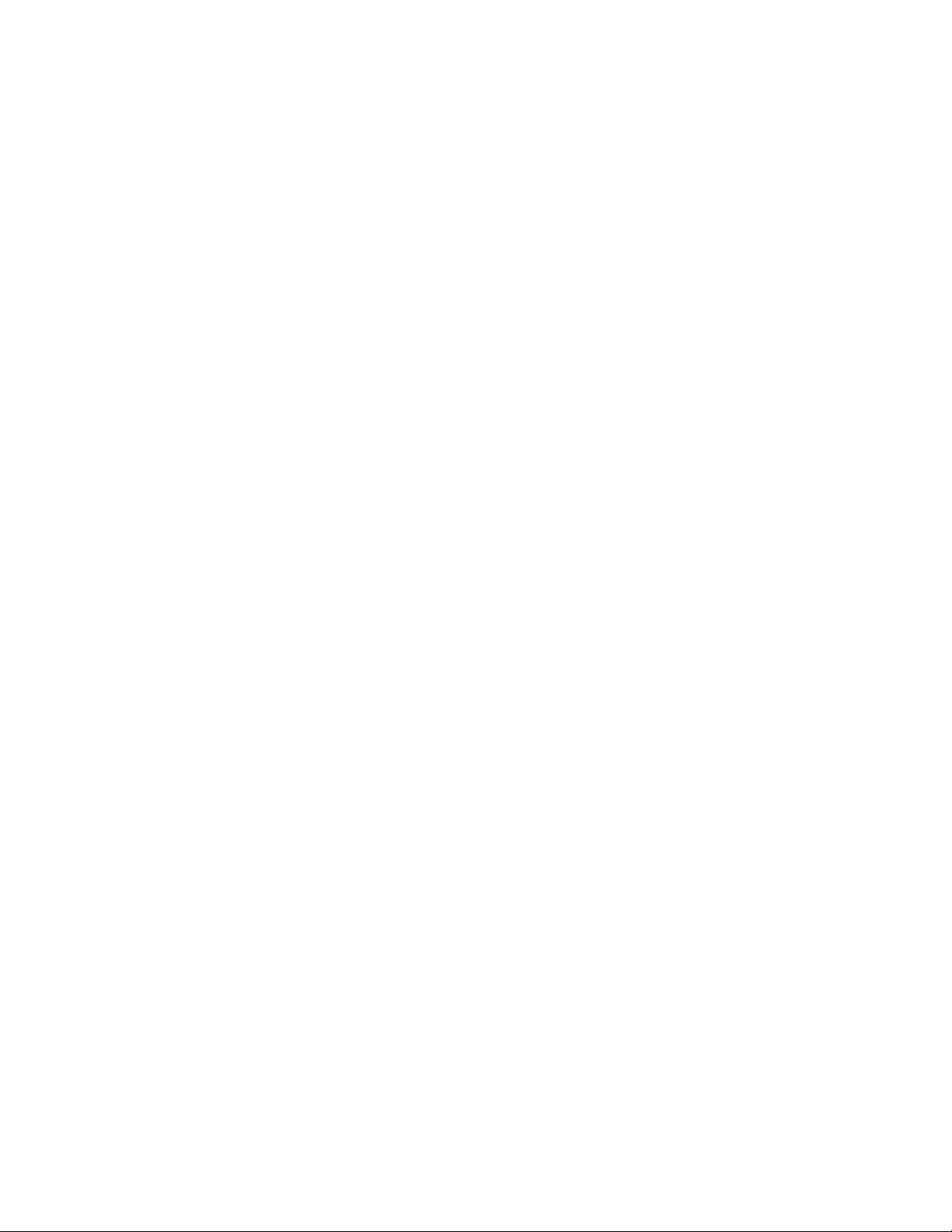
OpenAL 1.1 Specification and Reference
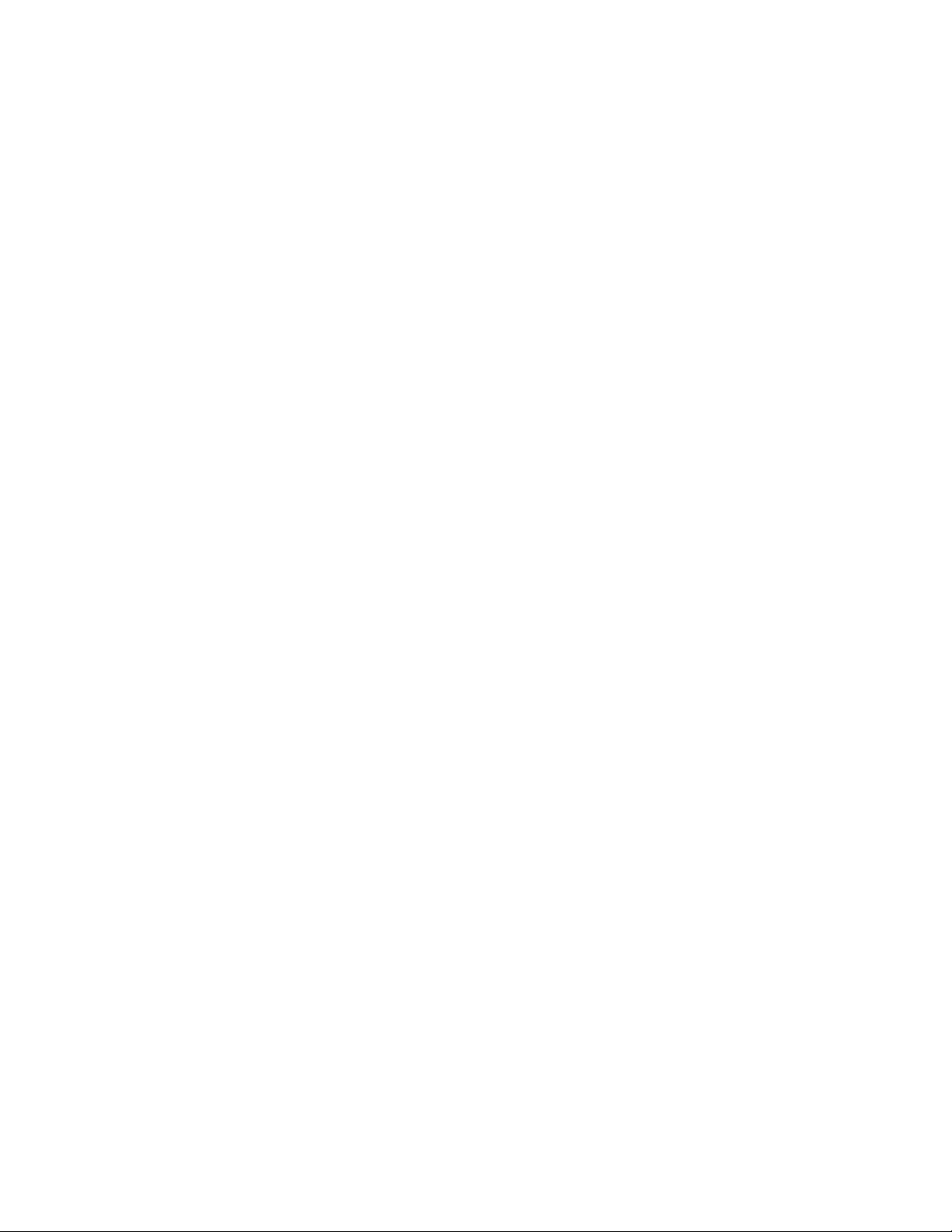
Specification and Reference
This is the OpenAL 1.1 Specification and Reference. This document is based upon the
older OpenAL Specification and Reference (1.0), published in June 2000. Both copyright
notices are presented below.
Version 1.1
Published June 2005
Copyright © 2005 by authors
Version 1.0 Draft Edition
Published June 2000
Copyright © 1999-2000 by Loki Software
Permission is granted to make and distribute verbatim copies of this manual provided the
copyright notice and this permission notice are preserved on all copies.
Permission is granted to copy and distribute translations of this manual into another
language, under the above conditions for modified versions, except that this permission
notice may be stated in a translation approved by the copyright owners.
BeOS is a trademark of PalmSource, Inc.
Linux is a trademark of Linus Torvalds.
Macintosh and Apple are trademarks of Apple Computer, Inc.
OpenAL is a trademark of Creative Labs, Inc.
OpenGL is a trademark of Silicon Graphics, Inc.
UNIX is a trademark of X/Open Group.
Windows is a trademark of Microsoft Corp.
X Window System is a trademark of X Consortium, Inc.
All other trademarks are property of their respective owners.
2
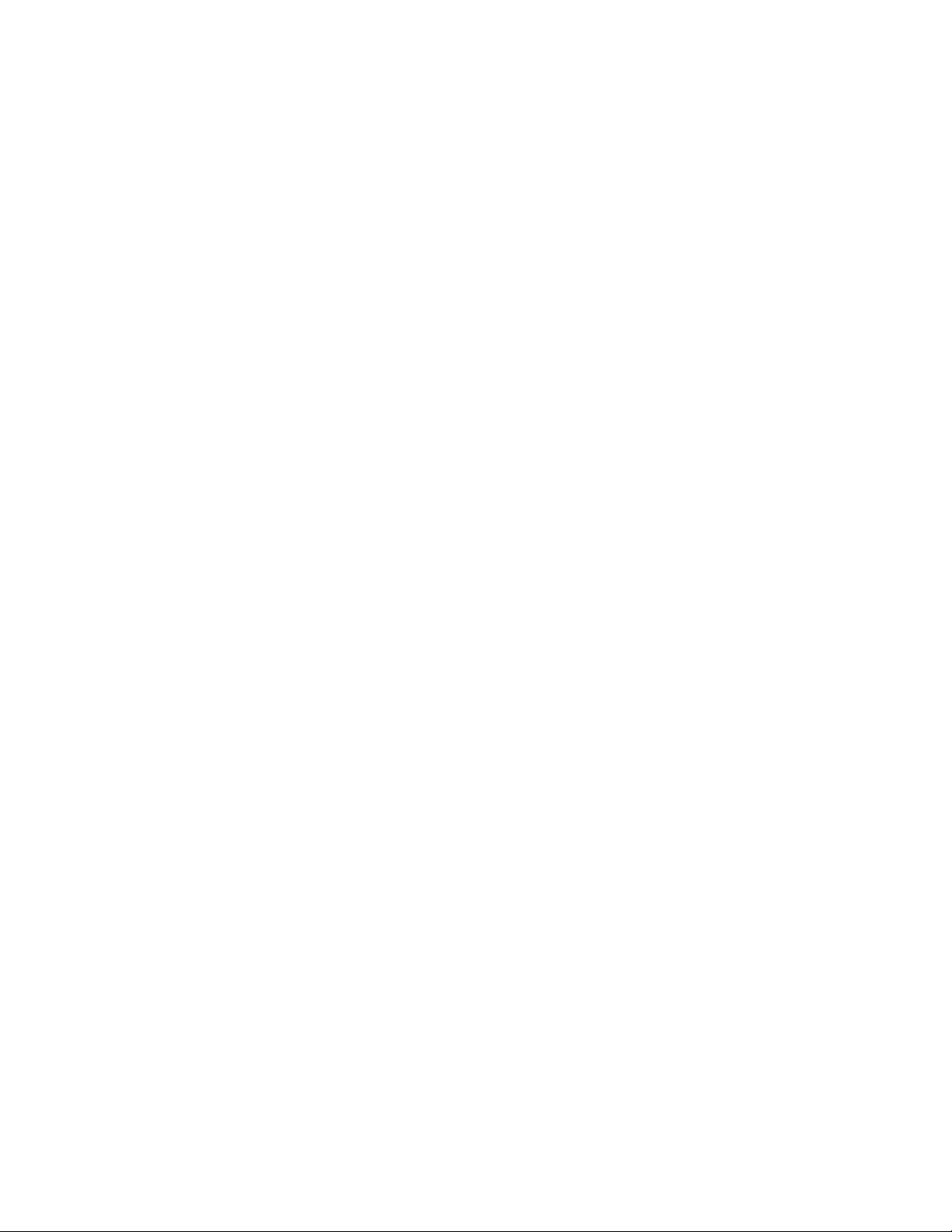
Table of Contents
1. Introduction......................................................................................................................7
1.1. Revision History, 1.1 Document..............................................................................7
1.2. A Brief History of OpenAL......................................................................................8
1.3. What Is the OpenAL Audio System?.......................................................................8
1.4. Differences Between OpenAL 1.1 and OpenAL 1.0................................................9
1.4.1. Recording API..................................................................................................9
1.4.2. Get/Set Offset...................................................................................................9
1.4.3. Linear Distance Models....................................................................................9
1.4.4. Exponential Distance Models...........................................................................9
1.4.5. Doppler.............................................................................................................9
1.4.6. Mono/Stereo Hints............................................................................................9
1.4.7. Standard Extensions Listings...........................................................................9
1.4.8. Standard Suspend/Process Behavior................................................................9
1.4.9. ALUT Revisions.............................................................................................10
1.4.10. Streaming Clarifications...............................................................................10
1.4.11. Error Codes...................................................................................................10
1.4.12. Pitch Shifting Limits.....................................................................................10
1.4.13. New ALchar and ALCchar types..................................................................10
1.4.14. alcCloseDevice Return Value.......................................................................10
1.4.15. Versioning Changes......................................................................................10
1.5. Programmer's View of OpenAL............................................................................10
1.6. Implementor's View of OpenAL............................................................................11
1.7. Our View................................................................................................................11
1.8. Requirements, Conformance and Extensions.........................................................11
1.9. Architecture Review and Acknowledgments.........................................................12
2. OpenAL Operation.........................................................................................................13
2.1. OpenAL Fundamentals...........................................................................................13
2.2. Primitive Types......................................................................................................13
2.3. Floating-Point Computation...................................................................................14
2.4. AL State..................................................................................................................14
2.5. AL Command Syntax.............................................................................................15
2.6. Basic AL Operation................................................................................................15
2.7. AL Errors................................................................................................................16
2.8. Controlling AL Execution......................................................................................17
2.9. Object Paradigm.....................................................................................................18
2.9.1. Object Categories...........................................................................................18
2.10. Static vs. Dynamic Objects..................................................................................18
2.11. Object Names.......................................................................................................18
2.12. Requesting Object Names....................................................................................19
2.13. Releasing Object Names......................................................................................19
2.14. Validating an Object Name..................................................................................19
2.15. Setting Object Attributes......................................................................................20
2.16. Querying Object Attributes..................................................................................21
3
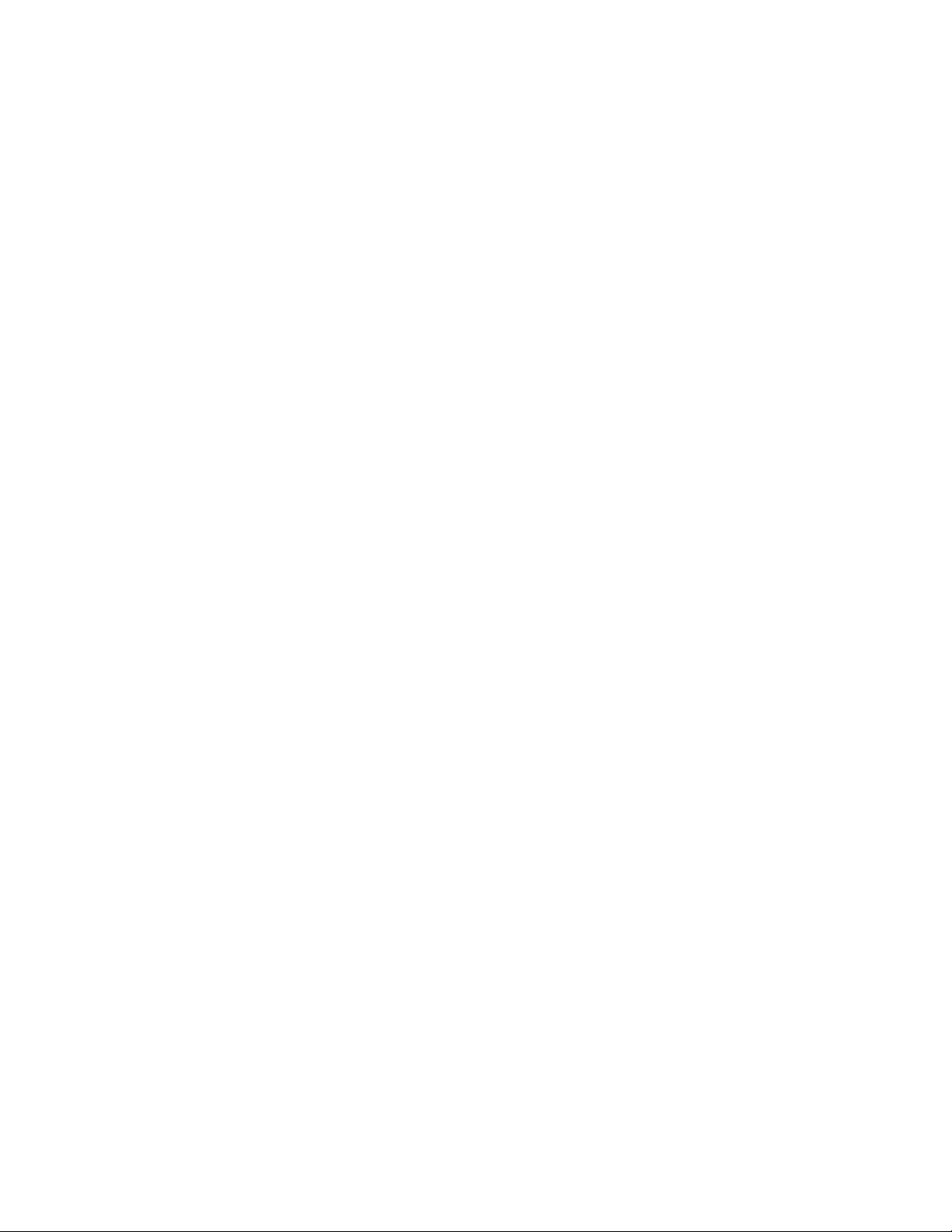
2.17. Object Attributes..................................................................................................21
3. State and State Requests................................................................................................22
3.1. Querying OpenAL State.........................................................................................22
3.1.1. Simple Queries...............................................................................................22
3.1.2. String Queries.................................................................................................23
3.2. Time and Frequency...............................................................................................23
3.3. Space and Distance.................................................................................................23
3.4. Attenuation By Distance........................................................................................23
3.4.1. Inverse Distance Rolloff Model.....................................................................24
3.4.2. Inverse Distance Clamped Model...................................................................25
3.4.3. Linear Distance Rolloff Model.......................................................................25
3.4.4. Linear Distance Clamped Model....................................................................25
3.4.5. Exponential Distance Rolloff Model..............................................................26
3.4.6. Exponential Distance Clamped Model...........................................................26
3.5. Evaluation of Gain/Attenuation Related State.......................................................26
3.5.1. No Culling By Distance.................................................................................26
3.5.2. Velocity Dependent Doppler Effect...............................................................27
4. Listener and Sources......................................................................................................30
4.1. Basic Listener and Source Attributes.....................................................................30
4.2. Listener Object.......................................................................................................31
4.2.1. Listener Attributes..........................................................................................31
4.2.2. Changing Listener Attributes..........................................................................32
4.2.3. Querying Listener Attributes..........................................................................32
4.3. Source Objects........................................................................................................32
4.3.1. Managing Source Names................................................................................32
Requesting a Source Name..................................................................................32
Releasing Source Names.....................................................................................32
Validating a Source Name...................................................................................33
4.3.2. Source Attributes............................................................................................33
Source Positioning...............................................................................................33
Source Type.........................................................................................................33
Buffer Looping.....................................................................................................34
Current Buffer......................................................................................................34
Queue State Queries.............................................................................................34
Bounds on Gain...................................................................................................35
Distance Model Attributes...................................................................................36
Frequency Shift by Pitch......................................................................................36
Direction and Cone..............................................................................................37
Offset...................................................................................................................38
4.3.3. Changing Source Attributes............................................................................39
4.3.4. Querying Source Attributes............................................................................39
4.3.5. Queuing Buffers with a Source......................................................................40
Queuing Command..............................................................................................40
Unqueuing Command..........................................................................................41
4.3.6. Managing Source Execution...........................................................................41
4
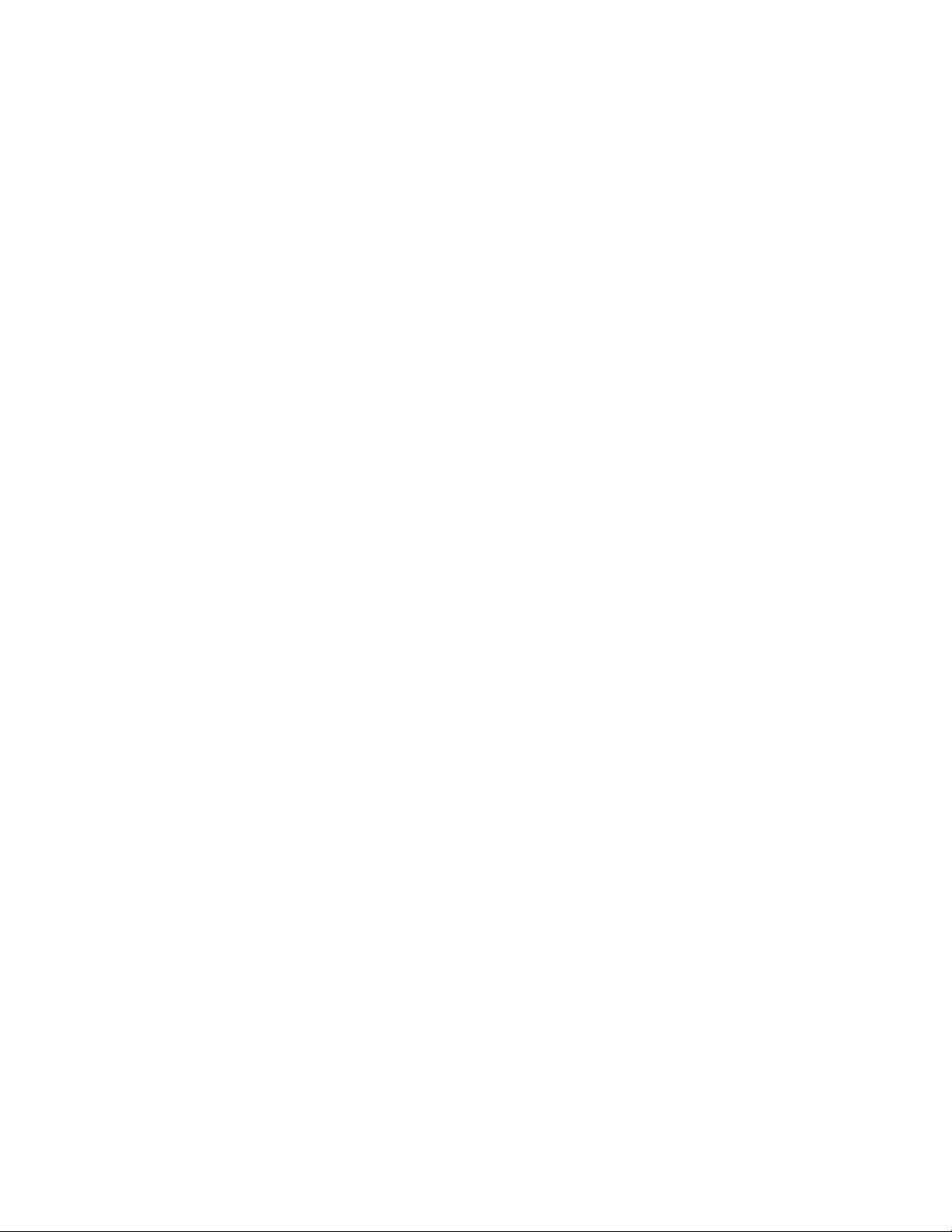
Source State Query..............................................................................................41
State Transition Commands.................................................................................41
Resetting Configuration.......................................................................................43
5. Buffers............................................................................................................................44
5.1. Buffer States...........................................................................................................44
5.2. Managing Buffer Names........................................................................................45
5.2.1. Requesting Buffers Names.............................................................................45
5.2.2. Releasing Buffer Names.................................................................................45
5.2.3. Validating a Buffer Name...............................................................................45
5.3. Manipulating Buffer Attributes..............................................................................45
5.3.1. Buffer Attributes.............................................................................................45
5.3.2. Changing Buffer Attributes............................................................................46
5.3.3. Querying Buffer Attributes.............................................................................46
5.3.4. Specifying Buffer Content..............................................................................47
6. AL Contexts and the ALC API......................................................................................48
6.1. Managing Devices..................................................................................................48
6.1.1. Connecting to a Device...................................................................................48
6.1.2. Disconnecting from a Device.........................................................................48
6.2. Managing Rendering Contexts...............................................................................48
6.2.1. Context Attributes..........................................................................................49
6.2.2. Creating a Context..........................................................................................49
6.2.3. Selecting a Context for Operation..................................................................49
6.2.4. Initiate Context Processing.............................................................................50
6.2.5. Suspend Context Processing...........................................................................50
6.2.6. Destroying a Context......................................................................................50
6.3. ALC Queries..........................................................................................................51
6.3.1. Query for Current Context..............................................................................51
6.3.2. Query for a Context's Device..........................................................................51
6.3.3. Query For Extensions.....................................................................................51
6.3.4. Query for Function Entry Addresses..............................................................51
6.3.5. Retrieving Enumeration Values......................................................................51
6.3.6. Query for Error Conditions.............................................................................52
6.3.7. String Query...................................................................................................52
6.3.8. Integer Query..................................................................................................53
6.4. Shared Objects........................................................................................................54
6.4.1. Shared Buffers................................................................................................54
6.4.2. Capture...........................................................................................................54
Procedures and Functions....................................................................................54
Tokens..................................................................................................................55
Audio capture.......................................................................................................56
7. Appendix: Extensions....................................................................................................57
7.1. Extension Query.....................................................................................................57
7.2. Retrieving Function Entry Addresses.....................................................................57
7.3. Retrieving Enumeration Values.............................................................................57
8. Appendix: Extension Process........................................................................................59
5