' **********************************************************************************
' * WinLicence.vb
' * Proxy Functions for WinLicence protected assemblies. Written in .NET
' *
' * Author: Mike Wilson (http://blog.evolvedsoftwarestudios.com)
' * Evolved Software Studios Ltd (http://www.evolvedsoftwarestudios.com)
' *
' * Version: 0.1
' * License: Freely distribute, but keep this comment header.
' *
' * Comments: You will need to modify the bespoke code below
' * in the region "Evolved Software Studios Licensing Code"
' * to your own needs. I have provided this as a demonstration only.
' *
' * Disclaimer: Use at your own risk. Think of this as a quick-start to using
' * WinLicence with VB.NET. Then improve the code and let me have the latest
' * version please :@)
' **********************************************************************************
Module WinLicence
Private _bufferVarValue As New System.Text.StringBuilder(100)
Public Declare Function GetEnvironmentVariable Lib "kernel32.dll" Alias "GetEnvironmentVariableA" (ByVal lpName As String, ByVal lpBuffer As System.Text.StringBuilder, ByVal nSize As Integer) As Integer
Public Declare Function WLLoadWinlicenseDll Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLGenTrialExtensionFileKey Lib "WinlicenseSDK.dll" (ByVal TrialHash As String, ByVal Level As Short, ByVal NumDays As Short, ByVal NumExec As Short, ByRef NewDate As Date, ByVal NumMinutes As Short, ByVal TimeRuntime As Short, ByVal BufferOut As String) As Short
'Public Declare Function WLGenTrialExtensionRegistryKey Lib "WinlicenseSDK.dll" (ByVal TrialHash As String, ByVal Level As Short, ByVal NumDays As Short, ByVal NumExec As Short, ByRef NewDate As Date, ByVal NumMinutes As Short, ByVal TimeRuntime As Short, ByVal pKeyName As String, ByVal pKeyValueName As String, ByVal BufferOut As String) As Short
'Public Declare Function WLGenPassword Lib "WinlicenseSDK.dll" (ByVal PasswordHash As String, ByVal UserName As String, ByVal BufferOut As String) As Short
'Public Declare Function WLGenLicenseTextKey Lib "WinlicenseSDK.dll" (ByVal LicenseHash As String, ByVal UserName As String, ByVal Organization As String, ByVal CustomData As String, ByVal MachineID As String, ByVal NumDays As Short, ByVal NumExec As Short, ByRef NewDate As Date, ByVal CountryId As Short, ByVal Runtime As Short, ByVal GlobalMinutes As Short, ByVal BufferOut As String) As Short
'Public Declare Function WLGenLicenseSmartKey Lib "WinlicenseSDK.dll" (ByVal LicenseHash As String, ByVal UserName As String, ByVal Organization As String, ByVal CustomData As String, ByVal MachineID As String, ByVal NumDays As Short, ByVal NumExec As Short, ByRef NewDate As Date, ByVal BufferOut As String) As Short
'Public Declare Function WLGenLicenseFileKey Lib "WinlicenseSDK.dll" (ByVal LicenseHash As String, ByVal UserName As String, ByVal Organization As String, ByVal CustomData As String, ByVal MachineID As String, ByVal NumDays As Short, ByVal NumExec As Short, ByRef NewDate As Date, ByVal CountryId As Short, ByVal Runtime As Short, ByVal GlobalMinutes As Short, ByVal BufferOut As String) As Short
'Public Declare Function WLGenLicenseRegistryKey Lib "WinlicenseSDK.dll" (ByVal LicenseHash As String, ByVal UserName As String, ByVal Organization As String, ByVal CustomData As String, ByVal MachineID As String, ByVal NumDays As Short, ByVal NumExec As Short, ByRef NewDate As Date, ByVal CountryId As Short, ByVal Runtime As Short, ByVal GlobalMinutes As Short, ByVal RegName As String, ByVal RegValueName As String, ByVal BufferOut As String) As Short
'Public Declare Function WLRegGetStatus Lib "WinlicenseSDK.dll" (ByVal ExtendedInfo As Short) As Short
'Public Declare Function WLTrialGetStatus Lib "WinlicenseSDK.dll" (ByVal ExtendedInfo As Short) As Short
'Public Declare Function WLTrialExtGetStatus Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLRegGetLicenseInfo Lib "WinlicenseSDK.dll" (ByVal UserName As String, ByVal Organization As String, ByVal CustomData As String) As Boolean
'Public Declare Function WLTrialTotalDays Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLTrialTotalExecutions Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLTrialDaysLeft Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLTrialExecutionsLeft Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLTrialExpirationDate Lib "WinlicenseSDK.dll" (ByRef ExpDate As Date) As Short
'Public Declare Function WLTrialGlobalTimeLeft Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLTrialRuntimeLeft Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLTrialLockedCountry Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLRegDaysLeft Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLRegExecutionsLeft Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLRegExpirationDate Lib "WinlicenseSDK.dll" (ByRef ExpDate As Date) As Short
'Public Declare Function WLRegTotalExecutions Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLRegTotalDays Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLHardwareGetID Lib "WinlicenseSDK.dll" (ByVal HardId As String) As Boolean
'Public Declare Function WLHardwareCheckID Lib "WinlicenseSDK.dll" (ByVal HardId As String) As Boolean
'Public Declare Function WLRegSmartKeyCheck Lib "WinlicenseSDK.dll" (ByVal UserName As String, ByVal Organization As String, ByVal CustomData As String, ByVal AsciiKey As String) As Boolean
'Public Declare Function WLRegNormalKeyCheck Lib "WinlicenseSDK.dll" (ByVal AsciiKey As String) As Boolean
'Public Declare Function WLRegNormalKeyInstallToFile Lib "WinlicenseSDK.dll" (ByVal AsciiKey As String) As Boolean
'Public Declare Function WLRegNormalKeyInstallToRegistry Lib "WinlicenseSDK.dll" (ByVal AsciiKey As String) As Boolean
'Public Declare Function WLRegSmartKeyInstallToFile Lib "WinlicenseSDK.dll" (ByVal UserName As String, ByVal Organization As String, ByVal CustomData As String, ByVal AsciiKey As String) As Boolean
'Public Declare Function WLRegSmartKeyInstallToRegistry Lib "WinlicenseSDK.dll" (ByVal UserName As String, ByVal Organization As String, ByVal CustomData As String, ByVal AsciiKey As String) As Boolean
'Public Declare Function WLTrialCustomCounterInc Lib "WinlicenseSDK.dll" (ByVal Value As Short, ByVal CounterID As Short) As Short
'Public Declare Function WLTrialCustomCounterDec Lib "WinlicenseSDK.dll" (ByVal Value As Short, ByVal CounterID As Short) As Short
'Public Declare Function WLTrialCustomCounter Lib "WinlicenseSDK.dll" (ByVal CounterID As Short) As Short
'Public Declare Function WLTrialCustomCounterSet Lib "WinlicenseSDK.dll" (ByVal Value As Short, ByVal CounterID As Short) As Short
'Public Declare Function WLRestartApplication Lib "WinlicenseSDK.dll" () As Boolean
'Public Declare Function WLRegLockedCountry Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLRegRuntimeLeft Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLRegGlobalTimeLeft Lib "WinlicenseSDK.dll" () As Short
'Public Declare Function WLRegDisableCurrentKey Lib "WinlicenseSDK.dll" (ByVal DisableFlags As Short) As Boolean
'Public Declare Function WLRegRemoveCurrentKey Lib "WinlicenseSDK.dll" () As Boolean
'Public Declare Function WLHardwareGetFormattedID Lib "WinlicenseSDK.dll" (ByVal NumCharBlock As Short, ByVal Uppercase As Short, ByVal Buffer As String) As Boolean
'Public Declare Function WLPasswordCheck Lib "WinlicenseSDK.dll" (ByVal UserName As String, ByVal Password As String) As Boolean
'Public Declare Function WLTrialExpireTrial Lib "WinlicenseSDK.dll" () As Boolean
没有合适的资源?快使用搜索试试~ 我知道了~
WinLicense V 2.1.0
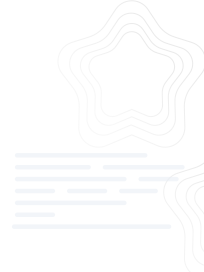
共923个文件
h:63个
old:63个
cpp:52个


温馨提示
WinLicense是一个强劲的保护系统, 专为了那些想保护自己的程式不被先进的反向工程和黑客软件破解的软件开发者而开发的。开发者不需要更改任何的原代码,和不需要程式编制的经验使用WinLicense。 WinLicense使用SecureEngine®的保护技术。它能够以最高的优先等级运行,这些保护技巧是从来都没在电脑防御技术领域出现过,使它最大程度地保护 任何程式 。 以下是WinLicense保护功能特点 多层的加密措施来保护程式的代码和资料。 黑客工具的监测。 以最高优先等级来启动代码,从来都没在电脑防御技术领域出现过。 扰乱 程式 的运行代码,资料和 APIs ,使软件破解者无法对 程式 还原成原代码 对于反汇编器和反编译器的保护 SDK为SecureEngine®和受保护的程式提供一个双向的沟通。 阻止从内存转送到磁盘上的高级技术。 完全自定义的保护选项和讯息。
资源推荐
资源详情
资源评论
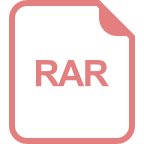
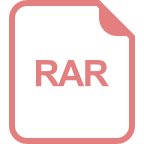
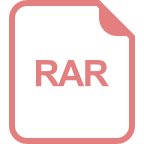
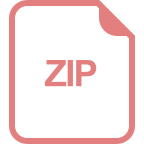
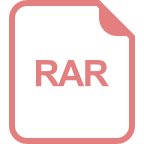
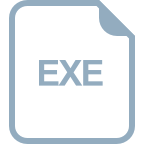
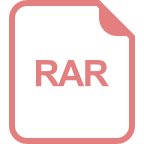
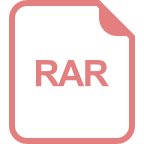
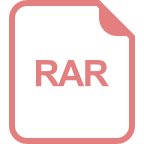
收起资源包目录

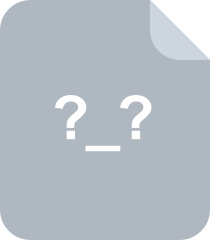
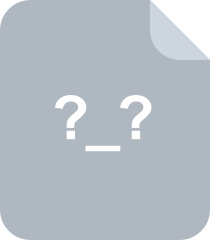
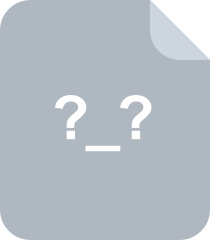
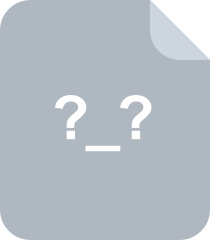
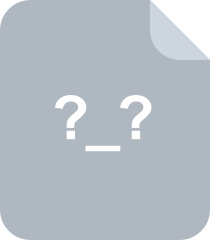
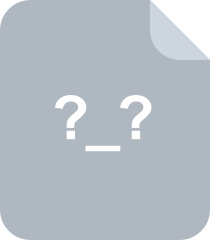
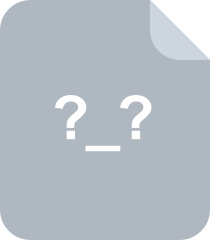
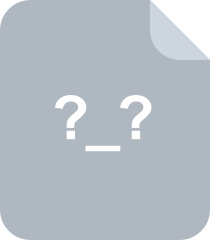
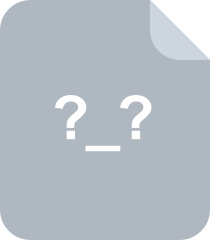
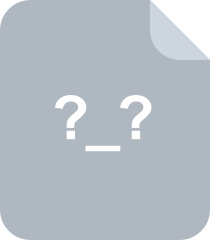
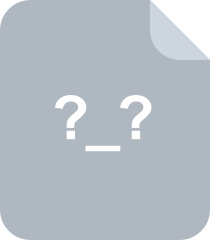
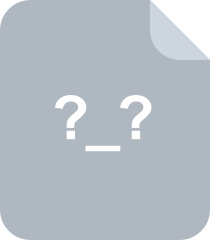
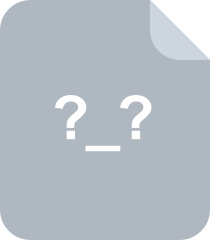
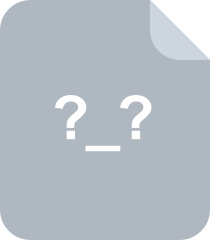
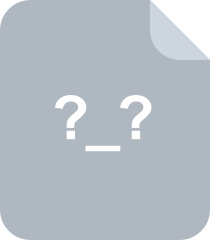
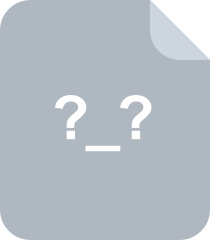
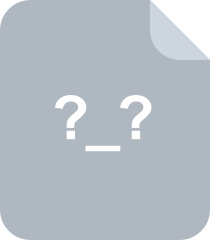
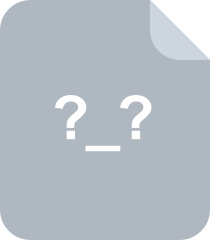
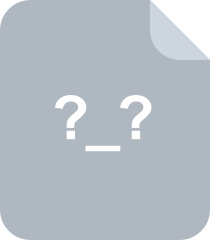
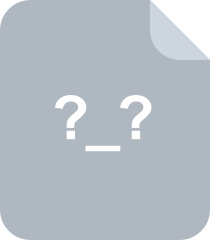
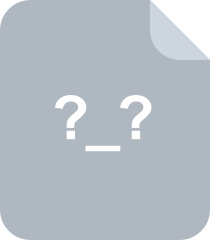
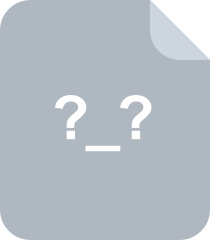
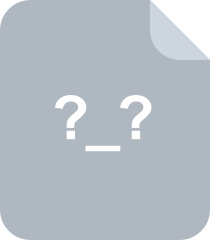
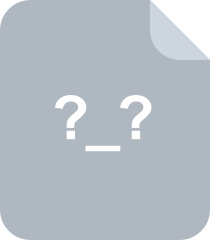
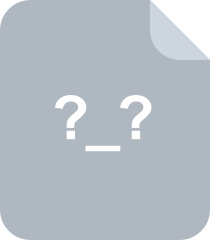
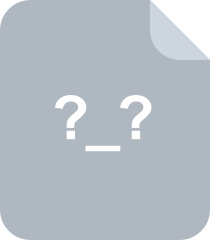
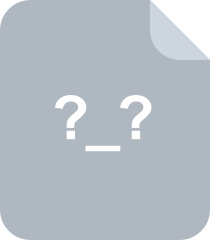
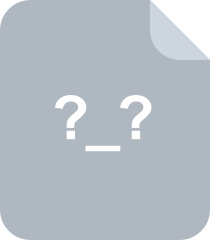
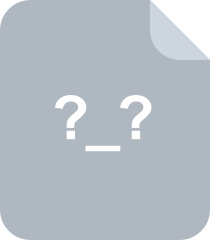
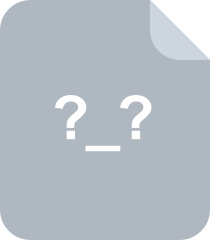
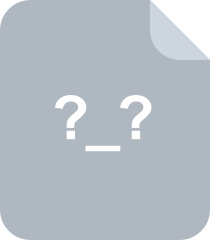
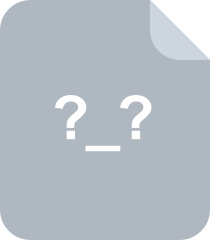
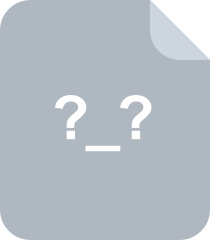
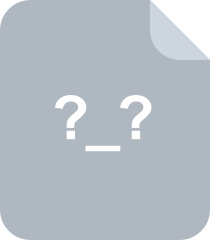
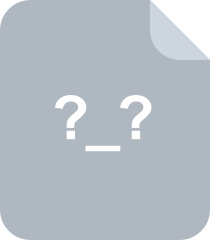
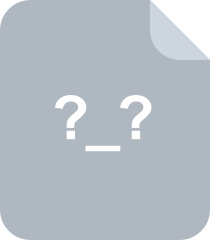
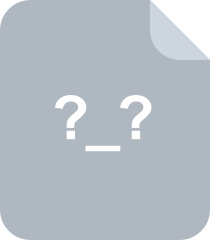
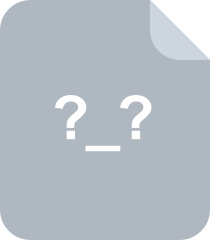
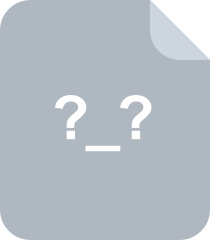
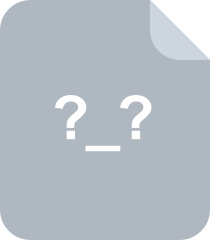
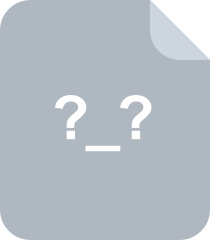
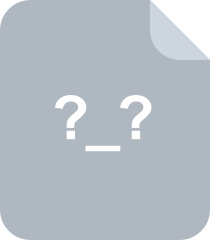
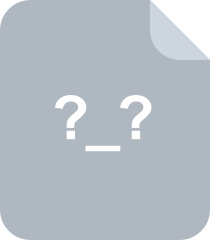
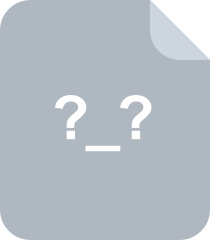
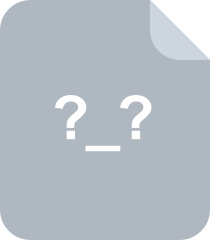
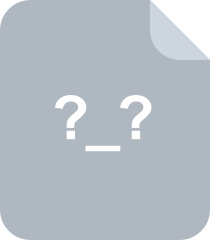
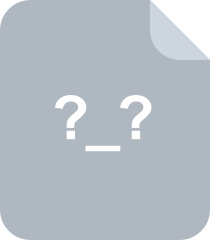
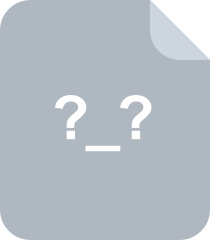
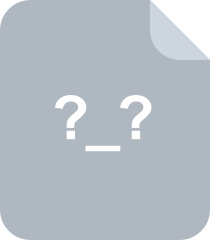
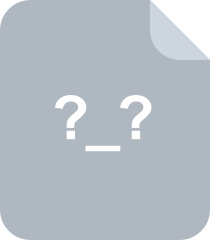
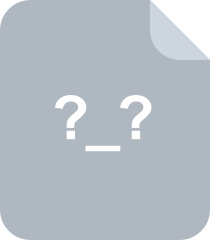
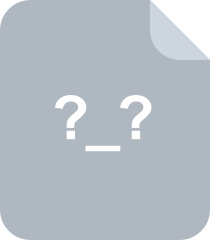
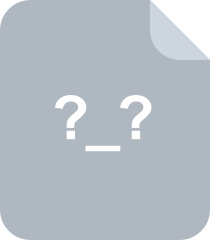
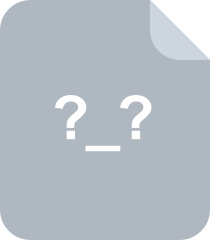
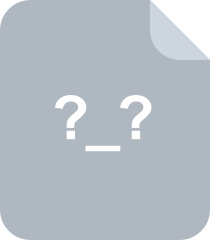
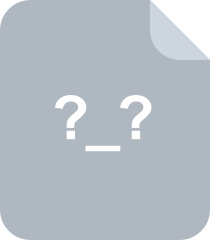
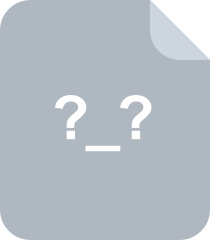
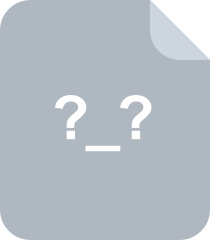
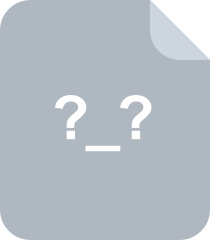
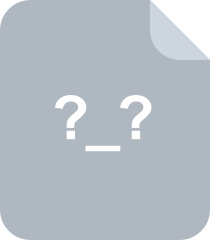
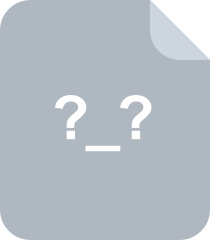
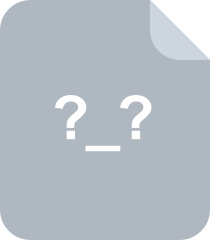
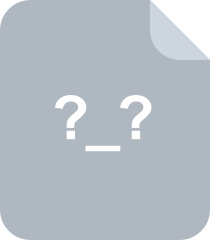
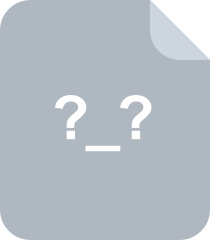
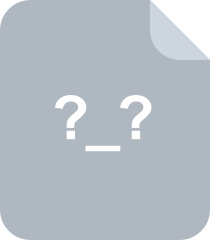
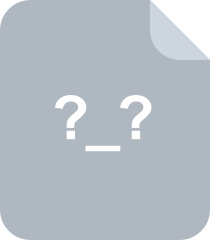
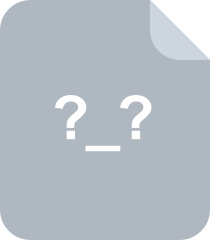
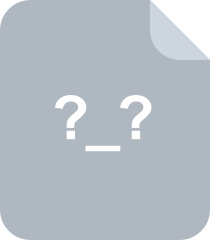
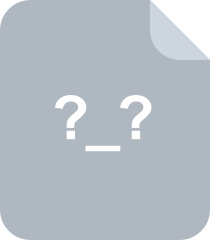
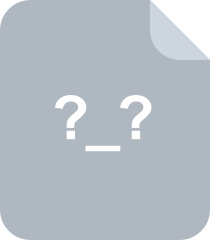
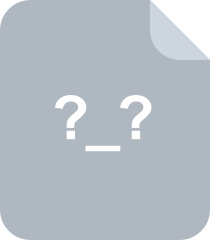
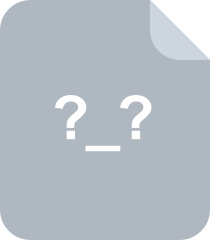
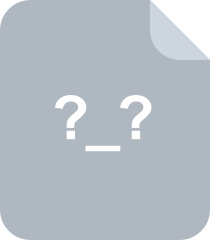
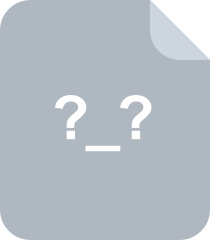
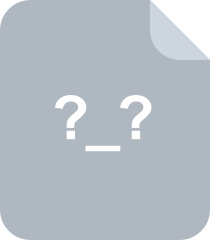
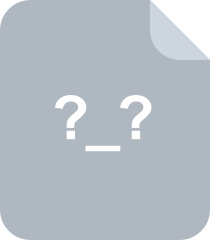
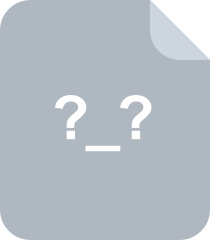
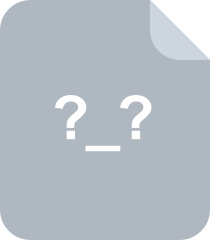
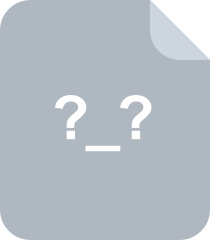
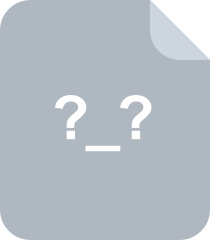
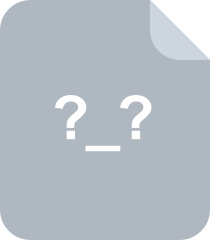
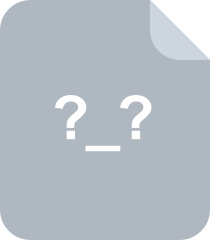
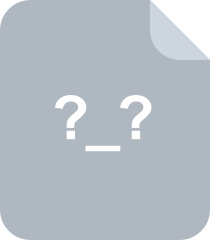
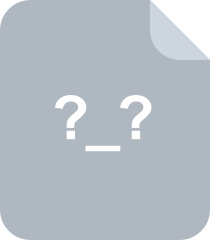
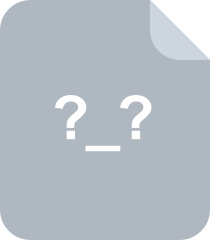
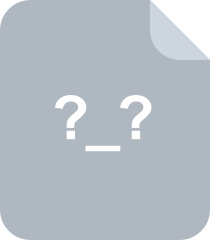
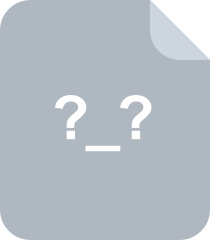
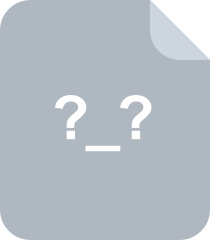
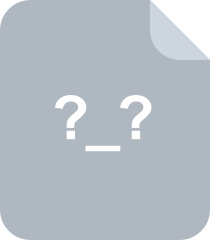
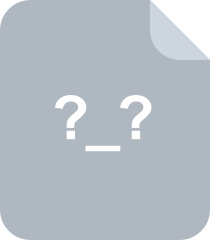
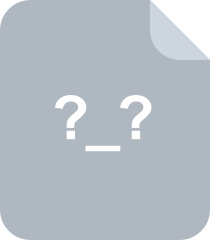
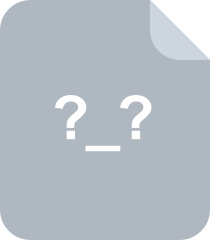
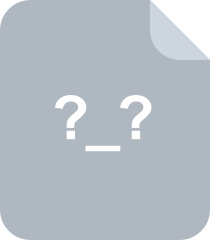
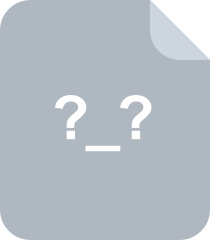
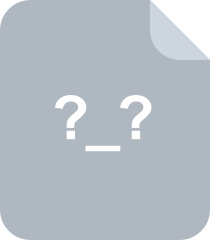
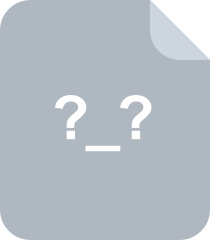
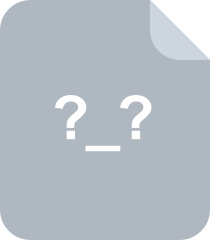
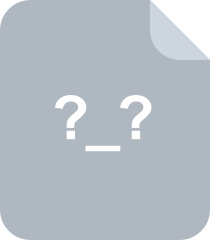
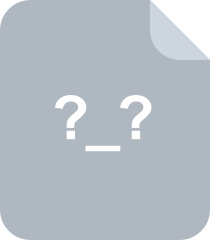
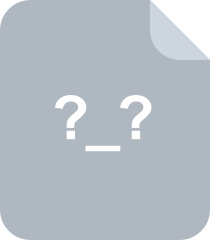
共 923 条
- 1
- 2
- 3
- 4
- 5
- 6
- 10
资源评论
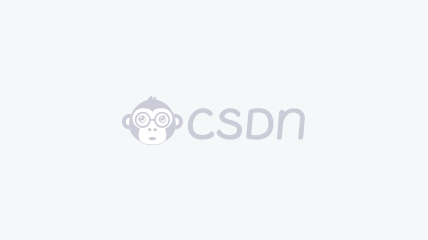
- wunaibinmate2012-12-20不错,本来是想加密按键精灵生成的小精灵的,结果用这个相当于是二次加密,可惜不能用啊。。
- 纳兰龙珠2011-09-11还可以版本比较高就是报马啊

hezhaoyang1972
- 粉丝: 0
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

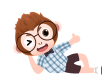
最新资源
- Digital-image-processing-system-main.zip
- 自动驾驶规划控制常用算法c++代码实现
- 旅行商问题旅行商问题旅行商问题旅行商问题旅行商问题.txt
- vuevuevuevuevuevuevuevuevuevuevuevuevuevuevuevue.txt
- c语言文件读写操作代码c语言文件读写操作代码c语言文件读写操作代码c语言文件读写操作代码.txt
- 基于新唐N76E003单片机SPI接口配置为SPI-Master+Slave 模式软件例程源码.zip
- android开发期末大作业基于Androidstudio的医疗系统app源码(高分项目).zip
- assets_e4b6f25e1c38b56b464c56c31c1a361d.mp4
- 基于Android studio设计的图书借阅管理系统APP期末大作业(高分项目).zip
- 安卓期末大作业-音乐播放器App-AndroidStudio开发(高分项目)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


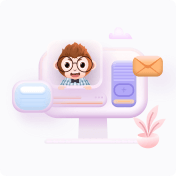
安全验证
文档复制为VIP权益,开通VIP直接复制
