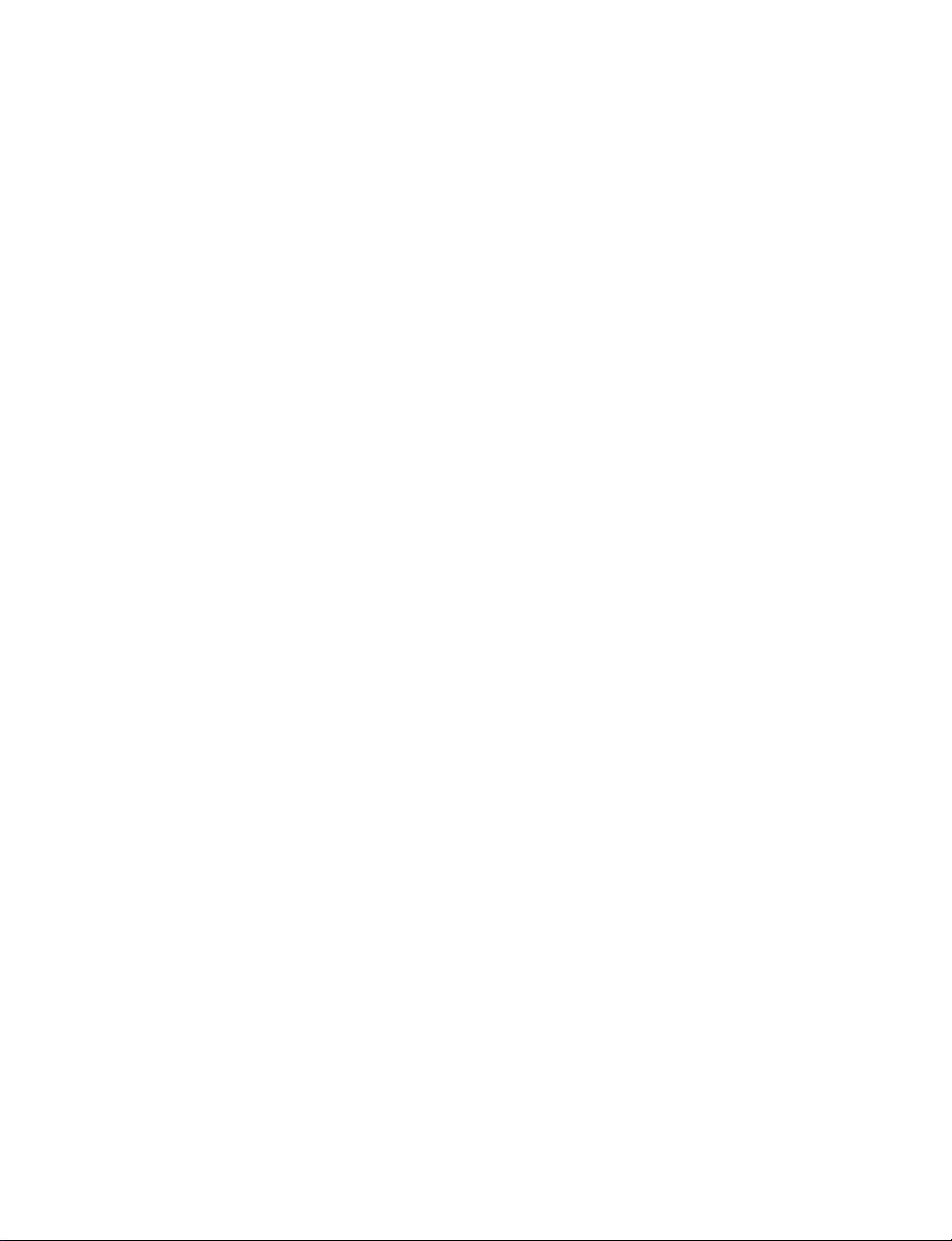
The Java
®
Language
Specification
Java SE 9 Edition
James Gosling
Bill Joy
Guy Steele
Gilad Bracha
Alex Buckley
Daniel Smith
2017-08-07
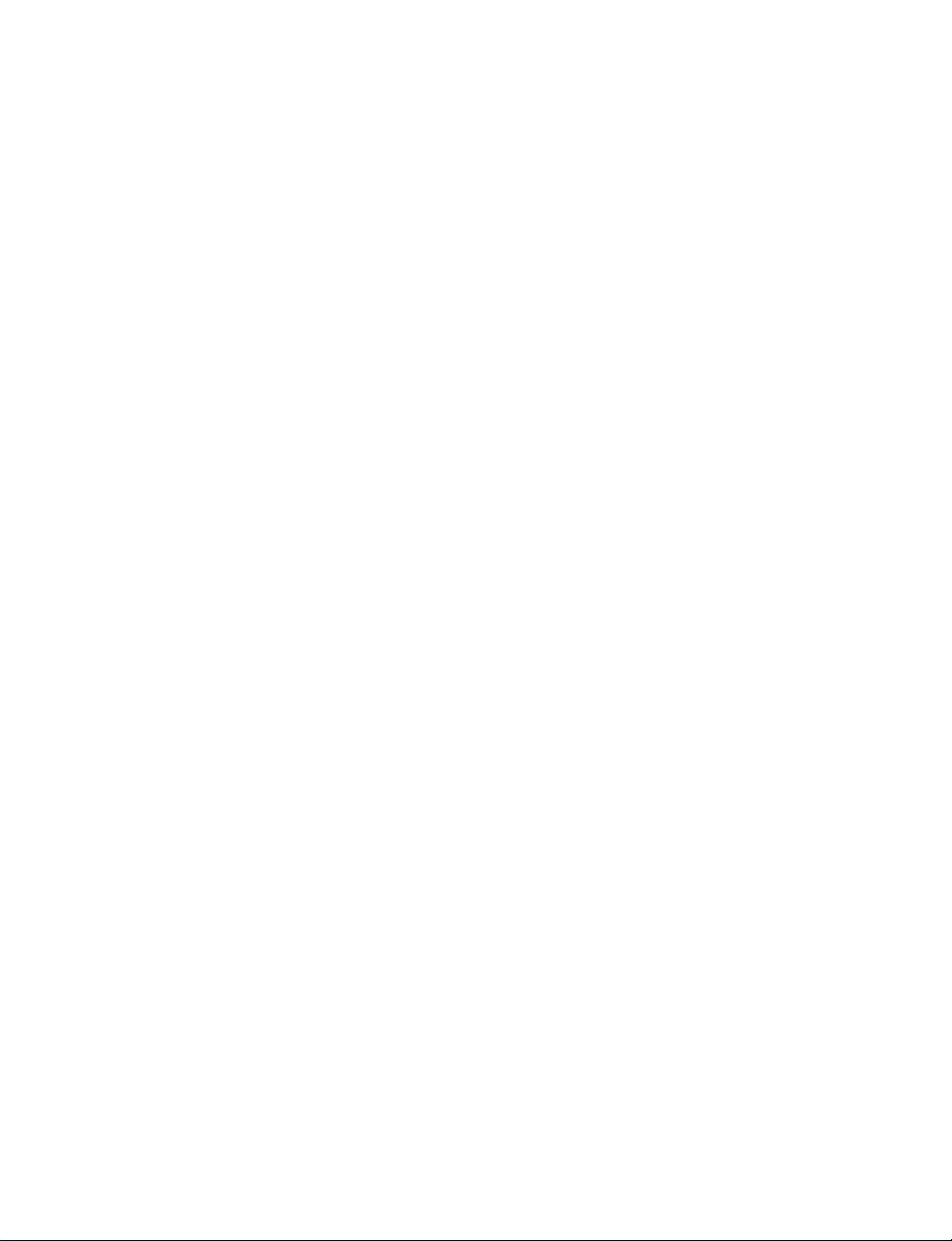
Specification: JSR-379 Java
®
SE 9 Release Contents ("Specification")
Version: 9
Status: Final Release
Release: September 2017
Copyright © 1997, 2017, Oracle America, Inc. and/or its affiliates.
500 Oracle Parkway, Redwood City, California 94065, U.S.A.
All rights reserved.
Oracle and Java are registered trademarks of Oracle and/or its affiliates. Other names may
be trademarks of their respective owners.
The Specification provided herein is provided to you only under the Limited License Grant
included herein as Appendix A. Please see Appendix A, Limited License Grant.
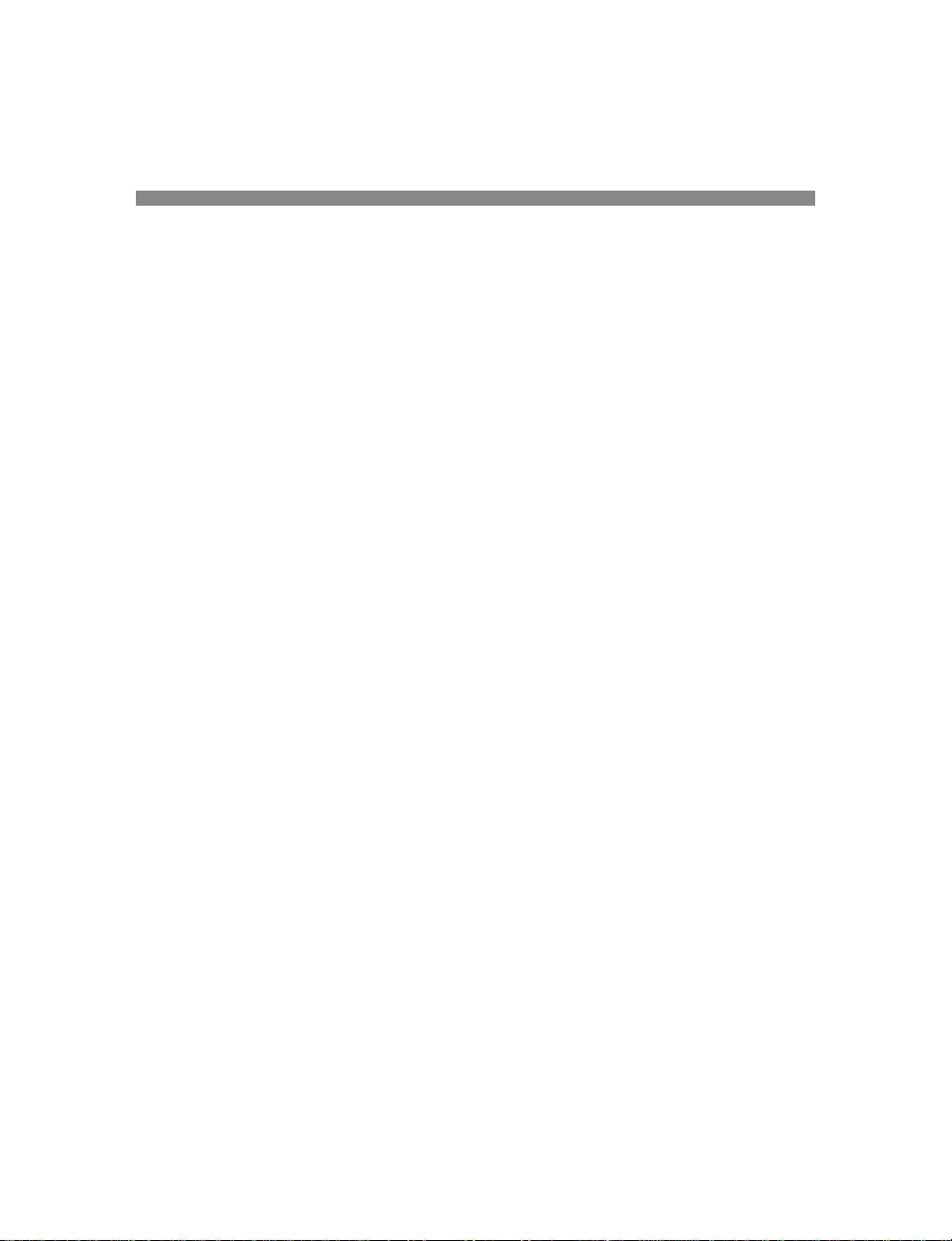
iii
Table of Contents
1
Introduction 1
1.1 Organization of the Specification 2
1.2 Example Programs 6
1.3 Notation 6
1.4 Relationship to Predefined Classes and Interfaces 7
1.5 Feedback 7
1.6 References 7
2
Grammars 9
2.1 Context-Free Grammars 9
2.2 The Lexical Grammar 9
2.3 The Syntactic Grammar 10
2.4 Grammar Notation 10
3
Lexical Structure 15
3.1 Unicode 15
3.2 Lexical Translations 16
3.3 Unicode Escapes 17
3.4 Line Terminators 19
3.5 Input Elements and Tokens 19
3.6 White Space 20
3.7 Comments 21
3.8 Identifiers 22
3.9 Keywords 24
3.10 Literals 25
3.10.1 Integer Literals 25
3.10.2 Floating-Point Literals 32
3.10.3 Boolean Literals 35
3.10.4 Character Literals 35
3.10.5 String Literals 36
3.10.6 Escape Sequences for Character and String Literals 38
3.10.7 The Null Literal 39
3.11 Separators 40
3.12 Operators 40
4
Types, Values, and Variables 41
4.1 The Kinds of Types and Values 41
4.2 Primitive Types and Values 42
4.2.1 Integral Types and Values 43
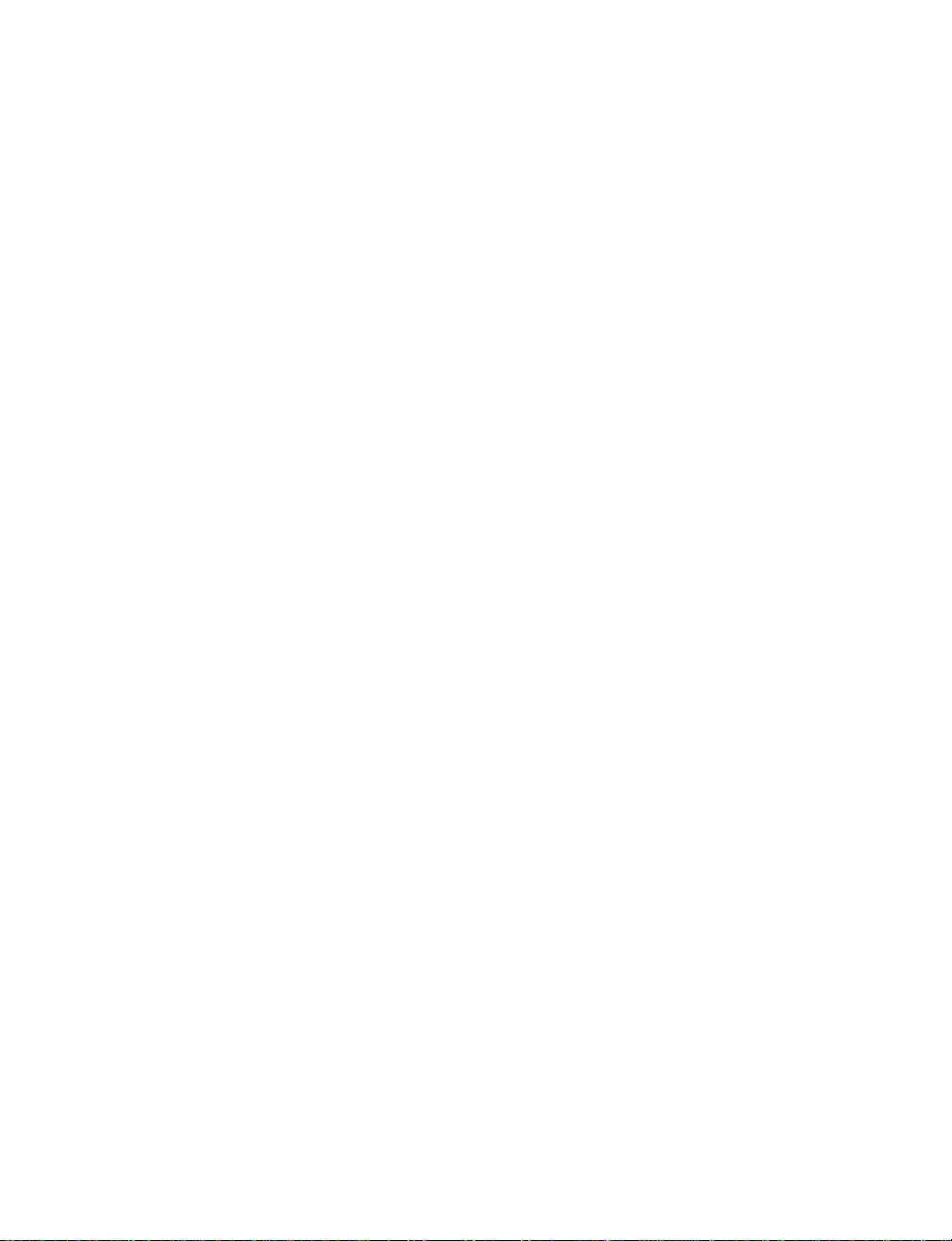
The Java
®
Language Specification
iv
4.2.2 Integer Operations 43
4.2.3 Floating-Point Types, Formats, and Values 45
4.2.4 Floating-Point Operations 48
4.2.5 The boolean Type and boolean Values 51
4.3 Reference Types and Values 52
4.3.1 Objects 53
4.3.2 The Class Object 56
4.3.3 The Class String 56
4.3.4 When Reference Types Are the Same 57
4.4 Type Variables 57
4.5 Parameterized Types 59
4.5.1 Type Arguments of Parameterized Types 60
4.5.2 Members and Constructors of Parameterized Types 63
4.6 Type Erasure 64
4.7 Reifiable Types 65
4.8 Raw Types 66
4.9 Intersection Types 70
4.10 Subtyping 71
4.10.1 Subtyping among Primitive Types 71
4.10.2 Subtyping among Class and Interface Types 72
4.10.3 Subtyping among Array Types 73
4.10.4 Least Upper Bound 73
4.11 Where Types Are Used 76
4.12 Variables 80
4.12.1 Variables of Primitive Type 81
4.12.2 Variables of Reference Type 81
4.12.3 Kinds of Variables 83
4.12.4 final Variables 85
4.12.5 Initial Values of Variables 87
4.12.6 Types, Classes, and Interfaces 88
5
Conversions and Contexts 93
5.1 Kinds of Conversion 96
5.1.1 Identity Conversion 96
5.1.2 Widening Primitive Conversion 96
5.1.3 Narrowing Primitive Conversion 98
5.1.4 Widening and Narrowing Primitive Conversion 101
5.1.5 Widening Reference Conversion 101
5.1.6 Narrowing Reference Conversion 101
5.1.6.1 Allowed Narrowing Reference Conversion 102
5.1.6.2 Checked and Unchecked Narrowing Reference
Conversions 103
5.1.6.3 Narrowing Reference Conversions at Run Time 103
5.1.7 Boxing Conversion 105
5.1.8 Unboxing Conversion 107
5.1.9 Unchecked Conversion 108
5.1.10 Capture Conversion 109
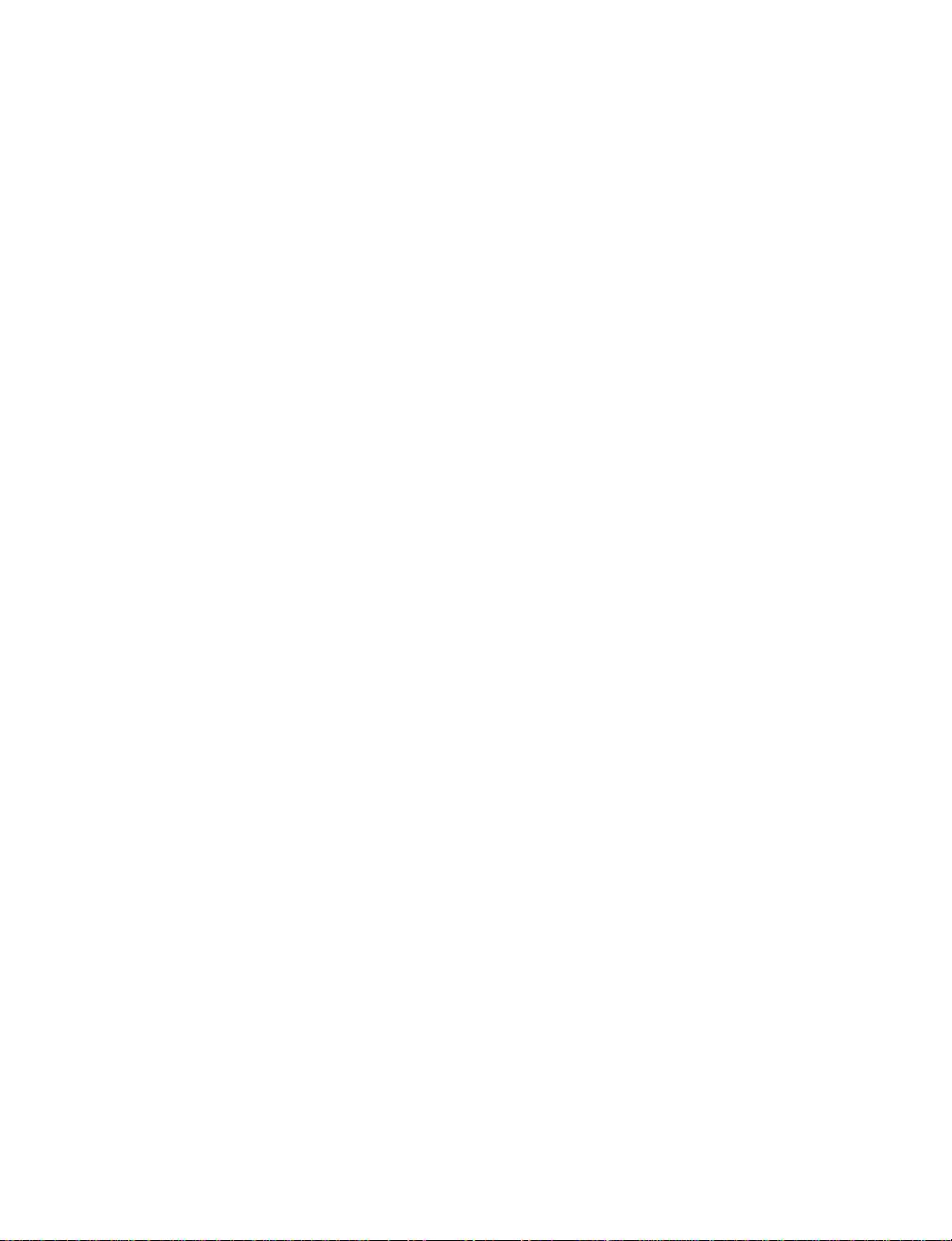
The Java
®
Language Specification
v
5.1.11 String Conversion 111
5.1.12 Forbidden Conversions 112
5.1.13 Value Set Conversion 112
5.2 Assignment Contexts 113
5.3 Invocation Contexts 118
5.4 String Contexts 120
5.5 Casting Contexts 120
5.6 Numeric Contexts 126
5.6.1 Unary Numeric Promotion 127
5.6.2 Binary Numeric Promotion 128
6
Names 131
6.1 Declarations 132
6.2 Names and Identifiers 139
6.3 Scope of a Declaration 141
6.4 Shadowing and Obscuring 145
6.4.1 Shadowing 147
6.4.2 Obscuring 150
6.5 Determining the Meaning of a Name 151
6.5.1 Syntactic Classification of a Name According to Context 152
6.5.2 Reclassification of Contextually Ambiguous Names 155
6.5.3 Meaning of Module Names and Package Names 157
6.5.3.1 Simple Package Names 157
6.5.3.2 Qualified Package Names 158
6.5.4 Meaning of PackageOrTypeNames 158
6.5.4.1 Simple PackageOrTypeNames 158
6.5.4.2 Qualified PackageOrTypeNames 158
6.5.5 Meaning of Type Names 158
6.5.5.1 Simple Type Names 158
6.5.5.2 Qualified Type Names 158
6.5.6 Meaning of Expression Names 159
6.5.6.1 Simple Expression Names 159
6.5.6.2 Qualified Expression Names 160
6.5.7 Meaning of Method Names 163
6.5.7.1 Simple Method Names 163
6.6 Access Control 164
6.6.1 Determining Accessibility 165
6.6.2 Details on protected Access 169
6.6.2.1 Access to a protected Member 170
6.6.2.2 Access to a protected Constructor 170
6.7 Fully Qualified Names and Canonical Names 172
7
Packages and Modules 175
7.1 Package Members 176
7.2 Host Support for Modules and Packages 177
7.3 Compilation Units 180
7.4 Package Declarations 181