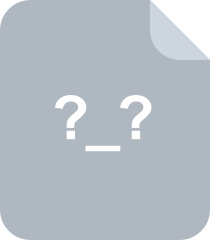
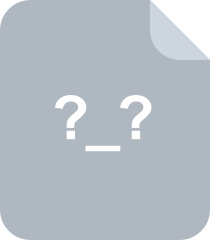
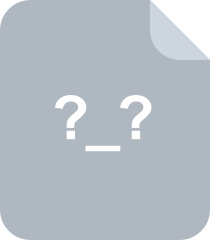
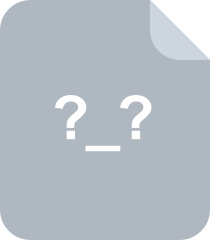
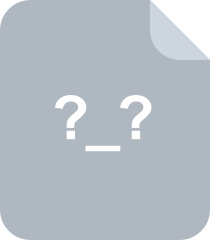
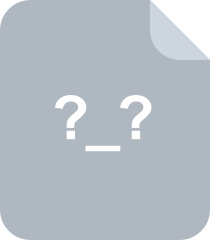
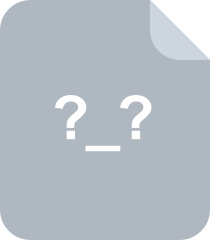
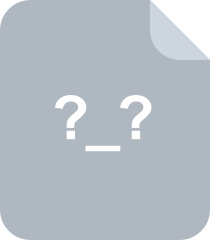
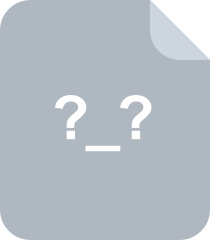
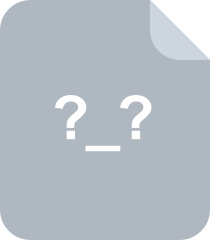
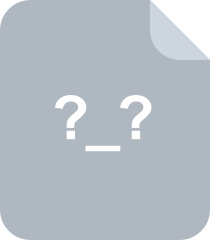
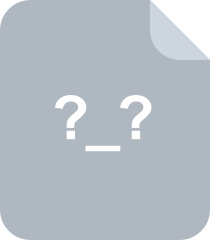
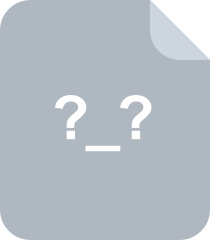
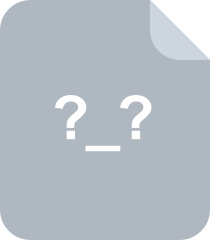
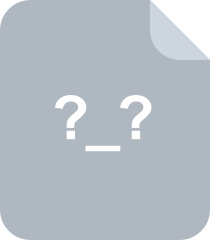
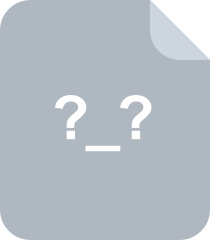
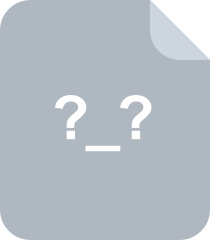
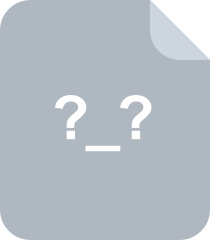
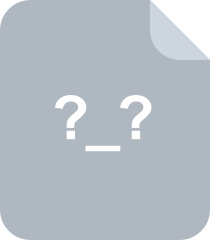
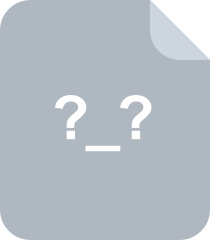
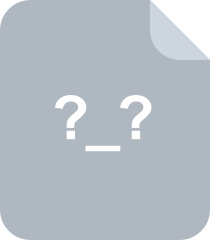
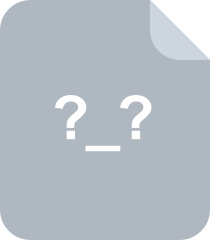
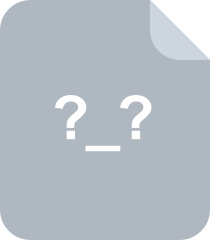
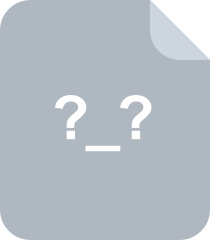
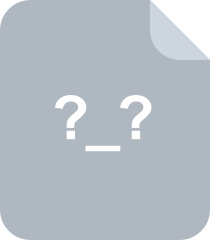
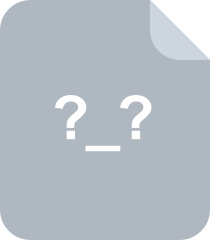
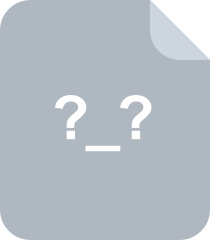
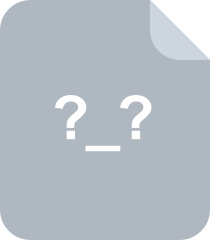
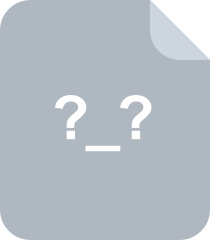
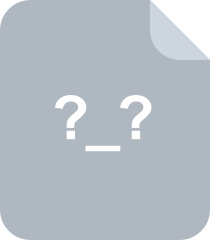
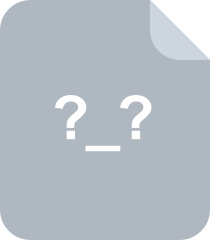
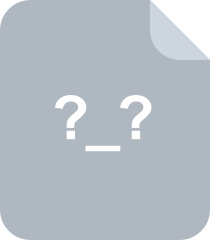
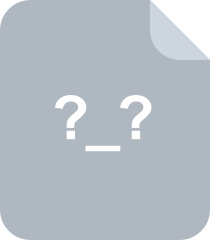
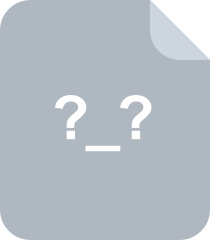
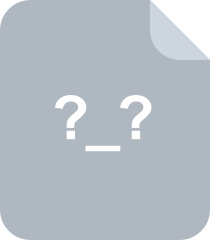
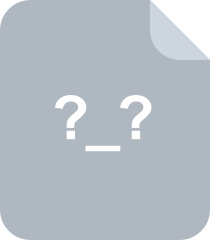
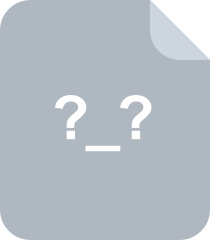
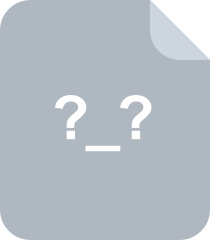
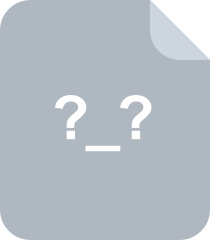
共 2612 条
- 1
- 2
- 3
- 4
- 5
- 6
- 27

创新境界
- 粉丝: 61
- 资源: 6
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

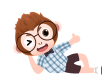
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
前往页