package userUI;
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.MouseAdapter;
import org.eclipse.swt.events.MouseEvent;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Combo;
import org.eclipse.swt.widgets.Composite;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.TabFolder;
import org.eclipse.swt.widgets.TabItem;
import org.eclipse.swt.widgets.Table;
import org.eclipse.swt.widgets.TableColumn;
import org.eclipse.swt.widgets.TableItem;
import org.eclipse.swt.widgets.Text;
import com.swtdesigner.SWTResourceManager;
import dbController.ScheduleTabController;
import dbController.StuScheduleBean;
import dbController.ClassTab1Ctrl;
import dbController.TeacherScheduleBean;
import dbController.RoomInfoController;
import dbController.AdjustController;
import java.util.*;
public class AdjustUI {
private Text text_4;
private Combo combo_9;
private Combo combo_8;
private Combo combo_7;
private Combo combo_6;
private Combo combo_5;
private Table table_2;
private Table table_1;
private Text text_3;
private Text text_2;
private Combo combo_4;
private Combo combo_3;
private Text text_1;
private Table table;
private Combo combo_2;
private Combo combo_1;
private Combo combo;
private Text text;
protected Shell shell;
private CountTimer countTimer=new CountTimer();
/**
* Launch the application
* @param args
*/
public static void main(String[] args) {
try {
AdjustUI window = new AdjustUI();
window.open();
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* Open the window
*/
public void open() {
final Display display = Display.getDefault();
createContents();
shell.open();
shell.layout();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
}
/**
* Create contents of the window
*/
protected void createContents() {
shell = new Shell(SWT.MIN);
shell.setSize(777, 523);
shell.setText("排课系统-调整课程");
final TabFolder tabFolder = new TabFolder(shell, SWT.NONE);
tabFolder.setBounds(10, 80, 751, 387);
final TabItem tabItem = new TabItem(tabFolder, SWT.NONE);
tabItem.setText("班级");
final Composite composite_1 = new Composite(tabFolder, SWT.NONE);
final Composite composite_2 = new Composite(composite_1, SWT.BORDER);
composite_2.setBounds(0, 10, 721, 64);
final Composite composite_3 = new Composite(composite_1, SWT.BORDER);
composite_3.setBounds(0, 80, 733, 272);
final Label label = new Label(composite_2, SWT.NONE);
label.setFont(SWTResourceManager.getFont("华文新魏", 12, SWT.NONE));
label.setAlignment(SWT.CENTER);
label.setText("查找班级");
label.setBounds(10, 0, 71, 19);
final Composite composite_4 = new Composite(composite_2, SWT.BORDER);
composite_4.setBounds(91, 12, 198, 42);
final Label label_1 = new Label(composite_4, SWT.NONE);
label_1.setText("班号");
label_1.setBounds(10, 10, 39, 28);
text = new Text(composite_4, SWT.BORDER);
text.setBounds(55, 7, 101, 25);
final Composite composite_5 = new Composite(composite_2, SWT.BORDER);
composite_5.setBounds(289, 12, 422, 42);
final Label label_2 = new Label(composite_5, SWT.NONE);
label_2.setText("年级");
label_2.setBounds(144, 10, 40, 22);
combo = new Combo(composite_5, SWT.NONE);
combo.addMouseListener(new MouseAdapter() {
public void mouseUp(final MouseEvent e) {
String[] classID=new ClassTab1Ctrl().getClassID(combo.getText(),combo_1.getText());
combo_2.setItems(classID);
}
});
combo.setItems(new String[] {"大一", "大二", "大三", "大四"});
combo.setBounds(192, 7, 72, 25);
final Label label_3 = new Label(composite_5, SWT.NONE);
label_3.setText("专业");
label_3.setBounds(10, 7, 40, 22);
combo_1 = new Combo(composite_5, SWT.NONE);
combo_1.setItems(new String[] {"计算机科学与技术", "网络工程", "软件工程"});
combo_1.setBounds(56, 7, 72, 25);
final Label label_4 = new Label(composite_5, SWT.NONE);
label_4.setText("班号");
label_4.setBounds(290, 10, 40, 22);
combo_2 = new Combo(composite_5, SWT.NONE);
combo_2.setBounds(336, 7, 72, 25);
final TableItem[][] item=new TableItem[4][4];
final TableItem[] item1=new TableItem[4];
final Label label_6 = new Label(composite_3, SWT.NONE);
label_6.setAlignment(SWT.CENTER);
label_6.setBackground(SWTResourceManager.getColor(255, 128, 128));
label_6.setText("当前没有可显示项");
label_6.setBounds(84, 0, 645, 18);
final Button button = new Button(composite_2, SWT.NONE);
button.addMouseListener(new MouseAdapter() {
public void mouseUp(final MouseEvent e) {
String classID=null;
if(combo_2.getText()!=null||text.getText()!=null){
if(text.getText()!=""){
classID=text.getText();
}else{
classID=combo_2.getText();
}
}else{
label_6.setText("请输入正确的班号");
}
StuScheduleBean[] scheduleInfo=new ScheduleTabController().searchStuSchedule(classID);
for(int i=0;i<4;i++){
for(int j=0;j<4;j++){
item[i][j]=new TableItem(table,SWT.SINGLE);
if(j==0){
item[i][j].setText(0,"第"+(i+1)+"大节");
item[i][j].setText(1,scheduleInfo[0*4+i].getCourseName());
item[i][j].setText(2,scheduleInfo[1*4+i].getCourseName());
item[i][j].setText(3,scheduleInfo[2*4+i].getCourseName());
item[i][j].setText(4,scheduleInfo[3*4+i].getCourseName());
item[i][j].setText(5,scheduleInfo[4*4+i].getCourseName());
}else
if(j==1){
item[i][j].setText(0," ");
item[i][j].setText(1,scheduleInfo[0*4+i].getTeacherName());
item[i][j].setText(2,scheduleInfo[1*4+i].getTeacherName());
item[i][j].setText(3,scheduleInfo[2*4+i].getTeacherName());
item[i][j].setText(4,scheduleInfo[3*4+i].getTeacherName());
item[i][j].setText(5,scheduleInfo[4*4+i].getTeacherName());
}else
if(j==2){
String[] totalWeek=new String[5];
for(int k=0;k<5;k++){
if(scheduleInfo[k*4+i].getTotalWeek()!=0){
totalWeek[k]=" 共"+scheduleInfo[k*4+i].getTotalWeek()+"周";
}else{
totalWeek[k]=" ";
}
}
item[i][j].setText(0," ");
item[i][j].setText(1,scheduleInfo[0*4+i].getRoomID()+totalWeek[0]);
item[i][j].setText(2,scheduleInfo[1*4+i].getRoomID()+totalWeek[1]);
item[i][j].setText(3,scheduleInfo[2*4+i].getRoomID()+totalWeek[2]);
item[i][j].setText(4,scheduleInfo[3*4+i].getRoomID()+totalWeek[3]);
item[i][j].setText(5,scheduleInfo[4*4+i].getRoomID()+totalWeek[4]);
}else{
item[i][j].setText(0," ");
item[i][j].setText(1," ");
item[i][j].setText(2," ");
item[i][j].setText(3," ");
item[i][j].setText(4," ");
item[i][j].setText(5," ");
}
}
}
label_6.setText(classID+"班的课表:");
}
});
button.setFont(SWTResourceManager.getFont("楷体_GB2312", 14, SWT.NONE));
button.setText("搜");
button.setBounds(10, 25, 71, 29);
table = new Table(composite_3, SWT.BORDER);
table.setLinesVisible(true);
table.setHeaderVisible(true);
table.setBounds(0, 25, 719, 243);
final TableColumn newColumnTableColumn = new TableColumn(table, SWT.NONE);
newColumnTableColumn.setWidth(50);
newColumnTableColumn.setText("时间");
final TableColumn newColumnTableColumn_1 = new TableColumn(table, SWT.NONE);
newColumnTableColumn_1.setWidth(130);
newColumnTableColumn_1.setText("星期一");
final TableColumn newColumnTableColumn_2 = new TableColumn(table, SWT.NONE);
newColumnTableColumn_2.setWidth(130);
newColumnTableColumn_2.setText("星期二");
final Tabl
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于ECLIPSE + SWT Designer平台开发的java的排课系统,数据库采用的sql server2000,采用odbc-jdbc桥的方式连接数据库!补充:有楼友说没有界面,本系统界面是在Eclipse下基于SWTDesigner插件开发的,请先安装该插件;有楼友说没有数据库,那么MDF和LDF文件干嘛用的呢?
资源推荐
资源详情
资源评论
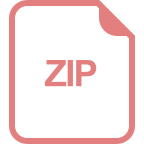
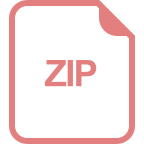
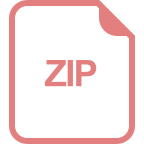
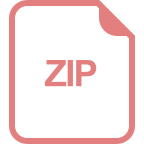
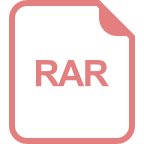
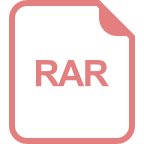
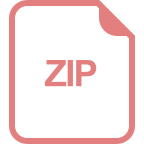
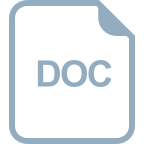
收起资源包目录

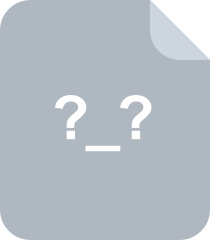
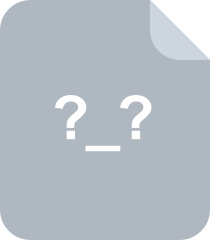




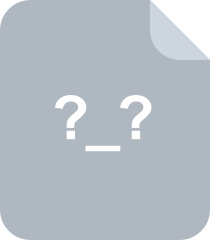

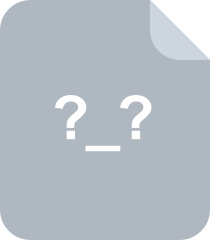
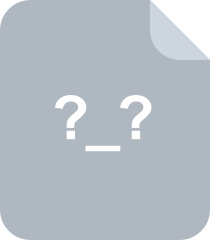
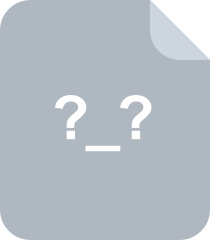
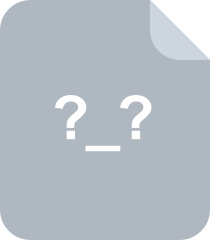
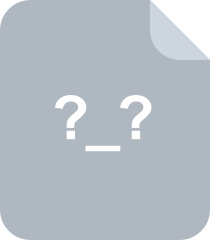
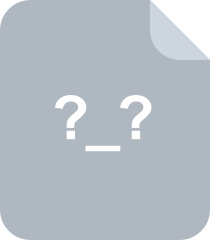
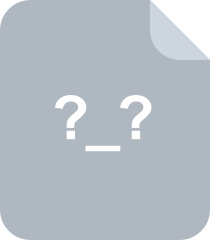
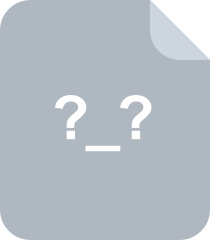
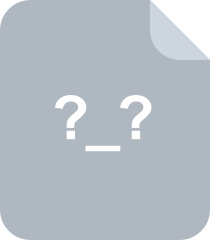
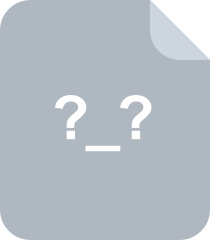
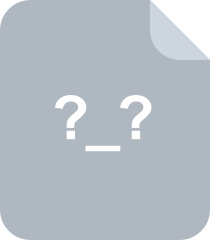
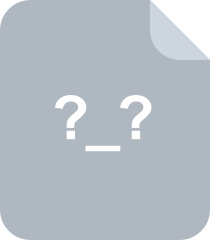
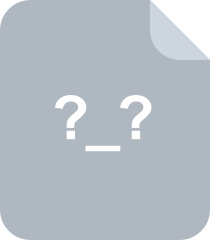
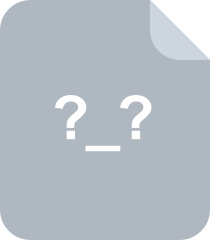

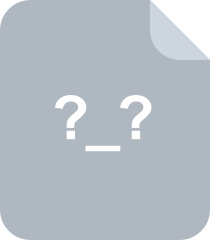
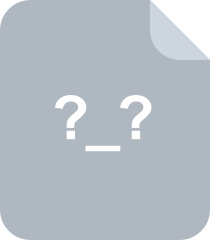
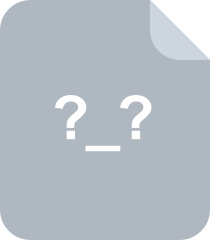
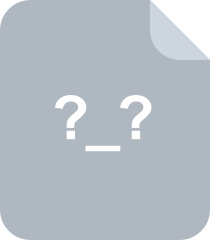
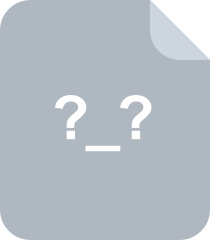
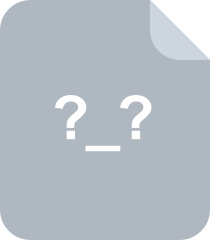
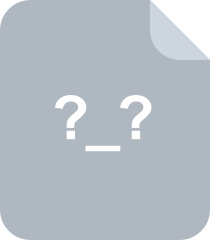



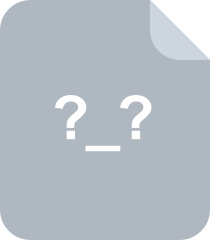
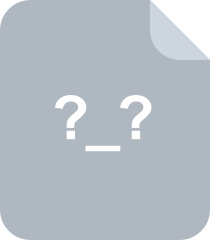

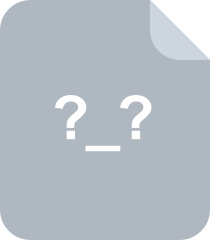
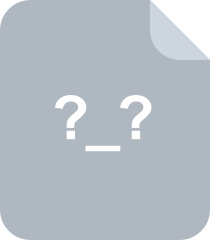
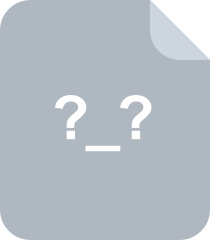
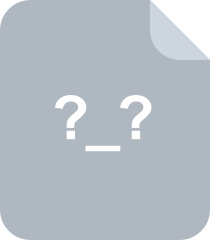
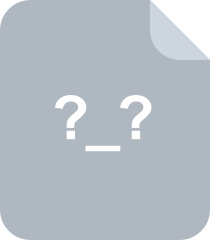
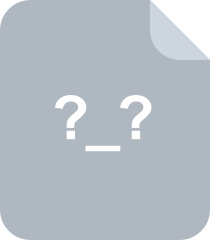
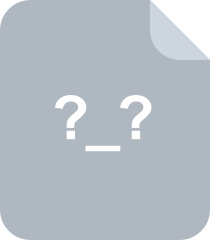
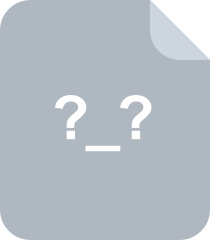
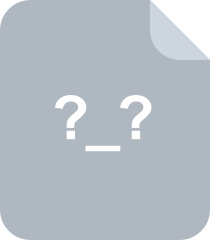
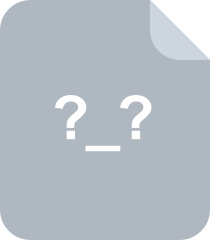
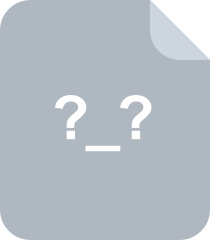
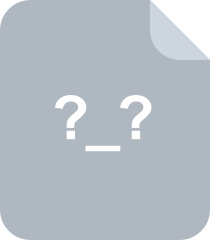
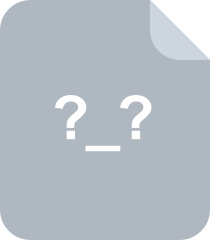
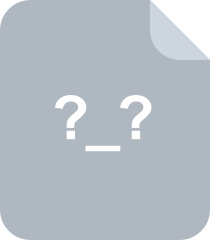

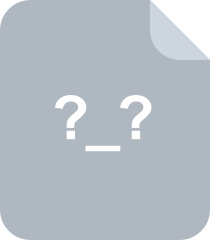
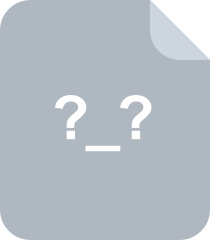
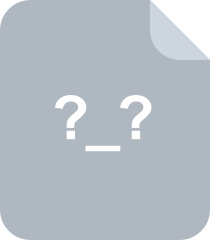
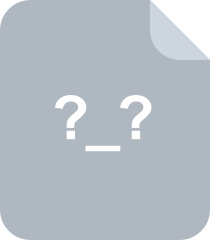
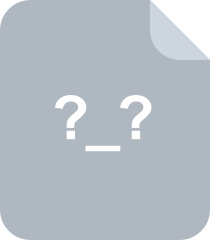
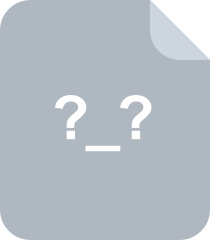
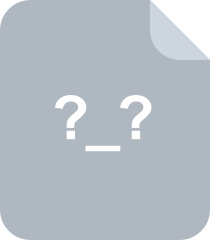
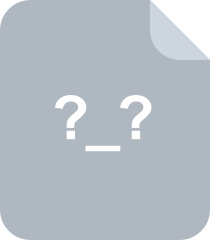
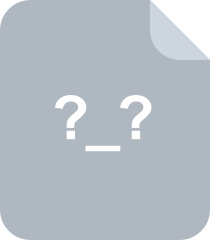
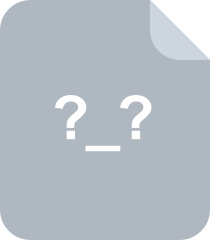
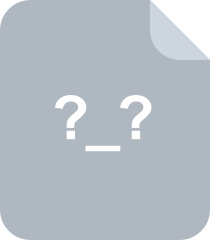
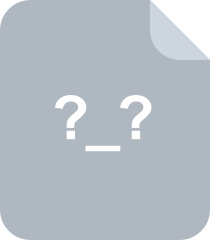
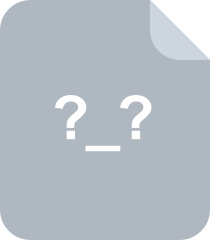
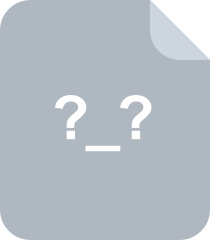
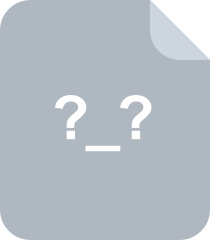
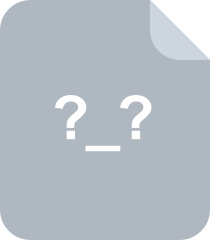
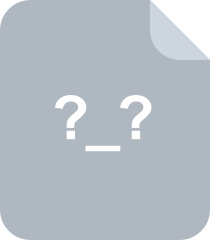
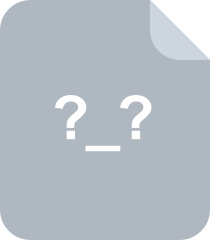
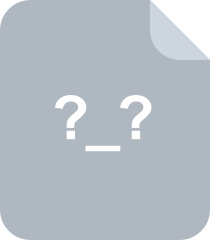
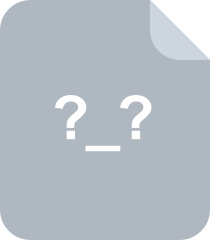
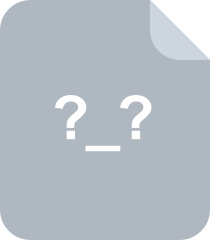
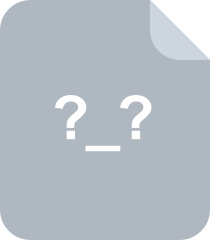
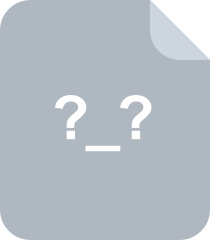
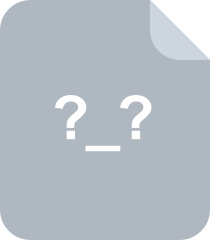
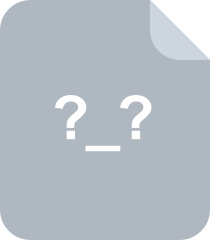
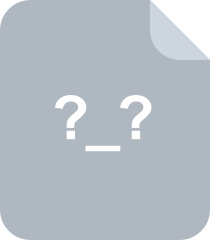
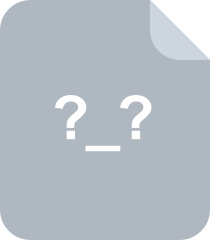
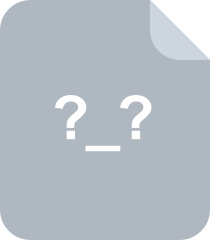
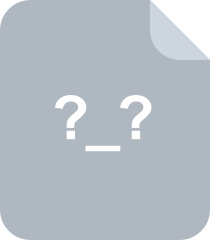
共 69 条
- 1

ikeycn
- 粉丝: 3
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

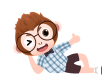
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


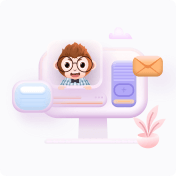
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
前往页