package com.forest.communityproperty.contoller;
import com.forest.communityproperty.entity.*;
import com.forest.communityproperty.global.Forest_variable;
import com.forest.communityproperty.service.*;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@RestController
public class Forest_roomnamemessageController {
//map存储数据
private Map<String, Object> map = new HashMap<>();
//使用数据存储房间编号,楼房编号,业主编号
private int[] arr = new int[3];
//统计数据的页数和单页数据
private int count, num;
//日常统计信息
public Forest_currentEntry f = new Forest_currentEntry();
/**
* 系统管理业务层
*/
//楼房与房间的关联service层
@Autowired
private Forest_roomnamemessageService forest_roomnamemessageService;
//日常信息的service层
@Autowired
private Forest_currentEntryService forest_currentEntryService;
//房间的service层
@Autowired
private Forest_roomnameService forest_roomnameService;
//楼房的service层
@Autowired
private Forest_roommessageService forest_roommessageService;
/**
* floorSelect
* 首次加载页面
*
* @RequestBody Forest_roomnamemessage model, HttpServletRequest request
*/
@RequestMapping("/floorSelect")
public Map<String, Object> insertSelective(@RequestBody Forest_roomnamemessage model, HttpServletRequest request) {
//判断用户是否存在登录了
if (new Forest_variable().variableNameSession(request) == 500) {
//状态码 500错误
map.put("code", 500);
return map;
}
//求出统计的数据
num = count(model);
//查询的业主、房间、楼房、关联四表的数据
List<Forest_roomnamemessage> list = forest_roomnamemessageService.selectEmployee(model);
//系统物业人员的账号名称
map.put("name", new Forest_variable().sessionName(request));
//存储的业主、房间、楼房、关联四表的数据
map.put("user", list);
//统计出来的页数
map.put("num", num);
//状态码 200正确
map.put("code", 200);
return map;
}
/**
* 求出统计的数据
*/
public int count(Forest_roomnamemessage model) {
//查询统计的数据
count = forest_roomnamemessageService.findSelectCount();
//通过计算判断页数
if (count % model.getSize() == 0) {
num = count / model.getSize();
} else {
num = count / model.getSize() + 1;
}
//如果大于8时只能返回8
if (num >= 8) {
return 8;
}
return num;
}
/**
* yeZhuSelectFenYe
* 分页查询
*/
@RequestMapping("/floorSelectFenYe")
public Map<String, Object> yeZhuSelectFenYe(@RequestBody Forest_roomnamemessage model, HttpServletRequest request) {
//求出统计的数据
num = count(model);
//计算起始的值
int ss = model.getNum() * model.getSize();
model.setNum(ss);
//查询的业主、房间、楼房、关联四表的数据
List<Forest_roomnamemessage> list = forest_roomnamemessageService.selectEmployee(model);
//存储的业主、房间、楼房、关联四表的数据
map.put("user", list);
//统计出来的页数
map.put("num", num);
//状态码 200正确
map.put("code", 200);
return map;
}
/**
* floorDelete
* 删除楼房信息
*/
@RequestMapping("/floorDelete")
public Map<String, Object> deleteByPrimaryKey(@RequestParam("roomNameID") int model, HttpServletRequest request, HttpSession session) {
//关联表的实例化
Forest_roomnamemessage ds = new Forest_roomnamemessage();
//设置关联编号
ds.setRoomNameID(model);
//删除关联信息
int shanchu = forest_roomnamemessageService.deleteByPrimaryKey(model);
//判断删除是否成功
if (shanchu == 1) {
//通过关联编号查询业主编号
Forest_roomnamemessage l = forest_roomnamemessageService.findSelectCountID(ds);
/**
* 添加操作信息
* */
//设置业主编号
f.setYeZhuID(l.getYeZhuID());
//设置操作类型
f.setStyleID(3);
//获取session中系统管理员姓名name值
String name = (String) session.getAttribute("name");
//获取session中系统管理员的编号id值
int id = (int) session.getAttribute("id");
//赋值给entity的方法
f.setCurrentEntryName(name);
f.setXtYongHuID(id);
//新增日常信息
int list = forest_currentEntryService.insertSelectiveS(f);
//状态码 成功返回200
map.put("code", 200);
return map;
}
//状态码 失败返回400
map.put("code", 400);
return map;
}
/**
* floorSelectAgo
* 查询之前楼房信息
*/
@RequestMapping("/floorSelectAgo")
public Map<String, Object> floorSelectAgo() {
//查询房间信息
List<Forest_roomname> room = forest_roomnameService.selectEmployee();
//查询楼房信息
List<Forest_roommessage> floor = forest_roommessageService.selectEmployee();
//存储楼房信息
map.put("floor", floor);
//存储房间信息
map.put("room", room);
//状态码 成功返回200
map.put("code", 200);
return map;
}
/**
* floorInsert
* 新增关联信息
*/
@RequestMapping("/floorInsert")
public Map<String, Object> floorInsert(@RequestBody Forest_roomnamemessage model, HttpServletRequest request, HttpSession session) {
//设置时间的格式
SimpleDateFormat sf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
//获取时间的方法
Date d = new Date();
//将获取的时间转换成设置的时间格式进行存储
model.setRoomNameDate(sf.format(d));
//查询楼房和房间是否已存储
List<Forest_roomnamemessage> list = forest_roomnamemessageService.selectByPrimaryKeys(model);
//如果存在
if (list.size() == 1) {
//状态码 返回400
map.put("code", 400);
return map;
} else {
//存储用户的数据
map.put("list", list);
//新增加业主信息
int add = forest_roomnamemessageService.insertSelective(model);
//进行评判
if (add == 1) {
//设置业主编号
f.setYeZhuID(model.getYeZhuID());
//设置日常操作类型
f.setStyleID(1);
//获取session的物业登录名
String name = (String) session.getAttribute("name");
//获取session的物业编号
int id = (int) session.getAttribute("id");
//设置物业登录名
f.setCurrentEntryName(name);
//设置物业编号
f.setXtYongHuID(id);
//存储日常操作信息
int sist = forest_currentEntryService.insertSelectiveS(f);
//状态码 返回200
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
# springboot+ajax+小区物业项目 #### Description springboot的第一个合格项目 小区物业管理系统 #### Software Architecture Software architecture description #### Installation 1. xxxx 2. xxxx 3. xxxx #### Instructions 1. xxxx 2. xxxx 3. xxxx #### Contribution 1. Fork the repository 2. Create Feat_xxx branch 3. Commit your code 4. Create Pull Request
资源推荐
资源详情
资源评论
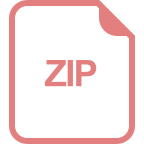
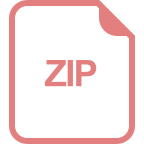
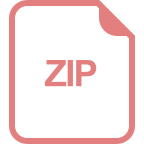
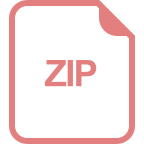
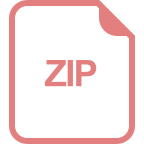
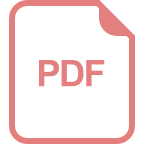
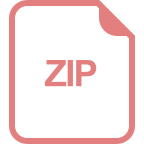
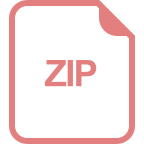
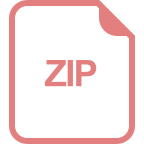
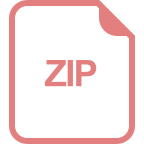
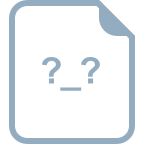
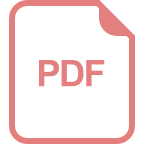
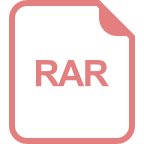
收起资源包目录

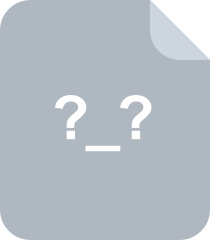
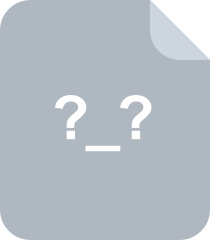
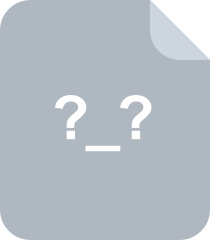
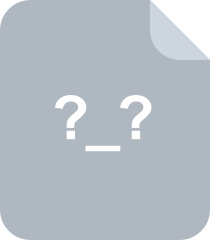
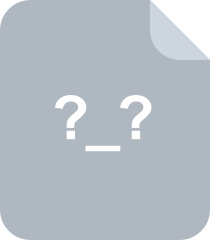
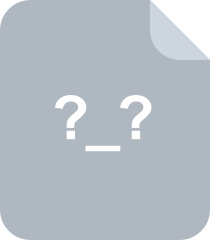
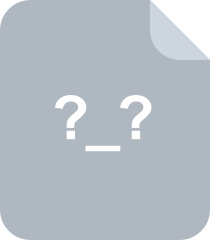
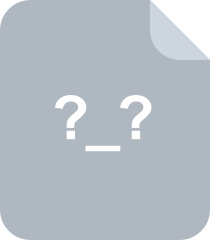
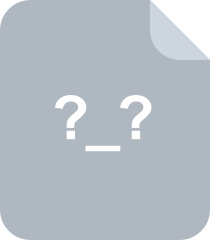
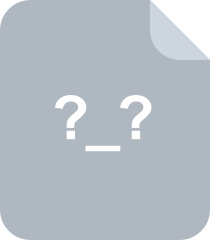
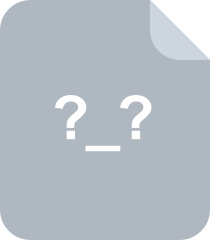
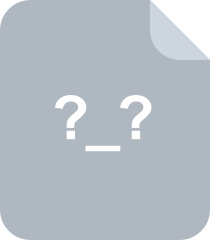
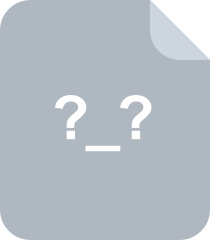
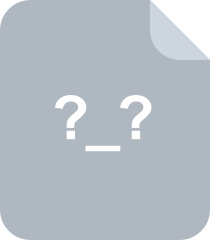
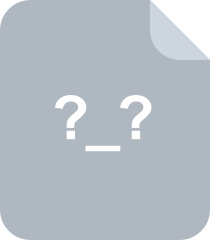
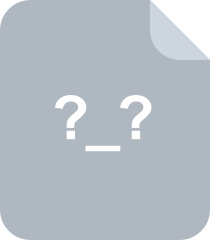
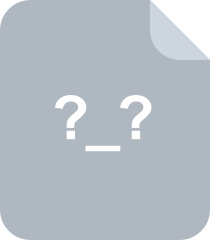
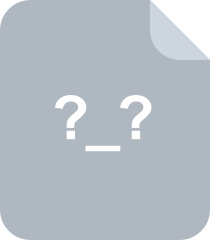
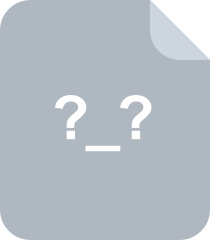
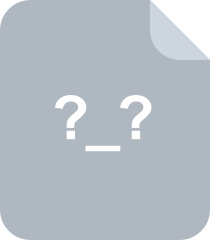
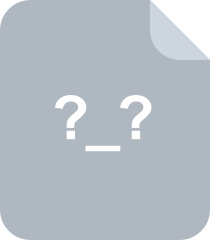
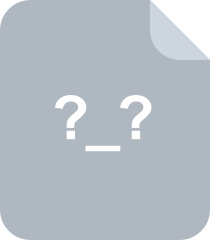
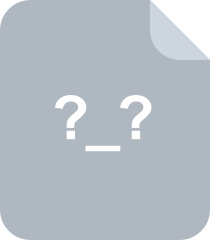
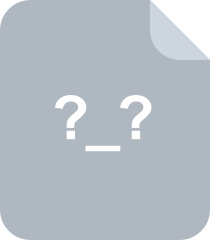
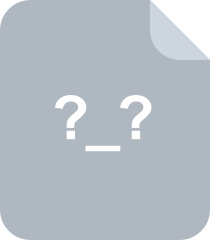
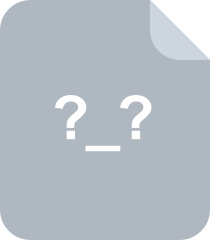
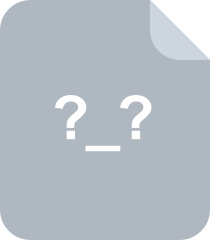
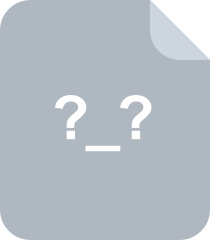
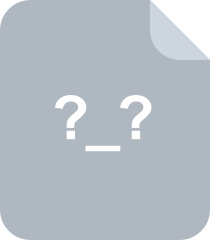
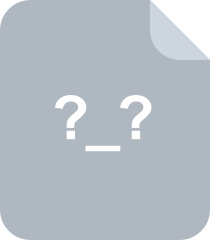
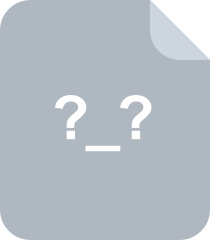
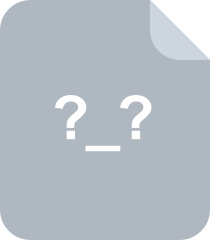
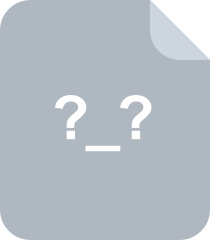
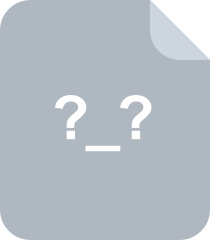
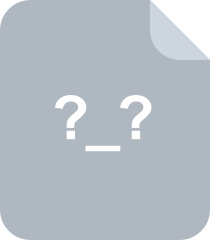
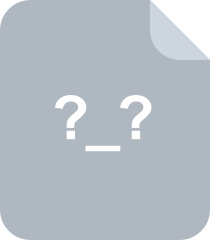
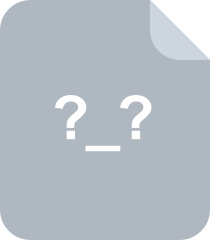
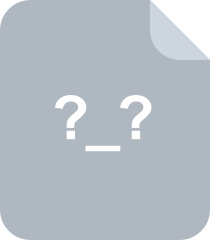
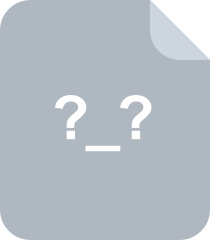
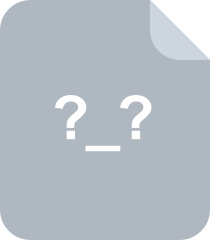
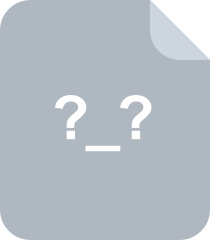
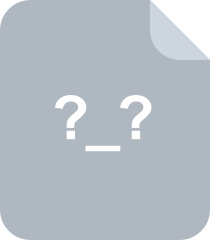
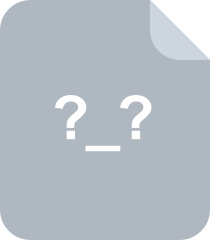
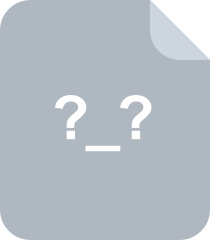
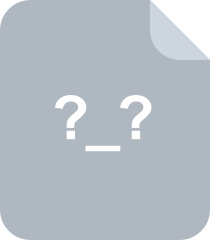
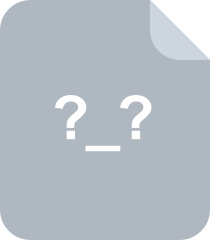
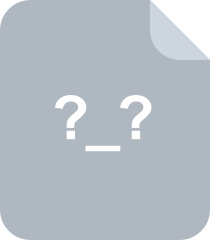
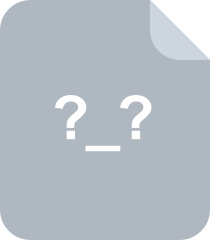
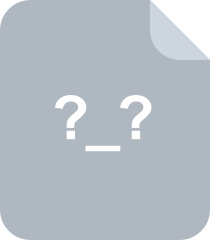
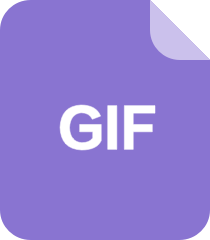
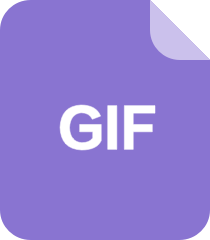
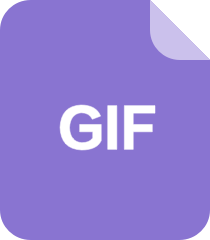
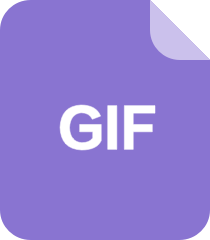
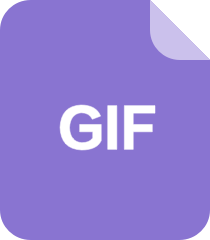
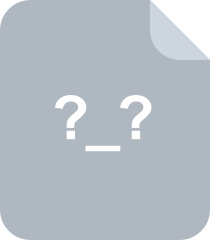
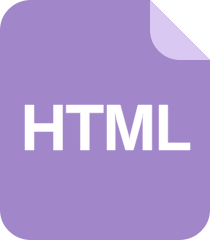
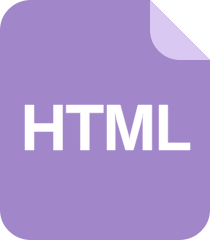
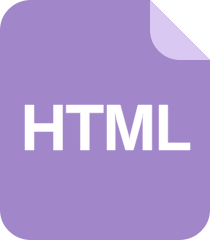
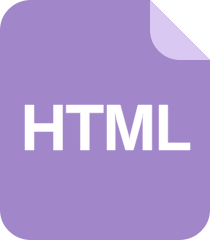
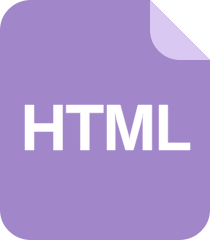
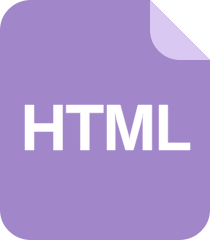
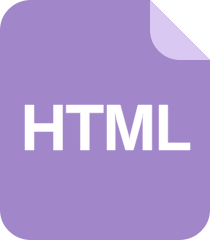
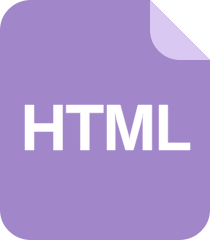
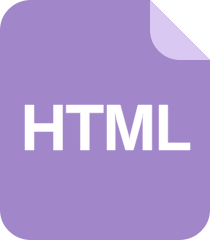
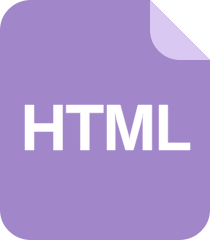
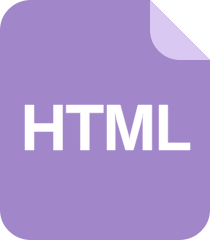
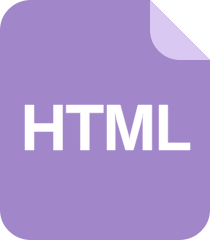
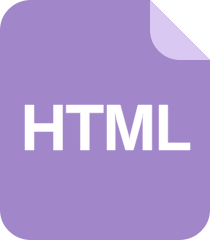
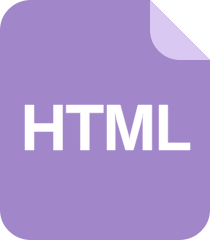
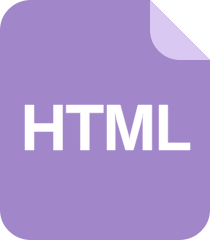
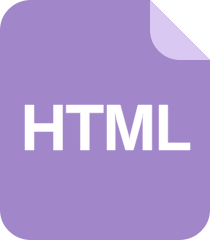
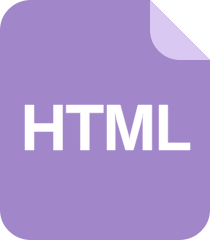
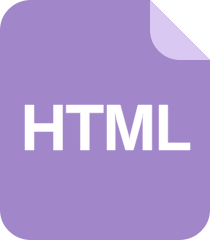
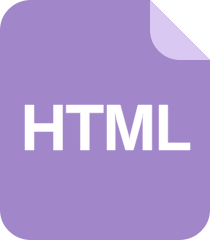
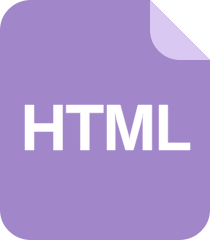
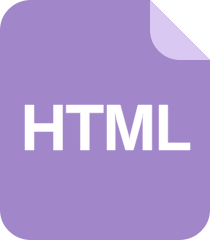
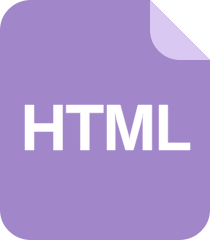
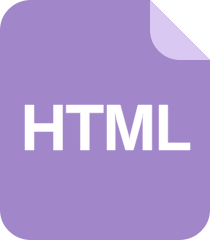
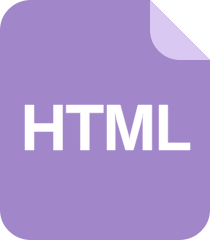
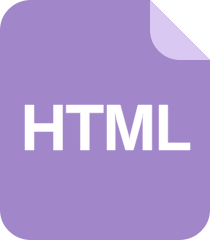
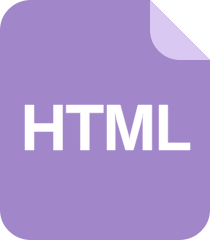
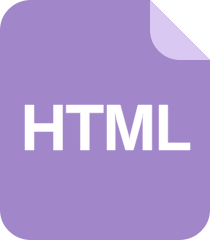
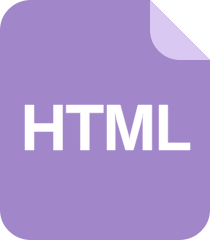
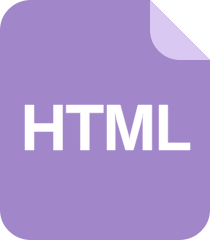
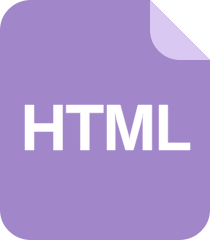
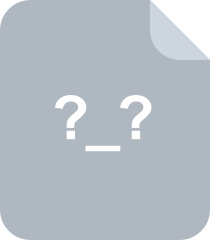
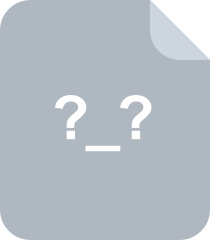
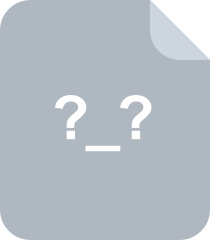
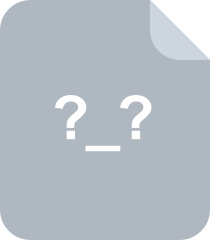
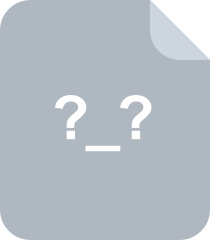
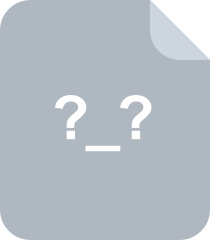
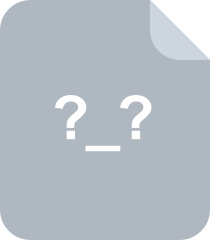
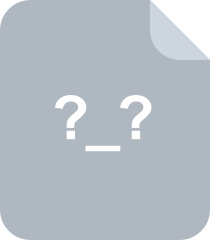
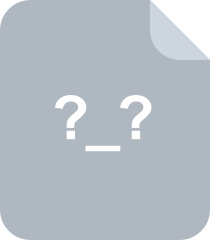
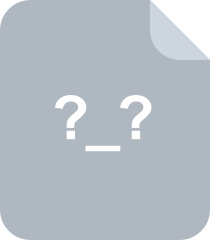
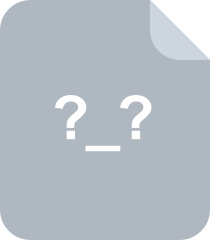
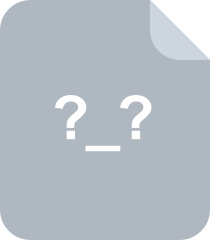
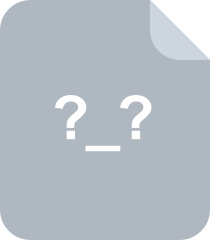
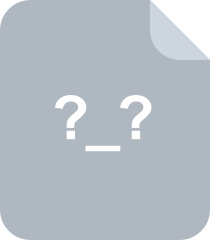
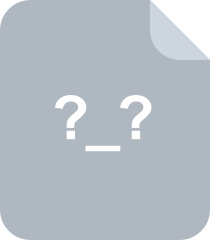
共 618 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
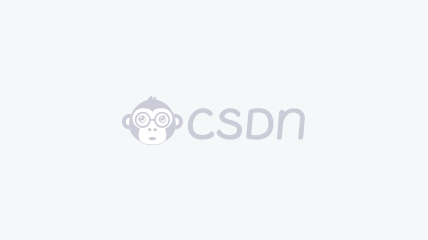

一只会写程序的猫
- 粉丝: 1w+
- 资源: 866
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

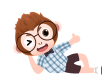
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


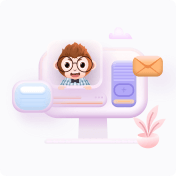
安全验证
文档复制为VIP权益,开通VIP直接复制
