<?php
/**
* Project: Smarty: the PHP compiling template engine
* File: Smarty_Compiler.class.php
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* @link http://smarty.php.net/
* @author Monte Ohrt <monte at ohrt dot com>
* @author Andrei Zmievski <andrei@php.net>
* @version 2.6.26
* @copyright 2001-2005 New Digital Group, Inc.
* @package Smarty
*/
/* $Id: Smarty_Compiler.class.php 3163 2009-06-17 14:39:24Z monte.ohrt $ */
/**
* Template compiling class
* @package Smarty
*/
class Smarty_Compiler extends Smarty {
// internal vars
/**#@+
* @access private
*/
var $_folded_blocks = array(); // keeps folded template blocks
var $_current_file = null; // the current template being compiled
var $_current_line_no = 1; // line number for error messages
var $_capture_stack = array(); // keeps track of nested capture buffers
var $_plugin_info = array(); // keeps track of plugins to load
var $_init_smarty_vars = false;
var $_permitted_tokens = array('true','false','yes','no','on','off','null');
var $_db_qstr_regexp = null; // regexps are setup in the constructor
var $_si_qstr_regexp = null;
var $_qstr_regexp = null;
var $_func_regexp = null;
var $_reg_obj_regexp = null;
var $_var_bracket_regexp = null;
var $_num_const_regexp = null;
var $_dvar_guts_regexp = null;
var $_dvar_regexp = null;
var $_cvar_regexp = null;
var $_svar_regexp = null;
var $_avar_regexp = null;
var $_mod_regexp = null;
var $_var_regexp = null;
var $_parenth_param_regexp = null;
var $_func_call_regexp = null;
var $_obj_ext_regexp = null;
var $_obj_start_regexp = null;
var $_obj_params_regexp = null;
var $_obj_call_regexp = null;
var $_cacheable_state = 0;
var $_cache_attrs_count = 0;
var $_nocache_count = 0;
var $_cache_serial = null;
var $_cache_include = null;
var $_strip_depth = 0;
var $_additional_newline = "\n";
/**#@-*/
/**
* The class constructor.
*/
function Smarty_Compiler()
{
// matches double quoted strings:
// "foobar"
// "foo\"bar"
$this->_db_qstr_regexp = '"[^"\\\\]*(?:\\\\.[^"\\\\]*)*"';
// matches single quoted strings:
// 'foobar'
// 'foo\'bar'
$this->_si_qstr_regexp = '\'[^\'\\\\]*(?:\\\\.[^\'\\\\]*)*\'';
// matches single or double quoted strings
$this->_qstr_regexp = '(?:' . $this->_db_qstr_regexp . '|' . $this->_si_qstr_regexp . ')';
// matches bracket portion of vars
// [0]
// [foo]
// [$bar]
$this->_var_bracket_regexp = '\[\$?[\w\.]+\]';
// matches numerical constants
// 30
// -12
// 13.22
$this->_num_const_regexp = '(?:\-?\d+(?:\.\d+)?)';
// matches $ vars (not objects):
// $foo
// $foo.bar
// $foo.bar.foobar
// $foo[0]
// $foo[$bar]
// $foo[5][blah]
// $foo[5].bar[$foobar][4]
$this->_dvar_math_regexp = '(?:[\+\*\/\%]|(?:-(?!>)))';
$this->_dvar_math_var_regexp = '[\$\w\.\+\-\*\/\%\d\>\[\]]';
$this->_dvar_guts_regexp = '\w+(?:' . $this->_var_bracket_regexp
. ')*(?:\.\$?\w+(?:' . $this->_var_bracket_regexp . ')*)*(?:' . $this->_dvar_math_regexp . '(?:' . $this->_num_const_regexp . '|' . $this->_dvar_math_var_regexp . ')*)?';
$this->_dvar_regexp = '\$' . $this->_dvar_guts_regexp;
// matches config vars:
// #foo#
// #foobar123_foo#
$this->_cvar_regexp = '\#\w+\#';
// matches section vars:
// %foo.bar%
$this->_svar_regexp = '\%\w+\.\w+\%';
// matches all valid variables (no quotes, no modifiers)
$this->_avar_regexp = '(?:' . $this->_dvar_regexp . '|'
. $this->_cvar_regexp . '|' . $this->_svar_regexp . ')';
// matches valid variable syntax:
// $foo
// $foo
// #foo#
// #foo#
// "text"
// "text"
$this->_var_regexp = '(?:' . $this->_avar_regexp . '|' . $this->_qstr_regexp . ')';
// matches valid object call (one level of object nesting allowed in parameters):
// $foo->bar
// $foo->bar()
// $foo->bar("text")
// $foo->bar($foo, $bar, "text")
// $foo->bar($foo, "foo")
// $foo->bar->foo()
// $foo->bar->foo->bar()
// $foo->bar($foo->bar)
// $foo->bar($foo->bar())
// $foo->bar($foo->bar($blah,$foo,44,"foo",$foo[0].bar))
$this->_obj_ext_regexp = '\->(?:\$?' . $this->_dvar_guts_regexp . ')';
$this->_obj_restricted_param_regexp = '(?:'
. '(?:' . $this->_var_regexp . '|' . $this->_num_const_regexp . ')(?:' . $this->_obj_ext_regexp . '(?:\((?:(?:' . $this->_var_regexp . '|' . $this->_num_const_regexp . ')'
. '(?:\s*,\s*(?:' . $this->_var_regexp . '|' . $this->_num_const_regexp . '))*)?\))?)*)';
$this->_obj_single_param_regexp = '(?:\w+|' . $this->_obj_restricted_param_regexp . '(?:\s*,\s*(?:(?:\w+|'
. $this->_var_regexp . $this->_obj_restricted_param_regexp . ')))*)';
$this->_obj_params_regexp = '\((?:' . $this->_obj_single_param_regexp
. '(?:\s*,\s*' . $this->_obj_single_param_regexp . ')*)?\)';
$this->_obj_start_regexp = '(?:' . $this->_dvar_regexp . '(?:' . $this->_obj_ext_regexp . ')+)';
$this->_obj_call_regexp = '(?:' . $this->_obj_start_regexp . '(?:' . $this->_obj_params_regexp . ')?(?:' . $this->_dvar_math_regexp . '(?:' . $this->_num_const_regexp . '|' . $this->_dvar_math_var_regexp . ')*)?)';
// matches valid modifier syntax:
// |foo
// |@foo
// |foo:"bar"
// |foo:$bar
// |foo:"bar":$foobar
// |foo|bar
// |foo:$foo->bar
$this->_mod_regexp = '(?:\|@?\w+(?::(?:\w+|' . $this->_num_const_regexp . '|'
. $this->_obj_call_regexp . '|' . $this->_avar_regexp . '|' . $this->_qstr_regexp .'))*)';
// matches valid function name:
// foo123
// _foo_bar
$this->_func_regexp = '[a-zA-Z_]\w*';
// matches valid registered object:
// foo->bar
$this->_reg_obj_regexp = '[a-zA-Z_]\w*->[a-zA-Z_]\w*';
// matches valid parameter values:
// true
// $foo
// $foo|bar
// #foo#
// #foo#|bar
// "text"
// "text"|bar
// $foo->bar
$this->_param_regexp = '(?:\s*(?:' . $this->_obj_call_regexp . '|'
. $this->_var_regexp . '|' . $this->_num_const_regexp . '|\w+)(?>' . $this->_mod_regexp . '*)\s*)';
// matches valid parenthesised function parameters:
//
// "text"
// $foo, $bar, "text"
// $foo|bar, "foo"|bar, $foo->bar($foo)|bar
$this->_parenth_param_regexp = '(?:\((?:\w+|'
. $
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
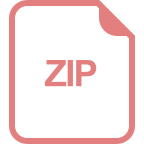
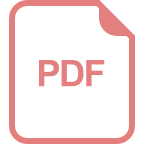
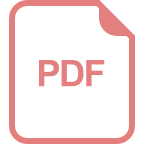
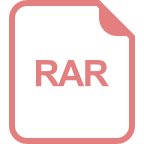
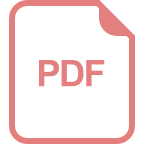
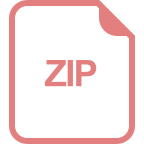
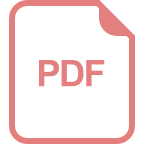
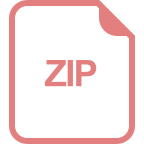
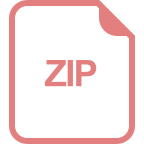
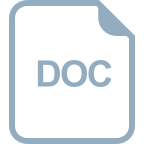
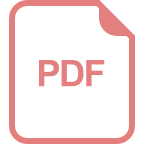
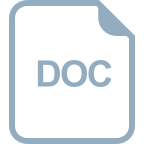
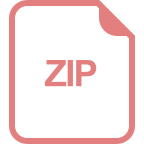
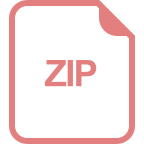
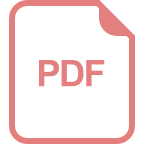
收起资源包目录

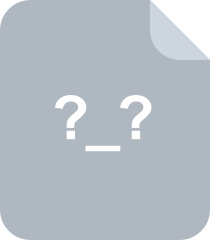
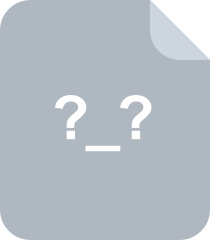
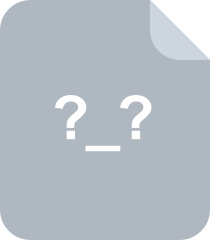
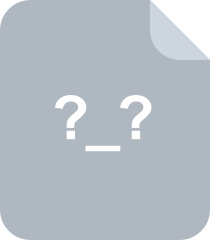
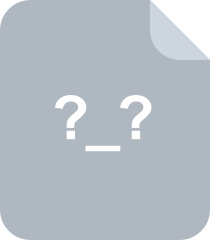
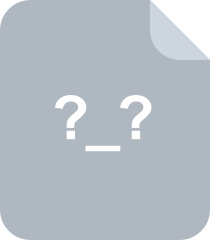
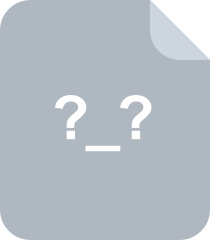
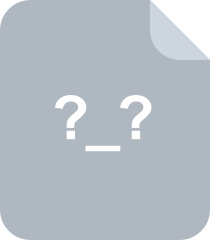
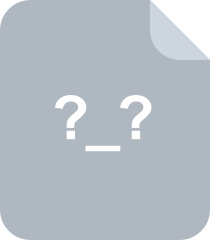
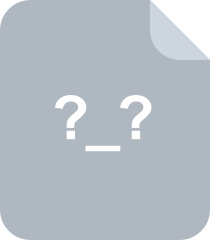
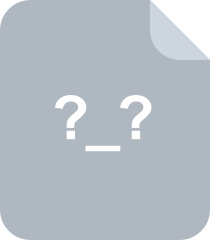
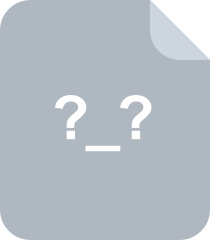
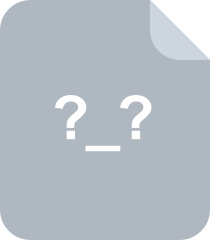
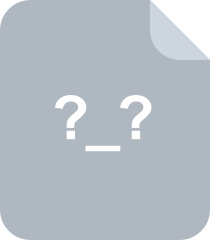
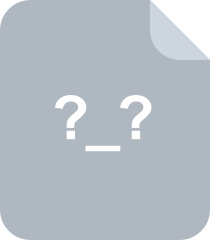
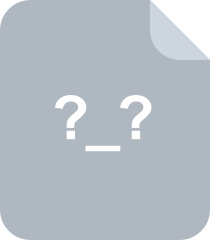
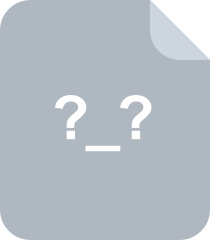
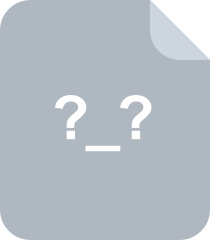
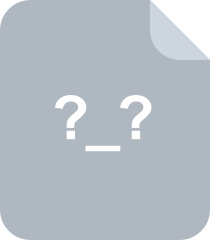
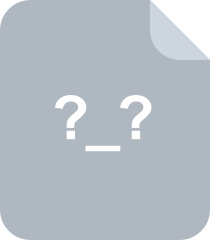
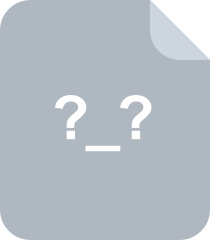
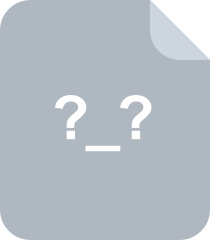
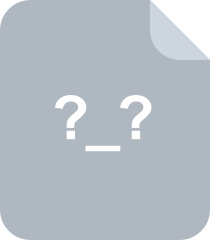
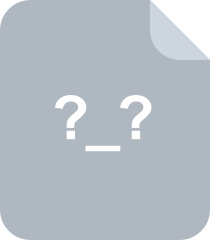
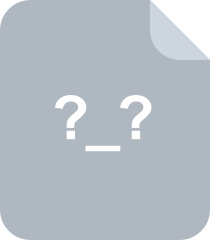
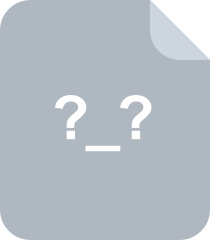
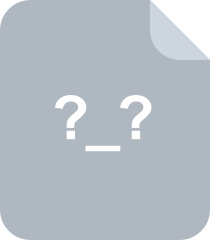
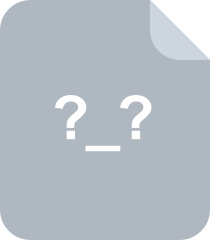
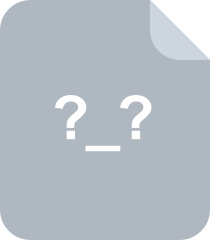
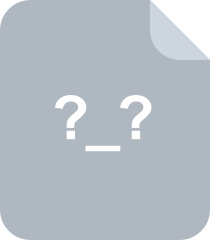
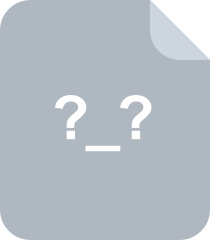
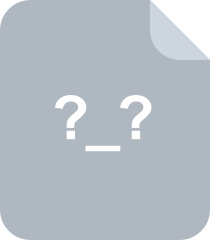
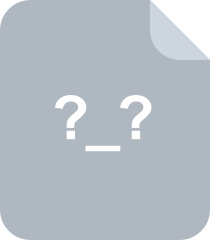
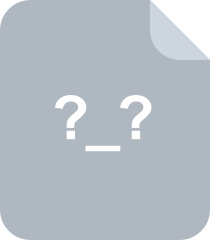
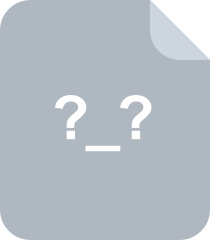
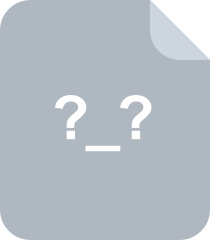
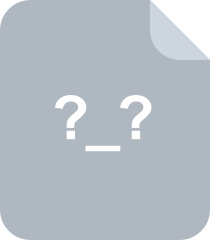
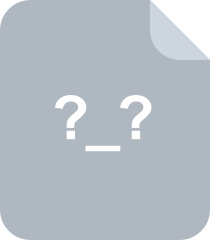
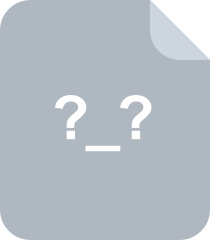
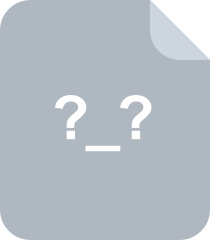
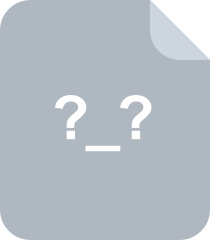
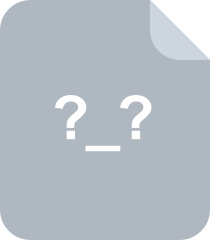
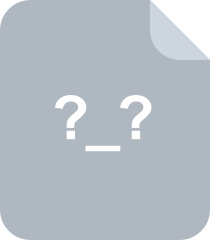
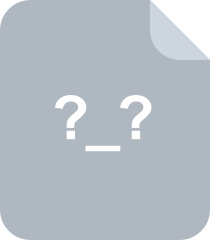
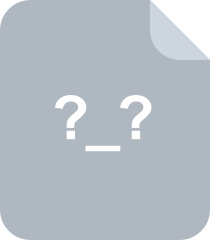
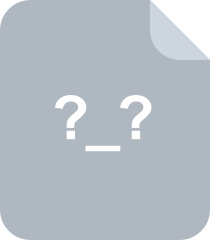
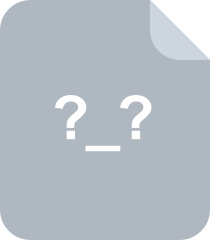
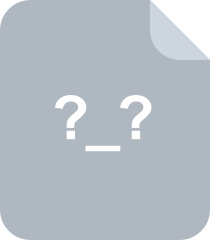
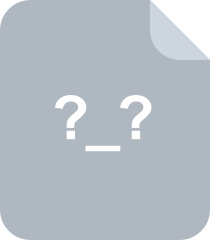
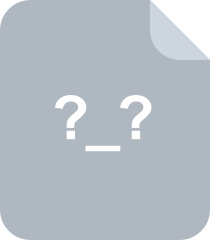
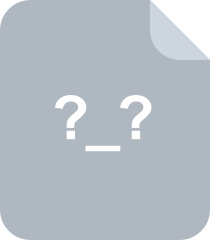
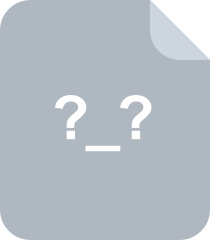
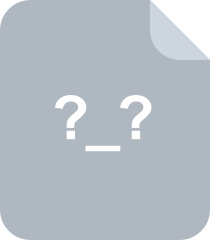
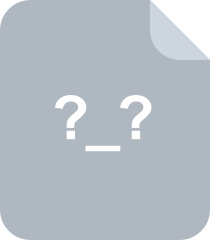
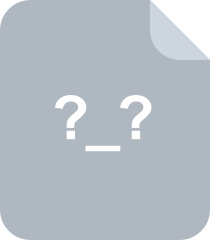
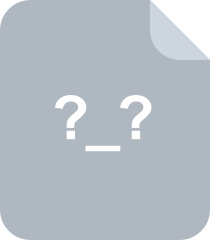
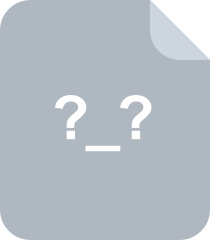
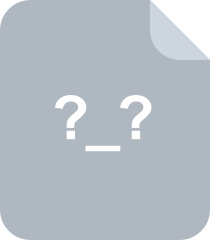
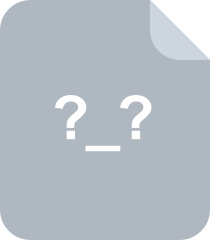
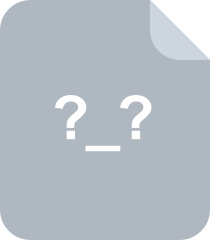
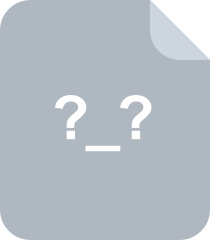
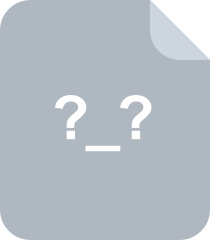
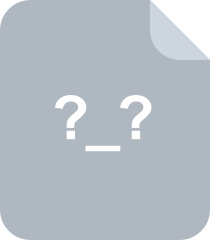
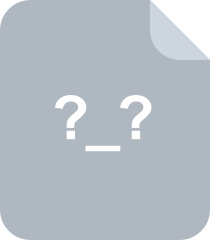
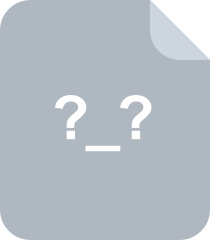
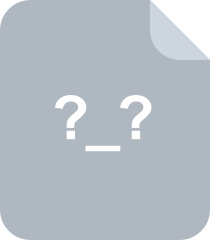
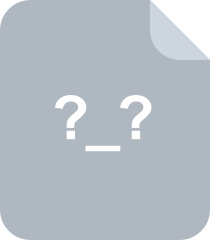
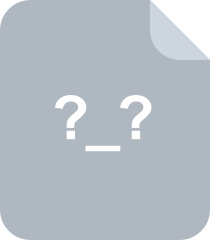
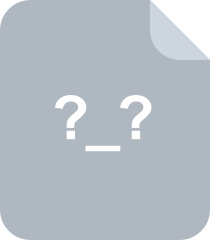
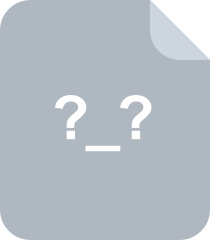
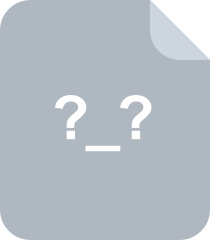
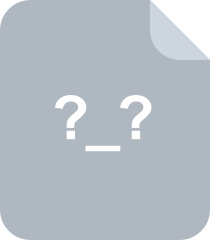
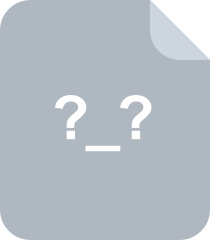
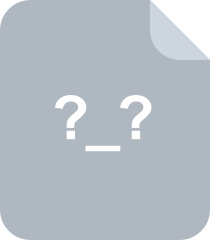
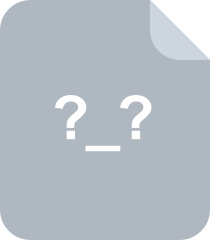
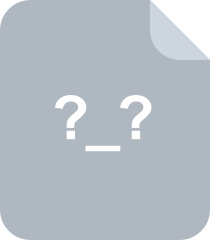
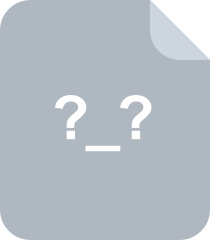
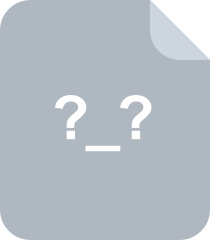
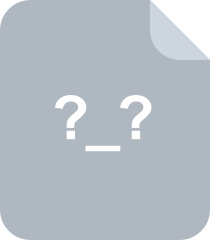
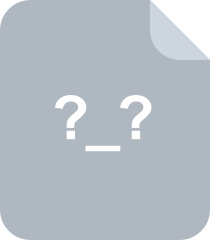
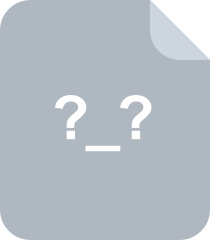
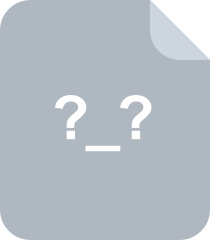
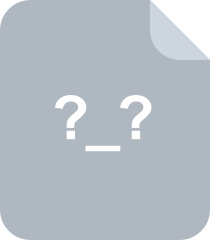
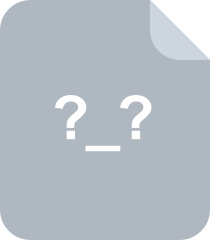
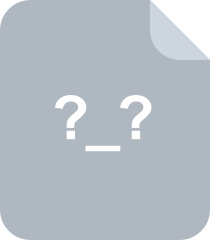
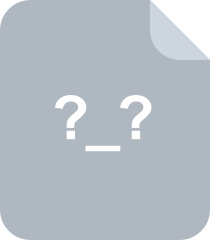
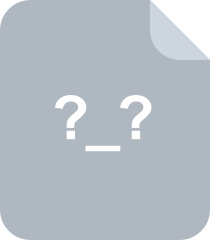
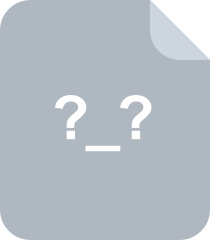
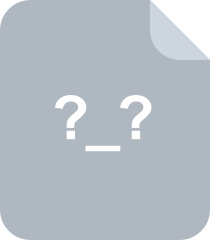
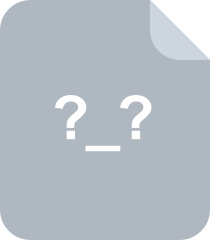
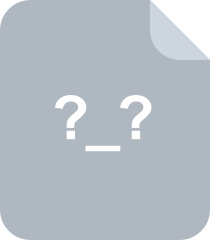
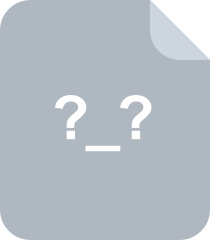
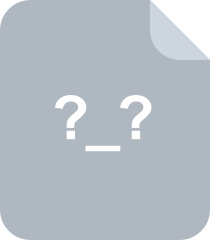
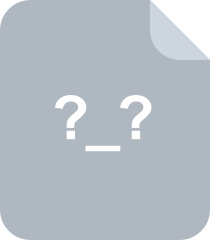
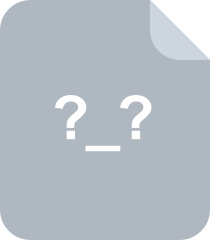
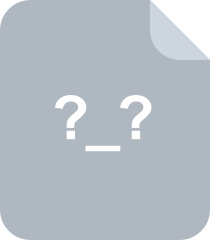
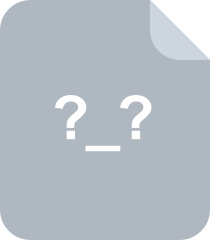
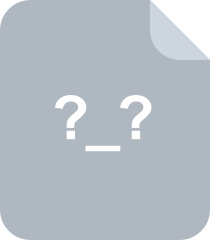
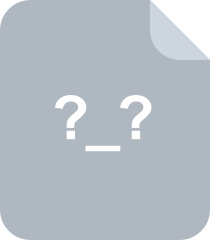
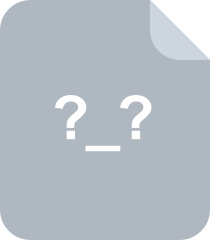
共 141 条
- 1
- 2
资源评论
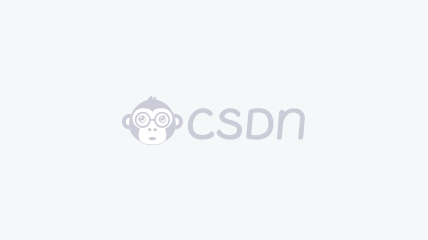
- 丨十一丨2020-12-30这个是php的,其他人下载前注意下

hm446434459
- 粉丝: 4
- 资源: 18
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

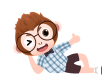
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


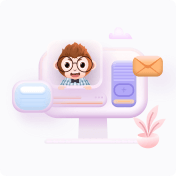
安全验证
文档复制为VIP权益,开通VIP直接复制
