/*
* Copyright (C) 2007-2013 Geometer Plus <contact@geometerplus.com>
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301, USA.
*/
package org.geometerplus.zlibrary.text.view;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.Set;
import java.util.TreeSet;
import org.geometerplus.zlibrary.core.application.ZLApplication;
import org.geometerplus.zlibrary.core.filesystem.ZLFile;
import org.geometerplus.zlibrary.core.util.ZLColor;
import org.geometerplus.zlibrary.core.view.ZLPaintContext;
import org.geometerplus.zlibrary.text.hyphenation.ZLTextHyphenationInfo;
import org.geometerplus.zlibrary.text.hyphenation.ZLTextHyphenator;
import org.geometerplus.zlibrary.text.model.ZLTextAlignmentType;
import org.geometerplus.zlibrary.text.model.ZLTextMark;
import org.geometerplus.zlibrary.text.model.ZLTextModel;
import org.geometerplus.zlibrary.text.model.ZLTextParagraph;
import org.geometerplus.zlibrary.text.view.style.ZLTextStyleCollection;
public abstract class ZLTextView extends ZLTextViewBase {
public static final int MAX_SELECTION_DISTANCE = 10;
public interface ScrollingMode {
int NO_OVERLAPPING = 0;
int KEEP_LINES = 1;
int SCROLL_LINES = 2;
int SCROLL_PERCENTAGE = 3;
};
private ZLTextModel myModel;
private interface SizeUnit {
int PIXEL_UNIT = 0;
int LINE_UNIT = 1;
};
private int myScrollingMode;
private int myOverlappingValue;
private ZLTextPage myPreviousPage = new ZLTextPage();
private ZLTextPage myCurrentPage = new ZLTextPage();
private ZLTextPage myNextPage = new ZLTextPage();
private final HashMap<ZLTextLineInfo,ZLTextLineInfo> myLineInfoCache = new HashMap<ZLTextLineInfo,ZLTextLineInfo>();
private ZLTextRegion.Soul mySelectedRegionSoul;
private boolean myHighlightSelectedRegion = true;
private final ZLTextSelection mySelection = new ZLTextSelection(this);
private final Set<ZLTextHighlighting> myHighlightings =
Collections.synchronizedSet(new TreeSet<ZLTextHighlighting>());
public ZLTextView(ZLApplication application) {
super(application);
}
public synchronized void setModel(ZLTextModel model) {
ZLTextParagraphCursorCache.clear();
mySelection.clear();
myHighlightings.clear();
myModel = model;
myCurrentPage.reset();
myPreviousPage.reset();
myNextPage.reset();
if (myModel != null) {
final int paragraphsNumber = myModel.getParagraphsNumber();
if (paragraphsNumber > 0) {
myCurrentPage.moveStartCursor(ZLTextParagraphCursor.cursor(myModel, 0));
}
}
Application.getViewWidget().reset();
}
public ZLTextModel getModel() {
return myModel;
}
public ZLTextWordCursor getStartCursor() {
if (myCurrentPage.StartCursor.isNull()) {
preparePaintInfo(myCurrentPage);
}
return myCurrentPage.StartCursor;
}
public ZLTextWordCursor getEndCursor() {
if (myCurrentPage.EndCursor.isNull()) {
preparePaintInfo(myCurrentPage);
}
return myCurrentPage.EndCursor;
}
private synchronized void gotoMark(ZLTextMark mark) {
if (mark == null) {
return;
}
myPreviousPage.reset();
myNextPage.reset();
boolean doRepaint = false;
if (myCurrentPage.StartCursor.isNull()) {
doRepaint = true;
preparePaintInfo(myCurrentPage);
}
if (myCurrentPage.StartCursor.isNull()) {
return;
}
if (myCurrentPage.StartCursor.getParagraphIndex() != mark.ParagraphIndex ||
myCurrentPage.StartCursor.getMark().compareTo(mark) > 0) {
doRepaint = true;
gotoPosition(mark.ParagraphIndex, 0, 0);
preparePaintInfo(myCurrentPage);
}
if (myCurrentPage.EndCursor.isNull()) {
preparePaintInfo(myCurrentPage);
}
while (mark.compareTo(myCurrentPage.EndCursor.getMark()) > 0) {
doRepaint = true;
scrollPage(true, ScrollingMode.NO_OVERLAPPING, 0);
preparePaintInfo(myCurrentPage);
}
if (doRepaint) {
if (myCurrentPage.StartCursor.isNull()) {
preparePaintInfo(myCurrentPage);
}
Application.getViewWidget().reset();
Application.getViewWidget().repaint();
}
}
public synchronized void gotoHighlighting(ZLTextHighlighting highlighting) {
myPreviousPage.reset();
myNextPage.reset();
boolean doRepaint = false;
if (myCurrentPage.StartCursor.isNull()) {
doRepaint = true;
preparePaintInfo(myCurrentPage);
}
if (myCurrentPage.StartCursor.isNull()) {
return;
}
if (!highlighting.intersects(myCurrentPage)) {
gotoPosition(highlighting.getStartPosition().getParagraphIndex(), 0, 0);
preparePaintInfo(myCurrentPage);
}
if (myCurrentPage.EndCursor.isNull()) {
preparePaintInfo(myCurrentPage);
}
while (!highlighting.intersects(myCurrentPage)) {
doRepaint = true;
scrollPage(true, ScrollingMode.NO_OVERLAPPING, 0);
preparePaintInfo(myCurrentPage);
}
if (doRepaint) {
if (myCurrentPage.StartCursor.isNull()) {
preparePaintInfo(myCurrentPage);
}
Application.getViewWidget().reset();
Application.getViewWidget().repaint();
}
}
public synchronized int search(final String text, boolean ignoreCase, boolean wholeText, boolean backward, boolean thisSectionOnly) {
if (text.length() == 0) {
return 0;
}
int startIndex = 0;
int endIndex = myModel.getParagraphsNumber();
if (thisSectionOnly) {
// TODO: implement
}
int count = myModel.search(text, startIndex, endIndex, ignoreCase);
myPreviousPage.reset();
myNextPage.reset();
if (!myCurrentPage.StartCursor.isNull()) {
rebuildPaintInfo();
if (count > 0) {
ZLTextMark mark = myCurrentPage.StartCursor.getMark();
gotoMark(wholeText ?
(backward ? myModel.getLastMark() : myModel.getFirstMark()) :
(backward ? myModel.getPreviousMark(mark) : myModel.getNextMark(mark)));
}
Application.getViewWidget().reset();
Application.getViewWidget().repaint();
}
return count;
}
public boolean canFindNext() {
final ZLTextWordCursor end = myCurrentPage.EndCursor;
return !end.isNull() && (myModel != null) && (myModel.getNextMark(end.getMark()) != null);
}
public synchronized void findNext() {
final ZLTextWordCursor end = myCurrentPage.EndCursor;
if (!end.isNull()) {
gotoMark(myModel.getNextMark(end.getMark()));
}
}
public boolean canFindPrevious() {
final ZLTextWordCursor start = myCurrentPage.StartCursor;
return !start.isNull() && (myModel != null) && (myModel.getPreviousMark(start.getMark()) != null);
}
public synchronized void findPrevious() {
final ZLTextWordCursor start = myCurrentPage.StartCursor;
if (!start.isNull()) {
gotoMark(myModel.getPreviousMark(start.getMark()));
}
}
public void clearFindResults() {
if (!findResultsAreEmpty()) {
myModel.removeAllMarks();
rebuildPaintInfo();
Application.getViewWidget().reset();
Application.getViewWidget().repaint();
}
}
public boolean findResultsAreEmpty() {
return (myModel == null) || myModel.getMarks().isEmpty();
}
@Override
public synchronized void onScrollingFinished(PageIndex pageIndex) {
switch (pageIndex) {
case current:
break;
case previous:
{
final ZLTextPage swap = myNextPage;
myNextPage = myCurrentPage;
myCurrentPage = myPreviousPage;
myPreviousPage = swap;
myPreviousPage.reset();
if (myCurrentPage.PaintState == PaintStateEnum.NOTHING_TO_PAINT) {
preparePaintInfo(myNextPage);
没有合适的资源?快使用搜索试试~ 我知道了~
android 阅读器
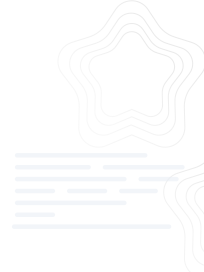
共1963个文件
class:781个
java:449个
png:374个

需积分: 10 1 下载量 186 浏览量
2022-07-25
20:58:12
上传
评论 1
收藏 8.79MB ZIP 举报
温馨提示
android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器android 阅读器
资源详情
资源评论
资源推荐
收起资源包目录

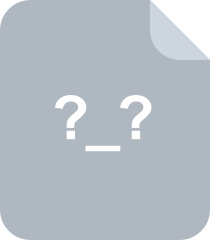
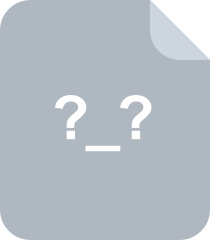
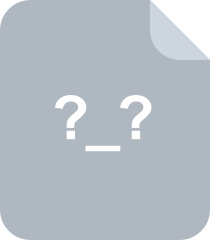
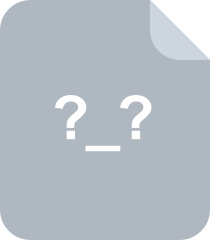
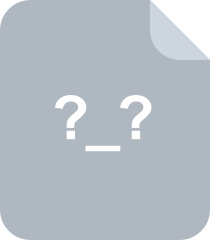
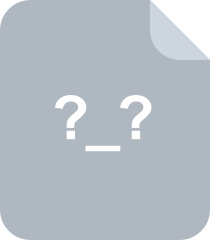
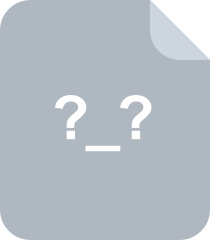
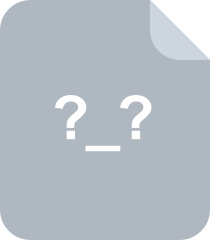
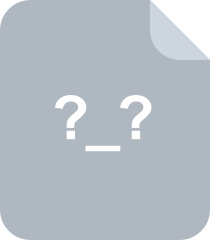
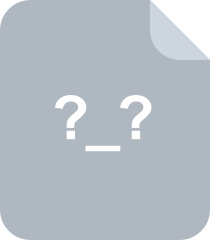
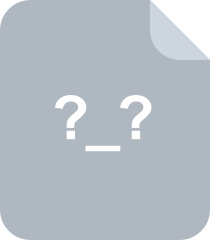
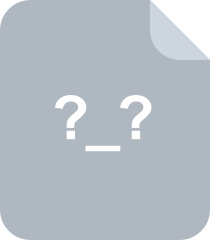
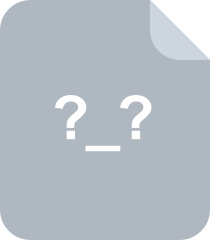
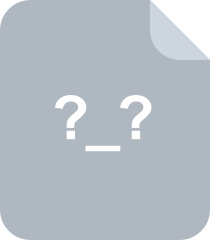
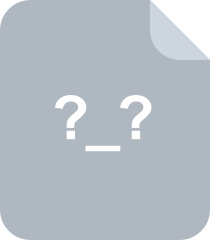
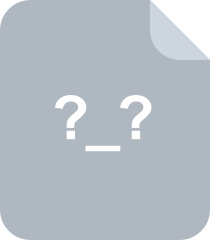
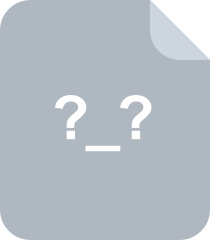
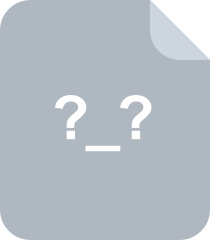
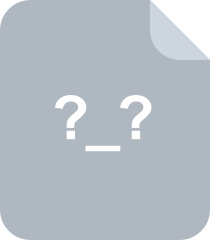
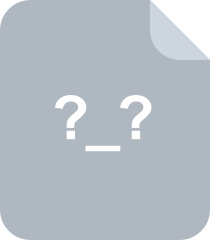
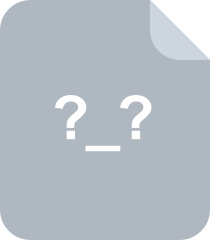
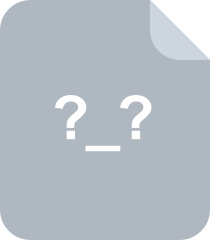
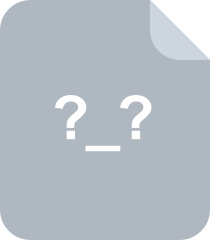
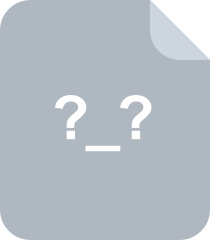
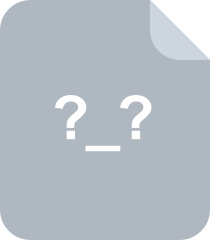
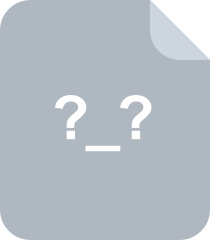
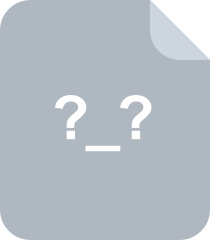
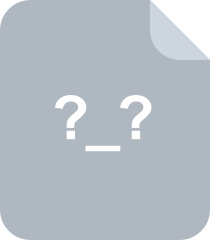
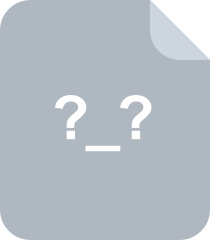
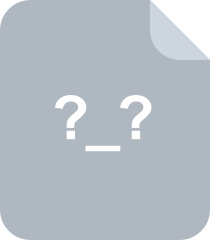
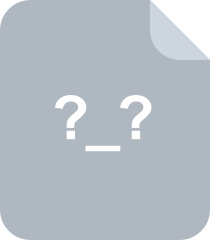
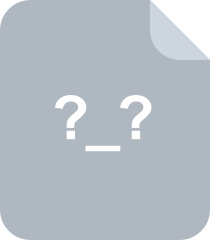
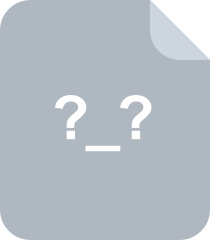
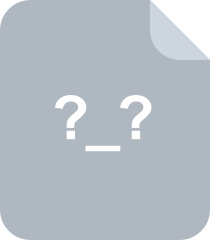
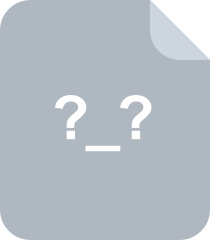
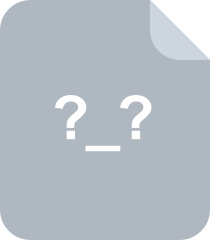
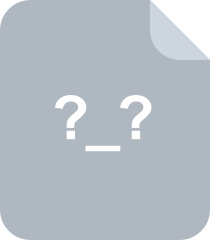
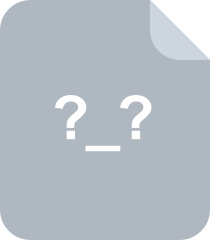
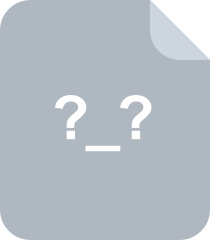
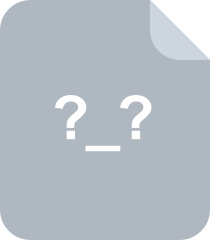
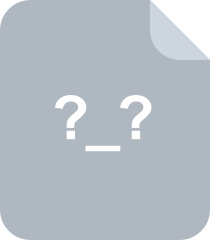
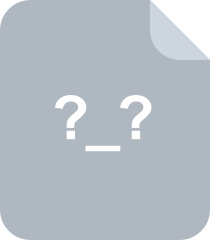
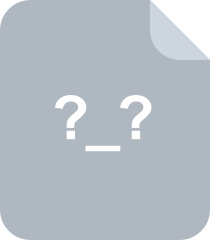
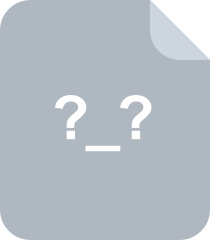
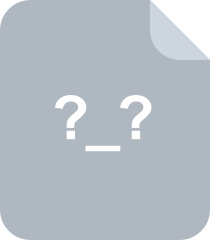
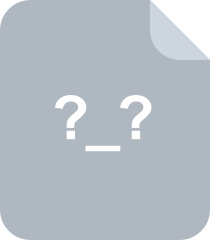
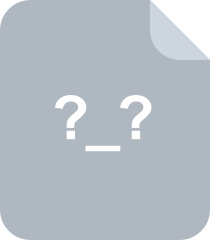
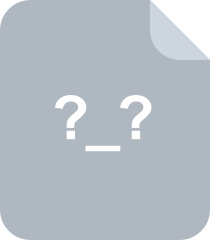
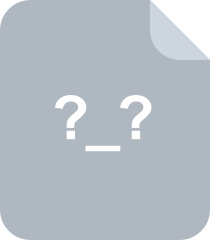
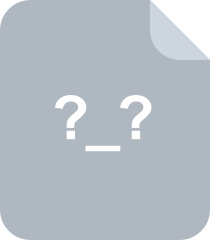
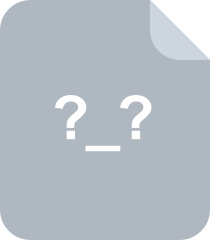
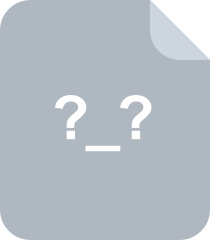
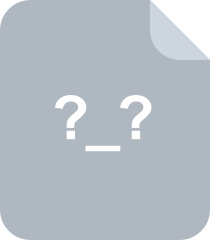
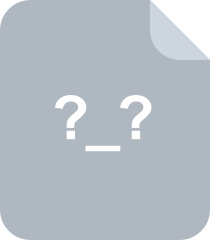
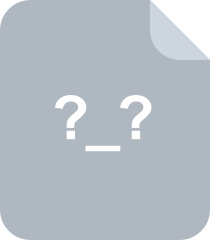
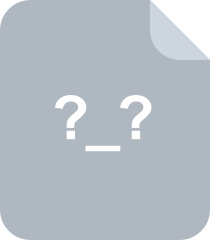
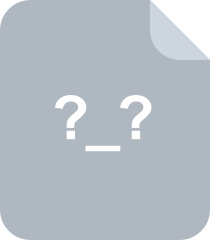
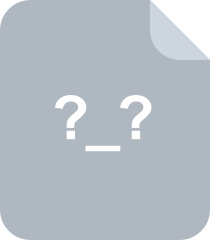
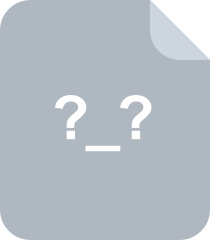
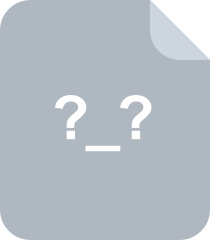
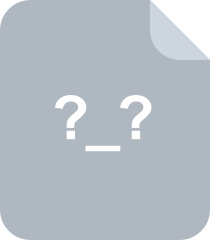
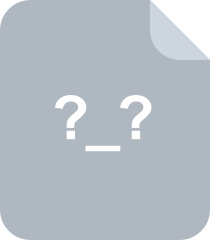
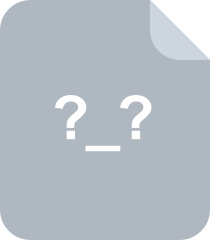
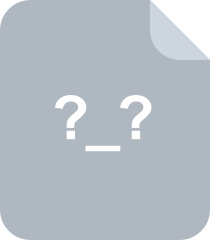
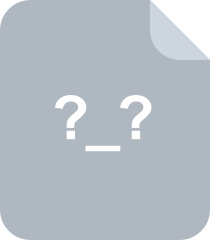
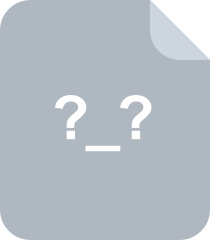
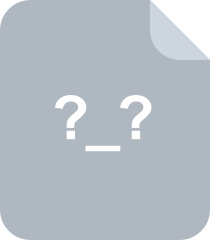
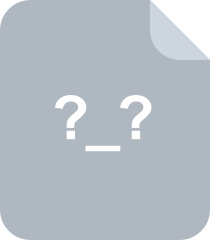
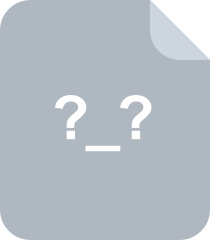
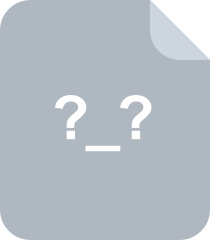
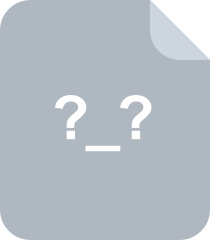
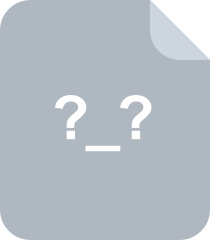
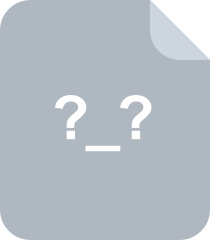
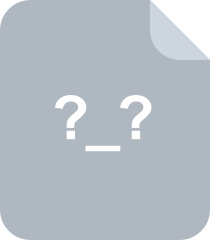
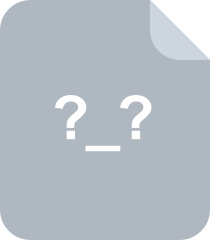
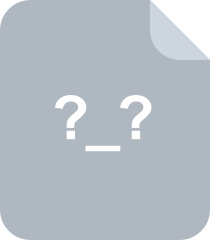
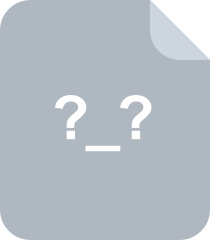
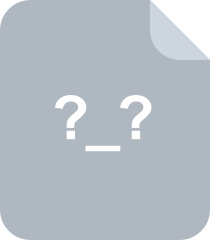
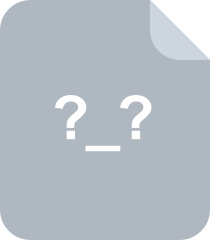
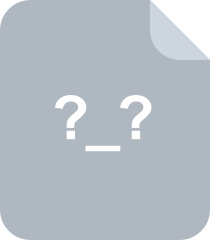
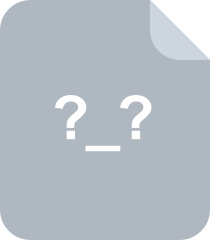
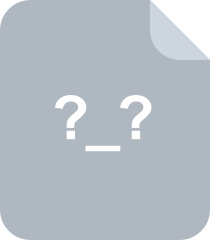
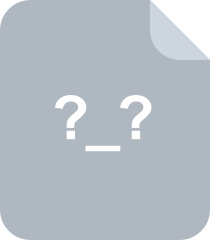
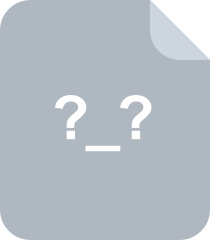
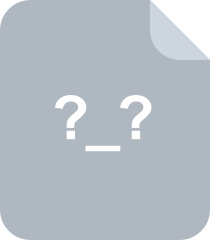
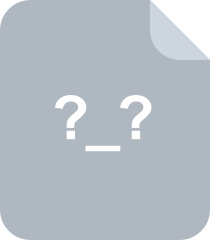
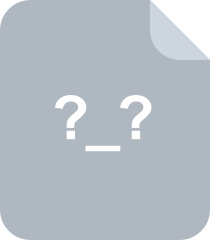
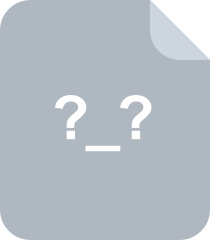
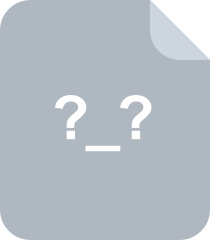
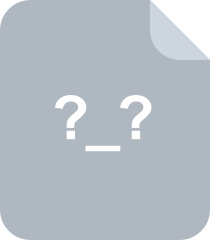
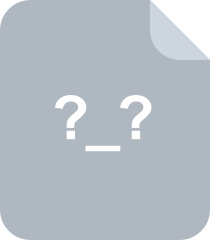
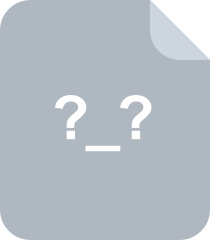
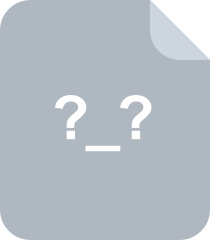
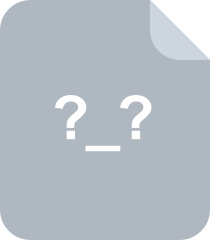
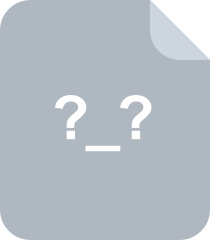
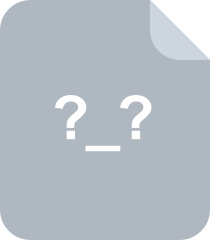
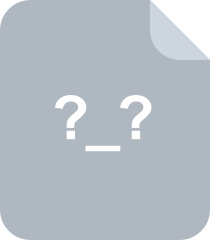
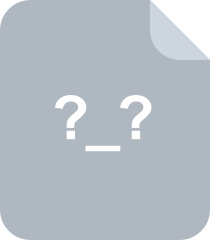
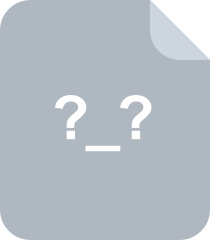
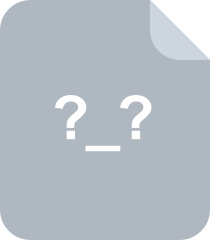
共 1963 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
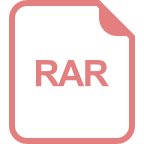
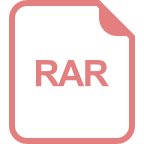
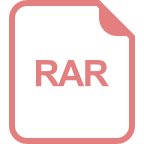
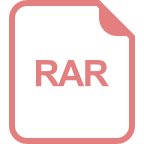
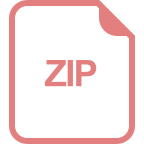
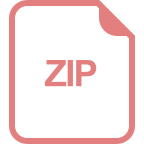
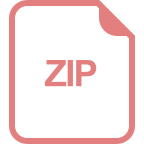
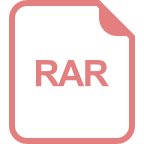
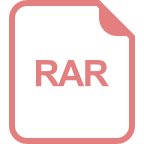
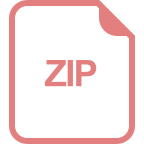
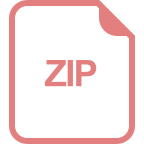

hwbbbb
- 粉丝: 4
- 资源: 260
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

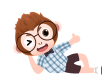
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


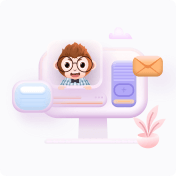
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0