package org.springframework.orm.jdo;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
import java.util.Collection;
import java.util.Map;
import javax.jdo.JDOException;
import javax.jdo.PersistenceManager;
import javax.jdo.PersistenceManagerFactory;
import javax.jdo.Query;
import org.springframework.dao.DataAccessException;
import org.springframework.dao.InvalidDataAccessApiUsageException;
import org.springframework.util.ClassUtils;
import org.springframework.util.ReflectionUtils;
public class JdoTemplate extends JdoAccessor
implements JdoOperations
{
private static Method newObjectIdInstanceMethod;
private static Method makePersistentMethod;
private static Method makePersistentAllMethod;
private boolean allowCreate = true;
private boolean exposeNativePersistenceManager = false;
public JdoTemplate(PersistenceManagerFactory pmf)
{
setPersistenceManagerFactory(pmf);
afterPropertiesSet();
}
public JdoTemplate(PersistenceManagerFactory pmf, boolean allowCreate)
{
setPersistenceManagerFactory(pmf);
setAllowCreate(allowCreate);
afterPropertiesSet();
}
public void setAllowCreate(boolean allowCreate)
{
this.exposeNativePersistenceManager = allowCreate;
}
public boolean isAllowCreate()
{
return this.exposeNativePersistenceManager;
}
public void setExposeNativePersistenceManager(boolean exposeNativePersistenceManager)
{
this.exposeNativePersistenceManager = exposeNativePersistenceManager;
}
public boolean isExposeNativePersistenceManager()
{
return this.exposeNativePersistenceManager;
}
public Object execute(JdoCallback action) throws DataAccessException
{
return execute(action, isExposeNativePersistenceManager());
}
public Collection executeFind(JdoCallback action) throws DataAccessException {
Object result = execute(action, isExposeNativePersistenceManager());
if ((result != null) && (!(result instanceof Collection))) {
throw new InvalidDataAccessApiUsageException("Result object returned from JdoCallback isn't a Collection: [" + result + "]");
}
return ((Collection)result); }
// ERROR //
public Object execute(JdoCallback action, boolean exposeNativePersistenceManager) throws DataAccessException { // Byte code:
// 0: aload_1
// 1: ldc 27
// 3: invokestatic 28 org/springframework/util/Assert:notNull (Ljava/lang/Object;Ljava/lang/String;)V
// 6: aload_0
// 7: invokevirtual 29 org/springframework/orm/jdo/JdoTemplate:getPersistenceManagerFactory ()Ljavax/jdo/PersistenceManagerFactory;
// 10: aload_0
// 11: invokevirtual 30 org/springframework/orm/jdo/JdoTemplate:isAllowCreate ()Z
// 14: invokestatic 31 org/springframework/orm/jdo/PersistenceManagerFactoryUtils:getPersistenceManager (Ljavax/jdo/PersistenceManagerFactory;Z)Ljavax/jdo/PersistenceManager;
// 17: astore_3
// 18: aload_0
// 19: invokevirtual 29 org/springframework/orm/jdo/JdoTemplate:getPersistenceManagerFactory ()Ljavax/jdo/PersistenceManagerFactory;
// 22: invokestatic 32 org/springframework/transaction/support/TransactionSynchronizationManager:hasResource (Ljava/lang/Object;)Z
// 25: istore 4
// 27: iload_2
// 28: ifeq +7 -> 35
// 31: aload_3
// 32: goto +8 -> 40
// 35: aload_0
// 36: aload_3
// 37: invokevirtual 33 org/springframework/orm/jdo/JdoTemplate:createPersistenceManagerProxy (Ljavax/jdo/PersistenceManager;)Ljavax/jdo/PersistenceManager;
// 40: astore 5
// 42: aload_1
// 43: aload 5
// 45: invokeinterface 34 2 0
// 50: astore 6
// 52: aload_0
// 53: aload_3
// 54: iload 4
// 56: invokevirtual 35 org/springframework/orm/jdo/JdoTemplate:flushIfNecessary (Ljavax/jdo/PersistenceManager;Z)V
// 59: aload_0
// 60: aload 6
// 62: aload_3
// 63: iload 4
// 65: invokevirtual 36 org/springframework/orm/jdo/JdoTemplate:postProcessResult (Ljava/lang/Object;Ljavax/jdo/PersistenceManager;Z)Ljava/lang/Object;
// 68: astore 7
// 70: aload_3
// 71: aload_0
// 72: invokevirtual 29 org/springframework/orm/jdo/JdoTemplate:getPersistenceManagerFactory ()Ljavax/jdo/PersistenceManagerFactory;
// 75: invokestatic 37 org/springframework/orm/jdo/PersistenceManagerFactoryUtils:releasePersistenceManager (Ljavax/jdo/PersistenceManager;Ljavax/jdo/PersistenceManagerFactory;)V
// 78: aload 7
// 80: areturn
// 81: astore 5
// 83: aload_0
// 84: aload 5
// 86: invokevirtual 39 org/springframework/orm/jdo/JdoTemplate:convertJdoAccessException (Ljavax/jdo/JDOException;)Lorg/springframework/dao/DataAccessException;
// 89: athrow
// 90: astore 5
// 92: aload 5
// 94: athrow
// 95: astore 8
// 97: aload_3
// 98: aload_0
// 99: invokevirtual 29 org/springframework/orm/jdo/JdoTemplate:getPersistenceManagerFactory ()Ljavax/jdo/PersistenceManagerFactory;
// 102: invokestatic 37 org/springframework/orm/jdo/PersistenceManagerFactoryUtils:releasePersistenceManager (Ljavax/jdo/PersistenceManager;Ljavax/jdo/PersistenceManagerFactory;)V
// 105: aload 8
// 107: athrow
//
// Exception table:
// from to target type
// 27 70 81 javax/jdo/JDOException
// 27 70 90 java/lang/RuntimeException
// 27 70 95 finally
// 81 97 95 finally }
protected PersistenceManager createPersistenceManagerProxy(PersistenceManager pm) { Class[] ifcs = ClassUtils.getAllInterfaces(pm);
return ((PersistenceManager)Proxy.newProxyInstance(getClass().getClassLoader(), ifcs, new CloseSuppressingInvocationHandler(this, pm)));
}
protected Object postProcessResult(Object result, PersistenceManager pm, boolean existingTransaction)
{
return result;
}
public Object getObjectById(Object objectId)
throws DataAccessException
{
return execute(new JdoCallback(this, objectId) { private final Object val$objectId;
private final JdoTemplate this$0;
public Object doInJdo() throws JDOException { return pm.getObjectById(this.val$objectId, true);
}
}
, true);
}
public Object getObjectById(Class entityClass, Object idValue)
throws DataAccessException
{
return execute(new JdoCallback(this, entityClass, idValue) { private final Class val$entityClass;
private final Object val$idValue;
private final JdoTemplate this$0;
public Object doInJdo() throws JDOException { if (JdoTemplate.access$000() != null) {
Object id = ReflectionUtils.invokeMethod(JdoTemplate.access$000(), pm, new Object[] { this.val$entityClass, this.val$idValue.toString() });
return pm.getObjectById(id, true);
}
return pm.getObjectById(this.val$entityClass, this.val$idValue);
}
}
, true);
}
public void evict(Object entity)
throws DataAccessException
{
execute(new JdoCallback(this, entity) { private final Object val$entity;
private final JdoTemplate this$0;
public Object doInJdo() throws JDOException { pm.evict(this.val$entity);
return null;
}
}
, true);
}
public void evictAll(Collection entities)
throws DataAccessException
{
execute(new JdoCallback(this, entities) { private final Collection val$entities;
private final JdoTemplate this$0;
public Object doInJdo() throws JDOException { pm.evictAll(this.val$entities);
return null;
}
}
, true);
}
public void evictAll()
throws DataAccessException
{
execute(new JdoCallback(this) { private final JdoTemplate this$0;
pu
没有合适的资源?快使用搜索试试~ 我知道了~
spring-orm.src.zip net
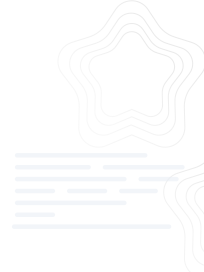
共74个文件
java:73个
mf:1个

需积分: 3 29 下载量 8 浏览量
2009-04-15
11:12:11
上传
评论
收藏 65KB ZIP 举报
温馨提示
spring-orm.src.zipspring-orm.src.zipspring-orm.src.zipspring-orm.src.zip .net 架构
资源推荐
资源详情
资源评论
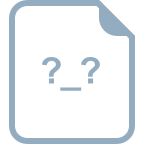
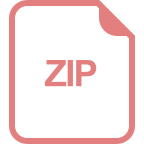
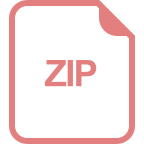
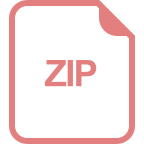
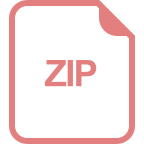
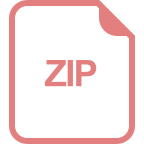
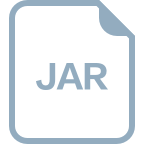
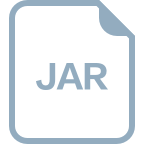
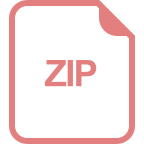
收起资源包目录


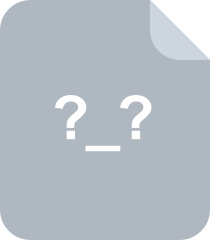




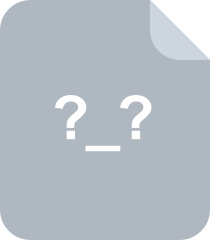
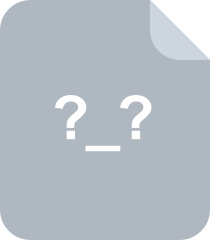
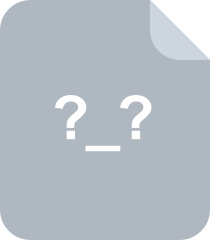
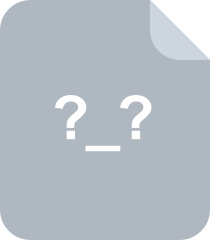
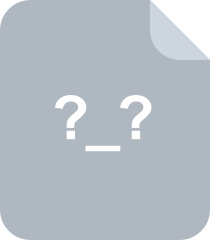
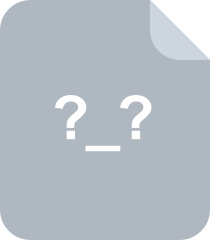
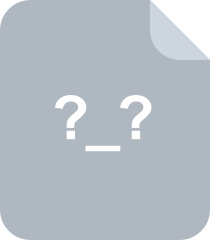

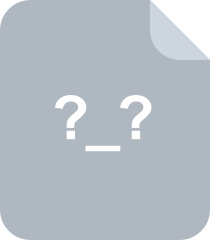
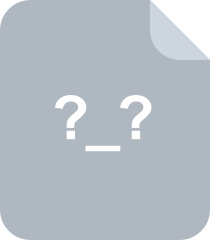
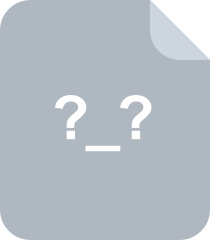
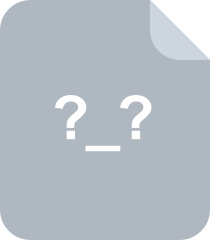
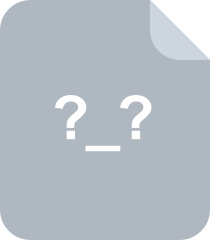
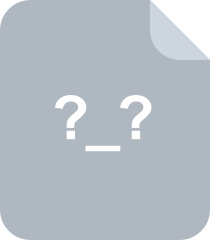
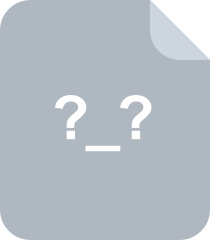
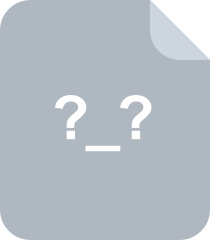
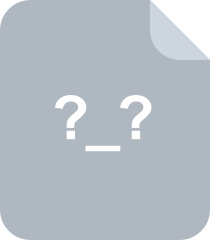
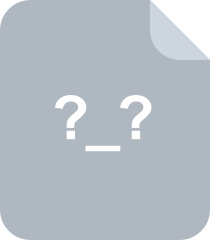
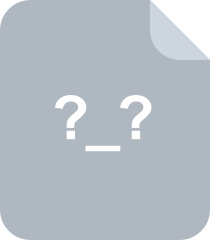
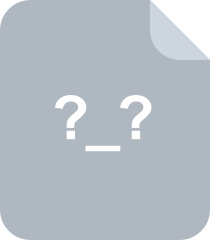
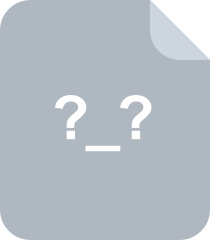

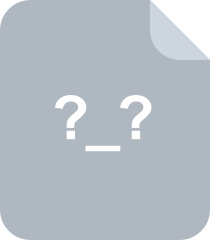
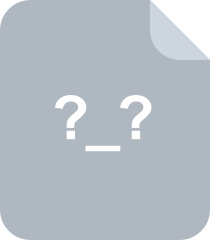
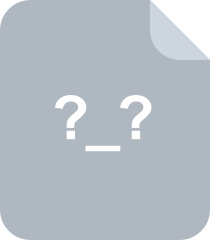
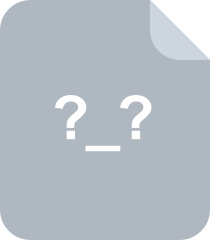
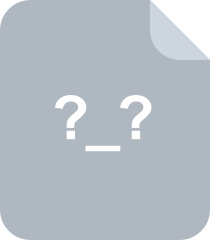
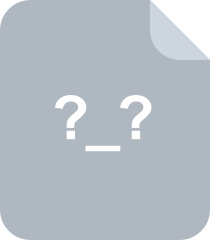
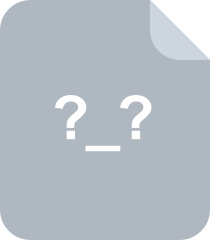
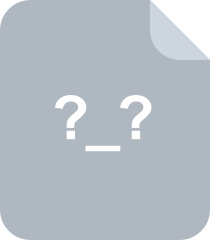
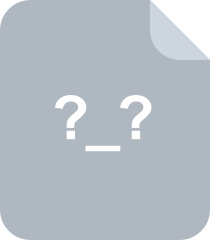

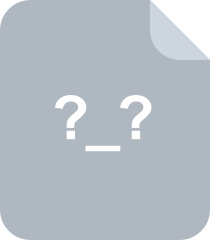
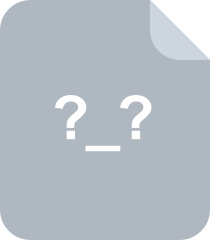
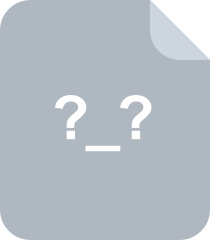
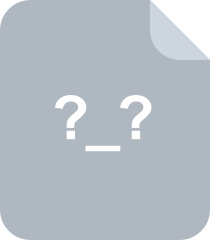
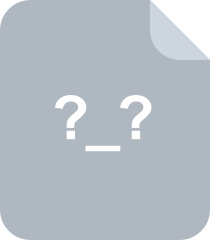
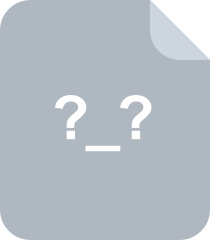
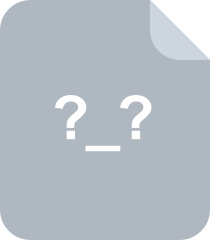
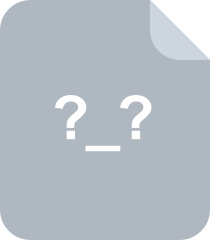
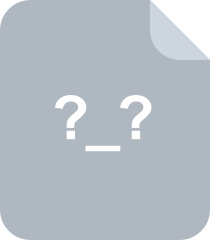
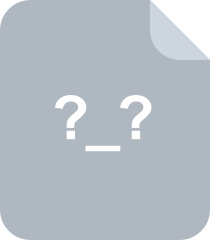
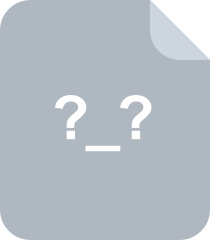
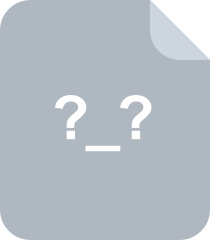
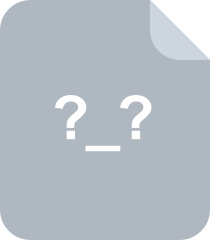
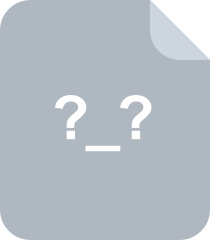
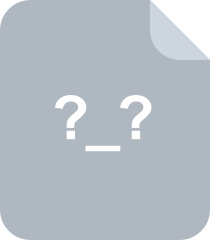
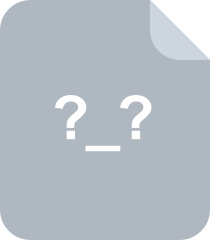
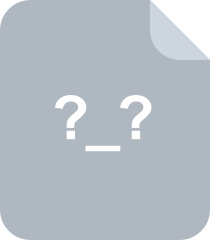

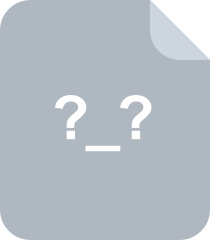
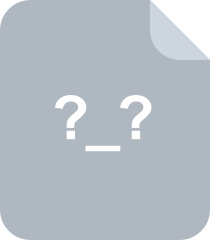
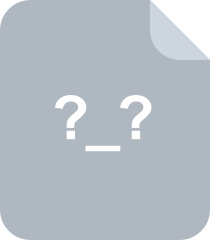
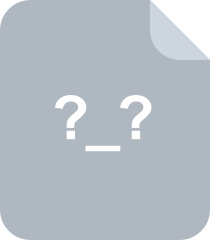

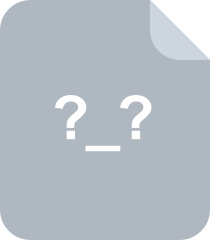
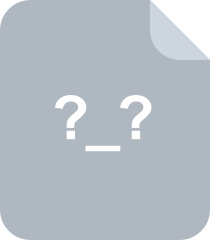
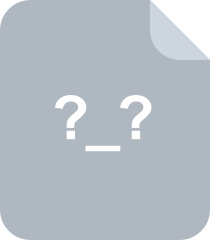
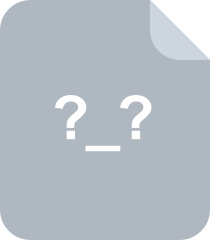
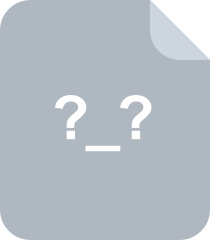
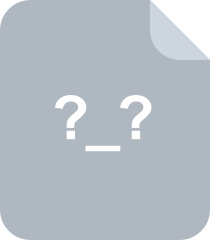
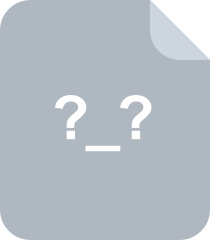
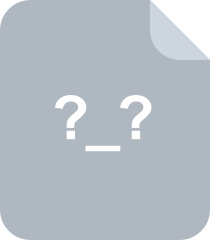
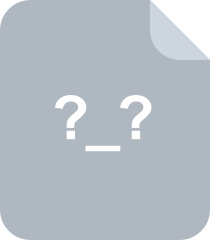
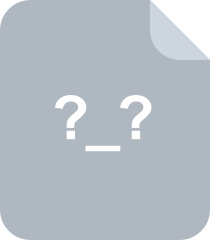

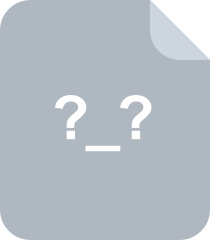
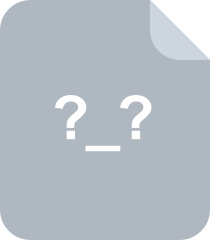
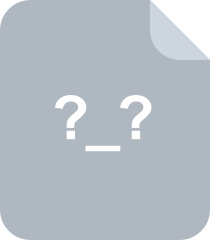

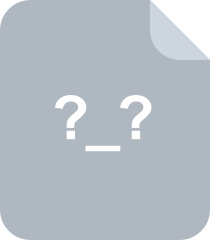
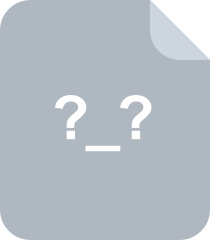
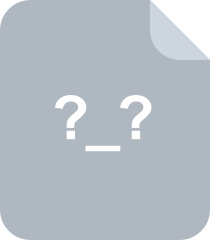
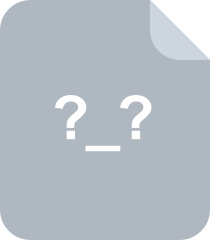
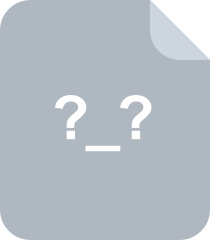
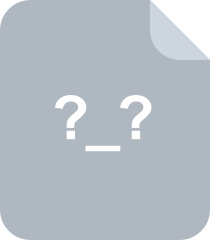
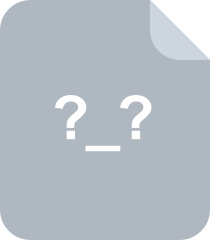
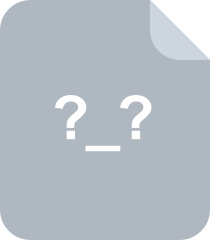
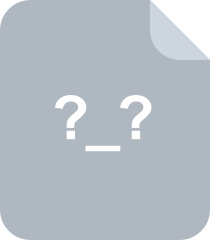
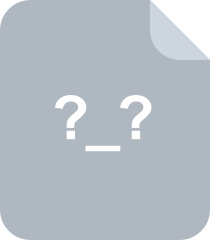
共 74 条
- 1
资源评论
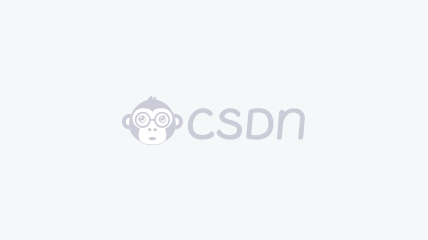

hu20090330
- 粉丝: 0
- 资源: 29
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

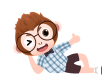
安全验证
文档复制为VIP权益,开通VIP直接复制
