"use strict";
var __spreadArray = (this && this.__spreadArray) || function (to, from, pack) {
if (pack || arguments.length === 2) for (var i = 0, l = from.length, ar; i < l; i++) {
if (ar || !(i in from)) {
if (!ar) ar = Array.prototype.slice.call(from, 0, i);
ar[i] = from[i];
}
}
return to.concat(ar || Array.prototype.slice.call(from));
};
var __importDefault = (this && this.__importDefault) || function (mod) {
return (mod && mod.__esModule) ? mod : { "default": mod };
};
Object.defineProperty(exports, "__esModule", { value: true });
var component_1 = require("../common/component");
var utils_1 = require("./utils");
var toast_1 = __importDefault(require("../toast/toast"));
var utils_2 = require("../common/utils");
var initialMinDate = (0, utils_1.getToday)().getTime();
var initialMaxDate = (function () {
var now = (0, utils_1.getToday)();
return new Date(now.getFullYear(), now.getMonth() + 6, now.getDate()).getTime();
})();
var getTime = function (date) {
return date instanceof Date ? date.getTime() : date;
};
(0, component_1.VantComponent)({
props: {
title: {
type: String,
value: '日期选择',
},
color: String,
show: {
type: Boolean,
observer: function (val) {
if (val) {
this.initRect();
this.scrollIntoView();
}
},
},
formatter: null,
confirmText: {
type: String,
value: '确定',
},
confirmDisabledText: {
type: String,
value: '确定',
},
rangePrompt: String,
showRangePrompt: {
type: Boolean,
value: true,
},
defaultDate: {
type: null,
observer: function (val) {
this.setData({ currentDate: val });
this.scrollIntoView();
},
},
allowSameDay: Boolean,
type: {
type: String,
value: 'single',
observer: 'reset',
},
minDate: {
type: Number,
value: initialMinDate,
},
maxDate: {
type: Number,
value: initialMaxDate,
},
position: {
type: String,
value: 'bottom',
},
rowHeight: {
type: null,
value: utils_1.ROW_HEIGHT,
},
round: {
type: Boolean,
value: true,
},
poppable: {
type: Boolean,
value: true,
},
showMark: {
type: Boolean,
value: true,
},
showTitle: {
type: Boolean,
value: true,
},
showConfirm: {
type: Boolean,
value: true,
},
showSubtitle: {
type: Boolean,
value: true,
},
safeAreaInsetBottom: {
type: Boolean,
value: true,
},
closeOnClickOverlay: {
type: Boolean,
value: true,
},
maxRange: {
type: null,
value: null,
},
firstDayOfWeek: {
type: Number,
value: 0,
},
readonly: Boolean,
},
data: {
subtitle: '',
currentDate: null,
scrollIntoView: '',
},
created: function () {
this.setData({
currentDate: this.getInitialDate(this.data.defaultDate),
});
},
mounted: function () {
if (this.data.show || !this.data.poppable) {
this.initRect();
this.scrollIntoView();
}
},
methods: {
reset: function () {
this.setData({ currentDate: this.getInitialDate() });
this.scrollIntoView();
},
initRect: function () {
var _this = this;
if (this.contentObserver != null) {
this.contentObserver.disconnect();
}
var contentObserver = this.createIntersectionObserver({
thresholds: [0, 0.1, 0.9, 1],
observeAll: true,
});
this.contentObserver = contentObserver;
contentObserver.relativeTo('.van-calendar__body');
contentObserver.observe('.month', function (res) {
if (res.boundingClientRect.top <= res.relativeRect.top) {
// @ts-ignore
_this.setData({ subtitle: (0, utils_1.formatMonthTitle)(res.dataset.date) });
}
});
},
limitDateRange: function (date, minDate, maxDate) {
if (minDate === void 0) { minDate = null; }
if (maxDate === void 0) { maxDate = null; }
minDate = minDate || this.data.minDate;
maxDate = maxDate || this.data.maxDate;
if ((0, utils_1.compareDay)(date, minDate) === -1) {
return minDate;
}
if ((0, utils_1.compareDay)(date, maxDate) === 1) {
return maxDate;
}
return date;
},
getInitialDate: function (defaultDate) {
var _this = this;
if (defaultDate === void 0) { defaultDate = null; }
var _a = this.data, type = _a.type, minDate = _a.minDate, maxDate = _a.maxDate;
var now = (0, utils_1.getToday)().getTime();
if (type === 'range') {
if (!Array.isArray(defaultDate)) {
defaultDate = [];
}
var _b = defaultDate || [], startDay = _b[0], endDay = _b[1];
var start = this.limitDateRange(startDay || now, minDate, (0, utils_1.getPrevDay)(new Date(maxDate)).getTime());
var end = this.limitDateRange(endDay || now, (0, utils_1.getNextDay)(new Date(minDate)).getTime());
return [start, end];
}
if (type === 'multiple') {
if (Array.isArray(defaultDate)) {
return defaultDate.map(function (date) { return _this.limitDateRange(date); });
}
return [this.limitDateRange(now)];
}
if (!defaultDate || Array.isArray(defaultDate)) {
defaultDate = now;
}
return this.limitDateRange(defaultDate);
},
scrollIntoView: function () {
var _this = this;
(0, utils_2.requestAnimationFrame)(function () {
var _a = _this.data, currentDate = _a.currentDate, type = _a.type, show = _a.show, poppable = _a.poppable, minDate = _a.minDate, maxDate = _a.maxDate;
// @ts-ignore
var targetDate = type === 'single' ? currentDate : currentDate[0];
var displayed = show || !poppable;
if (!targetDate || !displayed) {
return;
}
var months = (0, utils_1.getMonths)(minDate, maxDate);
months.some(function (month, index) {
if ((0, utils_1.compareMonth)(month, targetDate) === 0) {
_this.setData({ scrollIntoView: "month".concat(index) });
return true;
}
return false;
});
});
},
onOpen: function () {
this.$emit('open');
},
onOpened: function () {
this.$emit('opened');
},
onClose: function () {
this.$emit('close');
},
onClosed: function () {
this.$emit('closed');
},
onClickDay: function (event) {
if (this.data.readonly) {
return;
}
var date = event.detail.date;
var _a = this.data, type =
miniprogram-demo
需积分: 0 196 浏览量
2023-06-29
12:12:37
上传
评论
收藏 4.02MB ZIP 举报

》》》飞得更高《《《
- 粉丝: 0
- 资源: 3
最新资源
- 160501_20161201064907.zip
- 一个简洁的Python实现,使用了字典来存储图的信息,并构建了一个函数 dijkstra 来找到源点到所有其他节点的最短路径
- STM32输出PWM电压值
- apache-doris-build-env-for-2.0.d
- 使用Python编写一个分段函数,并将代码保存在一个文件中
- vscode插件合集vscode插件合集
- 基于OpenCV-Python 相机标定及矫正,张正友相机标定法
- tkinter的ttk主题:sun-valley-ttk-theme-main
- 基于 python实现的读取PDF中特定信息,插入到Word文档特定位置
- tkinter的ttk主题
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


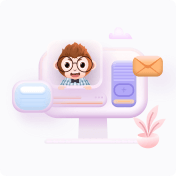