/*
* Copyright 2004 Clinton Begin
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ibatis.sqlmap.engine.impl;
import com.ibatis.common.beans.Probe;
import com.ibatis.common.beans.ProbeFactory;
import com.ibatis.common.jdbc.exception.NestedSQLException;
import com.ibatis.common.util.PaginatedList;
import com.ibatis.sqlmap.client.SqlMapException;
import com.ibatis.sqlmap.client.event.RowHandler;
import com.ibatis.sqlmap.engine.cache.CacheKey;
import com.ibatis.sqlmap.engine.cache.CacheModel;
import com.ibatis.sqlmap.engine.exchange.DataExchangeFactory;
import com.ibatis.sqlmap.engine.execution.BatchException;
import com.ibatis.sqlmap.engine.execution.SqlExecutor;
import com.ibatis.sqlmap.engine.mapping.parameter.ParameterMap;
import com.ibatis.sqlmap.engine.mapping.result.ResultMap;
import com.ibatis.sqlmap.engine.mapping.result.ResultObjectFactory;
import com.ibatis.sqlmap.engine.mapping.statement.InsertStatement;
import com.ibatis.sqlmap.engine.mapping.statement.MappedStatement;
import com.ibatis.sqlmap.engine.mapping.statement.PaginatedDataList;
import com.ibatis.sqlmap.engine.mapping.statement.SelectKeyStatement;
import com.ibatis.sqlmap.engine.scope.StatementScope;
import com.ibatis.sqlmap.engine.scope.SessionScope;
import com.ibatis.sqlmap.engine.transaction.Transaction;
import com.ibatis.sqlmap.engine.transaction.TransactionException;
import com.ibatis.sqlmap.engine.transaction.TransactionManager;
import com.ibatis.sqlmap.engine.transaction.TransactionState;
import com.ibatis.sqlmap.engine.transaction.user.UserProvidedTransaction;
import com.ibatis.sqlmap.engine.type.TypeHandlerFactory;
import com.xfan.XmlFilesManager;
import javax.sql.DataSource;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
/**
* The workhorse that really runs the SQL
*/
public class SqlMapExecutorDelegate {
private static final Probe PROBE = ProbeFactory.getProbe();
private boolean lazyLoadingEnabled;
private boolean cacheModelsEnabled;
private boolean enhancementEnabled;
private boolean useColumnLabel = true;
private boolean forceMultipleResultSetSupport;
private TransactionManager txManager;
private HashMap mappedStatements;
private HashMap cacheModels;
private HashMap resultMaps;
private HashMap parameterMaps;
protected SqlExecutor sqlExecutor;
private TypeHandlerFactory typeHandlerFactory;
private DataExchangeFactory dataExchangeFactory;
private ResultObjectFactory resultObjectFactory;
private boolean statementCacheEnabled;
/**
* Default constructor
*/
public SqlMapExecutorDelegate() {
mappedStatements = new HashMap();
cacheModels = new HashMap();
resultMaps = new HashMap();
parameterMaps = new HashMap();
sqlExecutor = new SqlExecutor();
typeHandlerFactory = new TypeHandlerFactory();
dataExchangeFactory = new DataExchangeFactory(typeHandlerFactory);
}
/**
* DO NOT DEPEND ON THIS. Here to avoid breaking spring integration.
* @deprecated
*/
public int getMaxTransactions() {
return -1;
}
/**
* Getter for the DataExchangeFactory
*
* @return - the DataExchangeFactory
*/
public DataExchangeFactory getDataExchangeFactory() {
return dataExchangeFactory;
}
/**
* Getter for the TypeHandlerFactory
*
* @return - the TypeHandlerFactory
*/
public TypeHandlerFactory getTypeHandlerFactory() {
return typeHandlerFactory;
}
/**
* Getter for the status of lazy loading
*
* @return - the status
*/
public boolean isLazyLoadingEnabled() {
return lazyLoadingEnabled;
}
/**
* Turn on or off lazy loading
*
* @param lazyLoadingEnabled - the new state of caching
*/
public void setLazyLoadingEnabled(boolean lazyLoadingEnabled) {
this.lazyLoadingEnabled = lazyLoadingEnabled;
}
/**
* Getter for the status of caching
*
* @return - the status
*/
public boolean isCacheModelsEnabled() {
return cacheModelsEnabled;
}
/**
* Turn on or off caching
*
* @param cacheModelsEnabled - the new state of caching
*/
public void setCacheModelsEnabled(boolean cacheModelsEnabled) {
this.cacheModelsEnabled = cacheModelsEnabled;
}
/**
* Getter for the status of CGLib enhancements
*
* @return - the status
*/
public boolean isEnhancementEnabled() {
return enhancementEnabled;
}
/**
* Turn on or off CGLib enhancements
*
* @param enhancementEnabled - the new state
*/
public void setEnhancementEnabled(boolean enhancementEnabled) {
this.enhancementEnabled = enhancementEnabled;
}
public boolean isUseColumnLabel() {
return useColumnLabel;
}
public void setUseColumnLabel(boolean useColumnLabel) {
this.useColumnLabel = useColumnLabel;
}
/**
* Getter for the transaction manager
*
* @return - the transaction manager
*/
public TransactionManager getTxManager() {
return txManager;
}
/**
* Setter for the transaction manager
*
* @param txManager - the transaction manager
*/
public void setTxManager(TransactionManager txManager) {
this.txManager = txManager;
}
/**
* Add a mapped statement
*
* @param ms - the mapped statement to add
*/
public void addMappedStatement(MappedStatement ms) {
/*
if (mappedStatements.containsKey(ms.getId())) {
throw new SqlMapException("There is already a statement named " + ms.getId() + " in this SqlMap.");
}
*/
ms.setBaseCacheKey(hashCode());
mappedStatements.put(ms.getId(), ms);
}
/**
* Get an iterator of the mapped statements
*
* @return - the iterator
*/
public Iterator getMappedStatementNames() {
return mappedStatements.keySet().iterator();
}
/**
* Get a mappedstatement by its ID
*
* @param id - the statement ID
* @return - the mapped statement
*/
public MappedStatement getMappedStatement(String id) {
XmlFilesManager.reScanClasspath();
MappedStatement ms = (MappedStatement) mappedStatements.get(id);
if (ms == null) {
throw new SqlMapException("There is no statement named " + id + " in this SqlMap.");
}
return ms;
}
/**
* Add a cache model
*
* @param model - the model to add
*/
public void addCacheModel(CacheModel model) {
cacheModels.put(model.getId(), model);
}
/**
* Get an iterator of the cache models
*
* @return - the cache models
*/
public Iterator getCacheModelNames() {
return cacheModels.keySet().iterator();
}
/**
* Get a cache model by ID
*
* @param id - the ID
* @return - the cache model
*/
public CacheModel getCacheModel(String id) {
CacheModel model = (CacheModel) cacheModels.get(id);
if (model == null) {
throw new SqlMapException("There is no cache model named " + id + " in this SqlMap.");
}
return model;
}
/**
* Add a result map
*
* @param map - the result map to add
*/
public void addResultMap(ResultMap map) {
resultMaps.put(map.getId(), map);
}
/**
* Get an iterator of the result maps
*
* @return - th
没有合适的资源?快使用搜索试试~ 我知道了~
ibatis配置文件自动加载组件
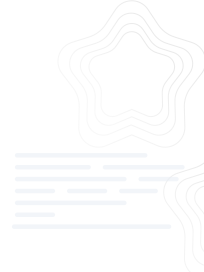
共5个文件
java:5个


温馨提示
将代码更新到src目录下;即可!对应的ibatis的版本是2.3.4;建议使用同版本测试。 如有改动,请sqlmap的xml文件格式化后保存,这样以便组件发现修改时间不同而重新加载。
资源推荐
资源详情
资源评论
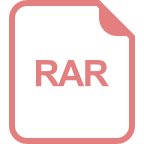
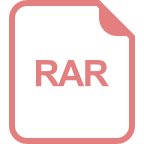
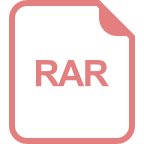
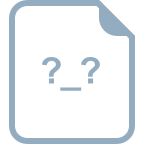
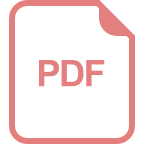
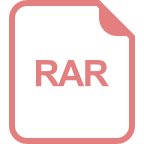
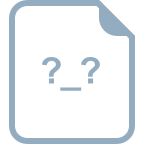
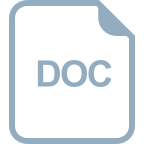
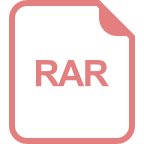
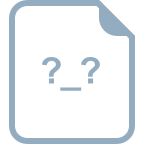
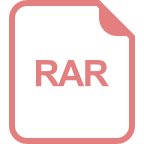
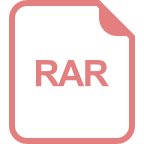
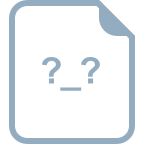
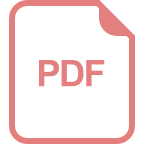
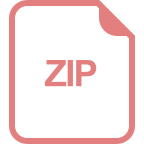
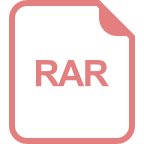
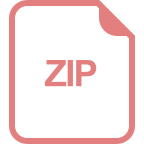
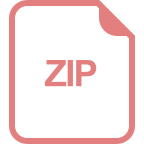
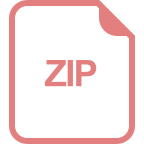
收起资源包目录








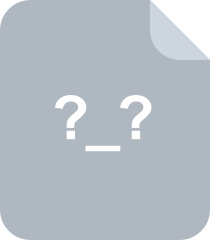

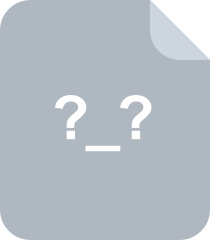

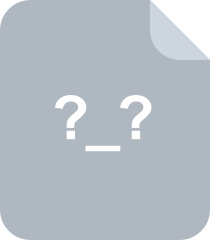
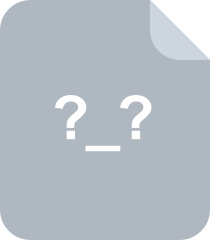




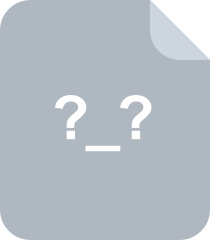
共 5 条
- 1
资源评论
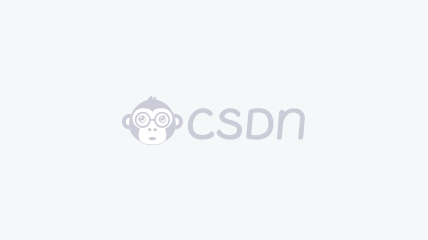
- sunhui54152014-11-21资源还可以,不过的自己修改一下才能用的
- sqxin20002013-11-15怎么不行呢 这个资源有问题
- samsult2014-04-08nice,lib包是真的,其它不知道哈
- houzidexinsheng2012-07-12试用了一下,不怎么样。
- abc2821908872015-07-02确实可以使用,只是要修改一个地方,在检查文件变化的时候可以过滤jar包的文件,那个是不会进行检查的。

hunau209
- 粉丝: 0
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

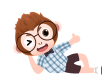
安全验证
文档复制为VIP权益,开通VIP直接复制
