# Deploy Spring Boot and Docker Microservices to AWS using ECS and AWS Fargate
[](https://www.udemy.com/course/deploy-spring-microservices-to-aws-with-ecs-and-aws-fargate/)
## Learn Amazon Web Services - AWS - deploying Spring Boot and Docker Microservices to AWS Fargate. Implement Service Discovery, Load Balancing, Auto Discovery, Centralized Configuration and Distributed Tracing in AWS.
Spring Boot is the No 1 Java Framework to develop REST API and Microservices. AWS (Amazon Web Services) is the No 1 Cloud Service Provider today.
How about learning AWS by deploying Spring Boot Docker Containers to Amazon Web Services using Elastic Container Service - ECS and AWS Fargate?
## Getting Started
- [Video - Docker in 5 Steps](https://youtu.be/Rt5G5Gj7RP0)
- [Video - Spring in 10 Steps](https://www.youtube.com/watch?v=edgZo2g-LTM)
- [Video - Spring Boot in 10 Steps](https://www.youtube.com/watch?v=pcdpk3Yd1EA)
- [Video - JPA/Hibernate in 10 Steps](https://www.youtube.com/watch?v=MaI0_XdpdP8)
- [AWS Code Pipeline Github Repo](https://github.com/in28minutes/hello-world-rest-api-aws-ecs-codepipeline)
## Container Images
| Application | Container |
| ------------------------------- | --------------------------------------------- |
| Hello World | in28min/aws-hello-world-rest-api:1.0.0-RELEASE |
| Simple Task | in28min/aws-simple-spring-task:1.0.0-RELEASE |
| CurrencyExchangeMicroservice-H2 | in28min/aws-currency-exchange-service-h2:0.0.1-SNAPSHOT |
| CurrencyExchangeMicroservice-H2 - V2 | in28min/aws-currency-exchange-service-h2:1.0.1-RELEASE|
| CurrencyExchangeMicroSevice-MySQL| in28min/aws-currency-exchange-service-mysql:0.0.1-SNAPSHOT|
| CurrencyConversionMicroservice | in28min/aws-currency-conversion-service:0.0.1-SNAPSHOT |
| Currency Exchange - X Ray | in28min/aws-currency-exchange-service-h2-xray:0.0.1-SNAPSHOT|
| Currency Conversion - X Ray | in28min/aws-currency-conversion-service-xray:0.0.1-SNAPSHOT|
| Utility | Container Image |
| ------------- | ------------------------- |
| aws-xray-daemon| amazon/aws-xray-daemon:1|
## Microservice URLs and Details
### Currency Exchange Service
- PORT - 8000
- URL - `http://localhost:8000/api/currency-exchange-microservice/currency-exchange/from/EUR/to/INR`
- HEALTH URL - `http://localhost:8000/api/currency-exchange-microservice/manage/health`
- Enviroment Variables
- SSM URN - `arn:aws:ssm:us-east-1:<account-id>:parameter/<name>`
- /dev/currency-exchange-service/RDS_DB_NAME - exchange_db
- /dev/currency-exchange-service/RDS_HOSTNAME
- /dev/currency-exchange-service/RDS_PASSWORD
- /dev/currency-exchange-service/RDS_PORT - 3306
- /dev/currency-exchange-service/RDS_USERNAME - exchange_db_user
### Currency Conversion Service
- PORT - 8100
- URL - `http://localhost:8100/api/currency-conversion-microservice/currency-converter/from/USD/to/INR/quantity/10`
- HEALTH URL - `http://localhost:8100/api/currency-conversion-microservice/manage/health`
- Enviroment Variables
- SSM URN - `arn:aws:ssm:us-east-1:<account-id>:parameter/<name>`
- /dev/currency-conversion-service/CURRENCY_EXCHANGE_URI
## Enviroment Variables
SSM URN - `arn:aws:ssm:us-east-1:<account-id>:parameter/<name>`
- /dev/currency-conversion-service/CURRENCY_EXCHANGE_URI
- /dev/currency-exchange-service/RDS_DB_NAME - exchange_db
- /dev/currency-exchange-service/RDS_HOSTNAME
- /dev/currency-exchange-service/RDS_PASSWORD
- /dev/currency-exchange-service/RDS_PORT - 3306
- /dev/currency-exchange-service/RDS_USERNAME - exchange_db_user
## Setting up App Mesh
#### Virtual nodes
- currency-exchange-service-vn - currency-exchange-service.in28minutes-dev.com
- currency-conversion-service-vn - currency-conversion-service.in28minutes-dev.com
#### Virtual services
- currency-exchange-service.in28minutes-dev.com -> currency-exchange-service-vn
- currency-conversion-service.in28minutes-dev.com -> currency-conversion-service-vn
#### Backend Registration
- currency-conversion-service-vn -> currency-exchange-service.in28minutes-dev.com
#### Task Definition Updates
- aws-currency-conversion-service
- aws-currency-exchange-service-h2
- ```ENVOY_LOG_LEVEL-trace, ENABLE_ENVOY_XRAY_TRACING-1```
#### Service Updates
- aws-currency-conversion-service-appmesh
- aws-currency-exchange-service-appmesh
## Deploying Version 2 of Currency Exchange Service to ECS and App Mesh
#### App Mesh - New Virtual Node
currency-exchange-service-v2-vn - currency-exchange-service-v2.in28minutes-dev.com
#### ECS Fargate - Update Task Definition
aws-currency-exchange-service-h2
- in28min/aws-currency-exchange-service-h2:1.0.1-RELEASE
- Use New Virtual Node
#### ECS Fargate - Create New Service
aws-currency-exchange-service-v2-appmesh
- Service Discovery - currency-exchange-service-v2
#### App Mesh - Create Virtual Router
currency-exchange-service-vr distributing traffic to
- currency-exchange-service-vn
- currency-exchange-service-v2-vn
#### App Mesh - Update Service to Use Virtual Router
currency-exchange-service.in28minutes-dev.com -> currency-exchange-service-vr
#### jq
```
sudo yum install jq
```
#### AWS CLI Hosted Zones
```
aws --version
aws configure
aws servicediscovery list-services
aws servicediscovery delete-service --id={id}
aws servicediscovery list-services
aws servicediscovery list-namespaces
aws servicediscovery delete-namespace --id={id}
aws servicediscovery list-namespaces
aws servicediscovery delete-service --id=srv-7q3fkztnbo6aa5kc
aws servicediscovery delete-service --id=srv-mdybugm4bh5u4ugx
aws servicediscovery delete-service --id=srv-7upzjx3mhfleyfoz
aws servicediscovery delete-namespace --id=ns-ctvtysasurklojm3
```
## Installation Guides
#### Required Tools
- Java 8+
- Eclipse - Oxygen+ - (Embedded Maven From Eclipse)
- Git
- Docker
#### Installing Guides
- [Playlist - Installing Java, Eclipse & Embedded Maven](https://www.youtube.com/playlist?list=PLBBog2r6uMCSmMVTW_QmDLyASBvovyAO3)
#### Troubleshooting Installations
- Eclipse and Embedded Maven
- Troubleshooting Guide : https://github.com/in28minutes/in28minutes-initiatives/tree/master/The-in28Minutes-TroubleshootingGuide-And-FAQ#tip--troubleshooting-embedded-maven-in-eclipse
- PDF : https://github.com/in28minutes/SpringIn28Minutes/blob/master/InstallationGuide-JavaEclipseAndMaven_v2.pdf
- GIT Repository For Installation : https://github.com/in28minutes/getting-started-in-5-steps
## Course Overview
This course would be a perfect first step as an introduction to Amazon Web Services - AWS and the Cloud.
In this course, we deploy a variety of Java Spring Boot Microservices to Amazon Web Services using AWS Fargate and ECS - Elastic Container Service.
You will learn the basics of implementing Container Orchestration with ECS (Elastic Container Service) - Cluster, Task Definitions, Tasks, Containers and Services. You will learn about the two launch types of ECS - EC2 and AWS Fargate. In this course, we would focus extensively on AWS Fargate to simplify your Container Orchestration. You will learn to deploy multiple containers in the same ECS task.
You will learn to Build Container Images for your Java Spring Boot Microservice Projects.
You will implement the following features for your Microservices
- Centralized Configuration Management with AWS Parameter Store
- Distributed Tracing with AWS X Ray
- Auto Scaling and Load Balancing with ECS, Elastic Load Balancers and Target Groups
- Service Mesh using AWS App Mesh. You will learn the basics of AWS App Mesh - Mesh, Virtual Nodes and Virtual Services. You will learn to perform
没有合适的资源?快使用搜索试试~ 我知道了~
deploy-spring-microservices-to-aws-ecs-fargate毕业设计&课设_.zip
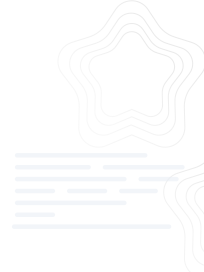
共25个文件
java:12个
md:4个
xml:3个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 23 浏览量
2024-11-14
17:42:18
上传
评论
收藏 23KB ZIP 举报
温馨提示
1、资源项目源码均已通过严格测试验证,保证能够正常运行; 2、项目问题、技术讨论,可以给博主私信或留言,博主看到后会第一时间与您进行沟通; 3、本项目比较适合计算机领域相关的毕业设计课题、课程作业等使用,尤其对于人工智能、计算机科学与技术等相关专业,更为适合; 4、下载使用后,可先查看README.md文件(如有),本项目仅用作交流学习参考,请切勿用于商业用途。
资源推荐
资源详情
资源评论
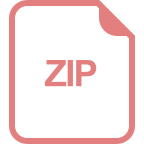
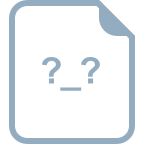
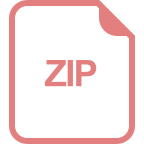
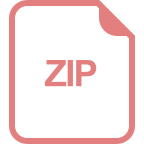
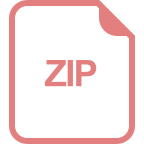
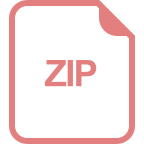
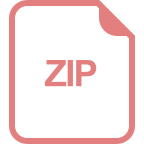
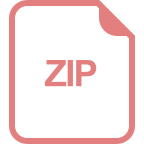
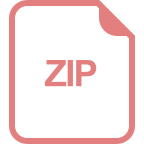
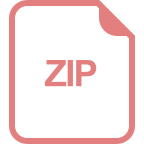
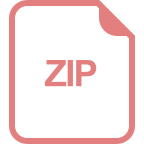
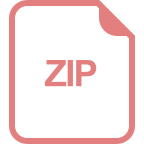
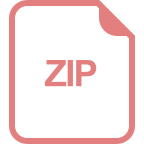
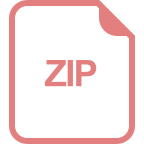
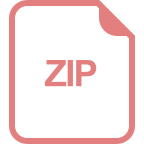
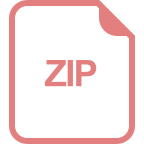
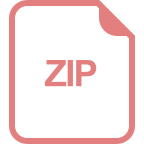
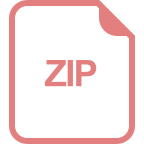
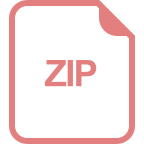
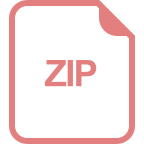
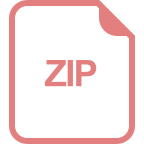
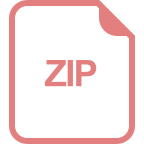
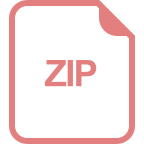
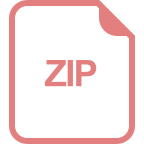
收起资源包目录



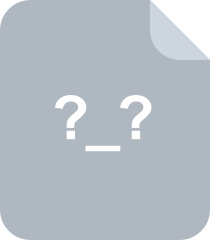








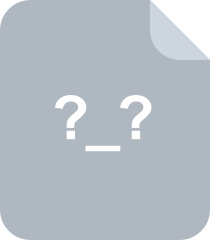


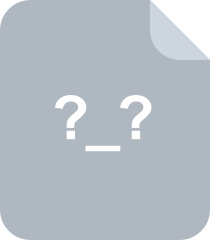
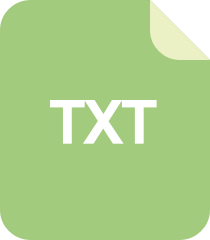






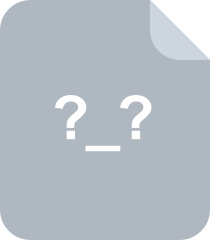
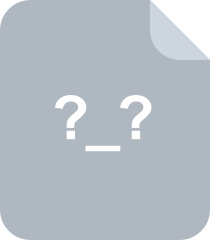
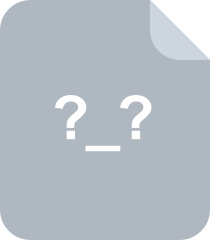
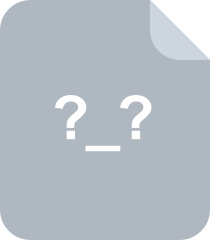
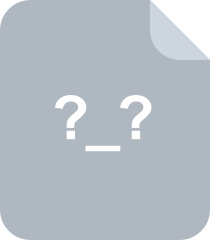

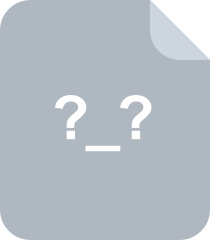








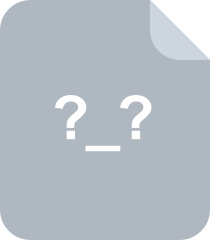


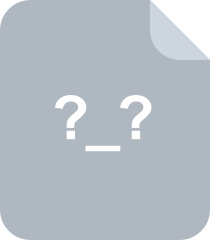






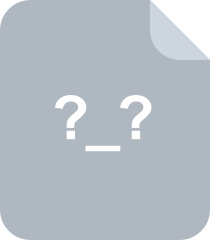
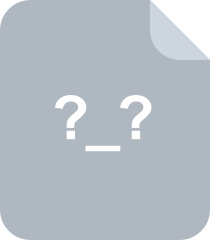
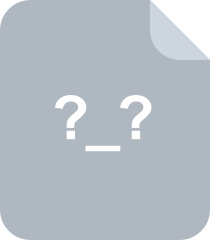
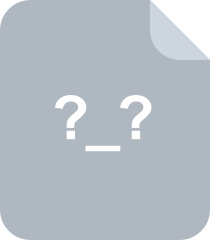
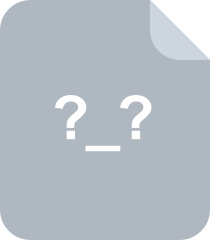

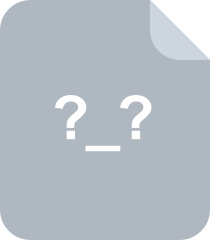







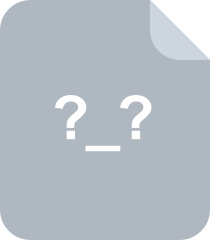

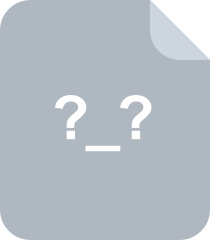
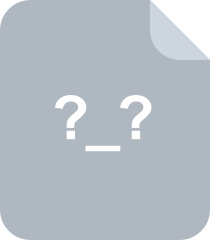
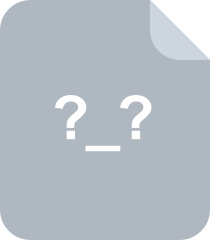
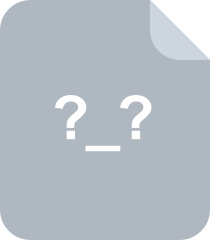
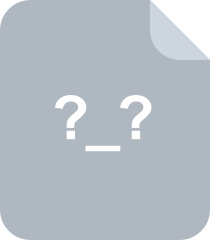
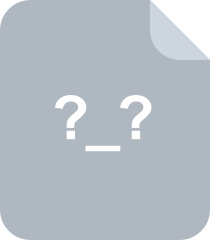
共 25 条
- 1
资源评论
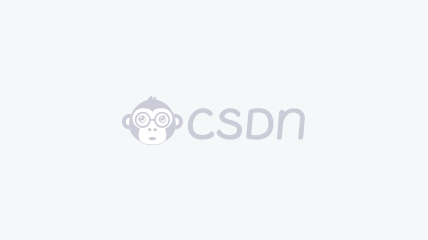

pk_xz123456
- 粉丝: 2860
- 资源: 4045
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

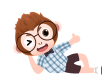
最新资源
- XX人民法院大楼安保系统整体解决方案Word(103页).docx
- 法院大楼安保系统整体解决方案PPT(25页).pptx
- 法院办公楼智能化规划设计方案PPT(96页).pptx
- 法院安防系统解决方案Word(77页).docx
- 法院高清智能庭审系统解决方案PPT(28页).pptx
- 法院大楼无线网络解决方案Word(26页).doc
- 法院大楼安保系统整体解决方案Word(85页).docx
- 法院执行指挥调度系统Word(33页).docx
- 法院执行指挥调度系统解决方案PPT(31页).pptx
- 法院执行指挥调度系统解决方案Word(57页).docx
- 法院综合安全监管平台解决方案PPT(53页).pptx
- 法院综合安全监管平台解决方案(深信服)PPT(53页).pptx
- 53页-智慧法院解决方案.pdf
- 43页-智慧法院庭审系统解决方案.pdf
- 学生作业-QQ音乐首页 该项目为html前端项目,主要QQ音乐首页列表 涉及html、js、css
- 基于QT的智慧交通管理系统(Day1)中的image文件
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


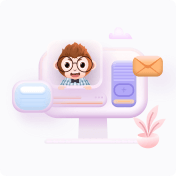
安全验证
文档复制为VIP权益,开通VIP直接复制
