#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <string.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/mman.h>
#include <linux/input.h>
#include <dirent.h>
#define PIC_PATH "./dirname"
#define PICTURE "./picture"
#define LCD_PATH "/dev/fb0"
#define TS_PATH "/dev/input/event0"
#define Slide_STATIC 0
#define Slide_UP 1
#define Slide_DOWN 2
#define Slide_LEFT 3
#define Slide_RIGHT 4
typedef struct node
{
char picpath[64];
struct node *next;
struct node *prev;
} D_NODE, *pD_node;
int lcd_fd;
int *FB;
void Lcd_Init(void);
void Lcd_Uninit(void);
void Show_Bmp(int win, int high, int x_s, int y_s, char *picname);
int Search_pic(char *dirname, pD_node pic_list);
int getxy(int *x, int *y);
int Get_Slide(int ts_fd);
int ts_open(void);
int ts_close(int fd);
char *auto_pic[10] ={
"./dirname/1.bmp",
"./dirname/2.bmp",
"./dirname/3.bmp",
"./dirname/4.bmp",
"./dirname/5.bmp",
"./dirname/6.bmp",
"./dirname/7.bmp",
"./dirname/8.bmp",
"./dirname/9.bmp",
"./dirname/10.bmp"
};
pD_node d_list_Create();
bool d_list_Insert_Head(pD_node Head, char *pic_name);
int d_list_Destroy(pD_node Head);
void D_Loop_List_Display(pD_node Head);
bool D_Loop_List_Insert_End(pD_node head, char *pic_name);
bool D_Loop_List_Remove(pD_node Node);
pD_node d_list_Create()
{
//0.申请头节点空??
pD_node Head = (pD_node)malloc(sizeof(D_NODE));
if (Head == NULL)
{
perror("malloc failed!");
return NULL;
}
//1.头节点赋
Head->next = Head;
Head->prev = Head;
//2.返回头节
return Head;
}
//1.头插
bool d_list_Insert_Head(pD_node Head, char *pic_name)
{
//0.新节点申请空??
pD_node Newnode = (pD_node)malloc(sizeof(D_NODE));
if (Newnode == NULL)
{
perror("malloc failed!");
return false;
}
//1.新节点赋
strcpy(Newnode->picpath, pic_name);
Newnode->prev = Newnode;
Newnode->next = Newnode;
//2.头插法插入链
Newnode->next = Head->next;
Head->next = Newnode;
Newnode->next->prev = Newnode;
Newnode->prev = Head;
return true;
}
//尾插
bool D_Loop_List_Insert_End(pD_node head, char *pic_name)
{
//1,新节点申请堆空间
pD_node newnode = (pD_node)malloc(sizeof(D_NODE));
if (NULL == newnode)
{
perror("malloc newnode failed");
return false;
}
//2,对新节点进行赋
strcpy(newnode->picpath, pic_name); //把传入的字符串拷贝到新的节点
newnode->prev = newnode;
newnode->next = newnode;
//尾插法插入节
//1)处理前继指针
newnode->prev = head->prev;
head->prev = newnode;
//2)处理后继指针
newnode->prev->next = newnode;
newnode->next = head;
return true;
}
//3.显示
void D_Loop_List_Display(pD_node Head)
{
pD_node p = Head->next;
while (p != Head)
{
printf("%s\n", p->picpath);
p = p->next;
}
}
int d_list_Destroy(pD_node Head)
{
pD_node p = Head;
pD_node q = p->next;
int i = 0;
while (q != Head)
{
p = q;
free(p);
i++;
q = q->next;
}
free(Head);
return i;
}
int ts_open(void)
{
int fd = open(TS_PATH, O_RDWR);
if (-1 == fd)
{
perror("open ts failed");
return -1;
}
return fd;
}
int ts_close(int fd)
{
close(fd);
}
int Get_Slide(int ts_fd)
{
int x1, x2, y1, y2;
struct input_event xy;
//1,获取滑动过程中起点坐标和终点坐??
for (;;) //获取起点坐标
{
read(ts_fd, &xy, sizeof(xy));
if (xy.type == EV_ABS && xy.code == ABS_X)
{
x1 = xy.value;
}
if (xy.type == EV_ABS && xy.code == ABS_Y)
{
y1 = xy.value;
}
if (xy.type == EV_KEY && xy.code == BTN_TOUCH && xy.value == 1)
{
printf("起点坐标(%d,%d)\n", x1, y1);
break;
}
}
x2 = x1;
y2 = y1;
for (;;) //获取终点坐标
{
read(ts_fd, &xy, sizeof(xy));
if (xy.type == EV_ABS && xy.code == ABS_X)
{
x2 = xy.value;
}
if (xy.type == EV_ABS && xy.code == ABS_Y)
{
y2 = xy.value;
}
if (xy.type == EV_KEY && xy.code == BTN_TOUCH && xy.value == 0)
{
printf("终点坐标(%d,%d)\n", x2, y2);
break;
}
}
//2,判断滑动方块
/*右滑*/
if (x2 > x1 && (x2 - x1) * (x2 - x1) > (y2 - y1) * (y2 - y1))
{
printf("右滑\n");
return Slide_RIGHT;
}
if (x2 < x1 && (x2 - x1) * (x2 - x1) > (y2 - y1) * (y2 - y1))
{
printf("左滑\n");
return Slide_LEFT;
}
if (y2 > y1 && (x2 - x1) * (x2 - x1) < (y2 - y1) * (y2 - y1))
{
printf("下滑\n");
return Slide_DOWN;
}
if (y2 < y1 && (x2 - x1) * (x2 - x1) < (y2 - y1) * (y2 - y1))
{
printf("上滑\n");
return Slide_UP;
}
if (x1 == x2 && y1 == y2)
{
printf("未滑动\n");
return Slide_STATIC;
}
}
int getxy(int *x, int *y)
{
//1.打开触摸屏文件
int fd = open(TS_PATH, O_RDWR);
if (fd == -1)
{
perror("open failed!");
return -1;
}
//2.读取触摸屏文件数据
struct input_event xy;
int flag = 0; //记录当前获取坐标的信标
while (1)
{
read(fd, &xy, sizeof(xy));
if (xy.type == EV_ABS && xy.code == ABS_X)
{
*x = xy.value * 800 / 1024;
flag = 1;
}
if (xy.type == EV_ABS && xy.code == ABS_Y)
{
*y = xy.value * 480 / 600;
flag = 2;
}
if (flag == 2)
{
printf("(%d,%d)\n", *x, *y);
flag = 0;
break;
}
}
//3.关闭触摸屏文件
close(fd);
return 0;
}
int Search_pic(char *dirname, pD_node pic_list)
{
//0.打开目录
DIR *dp = opendir(dirname);
if (NULL == dp)
{
perror("opendir failed!");
return -1;
}
//1.循环读取目录
struct dirent *ep;
int picnum = 0;
char picname[64];
while (1)
{
memset(picname, 0, sizeof(picname));
ep = readdir(dp);
if (ep == NULL)
break;
if (strcmp(".", ep->d_name) == 0 || strcmp("..", ep->d_name) == 0)
continue;
//检索目录下??.bmp文件
if (strcmp(".bmp", ep->d_name + strlen(ep->d_name) - 4) == 0)
{
sprintf(picname, "%s/%s", dirname, ep->d_name);
D_Loop_List_Insert_End(pic_list, picname); //尾插把指定目录下的图片插入到链表
}
picnum++;
}
//2.关闭目录
closedir(dp);
return picnum;
}
void Lcd_Init(void)
{
//1,打开LCD文件
lcd_fd = open(LCD_PATH, O_RDWR);
if (-1 == lcd_fd)
{
perror("open lcd failed");
return;
}
//2,lcd映射到用户空间
FB = mmap(NULL, 800 * 480 * 4, PROT_READ | PROT_WRITE, MAP_SHARED, lcd_fd, 0);
if (MAP_FAILED == FB)
{
perror("mmap lcd failed");
return;
}
}
//2,LCD释放函数
void Lcd_Uninit(void)
{
//4,解除映射,关闭文件
munmap(FB, 800 * 480 * 4);
close(lcd_fd);
}
//3,显示宽度为win,高度为high的bmp图片 在起点坐标为(x_s, y_s)这个点开始显示图??
void Show_Bmp(int win, int high, int x_s, int y_s, char *picname)
{
int i, j;
int tmp;
char buf[win * high * 3]; //存放图片的原始数??
int bmp_buf[win * high]; //存放转码之后的ARGB数据
//1,lcd初始??
Lcd_Init();
//2,读取图片数据,并且进行转换 RGB -> ARGB
//打开图片
FILE *fp = fopen(picname, "r");
if (NULL == fp)
{
perror("fopen failed");
return;
}
//读取图片像素点原始数据
fseek(fp, 54, SEEK_SET);
fread(buf, 3, win * high, fp);
//将读取的数据进行转码
for (i = 0; i < win * high; i++)
{
//ARGB R G B
bmp_buf[i] = buf[3 * i + 2] << 16 | buf[3 * i + 1] << 8 | buf[3 * i];
}
//将转码的数据进行倒转 把第i行,第j列的点跟??479-i行,第j列的点进行交??
for (i = 0; i < high / 2; i++) //0~239??
{
for (j = 0; j < win; j++) //0~799??
{
//
没有合适的资源?快使用搜索试试~ 我知道了~
关于21物联一linux“gec6818”如何替换图片(附带资源)
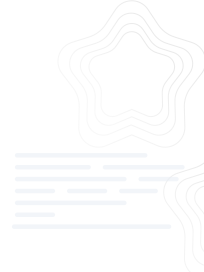
共39个文件
bmp:29个
rar:4个
url:1个

需积分: 0 0 下载量 201 浏览量
2023-11-11
02:29:20
上传
评论
收藏 36.09MB ZIP 举报
温馨提示
代码和素材,驱动最好用教室里的版本,我这个版本可能有一些问题。
资源推荐
资源详情
资源评论
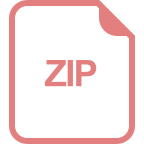
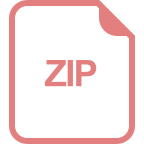
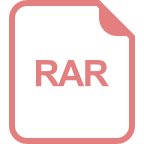
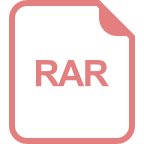
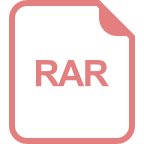
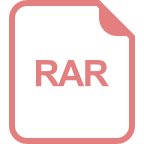
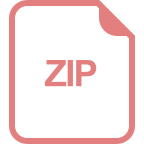
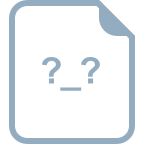
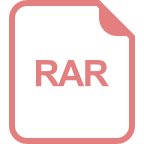
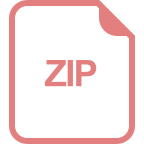
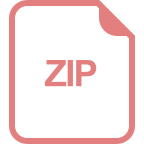
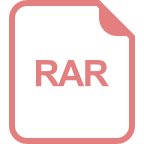
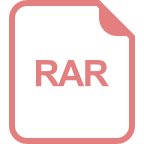
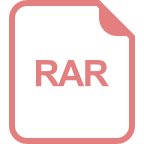
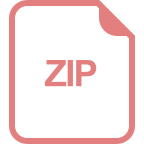
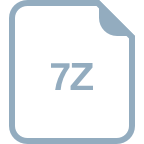
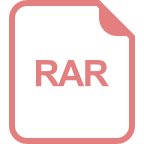
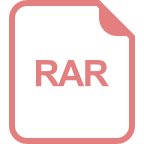
收起资源包目录




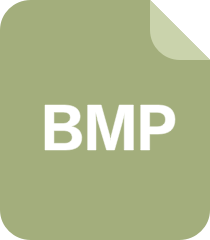
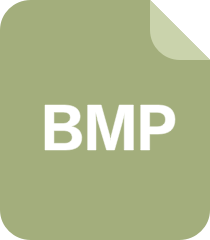
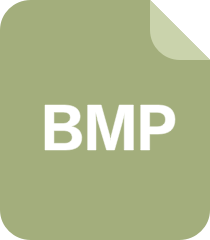
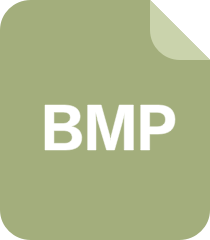
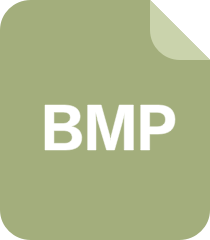
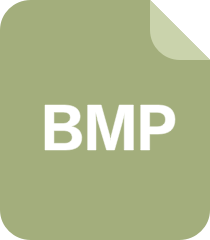
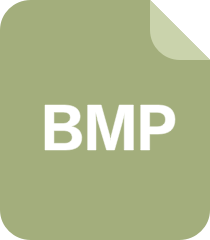

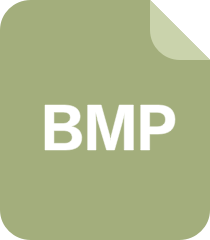
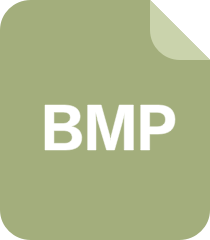
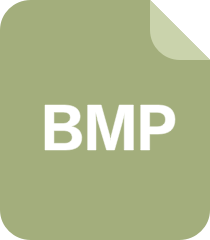
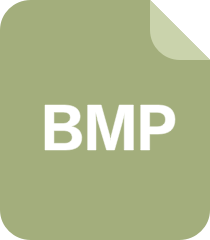
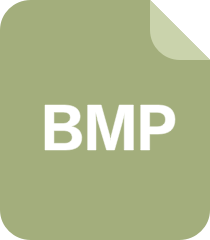
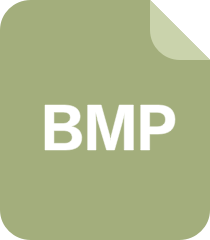
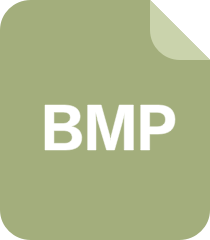
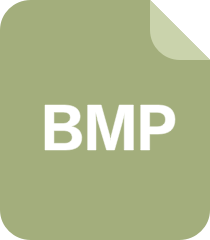
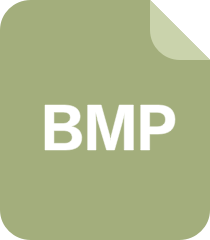
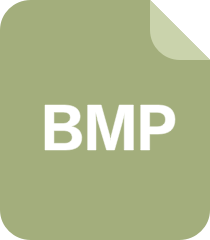

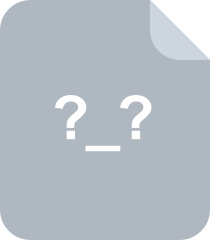
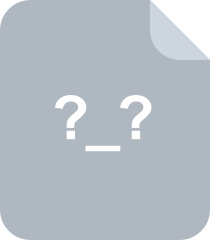
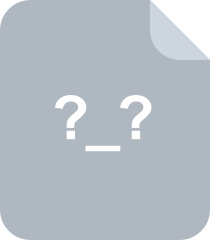

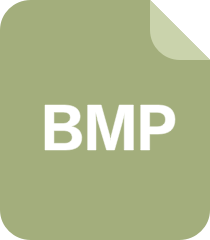
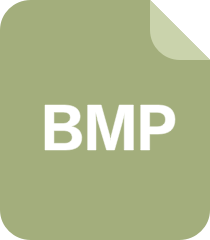
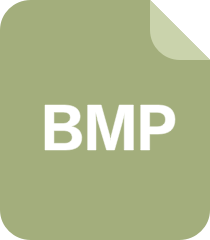
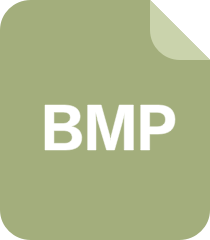
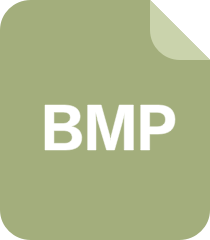
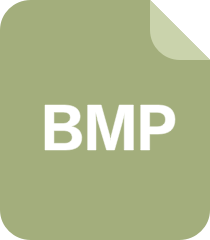
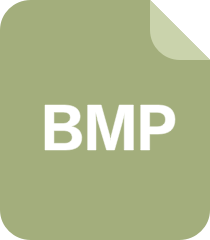
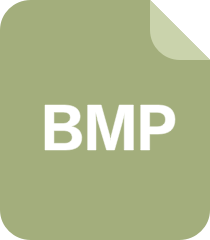
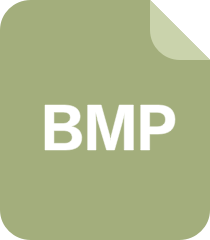
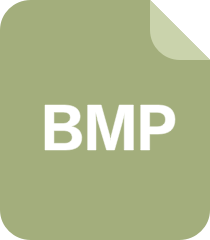


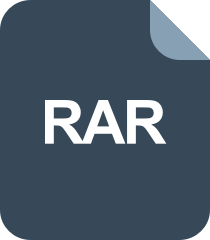
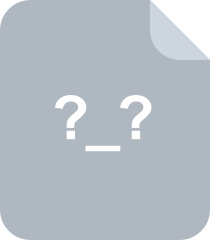
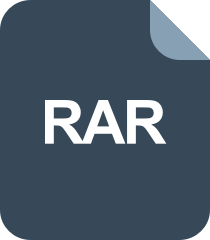
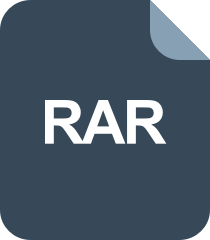
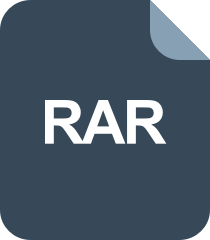
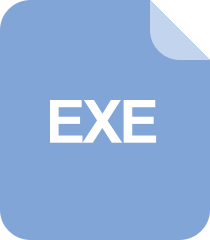

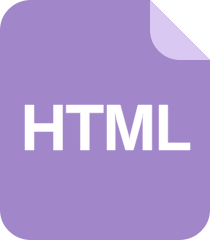

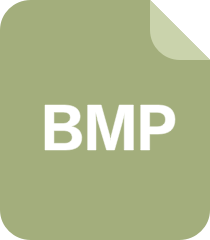
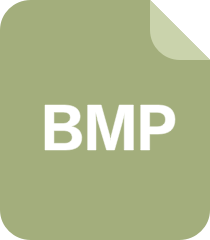
共 39 条
- 1
资源评论
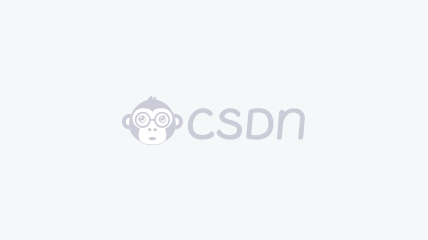

hu1302062194
- 粉丝: 9
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

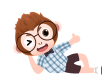
安全验证
文档复制为VIP权益,开通VIP直接复制
