/*++
Copyright(c) 1992-2000 Microsoft Corporation
Module Name:
protocol.c
Abstract:
Ndis Intermediate Miniport driver sample. This is a passthru driver.
Author:
Environment:
Revision History:
--*/
#include "precomp.h"
#pragma hdrstop
#define MAX_PACKET_POOL_SIZE 0x0000FFFF
#define MIN_PACKET_POOL_SIZE 0x000000FF
NDIS_STATUS AnalysisPacket(PNDIS_PACKET Packet, BOOLEAN bRecOrSend);
//
// NDIS version as 0xMMMMmmmm, where M=Major/m=minor (0x00050001 = 5.1);
// initially unknown (0)
//
ULONG NdisDotSysVersion = 0x0;
#define NDIS_SYS_VERSION_51 0x00050001
VOID
PtBindAdapter(
OUT PNDIS_STATUS Status,
IN NDIS_HANDLE BindContext,
IN PNDIS_STRING DeviceName,
IN PVOID SystemSpecific1,
IN PVOID SystemSpecific2
)
/*++
Routine Description:
Called by NDIS to bind to a miniport below.
Arguments:
Status - Return status of bind here.
BindContext - Can be passed to NdisCompleteBindAdapter if this call is pended.
DeviceName - Device name to bind to. This is passed to NdisOpenAdapter.
SystemSpecific1 - Can be passed to NdisOpenProtocolConfiguration to read per-binding information
SystemSpecific2 - Unused
Return Value:
NDIS_STATUS_PENDING if this call is pended. In this case call NdisCompleteBindAdapter
to complete.
Anything else Completes this call synchronously
--*/
{
NDIS_HANDLE ConfigHandle = NULL;
PNDIS_CONFIGURATION_PARAMETER Param;
NDIS_STRING DeviceStr = NDIS_STRING_CONST("UpperBindings");
NDIS_STRING NdisVersionStr = NDIS_STRING_CONST("NdisVersion");
PADAPT pAdapt = NULL;
NDIS_STATUS Sts;
UINT MediumIndex;
ULONG TotalSize;
BOOLEAN NoCleanUpNeeded = FALSE;
UNREFERENCED_PARAMETER(BindContext);
UNREFERENCED_PARAMETER(SystemSpecific2);
DBGPRINT(("==> Protocol BindAdapter\n"));
do
{
//
// Access the configuration section for our binding-specific
// parameters.
//
NdisOpenProtocolConfiguration(Status,
&ConfigHandle,
SystemSpecific1);
if (*Status != NDIS_STATUS_SUCCESS)
{
break;
}
if (NdisDotSysVersion == 0)
{
NdisReadConfiguration(Status,
&Param,
ConfigHandle,
&NdisVersionStr, // "NdisVersion"
NdisParameterInteger);
if (*Status != NDIS_STATUS_SUCCESS)
{
break;
}
NdisDotSysVersion = Param->ParameterData.IntegerData;
}
//
// Read the "UpperBindings" reserved key that contains a list
// of device names representing our miniport instances corresponding
// to this lower binding. Since this is a 1:1 IM driver, this key
// contains exactly one name.
//
// If we want to implement a N:1 mux driver (N adapter instances
// over a single lower binding), then UpperBindings will be a
// MULTI_SZ containing a list of device names - we would loop through
// this list, calling NdisIMInitializeDeviceInstanceEx once for
// each name in it.
//
NdisReadConfiguration(Status,
&Param,
ConfigHandle,
&DeviceStr,
NdisParameterString);
if (*Status != NDIS_STATUS_SUCCESS)
{
break;
}
//
// Allocate memory for the Adapter structure. This represents both the
// protocol context as well as the adapter structure when the miniport
// is initialized.
//
// In addition to the base structure, allocate space for the device
// instance string.
//
TotalSize = sizeof(ADAPT) + Param->ParameterData.StringData.MaximumLength;
NdisAllocateMemoryWithTag(&pAdapt, TotalSize, TAG);
if (pAdapt == NULL)
{
*Status = NDIS_STATUS_RESOURCES;
break;
}
//
// Initialize the adapter structure. We copy in the IM device
// name as well, because we may need to use it in a call to
// NdisIMCancelInitializeDeviceInstance. The string returned
// by NdisReadConfiguration is active (i.e. available) only
// for the duration of this call to our BindAdapter handler.
//
NdisZeroMemory(pAdapt, TotalSize);
pAdapt->DeviceName.MaximumLength = Param->ParameterData.StringData.MaximumLength;
pAdapt->DeviceName.Length = Param->ParameterData.StringData.Length;
pAdapt->DeviceName.Buffer = (PWCHAR)((ULONG_PTR)pAdapt + sizeof(ADAPT));
NdisMoveMemory(pAdapt->DeviceName.Buffer,
Param->ParameterData.StringData.Buffer,
Param->ParameterData.StringData.MaximumLength);
NdisInitializeEvent(&pAdapt->Event);
NdisAllocateSpinLock(&pAdapt->Lock);
//
// Allocate a packet pool for sends. We need this to pass sends down.
// We cannot use the same packet descriptor that came down to our send
// handler (see also NDIS 5.1 packet stacking).
//
NdisAllocatePacketPoolEx(Status,
&pAdapt->SendPacketPoolHandle,
MIN_PACKET_POOL_SIZE,
MAX_PACKET_POOL_SIZE - MIN_PACKET_POOL_SIZE,
sizeof(SEND_RSVD));
if (*Status != NDIS_STATUS_SUCCESS)
{
break;
}
//
// Allocate a packet pool for receives. We need this to indicate receives.
// Same consideration as sends (see also NDIS 5.1 packet stacking).
//
NdisAllocatePacketPoolEx(Status,
&pAdapt->RecvPacketPoolHandle,
MIN_PACKET_POOL_SIZE,
MAX_PACKET_POOL_SIZE - MIN_PACKET_POOL_SIZE,
PROTOCOL_RESERVED_SIZE_IN_PACKET);
if (*Status != NDIS_STATUS_SUCCESS)
{
break;
}
//
// Now open the adapter below and complete the initialization
//
NdisOpenAdapter(Status,
&Sts,
&pAdapt->BindingHandle,
&MediumIndex,
MediumArray,
sizeof(MediumArray)/sizeof(NDIS_MEDIUM),
ProtHandle,
pAdapt,
DeviceName,
0,
NULL);
if (*Status == NDIS_STATUS_PENDING)
{
NdisWaitEvent(&pAdapt->Event, 0);
*Status = pAdapt->Status;
}
if (*Status != NDIS_STATUS_SUCCESS)
{
break;
}
PtReferenceAdapt(pAdapt);
#pragma prefast(suppress: __WARNING_POTENTIAL_BUFFER_OVERFLOW, "Ndis guarantees MediumIndex to be within bounds");
pAdapt->Medium = MediumArray[MediumIndex];
//
// Now ask NDIS to initialize our miniport (upper) edge.
// Set the flag below to synchronize with a
没有合适的资源?快使用搜索试试~ 我知道了~
简单的防火墙驱动程序
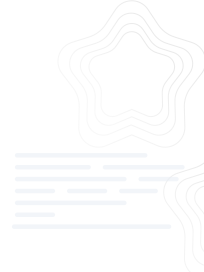
共82个文件
obj:11个
h:10个
pdb:6个


温馨提示
本程序是通过在小端口层和协议层之间添加一些过滤规则,来实现简单的网络信息过滤
资源推荐
资源详情
资源评论
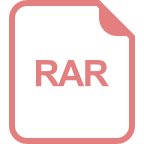
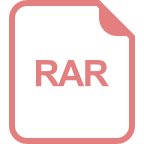
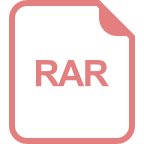
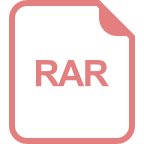
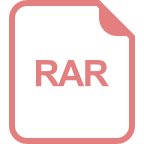
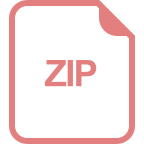
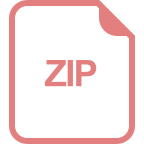
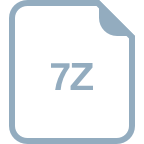
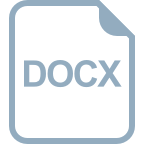
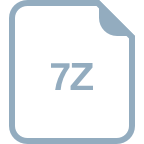
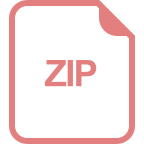
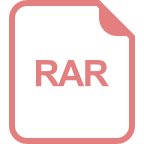
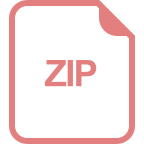
收起资源包目录




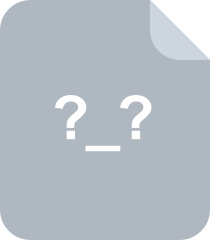
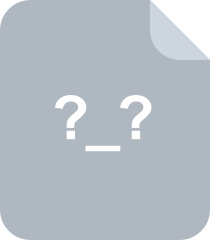
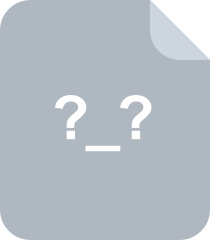
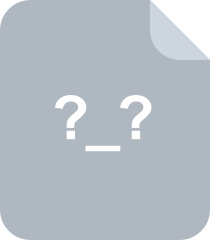
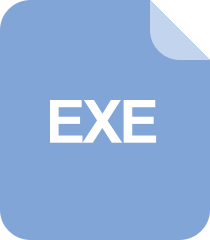
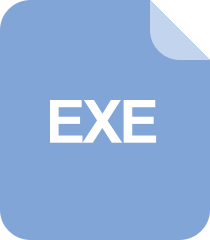
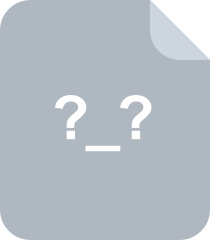
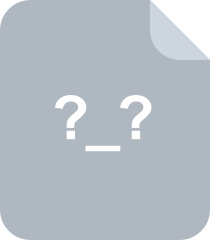

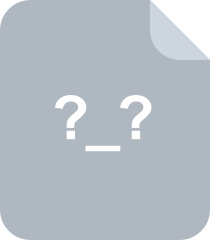
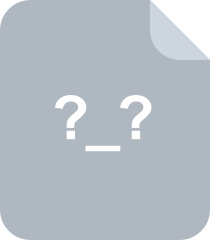
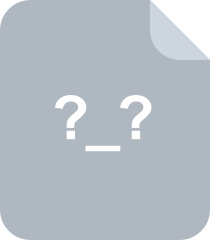
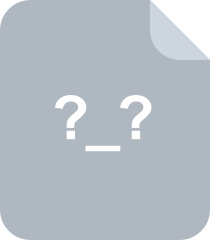
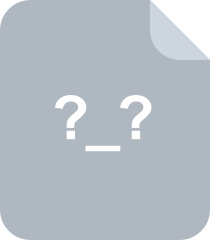

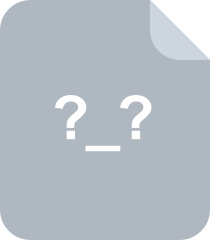
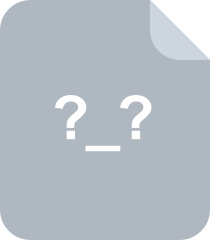
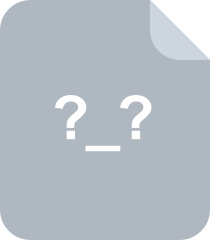
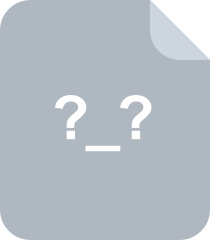
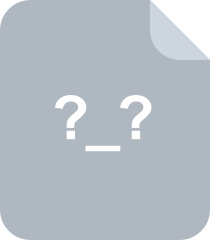
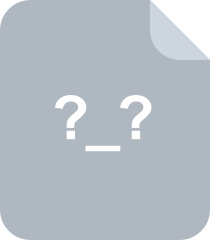
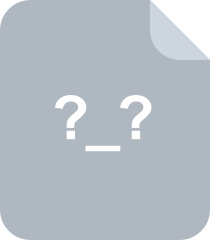
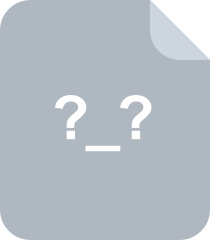
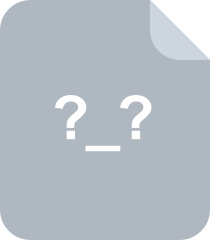
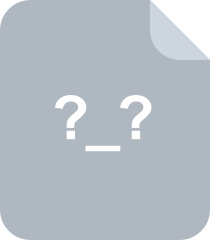
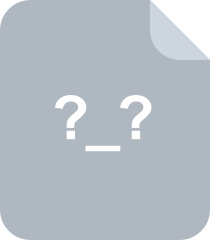
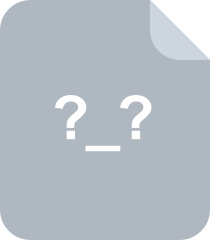
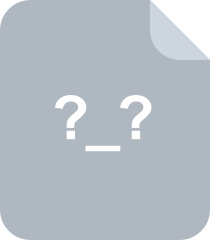
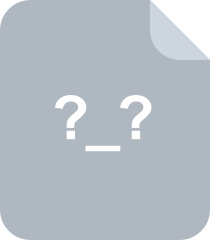
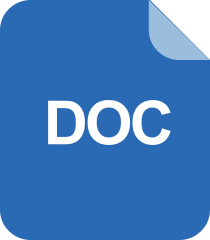

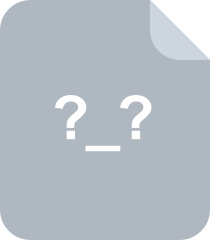
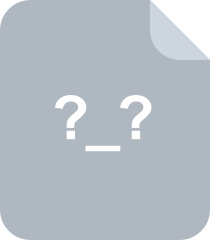
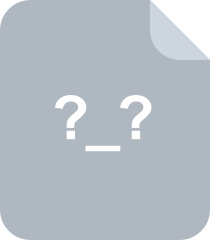
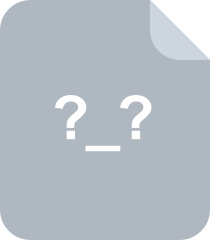
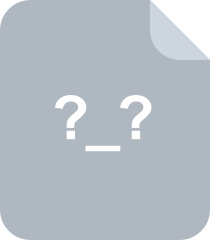
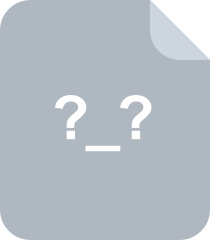
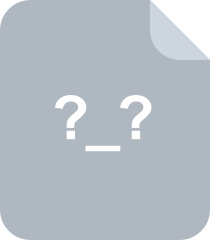
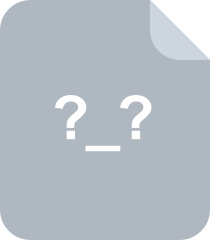
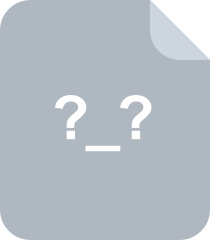
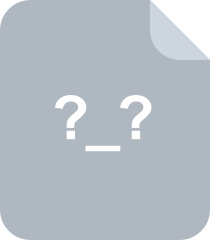
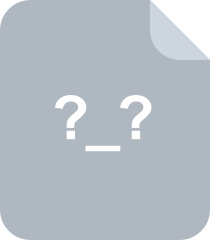

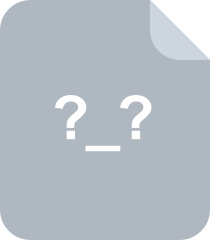
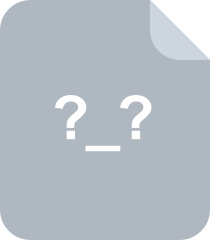
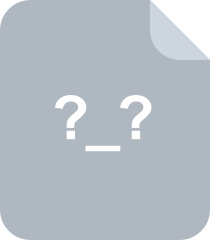
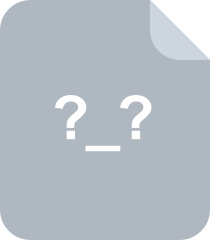




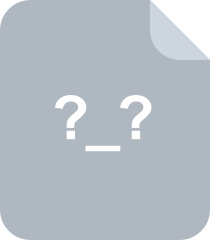
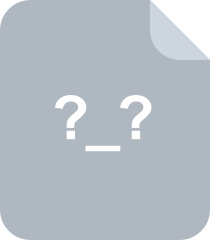
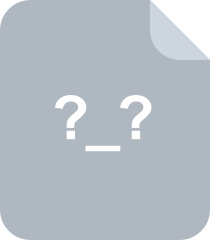
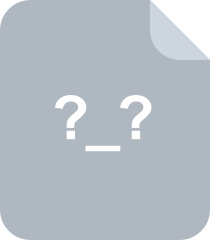
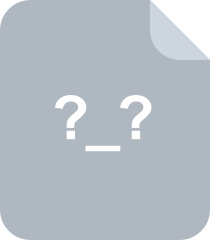
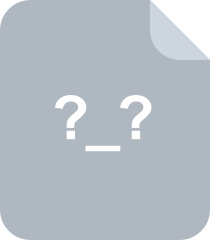
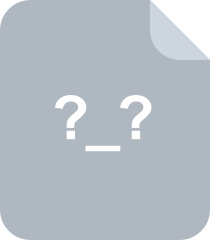
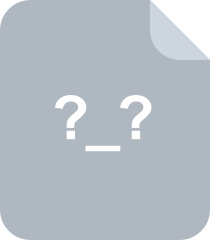
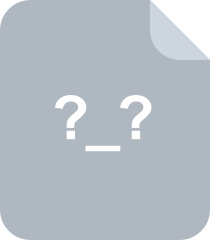
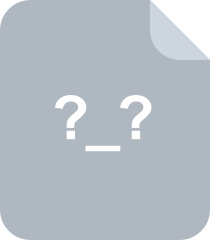
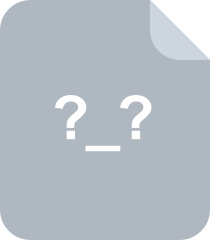
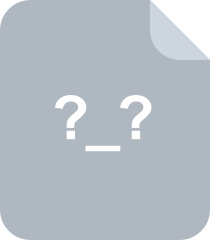
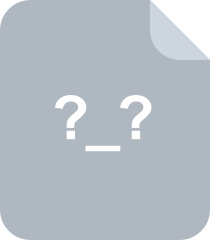
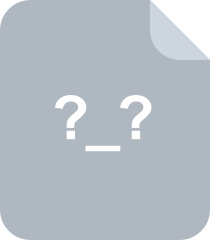
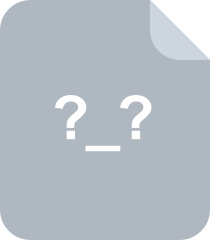
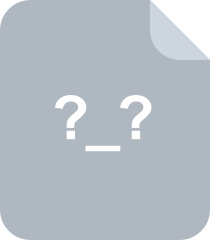
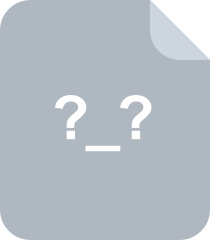

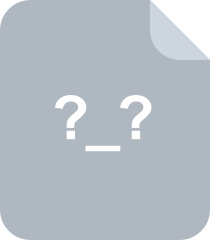
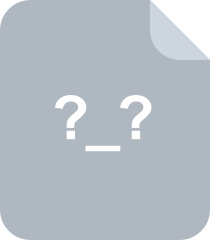
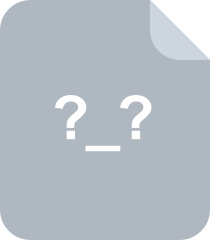
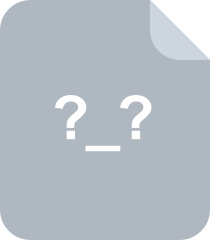
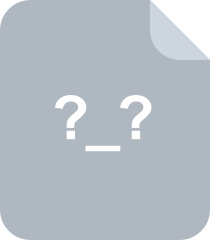
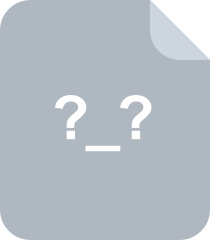

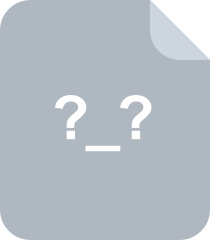
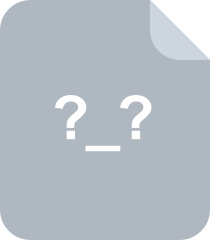
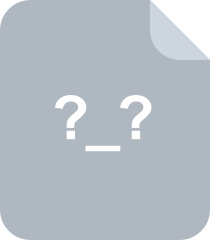
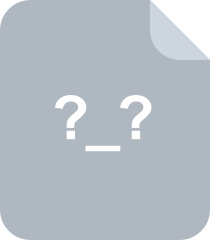
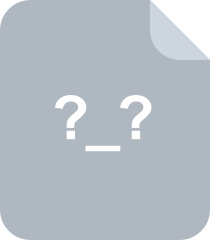
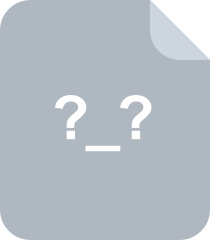
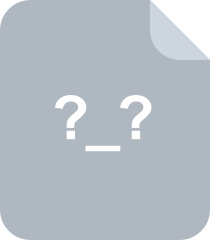
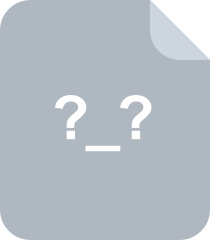
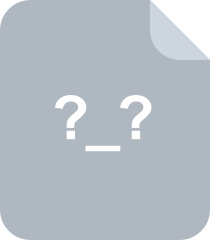
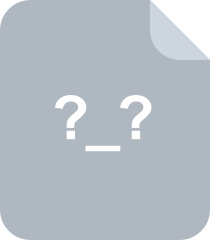
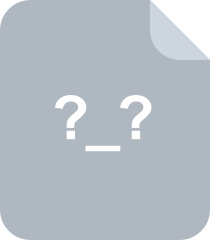
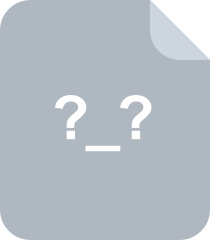
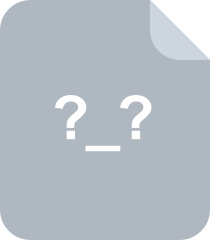

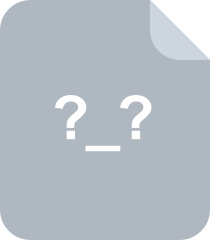
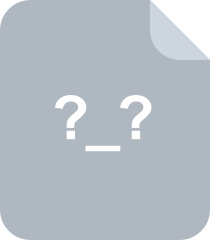
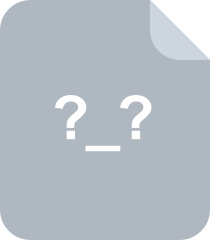
共 82 条
- 1
资源评论
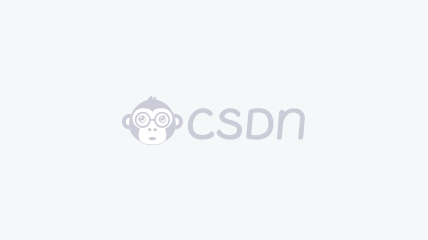
- zj88231292012-12-17程序还不错,但是文档不是很清晰
- y469222013-01-02还可以 有些有用
- kmxierong2012-07-08这个程序不错,可以用

huangjinli73
- 粉丝: 1
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

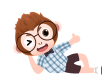
安全验证
文档复制为VIP权益,开通VIP直接复制
