<?php
/**
* 本文件百度云服务PHP版本SDK的公共网络交互功能
*
* @author 百度移动.云事业部
* @copyright Copyright (c) 2012-2020 百度在线网络技术(北京)有限公司
* @version 2.0.0
* @package
*/
class RequestCore {
/**
* The URL being requested.
*/
public $request_url;
/**
* The headers being sent in the request.
*/
public $request_headers;
/**
* The body being sent in the request.
*/
public $request_body;
/**
* The response returned by the request.
*/
public $response;
/**
* The headers returned by the request.
*/
public $response_headers;
/**
* The body returned by the request.
*/
public $response_body;
/**
* The HTTP status code returned by the request.
*/
public $response_code;
/**
* Additional response data.
*/
public $response_info;
/**
* The handle for the cURL object.
*/
public $curl_handle;
/**
* The method by which the request is being made.
*/
public $method;
/**
* Stores the proxy settings to use for the request.
*/
public $proxy = null;
/**
* The username to use for the request.
*/
public $username = null;
/**
* The password to use for the request.
*/
public $password = null;
/**
* Custom CURLOPT settings.
*/
public $curlopts = null;
/**
* The state of debug mode.
*/
public $debug_mode = false;
/**
* The default class to use for HTTP Requests (defaults to <RequestCore>).
*/
public $request_class = 'RequestCore';
/**
* The default class to use for HTTP Responses (defaults to <ResponseCore>).
*/
public $response_class = 'ResponseCore';
/**
* Default useragent string to use.
*/
public $useragent = 'RequestCore/1.4.2';
/**
* File to read from while streaming up.
*/
public $read_file = null;
/**
* The resource to read from while streaming up.
*/
public $read_stream = null;
/**
* The size of the stream to read from.
*/
public $read_stream_size = null;
/**
* The length already read from the stream.
*/
public $read_stream_read = 0;
/**
* File to write to while streaming down.
*/
public $write_file = null;
/**
* The resource to write to while streaming down.
*/
public $write_stream = null;
/**
* Stores the intended starting seek position.
*/
public $seek_position = null;
/**
* The user-defined callback function to call when a stream is read from.
*/
public $registered_streaming_read_callback = null;
/**
* The user-defined callback function to call when a stream is written to.
*/
public $registered_streaming_write_callback = null;
/*%******************************************************************************************%*/
// CONSTANTS
/**
* GET HTTP Method
*/
const HTTP_GET = 'GET';
/**
* POST HTTP Method
*/
const HTTP_POST = 'POST';
/**
* PUT HTTP Method
*/
const HTTP_PUT = 'PUT';
/**
* DELETE HTTP Method
*/
const HTTP_DELETE = 'DELETE';
/**
* HEAD HTTP Method
*/
const HTTP_HEAD = 'HEAD';
/*%******************************************************************************************%*/
// CONSTRUCTOR/DESTRUCTOR
/**
* Constructs a new instance of this class.
*
* @param string $url (Optional) The URL to request or service endpoint to query.
* @param string $proxy (Optional) The faux-url to use for proxy settings. Takes the following format: `proxy://user:pass@hostname:port`
* @param array $helpers (Optional) An associative array of classnames to use for request, and response functionality. Gets passed in automatically by the calling class.
* @return $this A reference to the current instance.
*/
public function __construct($url = null, $proxy = null, $helpers = null) {
// Set some default values.
$this->request_url = $url;
$this->method = self::HTTP_GET;
$this->request_headers = array ();
$this->request_body = '';
// Set a new Request class if one was set.
if (isset ( $helpers ['request'] ) && ! empty ( $helpers ['request'] )) {
$this->request_class = $helpers ['request'];
}
// Set a new Request class if one was set.
if (isset ( $helpers ['response'] ) && ! empty ( $helpers ['response'] )) {
$this->response_class = $helpers ['response'];
}
if ($proxy) {
$this->set_proxy ( $proxy );
}
return $this;
}
/**
* Destructs the instance. Closes opened file handles.
*
* @return $this A reference to the current instance.
*/
public function __destruct() {
if (isset ( $this->read_file ) && isset ( $this->read_stream )) {
fclose ( $this->read_stream );
}
if (isset ( $this->write_file ) && isset ( $this->write_stream )) {
fclose ( $this->write_stream );
}
return $this;
}
/*%******************************************************************************************%*/
// REQUEST METHODS
/**
* Sets the credentials to use for authentication.
*
* @param string $user (Required) The username to authenticate with.
* @param string $pass (Required) The password to authenticate with.
* @return $this A reference to the current instance.
*/
public function set_credentials($user, $pass) {
$this->username = $user;
$this->password = $pass;
return $this;
}
/**
* Adds a custom HTTP header to the cURL request.
*
* @param string $key (Required) The custom HTTP header to set.
* @param mixed $value (Required) The value to assign to the custom HTTP header.
* @return $this A reference to the current instance.
*/
public function add_header($key, $value) {
$this->request_headers [$key] = $value;
return $this;
}
/**
* Removes an HTTP header from the cURL request.
*
* @param string $key (Required) The custom HTTP header to set.
* @return $this A reference to the current instance.
*/
public function remove_header($key) {
if (isset ( $this->request_headers [$key] )) {
unset ( $this->request_headers [$key] );
}
return $this;
}
/**
* Set the method type for the request.
*
* @param string $method (Required) One of the following constants: <HTTP_GET>, <HTTP_POST>, <HTTP_PUT>, <HTTP_HEAD>, <HTTP_DELETE>.
* @return $this A reference to the current instance.
*/
public function set_method($method) {
$this->method = strtoupper ( $method );
return $this;
}
/**
* Sets a custom useragent string for the class.
*
* @param string $ua (Required) The useragent string to use.
* @return $this A reference to the current instance.
*/
public function set_useragent($ua) {
$this->useragent = $ua;
return $this;
}
/**
* Set the body to send in the request.
*
* @param string $body (Required) The textual content to send along in the body of the request.
* @return $this A reference to the current instance.
*/
public function set_body($body) {
$this->request_body = $body;
return $this;
}
/**
* Set the URL to make the request to.
*
* @param string $url (Required) The URL to make the request to.
* @return $this A reference to the current instance.
*/
public function set_request_url($url) {
$this->request_url = $url;
return $this;
}
/**
* Set additional CURLOPT settings. These will merge with the default settings, and override if
* there is a duplicate.
*
* @param array $curlopts (Optional) A set of key-value pairs that set `CURLOPT` options. These will merge with the existing CURLOPTs, and ones passed here will override the defaults. Keys should be the `CURLOPT_*` constants, not strings.
* @return $this A reference to the current instance.
*/
public function set_curlopts($curlopts) {
$this->curlopts = $curlopts;
return $this;
}
/**
* Sets the length in bytes to read from the stream while streaming up.
*
* @param integer $size (Required) The length in bytes to read from the stream.
*
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
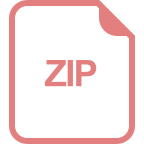
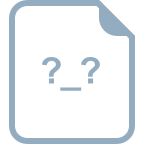
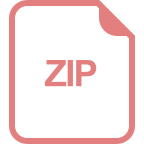
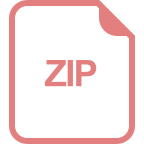
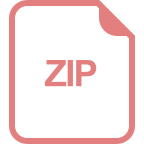
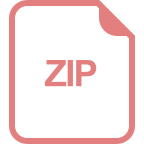
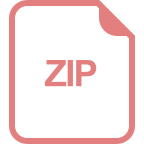
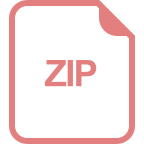
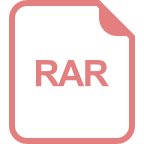
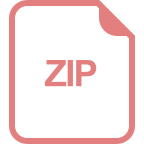
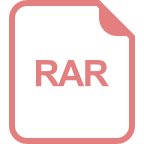
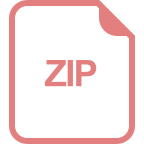
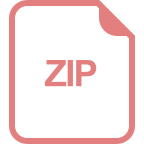
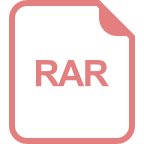
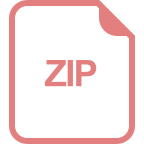
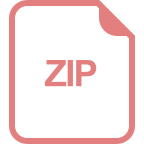
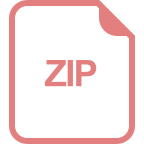
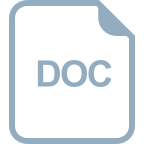
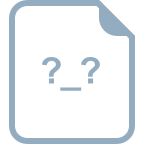
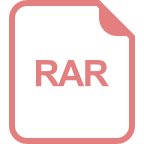
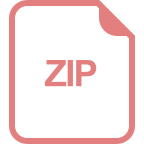
收起资源包目录



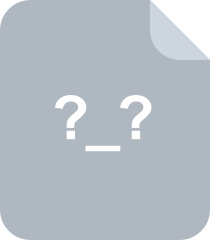
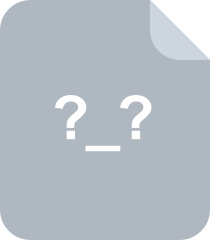
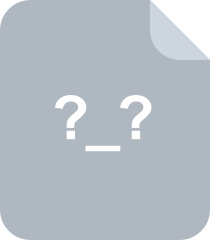
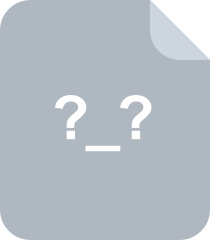
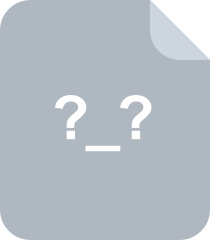
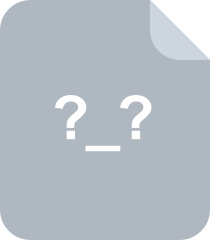
共 6 条
- 1

huqingyin1991
- 粉丝: 0
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

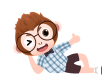
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


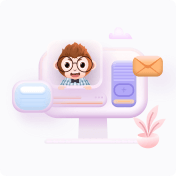
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页