#include <afxwin.h>
#include "VisualKB.h"
CMyApp myApp;
/////////////////////////////////////////////////////////////////////////
// CMyApp member functions
BOOL CMyApp::InitInstance ()
{
m_pMainWnd = new CMainWindow;
m_pMainWnd->ShowWindow (m_nCmdShow);
m_pMainWnd->UpdateWindow ();
return TRUE;
}
/////////////////////////////////////////////////////////////////////////
// CMainWindow message map and member functions
BEGIN_MESSAGE_MAP (CMainWindow, CWnd)
ON_WM_CREATE ()
ON_WM_PAINT ()
ON_WM_SETFOCUS ()
ON_WM_KILLFOCUS ()
ON_WM_SETCURSOR ()
ON_WM_LBUTTONDOWN ()
ON_WM_KEYDOWN ()
ON_WM_KEYUP ()
ON_WM_SYSKEYDOWN ()
ON_WM_SYSKEYUP ()
ON_WM_CHAR ()
ON_WM_SYSCHAR ()
END_MESSAGE_MAP ()
CMainWindow::CMainWindow ()
{
m_nTextPos = 0;
m_nMsgPos = 0;
//
// Load the arrow cursor and the I-beam cursor and save their handles.
//
m_hCursorArrow = AfxGetApp ()->LoadStandardCursor (IDC_ARROW);
m_hCursorIBeam = AfxGetApp ()->LoadStandardCursor (IDC_IBEAM);
//
// Register a WNDCLASS.
//
CString strWndClass = AfxRegisterWndClass (
0,
NULL,
(HBRUSH) (COLOR_3DFACE + 1),
AfxGetApp ()->LoadStandardIcon (IDI_WINLOGO)
);
//
// Create a window.
//
CreateEx (0, strWndClass, _T ("Visual Keyboard"),
WS_OVERLAPPED | WS_SYSMENU | WS_CAPTION | WS_MINIMIZEBOX,
CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT,
NULL, NULL);
}
int CMainWindow::OnCreate (LPCREATESTRUCT lpCreateStruct)
{
if (CWnd::OnCreate (lpCreateStruct) == -1)
return -1;
//
// Initialize member variables whose values are dependent upon screen
// metrics.
//
CClientDC dc (this);
TEXTMETRIC tm;
dc.GetTextMetrics (&tm);
m_cxChar = tm.tmAveCharWidth;
m_cyChar = tm.tmHeight;
m_cyLine = tm.tmHeight + tm.tmExternalLeading;
m_rcTextBoxBorder.SetRect (16, 16, (m_cxChar * 64) + 16,
((m_cyChar * 3) / 2) + 16);
m_rcTextBox = m_rcTextBoxBorder;
m_rcTextBox.InflateRect (-2, -2);
m_rcMsgBoxBorder.SetRect (16, (m_cyChar * 4) + 16,
(m_cxChar * 64) + 16, (m_cyLine * MAX_STRINGS) +
(m_cyChar * 6) + 16);
m_rcScroll.SetRect (m_cxChar + 16, (m_cyChar * 6) + 16,
(m_cxChar * 63) + 16, (m_cyLine * MAX_STRINGS) +
(m_cyChar * 5) + 16);
m_ptTextOrigin.x = m_cxChar + 16;
m_ptTextOrigin.y = (m_cyChar / 4) + 16;
m_ptCaretPos = m_ptTextOrigin;
m_nTextLimit = (m_cxChar * 63) + 16;
m_ptHeaderOrigin.x = m_cxChar + 16;
m_ptHeaderOrigin.y = (m_cyChar * 3) + 16;
m_ptUpperMsgOrigin.x = m_cxChar + 16;
m_ptUpperMsgOrigin.y = (m_cyChar * 5) + 16;
m_ptLowerMsgOrigin.x = m_cxChar + 16;
m_ptLowerMsgOrigin.y = (m_cyChar * 5) +
(m_cyLine * (MAX_STRINGS - 1)) + 16;
m_nTabStops[0] = (m_cxChar * 24) + 16;
m_nTabStops[1] = (m_cxChar * 30) + 16;
m_nTabStops[2] = (m_cxChar * 36) + 16;
m_nTabStops[3] = (m_cxChar * 42) + 16;
m_nTabStops[4] = (m_cxChar * 46) + 16;
m_nTabStops[5] = (m_cxChar * 50) + 16;
m_nTabStops[6] = (m_cxChar * 54) + 16;
//
// Size the window.
//
CRect rect (0, 0, m_rcMsgBoxBorder.right + 16,
m_rcMsgBoxBorder.bottom + 16);
CalcWindowRect (&rect);
SetWindowPos (NULL, 0, 0, rect.Width (), rect.Height (),
SWP_NOZORDER | SWP_NOMOVE | SWP_NOREDRAW);
return 0;
}
void CMainWindow::PostNcDestroy ()
{
delete this;
}
void CMainWindow::OnPaint ()
{
CPaintDC dc (this);
//
// Draw the rectangles surrounding the text box and the message list.
//
dc.DrawEdge (m_rcTextBoxBorder, EDGE_SUNKEN, BF_RECT);
dc.DrawEdge (m_rcMsgBoxBorder, EDGE_SUNKEN, BF_RECT);
//
// Draw all the text that appears in the window.
//
DrawInputText (&dc);
DrawMessageHeader (&dc);
DrawMessages (&dc);
}
void CMainWindow::OnSetFocus (CWnd* pWnd)
{
//
// Show the caret when the VisualKB window receives the input focus.
//
CreateSolidCaret (max (2, ::GetSystemMetrics (SM_CXBORDER)),
m_cyChar);
SetCaretPos (m_ptCaretPos);
ShowCaret ();
}
void CMainWindow::OnKillFocus (CWnd* pWnd)
{
//
// Hide the caret when the VisualKB window loses the input focus.
//
HideCaret ();
m_ptCaretPos = GetCaretPos ();
::DestroyCaret ();
}
BOOL CMainWindow::OnSetCursor (CWnd* pWnd, UINT nHitTest, UINT message)
{
//
// Change the cursor to an I-beam if it's currently over the text box,
// or to an arrow if it's positioned anywhere else.
//
if (nHitTest == HTCLIENT) {
DWORD dwPos = ::GetMessagePos ();
CPoint point (LOWORD (dwPos), HIWORD (dwPos));
ScreenToClient (&point);
::SetCursor (m_rcTextBox.PtInRect (point) ?
m_hCursorIBeam : m_hCursorArrow);
return TRUE;
}
return CWnd::OnSetCursor (pWnd, nHitTest, message);
}
void CMainWindow::OnLButtonDown (UINT nFlags, CPoint point)
{
//
// Move the caret if the text box is clicked with the left mouse button.
//
if (m_rcTextBox.PtInRect (point)) {
m_nTextPos = GetNearestPos (point);
PositionCaret ();
}
}
void CMainWindow::OnKeyDown (UINT nChar, UINT nRepCnt, UINT nFlags)
{
ShowMessage (_T ("WM_KEYDOWN"), nChar, nRepCnt, nFlags);
//
// Move the caret when the left, right, Home, or End key is pressed.
//
switch (nChar) {
case VK_LEFT:
if (m_nTextPos != 0) {
m_nTextPos--;
PositionCaret ();
}
break;
case VK_RIGHT:
if (m_nTextPos != m_strInputText.GetLength ()) {
m_nTextPos++;
PositionCaret ();
}
break;
case VK_HOME:
m_nTextPos = 0;
PositionCaret ();
break;
case VK_END:
m_nTextPos = m_strInputText.GetLength ();
PositionCaret ();
break;
}
}
void CMainWindow::OnChar (UINT nChar, UINT nRepCnt, UINT nFlags)
{
ShowMessage (_T ("WM_CHAR"), nChar, nRepCnt, nFlags);
CClientDC dc (this);
//
// Determine which character was just input from the keyboard.
//
switch (nChar) {
case VK_ESCAPE:
case VK_RETURN:
m_strInputText.Empty ();
m_nTextPos = 0;
break;
case VK_BACK:
if (m_nTextPos != 0) {
m_strInputText = m_strInputText.Left (m_nTextPos - 1) +
m_strInputText.Right (m_strInputText.GetLength () -
m_nTextPos);
m_nTextPos--;
}
break;
default:
if ((nChar >= 0) && (nChar <= 31))
return;
if (m_nTextPos == m_strInputText.GetLength ()) {
m_strInputText += nChar;
m_nTextPos++;
}
else
m_strInputText.SetAt (m_nTextPos++, nChar);
CSize size = dc.GetTextExtent (m_strInputText,
m_strInputText.GetLength ());
if ((m_ptTextOrigin.x + size.cx) > m_nTextLimit) {
m_strInputText = nChar;
m_nTextPos = 1;
}
break;
}
//
// Update the contents of the text box.
//
HideCaret ();
DrawInputText (&dc);
PositionCaret (&dc);
ShowCaret ();
}
void CMainWindow::OnKeyUp (UINT nChar, UINT nRepCnt, UINT nFlags)
{
ShowMessage (_T ("WM_KEYUP"), nChar, nRepCnt, nFlags);
CWnd::OnKeyUp (nChar, nRepCnt, nFlags);
}
void CMainWindow::OnSysKeyDown (UINT nChar, UINT nRepCnt, UINT nFlags)
{
ShowMessage (_T ("WM_SYSKEYDOWN"), nChar, nRepCnt, nFlags);
CWnd::OnSysKeyDown (nChar, nRepCnt, nFlags);
}
void CMainWindow::OnSysChar (UINT nChar, UINT nRepCnt, UINT nFlags)
{
ShowMessage (_T ("WM_SYSCHAR"), nChar, nRepCnt, nFlags);
CWnd::OnSysChar (nChar, nRepCnt, nFlags);

五一编程
- 粉丝: 1w+
- 资源: 882
最新资源
- 音乐网站(JSP+SERVLET).rar
- 抢购软件:快速复制信息
- oracle错误代码和信息速查手册chm版最新版本
- MATLAB【逆变器二次调频模型】 微电网分布式电源逆变器DROOP控制二次调频模型,加入二次控制实现二次调频控制,及二次调压控制,程序可实现上图功能,工况有所改变 需要matlab2021A版
- 基于python的网页自动化工具项目全套技术资料100%好用.zip
- Oracle数据库命令速查手册doc版最新版本
- 程序名称:转向设计计算程序 开发平台:基于matlab平台 计算内容:阿克曼转角,转弯半径,转向阻力矩,回正力矩,转向主参数,转向传动比,力矩波动,转向梯形,EPS匹配,HPS匹配,齿轮齿条传动比,循
- 基于二阶自抗扰ADRC的轨迹跟踪控制,对车辆的不确定性和外界干扰具有一定抗干扰性,基于carsim和simulink仿真 跟踪轨迹为双移线,效果良好,有对应复现资料,是学习自抗扰技术快速入门很好的资料
- TianleSoftwareOracle学习手册中文pdf格式最新版本
- MATLAB代码:基于分布式ADMM算法的考虑碳排放交易的电力系统优化调度研究 关键词:分布式调度 ADMM算法 交替方向乘子法 碳排放 最优潮流 仿真平台:MATLAB+CPLEX GUROBI
- Oracle安装配置使用WORD文档doc格式最新版本
- 西门子840D HMI ADVANCED FOR PC 也可用于810D,840DSL中文版 1、软件可安装到台式机或笔记本上,可以连接到机床的NCU进行NC与PLC的数据备份与恢复,备份和恢复的数
- OraclePLSQL简单安装指南WORD文档doc格式最新版本
- 网页数据采集软件项目全套技术资料100%好用.zip
- Oracle高级SQL培训与讲解WORD文档doc格式最新版本
- 超智能体写的人工智能深度学习pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


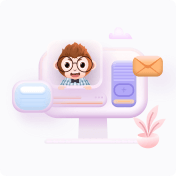