<?php
namespace WY\app\libs\Mailer;
use WY\app\libs\Mailer\SMTP;
use Exception;
use WY\app\libs\Mailer\phpmailerException;
/**
* PHPMailer - PHP email creation and transport class.
* PHP Version 5
* @package PHPMailer
* @link https://github.com/PHPMailer/PHPMailer/ The PHPMailer GitHub project
* @author Marcus Bointon (Synchro/coolbru) <phpmailer@synchromedia.co.uk>
* @author Jim Jagielski (jimjag) <jimjag@gmail.com>
* @author Andy Prevost (codeworxtech) <codeworxtech@users.sourceforge.net>
* @author Brent R. Matzelle (original founder)
* @copyright 2012 - 2014 Marcus Bointon
* @copyright 2010 - 2012 Jim Jagielski
* @copyright 2004 - 2009 Andy Prevost
* @license http://www.gnu.org/copyleft/lesser.html GNU Lesser General Public License
* @note This program is distributed in the hope that it will be useful - WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE.
*/
/**
* PHPMailer - PHP email creation and transport class.
* @package PHPMailer
* @author Marcus Bointon (Synchro/coolbru) <phpmailer@synchromedia.co.uk>
* @author Jim Jagielski (jimjag) <jimjag@gmail.com>
* @author Andy Prevost (codeworxtech) <codeworxtech@users.sourceforge.net>
* @author Brent R. Matzelle (original founder)
*/
class PHPMailer
{
/**
* The PHPMailer Version number.
* @var string
*/
public $Version = '5.2.14';
/**
* Email priority.
* Options: null (default), 1 = High, 3 = Normal, 5 = low.
* When null, the header is not set at all.
* @var integer
*/
public $Priority = null;
/**
* The character set of the message.
* @var string
*/
public $CharSet = 'iso-8859-1';
/**
* The MIME Content-type of the message.
* @var string
*/
public $ContentType = 'text/plain';
/**
* The message encoding.
* Options: "8bit", "7bit", "binary", "base64", and "quoted-printable".
* @var string
*/
public $Encoding = '8bit';
/**
* Holds the most recent mailer error message.
* @var string
*/
public $ErrorInfo = '';
/**
* The From email address for the message.
* @var string
*/
public $From = 'root@localhost';
/**
* The From name of the message.
* @var string
*/
public $FromName = 'Root User';
/**
* The Sender email (Return-Path) of the message.
* If not empty, will be sent via -f to sendmail or as 'MAIL FROM' in smtp mode.
* @var string
*/
public $Sender = '';
/**
* The Return-Path of the message.
* If empty, it will be set to either From or Sender.
* @var string
* @deprecated Email senders should never set a return-path header;
* it's the receiver's job (RFC5321 section 4.4), so this no longer does anything.
* @link https://tools.ietf.org/html/rfc5321#section-4.4 RFC5321 reference
*/
public $ReturnPath = '';
/**
* The Subject of the message.
* @var string
*/
public $Subject = '';
/**
* An HTML or plain text message body.
* If HTML then call isHTML(true).
* @var string
*/
public $Body = '';
/**
* The plain-text message body.
* This body can be read by mail clients that do not have HTML email
* capability such as mutt & Eudora.
* Clients that can read HTML will view the normal Body.
* @var string
*/
public $AltBody = '';
/**
* An iCal message part body.
* Only supported in simple alt or alt_inline message types
* To generate iCal events, use the bundled extras/EasyPeasyICS.php class or iCalcreator
* @link http://sprain.ch/blog/downloads/php-class-easypeasyics-create-ical-files-with-php/
* @link http://kigkonsult.se/iCalcreator/
* @var string
*/
public $Ical = '';
/**
* The complete compiled MIME message body.
* @access protected
* @var string
*/
protected $MIMEBody = '';
/**
* The complete compiled MIME message headers.
* @var string
* @access protected
*/
protected $MIMEHeader = '';
/**
* Extra headers that createHeader() doesn't fold in.
* @var string
* @access protected
*/
protected $mailHeader = '';
/**
* Word-wrap the message body to this number of chars.
* Set to 0 to not wrap. A useful value here is 78, for RFC2822 section 2.1.1 compliance.
* @var integer
*/
public $WordWrap = 0;
/**
* Which method to use to send mail.
* Options: "mail", "sendmail", or "smtp".
* @var string
*/
public $Mailer = 'mail';
/**
* The path to the sendmail program.
* @var string
*/
public $Sendmail = '/usr/sbin/sendmail';
/**
* Whether mail() uses a fully sendmail-compatible MTA.
* One which supports sendmail's "-oi -f" options.
* @var boolean
*/
public $UseSendmailOptions = true;
/**
* Path to PHPMailer plugins.
* Useful if the SMTP class is not in the PHP include path.
* @var string
* @deprecated Should not be needed now there is an autoloader.
*/
public $PluginDir = '';
/**
* The email address that a reading confirmation should be sent to, also known as read receipt.
* @var string
*/
public $ConfirmReadingTo = '';
/**
* The hostname to use in the Message-ID header and as default HELO string.
* If empty, PHPMailer attempts to find one with, in order,
* $_SERVER['SERVER_NAME'], gethostname(), php_uname('n'), or the value
* 'localhost.localdomain'.
* @var string
*/
public $Hostname = '';
/**
* An ID to be used in the Message-ID header.
* If empty, a unique id will be generated.
* @var string
*/
public $MessageID = '';
/**
* The message Date to be used in the Date header.
* If empty, the current date will be added.
* @var string
*/
public $MessageDate = '';
/**
* SMTP hosts.
* Either a single hostname or multiple semicolon-delimited hostnames.
* You can also specify a different port
* for each host by using this format: [hostname:port]
* (e.g. "smtp1.example.com:25;smtp2.example.com").
* You can also specify encryption type, for example:
* (e.g. "tls://smtp1.example.com:587;ssl://smtp2.example.com:465").
* Hosts will be tried in order.
* @var string
*/
public $Host = 'localhost';
/**
* The default SMTP server port.
* @var integer
* @TODO Why is this needed when the SMTP class takes care of it?
*/
public $Port = 25;
/**
* The SMTP HELO of the message.
* Default is $Hostname. If $Hostname is empty, PHPMailer attempts to find
* one with the same method described above for $Hostname.
* @var string
* @see PHPMailer::$Hostname
*/
public $Helo = '';
/**
* What kind of encryption to use on the SMTP connection.
* Options: '', 'ssl' or 'tls'
* @var string
*/
public $SMTPSecure = '';
/**
* Whether to enable TLS encryption automatically if a server supports it,
* even if `SMTPSecure` is not set to 'tls'.
* Be aware that in PHP >= 5.6 this requires that the server's certificates are valid.
* @var boolean
*/
public $SMTPAutoTLS = true;
/**
* Whether to use SMTP authentication.
* Uses the Username and Password properties.
* @var boolean
* @see PHPMailer::$Username
* @see PHPMailer::$Password
*/
public $SMTPAuth = false;
/**
* Options array passed to stream_context_create when connecting via SMTP.
* @var array
*/
public $SMTPOptions = array();
/**
* SMTP username.
* @var string
*/
public $Username = '';
/**
* SMTP password.
* @var string
*/
public $Password = '';
/**
* SMTP auth type.
* Options
没有合适的资源?快使用搜索试试~ 我知道了~
PHP聚合通多平台支付平台源码.zip
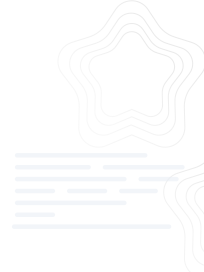
共2000个文件
php:551个
png:429个
gif:293个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 147 浏览量
2024-06-13
19:32:07
上传
评论
收藏 29.33MB ZIP 举报
温馨提示
php聚合通多平台支付平台源码,源码搭建了一下,这个源码不复杂,修改一下数据库账号密码然后导入数据库就可以,和网站恢复备份一样简单!
资源推荐
资源详情
资源评论
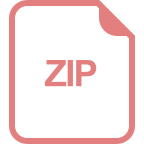
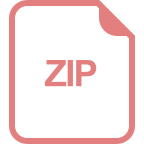
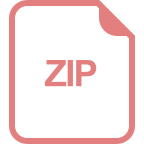
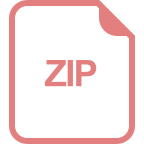
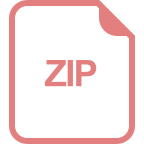
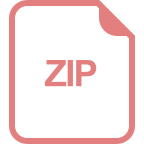
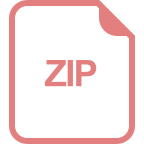
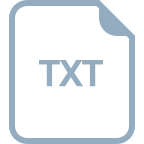
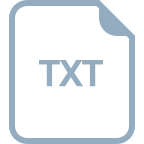
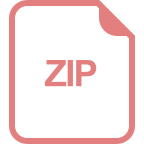
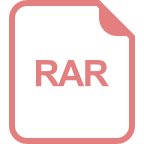
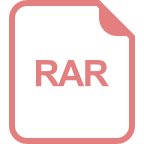
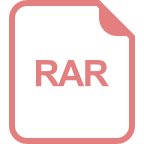
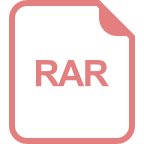
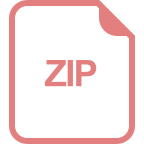
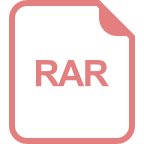
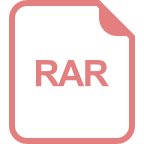
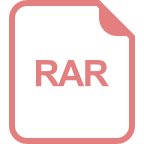
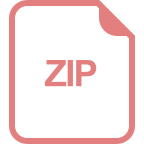
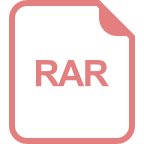
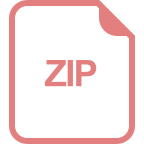
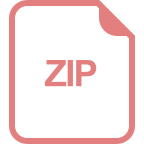
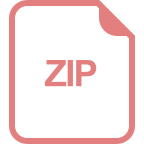
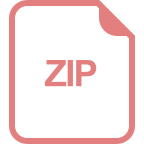
收起资源包目录

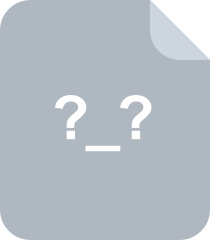
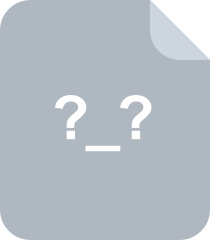
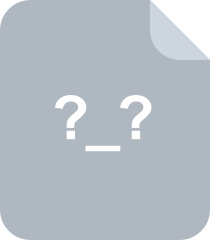
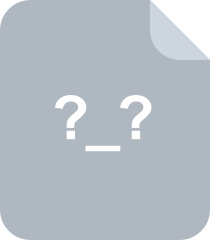
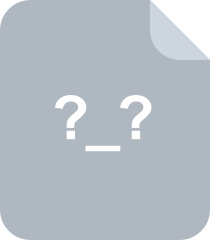
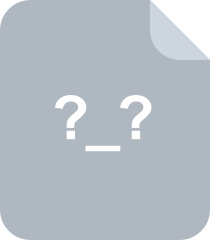
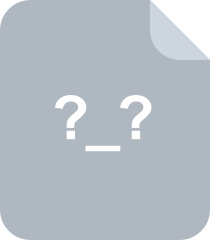
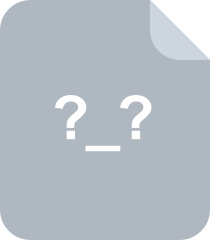
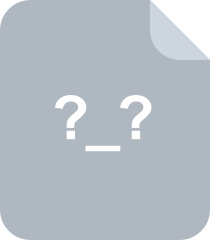
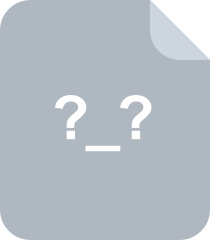
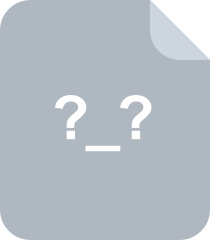
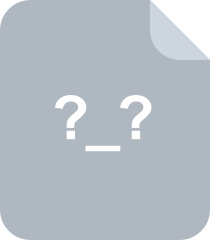
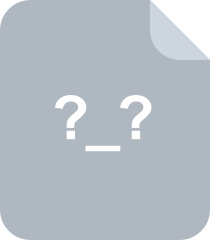
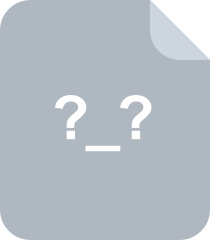
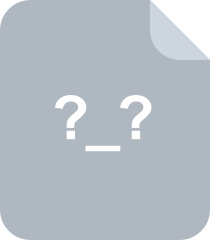
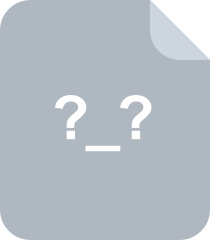
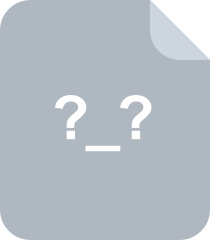
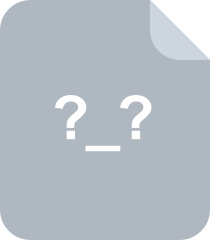
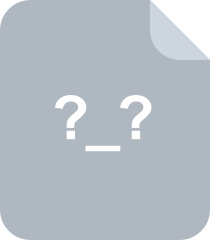
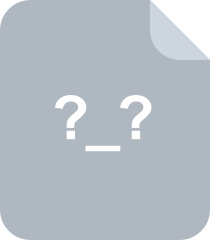
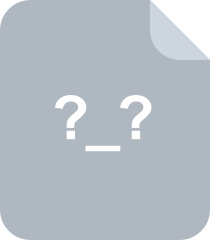
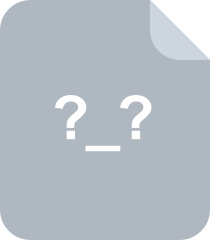
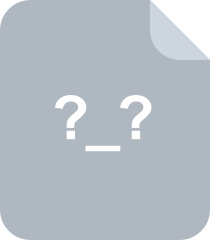
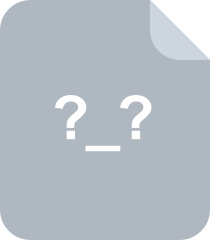
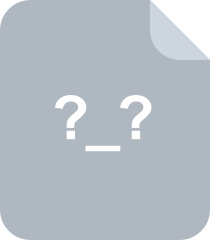
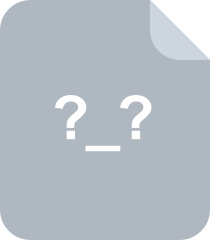
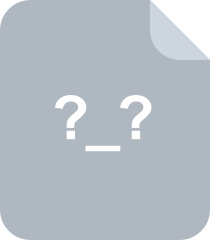
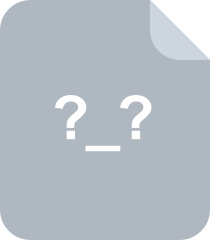
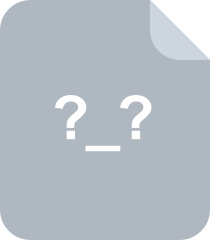
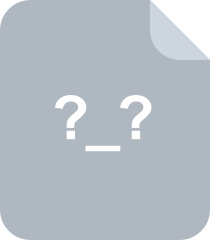
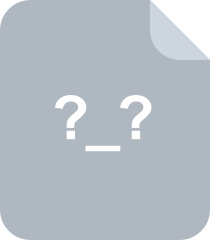
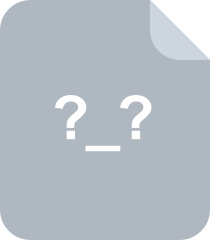
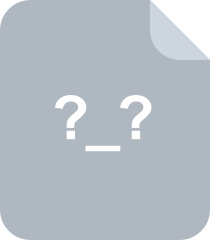
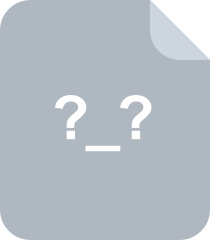
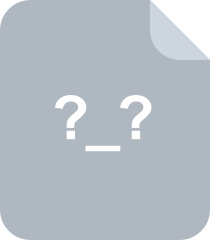
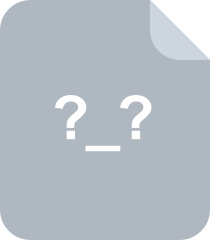
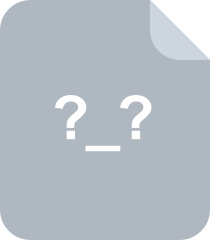
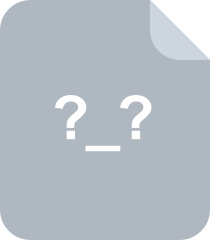
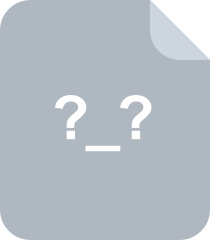
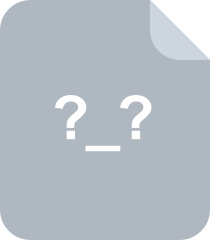
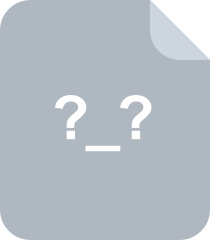
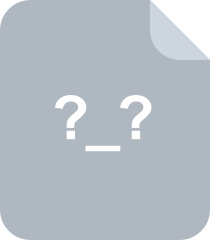
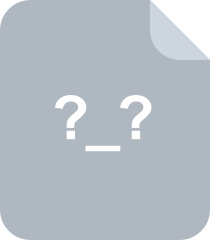
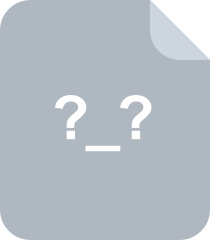
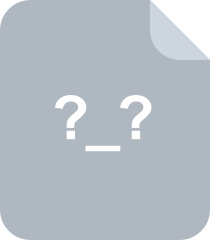
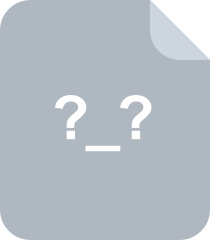
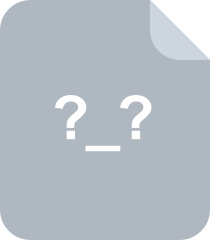
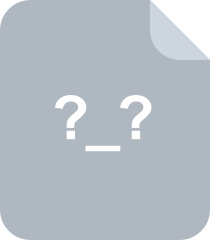
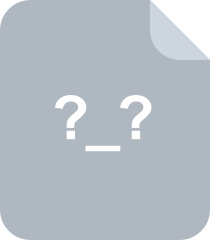
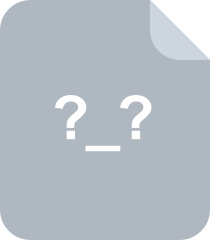
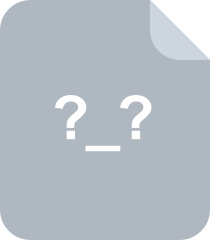
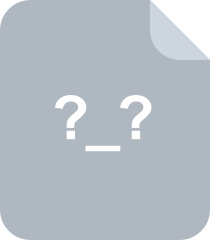
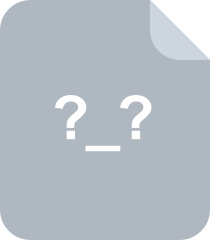
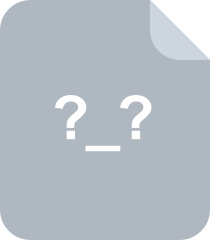
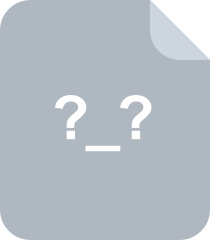
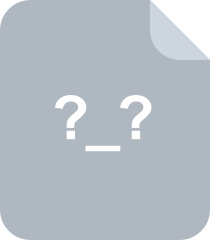
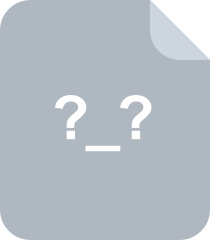
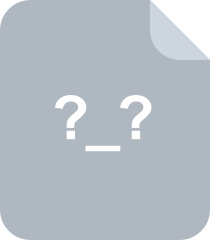
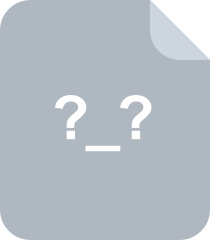
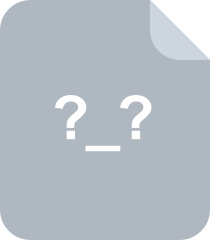
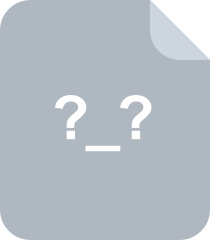
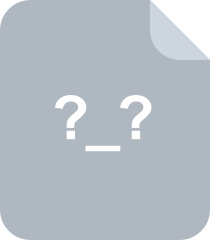
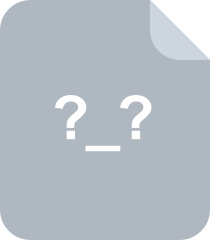
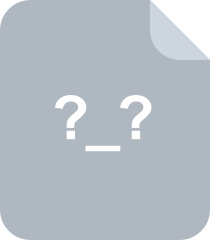
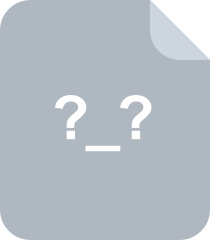
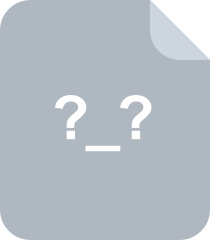
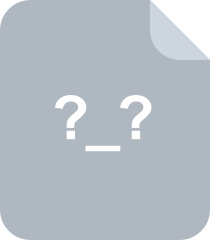
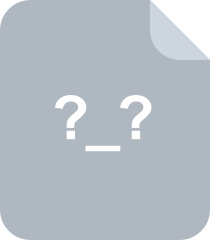
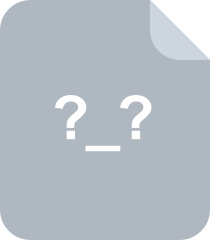
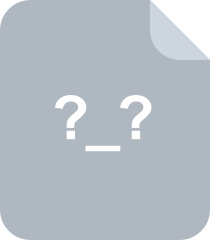
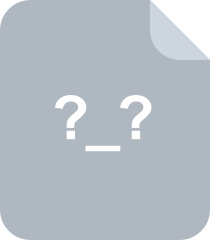
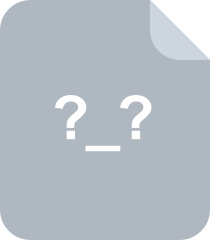
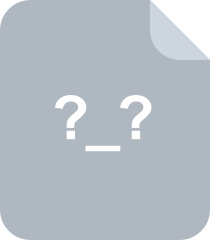
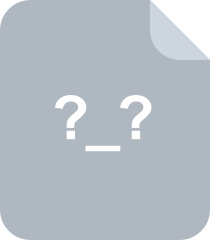
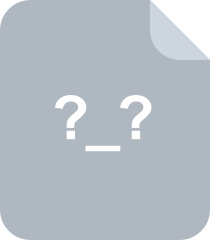
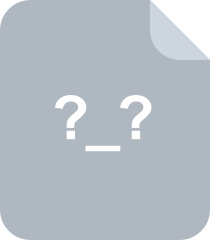
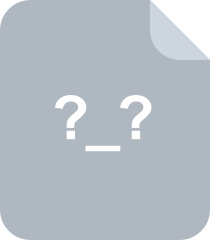
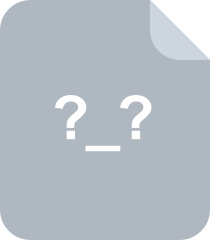
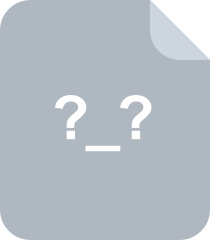
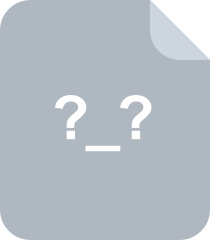
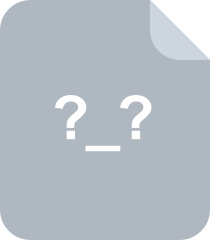
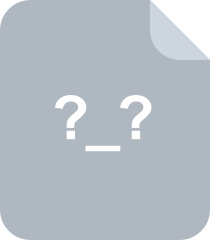
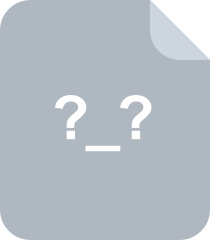
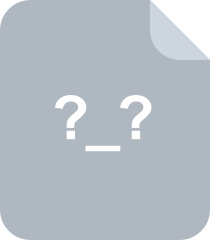
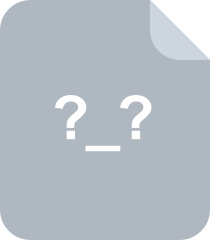
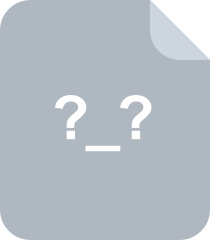
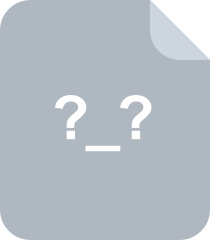
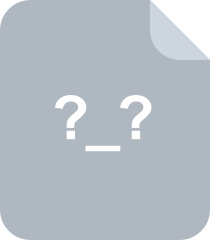
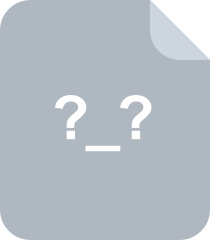
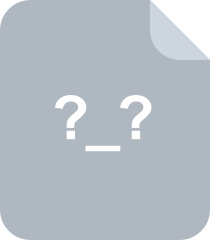
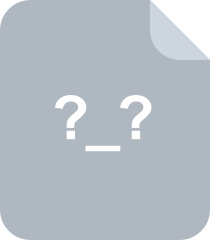
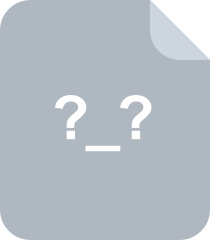
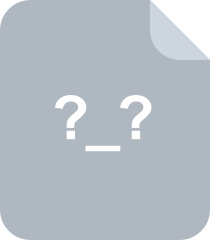
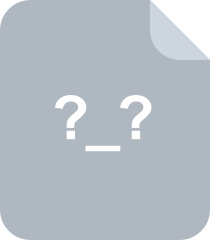
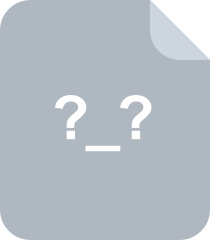
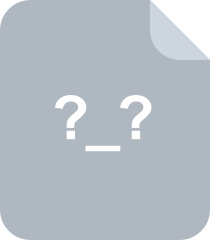
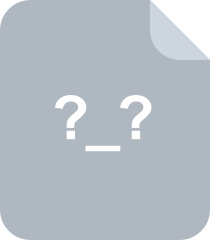
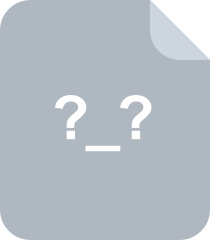
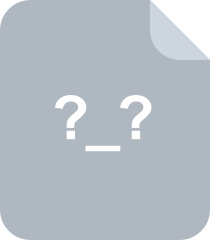
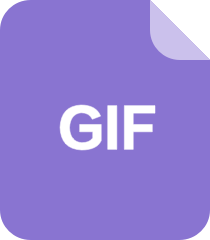
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
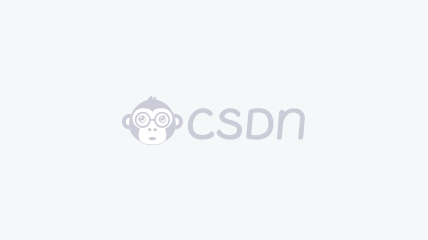

酷爱码
- 粉丝: 6713
- 资源: 1088

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

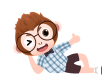
安全验证
文档复制为VIP权益,开通VIP直接复制
