#include <iostream>
#include <opencv2/opencv.hpp>
#include <unistd.h>
#include <error.h>
#include <errno.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <sys/types.h>
#include <pthread.h>
#include <linux/videodev2.h>
#include <sys/mman.h>
#include <opencv2/core/core.hpp>
#include <opencv2/highgui/highgui.hpp>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <iostream>
#include <iomanip>
#include <string>
using namespace std;
using namespace cv;
int main(int argc, char ** argv)
{
std::string videoFile = "top_view.mp4";//视频的路径
std::string videoFile1 = "fusion_view.mp4";//视频的路径
/** 打开第一个视频文件 */
VideoCapture cap; //视频句柄变量
cap.open(videoFile);//打开视频
if(!cap.isOpened()) //判断是否打开了
{
printf("cap.isOpened is error\n");
return -1;
}
/** 打开第二个视频文件 */
VideoCapture cap1; //视频句柄变量
cap1.open(videoFile1);//打开视频
if(!cap1.isOpened()) //判断是否打开了
{
printf("cap.isOpened is error\n");
return -1;
}
/** 打开第一个视频文件的帧数 */
int frame_num = cap.get(cv::CAP_PROP_FRAME_COUNT);
std::cout << "videoFile total frame number is: " << frame_num << std::endl;
/** 打开第二个视频文件的帧数 */
int frame_num1 = cap1.get(cv::CAP_PROP_FRAME_COUNT);
std::cout << "videoFile1 total frame number is: " << frame_num1 << std::endl;
/** 打开第一个视频文件的帧率 */
int fps = cap.get(cv::CAP_PROP_FPS);
std::cout << "videoFile fps: " << fps << std::endl;
/** 打开第二个视频文件的帧率 */
int fps1 = cap1.get(cv::CAP_PROP_FPS);
std::cout << "videoFile1 fps1: " << fps1 << std::endl;
/** 打开第一个视频文件的宽度 */
int image_width = cap.get(cv::CAP_PROP_FRAME_WIDTH);
std::cout << "videoFile image width is: " << image_width << std::endl;
/** 打开第二个视频文件的宽度 */
int image_width1 = cap1.get(cv::CAP_PROP_FRAME_WIDTH);
std::cout << "videoFile1 image width is: " << image_width1 << std::endl;
/** 打开第一个视频文件的高度 */
int image_height = cap.get(cv::CAP_PROP_FRAME_HEIGHT);
std::cout << "videoFile image height: " << image_height << std::endl;
/** 打开第二个视频文件的高度 */
int image_height1 = cap1.get(cv::CAP_PROP_FRAME_HEIGHT);
std::cout << "videoFile1 image height: " << image_height1 << std::endl;
/** 打开第一个视频文件的矩阵对象的格式*/
int frame_format = cap.get(cv::CAP_PROP_FORMAT);
std::cout << "videoFile frame format: " << frame_format << std::endl;
/** 打开第二个视频文件的矩阵对象的格式 */
int frame_format1 = cap1.get(cv::CAP_PROP_FORMAT);
std::cout << "videoFile1 image height: " << frame_format1 << std::endl;
/** 合并视频初始化 */
std::string mergeVideooFile = "mergeVideo.avi";
int mergeVideooHeight = 600;
int mergeVideooWidth = 1250;
float mergeVideooFps = 10.0;
int mergeVideooFpsFrameFormat = CV_8UC3;
/*
mergeVideooHeight = image_height + image_height1 + 10;
mergeVideooWidth = image_width + image_width1 + 10;
mergeVideooFps = fps;
if(fps1>fps)
mergeVideooFps = fps1;
mergeVideooFps = 10;
mergeVideooFpsFrameFormat = frame_format;
*/
cv::VideoWriter track_writer;
Mat img = cv::Mat::zeros(mergeVideooWidth, mergeVideooHeight, mergeVideooFpsFrameFormat);
track_writer.open (mergeVideooFile, cv::VideoWriter::fourcc('M', 'P', '4', '2'), mergeVideooFps, cv::Size(mergeVideooWidth, mergeVideooHeight));
if(!track_writer.isOpened())
{
assert("track writer open failed!\n");
}
Mat frame;
Mat frame1;
while(1)
{
cap.read(frame);//从第一个视频获取一帧图片
cap1.read(frame1);//从第二个视频获取一帧图片
if(frame.empty()) break; //是否加载成功
//std::cout << "Width : " << frame1.cols << std::endl;
//std::cout << "Height: " << frame1.rows << std::endl;
// std::cout << "Width : " << frame.cols << std::endl;
//std::cout << "Height: " << frame.rows << std::endl;
//cout << "======================" << endl;
// 设定ROI区域:截取一部分进行合并
Mat imageROI= frame(Rect(400,0,400,600));
Mat imageROI1= frame1(Rect(200,0,850,450));
// 大小转换
Mat imageROI1dst = Mat::zeros(600,850 , CV_8UC3); //我要转化为850*600大小的
resize(imageROI1, imageROI1dst, imageROI1dst.size());
// 视频写字
putText(imageROI1dst, videoFile, Point(5, 55),FONT_HERSHEY_PLAIN,2.0,(0, 255, 255),2);
putText(imageROI, videoFile1, Point(5, 55),FONT_HERSHEY_PLAIN,2.0,(0, 255, 255),2);
//创建目标Mat
Mat des;
des.create(mergeVideooHeight,mergeVideooWidth, imageROI1dst.type());
// 视频帧合并
Mat r1 = des(Rect(0, 0, 850, 600));
imageROI1dst.copyTo(r1);
Mat r2 = des(Rect(850, 0, 400, 600));
imageROI.copyTo(r2);
// 格式化要保存的视频帧
cv::resize(des, img , cv::Size(mergeVideooWidth, mergeVideooHeight));
// 保存视频
track_writer.write(img);
// 可视化
imshow("mergeVideoo",des);
if (cv::waitKey(30) == 'q')//可以用开控制播放或者获取图片的速度,毫秒级别
{
break;
}
}
destroyWindow("mergeVideoo");//关闭窗口bb;
destroyAllWindows();//关闭所有的窗口
cap.release();//释放视频句柄
track_writer.release();
return 0;
}
视频合并;将两个视频合并为一个文件,并在视频中打标签
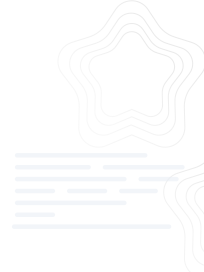

在IT领域,视频编辑是一项常见的任务,特别是在多媒体创作、教育、娱乐和社交媒体分享等方面。本文将详细介绍如何将两个视频合并为一个文件,并在视频中添加标签,这是一个实用且有趣的功能,能够帮助用户创造更具表现力的视听内容。 我们要理解“视频合并”这一过程。视频合并通常涉及到将两个或多个独立的视频片段拼接在一起,形成一个新的连续视频文件。这个过程可以通过各种视频编辑软件来完成,包括专业级的如Adobe Premiere Pro、Final Cut Pro,以及更适合普通用户的免费工具如iMovie、Shotcut等。这些工具提供了直观的界面和丰富的功能,使得视频合并变得简单易行。 合并视频的基本步骤如下: 1. **导入视频**:打开你选择的视频编辑软件,点击“导入”或“添加媒体”按钮,将你需要合并的两个视频文件导入到软件的工作区。 2. **排列顺序**:将视频按照你希望播放的顺序拖放到时间线。通常,时间线上的顶部视频会先播放,底部的视频随后播放。 3. **添加过渡效果**(可选):如果你希望视频之间有平滑的切换,可以在两段视频的交界处添加过渡效果。这可以是简单的淡入淡出,也可以是更复杂的特效。 4. **调整长度**:如果需要,你可以裁剪视频的部分片段,以适应你的需求。 5. **保存与导出**:完成编辑后,选择合适的视频格式和质量进行导出。确保导出设置能保留原始视频的质量,除非你有特定的文件大小或格式要求。 接下来,我们讨论如何在视频中“打标签”。这通常指的是在视频画面上添加文字、图像或图形,以便提供说明、标注或者装饰。这可以通过视频编辑软件的“叠加”或“标题”功能实现。 1. **创建标签**:在软件的“标题”或“文本”工具中,输入你要显示的标签内容。你可以自定义字体、颜色、大小和动画效果。 2. **定位标签**:在时间线上选择你希望标签出现的时间点,然后将其拖放到相应位置。 3. **调整标签持续时间**:通过拉伸或缩短标题轨道,可以控制标签在屏幕上的显示时长。 4. **预览与修改**:在预览窗口检查标签的效果,如果需要,可以随时调整其样式和位置。 提到的“mergeVideo”可能是一个具体的工具或脚本,用于自动化视频合并的过程。在一些编程环境中,例如Python,可以使用库如OpenCV或moviepy来合并视频,并添加文字标签。这需要一定的编程知识,但能够实现更高级的定制化操作。 视频合并和标签添加是丰富视频内容、提升观看体验的有效手段。无论你是专业视频制作者还是业余爱好者,掌握这些技能都能帮助你更好地表达创意,创造出更具吸引力的视频作品。


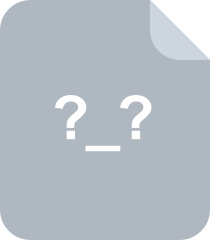
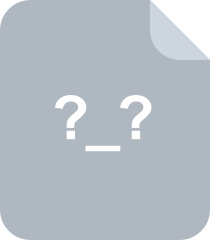
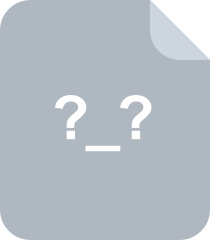
- 1




















- 粉丝: 686
- 资源: 24
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

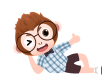
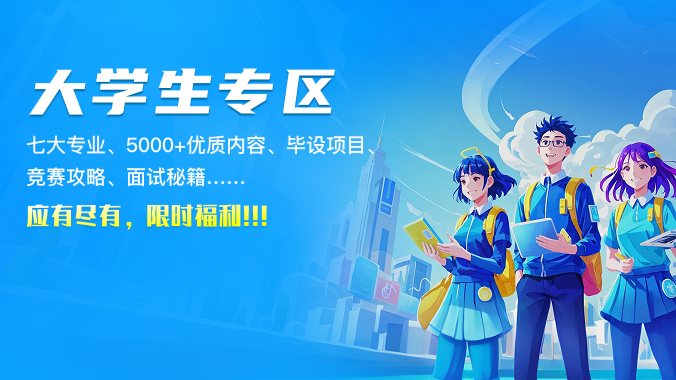
最新资源
- 淬火槽输送线sw20可编辑_三维3D设计图纸_包括零件图_机械3D图可修改打包下载_三维3D设计图纸_包括零件图_机械3D图可修改打包下载.zip
- 单信道超外差AM发射机设计与Multisim仿真验证:调制器与A类高频谐振功放
- 简明TypeScript书:TypeScript有效开发的简明指南
- (源码)基于低代码引擎的低代码开发演示项目.zip
- 卧式抛光机sw20可编辑_三维3D设计图纸_包括零件图_机械3D图可修改打包下载_三维3D设计图纸_包括零件图_机械3D图可修改打包下载.zip
- 车身翻转车sw20_三维3D设计图纸_包括零件图_机械3D图可修改打包下载_三维3D设计图纸_包括零件图_机械3D图可修改打包下载.zip
- 大理石幕墙板加工线sw20_三维3D设计图纸_包括零件图_机械3D图可修改打包下载_三维3D设计图纸_包括零件图_机械3D图可修改打包下载.zip
- MATLAB中切比雪夫I型IIR高通滤波器设计与低频噪声滤除验证
- STM32单片机开发-单片机开发资源
- (源码)基于PHPLaravel框架的在线教育平台.zip
- 永磁同步电机MATLAB Simulink仿真:PI控制、Clark-Park变换及SVPWM模块的实现与优化
- 工控机工业sw20可编辑_三维3D设计图纸_包括零件图_机械3D图可修改打包下载_三维3D设计图纸_包括零件图_机械3D图可修改打包下载.zip
- 汽车稳定杆喷涂设备sw20可编辑_三维3D设计图纸_包括零件图_机械3D图可修改打包下载_三维3D设计图纸_包括零件图_机械3D图可修改打包下载.zip
- 三坐标调整座sw16_三维3D设计图纸_包括零件图_机械3D图可修改打包下载_三维3D设计图纸_包括零件图_机械3D图可修改打包下载.zip
- (源码)基于Robotics与Arduino编程的Sumobot团队入门引导.zip
- 解决问题应用题(比多少)

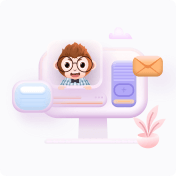

评论0