/*
* Generated by MyEclipse Struts
* Template path: templates/java/JavaClass.vtl
*/
package com.xpai.web;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionForward;
import org.apache.struts.action.ActionMapping;
import org.apache.struts.upload.FormFile;
import com.xpai.biz.BidBiz;
import com.xpai.biz.GoodsBiz;
import com.xpai.entity.Bid;
import com.xpai.entity.Goods;
import com.xpai.entity.User;
import com.xpai.util.CosUtil;
import com.xpai.util.JbUtils;
import com.xpai.util.Page;
import com.xpai.util.SpecialFilter;
public class GoodsAction extends BaseAction {
/*
* Generated Methods
*/
private GoodsBiz goodsBiz = null;
private BidBiz bidBiz = null;
public ActionForward toAdd(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
User saler = (User) request.getSession(false).getAttribute("user");
if(saler==null){
return new ActionForward("/index.jsp");
}
return mapping.findForward("add_goods");
}
public ActionForward upload(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws Exception {
String fileName = CosUtil.upload(request);
System.out.println(fileName);
return null;
}
public ActionForward uploadImage(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws Exception {
// response.setContentType("text/html;charset=GBK");
// // 获取当前项目在Tomcat中的路径
// String path =
// request.getSession().getServletContext().getRealPath("images");
// GoodsForm pf = (GoodsForm) form;
// FormFile file = pf.getEditorImg();
// FileOutputStream fos = new FileOutputStream(path + "/" +
// file.getFileName());
// // 将文件写入指定路径
// fos.write(file.getFileData());
// fos.flush();
// fos.close();
// PrintWriter out = response.getWriter();
// out.println(file.getFileName());
// return null;
request.setCharacterEncoding("GBK");
GoodsForm goodsForm = (GoodsForm) form;
// Goods item = goodsForm.getItem();
FormFile imageFile = goodsForm.getEditorImg();
// 文件名:不包含路径
String filename = imageFile.getFileName();
response.setContentType("text/html;charset=GBK");
response.getWriter().println(filename);
// byte[] content=imageFile.getFileData(); 用这个byte[]就死了,outof
// memory;提供吸管
InputStream contentStream = imageFile.getInputStream();
// 得到保存目录
// savedir=this.getServlet().getServletContext().getRealPath("/");
String savedir = request.getSession().getServletContext().getRealPath(
"images");
// 构建file
File fileImage = new File(savedir, filename);
// 文件流:文件输出流
FileOutputStream fos = new FileOutputStream(fileImage);
// 输入流内容导入到输出流里面去
int len = 0;
byte[] buf = new byte[1024];
while ((len = contentStream.read(buf)) != -1) {// 读到了内容
fos.write(buf, 0, len);
}
// 关门:不关以后会out of memory,JVM会回收对象,但不会回收对象关联的系统资源
contentStream.close();
fos.close();
return null;
}
public ActionForward doAdd(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws IOException {
request.setCharacterEncoding("GBK");
User saler = (User) request.getSession(false).getAttribute("user");
if(saler==null){
return new ActionForward("/index.jsp");
}
GoodsForm goodsForm = (GoodsForm) form;
// Goods item = goodsForm.getItem();
FormFile imageFile = goodsForm.getEditorImg();
// 文件名:不包含路径
String filename = imageFile.getFileName();
response.setContentType("text/html;charset=GBK");
response.getWriter().println(filename);
if (!((imageFile.getFileSize() == 0) || filename.trim().equals(""))) {
// byte[] content=imageFile.getFileData(); 用这个byte[]就死了,outof
// memory;提供吸管
InputStream contentStream = imageFile.getInputStream();
//String savedir=this.getServlet().getServletContext().getRealPath("images");
String savedir = request.getSession().getServletContext().getRealPath("images");
// 构建file
File fileImage = new File(savedir, filename);
// 文件流:文件输出流
FileOutputStream fos = new FileOutputStream(fileImage);
// 输入流内容导入到输出流里面去
int len = 0;
byte[] buf = new byte[1024];
while ((len = contentStream.read(buf)) != -1) {// 读到了内容
fos.write(buf, 0, len);
}
// 关门:不关以后会out of memory,JVM会回收对象,但不会回收对象关联的系统资源
contentStream.close();
fos.close();
}
Goods item = goodsForm.getItem();
item.setGoodsPic("images/" + filename);
User user = getCurrUser(request);
this.goodsBiz.addGoods(item, user);
return mapping.findForward("index");
}
public ActionForward doBid(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws IOException, ServletException {
String goodsID = request.getParameter("goods_id");
Double myPrice = JbUtils.parseDouble(request.getParameter("myPrice"));
if (myPrice == -1d) {
request.getRequestDispatcher("/index.jsp").forward(request,
response);
}
Long goodId = Long.parseLong(goodsID);
Goods goods = goodsBiz.getGoods(goodId);
User buyer = (User) request.getSession(false).getAttribute("user");
boolean goodIsSold = (goods.getGoodsStatus() == GoodsBiz.GOODS_STATUS_OUT) ? true : false;
response.setCharacterEncoding("GBK");
PrintWriter out = response.getWriter();
if (goodIsSold) {
out.write("sold");
}
Double maxBidInHistory = goodsBiz.getMaxBidByGoods(goods);
Double price = goods.getGoodsPrice();
if (maxBidInHistory == null) {
if (myPrice <= price) {
out.write("less");
} else if (myPrice > price) {
bidBiz.addBid(goods, buyer, myPrice);
out.write("ok");
}
} else if (maxBidInHistory != null) {
if (myPrice <= maxBidInHistory) {
out.write("less");
} else if (myPrice > maxBidInHistory) {
bidBiz.addBid(goods, buyer, myPrice);
out.write("ok");
}
}
return null;
// return new ActionForward("/user.do?operate=doLogin");
}
public ActionForward doBidNormal(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws IOException, ServletException {
String goodsID = request.getParameter("goods_id");
Double myPrice = JbUtils.parseDouble(request.getParameter("myPrice"));
if (myPrice == -1d) {
request.setAttribute("msg","Bid Price Invalid!You can't buy it or bid for it..");
request.getRequestDispatcher("msg.jsp").forward(request,response);
}
Long goodId = Long.parseLong(goodsID);
Goods goods = goodsBiz.getGoods(goodId);
User buyer = (User) request.getSession(false).getAttribute("user");
boolean goodIsSold = (goods.getGoodsStatus() == GoodsBiz.GOODS_STATUS_OUT) ? true : false;
if (goodIsSold) {
request.setAttribute("msg","Goods sold out! You can't buy it or bid for it..");
}
Double maxBidInHistory = goodsBiz.getMaxBidByGoods(goods);
Double price = goods.getGoodsPrice();
if (maxBidInHistory == null) {
if (myPrice <= price) {
request.setAttribute("msg","You bid too low.You can't buy it or bid for it..");
} else if (myPrice > price) {
bidBiz.addBid(goods, buyer, myPrice);
request.setAttribute("msg","竞拍成功");
}
} else if (maxBidInHistory != null) {
if (myPrice <= maxBidInHistory) {
request.setAttribute("msg","You bid too low.You can't buy
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
ACCP Y2毕业设计项目。采用SSH框架。最终版。 使用MYECLIPSE5.5+SQL SERVER2005. 本系统是一个C2C[客户对客户]在线交易平台, 功能: 注册,商品分页列表,成交等等。 *表格验证姓名是否存在,是否非空,是否符合格式; 增加功能: *首页样式重新设计,模仿163。 *上传图片控件。 *项目增加AJAX搜索智能提示,AJAX分页, *表格排序,存储过程分页。 *使用Javascript库实现脚本和HTML的分离。 PS:因CSDN限制,不能上传大附件,所以去掉了SSH的JAR包,请自行添加。
资源推荐
资源详情
资源评论
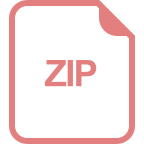
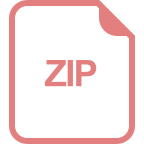
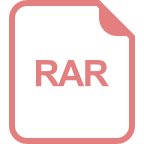
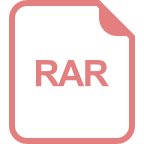
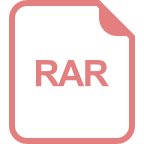
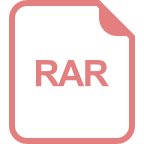
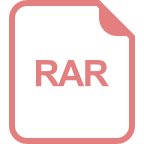
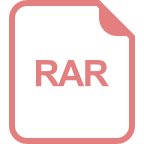
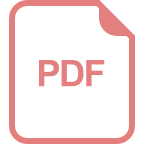
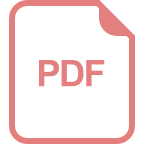
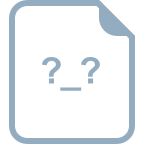
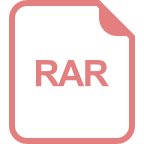
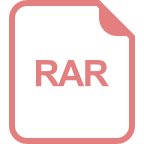
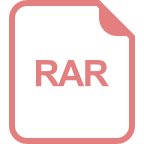
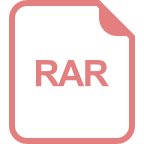
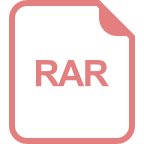
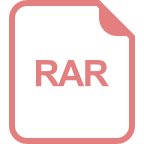
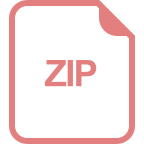
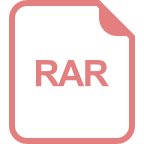
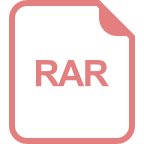
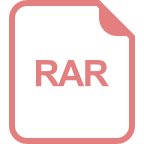
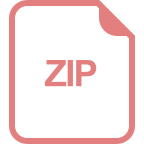
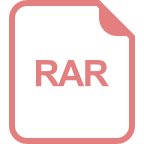
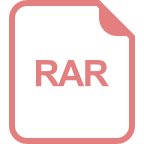
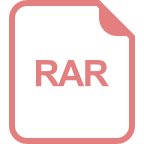
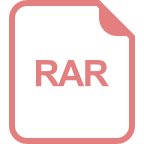
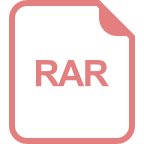
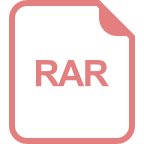
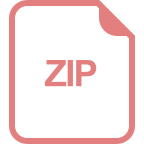
收起资源包目录

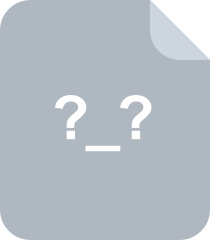
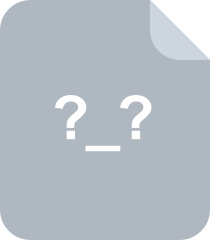
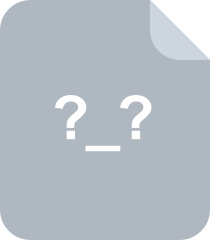
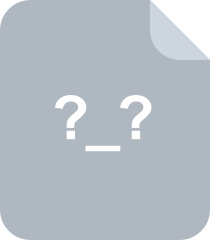
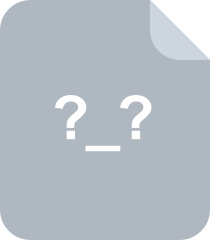
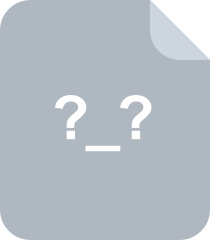
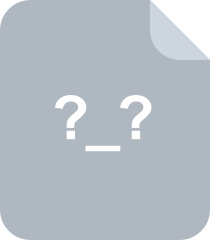
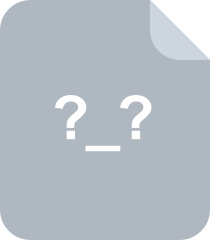
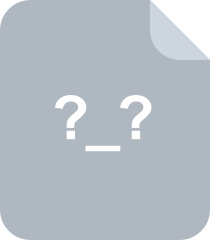
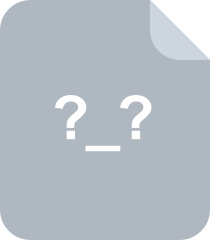
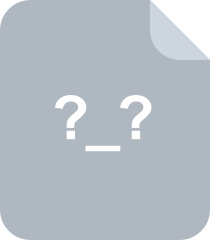
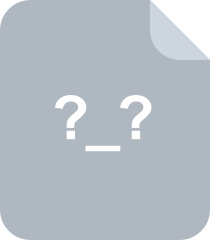
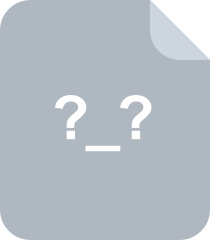
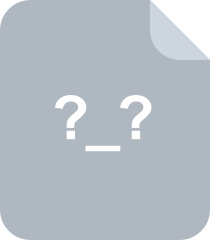
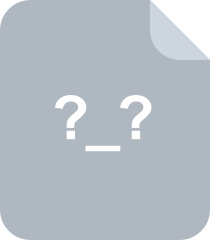
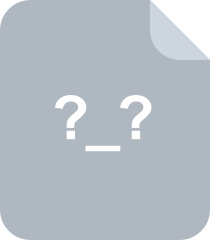
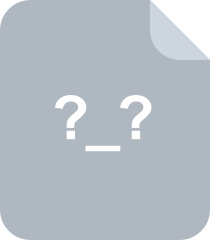
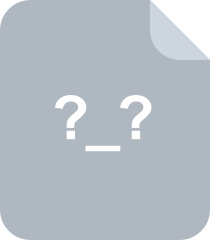
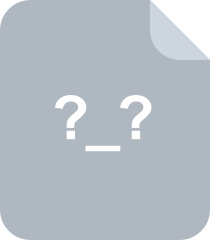
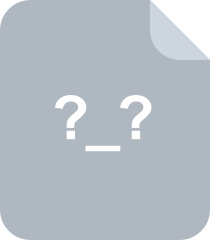
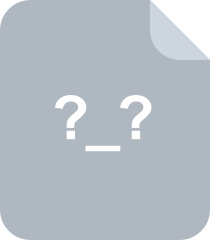
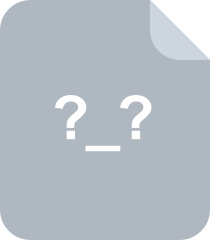
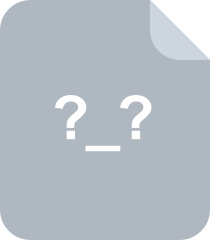
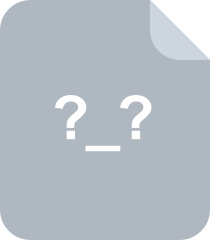
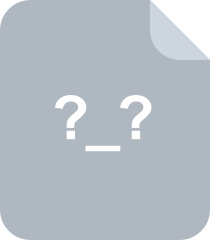
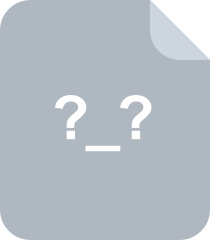
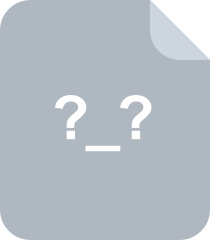
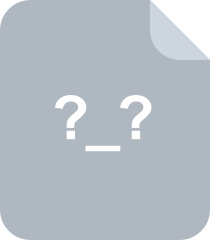
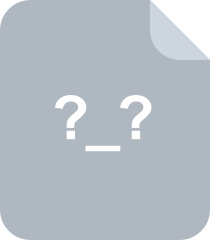
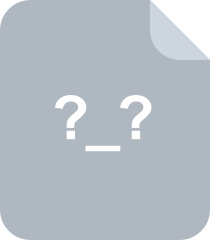
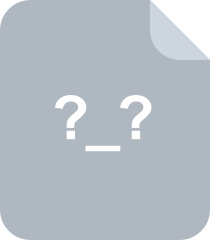
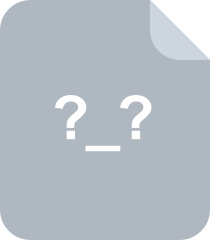
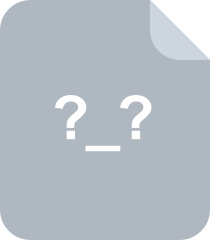
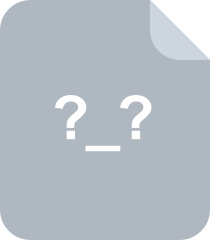
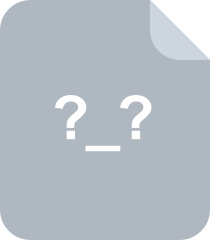
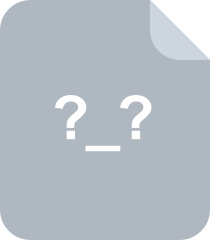
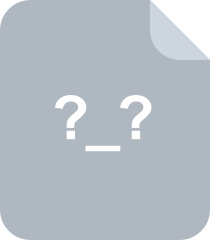
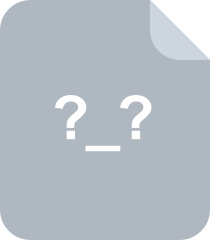
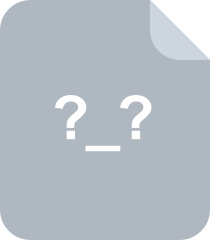
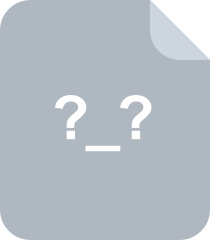
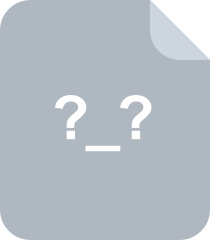
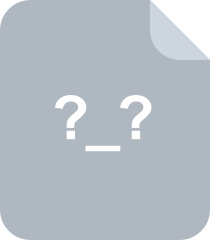
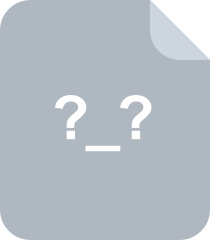
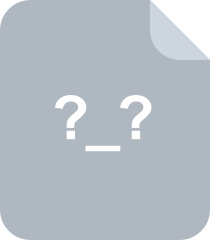
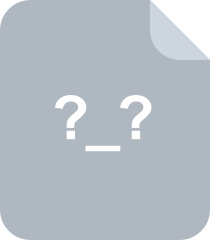
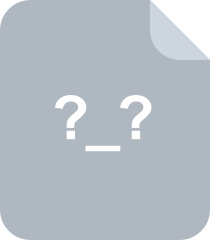
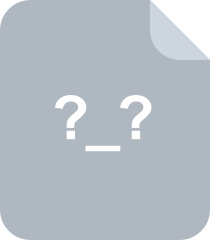
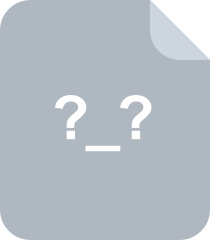
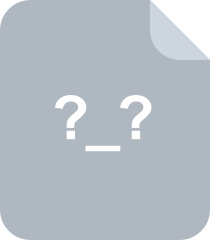
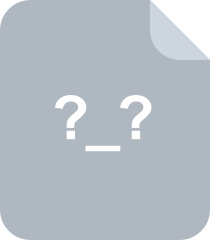
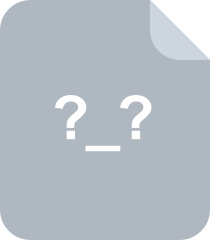
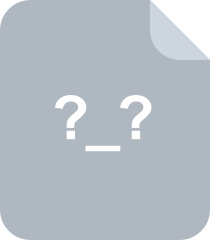
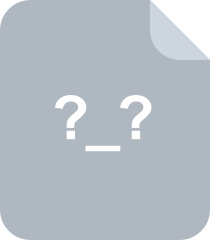
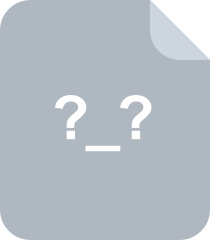
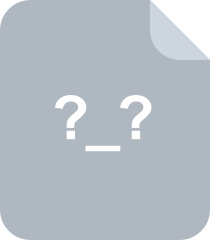
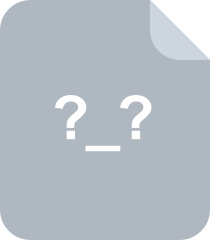
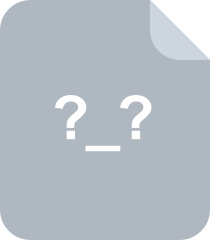
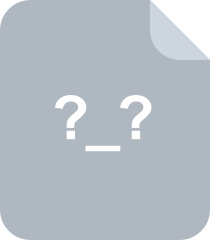
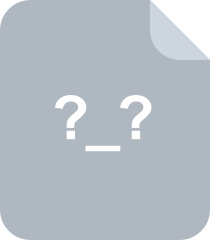
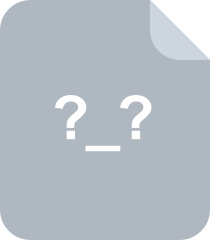
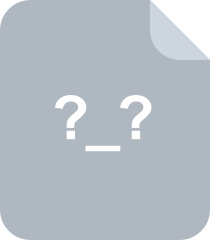
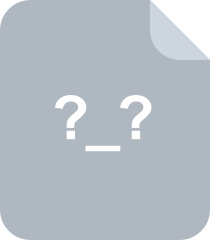
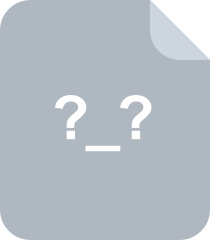
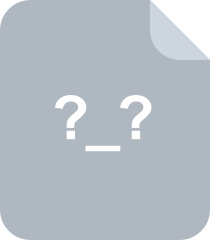
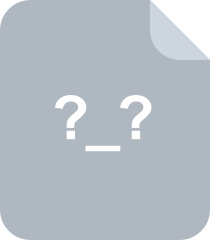
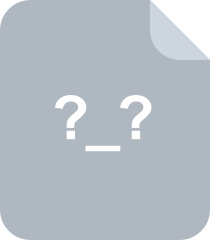
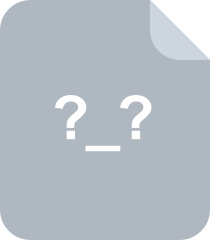
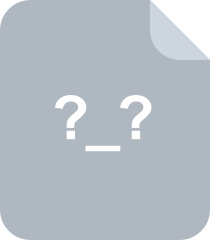
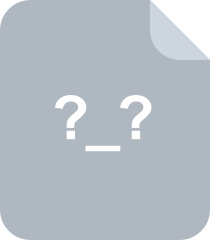
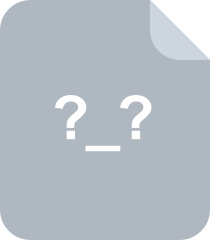
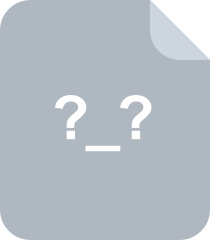
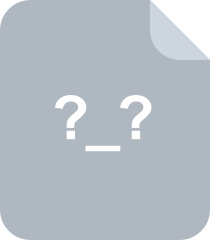
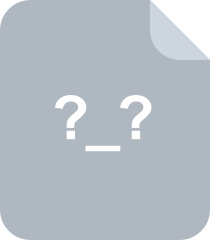
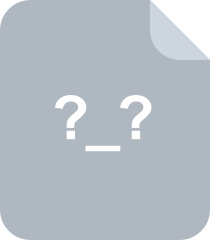
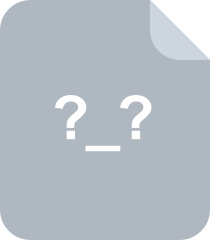
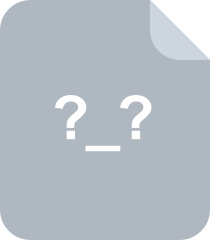
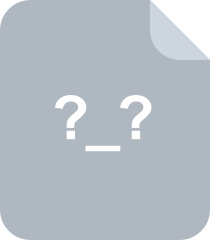
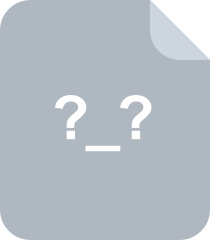
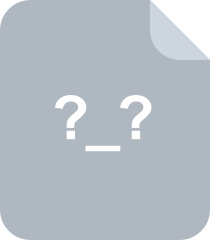
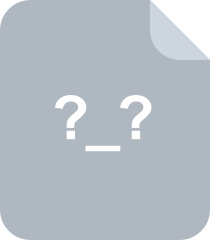
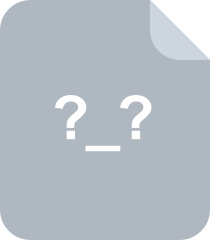
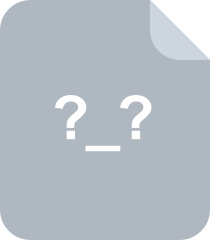
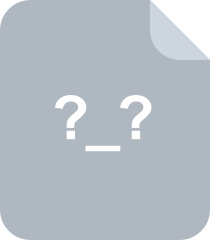
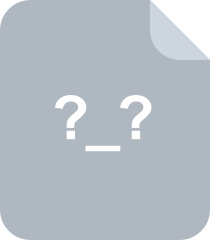
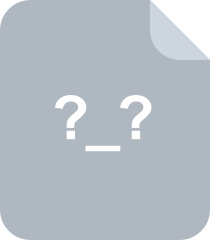
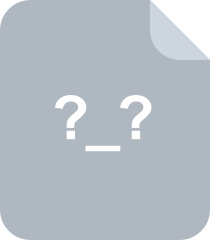
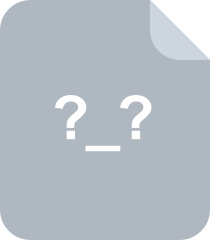
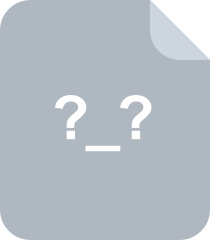
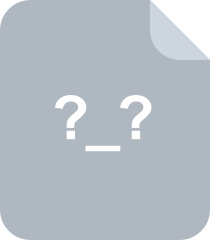
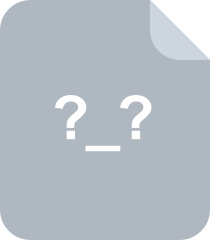
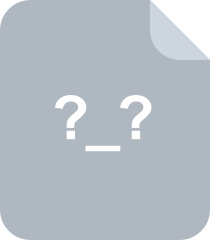
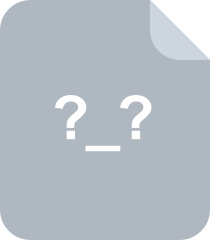
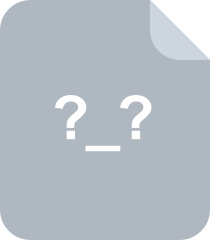
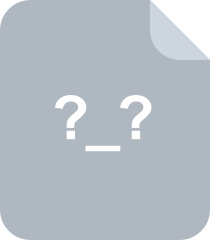
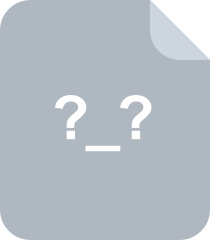
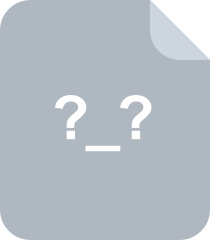
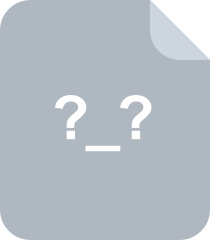
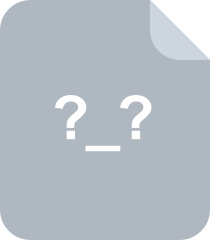
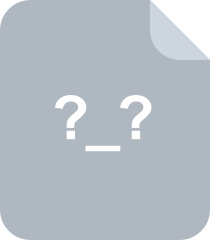
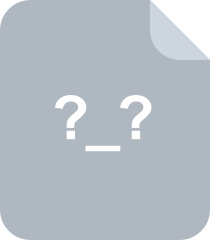
共 434 条
- 1
- 2
- 3
- 4
- 5
资源评论
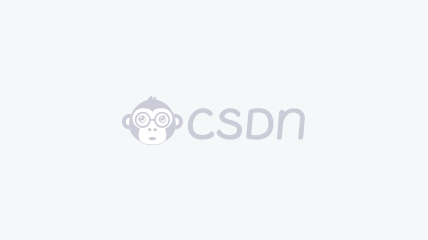
- weixs2012-07-17采用SSH框架,使用MYECLIPSE5.5环境和SQL SERVER2005数据库
- huzhancheng2012-08-23jsp+mysql+struts+spring+hibernate+jsp整合的项目,但是没有数据库,需要自己建库。

hqula
- 粉丝: 2
- 资源: 30
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

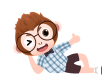
最新资源
- 基于GPRS的环境监测数据传输系统的研究
- 基于FPGA的TFT-LCD视频驱动系统设计
- 基于MSP430的智能家居系统的设计与实现
- 基于LabVIEW喷雾干燥机模糊控制系统的设计
- calibre-7.23.0.dmg
- 机械设计汽车车身底板装配线sw21全套设计资料100%好用.zip.zip
- 机械设计汽车玻璃延时料架设备(sw18可编辑+工程图+BOM)全套设计资料100%好用.zip.zip
- 机械设计平板主板整流罩贴泡棉机sw18可编辑全套设计资料100%好用.zip.zip
- 机械设计汽车天窗装配线sw16全套设计资料100%好用.zip.zip
- 机械设计汽车天窗底涂工作站(sw18可编辑+工程图+BOM)全套设计资料100%好用.zip.zip
- 机械设计汽车连接器插端包装一体机sw2016可编辑全套设计资料100%好用.zip.zip
- 机械设计汽车头枕盖自动去毛刺设备sw2016全套设计资料100%好用.zip.zip
- 机械设计全自动导管检测机(sw18可编辑+BOM)全套设计资料100%好用.zip.zip
- 机械设计全自动动平衡量测移载机stp全套设计资料100%好用.zip.zip
- 机械设计全自动上料双工位锁螺丝机stp全套设计资料100%好用.zip.zip
- 机械设计全自动端子插针机sw18全套设计资料100%好用.zip.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


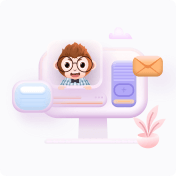
安全验证
文档复制为VIP权益,开通VIP直接复制
