package cn.xpool.ats.sui;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.FocusEvent;
import java.awt.event.FocusListener;
import java.util.HashSet;
import java.util.Set;
import javax.swing.JButton;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JSplitPane;
import javax.swing.JTabbedPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableModel;
import cn.xpool.ats.item.AirplaneModel;
import cn.xpool.ats.item.Airport;
import cn.xpool.ats.item.FlightScheduler;
import cn.xpool.ats.item.MyTable;
public class FlightSchedulerAssistantJPane extends JSplitPane {
private static final long serialVersionUID = 1L;
/**
* 航班计划辅助设置上方布局面板
*/
private JSplitPane upJSplitPane = null;
/**
* 机场信息面板
*/
private JTabbedPane airportInfoPane = null;
/**
* 机场信息内容面板
*/
private JPanel airportInfoContentPane = null;
/**
* 机场编码(三字码)文本框
*/
private JTextField airportNo = null;
/**
* 机场名文本框
*/
private JTextField airportName = null;
/**
* 机场所在城市名文本框
*/
private JTextField airportCity = null;
/**
* 机场所在省份文本框
*/
private JTextField airportProvince = null;
/**
* 机场信息列表面板
*/
private JScrollPane airportInfoScrollPane = null;
/**
* 机场信息列表表格
*/
private JTable airportInfoTable = null;
/**
* 航班计划辅助设置面板下方布局面板
*/
private JSplitPane downJSplitPane = null;
/**
* 飞机型号信息面板
*/
private JTabbedPane airplaneModelPane = null;
/**
* 飞机型号信息内容面板
*/
private JPanel airplaneModelContentPane = null;
/**
* 飞机信息列表面板
*/
private JScrollPane airplaneModelScrollPane = null;
/**
* 飞机信息列表表格
*/
private JTable airplaneModelTable = null;
/**
* 飞机型号文本框
*/
private JTextField airplaneModel = null;
/**
* 制造商文本框
*/
private JTextField manufacturer = null;
/**
* 最大航程文本框
*/
private JTextField maxSail = null;
/**
* 头等舱座位数文本框
*/
private JTextField fSits = null;
/**
* 公务舱座位数文本框
*/
private JTextField cSits = null;
/**
* 经济舱座位数文本框
*/
private JTextField ySits = null;
/**
* 构造航班计划辅助设置面板
*/
public FlightSchedulerAssistantJPane() {
initialize();
}
/**
* 初始化航班计划辅助设置面板
*/
private void initialize() {
this.setSize(760, 515);
this.setName("辅助设置");
this.setOrientation(JSplitPane.VERTICAL_SPLIT);
upJSplitPane = new JSplitPane();
upJSplitPane.setOrientation(JSplitPane.VERTICAL_SPLIT);
airportInfoTable = new MyTable();
airportInfoTable.setBackground(new Color(151, 220, 233));
airportInfoScrollPane = new JScrollPane(airportInfoTable);
airportInfoTable.setPreferredScrollableViewportSize(new Dimension(760,
70));
this.upRefreshTable(MainClass.fatiDao.getObj());
airportInfoContentPane = new JPanel();
airportInfoContentPane.setName("机场信息增删查改");
airportInfoContentPane.setLayout(new GridLayout(2, 1));
airportNo = new JTextField(8);
airportName = new JTextField(8);
airportCity = new JTextField(8);
airportProvince = new JTextField(8);
JPanel textFieldPane = new JPanel();
textFieldPane.add(new JLabel("三字码:"));
textFieldPane.add(airportNo);
textFieldPane.add(new JLabel("机场名:"));
textFieldPane.add(airportName);
textFieldPane.add(new JLabel("城市:"));
textFieldPane.add(airportCity);
textFieldPane.add(new JLabel("省份:"));
textFieldPane.add(airportProvince);
JPanel upButtonPane = new JPanel();
upButtonPane.setLayout(new FlowLayout(FlowLayout.CENTER, 50, 5));
JButton upAddButton = new JButton("增加");
upAddButton.addActionListener(new ActionListener() {
@SuppressWarnings("unchecked")
public void actionPerformed(ActionEvent e) {
if (airportNo.getText().equals("")
|| airportName.getText().equals("")
|| airportCity.getText().equals("")
|| airportProvince.getText().equals("")) {
JOptionPane.showMessageDialog(null, "机场信息填写不完整,请返回补充!",
"添加操作提示", JOptionPane.ERROR_MESSAGE);
return;
}
if (!isAirportNo(airportNo.getText())) {
JOptionPane.showMessageDialog(null,
"机场三字码不合格!\n\n机场三字码只能是三个大写英文字母。请返回修改!", "添加操作提示",
JOptionPane.ERROR_MESSAGE);
upClearTextField();
return;
}
Set<Airport> airportSet = (Set<Airport>) MainClass.fatiDao
.getObj();
for (Airport airport : airportSet) {
if (airport.getAirportNo().equals(airportNo.getText())) {
JOptionPane.showMessageDialog(null,
"添加机场失败,因为已经存在相同的机场!", "添加操作提示",
JOptionPane.ERROR_MESSAGE);
upClearTextField();
return;
}
}
if (MainClass.fatiDao.addAirport(new Airport(airportNo
.getText(), airportName.getText(), airportCity
.getText(), airportProvince.getText()))) {
JOptionPane.showMessageDialog(null, "成功添加了一个机场!", "添加操作提示",
JOptionPane.INFORMATION_MESSAGE);
upRefreshTable(MainClass.fatiDao.getObj());
String name = airportName.getText().trim();
String city = airportCity.getText().trim();
String province = airportProvince.getText().trim();
String airportListName = name.equals("") ? (city.equals("") ? province
: city)
: name;
MainFrame.fromCityList.addItem(airportListName);
MainFrame.toCityList.addItem(airportListName);
upClearTextField();
return;
}
JOptionPane.showMessageDialog(null, "添加机场失败!", "添加操作提示",
JOptionPane.ERROR_MESSAGE);
upClearTextField();
}
});
JButton upDelButton = new JButton("删除");
upDelButton.addActionListener(new ActionListener() {
@SuppressWarnings("unchecked")
public void actionPerformed(ActionEvent e) {
if (airportNo.getText().equals("")) {
JOptionPane.showMessageDialog(null,
"请先用机场三字码指定一个机场后再做删除操作!", "删除操作提示",
JOptionPane.ERROR_MESSAGE);
return;
}
String no = airportNo.getText();
if (!isAirportNo(no)) {
JOptionPane.showMessageDialog(null, "三字码不合格,请返回修改!",
"删除操作提示", JOptionPane.ERROR_MESSAGE);
return;
}
int selected = JOptionPane.showConfirmDialog(null, "确认要删除三字码为 "
+ no + " 的机场?", "删除操作提示", JOptionPane.YES_NO_OPTION,
JOptionPane.QUESTION_MESSAGE);
if (selected == JOptionPane.YES_OPTION) {
Set<Airport> tempSet = (Set<Airport>) MainClass.fatiDao
.queryAirport(no, "", "", "");
String name = null;
String city = null;
String province = null;
for (Airport deletedAirport : tempSet) {
name = deletedAirport.getAirportName().trim();
city = deletedAirport.getAirportCity().trim();
province = deletedAirport.getAirportProvince().trim();
}
String airportListName = name.equals("") ? (city.equals("") ? province
: city)
: name;
Set<FlightScheduler> flightSchedulerSet = (HashSet<FlightScheduler>) MainClass.ffsiDa
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
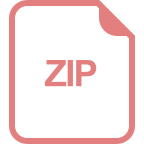
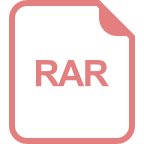
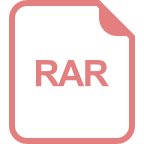
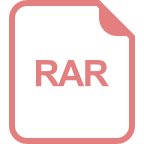
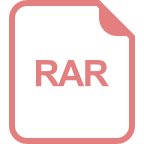
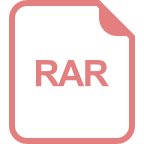
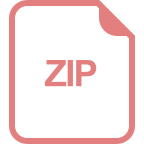
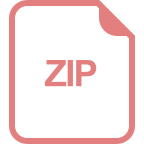
收起资源包目录

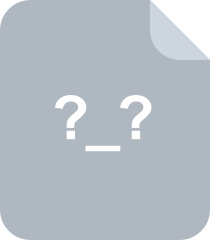
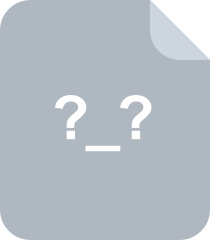
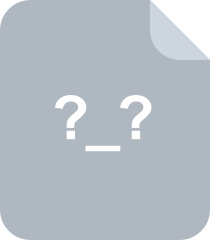
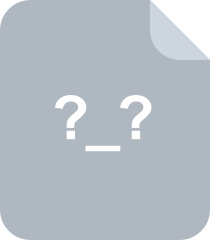
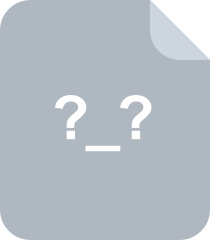
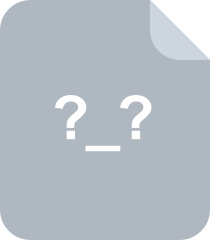
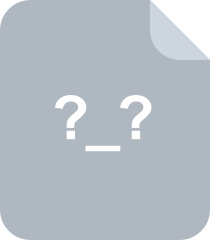
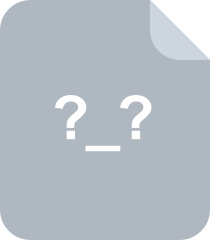
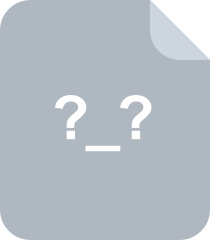
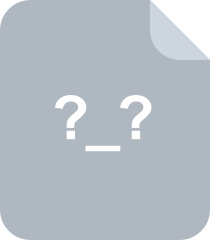
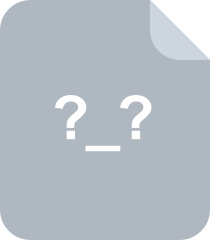
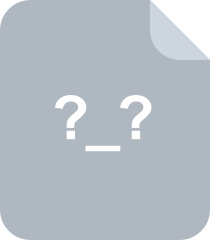
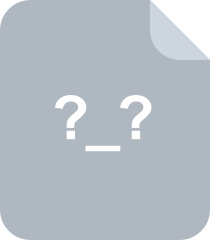
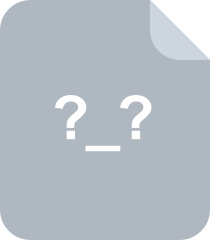
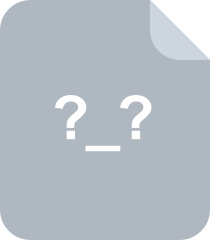
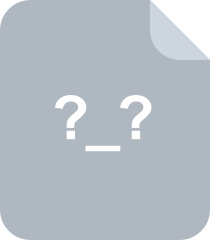
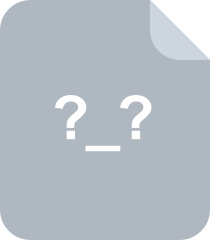
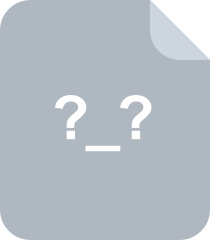
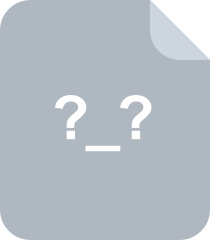
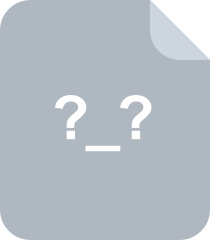
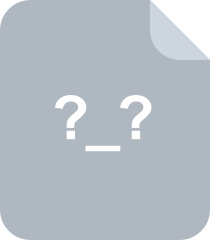
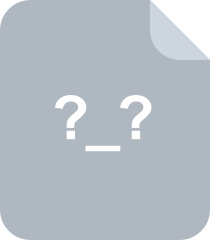
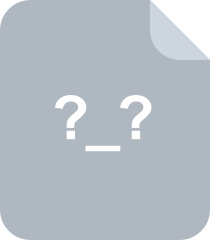
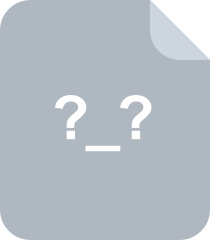
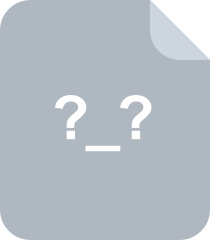
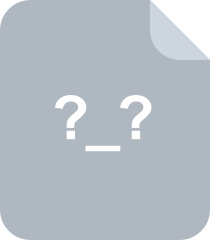
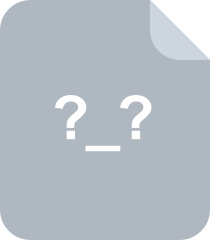
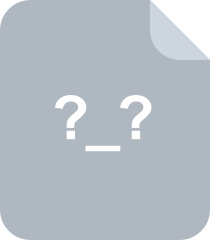
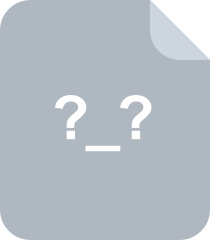
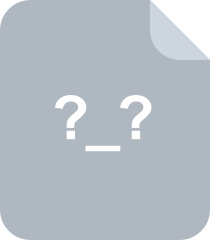
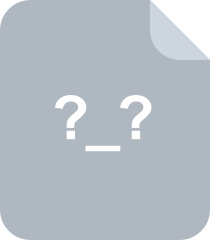
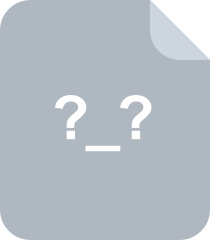
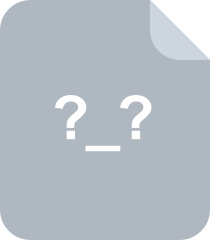
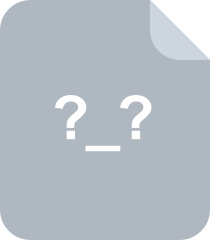
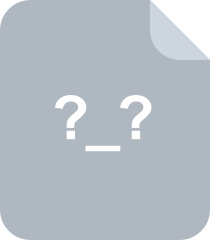
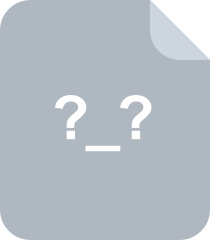
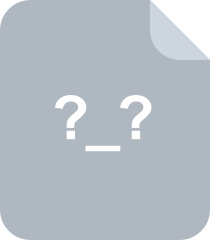
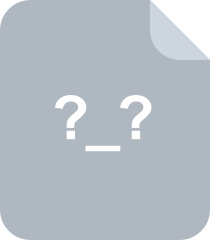
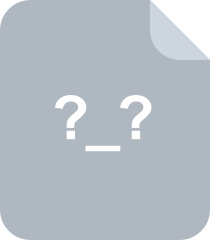
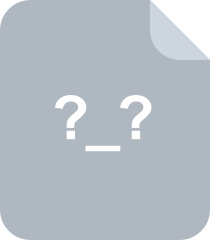
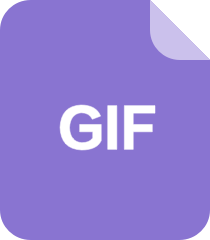
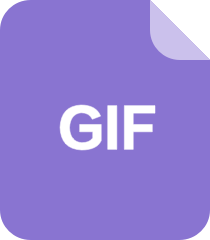
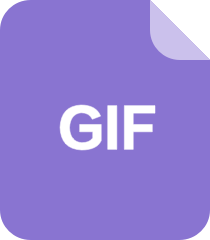
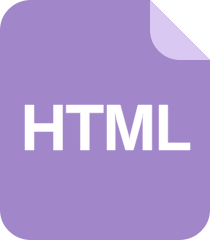
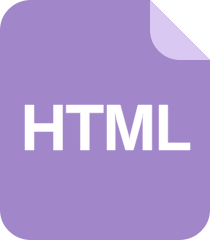
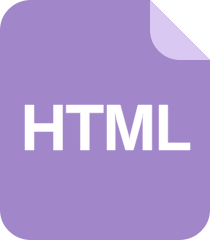
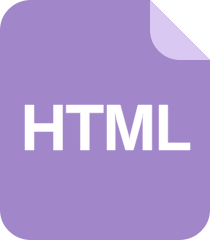
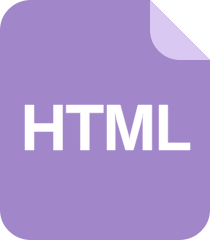
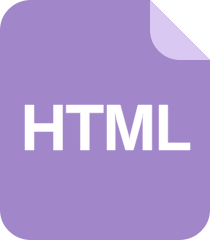
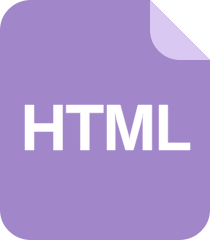
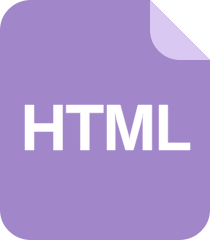
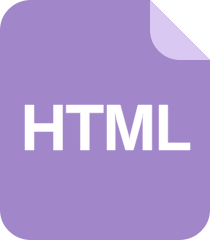
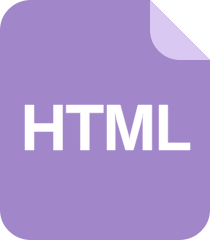
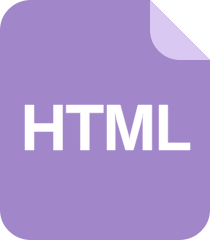
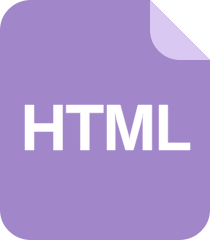
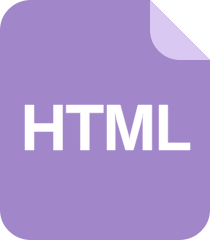
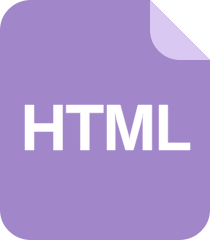
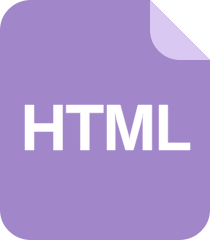
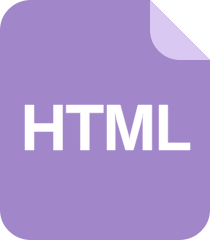
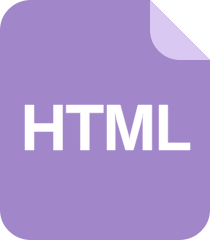
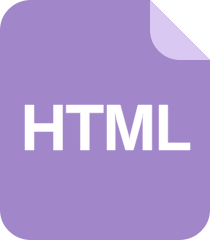
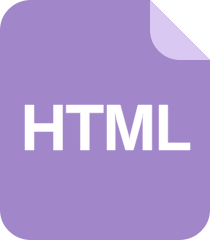
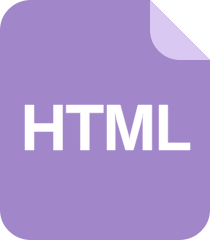
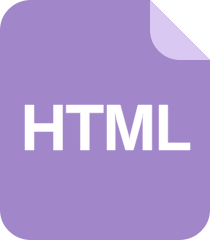
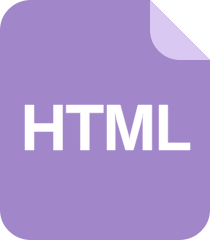
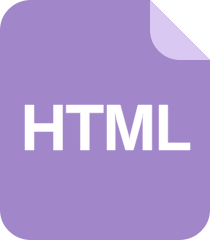
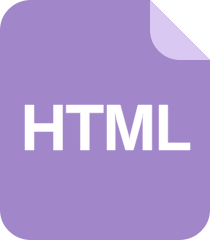
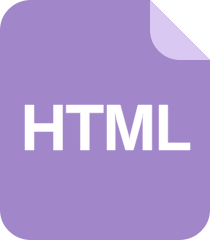
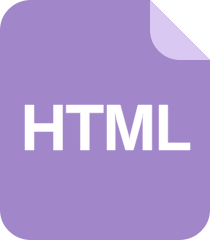
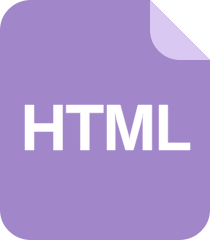
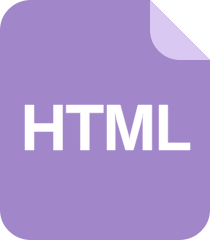
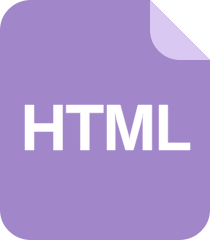
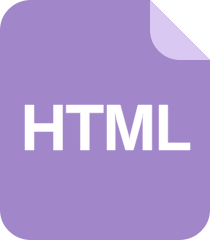
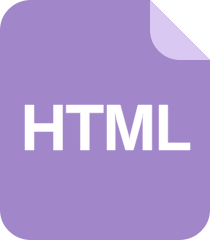
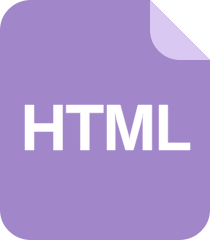
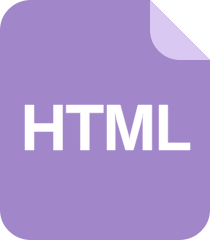
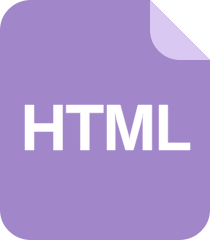
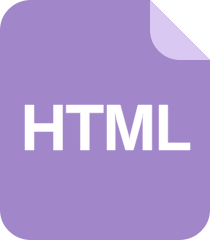
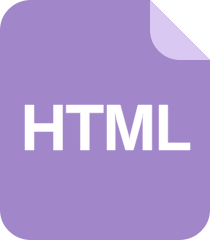
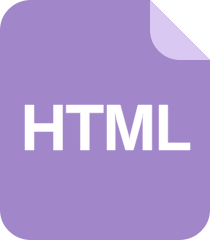
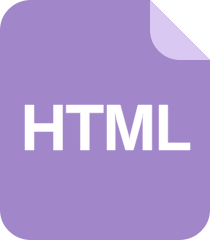
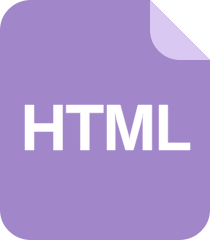
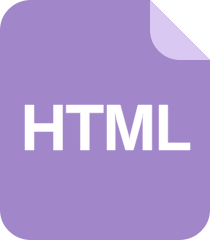
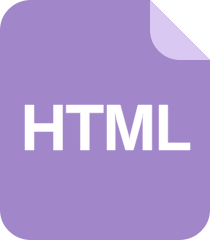
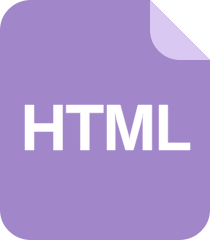
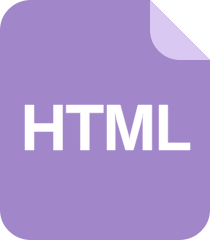
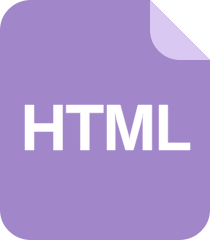
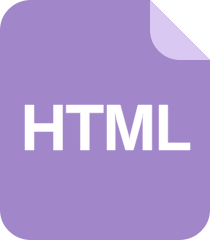
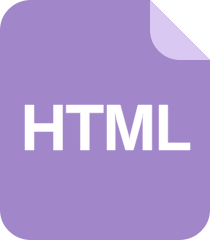
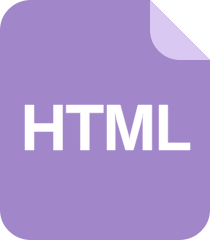
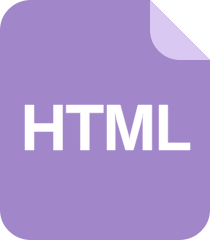
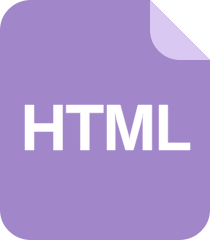
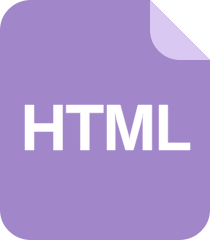
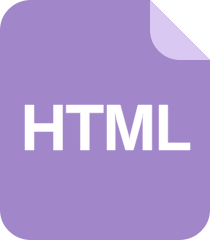
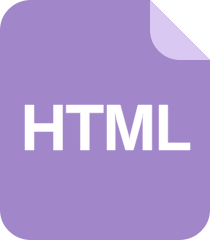
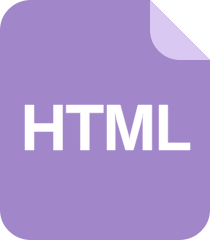
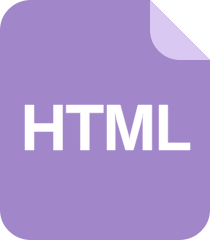
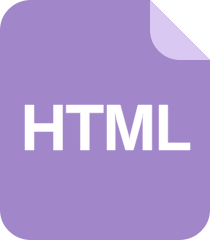
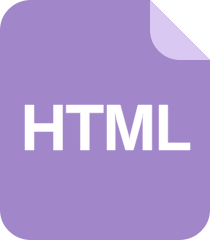
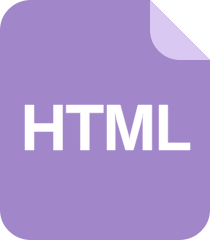
共 498 条
- 1
- 2
- 3
- 4
- 5

hsh678
- 粉丝: 3
- 资源: 31
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

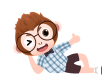
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


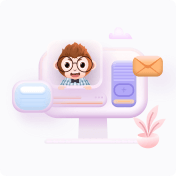
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页